Table of content:
- What Are Roots Of A Quadratic Equation?
- How To Find Roots Of Quadratic Equations?
- Steps To Write A C Program Find Roots Of Quadratic Equation
- Pseudo Code Of C Program To Find The Roots Of Quadratic Equations
- C Program To Find Roots Of Quadratic Equation Using If-Else Statement
- C Program To Find Roots Of Quadratic Equation Using Functions
- Conclusion
- Frequently Asked Questions
C Program To Find Roots Of Quadratic Equation With Detailed Examples
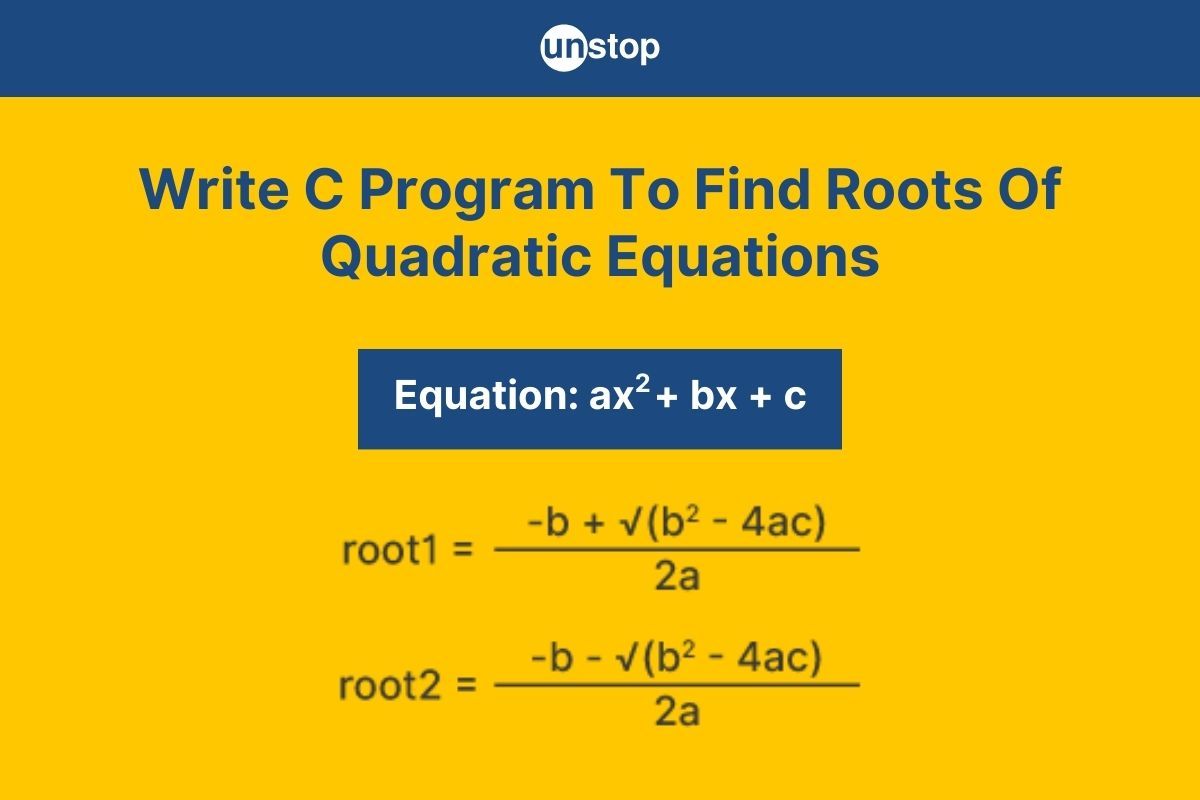
In mathematics, quadratic equations are polynomial expressions where the highest power of the variable is 2. The highest power in an equation is also called the degree of a quadratic equation. So, all equations with a degree of 2 are quadratic equations, written as:
ax^2 + bx + c,
where a ≠ 0 and a, b, and c are constants.
Most of us know how to solve quadratic equations mathematically, but can you get a computer to do this for you? Well, don’t worry. In this article, we will explore how to write and run a C program to find the roots of a quadratic equation.
What Are Roots Of A Quadratic Equation?
Before we begin, let’s understand what the roots of an equation are. In simple terms, any value which satisfies the equation is termed the root of the equation. A quadratic equation is a second-degree polynomial equation and has the general form:
ax2+bx+c=0
Here, x represents the variable, and a, b, and c are constants with a not equal to zero. The solutions for x are called the roots of the quadratic equation.
To find the roots, we need to solve the equation. There are various methods of solving a quadratic equation, like the quadratic formula or the factorization method. We will discuss the steps involved in solving the equation in the later part of this article. We will also discuss the process of solving a quadratic equation using the C programming language, but first, let’s discuss the nature of roots.
Nature Of Roots
It is important to understand the nature of roots, as it helps us ascertain how the solution is obtained and know a lot more about the equation we have. To figure out the nature of the roots, one has to look at the values of the coefficients in the equation. In mathematics, a term called discriminant (represented by D) is calculated on the basis of an expression containing the coefficients from the equation. and we can determine the nature of the roots, i.e., real or imaginary, based on the value of the discriminant.
For equation ax^2+bx+c, the discriminant D will be given by the expression b^2-4ac. Based on the value of D, i.e., the discriminant, we can have the following types of roots:
- For a positive discriminant, D>0: The quadratic equation has two distinct and real roots.
- For a positive discriminant, D=0: The quadratic equation has real and equal roots (one real and repeated root).
- For a positive discriminant, D<0: The quadratic equation has no real root. Instead, it has complex roots, which occur in pairs.
How To Find Roots Of Quadratic Equations?
We already mentioned at the beginning of the article that quadratic equations could be solved using two methods, namely, the quadratic formula and the factorization method. Let’s discuss both of them in detail and know the step-by-step process.
Factorization
Factorization, as the name suggests, is the process of splitting the coefficients of the variable into factors and then calculating the roots. The process is as follows:
- We begin with an equation in the form of ax^2+bx+c=0. For example, x^2 - 8x + 15 = 0.
- Then, we factorize the equation into two binomial expressions. In the example above, this will be (x-3)(x-5)=0.
- We then equate the polynomials to zero. That is, x-3=0 and x-5=0.
- After equating it to zero, both the binomial expressions are individually solved for x. This is how we can obtain the roots. Which will be x=3, 5, in the example.
However, this method has its limitations, i.e., it can work only when the equation is factorable. For cases when it is not, we can use the quadratic formula, which is applicable in almost all cases.
Quadratic Formula Method
In this method, we use a general formula that uses the values of coefficients in the equation to find the solution of the quadratic equation. Say we have an equation, i.e., ax^2 + bx + c = 0. Then, the quadratic formula for the roots will be as follows:
x = (-b ± √(b^2 - 4ac)) / (2a)
Here,
- First, we have to identify the values of coefficients (a, b, c) so that we can put these values in the formula above and start solving. Note a ≠ 0.
- Simplifying the equation and calculating value within the root.
- Apply the plus-minus symbol and find both possible roots.
- The resulting values of x are roots of quadratic equations.
Note: Make sure the determinant (D) is not negative to make sure we obtain real roots.
Steps To Write A C Program Find Roots Of Quadratic Equation
We now know everything about quadratic equations, their roots, and ways to solve them mathematically. In this section, we will discuss how the basic C program to find roots of quadratic equations works and how you, too, can write one. Listed below is a step-by-step explanation of the same.
Steps to write a C program to find the roots of a quadratic equation:
- Include header files, <stdio.h> and <math.h>, this will help us to import the sqrt function from the math library in C.
- Declare variables a, b, and c, which will be the values of the coefficients in the equation.
- If the program takes the input from the user, prompt the user to enter values of a, b, c, or the coefficients in the quadratic equation. Otherwise, provide values in advance by initializing those variables.
- Calculate the discriminant D using the formula- b^2-4ac.
- Check the value of D and identify the nature of roots.
- Once the nature of the root is identified, provide values of roots according to the nature using the if-else blocks.
- Compile and run the program, input values of coefficients, and the program will display nature along with the value of the roots.
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
The flowchart given below shows how the flow of execution moves through a C program to find the roots of a quadratic equation.
Pseudo Code Of C Program To Find The Roots Of Quadratic Equations
Here's the pseudo-code (an outline of the logic and flow of the program without proper syntax) to solve the quadratic equation with the help of if-else blocks in C language.
- Include the math.h header file.
- Declare variables a, b, c, root1, root2, discriminant.
- Prompt the user to enter coefficients a, b, and c. And read the values of a, b, and c from the user.
- Calculate the discriminant using the formula b^2 - 4ac.
- If the discriminant is greater than 0, then-
- Calculate root1 and root2 using the quadratic formula.
- Print string message- Roots are real and distinct, and the values of root1 and root2.
- Else-if the discriminant is equal to 0, then-
- Calculate the root using the quadratic formula.
- Print the message- Roots are real and same, and the value of the root.
- Else (the discriminant is less than 0), do the following:
- Calculate the realPart and imaginaryPart using the quadratic formula.
- Print the message- Roots are complex and different, and the values of the complex roots.
- Program ends.
Now, let's take a look at an example that showcases the implementation of the method discussed above.
Example:
Suppose the values of a,b, and c, as entered by the user, are 1, -5, and 6, respectively. Then the equation at hand is- x^2 - 5x + 6. Now, to solve the equation, we will follow the steps given below-
Step1: Calculate the value of determinant (D)
D = b^2 - 4ac
D = (-5)^2 - 4*1*6
D = 1(Therefore D>0)
Step 2: Since D>0, the roots are real and distinct. Then-
root1 = (-b + sqrt(discriminant)) / (2 * a)
root1 = {-(-5) + sqrt(1)} / (2*1)
root1 = (5 + 1) / 2
root1 = 3
root2 = (-b - sqrt(discriminant)) / (2 * a)
root2 = {-(-5) - sqrt(1)} / (2*1)
root2 = (5 -1) / 2
root2 = 2
Step 3: The values of roots are 3 and 2
As is evident, we use the steps given in the pseudocode to calculate the roots which here are 3 and 2.
C Program To Find Roots Of Quadratic Equation Using If-Else Statement
An if-else statement is a control statement that helps execute different blocks of code depending on specific conditions being met. If the main statement condition is true, then the if block is executed, and if false, then the else-block is executed. Let's take a look at a sample C program to find the roots of quadratic equations using if-else.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxtYXRoLmg+CgppbnQgbWFpbigpIHsKZG91YmxlIGEsIGIsIGMsIHJvb3QxLCByb290MiwgZGlzY3JpbWluYW50OwoKcHJpbnRmKCJFbnRlciBjb2VmZmljaWVudHMgYSwgYiwgYW5kIGM6ICIpOwpzY2FuZigiJWxmICVsZiAlbGYiLCAmYSwgJmIsICZjKTsKCi8vQ2FsY3VsYXRpbmcgdGhlIGRpc2NyaW1pbmFudCB1c2luZyB0aGUgZm9ybXVsYQpkaXNjcmltaW5hbnQgPSBiICogYiAtIDQgKiBhICogYzsKCmlmIChkaXNjcmltaW5hbnQgPiAwKSB7CnJvb3QxID0gKC1iICsgc3FydChkaXNjcmltaW5hbnQpKSAvICgyICogYSk7CnJvb3QyID0gKC1iIC0gc3FydChkaXNjcmltaW5hbnQpKSAvICgyICogYSk7CnByaW50ZigiUm9vdHMgYXJlIHJlYWwgYW5kIGRpc3RpbmN0LlxuIik7CnByaW50ZigiUm9vdCAxID0gJS4ybGZcbiIsIHJvb3QxKTsKcHJpbnRmKCJSb290IDIgPSAlLjJsZlxuIiwgcm9vdDIpOwoKfSBlbHNlIGlmIChkaXNjcmltaW5hbnQgPT0gMCkgewpyb290MSA9IC1iIC8gKDIgKiBhKTsKcHJpbnRmKCJSb290cyBhcmUgcmVhbCBhbmQgc2FtZS5cbiIpOwpwcmludGYoIlJvb3QgMSA9IFJvb3QgMiA9ICUuMmxmXG4iLCByb290MSk7Cgp9IGVsc2Ugewpkb3VibGUgcmVhbFBhcnQgPSAtYiAvICgyICogYSk7CmRvdWJsZSBpbWFnaW5hcnlQYXJ0ID0gc3FydCgtZGlzY3JpbWluYW50KSAvICgyICogYSk7CnByaW50ZigiUm9vdHMgYXJlIGNvbXBsZXggYW5kIGRpZmZlcmVudC5cbiIpOwpwcmludGYoIlJvb3QgMSA9ICUuMmxmICsgJS4ybGZpXG4iLCByZWFsUGFydCwgaW1hZ2luYXJ5UGFydCk7CnByaW50ZigiUm9vdCAyID0gJS4ybGYgLSAlLjJsZmlcbiIsIHJlYWxQYXJ0LCBpbWFnaW5hcnlQYXJ0KTt9CgpyZXR1cm4gMDsKfQ==
Output:
Enter coefficients a, b, and c: 3 5 7
Roots are complex and different.
Root 1 = -0.83 + 1.28i
Root 2 = -0.83 - 1.28i
Explanation:
- We begin the C program example by including the header files for input and output operations, i.e., <stdio.h>, and mathematical functions, i.e., <math.h>.
- Then, we begin the main() function, which is the entry point of the program.
- We then declare six variables, i.e., a, b, c, root1, root2, and discriminant of double data type, to store the coefficients and roots of the quadratic equation.
- Next, we use the printf() function to prompt the user to input values for coefficients a, b, and c.
- We use the scanf() function to read these values and store them in respective coefficient variables using the address-of operator (&) and the %lf format specifier.
- After that, as mentioned in the code comments, we calculate the discriminant using the quadratic formula b²-4ac (i.e., b*b - 4*a*c).
- Next, we create an if-else-if-else structure to determine the nature of the roots based on the value of the discriminant.
- If the discriminant is greater than 0, it implies two real and distinct roots. We calculate the roots with the respective formula and print their values to the console.
- If the discriminant is equal to 0, it implies two real and identical roots. So, we calculate the root and print it.
- If the discriminant is less than 0, it implies complex roots. We thus calculate the real and imaginary parts and print the complex roots.
- Finally, the program returns 0 to indicate successful execution indicate no errors in program execution.
Time Complexity: The time complexity of the provided program is constant, denoted as O(1).
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
C Program To Find Roots Of Quadratic Equation Using Functions
There is one more way to implement the same functionality where we do not make use of if-else blocks. Instead, we create a function to find the roots of the equation. Below is an example C program to find roots of quadratic equations using a function.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxtYXRoLmg+Cgp2b2lkIGZpbmRSb290cyhkb3VibGUgYSwgZG91YmxlIGIsIGRvdWJsZSBjKSB7CmRvdWJsZSBkaXNjcmltaW5hbnQsIHJvb3QxLCByb290MjsKZGlzY3JpbWluYW50ID0gYiAqIGIgLSA0ICogYSAqIGM7CgppZiAoZGlzY3JpbWluYW50ID4gMCkgewpyb290MSA9ICgtYiArIHNxcnQoZGlzY3JpbWluYW50KSkgLyAoMiAqIGEpOwpyb290MiA9ICgtYiAtIHNxcnQoZGlzY3JpbWluYW50KSkgLyAoMiAqIGEpOwpwcmludGYoIlJvb3RzIGFyZSByZWFsIGFuZCBkaXN0aW5jdC5cbiIpOwpwcmludGYoIlJvb3QgMSA9ICUuMmxmXG4iLCByb290MSk7CnByaW50ZigiUm9vdCAyID0gJS4ybGZcbiIsIHJvb3QyKTsKCn0gZWxzZSBpZiAoZGlzY3JpbWluYW50ID09IDApIHsKcm9vdDEgPSAtYiAvICgyICogYSk7CnByaW50ZigiUm9vdHMgYXJlIHJlYWwgYW5kIHNhbWUuXG4iKTsKcHJpbnRmKCJSb290IDEgPSBSb290IDIgPSAlLjJsZlxuIiwgcm9vdDEpOwoKfSBlbHNlIHsKZG91YmxlIHJlYWxQYXJ0ID0gLWIgLyAoMiAqIGEpOwpkb3VibGUgaW1hZ2luYXJ5UGFydCA9IHNxcnQoLWRpc2NyaW1pbmFudCkgLyAoMiAqIGEpOwpwcmludGYoIlJvb3RzIGFyZSBjb21wbGV4IGFuZCBkaWZmZXJlbnQuXG4iKTsKcHJpbnRmKCJSb290IDEgPSAlLjJsZiArICUuMmxmaVxuIiwgcmVhbFBhcnQsIGltYWdpbmFyeVBhcnQpOwpwcmludGYoIlJvb3QgMiA9ICUuMmxmIC0gJS4ybGZpXG4iLCByZWFsUGFydCwgaW1hZ2luYXJ5UGFydCk7fQp9CgppbnQgbWFpbigpIHsKZG91YmxlIGEsIGIsIGM7CgpwcmludGYoIkVudGVyIGNvZWZmaWNpZW50cyBhLCBiLCBhbmQgYzogIik7CnNjYW5mKCIlbGYgJWxmICVsZiIsICZhLCAmYiwgJmMpOwoKZmluZFJvb3RzKGEsIGIsIGMpOwoKcmV0dXJuIDA7Cn0=
Output:
Enter coefficients a, b, and c: 1 -4 4
Roots are real and equal
Root 1 = Root 2 = 2.00
Explanation:
We begin the C code example by including the necessary header files. Then-
- We define a findRoots function to calculate and print the roots of a quadratic equation, which takes the coefficients a, b, and c of double type as arguments.
- Inside the function, we first declare double variables (discriminant, root1, and root2) and then calculate the discriminant using the quadratic formula- b²-4ac.
- Next, the function employs an if-else-if-else statement to calculate the roots and print their values based on the value of the discriminant.
- If the discriminant is greater than 0, then we have two real and distinct roots, which we calculate using the quadratic formula. Then, we use the printf() function with a formatted string to display a message to this effect, as well as the values of the roots (root1 and root2) with two decimal places.
- If the discriminant is equal to 0, we have two equal real roots, which we calculate as per the formula. The program then prints the message- Roots are real and same, with the value of the root to the console.
- If the discriminant is less than 0, then we have complex roots whose real and imaginary parts are calculated. Once again, we print a message and the values to the console.
- Note that we use the %lf format specifier, newline escape sequence, and arithmetic operators while making calculations and printing the values.
- Inside the main() function, we then declare three double variables (a, b, and c) to store the coefficients of the quadratic equation.
- Next, we prompt the user to input values for these coefficients and store them using printf() and scanf() functions, respectively.
- We then call the findRoots function by passing the provided coefficients as parameters.
- The program returns 0 to indicate successful execution.
Time Complexity: The time complexity of the provided program is constant, denoted as O(1).
Conclusion
In the article, we discussed what quadratic equations are, the discriminant of an equation, and how this discriminant determines the nature of roots, which can be imaginary or real. Further, we discussed the ways to solve these equations to find their roots, i.e., by using the quadratic formula and factorization in mathematics.
Then, we built an algorithm to show how to write a C program to find the roots of quadratic equations. The two primary methods we used here were the if-else statements and functions, along with the mathematical formulas. Understanding how to write a C program for different use cases and actual mathematical problems is critical if you want to improve your real-life programming skills.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. How do you find the number of roots in a quadratic equation?
The number of roots of a quadratic equation (say ax^2 + bx + c = 0) is determined by the discriminant (b^2 - 4ac). This happens as follows:
- If the discriminant is greater than zero, the equation has two distinct real roots. For example, if the equation is 2x^2 - 3x + 1 = 0, then the coefficients are 2, -3, and 1, and in this case, there are two distinct real roots.
- If the discriminant is equal to zero, the equation has two identical real roots. For example, if the equation is x^2 - 2x + 1 = 0, then the coefficients are 1, -2, and 1, and in this case, the roots are two identical real roots.
- If the discriminant is less than zero, the equation has no real roots (complex roots). For example, if the equation is 3x^2 - 2x + 1 = 0, then the coefficients are 3, -2, and 1, and in this case, roots are complex roots.
Q. How to find the roots of a quadratic equation by completing the square?
To find the roots of a quadratic function using the complete-square method, we need to follow these steps:
- Write the equation in the standard form, i.e., ax^2 + bx + c = 0, where a, b, and c are constants.
- Divide the equation by a to make the coefficient of x^2 as 1.
- Move the constant term (c/a) to the right side of the equation, i.e., x^2 + (b/a)x = -c/a.
- Take half of (b/a) and square it, i.e., (b/2a)^2.
- Add the squared term to both sides of the equation, i.e., x^2 + (b/a)x + (b/2a)^2 = -c/a + (b/2a)^2.
- Simplify the right side of the equation, i.e., x^2 + (b/a)x + (b/2a)^2 = (b^2 - 4ac) / (4a^2).
- Factor the left side of the equation, i.e., (x + b/2a)^2 = (b^2 - 4ac) / (4a^2).
- Take the square root of both sides, i.e., x + b/2a = ±√((b^2 - 4ac) / (4a^2)).
- Solve for x by using the formula, i.e., x = (-b ± √(b^2 - 4ac)) / (2a).
Let’s take an example with values to get a clearer picture of how this approach works. Say the equation is 2x^2 + 5x - 3 = 0. Then the solution will be as follows:
- x^2 + (5/2)x - 3/2 (Dividing by 2)
- x^2 + (5/2)x = 3/2 (Constant term to RHS)
- (5/4)^2 = 25/16. (Halved b/a and then squared)
- x^2 + (5/2)x + 25/16 = 3/2 + 25/16. (Added the term both sides)
- x^2 + (5/2)x + 25/16 = 49/16 (Simplifying RHS)
- (x + 5/4)^2 = 49/16 (Factoring LHS)
- x + 5/4 = ±√(49/16) (Taking square root on both sides)
- x = (-5/4 ± 7/4) (Solving for x)
- Roots : x = ½ and x = 3
Q. What is the fastest way to find the roots of a quadratic equation?
The fastest way to find the roots of a quadratic equation is by factoring or completing the square. The quadratic formula is derived from the complete-square method. Therefore, it is always best to give a shot using a complete square first, but if it turns complex, you can anytime use the quadratic formula to find roots directly.
Q. How to handle complex roots in a C program?
The <complex.h> header file in the library function provides us with the csqrt() function. It helps us handle the negative discriminant and return the imaginary and real parts of the root separately. The extraction of real and imaginary parts is also possible by using two other functions from the same header file, i.e., creal() and cimag().
- csqrt() - helps us to find the square root of a complex number.
- creal() - extracts the real part of a complex number.
- cimag() - extracts the imaginary part of a complex number
Let's take a look at an example to understand how these functions work in a C program to find the roots of quadratic equations.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxtYXRoLmg+CiNpbmNsdWRlIDxjb21wbGV4Lmg+CgppbnQgbWFpbigpIHsKZG91YmxlIGEsIGIsIGM7CmRvdWJsZSBkaXNjcmltaW5hbnQ7CmRvdWJsZSByb290MSwgcm9vdDI7CgpwcmludGYoIkVudGVyIGNvZWZmaWNpZW50cyBhLCBiLCBhbmQgYzogIik7CnNjYW5mKCIlbGYgJWxmICVsZiIsICZhLCAmYiwgJmMpOwpkaXNjcmltaW5hbnQgPSBiICogYiAtIDQgKiBhICogYzsKCmlmIChkaXNjcmltaW5hbnQgPj0gMCkgewpyb290MSA9ICgtYiArIGNzcXJ0KGRpc2NyaW1pbmFudCkpIC8gKDIgKiBhKTsKcm9vdDIgPSAoLWIgLSBjc3FydChkaXNjcmltaW5hbnQpKSAvICgyICogYSk7CnByaW50ZigiUm9vdCAxID0gJS4ybGYgKyAlLjJsZmlcbiIsIGNyZWFsKHJvb3QxKSwgY2ltYWcocm9vdDEpKTsKcHJpbnRmKCJSb290IDIgPSAlLjJsZiArICUuMmxmaVxuIiwgY3JlYWwocm9vdDIpLCBjaW1hZyhyb290MikpOwoKfSBlbHNlIHsKZG91YmxlIHJlYWxQYXJ0ID0gLWIgLyAoMiAqIGEpOwpkb3VibGUgaW1hZ2luYXJ5UGFydCA9IGNzcXJ0KC1kaXNjcmltaW5hbnQpIC8gKDIgKiBhKTsKcHJpbnRmKCJSb290IDEgPSAlLjJsZiArICUuMmxmaVxuIiwgcmVhbFBhcnQsIGltYWdpbmFyeVBhcnQpOwpwcmludGYoIlJvb3QgMiA9ICUuMmxmIC0gJS4ybGZpXG4iLCByZWFsUGFydCwgaW1hZ2luYXJ5UGFydCk7fQoKcmV0dXJuIDA7Cn0=
Output:
Enter coefficients a, b, and c: 5 2 3
Root 1 = -0.20 + 0.75i
Root 2 = -0.20 - 0.75i
Q. How can I handle cases where the coefficients are very large or very small floating-point values?
If coefficients are very large or very small floating point values, then it is advisable to use high precision data type. That is, instead of double, use long double for coefficient variables and roots as well. Additionally, you can adjust the formatting of the output using appropriate format specifiers in printf() to display the coefficients and roots with the desired precision and scientific notation if needed. Being knowledgeable in how to take note of such aspects will help you write the perfect C program to find the roots of quadratic equations and much more.
Here are some other amazing topics you must explore:
- Bitwise Operators In C Programming Explained With Code Examples
- Understanding Looping Statements In C [With Examples]
- Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
- 5 Types Of Literals In C & More Explained With Examples!
- Jump Statement In C | Types, Best Practices & More (+Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment