Goto Statement in C Explained (With Examples)
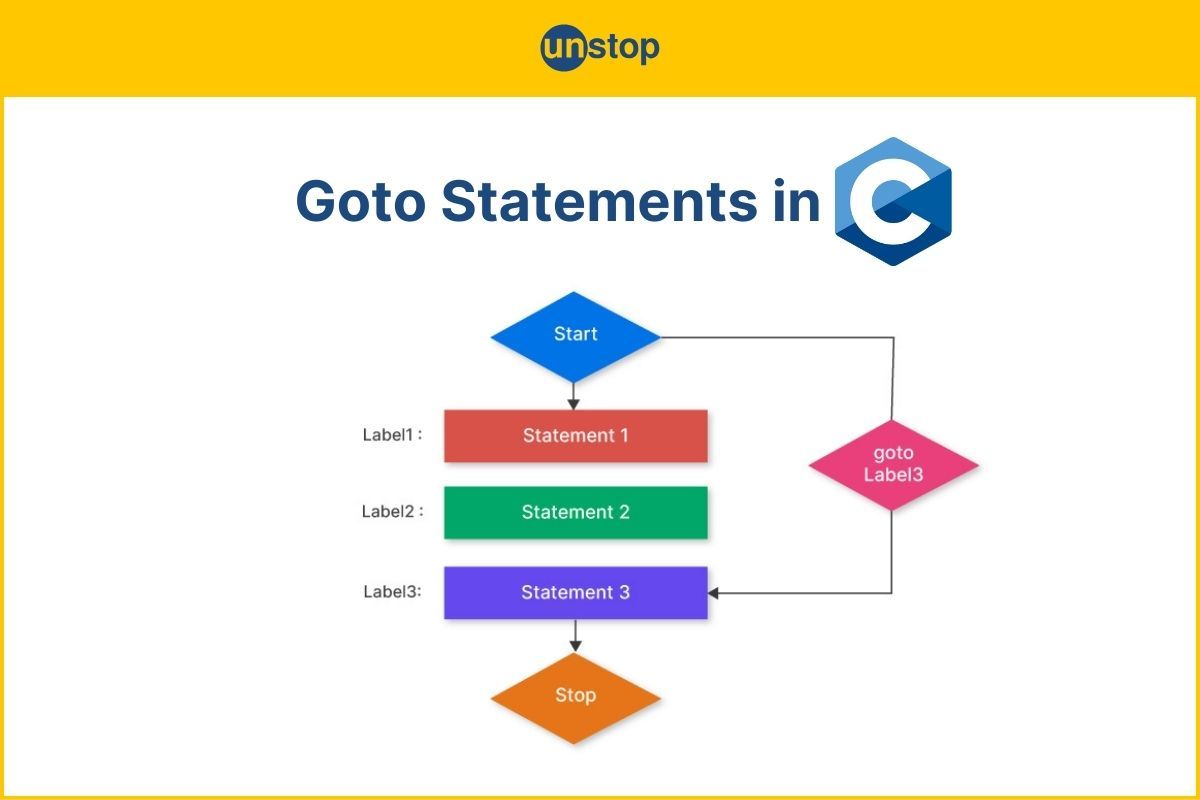
In the realm of programming languages, the C programming language is known for its simplicity, efficiency, and control it provides to developers. However, there is a controversial and often misunderstood feature that has been part of C since its early days—the infamous goto statement.
The goto statements in C allow programmers to transfer control within a program, jumping from one part of the code to another. While its usage has been widely discouraged due to concerns about code readability and maintainability, understanding the goto statement can shed light on its appropriate application and help developers make informed decisions.
Why do we use control statements?
In C, control statements assist the compiler in deciding whether to allow the control to flow through a particular set of statements or not when executing a specific logical statement. In other words, they shine a light on the execution of statements, given specific circumstances.
Control statements allow us to execute specified code blocks based on conditions or loop test expressions, increasing the efficiency, flexibility, and adaptability of our programs. The various types of control statements in C are:
Conditional Statements:
- If-Else
- Ternary/ Conditional Operator
- Switch
Looping/ Iterative Statements
- While Loops
- Do-While Loops
- For Loops
Jump Statements
- Break and Continue
- Goto
What is the goto statement in C?
The goto statement in C is an unqualified statement that hands power over to another section of the program. It has a well-deserved reputation for creating "spaghetti" code that is impossible to read. Since they are rarely necessary, it is generally best to avoid using them.
Nevertheless, the goto statements in C are useful and safe in a few specific situations. A goto statement enables navigation to a named label from any location inside the same function. If it becomes necessary to exit a highly nested structure, such as nested loops, a goto can be helpful in that circumstance. This is impossible for a break statement to accomplish because it can only exit one level at a time.
Controversies Surrounding Goto Statements in C:
The use of goto has been controversial since its inception. The primary argument against it is that it can lead to "spaghetti code" - a disorganized and hard-to-follow code structure. Code that relies heavily on goto statements can be difficult to maintain, understand, and debug.
Structured programming principles discourage the use of unconditional jumps, promoting constructs like loops and conditionals for better readability and maintainability. The presence of goto statements can complicate code reviews and make it harder for developers to reason about the program's behavior.
However, there are situations where judicious use of goto can lead to more efficient and cleaner code, especially in error handling and resource cleanup scenarios.
Syntax of goto statement in C
The syntax of the goto statement in C consists of the goto keyword and the label.
- Goto keyword: In C, it serves as a reserved keyword to denote the use of the goto statement.
- Label: A designation that is assigned to a specific area in the code and is accompanied by a colon (:) is referred to as a label. Control is passed from the goto statement to the labeled statement.
The syntax of the goto statement in C is as follows:
goto label;
//…
Label:
// Code to be executed when control is transferred here
When the goto statement is run, control will be handed to the labeled statement with the identifier "label" as its argument.
It's crucial to remember that the label and goto statements must both be specified in the same function. Additionally, since the goto statement cannot transfer control into another block, the label should not be defined within a block (such as inside a loop or conditional statement).
Flow Diagram for the Syntax of Goto Statement in C
Two styles of goto statements in C
Style 1 - Transferring the control from down to top
In this programming approach, the goto statement is used to move control from a lower to a higher level of code. Before quitting a function or program, this style is frequently used for cleanup or error-handling duties. The purpose of this approach is to make it simple and organized for developers to deal with unusual circumstances or faults. Control can be moved from the area of error or exceptional situation to a higher portion of code, allowing for the completion of any required cleanup work or error-handling activities before the function or program is terminated.
Syntax:
goto label;
// ...
label:
// Code to be executed when control reaches the label
Here:
- goto label; -The goto keyword is followed by a label, indicating the target point in the code where control will be transferred. It allows the program to jump to the specified label, even if it is located above the goto statement.
- label: - Labels are identifiers followed by a colon (:). They mark specific points in the code where control can be transferred using goto.
- The label is followed by the code to be executed when control reaches that point.
Code Example: Error Handling
Output:
ERROR!
Result: 20
Error: Negative number not allowed.
Error occurred. Exiting function.
Explanation:
- The code includes the standard input/output library (#include <stdio.h>).
- Defines a function, performOperation, that takes an integer parameter num and returns void.
- Checks if the input num is negative; if true, prints an error message and jumps to the error label using goto.
- If num is non-negative, performs the operation (multiplies num by 2), prints the result, and returns from the function.
- The error label is a point in the code where execution jumps in case of an error, printing an error message.
- The main function is the program's entry point.
- Declares and initializes two integer variables, num1 with a value of 10 and num2 with a value of -5.
- Calls the performOperation function with the values of num1 and num2 as arguments.
- Returns 0 from the main function, indicating that the program executed successfully.
Style 2 - Transferring the control from top to down
In this programming approach, the goto statement is used to move control from a higher to a lower level of code. This coding approach is frequently employed to implement loops or to exit nested loops. The purpose of this programming style is to provide developers with the ability to jump to a certain location in the code to regulate program flow. It allows for the implementation of jump-based program logic or the ability to exit loops based on particular situations or demands.
Syntax:
label:
// Code to be executed when control reaches the label
// ...
goto label;
Here:
- label:: -A label is declared with an identifier followed by a colon (:). It marks a point in the code where control can be transferred using goto.
- The code following the label is executed when control reaches that point.
- goto label; - The goto keyword is followed by a label, indicating the target point in the code where control will be transferred.
- In this style, the goto statement is typically placed after the label, creating a loop-like structure by jumping back to the labeled point.
Code Example: Jumping to Different Sections of Code
Output:
Menu:
1. Print 'Hello'
2. Print 'World'
3. Print 'Goodbye'
Enter your choice: 2
World!
End of program.
Explanation:
- Includes the standard input/output library (#include <stdio.h>).
- Declares the main function, which serves as the entry point for the program.
- Declares an integer variable choice to store the user's menu selection.
- Prints a menu with three options: "Print 'Hello'", "Print 'World'", and "Print 'Goodbye'".
- Prompts the user to enter their choice and reads the input into the choice variable using scanf.
- Jumps to the label using goto.
- Defines three labels: hello, world, and goodbye, each corresponding to a specific action based on the user's choice.
- Prints "Hello!" if the program reaches the hello label.
- Prints "World!" if the program reaches the world label.
- Prints "Goodbye!" if the program reaches the goodbye label.
- Jumps to the end label, indicating the end of the program.
- Prints "End of program." if the program reaches the end label.
- Returns 0, indicating successful program execution from the main function.
- The label section is a point in the code where execution jumps based on the user's choice using a switch statement.
- The switch statement checks the value of choice and jumps to the corresponding label (hello, world, or goodbye).
- If the user enters an invalid choice, prints "Invalid choice" and jumps to the end label.
This example shows how to build jump statement-based program logic using the goto statement, allowing control to move from a higher to a lower part of code depending on certain circumstances or user selections.
How does the goto statement work in C?
Control may be handed off unconditionally from one section of code to another in C thanks to the ‘goto’ statement. Let's go through the goto statement's operation step by step here:
- The 'goto' statement with the supplied label is encountered by the program.
- The label acts as a landmark for a particular place inside the code.
- After the 'goto' statement has been run, the program's control goes to the statement with the provided label.
- From the labeled statement onward, the program keeps running.
- Because the code between the 'goto' statement and the labeled statement is skipped, the execution flow is changed
Although the 'goto' statement allows for flexibility, it should only be applied in limited circumstances, in order to maintain code readability and avoid the development of convoluted program flows. It is recommended to use 'goto' instructions seldom and to give preference to structured programming techniques wherever possible.
Goto statement in C example
Let's understand the use of goto statements in C with a code example, where we create a menu-driven arithmetic calculator.
Code Example:
Output :
Menu:
- Add
- Subtract
- Multiply
- Divide
Enter your choice: 2
Enter two numbers: 8 4
Result: 4
End of program.
Explanation:
- The program displays a menu with options for addition, subtraction, multiplication, and division.
- It prompts the user to enter a choice and stores it in the variable choice.
- It checks if the user's choice is outside the valid range (1 to 4). If so, it prints an error message and jumps to the end label using goto.
- If the choice is valid, the program proceeds to prompt the user for two numbers (num1 and num2).
- It uses a switch statement to perform the selected operation based on the user's choice.
- If the user chooses division (case 4), it checks for division by zero and prints an error message if necessary, jumping to the end label using goto.
- After performing the operation, the program prints the result.
- Finally, it prints "End of program" and returns 0 to indicate successful execution.
Complexity Analysis:- The code has an O(1) complexity for processing input and performing arithmetic operations, with a worst-case cost of O(log N) for division, where N is the size of the dividend.
When should we use the goto statement in C?
- Breaking out of nested loops: A goto statement can be a useful method to exit numerous layers of nested loops at once in complex nested loops, obviating the need for flags or complicated control structures.
- Error handling: Using a goto statement to move to a common error treatment block enables quick cleaning and error handling when an error occurs deep within a function.
- Resource deallocation: When working with restricted resources like memory or file handles, a goto statement can be used to move to a block of code where resources are appropriately deallocated before the function exits.
- Code reduction: In some circumstances, a goto statement can be used to reduce code duplication or eliminate unnecessary checks. To ensure readability and maintainability of the code, this should be done sparingly and cautiously.
- Bailing out of a section of code: usually the body of a function, in the event of a mistake or other unanticipated occurrence. If the language included exceptions, there wouldn't be any need for this.
- Putting into practice an explicit finite state machine: The current state is represented by whatever block of code is presently being executed. In this situation, a goto statement directly relates to a notion in the problem domain by switching from one state to a certain other one.
Why must the use of the goto statement be avoided?
- Code Readability and Maintainability: The goto statement can produce difficult-to-read, difficult-to-understand, and difficult-to-maintain code. It can result in a non-linear control flow, which makes it more difficult to understand the program's logic. As a result, the code may have flaws and be difficult for other developers to understand and edit.
- Spaghetti Code: When goto instructions are overused, it can result in "spaghetti code," where the program's control flow is muddled and complicated. This increases the likelihood of introducing minor flaws and makes it more difficult to reason about the program's behavior.
- Lack of Structure: Bypassing the standard order of execution, the goto statement permits unstructured control flow. This can result in unpredictable behavior and make the code more difficult to reason about.
- Encourages Bad Practices: The use of goto can promote bad programming practices such as code duplication, a lack of encapsulation, and a disregard for modular design principles. It may make it more difficult to perform updates or improvements and restrict code reuse.
- Challenges in Debugging and Testing: The goto statement's lack of structure can make debugging and testing more challenging. It becomes more difficult to track the execution flow and pinpoint problems.
- Safety and Security Issues: Using goto in systems that are safety- or security-critical might result in risks and vulnerabilities. It can make it more difficult to evaluate if a program is proper, which could result in system failures or security flaws.
Example of the goto statements in C
Code Example: Determine the square root of a positive number
Output:
Enter a positive number: 6
Square root of 6.00 is 2.45
Explanation:
- The program includes the standard input/output library (<stdio.h>) and the math library (<math.h>), which provides the sqrt function for calculating square roots.
- The main function (main) is the entry point of the program.
- The program declares two double variables: number to store the user input and squareRoot to store the calculated square root.
- The input label is defined to allow the program to jump back in case of invalid input.
- The program prompts the user to enter a positive number and reads the input into the number variable using scanf.
- It checks if the entered number is negative. If so, it prints an error message, and using goto, it jumps back to the input label to prompt the user again.
- If the input is valid (non-negative), the program calculates the square root of the entered number using the sqrt function from the math library.
- It then prints the result, displaying both the original number and its square root with two decimal places.
- The program returns 0 to indicate successful execution.
Complexity Analysis: The code is O(1) in terms of both its temporal and spatial complexity.
Advantages and disadvantages of goto statements in C
Here's a table summarizing the advantages and disadvantages of goto statements in C:
Advantages of goto statements in C | Disadvantages of goto statements in C |
---|---|
1. Simplicity: goto can simplify certain control flow situations, such as breaking out of nested loops or handling errors by jumping to a common error-handling section. | 1. Readability: The use of goto can make code less readable and harder to understand. It can create spaghetti code, where the flow of control is difficult to follow. |
2. Efficiency: In some cases, using goto can lead to more efficient code, especially when breaking out of deeply nested loops or avoiding unnecessary conditions. | 2. Unstructured Code: goto can result in unstructured code, making it challenging to maintain and debug. It goes against the principles of structured programming. |
3. Error Handling: It can be useful for error handling, allowing the programmer to jump to a common error handling section when an error condition is encountered. | 3. Scope of Labels: Labels used with goto must be within the same function. Jumping across functions is not allowed, limiting its usefulness in certain situations. |
4. Looping: goto can be used to create loops in situations where the standard loop constructs are not suitable or efficient. | 4. Alternatives Available: In modern C programming, structured constructs like for, while, and do-while loops provide alternatives for creating loops without the need for goto. |
5. Legacy Code: In some legacy code or specific scenarios, the use of goto might be acceptable and even necessary to achieve certain functionalities. | 5. Discouraged Practice: The use of goto is generally discouraged in favor of structured programming constructs, and many coding standards recommend avoiding its use. |
Alternatives to the goto statement in C
- Loops: You can use loop structures like for, while, or do-while loops in place of goto to exit nested loops or control flows. You may regulate the code execution flow and break out of loops when particular criteria are fulfilled by utilizing loop conditions and control variables.
- Conditionals: You may modify the program's flow based on various situations by using conditional statements like if, else, and switch. You can control the program execution control without using unconditional jump statements by using the right conditional expressions.
- Functions: Dividing lines of code into individual functions encourages modularity and reuse. You may contain particular logic and control flow within each function by separating your code into several functions. This enhances the organization of the code and facilitates the analysis of program flow.
- Error Handling Techniques: You can use error handling techniques, such as error codes, exception handling, or structured error handling, to deal with errors or uncommon situations. These methods offer more methodical and dependable approaches to dealing with uncommon circumstances and regulating program normal flow appropriately.
- Control Flags: Control flags or boolean variables can be used to denote certain states or circumstances rather than using goto to jump between parts of code. You can arrange the program's flow by appropriately setting and inspecting these flags.
Conclusion
The goto statement in C remains a controversial topic, with strong arguments both for and against its use. While structured programming principles discourage its use, there are scenarios where judicious use of goto can lead to cleaner and more efficient code. Developers should use it with caution, always prioritizing readability and maintainability. Understanding the potential applications of goto and following best practices can help strike a balance between its advantages and disadvantages in C programming.
Frequently Asked Questions
Q1. What are the three types of loops?
There are generally three types of loops, which are as follows:
- For Loop: When the number of iterations is predetermined, a for loop is used. It is made up of a condition, an increment or decrement statement, and an initialization statement. Up until the condition is false, the loop remains in place.
- While Loop: A while loop is used when it is unknown in advance how many iterations will be made, and the loop runs as long as the condition is met.
- Do-while loop: While a do-while loop is similar to a while loop, the condition is verified after the body of the loop has been executed. By doing this, the loop body will always be run at least once.
Q2. What is the continue label in C?
To go to the next iteration of a loop and skip the remaining iterations, use the continue statement in the C language. It enables you to start the subsequent iteration without running the remaining code inside the loop's body.
To define which loop should be continued, the continue initial statement can also be used with a label. This is called the continue label statement. It enables you to go on to the following iteration of the loop without performing the remaining iterations of an outer loop or a particular labeled loop.
Syntax:
continue label;
The label of the loop that you want to continue is indicated by "label" in the aforementioned syntax. Within the same function or block, the labeled loop may be put wherever you like.
You can select to skip specific iterations of nested loops based on certain conditions by using the continue label statement to manage the execution normal flow.
Q3. What are label statements?
Some programming languages provide a feature called label statements that lets you give a label to a particular line or statement of your code. Label statements are sometimes referred to as labeled statements or target statements. The label serves as a point of reference that can be used to move control from another area of the code to that specific line or sentence.
A label is commonly defined by adding an identifier to the beginning of a line, followed by a colon (:), in languages that support label declarations.
Syntax:
Label:
// Code statement
Q4. Is there a label goto in Python?
No, unlike several other programming languages, such as C and C++, Python does not support labeled statements or contain a goto statement. The flow of execution constructs in Python does not include the goto expression. The goto statement is intentionally absent from Python because it can make code more challenging to read and maintain, particularly in bigger codebases. The goto statement isn't used in Python because of its design philosophy, which prioritizes readability and simplicity of code.
Q5. What is the use of the goto label in C?
The goto statement with a label's main applications in C are as follows:
- Breaking out of nested loops: If you have several nested infinite loops and you need to prematurely depart from each one of them due to a specific circumstance, you can use a goto statement with a label placed after the closing braces of the loops.
- Error handling and cleanup: In difficult situations when you must deal with errors and carry out cleanup procedures before leaving a function or a block, a goto statement with a label might offer a quick way to navigate to a typical error handling or cleanup area of your code.
- Putting finite state machines into practice: finite state machines frequently switch between various states in response to certain circumstances. State machine implementations are more condensed when states may be jumped between using the goto command with labeled statements.
Q6. What is the difference between exit and goto in C?
Criteria |
Exit |
Goto |
Purpose |
Terminates the execution of the program. |
Transfers control to a labeled destination statement. |
Result |
The entire program is affected. |
Performs actions within the current block or function. |
Use of label |
The statement is not marked as a label. |
The statement is marked as "label," and it is followed by a colon. |
Program ending |
Can end the program completely. |
Does not end the program completely. |
Syntax |
|
|
Suggested Reads:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment