Array Of Pointers In C & Dereferencing With Detailed Examples
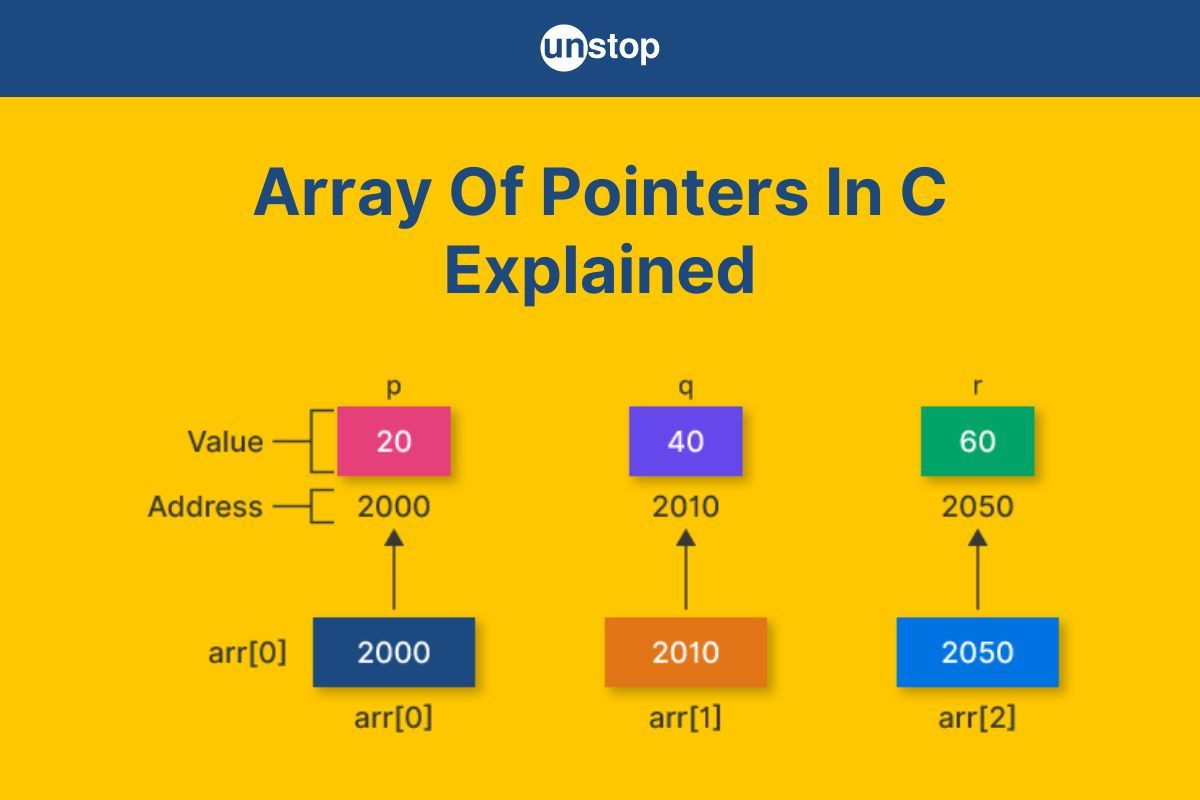
Arrays and pointers are fundamental concepts in the C programming language, each playing a crucial role in manipulating data efficiently. When these two concepts are combined, we get a powerful construct called an array of pointers in C. This advanced data structure increases flexibility and dynamic memory management in C programming. In this article, we will delve into the intricacies of the array of pointers in C, its uses, advantages, and how it enhances the capabilities of C programs.
What Is An Array?
An array is a collection of entities of the same data type stored in continuous memory locations. The size of an array is fixed. It can store data types such as int, char, float, pointers, structures, etc. We can also access any element present in the array by using indexes. Indexing starts from 0 in arrays, i.e. the first element of the array can be accessed by arr[0].
Syntax:
data_type array_name [size] = {element1, element2, ... elementSize}
Here,
- Data_type is the type of data the elements of the array are of.
- Array_name is the name of the array.
- Size is the no of elements present in the array.
- element1,element2,....elementSize are the elements of the array array_name.
Example:
- int arr[5] = {0,1,2,3,4}; //Here, we have declared an array of size 5 and initialized it with elements 0,1,2,3 and 4.
- We can access individual elements in the example above by using indexes, i.e., element 3 can be accessed by arr[3]. It is also possible to update elements, i.e., arr[0]=5, so now the array will have {10,1,2,3,4}
- We can also declare an array without mentioning size, i.e., int arr[]={0,10,20};
- We can initialize an array afterward using loops and also traverse all elements using them.
General Uses Of Arrays
- Sequential Storage: Store a collection of variables of the same data type in a contiguous memory block.
- Indexed Access: Access elements using indices for quick and direct retrieval.
- Loop Iteration: Simplify repetitive tasks by iterating over array elements using loops.
- Fixed-Size Collections: Create fixed-size collections of data.
- Strings: Represent and manipulate strings using character arrays.
- Efficient Memory Usage: Optimize memory usage by grouping related data in a structured manner.
- Function Parameters: Pass arrays to functions, enabling the manipulation of data within functions.
- Multi-dimensional Arrays: Represent and work with multi-dimensional data structures efficiently.
- Sorting and Searching: Implement sorting and searching algorithms on arrays for data analysis.
Read more- Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
What Is A Pointer?
A pointer is used to store the memory address of other variables(integers, char, etc), arrays, structures, functions, or even other pointers. This means that the pointer points towards the location (space in memory) where a value/ variable is stored. We can use pointers to access and manipulate the data stored in the respective memory location.
Syntax:
datatype *ptr;
Here,
- Datatype is the type of data the pointer variable ptr will point to.
- The indirection operator/ asterisk (*) is added to indicate that it is a pointer variable.
- ptr is the name of the pointer.
Example:
int x = 100;
int *ptr; // Pointer declaration
int *ptr = &x; //Declaration and initialization together
General Uses Of Pointers
- Dynamic Memory Allocation: Allocate memory dynamically during program execution.
- Function Parameters and Return Values: Pass arrays or structures efficiently to functions using pointers.
- Pointer Arithmetic: Efficiently traverse arrays and data structures.
- Arrays and Strings: Arrays and strings are implemented using pointers.
- Dynamic Data Structures: Create dynamic data structures like linked lists, trees, and graphs.
- Pointers to Functions: Pointers can point to functions, enabling dynamic function calls.
- File Handling: Used in file handling operations, such as reading and writing data to files.
What Is An Array Of Pointers In C?
An array of pointers in C language is a data structure that consists of a collection of pointers. That is, each element of an array of pointers holds the memory address of another variable or data structure. In this sense, an array of pointers can also be referred to as a collection of memory addresses.
Instead of storing actual data values, an array of pointers stores references to memory locations. This provides a level of indirection, allowing greater flexibility in managing and accessing data. Each element in the array is a pointer, and it can point to variables of different types or dynamically allocated memory blocks.
Arrays of pointers are commonly used in scenarios where dynamic memory allocation is required or when dealing with data structures with varying sizes. They offer advantages in terms of memory efficiency, flexibility, and ease of manipulation, especially when working with complex data structures or when the size of the data is not known at compile time.
Declaration And Initialization Of An Array Of Pointers In C
Arrays of pointers can be used when we want to point at multiple memory locations of similar data types. We can use these arrays for data types like characters, integers, strings, and different types.
To declare an array of pointers in C, we must specify the data type of the elements, the array name, and the size of the array of pointers. Also, note that we use an asterisk(*) to signify it is an array of pointers.
Syntax To Declare Array Of Pointers In C
data_type (*array_name)[size_of_array];
Here,
- data_type refers to the type of data the pointer variable is pointing to.
- array_name is the placeholder for the name you give to the array of pointers.
- size_of_array is the size of the array of pointers, i.e., the number of pointers in the array.
- The asterisk (*) is used to specify that it is an array of pointers.
For Example: int *arr[5];
In this example, we have declared an array of pointers with the size 5. That is, the array named arr can have 5 elements of integer data type.
Here, we have not initialized the array, i.e., we have not assigned the elements/ values. To initialize the array of pointers, we use the referencing/ address-of operator (&) to point to the respective data types by simply assigning the addresses to the array of pointers.
Syntax For Initializing Of Array Of Pointers In C
data_type (*array_name)[size_of_array] = {add1,add2,...addsize};
Here,
- data_type is the type of the data the pointer variable is pointing to.
- array_name is the name of an array of pointers.
- size_of_array is the size of the array of pointers.
- The asterisk (*), also known as the indirection operator, specifies that it is an array of pointers.
- add1,add2,...addsize are the elements of the array, i.e., addresses of the data type the particular pointers point to.
We can access the addresses of the data types inside the array by using the address-of (&) operator.
For Example:
int v1=11,v2=22,v3=33;
int *arr[3] = {&v1,&v2,&v3};
Here v1,v2 and v3 are 3 integer variables. The array of pointers, i.e., arr, contains the memory locations of v1,v2, and v3, assigned using the & operator.
Code Example:
Output:
0x7ffc53220110
0x7ffc53220114
0x7ffc53220118
0x7ffc5322011c
Explanation:
In this simple C program, we see how to declare and initialize an array of integer pointers and print the addresses/ array elements to the console. We begin by including the <stdio.h> header file for input/ output operations.
- Inside the main() function, we declare and initialize an integer array t with four elements, i.e., {1, 2, 3, 4} separated by commas.
- Next, we declare an array of integer pointers arr, and each element of this array is assigned the address of the corresponding element in the array t.
- As mentioned in the code comments, we then use a for loop to initialize the elements of the array of pointers.
- The loop control variable i, initially set at 0, assigns the address of the ith element of the integer array to the ith element of an array of pointers using the address-of operator (&).
- After every iteration, the value i is incremented by 1, and the loop continues until the condition i<4 remains true.
- We then use another for loop to traverse the array of pointers and print its elements using the printf() function. The %p format specifier indicates the pointer value, and the newline escape sequence moves the cursor to the next line.
- The output of the program is a series of hexadecimal memory addresses, each corresponding to the memory location of an element in the array t.
- Finally, the main function returns 0, indicating successful execution without any errors.
Time Complexity: O(size of pointer array)
Working Mechanism Of Array Of Pointers In C
The basic definition of an array of pointers in C is a collection of numerous pointer variables stored in contiguous memory locations. Each pointer variable in the simple array stores the memory location of some other variables of the same data type.
The mechanism of creation of an array of pointers in C is as follows:
- The first step is to create variables that, when initialized, take up a space in the memory.
- Then, we define an array of pointers of a certain fixed size by specifying the data type of the elements the pointers will point to, the name of the array of pointers, and the number of pointers (elements) it will have.
- Every pointer in this array will point to the address of the variables initialized in the first step. Note that the data type of all these elements must be the same.
- So, we assign the address to the elements of the array of pointers using the address operator (&) and assignment operator (=).
- Once the addresses are attached to the elements of the array of pointers, we can perform various operations on these elements. For example, we can access and print the addresses using the dereference operator (*) and the index.
Code Example:
Output:
Value of v1: 10 Address: 0x7fff1ac65480
Value of v2: 20 Address: 0x7fff1ac65484
Value of v3: 30 Address: 0x7fff1ac65488
Explanation:
In this example C code-
- We declare and initialize three integer variables, i.e., v1, v2, and v3, and initialize them with values 10, 20, and 30, respectively.
- Then, we declare an array of integer pointers, i.e., parr and initialize its elements with the addresses of the variables above, using the address-of operator (&).
- Next, we use a for loop to iterate through each element of the array of pointers.
- The loop contains a printf() statement, which displays the value of each variable and its corresponding address.
- The formatted string in the statement uses %d and %p format specifiers to indicate the place where integer and pointer values will go.
- The newline and tab escape sequences shift the cursor to the next like and by a tab space, respectively.
- It prints the index-adjusted variable name (var1, var2, var3), the value of the variable (i.e., *parr[i]), and the memory address of the variable (i.e., parr[i]).
- Finally, the main function returns 0, indicating successful program execution.
The program demonstrates the relationship between the array of pointers and the original variables.
Dereferencing Array Of Pointer In C
As mentioned before, an array of pointers in C is a collection of memory addresses/ numerous pointer variables stored in contiguous memory locations. Every pointer variable in the array holds the address of variables of a certain data type.
We can access the addresses or pointer values using the address-of operator (&) and the data or variable value stored at these addresses using the indirection operator (*). This operator is also called the dereference operator, which is used to access and modify the value of the element whose address is stored in the pointer variable.
Code Example:
Output:
The value at index 0 of num is 10
The value at index 1 of num is 20
The value at index 2 of num is 30
The value at index 3 of num is 40
The value at index 4 of num is 50
Explanation:
In this sample C program, we show how to access the elements in the original array by using the pointers stored inside the array of pointers.
- We begin by declaring and initializing an integer array num with five elements: {10, 20, 30, 40, 50}.
- Then, we declare an array of integer pointers ptr and use a for loop to initialize its elements. The loop begins with the control variable x set to index 0 and continues until x<5 is true.
- It uses the address-of operator (&) to assign the address of the xth element of the num array to the xth element of the array of pointers.
- We then use another for loop to iterate through each element of the array ptr and print the values inside the num array based on the index position.
- The printf() statement inside this loop prints the value at each index of the array num by dereferencing the pointer using the deference operator (*).
- The output of the program displays the index and value of each element in the array num, as accessed through the array of pointers ptr.
- Finally, the main function terminates with a return 0 statement.
Relationship Between Pointers And Arrays In C
In C, pointers and arrays are closely related, and understanding their relationship is crucial for effective programming. Here are key aspects of their relationship:
- Arrays as Pointers: The name of an array in C is essentially a pointer to the first element of the array. For example, in the array- int numbers[5], the identifier numbers points to the first element, making it a pointer. The expression, i.e., &numbers[0], is equivalent to numbers.
- Pointer Arithmetic: Pointers can be incremented and decremented, and this behavior extends to array names. For example, if ptr is a pointer, ptr++ and ptr-- will move the pointer to the next and previous memory locations, respectively. Similarly, for an array arr, arr++ and arr-- behave the same way.
- Array Access using Pointers: Elements of an array can be accessed using pointers and pointer arithmetic. For example, arr[i] is equivalent to *(arr + i), i.e., both expressions access the ith element of the array.
- Pointer Initialization with Arrays: Pointers can be initialized with the address of the first element of an array. For example, int numbers[5] = {1, 2, 3, 4, 5};
int *ptr = numbers; // ptr now points to the first element of the array - Dynamic Memory Allocation: Pointers are commonly used when dynamically allocating memory for arrays using functions like malloc() and calloc(). For example, int *dynamicArray = (int*)malloc(sizeof(int) * 10);
Take a look at the C code example below to understand this relationship between arrays and pointers.
Code Example:
Output:
arr : 63744176, Value : 12
&arr : 63744176, Value : 12
&arr[0] : 63744176, Value : 12
Explanation:
The program aims to demonstrate different ways of accessing the first element of the array and printing its value and memory address. In this C code-
- We begin by declaring and initializing an integer array arr with five elements, i.e., {12, 24, 36, 48, 60} inside the main() function.
- We then use three printf() statements to display the information of the first element of the array. In these statements-
- The first statement prints the memory address of the array arr and the value stored at this address (*arr). It shows how to access the array directly without using the address-of operator (&).
- The second statement prints the memory address of the array arr using the address-of operator (&arr) and the value stored at this address (*(arr)). It demonstrates taking the address of the array itself.
- The third statement prints the memory address of the first element of the array arr (&arr[0]) and the value stored at this address (*(&arr[0])). It shows how we can get the address of the first element using the address-of operator applied to arr[0].
- The program displays the memory addresses and values of the first element of the array arr, showcasing different methods of accessing and dereferencing pointers in C.
Applications Of Arrays Of Pointers In C With Examples
In C programming, arrays of pointers can be a powerful and flexible way to manage data, especially when dealing with dynamic memory allocation and structures. Here are some common applications and scenarios where arrays of pointers are useful:
1. Dynamic Memory Allocation: Arrays of pointers in C are often used when dynamically allocating memory for arrays of different sizes. Instead of having a fixed-size array, you can use an array of pointers, and each pointer points to a dynamically allocated memory block. This allows for more flexibility in managing memory resources.
int* dynamicArray[5]; // Array of pointers to int
for (int i = 0; i < 5; ++i) {
dynamicArray[i] = (int*)malloc(sizeof(int) * (i + 1));
}
// Use dynamicArray like a 2D array
2. Array of Strings: An array of pointers in C can also be used to store an array of strings. Here, each element of the array is a pointer will be pointing to a character array (individual string).
char* words[] = {"apple", "banana", "orange", "grape"};
for (int i = 0; i < 4; ++i) {
printf("%s\n", words[i]);}
3. Array of Structures: Arrays of pointers are useful when dealing with arrays of structures. Each element of the array can be a pointer to a structure, allowing for more complex data organization.
struct Point{
int x;
int y;
};
struct Point* pointArray[5]; // Array of pointers to Point structures
for (int i = 0; i < 5; ++i) {
pointArray[i] = (struct Point*)malloc(sizeof(struct Point));
pointArray[i]->x = i;
pointArray[i]->y = i * 2;}
// Access and manipulate data through pointArray
4. Function Pointers: Arrays of function pointers are useful for implementing things like function tables, callbacks, or state machines.
void add(int a, int b) {
printf("Sum: %d\n", a + b);}void subtract(int a, int b) {
printf("Difference: %d\n", a - b);}void multiply(int a, int b) {
printf("Product: %d\n", a * b);}void (*operation[])(int, int) = {add, subtract, multiply};
// Use function pointers to call different operations
operation[0](5, 3); // Calls add
operation[1](5, 3); // Calls subtract
operation[2](5, 3); // Calls multiply
Pointer To Arrays In C
We already know what is an array of pointers in C programming; now let's see what a pointer to an array means. Well, it is simply a pointer which is pointing to an array. So, we just have to store the base address of the array in the pointer variable, and the rest all the elements can be accessed easily.
Suppose we initialize a pointer ptr, which contains the base address of the array, i.e., the first element of the array. Then, we can access all the elements of the array by using the (*) dereferencing operator. That is, we can use the pointer expression *(ptr+i) to access the ith element of the array. So, ptr = &arr[0], where arr is the array, then ptr is the pointer to the first element of the array.
Syntax:
int *ptr = &arr;
int *ptr = arr;
int *ptr = &arr[0];
Here,
- We have taken an array of integers so the data type of the pointer will be int.
- * is used to denote that it is a pointer variable
- ptr is the name of the pointer variable.
- Name of the array itself points to the base address of the array so we can store the base address of the array by using arr,&arr and also &arr[0].
Example:
Output:
30 5 71 99 11
Explanation:
The primary goal of the program is to print the values of each element in the array using pointer arithmetic. In this C code-
- We declare and initialize an integer array arr of size 5, with the elements {30, 5, 71, 99, 11}.
- Then, we declare a pointer variable ptr and initialize it to point to the first element of the array arr.
- Next, we use a for loop to iterate through each element of the array and print the value of the array elements.
- The printf() statement inside the loop prints the value of the array element currently pointed to by the pointer (*(ptr + i)). Here, pointer arithmetic is applied to access the values of successive array elements as the loop iterates.
- The loop runs for five iterations, printing each array element in sequence.
- Finally, the main function returns 0.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Pointer To Multi-Dimensional Arrays In C
A multidimensional array in C is an array with more than one dimension. The most common type of multidimensional array is the two-dimensional array, although C supports arrays with more than two dimensions as well.
For example, a 3x4 integer array can be declared as:
int matrix[3][4];
This creates a matrix with 3 rows and 4 columns. The elements of the array can be accessed using indices, such as matrix[1][2], to access the element in the second row and third column.
This concept is particularly useful when dealing with functions that require passing multidimensional arrays as arguments or when dynamically allocating memory for such arrays. It allows for more efficient manipulation and traversal of the array elements by leveraging the principles of how arrays are stored in memory.
Pointer To 2D Arrays
A 2-D array is an array of arrays made up of n 1-D arrays stored in a linear manner in the memory. 2-D arrays can also be represented as a matrix in the form of rows and columns. In C, a pointer to a 2D array is a pointer that points to the base address of a 2D array. It allows us to access and manipulate the elements of the array using pointer arithmetic.
Syntax:
*(*(arr + i) + j)
It represents the element present at the ith row and jth column, respectively, which is equivalent to arr[i][j]. For example, we can declare a pointer to a 2D array of integers with the syntax- int (*ptr)[N], where N represents the number of columns in each row. This pointer can be incremented or decremented to navigate through the rows of the array, and array indexing can be used to access individual elements within each row.
Pointer to 3D Arrays
A 3-D array is an array of 2-D arrays or an array of arrays of arrays. They are made up of n 2-D arrays stored linearly in the memory. A pointer to a 3D array in C is a pointer that points to the base address of a 3D array. It allows for accessing and manipulating the elements of the array in three dimensions.
For example, int (*ptr)[M][N]; declares a pointer to a 3D array of integers, where M and N represent the number of rows and columns in each two-dimensional slice, respectively. This pointer can be used to navigate through the different slices of the array, and array indexing can be used to access individual elements within each slice.
Syntax:
*(*(*(arr + i) + j) + k)
It represents the element of an array arr at the index value of ith row and jth column of the kth array in the array arr; it is equivalent to the regular representation of 3-D array elements as arr[i][j][k].
Array Of Pointers To String (Characters) In C
We can also make an array of pointers to strings where each pointer in the array points to an element of character data type. That is, each pointer in the array points to the first character of the string. Generally, we use this when we want to store multiple strings of different lengths.
Syntax:
char *array_name [array_size];
Here,
- The term char indicates that the pointers inside the array are pointing to character values.
- The indirection operator (*) indicates that it is an array of pointers.
- The terms array_name and array_size refer to the name given to the array of pointers and the number of elements/ pointers inside it, respectively.
Note that this syntax only declares the array of pointers to strings in C. That is, it doesn't allocate memory for the actual strings (sequences of characters) but only for the pointers. You will need to allocate memory for each string individually and make each element of array_name point to the beginning of each string.
Code Example:
Output:
Cat
Dog
Rabbit
Explanation:
In the sample C code-
- We declare an array of pointers to character (char* arr[3]) and initialize it with three string literals, i.e., Cat, Dog, and Rabbit. Each element of this array (arr) stores the memory address of the respective string literal.
- Then, we use a for loop to iterate through each element of the array, starting from index 0 and ending at index 2.
- Within each iteration, a printf() statement displays the string value at the current array index using the %s format specifier.
- The loop ensures that each string in the array is printed to the console. The loop variable i is incremented in each iteration, allowing us to access each element of the array sequentially.
- Finally, the main function returns 0, indicating successful program execution.
In summary, this code demonstrates the usage of an array of pointers to strings, providing a convenient way to manage and print a collection of strings in a C program.
Array Of Pointers To Different Types
An array of pointers in C to different data types is a construct that allows us to store memory addresses of variables with varying data types, such as int, char, float, double, etc., within a single array. This flexibility is achieved by declaring an array of void pointers (void *), enabling the storage of addresses of values of different data types. Each element in the array is then assigned the address of a variable of a specific type.
Accessing the values involves careful type casting based on the expected data type, allowing for dynamic handling of heterogeneous data sets. This concept is particularly useful in scenarios where the data types may change dynamically or are not known at compile time, providing a versatile approach to managing diverse data structures within a single array.
Code Example:
Output:
Integer Value: 42
Float Value: 3.14
Char Value: A
Double Value: 2.718280
String Value: Hello, World!
Explanation:
In the given C code-
- We declare an array of void pointers ptrArray of size 5, to store the addresses of variables of different data types.
- Then, we declare and initialize 5 variables of different data types, i.e., intValue (int), floatValue (float), charValue (char), doubleValue (double type), and stringValue[] (string type).
- After that, we initialize the pointers in ptrArray with the addresses of these variables. We assign the address of the corresponding variable to each pointer in the array using the address-of operator (&) and index values.
- Note that we do not use the address-of operator when initializing ptrArray[4]. This is because the string itself is an array, in which case the pointer will automatically give the address of the first character of the string.
- Next, we use five printf() statements to display the values stored in the respective addresses.
- Inside each printf() statement, we first type cast the pointer to the appropriate data type. For example, (int *)ptrArray[0] casts the void pointer at position 0 to an integer pointer.
- Then, we deference the pointer to retrieve/ access the value stored at that address. For example, *(int *)ptrArray[0] accesses the integer value stored at the address pointed to by ptrArray[0].
- The printf() function then displays this value to the console. The same is done for all other pointers and values, and appropriate format specifiers are used as placeholders for the values.
- The program demonstrates how to print values of different data types stored in an array of pointers in C. It also provides an implementation of type casting and void pointers.
- Finally, the main function returns 0, indicating successful program execution.
Advantages And Disadvantages Of Array Of Pointers In C
Arrays of pointers in C offer numerous advantages and disadvantages, depending on the specific use case. In this section, we will look at a few key benefits and disadvantages of an array of pointers in C programming.
Advantages Of Array Of Pointers In C
- Dynamic Memory Allocation: Arrays of pointers in C allow for dynamic memory allocation, thus enabling flexible management of memory resources. Each element of the array can point to dynamically allocated memory, accommodating varying data sizes.
- Variable Size Arrays: Unlike traditional arrays, arrays of pointers in C can hold elements of different sizes. This is particularly useful when dealing with data structures like strings or arrays of structures with varying sizes.
- Memory Efficiency: Pointers facilitate efficient memory usage since only the necessary memory is allocated, and there's no need to reserve space for the maximum possible size of the data.
- Ease of Manipulation: Pointer arithmetic provides a convenient way to traverse and manipulate the elements of the array. This is especially valuable when dealing with complex data structures or multidimensional arrays.
- Function Pointers: Arrays of function pointers allow for the creation of function tables or implementing features like callbacks. This is powerful in scenarios where different operations need to be performed based on runtime conditions.
Disadvantages Of Array Of Pointers In C
- Increased Complexity: Arrays of pointers in C can introduce additional complexity, especially for programmers who are not familiar with pointer manipulation. Debugging and understanding the code may be more challenging.
- Memory Management Responsibility: Dynamic memory allocation using pointers requires careful memory management. Developers need to explicitly allocate and deallocate memory to prevent memory leaks or undefined behavior, which can be error-prone.
- Potential for Dangling Pointers: Incorrect handling of pointers can lead to dangling pointers, pointing to memory locations that have been freed. Accessing such pointers results in undefined behavior, and debugging such issues can be difficult.
- Overhead: There is some overhead associated with managing an array of pointers. Each pointer requires additional memory, and accessing the actual data involves an extra level of indirection.
- Potential for Memory Fragmentation: Frequent dynamic memory allocation and deallocation may lead to memory fragmentation, impacting the overall performance of the program.
Conclusion
In conclusion, the array of pointers in C is a powerful construct that combines the flexibility of pointers with the systematic organization of arrays. It empowers C programmers to manage memory consumption, create dynamic data structures efficiently, and implement advanced algorithms. Understanding how to declare, initialize, and use an array of pointers in C opens up new possibilities for writing more efficient and flexible C programs. As you delve deeper into C programming, mastering the array of pointers will undoubtedly enhance your ability to tackle complex problems and optimize your code.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. Is an array of pointers in C the same as a pointer to an array?
No, a pointer to an array differs from an array of pointers in C. In the former structure, we store the base address of the array in the pointer variable, and the rest of the elements can be accessed easily using pointer arithmetic.
In contrast, the latter structure is a collection of pointers present at contiguous locations. Every pointer has the memory location of a similar data type(int, char, double, etc.), which can be accessed by dereferencing that particular pointer. In contrast,
Example Code:
Output:
Value of var1: 10
Value of var2: 20
Value of var3: 30
Value at index 0 is 1
Value at index 1 is 2
Value at index 2 is 3
Explanation:
- Here, parr is an array of pointers pointing to the addresses of v1,v2, and v3, respectively.
- Whereas ptr is a pointer to the base address of array arr and we access the elements of the array arr by using pointer arithmetic and dereferencing operators.
Q. How do you assign a pointer to an array in C?
In the case of a pointer to an array in C, say ptr, we just have to store the base address of the array in the pointer variable, and the rest of the elements can be accessed easily. The address of the first element of the array can be accessed by the name of the array arr or &arr or &arr[0]. By using the dereferencing operator (*), we can access the elements of the array, i.e., by accessing the ith index element of the array, we will use *(arr+i).
Syntax:
*ptr = &arr;
*ptr = arr;
*ptr = &arr[0];
Example:
Output:
130 5 271 990 11
Explanation:
In this example, we have declared and initialized an integer array arr and then initialized a pointer ptr with it. We then initiate a for loop, where (ptr + i) will give the address of the array elements as the value of i changes from 0-4 as address(ptr + i) = address(arr[i]). We also use the referencing operator (*) to print the value at the provided address. That is, the expression *(ptr + i) will print the values of the array as the value of i changes using the printf() function.
Q. What is a pointer to a pointer?
A situation in which a pointer variable points to another pointer variable is known as a pointer to a pointer. A pointer points to the address of another variable, which we can access using a the dereferencing operator (*). A pointer to a pointer is a variable that stores the address of the pointer variable, which in turn points to another address of a variable. We dereference the pointer to a pointer 2 times to access the value of the variable stored by the first pointer.
Example:
int *p1,**p2,v=10;
p1=&v; p2=&p1;
Here, p2 is a pointer to a pointer p1, which holds the address of variable v.
Q. Write a C program to sort an array using pointers.
The array elements can be fetched with the help of pointers, with the pointer variable pointing to the base address of the array. Hence, to sort the array using pointers, we need to access the elements of the array using the (*) dereferencing operator, i.e., *(ptr+i) for the ith element of the array.
Code Example:
Output:
0 9 12 14 23
Explanation:
Here, we have an array of size 5, which we sort using the sort() function. The function takes the array and its size as parameters.
- We compare each element with the other and compare them using pointers to access them and swap the smaller one to the beginning of the array.
- At last, we print all the elements in a sorted order using the printf() function by accessing the elements by using the (*) dereferencing operator as *(ptr+i) for the ith element.
Q. What does the error ‘Null Pointer Assignment’ mean, and what causes this error?
A null pointer assignment error occurs when we try to dereference a null pointer. A null pointer is a pointer that does not point to any memory location. This error can be caused by any one of the 2, i.e., using an uninitialized pointer or using an uninitialized variable. So, to avoid this error, we must always initialize a pointer to a valid memory location first before using it.
Q. What is the advantage of an array of pointers?
There are many advantages of an array of pointers in Computer Science.
- It is most commonly used to store multiple strings.
- It is used in the chaining technique of collision resolving in hashing.
- It is used in sorting algorithms like bucket sort.
- It can be used with any pointer type, which is useful when we have separate declarations of multiple entities and we want to store them in a single place.
Q. Can we sort an array of strings?
Yes, we can sort an array of strings using the strcmp() function in the C programming language. Let us look at an example to see the implementation of the same.
Example:
Output:
Bumrah Chahal Hardik Jadeja Kuldeep Shami
Explanation:
Here,
- We have declared an array of strings and initialized n as the size of the array.
- We compare each string with the other using the strcmp() function in C and swap the smaller one to the beginning of the array.
- We use an if-statement with a temporary variable inside the nested for loop to get this done.
- At last, we print all the strings in a sorted order using the printf() function.
This brings us to the end of our discussion on the array of pointers in C. Here are a few other topics you must read:
- Ternary (Conditional) Operator In C Explained With Code Examples
- 6 Relational Operators In C & Precedence Explained (+Examples)
- Keywords In C Language | Properties, Usage, Examples & Detailed Explanation!
- Control Statements In C | The Beginner's Guide (With Examples)
- Constant In C | How To Define & Its Types Explained With Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment