Difference Between Abstract Class And Interface In C# (In Detail)
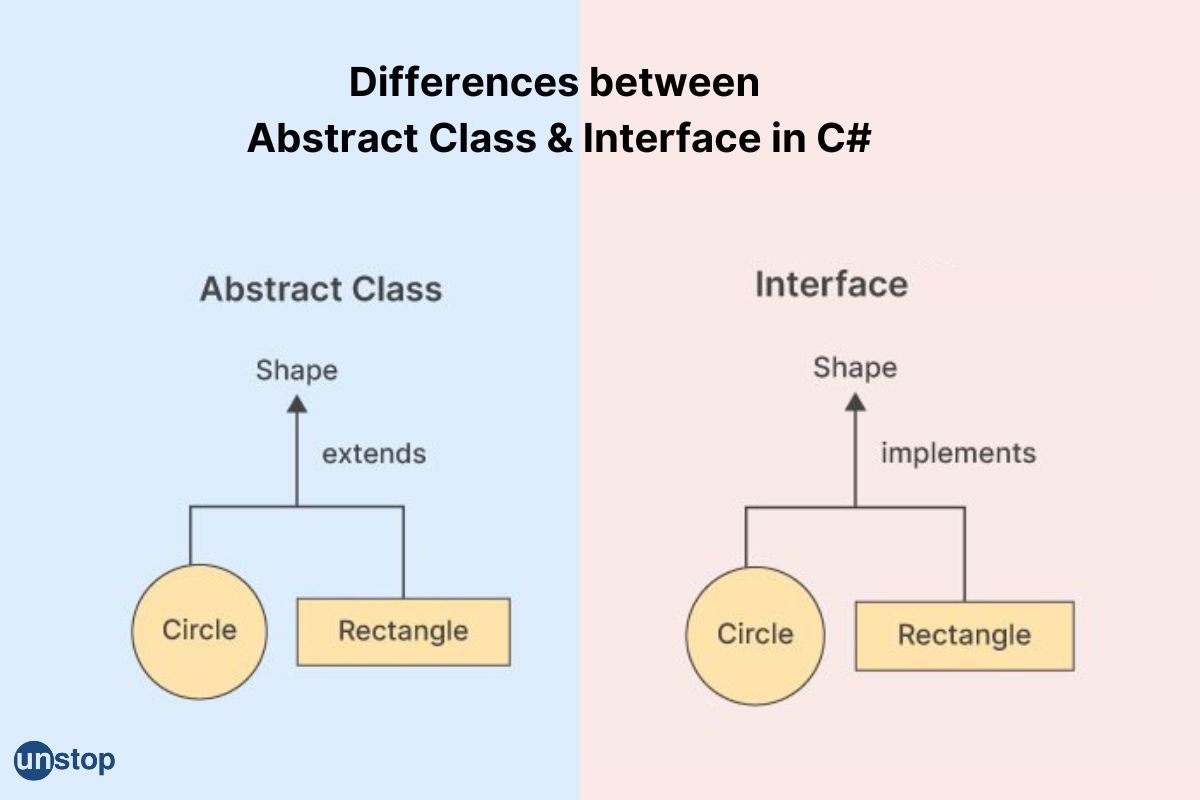
Microsoft created the object-oriented programming language C# as a part of the.NET framework. Since its initial release in 2000, it has become one of the most widely used languages for creating mobile apps, web services, and Windows programs.
C# is a strong yet user-friendly programming language integrating features from several other computer languages, including C++, Java, and Visual Basic. It offers development efficiency-enhancing features like type safety, garbage collection, scalability, interoperability with other languages via Common Language Runtime (CLR), and vast libraries.
One notable feature of C# is its support for creating platform-independent code using Xamarin or .NET Core frameworks. It allows building applications that can run on multiple platforms, including Windows desktops, iOS devices, Android devices, IoT devices, and more.
C# is widely used in industries ranging from finance to gaming due to its versatility and robustness. Its syntax resembles C-like languages making it accessible even for beginners while offering advanced functionality required by experienced programmers.
Abstraction in C
In C, abstraction hides unnecessary details and exposes only essential features or functionalities. It allows programmers to create complex systems by breaking them into smaller, more manageable parts.
