Pointer To Object In C++ | Declare, Usage & More (+Code Examples)
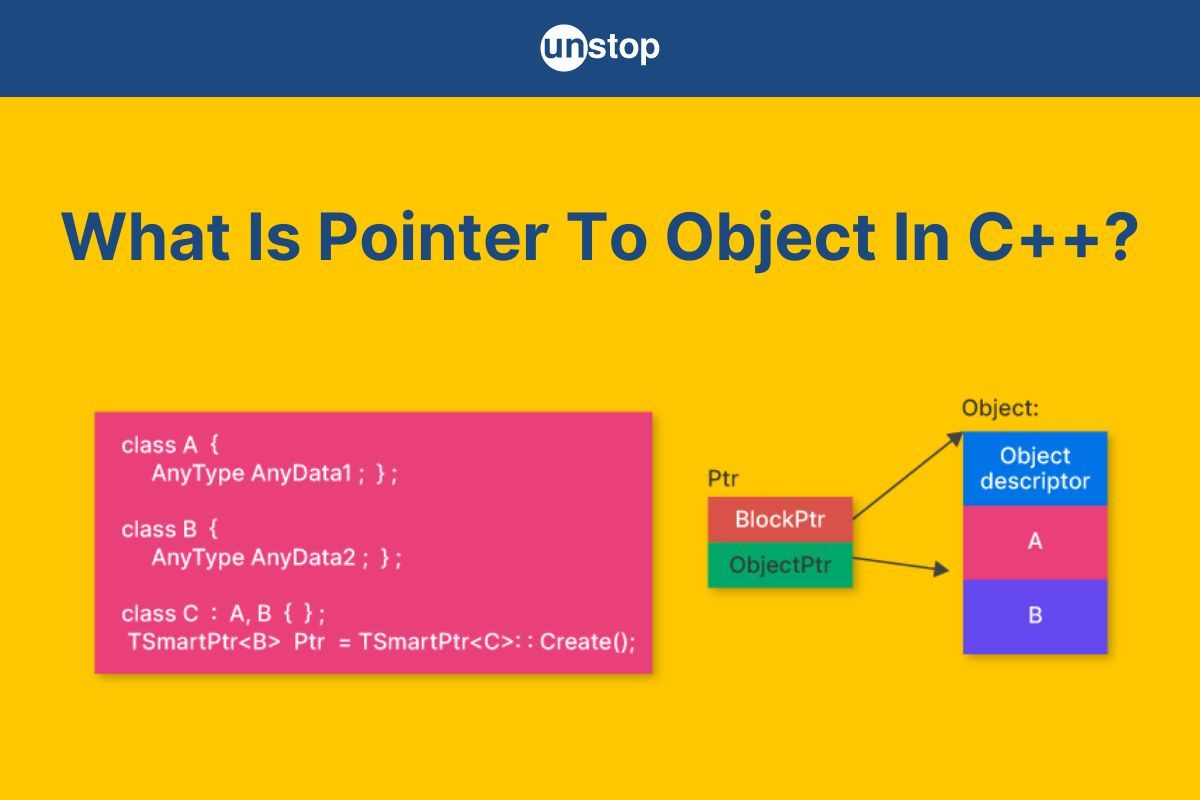
Pointers are a fundamental concept in C++, enabling programmers to manipulate objects indirectly (using the address for access) and dynamically allocate memory. In that, pointers to objects in C++ classes, provide powerful capabilities for memory management, allowing developers to allocate and deallocate memory dynamically. In this article, we will explore the concept of pointers to objects, their advantages, and best practices for using them effectively in C++ programming language.
Understanding Pointers In C++
In C++, a pointer is a variable that holds the memory address of another variable. Pointers allow for efficient memory utilization, as they enable dynamic memory allocation, deallocation, and indirect access to objects/ data. When declaring a pointer, you must specify the data type of the variable it will be pointing to. For example, int* ptr declares a pointer to an integer variable.
Syntax For Pointer Variable Declaration In C++
datatype *var_name;
int *ptr; // Ptr can direct attention to an address that contains int data.
Let's look at an example to understand the implementation of pointer variables in C++ programming.
Code Example:
Output:
The value of x is: 42
The address of x is: 0x7ffef459dc14
The value of ptr is: 0x7ffef459dc14
The value pointed to by ptr is: 42
The new value of x is: 100
Explanation:
In this example, we first include the <iostream> header for input/ output operations.
- Inside the main() function, we declare and initialize the integer variable x with a value of 42.
- Then, we declare a pointer variable ptr and assign it the address of variable x, using the address-of operator (&).
- After that, we use the std::cout commands to print the value of variable x (direct access), the value of the ptr/ address of x twice (first using &x and second using ptr directly), and the value pointed to by ptr using the pointer notation (*ptr).
- Next, we use the dereference operator (*) to modify the value being pointed to, by assigning the value 100 to ptr. The output shows that the modification was successful as we print the new value.
- Lastly, the main() function returns 0, indicating the successful execution of the program.
This C++ program shows how pointers can be used to access a variable's memory address and change its value indirectly.
For more, read: Pointers in C++ | A Roadmap To All Types Of Pointers With Examples
Pointer Reference Guide
Syntax |
Meaning |
int *p |
A pointer p is declared. |
p = new int |
Creates a dynamic memory integer variable and assigns its address to p. |
p = new int[7] |
Inserts the address of the first index of a dynamic array of size 7 in p. |
p = &var |
Pointer stores directly to the var variable. |
*p |
Reads the object's value that p points to |
*p = 7 |
Changes the object’s value that p points to |
p |
Reads the object's memory location that p corresponds to |
What Is Pointer To Object In C++?
A pointer to an object in C++ is a variable that holds the memory address of an object. It allows indirect access to the object, providing a way to manipulate and interact with the object dynamically. Pointers to objects are particularly useful when dealing with dynamically allocated memory, enabling flexible memory management and dynamic object creation.
When we create an object for a class, it occupies a specific memory location. Here, a pointer to object in C++ class stores the memory address of that object rather than the object itself. These pointers allow programmers to access and modify the object indirectly, without creating its copies. This is especially useful for efficient memory usage and passing objects to functions by reference. Below is an example that illustrates the concept of a pointer to object in C++.
Code Example:
Output:
Hello from MyClass!
Explanation:
In the example above,
- We define a class named MyClass, containing a public member function myFunction() that uses std::cout to print a message to the console.
- In the main() function, we create an instance/ object of MyClass named obj.
- Then, we declare a pointer ptr of type MyClass* and assign it the memory address of obj using the address-of operator (&).
- After that, we show how we can use this pointer along with the arrow operator/ 'this' pointer (->) to access the member function myFunction() of the obj object.
- As shown in the output, even though the myFunction() is a part of the class, we can access it using a pointer to object of that C++ class.
Pointers to objects provide flexibility in scenarios where objects need to be created dynamically, their lifetimes extend beyond a specific scope, or when objects are accessed indirectly for efficiency purposes. They are commonly used in data structures, dynamic memory allocation, object-oriented programming, and other advanced programming techniques.
Declaration And Use Of Object Pointers In C++
The process for creating object pointers is similar to that of ordinary pointers, i.e., appending it to the name of the pointer type. However, note that we use the arrow operator (->) instead of the dot operator when utilizing an object pointer to access members of an already-defined class.
The example program below illustrates the use of object pointers in C++.
Code Example:
Output:
Initializing data members using the object with values 1, 1, and 2000
Printing members using the object: The date is 1/1/2000
Printing members using the object pointer: The date is 1/1/2000Initializing data members using the object pointer, with values 31, 12, and 2023
Printing members using the object: The date is 31/12/2023
Printing members using the object pointer: The date is 31/12/2023
Explanation:
In the above code example-
- We start by defining a class Date with three private integer data members: day, month, and year.
- Then, we use the public access specifier and create a default constructor, Date(), that initializes the day, month, and year to 0.
- We then define a member function setData() to set the values of day, month, and year data members using the parameters d, m, and y. This allows us to change the date information later.
- Next, we define a printData() function that outputs the date in a day/month/year format using the std::cout command.
- In the main() function, we create an object of Date class, D1, and a pointer dptr of type Date (i.e., it will point to object of Date class).
- First, we use the dot operator to call the setData() function on object D1 to initialize its data members with values 1, 1, and 2000, respectively.
-
We then print these values using the printData() function, which outputs the date to the console. (i.e., D1.printData())
- Next, we assign the address of D1 to the pointer dptr using the address of operator(&). This will make the pointer dptr point to the same memory location as D1.
- After that, we call the printData() function using the pointer to the object, dptr, and arrow operator, i.e., dptr->printData().
- Then, we update the date using the pointer dptr by calling the setData() function with values 31, 12, and 2023. This modifies the values in the object D1 because both dptr and D1 refer to the same instance.
- Finally, we print the updated date by calling the printData() function twice, once by using the object name (D1) and once by using the pointer to the object (dptr).
- As seen in the output, both the calls to the function print the same value, showing modifications made using pointers to objects in C++ classes, modify the object.
This program shows C++ users how to declare a pointer to an object, use it, and access member functions. Aside from being presented as functions that take an object pointer in their implicit arguments, the said base pointer could also be utilized to reach the members and procedures of the object.
Advantages Of Pointer To Object In C++
The advantages of using a pointer to an object in C++ are as follows:
- Pointers grant us the power to dynamically distribute storage, enabling object creation at execution and releasing memory when it is not needed. It is especially useful in situations where either the generation of objects is dependent on user input, there are other runtime conditions, or knowledge of an object's size at compile time is lacking.
- When compared to passing by value, pointers to objects allow us to pass objects to functions by reference, which can be more effective. When we provide an object by value, a copy of the item is made, which for large objects can be time and memory-consuming. It is more effective to pass an object by reference, which merely passes the item's address.
- Incorporating polymorphism into our coding is a viable option, as instances of derived classes can be pointed to by including pointers from their base equivalents. As long as they share a base class, we can develop code that interacts with objects of various sorts.
- Smart pointers offer ownership semantics for objects that are allocated dynamically. Objects' lifespan can be governed using these effective programming tools to ensure they are properly disposed of when no longer necessary. This guarantees accurate deallocation and prevents any unintended consequences from the object's persistence.
Pointer To Objects In C++ With Arrow Operator
We've already seen the use of the arrow operator/ 'this' pointer in the examples above. In this section, we will discuss the use of this operator to access an object's members referenced by a pointer in detail.
- We should use the arrow operator instead of the (.) dot operator in cases where we possess an object pointer.
- This is because the arrow operator is a viable means to access members belonging to that specific object.
Also note that the arrow operator is a combination of the dereference operator (*) and the dot operator (.). It is used to access the member of the object that the pointer points to and dereference the pointer.
Syntax:
(pointer_name)->(variable_name)
Below is an example program that shows how to use the C++ arrow operator with pointers to objects.
Code Example:
Output:
The value of x is: 42
Explanation:
In this example, we define a class named MyClass with a public integer member variable x and a public member function print().
- The print() member function outputs the value of x using the std::cout command.
- In the main() function, we create an object obj of type MyClass.
- Next, we assign the value 42 to the member variable x of the object obj.
- We then declare a pointer ptr of type MyClass* and assign it the address of obj using the address-of-operator (&).
- Finally, we use the pointer ptr to call the print() function by using the arrow operator (->), which prints the value of x through the pointer.
This program shows how to use the arrow operator with object references and pointers to objects in C++ classes. In instances where simple functions necessitate the input of an object pointer as a parameter, one may utilize the arrow operator to gain access to both methods and members of the object.
An Array Of Objects Using Pointers In C++
A group of related data objects stored in contiguous memory locations can be accessed randomly using an array's indices in C++ or any other programming language. Arrays can be used to store elementary data types such as int, float, double, or char.
An array of objects:
No memory or storage is allocated when a class is defined; only the object's specification is defined. You must build objects in order to use the data and access the class's defined functions.
Syntax:
ClassName ObjectName[number of objects];
Code Example:
Output:
Jia is 25 years old.
Maira is 30 years old.
Bhaskar is 35 years old.
Explanation:
- In this program, we define a class named Person with two public data members: name (of type string) and age (of type int).
- Inside the main() function, we dynamically allocate an array of Person objects using the new operator and assign it to the pointer people.
- We then initialize the elements of the array individually, using index values and dot operator:
- For the first Person object (people[0]), we set the name to "Jia" and the age to 25.
- For the second Person object (people[1]), we set the name to "Maira" and the age to 30.
- For the third Person object (people[2]), we set the name to "Bhaskar" and the age to 35.
- Finally, we print the array elements by iterating through it using a for loop and dot notation to access the name and age member variables of each object.
- In order to prevent memory leaks, we then release the memory allocated by new using the delete[] operator.
Base Class Pointer For Derived Class Object In C++
In C++, a pointer to a base type class object may point to an object of a derived class. This is conceivable as a result of derived classes, which are classes that borrow characteristics from their base classes. It is possible to employ both a reference pointing towards an object of either the base class or derived class as they are compatible in type and offer several benefits. There exist diverse applications for working with these pointers to objects that belong to different classes.
Notes:
- A base class pointer referring to a derived class is a pointer to a derived class, but it will retain its aspect.
- The pointer of the base class is endowed with the ability to modify its distinct functions and variables while simultaneously being directed toward an object that originates from a subclass.
Here is a program to show a base class pointer can be used as the pointer to object of derived class.
Code Example:
Output:
This is a rectangle.
This is a circle.
Explanation:
In this program, we first include the standard Input/Output header file.
- We then create a base class named Shape with a public virtual function named display(). It prints a message to the console using the cout statement.
- Next, we create another class named Rectangle, which inherits the base class Shape and overrides the display() function. It prints a different method than the function from the base class, overriding it with its own definition.
- Then we create another class named Circle, which inherits the base class Shape and overrides the display() function, just like the Rectangle class.
- In the main() function, we declare the Shape class pointer (Shape*) and create objects r and c for the Rectangle and Circle classes, respectively.
- We then call the display() function of the derived class using the arrow operator (->) and point the base class pointer s to the object r.
- We will print an output to the console when the display() function is implemented within an instance of class Rectangle.
- Then we once again call the display() function of the derived class after we have pointed the base class pointer s to the object c.
- This time, it will invoke the Circle class' display() method and print the output to the console.
We can implement polymorphism in our program and create more adaptable, reusable code by employing a base class reference to point to derived class objects.
Conclusion
Within C++, the pointer variable is utilized to contain and manage the memory addresses of objects, permitting indirect entry into said objects. This approach enables us with a means of accessing objects outside their immediate scope without any difficulty or complication by using pointers instead.
When employing pointers to objects, we must take responsibility for memory management. Within the context of C++, pointers act as variables capable of storing and, more vitally, directing toward another variable's address. It is vital to note that these entities bear an identical data type in comparison with regular variables.
One may employ pointers to instantiate memory allocation and deallocation dynamically, create as well as alter dynamic data structures, and simulate call-by-reference functionality. Through the memory location it stores, a pointer indirectly references a value, and a reference enables us to operate an object using a pointer without having to manage memory manually.
Frequently Asked Questions
Q. How do you declare a pointer to an object in C++?
We use a particular syntax for declaring object pointers in the context of C++.
Syntax:
class-name *object-pointer-name
Here, the pointer variable is declared with the * operator.
Q. How can we write a program to illustrate pointers in C++?
Here is an example of how to write a program to show pointers in C++.
Code Example:
Output:
Before swapping: x = 10, y = 20
After swapping: x = 20, y = 10
Explanation:
This program describes the function swap, which swaps the values pointed to by two integer pointers as arguments using a temporary variable.
- The program declares and initializes the two integer variables x and y to 10 and 20, respectively.
- It prints their values prior to swapping, uses their addresses to invoke the swap function, and then prints their values following the swap.
- The dereference operator * is used by the swap function to access the values pointed to by the two integer pointers it receives as arguments.
- The addresses of x and y are passed to the swap function using the & operator.
Q. What is the difference between a pointer to an object and a reference to an object in C++?
In C++, one may find that a reference dwells as an alias to any given variable within existence, while alternatively, a pointer takes shape as an object meant for storing memory addresses assigned to other variables or objects. Although a reference has the ability to refer solely to one object, pointers have the capability of concurrently pointing toward multiple objects.
Q. Can a pointer point to another pointer in C++?
Yes, in C++, a pointer can point to another pointer. This is known as a pointer to a pointer or double pointer. A double pointer holds the address of another pointer, which in turn holds the address of a variable or object.
When working with class hierarchies in C++, what can hold the address of a pointer to the base class is another pointer to the base class or even a pointer to a pointer. This is useful when dealing with inheritance, polymorphism, and dynamically allocated objects, as it allows flexible handling of class pointers and facilitates dynamic binding in polymorphic scenarios.
Q. Can we return a pointer in C++?
Yes, we can return a pointer in C++. Here is an example of the same.
Code Example:
Output:
1 2 3 4 5
Explanation:
In this code fragment, the createArray() function, which accepts an integer argument size and returns a pointer to an integer, is defined in this program.
- The process employs the new operator to assign memory space for an array of integers.
- It then proceeds to initialize the aforementioned collection with certain values and consequently returns a pointer to the array's first element.
- Next, we invoke createArray() with argument 5 in the main() function, and we store the resultant pointer in the ptr variable.
- Then, by employing pointer arithmetic, we traverse through the array and output each entry.
- Finally, we use the delete[] operator to release the memory that the new operator had allocated.
Q. What are the different types of pointers in C++?
Here are the wide range of pointers available in C++:
- Null Pointer: A pointer that is initialized to nullptr (or NULL in older code), indicating it doesn't point to any valid memory location.
- Void Pointer: A generic pointer that can point to any data type but must be cast to another pointer type before dereferencing.
- Pointer to Object: A pointer that points to an object of a specific class or structure.
- Pointer to Member: A pointer that points to a member of a class (either a data member or a member function).
-
Pointer to Structure: A pointer that points to an instance of a struct. It allows access to the members of the structure through the pointer using the arrow operator (->).
- Pointer to Function: A pointer that points to a function, allowing functions to be called indirectly.
- Pointer to Pointer: A pointer that holds the address of another pointer, enabling multiple levels of indirection.
- Smart Pointer: A pointer provided by the C++ Standard Library that manages the lifetime of an object automatically. Examples include std::unique_ptr, std::shared_ptr, and std::weak_ptr.
- Array Pointer: A pointer that points to the first element of an array. It allows for array traversal and manipulation.
- Dynamic Pointer: A pointer used with dynamic memory allocation (e.g., new and delete operators) to manage memory at runtime.
- Dangling Pointer: A pointer that continues to hold the address of a memory location that has been deallocated or freed.
Q. Can two pointers point to the same object?
Yes, in C++ languages, two pointers can point at the same object. In the event two pointers are employed to refer to identical objects, they shall indicate the same value as a result of utilizing one and the same memory location.
Q. How do you free memory for an object using a pointer in C++?
You can use the delete operator to free memory for an object in C++ using a pointer. The snippet below will give you an idea of how this is done.
// Dynamically allocating an object
MyClass* ptr = new MyClass();
// Freeing memory for the object
delete ptr;
In this instance, we engage in the dynamic allocation of an object belonging to the predefined class known as MyClass through the utilization of the new operator. The pointer to said object is subsequently deposited within a variable named ptr. To free the memory allocated for the object, we use the delete operator with the pointer.
Q. What is the difference between delete and delete[] in C++?
Memory allotted by new and new[] is released using delete and delete[] in C++, accordingly. The two approaches of memory deallocation for arrays are fundamentally different from one another. Also, memory allotted for a single item can be freed via delete. To release memory reserved for an array of objects, use delete[].
This compiles our discussion on pointers to objects in C++, here are a few more interesting articles that you must check out:
- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
- Strings In C++ | Functions, How To Convert & More (With Examples)
- Difference Between C And C++| Features | Application & More!
- 10 Best C++ IDEs That Developers Mention The Most!
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment