Jump Statement In C | Types, Best Practices & More (+Examples)
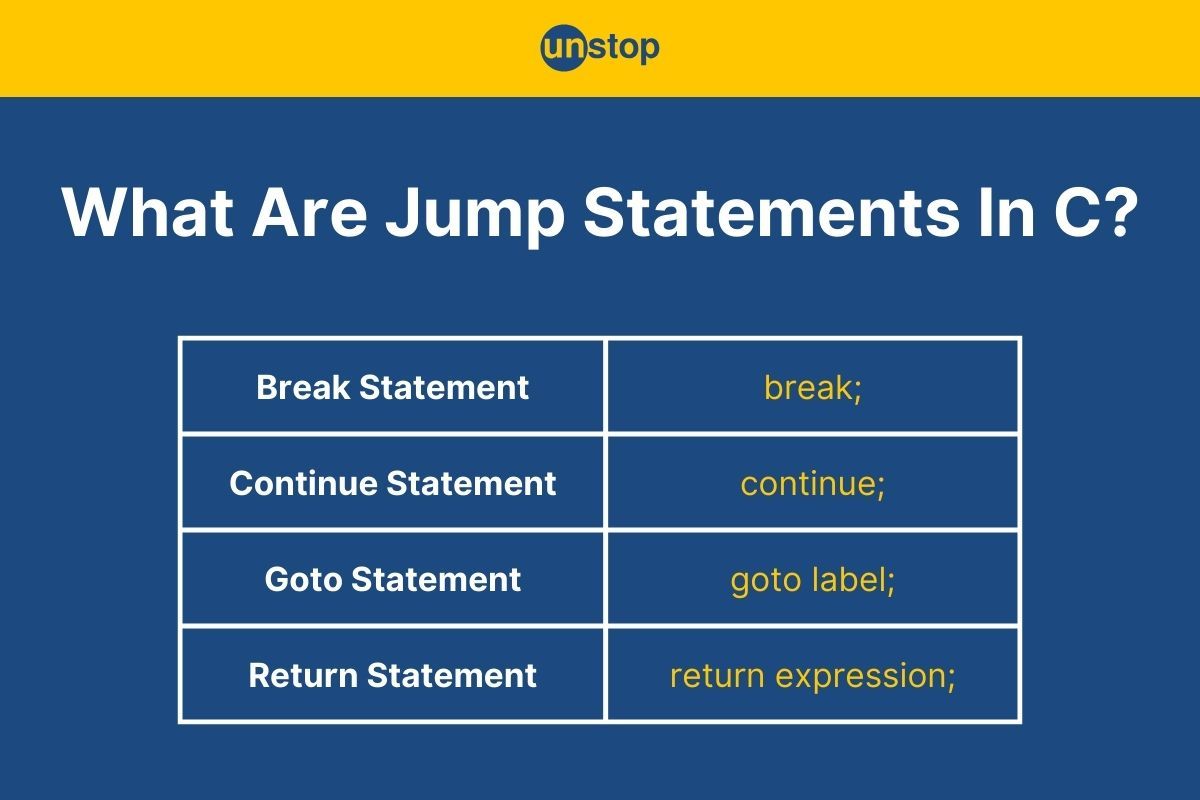
C stands as a robust and versatile programming language known for its efficiency and low-level control. One of the features that set C apart is its support for jump statements, which enable programmers to navigate control flow in a flexible manner. Jump statements provide the ability to transfer control from one part of a program to another, allowing developers to create more dynamic and responsive applications. In this article, we will delve into the world of jump statements in C language, exploring the different types and understanding how they can be employed effectively.
What Is A Jump Statement In C?
In C programming, a jump statement refers to a control statement that allows the program to alter its normal sequence of execution by transferring control to another part of the code. A jump statement in C provides a means to change the normal sequence of execution of statements, allowing for a more dynamic and flexible control flow.
What Is The Need For Jump Statements In C?
- Control Flow Flexibility: Implementation of jump statements in C programs provides a way to dynamically control the flow of program execution, allowing programmers to implement more flexible and responsive logic in their programs.
- Code Optimization: Jump statements, when used judiciously, can contribute to code optimization by allowing for early termination of loops or skipping unnecessary iterations.
- Error Handling: Jump statements in C programs can be useful for error handling, enabling the immediate termination of a sequence of code upon encountering an error condition.
- Simplifying Code Structures: In certain scenarios, jump statements can simplify code structures, making the program logic clearer and more concise.
Types Of Jump Statements In C
There are three primary types of control statements that work as jump statements in C, i.e., goto, break, and continue. Each serves a unique purpose, offering programmers the tools needed to manage program control flow efficiently.
- Break Statement: It is used to terminate a loop or switch block and transfer the program control to the line of code next to the loop or switch block of code.
- Continue Statement: It is used to skip the code after this statement in a loop, and the program control is transferred to the beginning of the loop again.
- Goto Statement: It is used to transfer the program control to a prespecified part of the code based on a label followed by a colon.
Break Jump Statement In C
The break statement is a type of jump statement/ control flow statement used primarily within loops (for loop, while loop, do-while loop) and switch statements. When encountered, the break statement terminates the nearest enclosing loop or switch statement, allowing the program to exit the loop prematurely. In other words, it causes the program flow to break when it is encountered and move out of the respective construct.
In the context of loops, it is often used to exit the loop based on a certain condition, providing a mechanism for early termination. In a switch statement, the break is employed to exit the switch block after a particular case is executed, preventing fall-through to subsequent cases. The break statement is valuable for altering program flow and achieving more flexible and responsive code execution in various situations.
Syntax of Break Jump Statement In C:
Loop or switch block{
if(break condition is true)
break;}
_ -> control comes here out of the block
Here,
- The break keyword marks the statement that is used inside a loop or switch case block.
- If the break condition is met, the program control comes out of the loop or switch case block immediately.
Code Example:
Output:
0 1 2 3 4
Loop terminated
Explanation:
In this simple C program, we begin by including the <stdio.h> header file, essential for input/ output operations.
- We then begin the main() function which is the entry point of the program.
- Then, we create a for loop with a loop variable i whose initial value is 0, and it continues till i is less than 10.
- Inside the loop, there is an if-else statement that uses the equality realtional operator to check if the current value of i is equal to 5.
- If the condition i==5 is true, then the break statement in the if-block is executed, and the loop terminates prematurely.
- If the condition is false, then the piece of code inside the else block is executed. The printf() statement displays the value of variable i, followed by a space. Here, the %d format specifiers are the placeholder for the integer value.
- After every iteration, the value of the control variable is incremented by 1. In this example, the loop runs for 5 iterations, after which the break statements come into effect.
- Once the loop terminates, the second printf() statement displays the string message- Loop terminated, to the console.
- The newline escape sequence before the string value moves the cursor to the next line before the message is printed.
- The return 0 statement indicates successful program execution without any errors.
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
Continue Jump Statement In C
The continue statement is a control flow statement used within loops (for, while, do-while). The purpose of this jump statement in C is to skip the rest of the statements inside the loop for the current iteration and move on to the next iteration.
When the continue statement is encountered, the program jumps directly to the loop's increment or update statement, bypassing any remaining code in the loop's body. This allows for selective execution of loop iterations based on specific conditions, providing a way to exclude certain statements within the loop for improved efficiency or logic. The continue statement is particularly useful when there is a need to skip specific iterations without prematurely terminating the entire loop.
Syntax of Continue Statement:
Loop or switch block{
if(condition)
continue; //line 3 and 4 are not executed for this iteration and we move on the next iteration
Line 3
Line 4}
Here,
- The if keyword marks the beginning of the if-statement, with the condition referring to the looping condition/ test expression.
- The continue keyword marks the continue statement, which, when triggered, makes the program control skip execution of Line 3 and Line 4 for the current iteration.
Control then proceeds to the next iteration of the loop or the next case in the switch block.
Code Example:
Output:
1 3 5 7 9
Loop terminated
Explanation:
In the sample C code above-
- We initiate a for loop with a loop control variable i initially set to 0 inside the main() function.
- The loop runs until the condition i<10 is true, after which it terminates. After every iteration, the value of the control variable is incremented by 1.
- Inside the loop, there is an if-statement where-
- We first calculate the remainder of the i divided by 2 using the modulo arithmetic operator and then check to see if it is 0, indicating an even number.
- If true, the if-block containing the continue statement is executed, and the program skips the rest of the loop and jumps to the next iteration.
- If false, the loop continues to the printf() statement displays the value of the control variable i to the console, followed by a space.
- After the loop terminates, another printf() statement is used to print the message- Loop terminated, to the console.
- Finally, the program terminates with a return 0 statement, indicating successful program execution.
Goto Jump Statement In C
The Goto statement is a jump statement in C that is used to transfer the control to any part of the code based on a label followed by a colon. It allows us to jump to a particular section of code that may be ahead of the goto statement or behind(namely forward goto and backward goto).
Syntax for Goto Jump Statement in C:
goto label;
Here,
- The goto keyword marks the beginning of the statement.
- The term label is a user-defined identifier followed by a colon marking a specific location in the code.
The Goto statement can take two forms depending on the place where the statement will lead the program execution. They are as follows:
Forward Goto Jump Statement In C
In this, the label is present below the goto statement in the code, i.e., the control is transferred to the label, which is present in the sections of code after the goto statement.
Syntax:
line 1
Goto X; //Forward Goto
line 2
X: - - - -> control comes here
Backward Goto Jump Statement In C
In this, the label is present above/ before the goto statement in the code, i.e., the control is transferred to the label that is present in the lines of code written before the goto statement.
Syntax:
line 1
X: - - - -> control comes here
Goto X; //Backward Goto
line 2
Now, let's take a look at a code example that showcases the implementation of both these types of Goto jump statements.
Code Example:
Output:
Choose an option:
1. Forward Goto
2. Backward Goto
1
Before forward goto
Jumped to forward_label
Explanation:
- We first declare a variable choice of integer data type inside the main() function.
- Then, as mentioned in the code comments, we display the menu options to the console using a set of printf() statements and prompt the user to enter their choice.
- The program reads the option entered using the scanf() function and saves it to the choice variable.
- Next, we create a switch case statement with goto statements to handle different cases based on the user's choice.
- If the user chooses option 1, the program executes the code for forward goto.
- If the user chooses option 2, the program executes the code for backward goto, resulting in an infinite loop.
- If the user enters an invalid choice, a default message is printed.
- The main function concludes by returning 0, indicating successful program execution.
- In summary, the code provides a menu for the user to choose between forward and backward goto scenarios.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Return Statement In C
In C programming, the return statement is a fundamental control flow statement used within functions to terminate their execution and to pass a value back to the calling function. It is crucial for providing output from functions, terminating function execution, and indicating the exit status of a program. Its usage depends on the specific requirements of the function and the desired behavior in the overall program structure.
A function can return a value or nothing based on the return type of the function.
Syntax:
return expression;
Here,
- The return keyword marks the beginning of the statement.
- The expression refers to the value the statement will return.
Code Example:
Output:
The square of 5 is: 25
Explanation:
In the example C code,
- We include the standard input-output library (stdio.h) to use functions like printf for output.
- We then define a function named square, which takes an integer num as an argument.
- Inside the function, we use the multiplication operator to calculate the square of num and store the outcome in a variable named result.
- The return result statement sends the calculated result back to the calling function.
- Inside the main() function, we declare an integer variable input and assign the value 5 to it.
- Next, we call the square function with user input as an argument, and we store the result in the variable result.
- Finally, we use the printf() function to display the result of the square calculation.
- The return 0 statement is used to indicate successful program execution to the operating system.
Advantages And Disadvantages Of Jump Statement In C
Jump statements in C, including goto, break, and continue, offer programmers a way to control the flow of execution within their programs. However, like any programming construct, they come with their own set of advantages and disadvantages.
Advantages Of Jump Statement In C
- Flexibility in Control Flow: Jump statements provide flexibility in controlling the flow of a program. They allow programmers to navigate to specific points in the code, break out of loops prematurely, or skip certain iterations, enabling more dynamic and responsive programming.
- Simplifying Control Structures: Jump statements in C programming can simplify complex control structures by providing a direct way to exit loops or switch cases or to jump to a particular label in the code. This can lead to cleaner and more readable code in situations where alternative control flow mechanisms might become convoluted.
- Optimization of Performance: In certain cases, judicious use of jump statements can lead to optimized performance. For instance, using a break statement to exit a loop early when a condition is met can prevent unnecessary iterations, potentially saving computation time.
- Useful in Error Handling: Jump statements in C can be useful in error handling scenarios, where immediate termination of a sequence of code is necessary upon encountering an error condition. This can help prevent further execution of code that might rely on erroneous data or states.
Disadvantages Of Jump Statement In C
- Complexity and Readability: One of the most significant disadvantages of jump statements in C, particularly goto, is their potential to introduce complexity and reduce code readability. Unrestricted use of goto can lead to spaghetti code, making it difficult to understand and maintain the program over time.
- Difficulty in Debugging: Programs that extensively use jump statements can be challenging to debug. Jumping around the code disrupts the natural flow of execution, making it harder to trace the logic and identify the source of bugs or unexpected behavior.
- Violation of Structured Programming Principles: Jump statements in C, especially goto, can violate the principles of structured programming, which emphasize the use of structured control flow constructs like loop statements and conditional statements. Uncontrolled jumps can make code harder to analyze and reason about, potentially leading to unintended consequences.
- Potential for Spaghetti Code: Overuse or misuse of jump statements, particularly goto, can result in spaghetti code, where the control flow becomes tangled and difficult to follow. This not only hampers maintainability but also increases the likelihood of introducing bugs during code modifications.
Common Mistakes While Using Jump Statements In C
As we've mentioned before, jump statements in C are used to transfer control from one part of the program to another. While these statements can be useful in some cases, they can also lead to errors if not used correctly. Some of the common mistakes to avoid while using jump statements in C are as follows:
- Improper labeling and usage: While using break or continue jump statements in C, it is quite possible to accidentally break out of the loop or skip over important code in the switch case block. To avoid this, be sure to label carefully and specify which loop you want to break out of or continue.
- Using goto statements: The goto jump statement in C can be useful in some situations, but it is generally considered bad practice to use in code (often leading to spaghetti code). They can make code harder to read as the flow of code is disturbed. However, they are used widely in error handling.
- Using break or continue in functions: Using break or continue to leave a current function can cause errors, as it can leave the function in an incorrect manner. This can further lead to an undefined state.
Alternatives To Jump Statement In C
Jump statements in C helps to control the flow of execution of code. However, using these can make our program difficult to understand and handle. There are many alternatives to jump statements in C that can be used to achieve the same outcome in a better and smoother way. They are:
- Looping Constructs: Use while, for, or do-while loops to control program flow. These constructs provide structured ways to repeat a block of code until a certain condition is met without the need for jumping around the code.
- Conditional Statements: Use if, else-if, and else statements to conditionally execute blocks of code based on certain conditions. This allows for branching based on logical conditions without needing to use the goto jump statement in C.
- Functions: Break your code into functions and call them as needed. Functions encapsulate a block of code, allowing you to call them from various parts of your program without the need for explicit jumps.
- Error Handling: Use error codes or exceptions to handle exceptional cases and guide the program flow accordingly. Instead of jumping to a specific location when an error occurs, handle errors gracefully within the flow of the program.
- State Machines: Code implementation state machines to model the behavior of your program. State machines have well-defined states and transitions between states, making the program flow easier to understand and control.
- Structured Programming: Follow the principles of structured programming, which emphasize the use of control structures like sequence, selection, and iteration to create clear and understandable code without resorting to goto jump statements in C.
- Recursion: Use recursion to solve problems that can be broken down into smaller, similar sub-problems. Recursion can often replace the need for goto by allowing functions to call themselves with modified parameters until a base case is reached.
- Exception Handling: If your C program supports it (e.g., C++ with exceptions), you can use try-catch blocks to handle exceptional situations, providing a more structured way to manage errors and unexpected conditions.
Best Practices: Jump Statement In C
The jump statement in C is very useful in programming and has many applications. Here are some of the best practices for using jump statements in C:
- Use them less: In general, it’s better to use conditional statements (like if/else statements) or loops (like for/while loops) to control the flow of execution. Jump statements should only be used when it’s absolutely necessary.
- Use of comments: It is best practice to use code comments to explain the usage of the jump statement and what they are doing. They make it easier for other developers and readers to understand, read, or modify the code.
- Error Handling: If you use a jump statement in C for error handling, consider alternatives like returning error codes or using exception handling (if supported). This can make error handling more structured and predictable.
- Consider Alternatives: Evaluate whether there are alternative ways to achieve the same goal without using a jump statement in C. Refactor your code to make use of loops, conditionals, and functions to maintain a more structured control flow.
- Testing and Code Review: Thoroughly test the code containing jump statements and ensure that it behaves as expected. Additionally, involve others in code reviews to get feedback on the usage of jump statements.
Conclusion
Jump statements in C provide programmers with a valuable set of tools for managing control flow within their programs. While the goto jump statement should be used cautiously to avoid creating spaghetti code, the break and continue jump statements offer cleaner and more structured ways to control loops.
Understanding how to leverage these jump statements in C programming can empower developers to write more efficient and readable code, enhancing their ability to craft robust and responsive applications. As with any programming feature, a balanced and thoughtful approach ensures the optimal use of jump statements in C programs.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. What is a jump statement in C?
A jump statement in C is a control statement that allows the program to alter its normal flow of execution by transferring control to another part of the code. Jump statements provide a mechanism for changing the sequence in which statements are executed, enabling a more dynamic and flexible control flow within a C program. The primary jump statements in C are goto, break, and continue.
- The goto statement allows the program to jump to a labeled statement elsewhere in the code, effectively changing the execution flow.
- The break statement is used to terminate the execution of a loop or switch statement prematurely, transferring control to the code section immediately following the terminated loop or switch.
- The continue statement is employed within loops to skip the rest of the current iteration and move on to the next one based on specific conditions.
Q. Why should we avoid the usage of goto jump statements in C?
Avoiding the usage of goto statements in C is advised due to concerns related to code readability, maintainability, and adherence to structured programming principles. This is because code containing goto statements tends to be less readable, as it disrupts the linear flow of execution, making it challenging for developers to comprehend the logic.
Excessive use of goto can result in something called spaghetti code, which is intricate and challenging to navigate. Structured programming principles advocate for the use of loops, conditionals, and functions to improve code clarity and reliability. Additionally, the use of goto can lead to scope issues with variables, making it harder to manage variable visibility within different code blocks.
Q. When do we use a return jump statement in C?
A return jump statement in C language is a control flow statement that is used to terminate a function immediately with or without a value, and the control is transferred to the line that made the function call. The return statement has 2 forms:
- Without value (when the function doesn’t have a return type or void return type)
- With value (the function returns a return value to the caller based on return type)
If a function has multiple return statements, only one of them will be executed, and the function will exit at that point. If a non-void function encounters a return statement without a return value, it can lead to a compilation error.
Q. What is a short jump and a long jump in C?
Short Jump: In assembly language or machine code, a short jump typically refers to a jump instruction that allows for a relatively small jump distance within the program. Short jumps are often used when the target location (the address to jump to) is within a limited range from the instruction itself. These jumps are more efficient in terms of instruction size and execution speed.
Long Jump: Conversely, a long jump refers to a jump instruction that can cover a larger distance within the program. This is necessary when the target location is farther away from the jump instruction. Long jumps may involve more complex addressing modes or additional information to specify the target address accurately.
Q. What is an unconditional jump statement in C?
An unconditional jump statement in C is a type of control flow statement that causes the program to transfer control to a specific location in the code unconditionally. This means that the jump always occurs, regardless of any conditions or expressions. The primary unconditional jump statement in C is the goto statement.
The goto statement is used to transfer control to a labeled statement within the same function. Its syntax is as follows:
goto label;
Here, a label is a user-defined identifier followed by a colon, marking a specific location in the code. The goto statement then directs the program's execution to the labeled statement.
While the goto statement allows for unconditional jumps, its use is generally discouraged in modern programming practices due to the potential for creating unreadable and hard-to-maintain code. Unrestricted use of the goto jump statement in C program can lead to spaghetti code, where the flow of execution becomes non-linear and difficult to follow.
You might also be interested in reading the following:
- Logical Operators In C Explained (Types With Examples)
- Ternary (Conditional) Operator In C Explained With Code Examples
- Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
- Bitwise Operators In C Programming Explained With Code Examples
- Constant In C | How To Define & Its Types Explained With Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment