Most-Asked Microsoft Interview Questions And Answers (2024)
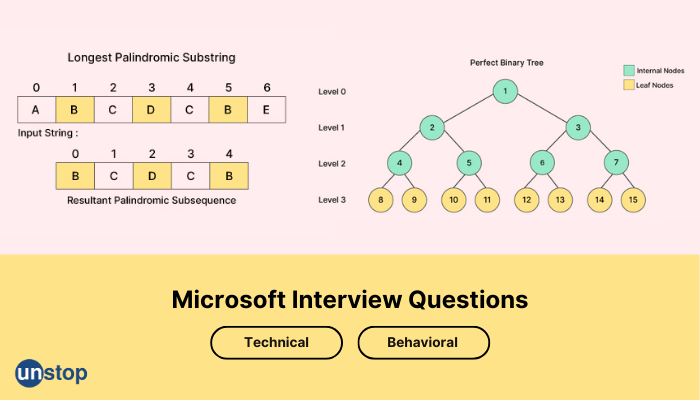
Table of content:
- Preparing for Microsoft interview questions
- Microsoft technical interview questions
- Microsoft behavioural interview questions
In 1975, when most Americans were relying on typewriters, long-time companions Bill Gates and Paul Allen founded Microsoft, an innovation-oriented tech company that made computer programs. The company gradually went on to dominate the personal computer operating system market with MS-DOS and Windows. Today, Microsoft is one of the largest software makers in the world.
Microsoft Corporation is based in Redmond, Washington, and is engaged in manufacturing computer software, personal computers, consumer electronics, and related services. Being a marquee brand, Microsoft attracts around 2 million applications per year from job seekers around the world. If you too want to be a part of the IT-giant as an engineer, we will guide you through. In this article, you will find the most important Microsoft interview questions that will bring you closer to your dream job.
Preparing yourself for Microsoft interview questions
For engineering roles, Microsoft seeks curious and innovative minds with a solid knowledge base. Hence, if you have made it to the interview round of Microsoft, handling the Microsoft interview questions like a pro needs good amount of practice. While brushing up on your basic concepts and practicing coding is absolutely important, good interpersonal skills are a must-have too.
The following sections cover Microsoft interview questions related to all the important topics that you must know. Since the interview process at Microsoft may be divided into technical and HR rounds, we have divided the questions into:
- Technical Microsoft interview questions
- Behavioral Microsoft interview questions
Microsoft Interview Questions: Technical
Like other tech companies, Microsoft assesses your technical skills through a range of questions from technical concepts like data structures, algorithm questions, dynamic programming, and online coding assessment. The technical interview may start with common coding questions, followed by topics like operating systems, time complexity, DSA, OOPS, concurrency basics and more.
Let's take you through the frequently asked Microsoft interview questions based on their difficulty level.
Microsoft Interview Questions - Level Easy
1. What is consumer electronics?
Consumer electronics refers to electronic devices that are designed and intended for everyday use by consumers. These are typically personal devices that people use in their homes, offices, or on-the-go, and are meant for entertainment, communication, or productivity purposes.
Examples of consumer electronics include smartphones, laptops, tablets, televisions, digital cameras, video game consoles, home theater systems, headphones, and portable music players. These devices often incorporate advanced technologies such as microprocessors, memory chips, sensors, and displays, and may be connected to the internet or other networks.
Consumer electronics are often marketed and sold through retail stores or online marketplaces and are subject to frequent updates and improvements in technology.
2. Give examples of PC or personal computers.
There are different types of personal computers (PCs), including desktops, laptops, 2-in-1s, and all-in-ones. Here are some examples of each type:
-
Desktop PC: A traditional desktop computer typically consists of a tower or box that contains the CPU, motherboard, memory, and storage components. Examples include the Dell OptiPlex, HP Pavilion, and Lenovo ThinkCentre.
-
Laptop PC: A laptop is a portable computer that integrates the CPU, motherboard, memory, storage, display, and keyboard into a single compact device. Examples include the Apple MacBook, Dell XPS, and Lenovo ThinkPad.
-
2-in-1 PC: A 2-in-1 PC is a hybrid device that combines the functionality of a laptop and a tablet. Examples include the Microsoft Surface Pro, Lenovo Yoga, and HP Spectre x360.
-
All-in-One PC: An all-in-one PC is a desktop computer that integrates the CPU, motherboard, memory, storage, and display into a single device. Examples include the Apple iMac, Dell Inspiron, and HP Envy.
3. Explain UX or User Experience.
User Experience (UX) refers to the overall experience that a user has when interacting with a product or service, such as a website, app, or physical device. It encompasses all aspects of a user's interaction, including how easy the product is to use, how enjoyable the experience is, and how well it meets the user's needs and expectations.
Good UX design involves a combination of factors, including usability, accessibility, design aesthetics, and user research. UX designers aim to create interfaces and experiences that are intuitive, efficient, and enjoyable, with a focus on delivering value to the user.
UX design involves a range of activities, including user research, prototyping, testing, and iterative design. It requires a deep understanding of user needs, behaviors, and preferences, as well as an understanding of the technical constraints and capabilities of the product or service being designed.
Ultimately, the goal of UX design is to create products and services that provide a positive experience for users, resulting in increased engagement, satisfaction, and loyalty.
4. Give an example of an efficient algorithm for solving problems.
One example of an efficient algorithm for solving problems is the "binary search" algorithm, which is used to search for a specific item in a sorted list of elements. Here's how it works:
- Start by selecting the middle element in the list.
- If the middle element matches the item you're searching for, then the search is complete.
- If the middle element is greater than the item you're searching for, then repeat the search on the left half of the list.
- If the middle element is less than the item you're searching for, then repeat the search on the right half of the list.
- Repeat steps 1-4 until either the item is found, or it's determined that the item is not on the list.
Binary search is an efficient algorithm because it reduces the search space in half with each iteration, resulting in a logarithmic time complexity of O(log n), where n is the number of elements in the list. This means that even for very large lists, the search can be completed in a relatively small number of steps, making it an ideal algorithm for searching sorted data.
5. What is the difference between array/string in Java?
In Java, an array is a collection of elements of the same data type, which are stored in contiguous memory locations. The elements of an array can be accessed using an index, which represents the position of the element in the array. Arrays in Java are mutable, which means that their values can be changed.
On the other hand, a string is a sequence of characters. In Java, strings are immutable, which means that once a string is created, its value cannot be changed. Strings are represented by the String class in Java, and they can be created using string literals (enclosed in double quotes) or using the new operator.
The main difference between arrays and strings in Java is that arrays are used to store a collection of values of the same data type, while strings are used to represent text. Arrays are mutable, while strings are immutable. Another important difference is that arrays can store any primitive data type or object reference, while strings can only store characters.
However, it's worth noting that strings can be converted to arrays of characters using the toCharArray() method, and arrays of characters can be converted to strings using the String constructor that takes an array of characters as an argument.
6. What is the frequency of a majority element in an array?
The frequency of a majority element in an array is the number of times that element appears in the array such that it occurs in more than half of the total number of elements. In other words, a majority element in an array is an element that appears more than n/2 times, where n is the total number of elements in the array.
For example, consider the following array:
[2, 3, 2, 2, 4, 2, 5, 2, 6]
In this array, the number 2 appears 5 times, which is more than half of the total number of elements (9). Therefore, 2 is the majority element in the array, and its frequency is 5.
Finding the frequency of a majority element in an array is an important problem in computer science and is used in various algorithms and applications, such as voting systems and data analysis. One common approach to solving this problem is to use the Boyer-Moore majority vote algorithm, which is an efficient algorithm that can find the majority element in linear time complexity (O(n)) and constant space complexity (O(1)).
7. How to find the largest element of an array in C?
To find the largest element of an array in C, you can use a simple loop that iterates through the array and compares each element to a variable that stores the current maximum value. Here's an example:
#include
int main() {
int arr[] = {10, 20, 30, 40, 50};
int n = sizeof(arr) / sizeof(arr[0]);
int max = arr[0];
for (int i = 1; i < n; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
printf("The largest element of the array is %d", max);
return 0;
}
In this example, we first define an array arr and its size n. We initialize a variable max to the first element of the array. We then use a for loop to iterate over the remaining elements of the array, comparing each element to the current maximum value and updating max if a larger value is found.
8. What is a 2D array in JavaScript?
In JavaScript, a 2D array is an array of arrays. This means that each element of the main array is itself an array. A 2D array can be thought of as a table or grid, where each element in the table is identified by a row and column index.
Here's an example of how to create and access a 2D array in JavaScript:
// Create a 2D array with 3 rows and 4 columns
let matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12]
];// Access an element in the matrix by row and column index
console.log(matrix[1][2]);
9. Differentiate between Subarray and Subsequence.
A subarray is a portion of an array, which can be either contiguous or non-contiguous.
A contiguous subarray, also called a substring, is a subarray in which all the elements are adjacent to each other. In other words, the contiguous subarray is a continuous segment of the original array.
For example, consider the following array:
[1,2,3,4,5]
Some examples of contiguous subarrays of this array are:
- [1,2,3]
- [2,3]
- [4,5]
On the other hand, a non-contiguous subarray can have gaps between the selected elements. For example, the following are some non-contiguous subarrays of the same array:
- [1,3,5]
- [2,4]
- [1,2,4,5]
In computer science, contiguous subarrays are often used in algorithms and problems related to data structures and dynamic programming, such as finding the maximum sum of a contiguous subarray or the longest increasing subsequence. Non-contiguous subarrays can be used in other types of problems, such as finding the maximum sum of a non-contiguous subarray.
10. What is an integer array in Java?
In Java, an integer array is an array that stores a collection of integers, where each element of the array is an integer value. An integer array can be declared and initialized in Java as follows:
int[] arr = new int[5]; // declare and initialize an integer array of size 5
// initialize the array with integer values
arr[0] = 10;
arr[1] = 20;
arr[2] = 30;
arr[3] = 40;
arr[4] = 50;
In this example, we declare an integer array arr of size 5 using the syntax int[] arr = new int[5];. We then initialize the elements of the array with integer values using the index notation arr[index] = value;.
11. Using JavaScript, how can you obtain values from an HTML input array?
Use input tags that have the same value for the “name”. This can tag multiple values which are stored under a single name. These can be accessed by using the name later. Use the given method for accessing all the input values:
var input = document.getElementsByName('array[]');
To obtain values from an HTML input array in JavaScript, you can use the querySelectorAll() method to select all the input elements with a specific name or class attribute, and then loop through the selected elements to get their values. Here's an example of how to obtain values from an HTML input array with the name attribute "myArray":
HTML:
<!-- HTML input array with name attribute "myArray" -->
<input type="text" name="myArray[]" value="1">
<input type="text" name="myArray[]" value="2">
<input type="text" name="myArray[]" value="3">
<input type="text" name="myArray[]" value="4">
JavaScript:
// Get all the input elements with the name attribute "myArray[]"
let inputs = document.querySelectorAll('input[name="myArray[]"]');
// Loop through the selected elements and get their values
let values = [];
for (let i = 0; i < inputs.length; i++) {
values.push(inputs[i].value);
}
// Print the obtained values to the console
console.log(values); // Output: ["1", "2", "3", "4"]
var input = document.getElementsByName('array[]');
12. What is a binary tree in C?
In C, a binary tree is a data structure that consists of nodes, where each node has at most two child nodes, referred to as the left child and the right child. The binary tree is a recursive data structure, meaning that each child node can also be the root of its own binary tree.
Here's an example of a binary tree in C:
struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
};
struct TreeNode* createNode(int val) {
struct TreeNode* node = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
// Example usage
int main() {
// Create a binary tree with root node value 1
struct TreeNode* root = createNode(1);
// Add left and right child nodes to the root
root->left = createNode(2);
root->right = createNode(3);
// Add left and right child nodes to the left child of the root
root->left->left = createNode(4);
root->left->right = createNode(5);
return 0;
}
13. What is a perfect binary tree?
A perfect binary tree is a type of binary tree in which all the internal nodes have exactly two children, and all the leaf nodes are at the same level or depth. In other words, all the levels of a perfect binary tree are filled, except possibly for the last level, which is filled from left to right.
Here's an example of a perfect binary tree:
1
/ \
2 3
/ \ / \
4 5 6 7
14. What is a binary search tree?
A binary search tree (BST) is a type of binary tree data structure in which each node has at most two children, referred to as the left child and the right child.
The binary search tree is constructed in such a way that the left child of a node contains a value less than or equal to the node's value, and the right child contains a value greater than the node's value. This property allows for efficient searching, insertion, and deletion operations, as the tree can be traversed in sorted order.
In a binary search tree, the root node serves as the starting point for the search, and subsequent nodes are visited based on whether their values are greater than or less than the value being searched for. This allows for efficient searching with a time complexity of O(log n), where n is the number of nodes in the tree.
15. What is a programming language?
A programming language is a formal language used to communicate with a computer and instruct it to perform certain tasks. It is a set of rules, symbols, and instructions that allow programmers to write software and create applications that run on computers and other devices.
Programming languages are designed to be used by humans to communicate with computers. They allow programmers to write code in a way that is readable, understandable, and maintainable. Programming languages can be high-level or low-level, depending on the level of abstraction they provide from the underlying hardware and operating system.
There are many different programming languages, each with its syntax, rules, and features. Some of the most popular programming languages include Java, Python, C++, JavaScript, Ruby, and PHP. Each language has its strengths and weaknesses and is suited for different types of applications and projects.
Programmers use programming languages to write code that instructs a computer to perform specific tasks, such as calculating a sum, sorting data, or displaying information on a screen. The code is then compiled or interpreted into machine code that can be executed by the computer.
16. According to the step approach, what are the steps in solving problems in programming?
There are several problem-solving approaches in programming, but one commonly used approach is the following five-step process:
-
Understanding the problem: The first step is to fully understand the problem you are trying to solve. This involves analyzing the problem and breaking it down into smaller, more manageable parts. You should also identify any constraints or requirements that need to be considered.
-
Designing a solution: Once you have a clear understanding of the problem, the next step is to design a solution. This involves choosing an appropriate algorithm or data structure and planning out how your program will implement it.
-
Writing code: With a plan in place, you can begin writing the code for your program. This involves translating your solution into actual code that can be executed by a computer.
-
Testing: After writing the code, you need to test it to ensure that it works as expected. This involves running the program with different inputs and verifying that it produces the correct outputs.
-
Debugging and maintenance: Finally, if there are any errors or bugs in your program, you need to debug them and fix the issues. You should also consider any potential issues that may arise in the future and plan for ongoing maintenance and updates to your program.
By following this five-step process, you can approach programming problems in a systematic and structured way, which can help you to solve problems more effectively and efficiently.
17. What do you mean by adjacent nodes?
Adjacent nodes in a graph are nodes that are connected by an edge. In other words, two nodes are adjacent if there is a direct connection between them in a graph.
For example, in a simple undirected graph, if node A is connected to node B with an edge, then node A and node B are adjacent nodes. Similarly, if node B is connected to node C with an edge, then node B and node C are also adjacent nodes.
In a directed graph, an edge has a direction, so adjacency is directional. If node A is connected to node B with a directed edge, then node A is said to be the parent of node B, and node B is said to be the child of node A. In this case, node A and node B are adjacent nodes in the direction of the edge, but not in the opposite direction.
18. What is the use of nodeValue?
In computer science and programming, a node value is a piece of data associated with a node in a data structure, such as a tree, linked list, or graph. The node value can represent any type of data, such as a number, a string, an object, or a reference to another node.
The node value is used to store information that is relevant to the particular node in the data structure. For example, in a binary search tree, the node value is typically used to store a key that can be used for searching and sorting the tree. In a linked list, the node value may store a data element, while the next pointer points to the next node in the list.
The node value can also be used to store additional information, such as metadata or auxiliary data that may be needed for certain operations or algorithms. For example, in a graph, the node value may store a label or a weight that can be used for shortest path algorithms.
19. What is a root node?
In a tree data structure, a root node is the topmost node of the tree. It is the node that has no parent node and is the starting point for traversing or navigating the tree. Every tree has exactly one root node, and all other nodes in the tree are descendants of the root node.
The root node is often used as a reference point for accessing or manipulating the tree. For example, when inserting a new node into a tree, the root node may be the starting point for finding the correct location for the new node. Similarly, when traversing a tree, the root node is typically the first node visited.
The root node can have any number of child nodes, depending on the type of tree being used. In a binary tree, for example, the root node has at most two child nodes, while in a general tree, the root node can have any number of child nodes.
20. How do you calculate maximum profit in dynamic programming?
Calculating the maximum profit in dynamic programming involves breaking down a problem into smaller subproblems and storing the results of each subproblem to avoid redundant calculations. The general steps for calculating maximum profit in dynamic programming are as follows:
-
Define the problem: Clearly define the problem you are trying to solve and identify the parameters and constraints that apply.
-
Identify the subproblems: Break down the problem into smaller subproblems that can be solved independently. In the context of calculating maximum profit, this might involve breaking down a larger problem into smaller subproblems involving subsets of items, for example.
-
Define the recursive relationship: Determine the relationship between the solutions to the subproblems and how they can be combined to solve the larger problem. This relationship should be expressed recursively, meaning that the solution to a larger problem can be expressed in terms of the solutions to smaller subproblems.
-
Implement a memoization or tabulation algorithm: To avoid redundant calculations, use either a memoization or tabulation algorithm to store the results of each subproblem as it is solved. Memoization involves storing the results of solved subproblems in a cache, while tabulation involves creating a table to store the results.
-
Determine the optimal solution: Once all subproblems have been solved and their results stored, use the results to determine the optimal solution to the original problem.
21. What is the typical data structure of an array?
An array is a common and simple data structure in programming that consists of a collection of elements of the same data type, which are stored in contiguous memory locations. The elements in an array can be accessed using an index or a subscript, which indicates the position of the element within the array.
In terms of its implementation, an array is typically represented in memory as a contiguous block of memory locations, with each location holding one element of the array. The size of the array is usually fixed at the time of creation, and it cannot be resized dynamically during runtime.
Arrays can be one-dimensional or multi-dimensional, depending on the number of indices required to access the elements. In a one-dimensional array, each element is accessed using a single index or subscript. In a multi-dimensional array, such as a matrix or a table, each element is accessed using multiple indices or subscripts.
Arrays have several advantages, including fast access to individual elements, efficient memory allocation, and easy implementation. They are commonly used in a wide range of programming applications, such as sorting and searching algorithms, numerical simulations, and data processing.
22. What is the use of input strings in Java?
In Java, input strings are used to represent textual data that is entered by the user or obtained from a file or other input source. Input strings are a fundamental data type in Java, and they are used extensively in input/output operations, text processing, and user interfaces.
Input strings can be manipulated in a variety of ways using Java's built-in string manipulation methods. These methods allow you to perform operations such as concatenation, splitting, substring extraction, searching, and replacing.
Some common use cases for input strings in Java include:
-
Reading user input from the console: Input strings are often used to read user input from the console in command-line applications.
-
Parsing text files: Input strings are useful for parsing text files and extracting information from them.
-
Building user interfaces: Input strings are often used to represent text input fields in graphical user interfaces.
-
Web development: Input strings are commonly used in web development to represent form data submitted by users through HTML forms.
Overall, input strings are a versatile and essential data type in Java, and they are used in a wide range of programming applications.
23. What are the head pointer modification functions?
In programming, a "head pointer" typically refers to a pointer that points to the first node in a linked list. Head pointer modification functions are functions that modify the head pointer of a linked list in some way. Here are some common head pointer modification functions:
-
Insertion at the beginning of a linked list: This function adds a new node to the beginning of a linked list. It first creates a new node with the given data, sets its next pointer to the current head node, and then sets the head pointer to point to the new node.
-
Deletion from the beginning of a linked list: This function removes the first node from a linked list. It first checks if the list is empty (i.e., if the head pointer is NULL). If not, it saves a pointer to the current head node, sets the head pointer to point to the next node in the list, and then frees the memory of the saved node.
-
Reverse a linked list: This function reverses the order of a linked list. It starts by setting three pointers: prev to NULL, current to the head node, and next to the second node in the list. It then iterates through the list, setting current->next to prev, moving prev and current one node forward in the list, and updating next to point to the next node after current. Finally, it sets the head pointer to point to the last node in the original list (which is now the first node in the reversed list).
-
Splitting a linked list: This function splits a linked list into two separate lists. It first sets the head of the first list to the original head, and then iterates through the list until it reaches the node just before the midpoint. It then sets the next pointer of this node to NULL, effectively separating the two lists. Finally, it sets the head of the second list to the node just after the midpoint.
24. How do you get the next sibling in a binary tree?
In a binary tree, the next sibling of a node is the node that shares the same parent as the given node and appears immediately to the right of the given node.
To get the next sibling of a given node in a binary tree, we can follow the following steps:
- Get the parent of the given node.
- Check if the parent has a right child, and if so, return the right child.
- If the parent does not have a right child, then recursively traverse up the tree until we find a node that is the left child of its parent, and return the right child of that parent.
Here's the Python code for the same:
def getNextSibling(root, node):
# Base case
if root is None:
return None
# If given node is root
if root == node:
return None
# If given node is the left child of its parent
if root.left == node:
return root.right
# If given node is the right child of its parent
if root.right == node:
# Check if parent has a right sibling
if root.parent and root.parent.right:
return root.parent.right
else:
return getNextSibling(root.parent, root)
# Recurse on the left and right subtree
left_sibling = getNextSibling(root.left, node)
if left_sibling:
return left_sibling
else:
return getNextSibling(root.right, node)
Note that this algorithm has a time complexity of O(h), where h is the height of the tree. This is because we may need to traverse up the tree to find the next sibling, and the height of the tree is the worst-case scenario for this operation.
25. What is the regular matching of expressions in Python?
Regular expression matching in Python is a way of searching for patterns in text data using regular expressions. Regular expressions (regex) are a sequence of characters that define a search pattern. In Python, we use the re-module for regular expression matching. The most commonly used functions in the re-module are search, match, findall, sub, and split.
Here's a brief overview of each function:
- search(pattern, string, flags=0): This function searches for the first occurrence of the pattern in the string and returns a match object if found. If no match is found, it returns None.
- match(pattern, string, flags=0): This function checks if the pattern matches the beginning of the string and returns a match object if found. If no match is found, it returns None.
- findall(pattern, string, flags=0): This function finds all occurrences of the pattern in the string and returns them as a list of strings.
- sub(pattern, repl, string, count=0, flags=0): This function replaces all occurrences of the pattern in the string with the replacement string repl and returns the modified string.
- split(pattern, string, maxsplit=0, flags=0): This function splits the string at every occurrence of the pattern and returns a list of strings.
26. How to generate single-letter palindromic characters from a string?
To generate all single-letter palindromic substrings from a given string, we can follow the following algorithm:
- Initialize an empty list to store all single-letter palindromic substrings.
- Iterate over all characters in the input string.
- For each character, add it to the list of single-letter palindromic substrings.
- After iterating over all characters, return the list of single-letter palindromic substrings.
Here's the Python code for the same:
def generateSingleLetterPalindromicCharacters(s):
palindromes = []
for char in s:
palindromes.append(char)
return palindromes
This algorithm has a time complexity of O(n), where n is the length of the input string, since we are iterating over all characters in the string. The space complexity is also O(n), since we are storing all single-letter palindromic substrings in a list
27. Find all non-single letter substrings.
To find all non-single letter substrings in a given string, we can use the following algorithm:
- Initialize an empty set to store all non-single letter substrings.
- Iterate over all substrings of the input string with a length of at least 2 (i.e., excluding single-letter substrings).
- For each substring, check if it consists of more than one unique character. If it does, add it to the set of non-single letter substrings.
- After iterating over all substrings, return the set of non-single letter substrings.
Here's the Python code for the same:
def findNonSingleLetterSubstrings(s):
non_single_letter_substrings = set()
for i in range(len(s)):
for j in range(i+2, len(s)+1):
substring = s[i:j]
if len(set(substring)) > 1:
non_single_letter_substrings.add(substring)
return non_single_letter_substrings
This algorithm has a time complexity of O(n^3), where n is the length of the input string, since we are iterating over all substrings of length at least 2. The space complexity is also O(n^3), since we are storing all non-single letter substrings in a set. However, we can improve the time complexity of this algorithm by using more advanced algorithms for substring search, such as suffix trees.
28. What are Palindrome Substrings?
A string is said to be a 'Palindrome' if it is read the same forwards and backwards. For example, “abba” is a palindrome, but “abbc” is not. A 'substring' is a contiguous sequence of characters within a string.
Palindrome substrings are substrings of a given string that are palindromes, i.e., they read the same backward as forward. A substring is a contiguous sequence of characters within the given string. For example, consider the string "racecar". The palindrome substrings of this string are "r", "a", "c", "e", "ca", "ace", "cec", and "racecar".
Finding palindrome substrings is a common problem in computer science, and there are several algorithms to do so efficiently. One common approach is to use dynamic programming to build a table that stores whether a substring is a palindrome or not, and then use this table to find all palindrome substrings. Another approach is to use the two-pointer technique, where two pointers move from the center of a potential palindrome towards its edges, checking whether the characters at both ends match.
29. What are a source node and a destination node?
A source node and a destination node are terms commonly used in graph theory to describe the starting and ending points of a path or route between two vertices in a graph.
A graph is a collection of vertices (also called nodes) and edges (also called arcs or links) that connect pairs of vertices. In a directed graph, each edge has a direction, indicating the flow of the relationship between the two vertices it connects.
In this context, a source node is the starting vertex of a path or route, while a destination node is the ending vertex of the same path or route. For example, if you want to find a path from node A to node D in a directed graph, A would be the source node and D would be the destination node.
Microsoft Interview Questions - Intermediate to Hard
30. Find the longest increasing subsequence of a given array of integers, A.
We can solve this problem using dynamic programming. We will define an array dp of length n, where dp[i] represents the length of the longest increasing subsequence that ends at index i.
Initially, we set dp[i] = 1 for all i, since a single element is always an increasing subsequence.
We then iterate over the array A, for each element A[i], we compare it with all the elements before it A[j] (0 <= j < i). If A[i] > A[j], then we can add A[i] to the increasing subsequence that ends at j, thus increasing its length by 1. We then take the maximum of all such lengths to get the length of the longest increasing subsequence that ends at index i.
At the end of the iteration, we take the maximum of all values in dp to get the length of the longest increasing subsequence in the entire array.
Here's the Python code for the same:
def longestIncreasingSubsequence(A):
n = len(A)
dp = [1] * n
for i in range(1, n):
for j in range(i):
if A[i] > A[j]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
The time complexity of this algorithm is O(n^2) and the space complexity is O(n). However, it's also possible to solve this problem in O(n log n) time using a more advanced algorithm called Patience Sorting.
31. N children stand in one line. Every child is allocated a value of rating.
Candies are being distributed according to the conditions mentioned:
-
Every child should have a minimum of one candy.
-
Higher-rated children receive more candies compared to their neighbors.
At least what number of candies must you give to all the children?
To distribute candies to the children such that higher-rated children receive more candies compared to their neighbors, we can use the following algorithm:
- Initialize an array of candies with all values set to 1, as every child should have a minimum of one candy.
- Traverse the array of ratings from left to right, and for each child i, compare their rating with that of the child to their left (if i > 0) and the child to their right (if i < n-1).
- If the child to the left has a lower rating than child i, then set the value of candies[i] to candies[i-1]+1.
- If the child to the right has a lower rating than child i, then set the value of candies[i] to max(candies[i], candies[i+1]+1).
After this traversal, we can sum up the values in the candies array to get the total number of candies needed to satisfy the above conditions.
The above algorithm ensures that higher-rated children receive more candies compared to their neighbors, while also satisfying the minimum requirement of one candy per child.
The minimum number of candies needed to satisfy the above conditions is the sum of all values in the candies array.
32. Given an array of size n, find the majority element. The majority element is the element that appears more than floor(n/2) times.
To find the majority element in an array of size n, which is the element that appears more than floor(n/2) times, you can use a variety of algorithms, but one of the most efficient ones is the Boyer-Moore voting algorithm.
Here's the Java code to implement this algorithm:
public int majorityElement(int[] nums) {
int candidate = nums[0];
int count = 1;
for (int i = 1; i < nums.length; i++) {
if (nums[i] == candidate) {
count++;
} else {
count--;
if (count == 0) {
candidate = nums[i];
count = 1;
}
}
}
// Verify that candidate is the majority element
int majorityCount = 0;
for (int i = 0; i < nums.length; i++) {
if (nums[i] == candidate) {
majorityCount++;
}
}
if (majorityCount > nums.length / 2) {
return candidate;
} else {
return -1; // No majority element
}
}
Note that the time complexity of this algorithm is O(n) and the space complexity is O(1), which makes it very efficient for large arrays.
Microsoft behavioral interview questions and answers
Generally, in the Microsoft interview process, the technical round is followed by the HR round which includes situation-based or behavioral interview questions. While technical Microsoft interview questions assess the knowledge base of the candidate, behavioral questions are used to judge the personality traits of the candidate.
Behavioral questions help to understand your core values based on which the company decides whether one would fit well with the company values, besides your technical knowledge and skills. Based on this, the hiring manager checks your ability to make knowledge-based decisions.
The following are sample behavioral Microsoft interview questions for your review:
33. Do you use Microsoft products or services? If so, which is your favorite, and why?
This question allows the hiring manager to measure your familiarity with Microsoft and its products and services.
You can use it as a chance to highlight your wide range of relevant skills (for example MS Office skills) and previous experiences where you utilized Microsoft products. Additionally, you can tweak your approach based on the Microsoft job positions you seek and discuss the product or service that aligns with the nature of this job description and role.
Sample answer:
"I do utilize Microsoft products and services. If I had to choose one, then I'd go with Microsoft Office 365. Because Office 365 documents are saved in the cloud, I can view them from any internet-connected device, including when on the field. It's a great solution for managing work from wherever you are and sticking to deadlines."
34. How do you build and maintain functional workplace relationships or professional relationships with colleagues working in other locations?
Like any other company, Microsoft also prefers candidates who can coordinate effectively and easily with colleagues.
Sample answer:
"I've discovered that communication is the most important factor in establishing and maintaining all professional connections. Because having a deep bond help us to be more productive, I take concious steps to make significant connections. For example, in my previous role, I used to organize a relaxed monthly video-based team event. This added a new element that made us connect more on the team level."
35. What steps do you take to keep your skills updated?
Hiring managers like applicants who are self-motivated to learn. Hence, such questions are intended to understand this aspect of the student.
Sample Answer:
"I start by signing up for industry publications and following thought leaders on social media. I keep up with developing trends. I read articles, use free online learning tools, and work on personal projects in areas that interest me. This gives me the opportunity to learn, grow, and improve myself, ensuring that my skills are always up to date."
36. Where do you see yourself in 5-10 years?
Here too, you can take the opportunity to talk about your core values that align with the company values. You can talk about the job profile that you would like to get into.
Sample answer:
"I am very ambitious in my professional life. I am always eager to learn more and contribute towards the growth of the company to the best of my abilities. So, with the passion I have for my work, I see myself as a Senior Executive at a successful corporation like Microsoft in the next 5 years."
37. Why did you give an application for this specific role?
Here is one of the ways you can answer such types of Microsoft interview questions.
Sample answer:
"I came across the openings for software developers on your careers page and wanted to grab the opportunity to learn from the best. I believe that Microsoft would be the best environment for me to apply the skills I acquired during my internship and Bachelor's degree."
38. Do you have any skills relevant to this role?
"In my college and internship, I developed native/web apps with Java. I am appropriately versed in all aspects of app development and deployment. I have also developed a counter app, an airline ticket booking app, and email client software. I can handle roles in the front end and back end. I am also good at algorithm application and logic handling."
39. What do you like about this job description?
This question intends to understand the presence of your mind. Here is how you can handle it.
Sample answer:
"The job description is greatly written and provides all the information that is required for this role."
40. Why are you leaving your previous job?
This question is for experienced candidates. One important point to remember here is to never badmouth your previous company. Don't mention anything about the salary or behavior of people at the previous organization. Here is how you can answer it.
Sample answer:
"My previous company was extremely great for exposure to a different set of responsibilities. I had worked through the hierarchy and grasped what was there to learn on the job. Due to the stagnancy in learning, I decided that it would be best to move on."
41. In what type of workplace will you be happy and most productive?
"I like workplaces where we are given both freedom and responsibility. Also, the teamwork at that place should be great. It would be great if there would be fewer interruptions during work hours. I would prefer if there is no strict dress code unless we need to meet partners or clients."
42. What are your salary expectations?
"I’ve done some research on the average salaries for this type of role in my area and I think I would expect this role to pay me somewhere between X and Y. But I think we can discuss this further at a later time if you think I’d be a good fit for the role. Could you tell me the salary range you have in mind?"
43. Can you think of any problems you faced in any of your previous companies or internships?
"There was a certain instance where we faced a logjam related to an unconventional development process. It required a lot of hit and trial before we finally came to a solution."
44. How did you tackle the problem?
"As I have mentioned, we used the hit-and-trial methodology to come to a solution. But before we began, we analyzed the problem and broke it into several parts. Then, we analyzed those parts and saw if they resembled any previously solved problem. Then, we developed code for solving that specific portion of the problem. Slowly, we unified all the components and came to a final solution."
45. What did you learn from that specific problem?
"We learned that a more significant problem could be solved by being broken into smaller parts. Also, we learned how to rely on logical and analytical deductions to come to a solution for a particular problem."
46. How do you deal with criticism?
"If somebody gives me constructive feedback in the form of criticism, then I readily take it and work on improving myself. If somebody is trying to dampen my spirit, I try to ignore them and continue working."
47. Tell me about the gap in your resume.
"After college, I was working for N years and it was high time I needed a break to focus on my family and health. So, I went on a cross-country trip/world tour to regroup myself and expose myself to newer domains and experiences."
48. Tell me about your biggest achievement so far.
"As a software developer, I have made many personal and professional achievements. But there was a time when our team had to prepare for a project where I was in the core team as a developer. We had to upskill and then develop and deploy the code. We worked round the clock and completed the project in N months, before the target deadline. We learned and grew as a team. This is my greatest professional as well as personal achievement to date."
49. Do you have any questions of your own?
You must keep a list of your questions ready before going to the interview. Here are some questions that you may ask the interviewer.
Sample answer:
"Could you please tell me how many steps are remaining in the hiring process? Anything in particular that I need to know about them? Are there any new technologies that are being launched by the company?"
We hope these Microsoft interview questions and answers will help you amp up your placement preparation. Good Luck!
Here are some more suggested reads:
Comments
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Mangali Anjali 1 year ago