Abstraction in C can be achieved through various mechanisms, such as:
1. Functions: By encapsulating a set of related instructions within a function, you can hide the implementation details from other parts of the program. This allows for code reuse and modular design.
2. Structs: In C programming language, structs define composite data types that group related variables together. They provide a way to abstract away multiple variables under one name.
3. Header files: By separating interface declarations (function prototypes) from implementation details in header files (.h), you can provide an abstract view of your functions to other modules without revealing their internal workings.
4. Typedefs: You can create new names for existing types or give custom names to structures or unions defined in your codebase. This helps improve readability and provides another level of abstraction by making code self-explanatory.
Broadly, abstract class and interface are used to achieve abstraction in C#. In this article, we'll study abstract class and interface in C#, key differences between the two, their advantages and disadvantages, and best practices. Read ahead:
What is Abstract Class?
An abstract class is a concept in object-oriented programming. It refers to a class that cannot be instantiated independently i.e. we cannot create objects of this type directly. But it can provide a common interface and functionality to its subclasses. It serves as a blueprint or template for other classes to inherit from.
Abstract classes are declared using the "abstract" keyword and may contain both regular methods with implementations and abstract methods with no implementation details.
One key usage of an abstract class is when you want to define some common behaviour across multiple related classes while also allowing customization in individual subclasses. Other important features are that it prevents direct instantiation, enforces use through derived classes, and provides structure and consistency within the inheritance hierarchy.
Example:
Output:
This is a shape.
The Area of the rectangle is: 50
Explanation of code:
- The abstract `Shape` class serves as a blueprint for shapes and has an abstract method `CalculateArea()` that must be implemented by any derived classes.
- The `Rectangle` class is a derived class from `Shape.` It implements the required `CalculateArea()` method, calculates the area of a rectangle using its length and width, and also inherits the non-abstract method (`Display()`) from its base.
- In the main program, we create an object of type Rectangle. We can call methods like Display(), inherited from Shape but cannot directly instantiate Shape because it is declared abstract. Then we calculate and display the area of this rectangle.
What is Interface?
In computer programming, an interface is a type that defines the methods or properties that a class must implement. It specifies each method's signature (name, parameters, and return type) without providing any implementation details.
An interface acts as a contract between different classes to achieve polymorphism and ensure consistency. Any class that implements an interface must define all its methods or properties declared in the interface.
Interfaces provide flexibility and allow multiple inheritance because a single class can implement multiple interfaces simultaneously. They also enable loose coupling since they separate the behaviour specification from its implementation.
Example:
Output:
The cat is eating
The cat is sleeping
Explanation of Code
We defined the 'Eat()' and 'Sleep()' methods for the 'IAnimal' interface. After that, we developed a " Cat " class that conforms to this interface. The 'Cat' class is where these methods are implemented.
Last but not least, in the main function of our program, we create an instance of the "Cat" class but assign it to a variable designated as type "IAnimal," which limits the methods we can call on it to those provided by the same interfaces ("Eat()" and "Sleep()").
Differences Between Abstract Class and Interface in C#
Let's take a look at the key differences between abstract class and interface in C#:
Abstract Class |
Interface |
It can have fields, properties, methods, and events, with or without implementation. |
It cannot have fields or implementation. It can have method, properties, and events, but only with signatures. |
Can provide a complete or partial implementation of a class. |
Only provides the definition; no implementation is allowed. |
Abstract class does not allow multiple inheritance |
Interface allows multiple inheritance. |
A derived class can inherit from an abstract class only once. |
Interface can implement multiple interfaces at once. |
Methods in abstract classes may contain access modifiers (public, private, etc.). |
All interface methods are public by default and do not allow access modifiers to be specified explicitly. |
Abstract classes can have constructors, including default ones, which are used for initializing the object's state when created. |
Interfaces do not allow constructors since they are not meant to create objects |
Similarities between Abstract Class and Interface
Let's take a look at the key similarities between abstract class and interface in C#:
Factor | Abstract Class & Interface |
Directly Instantiated | No. Both abstract class and interface in C# can't be instantiated directly. |
Provide common behavior | Yes, both abstract class and interface can provide common behavior to multiple classes. |
Polymorphism | Yes, both interface and abstract class can be used to achieve polymorphism (the ability of an object to behave differently depending on its type). |
Enforce Code Reuse | Yes, both interface and abstract class can be used to enforce code reuse i.e. use code that has already been written in other parts of the program. |
Advantages & Disadvantages of Abstract Class
Now that we've understood what an abstract class is, and how it's similar and different from interface, let's take a look at its advantages and disadvantages:
Advantages of Abstract Class:
1. Code Reusability: Abstract classes allow for code reusability, as multiple subclasses can inherit them.
2. Method implementation flexibility: An abstract class allows you to provide default implementations for some methods while leaving other methods to be implemented by its subclasses.
3. Encapsulation and Abstraction: Abstract classes help encapsulate common behaviour and characteristics of related objects into a single entity, promoting abstraction in the design.
4. Polymorphism support: Subclasses inherited from an abstract class can be treated as instances of their parent abstract class, facilitating polymorphic behaviour within a program.
5. Force implementation of specific methods: By defining certain methods as abstract in an abstract class, it enforces its derived classes to implement those specific methods.
Disadvantages of Abstract Class:
1. Limited inheritance: Java does not support multiple inheritance concerning classes; hence if a subclass already extends another base/abstract class or if there are restrictions on inheritance hierarchy due to architectural reasons, using an additional superclass (i.e., extending further) may become challenging when working with abstract classes.
2. Limited flexibility during runtime changes: Once a method is declared final within an abstract base class and provided with functionality inside it via concrete implementation, it cannot be overridden or modified by any subclass. This limits the flexibility to modify the behaviour of certain methods during runtime changes.
3. Rigid design structure: Abstract classes can lead to tight coupling between superclasses and subclasses since they rely on each other for implementation and inheritance. Changes in the superclass may require corresponding changes in its subclasses, leading to a rigid design structure.
4. Incomplete abstraction: Abstract classes are not completely abstract, as they can have both concrete and abstract methods within them. This makes them less pure than interfaces that only allow for abstraction through method declarations without any implementations.
5. Difficulty in testing: Since an abstract class cannot be instantiated directly, testing becomes difficult as we need to create concrete instances of subclasses derived from that particular abstract class.
Implementation of Abstract Classes in C#
To implement abstract classes in C#, follow these steps:
1. Start by defining the abstract class using the `abstract` keyword before the class name.
abstract class Vehicle
{
// ...
}
2. Declare any properties, methods, events, or indexers within the abstract class as you would in a regular class.
3. Any method-derived classes to implement must be declared with the `abstract` keyword and end with a semicolon instead of providing an implementation.
abstract void Drive();
4. Optionally, if desired, you can provide default implementations for some methods using either virtual or non-abstract non-virtual (concrete) methods inside your abstract class.
void TurnOnRadio()
{
Console.WriteLine("Radio is turned on.");
}
5. To inherit from an abstract base class and create concrete instances of derived classes, use the `: `<base-class-name>` syntax when declaring your child/descendant/derived classes.
6. Implement all inherited members marked as 'required' (`override`) from base (parent) classes.
Here's an example illustrating how to implement an abstract vehicle hierarchy:
Output:
Driving the car. It's a Toyota, manufactured in 2020.
The radio is turned on.
Riding the motorcycle. It's a Honda, manufactured in 2019.
The radio is turned on.
Explanation of the Code:
In this example, we have an abstract base class, `Vehicle` that defines two properties (`Brand` and `Year`) and an abstract method, `Drive().` The derived classes `Car` and `Motorcycle` inherit from the Vehicle class and implement their versions of the Drive() method. We also provide a default implementation for TurnOnRadio() inside the Vehicle class, which can be used by both Car and Motorcycle objects.
Advantages & Disadvantages of Interface
Now that we've understood what an interface is, and how it's similar and different from an abstract class, let's take a look at its advantages and disadvantages:
Advantages of Interface:
1. Ease of use: Interfaces provide a user-friendly environment that is easy to navigate and understand, making it convenient for users with varying levels of technical knowledge.
2. Accessibility: A well-designed interface ensures that persons with impairments may utilize tools like screen readers or keyboard navigation choices to properly access and interact with the system.
3. Efficiency: An intuitive interface allows users to complete tasks quickly and efficiently, reducing the learning curve associated with complex systems.
4. Consistency: Interfaces maintain consistency across different devices and platforms, providing a seamless user experience regardless of where the application is accessed.
5. Customization: Users can customize interfaces according to their preferences or requirements, allowing them to personalize their experience based on individual needs.
Disadvantages of Interface:
1. Complexity: In some cases, interfaces can be overly complicated, especially when dealing with complex software applications or extensive functionalities. This complexity may intimidate new users and require additional time for training or support.
2. Limited control options: Certain interfaces may limit user flexibility by offering only pre-determined methods for interaction without accommodating alternative approaches preferred by certain individuals (e.g., touchscreen-only mobile apps).
3. Learning curve: Although many interfaces aim for simplicity in design, there might still be a learning curve associated with understanding and getting used to a new interface, especially for users who need to become more familiar with similar systems or technologies.
4. Lack of personalization: While customization is generally an advantage, some interfaces may need more ability to personalize certain aspects or may have limited options for customization, which can result in a less tailored user experience.
5. Compatibility issues: Interfaces not designed to be compatible across different devices or platforms might lead to consistency in functionality and appearance when accessed from various sources (e.g., desktop vs mobile).
Implementation of Interface in C#
To implement an interface in C#, you need to follow these steps:
1. Define the interface: Define the interface with its methods and properties. For example, consider a simple interface for a shape:
public interface IShape {
double CalculateArea();
}
2. Implement the Interface: Next, create a class that implements this interface and provides the implementation for all its members (methods or properties). For example, let's create a class named "Rectangle" that implements the `IShape` interface:
public class Rectangle : IShape {
public double Width { get; set; }
public double Height { get; set; }public double CalculateArea() {
return Width * Height;
}
}
3. Use the implemented functionality: After implementing the required methods or properties defined in an interface, you can use them wherever needed.
class Program
{
static void Main(string[] args)
{
// Create an instance of Rectangle.
var rectangle = new Rectangle {Width = 5, Height = 4};// Call CalculateArea method from IShape.
var area = rectangle.CalculateArea();Console.WriteLine($"The area of rectangle is {area}");
}
}
4. Note that a single class can implement multiple interfaces. In such cases, separate the interface names with commas when implementing them.
public class Rectangle : IShape, IDrawable {
// Implementation of methods and properties from both interfaces goes here.
}
5. Make sure that your class follows an interface's declared contract by implementing it in C#. This enhances object-oriented programming's code organization, reuse, and polymorphism.
Example:
Output:
The area of the Rectangle is 20.
Explanation of the code:
In this program, we have defined an `IShape` interface with a single method, `CalculateArea().` Then, we implemented this interface in the `Rectangle` class by providing the implementation for its methods. In `Main()` method, we create an instance of `Rectangle,` set its width and height properties, and called its `CalculateArea()` to calculate and display the area.
When to Use an Abstract Class vs Interface in C#
In C#, both abstract classes and interfaces are used to define contracts for derived types. However, there are certain scenarios where using an abstract class is more appropriate, while in other cases, using an interface is the better option:
When to use Abstract Class
1. When you want to provide a common base implementation: Abstract classes can contain method implementations and abstract methods that derived classes must implement.
2. When you want to define shared member variables or properties: An abstract class can have fields and properties that its derived classes can access.
3. When you need versioning support: Adding new members or modifying existing ones in an abstract class does not break compatibility with already compiled code.
When to use Interface:
1. When multiple inheritance is required: A class in C# cannot inherit from multiple base classes but can implement multiple interfaces.
2. When different unrelated objects need similar functionality: Interfaces allow unrelated objects to share common behaviour without being tied together through inheritance hierarchies.
3. When creating contract-based programming models: Interfaces make it easier to create loosely coupled systems as they only specify what a type should do (contract), allowing flexibility on how it's implemented.
It's also worth noting that a single concrete type in C# may implement multiple interfaces but derive from only one abstract class. Therefore, an abstract class would be the better choice if you need to provide a common base implementation or define shared member variables. On the other hand, interfaces should be used if you require multiple inheritance or want to create contract-based programming models with unrelated objects.
Using Abstract Classes and Interfaces in Code: Best Practises
Both abstract classes and interfaces are commonly used in programming. Thus, here are some best practices for using the two:
1. When you need to offer a shared base implementation for several related classes, we use abstract classes. Abstract classes can contain concrete and abstract methods, allowing subclasses to override specific behaviours while inheriting the rest.
2. Use interfaces when you want to define a contract that all implementing classes must adhere to. Interfaces only contain method signatures without implementation details, providing flexibility in choosing different implementations based on the context.
3. Favor interfaces over abstract classes whenever possible, as they allow more flexible class hierarchies and avoid limitations imposed by single inheritance in languages like Java.
4. Carefully design your interface or abstract class hierarchy by identifying common behaviours or contracts among related classes before defining them.
5. Follow naming conventions that indicate whether a class is an interface (e.g., starting with "I" in C#) or an abstract class (using descriptive names).
6. Consider using default methods (available since Java 8) in interfaces if you need basic implementations shared across multiple implementing classes but want to avoid introducing an unnecessary base class hierarchy.
7. Treat interfaces as separate types rather than mere collections of methods; this allows better decoupling between components and promotes loose coupling principles such as Dependency Inversion Principle (DIP).
8. Use composition over inheritance whenever possible; prefer using interfaces to define the behaviour that a class should have rather than inheriting from abstract classes. This promotes code reusability and flexibility.
9. Use abstract classes when you want to provide common functionality or state across multiple subclasses, as they can contain instance variables, concrete methods, and abstract ones.
10. Be cautious about creating deep inheritance hierarchies using abstract classes, which can lead to complex and tightly coupled code. Instead, favour composition by combining smaller interfaces or using mixins (if the language supports it) for more modularity.
11. Document your interfaces clearly with comments describing their purpose, expected behaviour, and any pre-conditions or post-conditions that implementing classes must meet.
12. Ensure that your interface methods are well-named and self-explanatory; this makes it easier for other developers (including yourself in the future) to understand how the interface is intended to be used without diving into its implementation details.
13. Regularly review your usage of both interfaces and abstract classes during code reviews to ensure they align with design principles such as SOLID (Single Responsibility Principle), DRY (Don't Repeat Yourself), etc., promoting maintainable and extensible codebases.
Mistakes to Avoid in Abstract Classes and Interfaces
Programmers should look out for the following errors when using abstract classes and interfaces:
1. Not understanding the difference between abstract classes and interfaces: Abstract classes are partially implemented classes with both concrete and abstract methods. In contrast, interfaces only define method signatures without any implementation.
2. Overusing or incorrectly using abstract classes: It's important to use abstract classes when there is a clear relationship between the base class and its subclasses and when you want to provide some common functionality across multiple subclasses. However, using them can lead to more complexity in the inheritance hierarchy.
3. Confusing multiple inheritance with interfaces: Unlike other programming languages such as C++, Java doesn't support multiple inheritance of classes but does allow the implementation of multiple interfaces. The interface allows you to inherit behaviour from several unrelated types without limiting your ability to extend other class hierarchies.
4. Forgetting to implement all interface methods: When a class implements an interface, it must provide implementations for all methods defined in that interface; otherwise, it will result in compilation errors.
5. Using excessive abstraction levels: While abstraction is useful for creating modular code and reducing dependencies on specific implementation details, using too many layers of abstraction can make the code hard to understand and maintain.
6. Tight coupling with concrete implementations instead of abstractions: When working with abstract concepts like abstract classes or interfaces, it's essential not to depend too heavily on concrete implementations. Instead, focus on programming to the abstraction by using dependency injection and relying on interfaces or abstract classes as much as possible.
7. Ignoring the Liskov substitution principle: It states that any instance of a subtype should be able to be used in place of an instance of its supertype without affecting the correctness or behaviour of the program. Violating this principle can lead to unexpected runtime errors and incorrect results.
8. Not considering future changes: When designing abstract classes or interfaces, thinking about potential changes and extensions is essential. Failing to do so may result in tightly coupled code that requires significant refactoring when requirements change.
9. Not documenting interface contracts properly: Interfaces define a contract between different components/classes/modules/teams, etc. Developers who use these contracts (which invariably are other developers) must understand how they interact with them.
10. Not properly handling exceptions in abstract methods: Abstract methods can throw exceptions, and it's crucial to handle them appropriately in concrete implementations and when calling those implementations.
11. Mixing up inheritance with composition: While abstract classes and interfaces allow for code reusability through inheritance, they should not replace composition when appropriate. It's important to understand the differences between these two concepts and use them accordingly.
12. Overcomplicating code by using too many abstractions: Sometimes, developers overuse abstraction just for the sake of adhering to best practices or design patterns without considering if it adds real value or unnecessarily increases complexity.
13. Failing to follow naming conventions: When working with abstract classes or interfaces, following proper naming conventions is essential for the clarity and maintainability of code.
14. Not utilizing default method implementation (for Java 8 onwards): With Java 8 onward versions introducing default method implementation in interfaces, failing to utilize this feature may result in unnecessary boilerplate code or missed opportunities for adding common functionality across multiple implementing classes.
15. Poorly designing class hierarchies: A poorly designed hierarchy can make understanding relationships between different abstract classes or interface subclasses difficult.
Frequently Asked Questions
1. What is an abstract class and interface in C#?
An abstract class is a class that cannot be instantiated directly but can contain both concrete class and abstract methods. It acts as a blueprint for derived classes to inherit from.
Conversely, an interface is similar to an abstract class in that it also defines method signatures without implementations. However, unlike an abstract class, an interface cannot have any implementation or state variables.
2. How are abstract class and interface different from each other?
The major differences between an abstract class and an interface lie in their purpose and usage:
- Purpose: An abstract class provides common functionality among related classes by defining base behaviour through inheritance.
- Implementation: Abstract classes can provide default implementations for certain methods or properties while leaving others as purely virtual (abstract). Interfaces only declare method signatures without providing any implementation.
- Inheritance vs Multiple Implementations: A single subclass can only inherit from one parent (abstract) class due to C#'s lack of support for multiple inheritance to classes.
3. Can interfaces have static methods?
Interfaces cannot have static methods until C# version 8.0 (introduced in September 2019). In earlier versions of C#, only concrete types like classes were allowed to contain static members.
4. How does using an interface differ from using an abstract class?
Using an interface promotes loose coupling between components since it defines contracts without providing implementation details.
In contrast, using an abstract class establishes a tighter relationship between base and derived types, as the derived type inherits both behavior and structure from its parent.
5. What is a public access modifier in C#?
The public access modifier allows unrestricted access to the member or type from any program part. It provides the highest level of accessibility.
6. Can you give an example of an actual method with the public access modifier in C#?
Sure, here is an example of a method declared as public:
public void PrintMessage(string message)
{
Console.WriteLine(message);
}
7. What types of access modifiers are there in C#?
There are three other access modifiers in C# in addition to "public": "private," "protected," and "internal." These offer varying accessibility levels within diverse domains.
8. What exactly does the C# IEnumerable interface do?
The IEnumerable interface provides a method for iterating through a collection or sequence. It specifies techniques for iteratively looping through elements.
We hope this makes it clear how abstract class and interface function, the differences between the two and their advantages and disadvantages.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
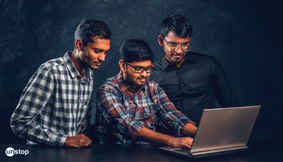
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
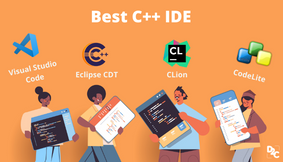
10 Best C++ IDEs That Developers Mention The Most!
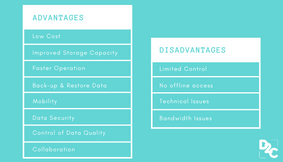
Advantages and Disadvantages of Cloud Computing that you should know!
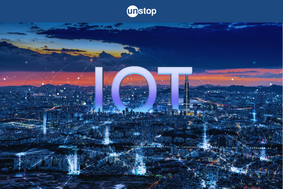
Comments
Add comment