100+ Top C Interview Questions With Answers (2024)
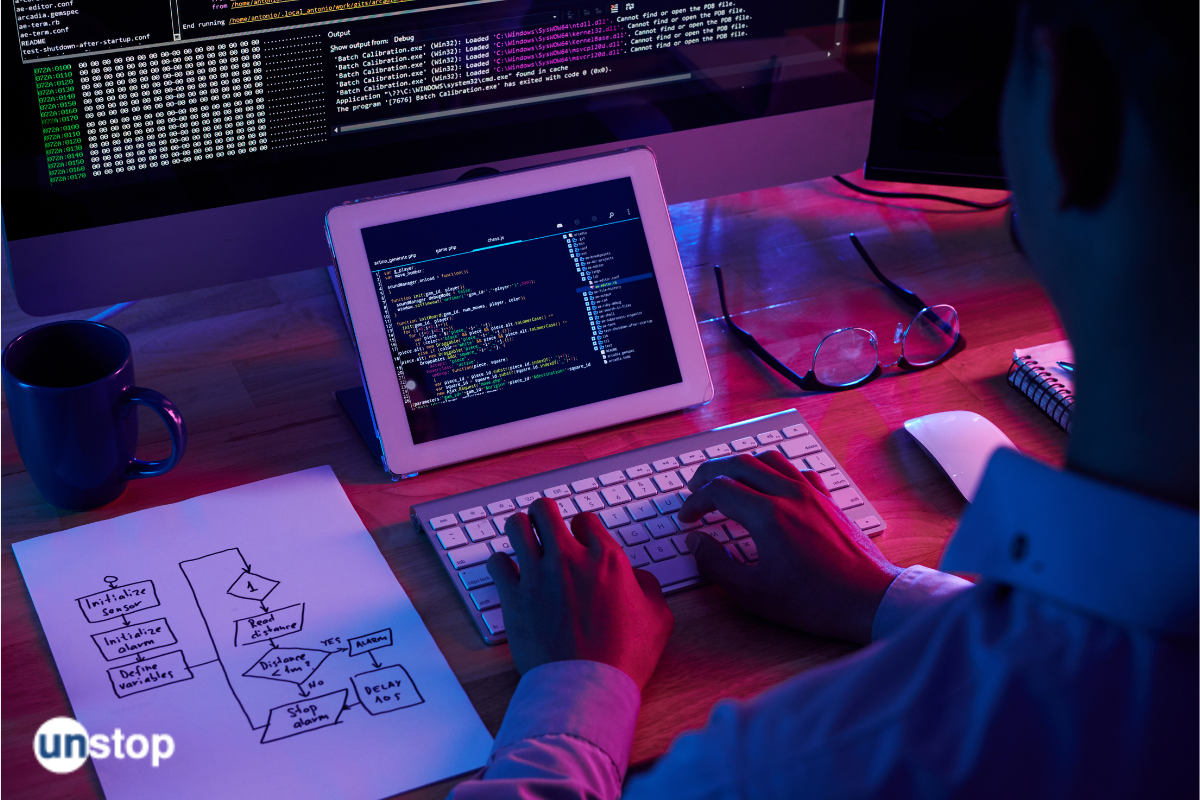
Whether you are preparing for an interview or just wanted to quickly brush up your C knowledge, you are at the right spot. We have made a list of curated C programming interview questions and answers that are very frequently asked in an interview. Let's get started.
Most important C interview questions and answers
Q1. What is the C language?
C is a procedural, general-purpose programming language that was created by C. Dennis Ritchie at Bell Labs in 1972. This machine-independent language is also called as mother of all programming languages.
Q2. Distinguish between the source code and object code in C.
Source Code: In a programming language, the set of instructions written by the programmer is source code. It is also known as a source program or human-readable code that humans can read and edit. Computers cannot directly execute it, and it needs to be compiled into object code (machine language) before execution. In C, source codes are saved with a ‘.c’ extension like a filename.c
Object Code: Object code refers to the output of compiling a computer program that consists of low-level machine language understandable only by machines/computers. In C, these files have an extension .o or .obj.
Q3. What is the main() function in C Language?
Every C program has one mandatory function that is named main(). The main() function controls the execution, and the function calls to other functions are made from the main() function.
Syntax: datatype main() { // code }
Q4. What is meant by the preprocessor directive in C?
In C language, before beginning actual compilation, the preprocessor directives instruct the compiler to preprocess the data. For this, the # sign comes before the preprocessor directives.
Example: #include<stdio.h>, the studio library will be included in the current program.
Q5. What is the full form for ANSI?
ANSI stands for American National Standards Institute. It is a private nonprofit organization that oversees the development of voluntary consensus standards for products, services, processes, systems, and personnel in the United States.
Q6. What is a macro in C?
A macro is a fragment of code in the program that replaces a macro value. Macro is defined by the #define directive. Whenever a macro name is found by a compiler, it replaces the name with the macro definition.
Example: #define Pi 3.14
Now, whenever the name Pi is used anywhere in the program, it is replaced by the value 3.14. There is no need to terminate the macro definition using semicolons.
Q7. What is necessary to construct a conditional statement within a sequential access file source file?
To construct a conditional statement within a sequential access file source file, you must provide the conditions that must be met for data to be accessed from the file. This involves using keywords or logical operators (such as "AND," "OR," and so on) in combination with expressions or values related to specific fields in the record.
For example, if your input record contains fields called "Name" and "Age," then a simple condition might look like this Name = 'John' AND Age > 18.
This represents an expression that finds all records with both names equal to John and the age exceeding 18. The exact syntax of these expressions may vary depending on your language, but you should generally follow this format.
Q8. In C, what is the volatile keyword?
The volatile keyword in C is a type qualifier used to indicate that a variable's value may be changed by something outside the control of the code, such as an external hardware device. It also prevents certain compiler optimizations from being applied to variables or pointers declared volatile; this ensures that instructions referring to them are not reordered and executed out-of-order.
This can be useful for preventing data races when writing multi-threaded programs since it informs the compiler that values should always be read directly from memory instead of stored in registers or caches.
Q9. What is the difference between the actual and formal parameters in C?
The values provided to a function when it is called are the actual arguments in the C programming language. Formal parameters refer to the names given in the definition of a function. They act as placeholders for information provided by whoever calls the function, which can then be referenced within its body using those formal parameter names. The way they bind together is known as parameter binding.
When you call a function with arguments (actual parameters), each argument value will replace or 'binds' into its respective formal parameter from left-to-right order until actual arguments have filled all variable positions.
For example, if your functions take three inputs like this:
int sum(int x1, int y1, int z1); Then any time you call "sum()" and pass three integers afterward – say 10 20 30 - these numbers would correspond one-by-one to x1=10, y2 =20 and z3 =30.
Q10. What is printf() function?
The printf() function, part of the C Standard Library, prints formatted output to the standard output device (often a screen). It can accept different parameters depending on the format string (specifier) provided. Format strings are composed of text and formatting characters with placeholders for data values that will be printed; these include %d for integers, %f for floating-point numbers, or %s for strings. When using print (), a format string and a list of values must be supplied as arguments. The function then parses this information and prints it out following the formatting characters specified within the string, along with the placeholder values from the list of arguments passed.
For example, "Hello%sWorld!", "Beautiful"
printf; would display "Hello Beautiful World!" on to standard output
Syntax:
printf(“%formatspecifier”, variablename);
Q11. What is scanf() function ?
To read the input in C, we can use built-in function like scanf().The scanf() method, in C, reads the value from the console as per the type specified.
Syntax:
scanf(“%formatspecifier”, &variablename);
Q12. What do you mean by format specifiers?
The format specifiers are used in C during standard input and output. They specify with what datatype we are dealing with.
Q13. What is the char const in C?
char const is a modifier used to give special meaning to the declaration of a character variable or constant. It indicates that the value assigned to this entity will not be modified.
Q14. In what order does the newline escape occur?
The newline escape sequence (\n) is the most commonly used in C, and it occurs first when a program gets executed. When encountered within a string or character literal, \n specifies that one line should be terminated and another started. In other words, it tells the compiler to move on to the next line after processing its current one. This allows for more readable code since each statement can be placed on separate lines instead of crammed into single ones.
Q15. What is a data type and name the data types present?
It specifies the type of data that a variable can store, The most basic data types in the C language are int, char, float, and double.
Q16. What is a variable?
A variable in C is a named unit of data that is assigned a value. The value-assigned variable can be modified.
Q17. What is a constant in C?
A variable whose values cannot be adjusted or changed during the entire program is called a constant.
Example: Value of Pi.
Q18. In C header files, are functions declared or defined?
In C header files, functions are declared rather than defined. Function declarations in a header file provide the compiler with information about what arguments each function takes and returns what type of value. This allows other code to use that function without seeing its implementation (i.e., the definition).
When a program links together multiple object files, it uses this type of declaration as an interface between them so that one module does not need access to another's code for their associated symbols (such as variables or functions) to be linked together correctly during compilation/linking stages of building your executable program from source code.
Q19. What distinguishes embedded C from other programming languages?
A collection of language extensions called embedded C is utilized for embedded programming. It is based on the standard ANSI C but includes additional functions and features that better suit the needs of embedded systems development. Embedded C provides facilities to develop code that interfaces hardware devices directly with microcontrollers or supports real-time applications with limited resources such as memory, power consumption, and computational capability.
Compared to other programming languages, one of its main advantages is its close connection between software and hardware components: it allows developers to optimize their program quickly by accessing peripheral registers directly to use fewer processor cycles (CPUs).
Q20. What is modular programming in C?
A modular programming software design method divides complicated programs into simpler, more manageable chunks. This makes debugging and testing each module simpler and allows you to reuse code on different projects. In modular programming, each module consists of well-defined interfaces which communicate with each other through input/output operations such as file I/O or passing parameters via function calls.
Modules can also be further subdivided into functions (or subroutines) specific to the tasks undertaken by the program at hand — this often helps reduce complexity in larger applications where hundreds or thousands of lines may need to be organized to achieve the desired functionality.
Q21. What are the differences between const char and single character variables in operating systems in C?
Const char is an immutable type, and a single character variable in C is a mutable type. Const char variables must remain constant throughout the program, while single character variables may be modified or changed at any time during runtime. Another difference between const char and single character variables is that const chars are always explicitly typed. In contrast, characters can be implicitly assigned to different types depending on context and use.
For example, you could assign 'A' as an int (65) and a char ('A'). Finally, when declaring multiple constants of the same value, it's more efficient with memory allocation to declare them as one string rather than individual characters because strings take up less space in memory compared to separate letters - this makes sense considering they contain much more data points such as size, length, etc. which all require extra bytes of storage for each letter declared separately versus having just one string declaration with all the information contained within it already allocated from beforehand.
Q22. Explain the input string in C.
In C, an input string is a sequence of characters entered into the computer from an external source. Common sources include keyboard entries or text files on disk. Input strings can be used for many purposes in programming, such as user input validation and checking for certain patterns within the string. They can also be modified by trimming whitespace or finding substrings for more advanced processing tasks. An input string in a program must first be read by calling functions like "scarf," "getcha," or others depending on your development environment and the language version being used.
Q23. Explain the unwinding phase and winding phase.
The unwinding phase and winding phase in C are related to the process of deallocating memory. In the unwinding step, objects stored on the stack or frames allocated during a function's execution will be freed up before control is passed to another part of the code outside the scope of this particular frame. This helps prevent memory leaks since it ensures that any variables which may have been created by calling functions inside this scope can no longer be used when returning from them due to being invalidated through deallocation.
On the other hand, in windings phases, registers are rewound as they become available for further use after their original values have been either overwritten or lost due to optimization techniques applied at compile time, such as register renaming and instruction scheduling.
Q24. What is a static variable?
A static variable is a unique type of variable that keeps its value through multiple calls to a function and/or runs of a program.
Its values persist until the end of the program, meaning they remain with their initial assigned value throughout all scopes in which it has been declared. Generally speaking, this allows for a single instance or object to access information without having multiple copies around memory while still being accessible from anywhere where it's required.
Static variables are helpful when needing to keep track of certain things throughout your codebase, such as counters or flags inside loops or methods; since you know, only one copy exists, this will make sure any changes made by other sections won't override each other.
Additionally, static variables can be utilized as constants because their values can never change once initialized - making them great for situations where there is no need for them ever changing at runtime due to some external influence (e.g., manifest files).
Q25. How do we store a value in a variable?
The assignment operator is used to store a value in a variable, and the = is the symbol of an assignment operator. Likewise, the == operator is used to check if two values are equal. This comes under the category of relational operators.
Q26. What is an identifier?
Identifiers are symbols used to uniquely identify entities such as variables and functions. An identifier is a combination of alphanumeric characters. An identifier can start with an underscore or an alphabet but no other special characters are allowed.
Q27. Give examples of a valid identifier and an invalid identifier.
valid identifiers : identiifer_1, value2, val3, val3_val4
Invalid identifiers : @abc, &val1, 12value
Q28. What is a pointer variable?
A pointer variable is one that contains the address of another variable, and that points to a location in memory.
Syntax : const char const //* Constant Pointer or Char Pointer
Q29. What is a far pointer?
The far pointer is a 32-bit pointer that can access memory outside the current segment. A far pointer is a type in the C programming language that can access data from any memory segment. It can be used on all systems, but it is particularly useful for accessing memory beyond a system's first 64K bytes (the default segment size) without resorting to elaborate techniques like bank switching.
A far pointer holds two values—a 16-bit "segment" value and a 16-bit "offset" value which together make up its 32-bit address that uniquely identifies its location in RAM or ROM. The offset refers to byte addresses within its segment, while the base provides an additional layer that allows each different program's segments—and therefore also their code and data—to coexist peacefully inside one single physical computer system
Q30. What is a dangling pointer variable?
A dangling pointer is a pointer variable that points to a memory address that has either been freed or was never initialized. They are often caused when the object they point to goes out of scope, such as occurs when a local variable defined within a function is destroyed upon exiting the function. Dangling pointers can cause unexpected and difficult-to-track bugs and resulting program crashes, making them especially dangerous for programming in C language.
Q31. What is a void pointer?
A void pointer in C is a special type of pointer. It has the following characteristics:
- Any data type, including objects and functions, can be referenced by a void pointer. Thus it is also referred to as the generic pointer.
- The size of a void pointer is platform dependent and usually matches the word size (typically 4 bytes). As such, it can store addresses up to 4 GB on 32-bit systems or more on 64-bit systems.
- Since they do not have an associated data type, operations normally performed by de-referencing them cannot be done directly with a void pointer; this requires first casting them into another specific kind of pointer before performing these operations.
Q32. When can a void pointer and null pointer be used in C?
A void pointer can be used in C to store a pointer that does not have an associated data type. It is helpful for situations where the exact data type of the pointer's value must not be known or when multiple types need to use a single common reference without casting from one specific type to another. Since there isn't any information about what kind of object a void* points at, you cannot dereference it directly; instead, you must cast it back into some other valid pointer (with appropriate conversions) before being able to access its content.
A null pointer is also used in C, which points nowhere. A null pointer and 0 are synonymous; they both represent nothingness but differ slightly, as 0 is an integer. At the same time, NULL has special meaning within various programming contexts since all pointers evaluate as false when tested against NULL (as opposed to non-zero values). You can assign this value explicitly by writing "NULL" or using the macro defined in <stdio> header file, which expands out as ((void*)0).
Q33. What does a function's call by reference mean?
In call by reference, the address of the variable is passed into the function call as the Actual Argument. Since the memory allocator is the same for both formal parameters and actual arguments, changes made to the parameter inside the function change the value of the actual parameter.
Q34. What is a call by value in functions?
When a function is called by value, the formal parameters copy the value of the actual parameters; any modifications performed inside the function have no bearing on the parameter's actual value. Here, distinct memory regions are allotted for the actual and formal arguments.
Syntax:
int *p; // integer pointer
Q35. What distinguishes a C function definition from a C function declaration?
A C function definition is the actual code that implements a particular behavior or action. It consists of three parts: the return type, name and parameters of the declared C function, and its body.
The parameter list can be empty if no arguments are needed to operate within a given block of code (e.g., int foo() {...}). A C function declaration tells the compiler about a certain set of functions that will later on take place when running/executing any program written in C. It contains information such as input parameters, output results, etc., without actually providing executable instructions for implementing them. An example would be:
void myFunction(int x); // This is just declaration
void myFunction(int x) { printf("Value Of X Is %d ",x); }
Q36. What are library functions and how do they work in C?
Library functions are pre-written blocks of code that can perform various tasks. They provide multiple operations, from manipulating strings to working with graphics and databases in C programming. Experts in the field have written library functions, and most compilers will include these libraries as part of their distribution packages. Using library functions is simpler than writing them from scratch since less time is needed to implement and debug them, allowing developers to focus more on problem-solving techniques instead.
To use such pre-existing library functions appropriately within your programs, you need both function headers (which declare the parameters being passed into the function) and compiled object files or shared libraries which contain its actual definition or body parts responsible for carrying out some task(s).
Q37. What is an array?
A collection of identically typed items is called an array, and the data it contains is kept in contiguous memory or in a position next to it.
Syntax:
Datatpe arrayname [arraysize];
Q38. How can we reduce memory consumption and identify unused memory in allocation of memory in C?
Several techniques can be employed to reduce memory consumption and identify unused memory in the memory allocation process. One of these is segmentation, wherein a program's code and data are pre-determined areas or segments assigned pre-determined properties such as read/write access rights, size restrictions, etc. This allows for more efficient utilization of resources as bytes associated with specific tasks cannot overlap, leading to less wastage and preventing bugs from occurring due to incompatible overlapping codes.
Additionally, to reduce memory consumption and identify unused memory in the process of allocation of memory, dynamic allocation can allow programs greater flexibility with regard to their resource use by allowing them only the exact amount they need at any given time rather than occupying huge amounts dedicated spaces regardless of usage levels (a practice which leads eventually lead ultimately results in significant wastage). This method allows applications to request certain blocks when needed, reducing wasted space significantly.
Q39. In C, what does static memory allocation refer to?
When using the static memory allocation technique, the compiler allocates a predetermined amount of memory at the time of compilation. Static memory allocation is managed via stack.
Q40. Describe dynamic memory allocation.
Dynamic memory allocation is the technique of assigning the memory space during the execution time or the run time. For example, if we create an array using dynamic memory allocation, we need not bother about the size of the array.
Q41. How can a wide range of functions be incorporated into the current source file in C?
A wide range of functions can be incorporated into the current source file in C using header files, preprocessor directives, and libraries. Header files add declarations associated with standard library functions such as printf() and scanf(). Preprocessor directives allow for conditional code compilation at compile time based on certain conditions specified within your program.
Libraries provide access to a large set of predefined data types and objects that may already have specific function definitions defined, which you can call from anywhere in your program. Finally, inline code allows for the direct insertion and execution of small pieces of code along with other regular lines within the main source file.
Q42. What are the advantages and disadvantages of using static functions?
Advantages
- Static functions can be called without creating an object of the class, making them more efficient when many calls need to be made.
- They are also helpful when it makes sense for certain methods to be associated exclusively with a particular class rather than its instances/objects, as is often seen in utility or library classes (e.g., Math).
- The scope of static members and functions remain within the file they're declared in, meaning other files will not have access which provides extra protection against namespace collisions when programming large-scale projects since this helps keep related variables together but separate from others that might share similar namespaces elsewhere in codebase due to their self-contained nature under one header file only accessible by including said header into source code before compilation occurs.
Disadvantages
- Static member doesn't take any object parameter. So they cannot operate on a per instance basis, nor do operations that rely upon different values belonging to each instance, such as initialization tasks as the constructor does. Instead, all objects created within the same type would get the same value assigned due to lack of flexibility.
Q43. How do function prototypes aid in understanding location in memory?
Function prototypes define the memory locations of functions in a program. Since function prototypes list the function’s name, parameters, and return type before it is defined or declared in code, understanding its location in memory makes it easier for the programmer and the compiler to interpret their code. This information is readily available at compile-time rather than run-time, speeds up compilation, and helps optimize performance since there is no need to search through all possible places where a particular function could be located.
Additionally, using a prototype declaration across multiple files that include functions with the same names but potentially different arguments gives developers control over how these conflicts will be handled during linking, which can lead to better bug-prevention practices overall.
Q44. How to control the flow of execution in C?
The flow of control can be defined as the order in which function calls, instructions, and statements are being executed. The flow of execution of a C program can be controlled using if conditions, switch statements, and labels.
Q45. What is Loop in C?
Loop is used to execute the block of code repeatedly until the given condition is satisfied. The loop consists of a control statement (i.e. condition) and body of the loop, There are different loops like while loop, for loop, do-while loop in c.
Syntax:
for (condition) { code to be repeated }
What is the break statement in C?
The break statement terminates the execution of the nearest for loop, while loop or a do-while loop statement
Syntax:
statement: break;
Q46. What is a label in C?
To relocate program execution to another statement, we can use the goto statement in the C programming language. A statement in the code is identified by a label. Control is given over in an unconditional manner.
Q47. Difference between while and do-while loop.
Do-while loop only executes the body statement once before testing the condition, in contrast to while loop, which checks the condition first.
Q48. What are the key features of Character Arrays in C Language?
Character arrays, also known as strings in C language, are null-terminated arrays of characters used to store a sequence of characters that ends with the special character NULL (\0). Key features include that they can be manipulated using standard string functions like strlen(), strcat() etc., and accessing individual elements within them via indexing like other ordinary one-dimensional arrays is possible.
Q49. How do multidimensional arrays work in C Language?
A multidimensional array contains multiple levels or dimensions where each level consists of its own set of data stored in the form of an array component, such as columns and rows. Each element present in this type of data structure can be accessed by specifying a row number followed by a column number corresponding to its position on a two-dimensional plane or vice versa using syntax similar to two single variables separated by a comma operator, i.e. [i][j].
Learn More About Arrays! Understand the advantages and disadvantages
Q50. What advantages does dynamic data structure have in C language?
Dynamic Data Structures allow for efficient memory utilization and manipulation due to their property allowing them to grow/shrink while being implemented, unlike static structures, whose size remains fixed throughout the program execution lifetime, reducing overall wastage space when needed memory becomes excessive, thus providing a great degree of flexibility with both during designing phase without the need for extra amount code rewriting once end users decide to change underlying storage requirements further down application development timeline
Q51. Explain how Bitwise Operators can effectively manipulate integer values in C language.
By performing operations like AND, etc., bitwise operators are used to manipulate the bits that are present at the lowest level (the bit bag) of an integer number, which in turn reflects the data stored within it. on them according to Boolean Algebra successfully, allowing the user/programmer to perform activities as simple as setting individual low-order or high-order values from memory address storing said word size quantity alongside more complex tasks such changing their values selectively depending upon certain criteria set during the design phase.
Q52. Describe Pointer to Pointer and its usage within C Language programming.
Pointer to pointer concept involves using two levels of pointing, i.e., one where the initial address specifies the location containing another pointed further having its own assigned type, thus increasing flexibility being offered when dealing with a limited amount of system resources, especially dynamic structure implementing a linked list for example wherein each node holds both next and previous reference whose total length may change across run time thus making allocating appropriately sized space most important factor thereby resulting into need above technique since the link between any particular elements cannot be pre-known until actual connection made leading up corresponding nodes creation runtime stage
Q53. Compare higher-level languages and their relationship with a lower-level language such as C.
Higher-level languages (HLLs) are programming languages that have abstracted machine-level operations like memory allocation, addressing, etc., by providing various inbuilt functions and statements to users, taking away the unnecessary burden of writing complex code lines most commonly found within low-level languages such as C/assembly primarily due multiple available supported library being present thereby maintaining a layer between hardware components actual user end making coding much simpler yet powerful task when compared its counterparts.
Q54. Name some major differences between various versions of C programming language.
Major differences existing amongst different versions of the same family belonging to Programming Language can be ones regarding minor syntax changes introduced for streamlining particular specific sections more robustly along with other additions made to device driver support or increased runtime speed etc., all having influenced their unique way over final compiled output delivered during the application execution phase.
Q55. What are the special functions of C language?
Special functions in C are pre-defined functions that perform specialized tasks like arithmetic and logical operations, string manipulation, input/output etc. They return a value when executed upon successfully completing their task. Examples include printf(), scanf(), strcpy() and many more such standard library of special functions.
Special functions in C are special-purpose routines that perform specific tasks. Examples of these functions include input and output (I/O) operations, string manipulation, dynamic memory allocation, error handling, sorting arrays and more.
Q56. How do function pointers work in C language?
Function pointers provide access to the address of a function or subroutine without having to directly write out its name or parameters into the program code each time it is used as part of an operation or expression. A pointer can provide indirect access – pointing at something instead of using its direct address to pass arguments during calls from one part (or layer) of the software architecture to another.
Q57. What types of function checks exist in the language?
In addition to syntax checking, which ensures correct implementation within source code files by detecting errors caused by discrepancies between different expected formats, other types of function checks exist, namely, flow control checks designed detect anomalies related to unexpected sequence changes while running routines, boundary condition check designs determine any type parameterization problems incorrect due bounds setting and finally variable propagation check examines values passed between objects looking for potential conflicts with existing elements before execution.
Q58. Explain how to implement a hash function using programming code in C language.
A hash function takes some data - often base types like integers strings but can also include complex data types. This data is passed through a hashing algorithm, producing an output value known as the hash code or digest. The size of these outputs is usually consistent and will remain constant regardless of input values; this allows for easier comparison operations when checking files that may have been changed while employing security measures to maintain integrity.
Q59. Can you explain what a piece of code is meant for coding operations with an example from C-language?
A piece of code can be defined as any set number of lines containing programming commands (implemented via high-level coding languages) with well-formed syntax formulated from operators, keywords and literals aimed at performing specific tasks within software programs; one example could be a segment implementing arithmetic expression where numbers declared identified with keyword 'int,' multiplication operator denoted '*' followed by equal sign completing statement required to execute desired results
Q60. What kinds of Library Files can be used while working on projects related to C-language?
Library Files provide library functions designed to facilitate various tasks commonly encountered during application development, which can help speed up the process by setting specific elements or components throughout source-code
Q61. How does one handle values assigned to variables while writing programs or codes using c-Language?
Values assigned to variables must first be declared by data type (exact or approximate) before they are used within any c program; these can consist of literal values, references to other objects and expressions
Q62. Which applications can be developed using the C programming language?
A variety of applications such as system utilities, internet programs, mobile applications and graphical user interfaces have been created using different flavours of C Programming language
Q63. Can you explain the types of casting processes available with various data type formats provided by C-language?
The types casting process involves conversion between incompatible formats with simple techniques like double-casting used to convert an integer into float. In contrast, complex methods use classes to create a hierarchy representing types required passed during operations, e.g. error handling mechanism-based polymorphism.
Q64. Explain complex data structures which could be helpful during programming operations through the use of c-Programming language.
Complex data structures provided through library files help organize information-associated tasks more effectively, creating custom collections from built elements, typically consisting of pointers memory address linking array list nodes allowing faster access retrieval specific records when requested.
Q65. What is a function?
A function is a group of statements that together perform a task. Every C program has one mandatory function that is named main. The main() function controls the execution. The function calls to other functions are made from the main() function.
Syntax:
datatype main() { // code }
Q66. What is recursion?
The function calling itself is known as a recursive function. The recursive function can run endlessly if the terminating condition is not defined.
Syntax:
void add() {
add(); // function calls itself
}
Q67. What is a command line argument?
The arguments sent to the program during the program execution are known as command line arguments, and they are often given following the program's name with a space.
Q68. Do all the functions require to return some value?
A function need not return a value to the calling function always, if the return type of function is void, then there is no need to return a value.
Q69. In C, what are built-in functions?
The functions provided by the system and these functions are stored in the library. For example, scanf(), print().
Q70. What is the smallest unit of memory that a processor can address?
The smallest unit of memory that a processor can address is the byte. A byte typically consists of 8 bits used to represent data in binary form, allowing computers to interpret and manipulate information quickly and accurately.
Q71. What is the interrupt latency in C?
The interrupt latency in C depends on the type of architecture and system. Generally, most architectures have an interrupt latency between 1-2 microseconds for context switching when executing a simple instruction such as push/pop. This can be increased if more complex instructions are required or multiple interrupts occur rapidly.
Q72. How do machine code programs control the operations of computer hardware components?
Machine code programs control operations on computer hardware components by providing instructions or commands directly to the processor. This allows for basic operational functions such as input, output, arithmetic/logical operations, and other low-level tasks like transferring data between main memory (RAM) and registers within the CPU core.
Q73. What are code blocks, and how are they used in programming languages?
Code blocks are chunks of code that, when run at run time, carry out specified tasks within a programming language; they typically contain statements surrounded in curly braces ({}). They can range from simple sequences consisting of only two lines up to complex nested structures with multiple levels containing dozens of lines each; it just depends on what you need your program to do!
Examples might include loops or conditional expressions where certain outcomes determine how further down execution proceeds depending on an evaluated condition being true or false, egressing out else if clauses until eventually a final block results, either indicating success if conditions have been met along the path taken through its logic gates resulting in some action taking place accordingly as designed; failure would occur should any given criteria
Q74. Describe compound statements and provide an example of one in action.
Compound statements are combinations of two more simple code blocks which together create a single unit that is executed as one command - it should be noted, however, that all the operations within must complete successfully for this to work correctly and not break something else along with it!
For example, you could have an if statement controlling some action followed by another block doing something different depending on what value has been assigned from the preceding conditional expression, such as incrementing numbers each time or adding extra text output into a string variable containing other data already gathered from elsewhere beforehand.
Q75. What is type casting, and what purpose does it serve when coding with different data types?
Type casting is a technique used to convert data from one type into another more suitable form which can then be utilized within an application; this may include changing integers into strings.
For example, when storing them in files and other external output devices or converting floating point numbers back again so calculations involving decimals etc. come out as accurately as possible ensuring no inaccuracies occur during run time potentially invalidating results otherwise lost due mismatches between incompatible types initially assigned beforehand leading them becoming unusable because of their non-compatibility status - thus avoiding undesirable outcomes which cannot always be foreseen thereby necessitating its use often times undesirably too but still required anyway regardless making sure operations proceed correctly without interruption near the end vital successions successfully returned validly worthy uptake credit worthiness ensuring safe harboring retained satisfactorily
Q76. Name some of the commonly used built-in functions.
1. printf(): Prints the output to the standard output device (usually a monitor).
2. scanf(): Reads formatted input from keyboard or file stream and stores it in designated variables.
3. getchar(): Used to read characters from standard input such as keyboard until the user presses the enter key, displaying each character on the screen one after another.
4. putchar(): Writes a single character when given an argument of type int with a value between 0-255 or EOF, which means the end of the file.
5. strcat(): Concatenates two strings together and appends them into one string.
Q77. What is an intrinsic function in C language?
An intrinsic function is a built-in or predefined C language function that performs some specific operation, such as accessing hardware registers, setting certain flags, etc. but doesn't link with any other library files to do its work. These functions are embedded into the core of the compiler by its implementer and increase productivity during the code development process up to a great extent since there's no need to include related libraries before using these functions.
Q78. Name some common output functions used in C language.
Print (), puts (), puts() and printf() are commonly used output functions in C programming language which print a specified message on the screen while executing program code along with manipulating string formatting if required simultaneously according to the requirement mentioned within the statement itself using special symbol/signs like %d (for decimal), %f (for float) and so on.
Q79. What Kinds of Function Calls exist in the C programming language?
Three main kind of Function Calls are available when working with coding projects written under a standard ANSI-compliant version of c language: call-by-value and call-by-reference. The first kinds of function calls (i.e. continuous) is simply calling one function from within another for running a task in a loop or repetitively at specific instances. Where second and third Kinds of function are mainly focused on passing values between two different functional blocks during the execution process, which eventually helps achieve desired output with a minimum number of lines written.
Q80. What is a callback function?
A callback function, also referred to as a "intrusive" function, is a section of code that passes as an argument to other code. When the latter code is executed, the former functioning block will be invoked or executed using specific arguments supplied externally at the initialization step, resulting in a more dynamic nature when dealing with large sets of data stored inside memory structures like arrays, lists, etc.
Q81. What do you mean by callee function?
A callee function refers to any user-defined programming block being called from the main program or indirectly through a chain reaction caused due nested loops introduced initially via some already existing higher-order codes either prescribed under standard library files or imported manually into the project folder before even starting coding section since these enable programmer(s) for writing lines of codes with minimum errors.
Q82. What do you mean by caller function?
A caller function refers to any user-defined programming block responsible for calling some other piece of code or function either directly or indirectly to carry out certain tasks at a given instance, which helps programmers during their working process and eventually increases overall efficiency while taking into account time constraints too during problem-solving methodology adopted under specific scenario frequently encountered throughout development stage leading up to completion point gradually with new features being added one after another as required using library files (if needed).
Q83. What is the code for size in C?
Size in C language is a relative concept. Different types have different sizes, depending on the system and compiler used. Size defines the amount of memory (in bytes) occupied by an object or data type. The size function can be used to determine the exact byte size for each type available in the C programming language:
• char: It occupies 1 byte of memory space. Example - sizeof(char);
• int: It occupies 2 or 4 bytes of memory space (on most 16-bit and 32-bit platforms).Example - sizeof(int);
• float: It occupies 4 bytes of memory space. Example –sizeof(float);
• double : It occupies 8bytes of memory space .Example – sizeof(double);
Q82. What is an interrupt handler function in C language?
An interrupt handler function, also known as a "top-level" routine, is a special type of coding structure used when handling interruption events received from external sources differently than usual way written inside major functions dedicated solely towards servicing data coming from different devices attached parallelly on existing network path followed through looping mechanism found via variable definitions inside source file before even running the program itself thus helping programmer(s) design better codes within limited amount allotted per project slated for completion later on periodical basis following stipulated guidelines already set down implicitly prior there to help bring it
Q83. What is Non-volatile memory in C?
Non-volatile memory in C is a type of storage that maintains its contents even when power is lost. It consists mainly of ROM, PROM and EPROM, which are used to store programs and data that need to be retained when the system’s power source gets switched off.
Q84. What is Flash Memory?
These types often come together since they both use electrons instead of ultraviolet radiation, similar to how RAM works, except these modifications don't require constant refreshing like dynamic RAM due to its ability to hold a charge longer than normal DRAM cells and thus retain anything written to it permanently.
Unlike PROM, these types can also be reprogrammed repeatedly with the addition of an external programmer or, in some cases, by directly programming them with a microcontroller such as Arduino boards.
Q85. What is cache memory in C?
Cache Memory: A special subset of fast-acting, non-volatile storage employed by most modern processors today with various levels separating L1/L2/L3 caches depending on their roles within computer architectures.
It is designed specifically to store frequently accessed pieces of code or literal values temporarily right next door near CPU cores themselves, allowing quicker access times over regular RAM while still providing enough space not to overcrowd precious Hard drive real.
Q86. What is Random Access Memory (RAM)?
The CPU can randomly read from and write to Random Access Memory (RAM), a type of computer memory.It is volatile, meaning its contents are lost when power is removed, but it has much faster access speeds than other forms of storage, such as hard disks and optical drives.
Q87. How does Direct Memory Access (DMA) differ from RAM?
Direct Memory Access (DMA) differs from RAM because while RAM requires CPU intervention for all data transfers, DMA allows an external device - like a disk drive - to directly connect with system memory to transfer large amounts of data without involving the main processor at every step. This makes it much faster than using CPU cycles for each transaction since there's no need for extra instructions or context switches between devices during transfers which consume time and resources on CPUs performing other tasks.
Q88. What are the key features of memory access instruction?
The key features of a memory access instruction include commands specific to accessing particular addresses within physical memory, transferring chunks of information between separate memory locations in one instruction cycle, writing words into blocks addressed sequentially, determining if an address contains valid data before attempting any operation using that address, handling corrupted values due to power failure or busy signals from slaves, etc., among others.
Q89. Define Left shift operator in C
Left shift operator (<<) shifts each bit towards the left side for certain places according to the given value on the right side, and it also adds 0 at starting because we don't have any space after the last bits, so it just changes the position of bits nothing else into same length binary number format only. This process can easily be done manually without using this operator. Still, if your data size becomes large, you need to use operate instead of manual work with a calculator, etc.
Q90. What are compile-Time errors in C?
Compile-time errors occur when compiling source code written in some language (e.g., C). These errors usually involve syntactical problems like missing symbols, incorrect usage of keywords, or functions/statements being out of scope/undefined, etc. They will cause compilation failure if solved after executing the program(s). As such, they must be resolved before proceeding further with development tasks, and execution begins since
Q91. How to check for compilation errors in C language?
To check for compilation errors in C language, the programmer must first compile their code using a suitable compiler. Once the source code is compiled and linked, errors will be presented as 'warnings' or 'errors'. Warnings indicate potential problems that should be addressed but may not stop compiling altogether, whereas an error indicates something that renders the program unusable until it is fixed.
The user can look up each warning/error message online for more information about why it occurred and how to fix it before proceeding with further development tasks.
Q92. What is an extern keyword in C language?
The extern keyword in C is a storage class specifier that tells the compiler to treat an object or function as being defined externally. It signals to the compiler that another source will provide and initialize something at compile-time or link times.
For example, if a variable is declared with 'extern int x', it means that it has been declared elsewhere, and its value can be found there rather than locally within your code.
Q93. Explain different types of statements in C.
1. Nested if-Else statements: These are conditional statements containing another if statement. The inner if statement will execute only when the outer one evaluates to true. This allows for multiple checks of a given condition to decide what action needs to be taken next by providing complex programming logic structures as needed.
2. Print Statements: A print statement is used in C language programming and other languages, mainly outputting messages or values from variables on screen or printer devices, depending on the command issued.
3. GOTO Statements: It provides an unconditional jump from the current program execution line, sequentially numbered by the compiler to some specific code segment location, either backward direction (backward)or forward direction provided through the argument specified within the goto keyword.
4. Initialization Statement: An initialization statement assigns values to variables before any actual use happens with them during the program runtime period.
5. Iteration Statements: Iterations help us execute certain sets of instructions repeatedly until the assigned conditions get evaluated purposely set false value termination occurs flags off, thus releasing control out of loop body section reaches upto top most loop instruction begins further fresh new iteration process begins again continues like this till the whole cycle completes successfully.
Q94. Define Bytes of Memory in C.
A byte of memory in C is a unit of data storage that can hold one character (a letter, number, or symbol). It consists of 8 bits and has a total capacity of 256 possible values. Each bit within the byte can store either 0 or 1. Bytes are typically organized into larger units such as words (16-bits) and double words (32-bits).
In C programming language, bytes are represented by char type, usually written as "char." This type may range from -128 to +127, depending on platform architecture. The size_t integer unsigned types hold unsigned integers related to memory allocations called `size of.`
Q95. What are the normal variables in C?
Normal variables in C are data variables that can store values of different types, such as int, char, float and double. They have declared with the syntax: type identifier;
Q96. What is the enter key in C?
The enter key in C is called the carriage return (or "CR"). The code character typically indicates that the cursor is being moved to a new line.
Q97. Explain the double-pointers on C.
Double pointers are variables that either store or reference another pointer variable. They can be used for many things, such as dynamic memory allocation, data transmission between functions, and the creation of linked lists. Double pointers offer an additional degree of abstraction that makes it simpler for developers to work with complex structures like trees or graphs by allowing access to objects through several indirection layers.
Q98. What is the huge pointer in C?
Huge pointers in C represent an extended version of traditional pointer variables. Unlike normal pointers, which point to individual bytes or words in memory, huge pointers can address up to 4 Gigabytes (GB) RAM in segmented memory mode or 1 GB RAM on a flat model architecture. Huge pointers must be declared using 'huge' combined with the other appropriate declarations like far, near, and ptrdiff_t qualifier before they can be used for large-scale computation purposes.
Q99. What are the different types of systems in C?
- Real-Time Operating System: It is designed to meet the needs of a specific application or device, such as controlling machinery in industrial settings. Real-time OS are typically used for embedded systems and aim to guarantee quick response times by rapidly responding to input events without significant delays.
- Automotive System: Automotive systems refer to any software, hardware, and networks employed in automobiles, trucks, and other motor vehicles. These consist of technologies like infotainment systems (i.e., navigation), communication devices (e.g., Bluetooth/WiFi connectivity), safety controls (such as ABS), and advanced driver assistance features (like lane keep assist). Many feature preinstalled applications which allow users access various functionalities while on the go, including emergency services contact information and live traffic updates, etc.
- Fault-Tolerant Systems: Fault Tolerant Systems are computer systems specifically engineered with built-in redundancy to continue operation even when individual components fail due to errors or malfunctions from external factors such as temperature changes or power surges. They often employ RAID storage solutions, hot-swappable hard drives, or redundant server configurations, among many other strategies, which can help seamlessly reduce downtime during outages caused by natural disasters or operations.
- General-Purpose Operating System: A general-purpose operating system is an all-purpose operating system designed to cater to the needs of various users in different scenarios and tasks such as networking, gaming, video editing, etc. It must be capable of running multiple applications concurrently while ensuring data security and stability with control mechanisms and access privileges built in from the ground up. Examples include Windows 10 or Mac OSX, which support various types of software programs alongside core functions like file management or memory allocation/deallocation.
Q100. What is a Stack?
Stack is a data structure that stores homogeneous data, in LIFO (Last-In-First-Out) manner. For example, a pile of books.
Q101. What is Stack Overflow in C?
Stack, a Last-In-First-Out (LIFO) data structure, is used to hold local variables that are used within functions. Stack Overflow errors happen when a program utilizes more memory than is allotted.
Q102. What is a struct?
A struct is a keyword in C that creates a user-defined data type. A structure is a collection of heterogeneous data-type elements.
Q103. What is a Union?
Similar to struct, Union is a user-defined datatype in C. Union is a collection of variables of heterogeneous datatypes in the same memory location.
Q104. What is the difference between struct and union?
In a struct, each data member occupies a unique memory space, whereas, in a union, data members share the memory space of the largest size variable.
Q105. What distinguishes malloc from calloc?
The calloc() procedure assigns several blocks of memory to a single variable, whereas the malloc() function produces a single block of memory of a given size.
Q106. What is the use of realloc() ?
A block of memory that has already been allocated using the malloc or calloc functions can be resized using the realloc() function.
Q107. What is Free()
The free() function in C is used ****to free up or for Deallocation Of Memory blocks that are previously allocated by calloc(), malloc() functions.
Q108. What are the non-volatile variables in C?
Non-volatile variables in C retain their value even after the program ends or is closed. These include global and static local variables and constants defined using the #define preprocessor directive.
Q109. What is meant by file extension in C?
File Extension in C refers to filename suffixes used by programming language compilers such as gcc, clang etc., for their source code files, typically with '.c' extension.
For example, 'example code. c'. The file extension indicates how different programs should interpret or compile a particular file on any system platform with the correct compiler/interpreter installed and configured correctly.
Q110. What is an infinite loop?
A loop becomes an infinite loop when there is no terminating condition, i.e., the condition will always be true. An infinite loop in the while loop can be achieved by keeping the condition as 1, the for loop can be made an infinite loop by using two semi-colons i.e. ;; Following is an example.
while(1)
{
body of loop
}
for(;;)
{
body of loop
}
Q111. Explain the datatype format specifier in C.
A datatype format specifier in C is a character that determines the way data would be printed on the output device, such as "%d" for integers, "%f" for floating point numbers, and " %s" for strings. It provides information to the compiler about how exactly the user wants to print variables, contents, or values on the console or any other output device like file, etc.
Each datatype format specifier has its specific meaning, and it works accordingly such that proper value is assigned during reading from input devices.
Q112. What are the base values and floating-point values in C?
- Base Values: These are the fundamental data types built-in to C language and represent basic values such as numbers, characters, strings, etc. The base values in C include int (integer), float (floating point number), char (character), and void.
- Floating Point Values: A floating point value is a sort of numeric representation that enables us to more accurately store real numbers than is possible with just integers. In order to restore a number's original value from memory storage, it divides it into two parts: the mantissa, or fractional part, and an exponent, or power of 10, that specifies how much it should be multiplied. For single precision floats, 32 bits are used, and for double precision values, 64 bits.
Q113. In C, what is a memory leak?
When a program allocates memory for use but forgets to deallocate it once the task is successfully performed, it is called a memory leak. Over time, this can cause the program's allocated memory to grow without bound and eventually produce an Out Of Memory Error if not caught in time.
Memory leaks are commonly seen in C and C++ applications because they manually manage dynamic variables and data structures using malloc()/free(). When objects dynamically created by these functions aren't disposed of correctly, they accumulate on the heap, wasting precious RAM resources until either garbage collection cleans them up or the application runs out of heap space.
Q114. List some features of memory management in C
Memory Management in C is a process of allocating and deallocating memory blocks to the programs as per their need at runtime. This memory management helps avoid wastage of unused allocated space during execution because, with proper utilization, maximum possible performance can be achieved from the system’s resources.
The main features associated with Memory Management in C are:
Dynamic Allocation & Deallocation: In this approach, an application has access to various functions that allow it to allocate or free up chunks o memory while running dynamically. The two most popular dynamic allocation/deallocation functions are malloc() and free(). Malloc() allocates space for data storage requested by the program; conversely, Free() releases previously allocated blocks when no longer needed by the program.
Pointer Arithmetic: Using pointers arithmetic is one-way programmers gain more control over how they use& manage memory within their applications; however, there are some inherent risks involved since pointer errors (e.g., accessing outside bounds into the non-existent region ) can cause unexpected behavior and lead to potential security vulnerabilities if not handled.
Array of Pointers: An array of pointers is a data structure that points to multiple memory locations. It helps the users manage many elements by storing them into a single variable, thereby reducing complexity and improving efficiency while accessing or manipulating data stored within those addresses pointed out by pointer variables.
Memory Alignment: This feature deals with how bytes are arranged in memory during the allocation process so that they can be efficiently accessed when needed, e.g., for integers, most architectures allow two-byte boundary alignment, whereas double words (32 bits) should reside at four-byte boundaries, etc.
Stack & Heap Management: In C language, these two aspects play a critical role when it comes to allocating& deallocating dynamic blocks as each provides a different approach towards the same task, i.e., stack uses last-in-first-out (LIFO ) technique/heap utilizes first -in first out ( FIFO).
Q115. Write a program to check if a given number is prime or Not.
We will take the input of a number using scanf(), then we will run a for loop and check if the number is divisible by any other number other than one and itself; if it is divisible by any other number set the notprime flag to 1, at the end, we will check the value of nonprime flag and print the result.
#include
int main() {
int n, i, notprime= 0;
printf("Enter a number: ");
scanf("%d", &n);
if (n == 0 || n == 1)
{
notprime = 1;
}
for (i = 2; i <= n / 2; ++i) {
if (n % i == 0) {
notprime = 1;
break;
}
}
if (notprime== 0)
printf("prime number.", n);
else
printf("Not a prime number.", n);
}
Q116. Write a program to print the first 10 Fibonacci numbers.
By default, the first two numbers of a Fibonacci series are 0, and 1 now we add these two to get the 3rd number and add the 2nd, and 3rd number to get the 4th number.
In the code, we will use a for loop to find the first 10 Fibinacci numbers.
#include
int main() {
int i, n=10;
int a = 0, b = 1;
int c = a + b;
// print the first two terms t1 and t2
printf("Fibonacci Series = %d %d, ", a, b);
// print 3rd to nth terms
for (i = 3; i <= n; ++i) {
printf("%d ", c);
a= b
b= c;
c= a+ b;
}
}
Q117. Write a program to find the factorial of a given number.
The factorial of n is the product of all positive descending integers. The factorial of n is denoted by n! So, for example, the factorial of 5 is 321, which is 6. In the following code, we will use a for loop to multiply the given number with all the numbers till 1.
#include
int main()
{
int i,factorial=1,n;
printf("Enter a num \\n ");
scanf("%d",&num);
for(i=1;i<=nu;i++){
factorial=factorial*i;
}
printf("Factorial = %d",factorial);
}
C is considered a robust language that has a rich set of built-in functions, data types and operators that can be used to write any complex program. Hope the above article helped you understand many basic concepts of the C programming language and helped you improve your grasp of it. Keep learning!
Suggested Reads:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
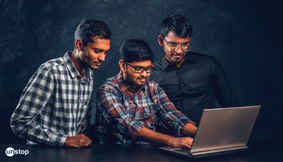
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
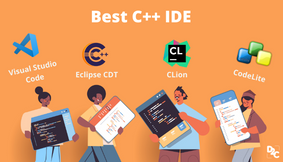
10 Best C++ IDEs That Developers Mention The Most!
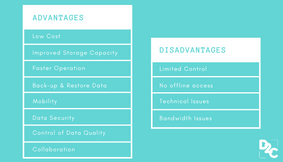
Advantages and Disadvantages of Cloud Computing that you should know!
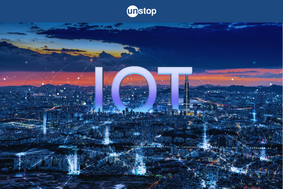
Comments
Add comment