Conditional/ If-Else Statements In C Explained With Code Examples
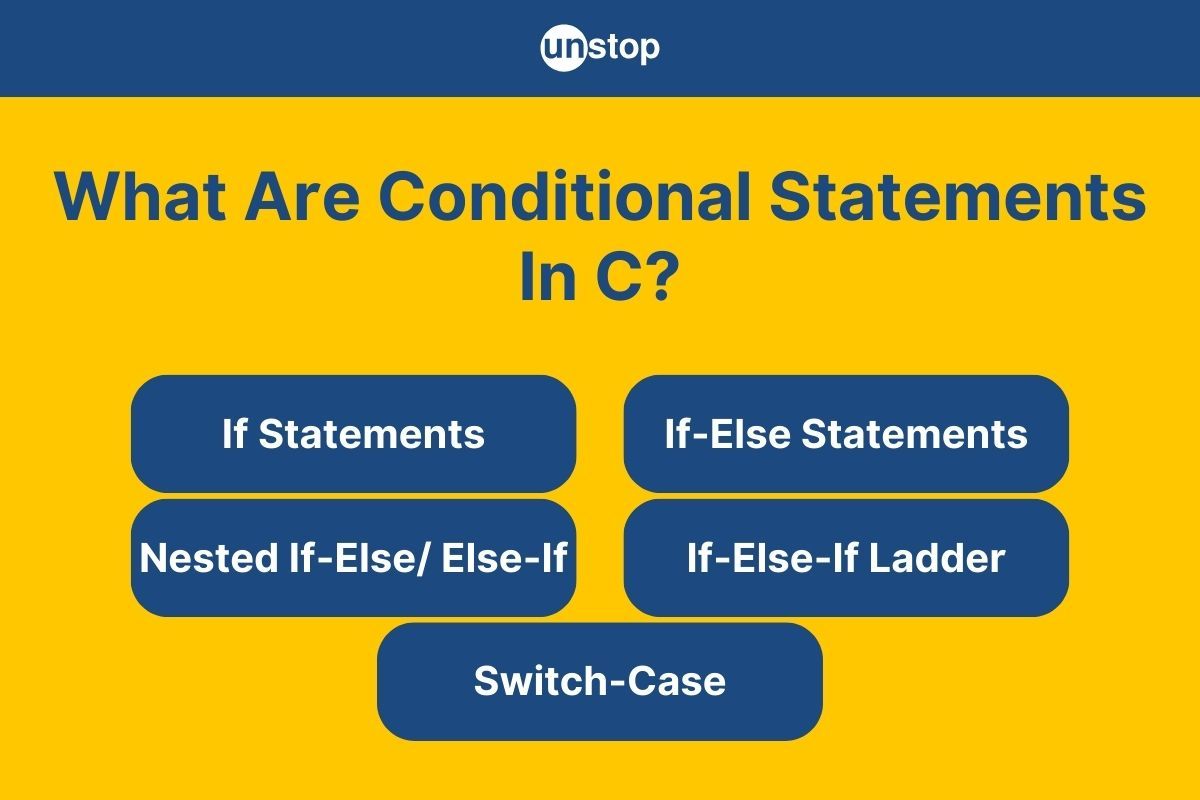
A fundamental building block in programming, if statements (also known as conditional statements in C) enable you to manage the flow of your code depending on certain circumstances. That is, depending on whether a given condition evaluates to true or false, you can run several code blocks.
When writing responsive and dynamic programs, conditional if-else statements in C language are essential since they let you manage a variety of scenarios, user interactions, and data conditions with ease.
What Is A Conditional Statement In C?
A conditional statement in C programming is a construct that enables you to manage the execution flow depending on a given set of circumstances.
- You can first set a condition and specify a block of code (task) that must be executed if that condition is met. That is, you can specify different tasks to perform for different situations.
- So, depending on whether a specific condition evaluates to true or false, a specific action or code block is executed.
- Conditional statements in C enable programmers to manage the execution flow in a program based on specified situations. Because not all tasks in a program can be completed using a linear sequence of instructions.
- It also adds a layer of flexibility to your code by allowing you to make decisions on the go (as per the conditions). In this way, conditional statements offer a potent mechanism to modify the course of C program execution based on particular circumstances.
These statements allow us to deal with changing circumstances by selectively running or skipping specific code blocks. Overall, conditional if-else statements in C increase the flexibility and reactivity of your code by enabling your program to decide what to do next and take different actions accordingly.
Types Of Conditional Statements In C
In C, there are various sorts of conditional statements that let you regulate the program flow based on specific circumstances. These include-
- If Statement
- If-Else Statement
- Nested If-Else Statement
- If-Else-if Statement
- If-Else Ladder Statement
We will discuss each of these conditional if-else statements in C in detail in the sections ahead, with the help of C programs, to show their implementation.
If Conditional Statement In C
An if statement is a control structure to make decisions based on specific circumstances. It establishes a direct relation between the condition being fulfilled and the task to be performed. That is, it enables the program to run a particular code block only when a particular condition is met.
- The statement begins with an expression or condition which, when evaluated, turns a boolean value, i.e., either true or false.
- The code block wrapped in the curly braces will only be run if the condition is true.
- Otherwise, it will be skipped, and the program will move on to the line of code that follows the if statement.
If Statement Syntax:
if (condition) {
// Code to execute if the condition is true
}
Here,
- The if keyword indicates the beginning of the conditional statement.
- The condition refers to the expression that is evaluated to determine whether the conditional code inside the curly braces will be executed.
The Working Mechanism Of If Conditional Statement In C
The if statement flowchart above depicts the working mechanism of this conditional statement in C, which is-
- When the program encounters an if-statement, it evaluates the specified condition within the parentheses.
- The condition is an expression that returns either true (non-zero) or false (zero).
- If the condition is true, the code block enclosed within the curly braces {} is executed. This code block contains the instructions you want to run when the condition is satisfied.
- If the condition is false, the code block is skipped, and the program moves to the next statement after the if-block.
If Statement Example In C: Now, let's take a look at a simple C program example to see the implementation of the simple if-statement.
Code Example:
Output:
The number is positive.
Explanation:
In this simple C program, we begin by including the header file for input/ output operations, i.e., <stdio.h>.
- We then define the main() function, which is the entry point for the program's execution.
- Inside the main, we declare a variable number and initialize it with the value 10.
- Then, we initiate an if statement, where the condition is to check if the value of the number variable is greater than 0, i.e., n > 0.
- If the condition istrue, the code inside the braces is executed. inside, we use the printf() function to display a message to the console. Here, the newline escape sequence shifts the cursor to the next line after printing.
- If the condition isfalse, then the program flow exists the if-statement.
- Since here, the value of number (10) is indeed greater than 0, the condition is true, and the code block within the if statement is executed.
- Finally, the program terminates with a return 0 statement indicating successful execution.
Complexity Analysis: The time complexity of an if statement in terms of the condition evaluation is typically O(1).
If-Else Statement In C
The if-else statement can be seen as an extension of the if conditional statement in C. This statement provides us with the option to execute a code block even if the condition evaluates to false.
- That is, if the condition is true, the code within the if block is executed. And if the condition is false, the code within the else block is executed.
- This statement controls the flow of execution in the program and provides alternatives based on the condition's evaluation.
The if-else statement in C enables you to easily regulate the flow of your program by branching it into distinct paths based on the results of a logical statement. It is a crucial tool for creating conditional behavior and decision-making in a variety of computer tasks.
If-Esle Syntax:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
Here,
- The statement begins with the if keyword and the (condition) expression is evaluated to determine the future course of action. The code in the curly brackets after if is executed in case the condition returns true.
- The else keyword gives the alternative, which comes into effect if the condition's result is false. In this case, the code inside this block of curly braces is executed.
The Working Mechanism Of If-Else Statement In C
The if-else statements in C work similarly to the if-statements but with an added step. Here is the working mechanism of these conditional statements (as also shown in the if-else flowchart above):
- The program begins by analyzing the condition inside the parentheses when it first comes across an if expression.
- The expression/ condition will be evaluated as either true (non-zero) or false (zero).
- The code block included in the curly braces is executed if the condition is true. The instructions you wish to execute when the condition is met are contained in this block of code.
- If the condition is false, the immediate block of code is skipped, and the program moves on to the else block, which is then executed.
If-Else Example: Let's look at a C program example that showcases how the if-else statement is defined and used.
Code Example:
Output:
The number is odd.
Explanation:
In the C code example-
- We declare a variable number of integer data type in the main() function and assign the value of 5 to it.
- Then, as mentioned in the code comments, we initiate an if-else statement, which checks the condition to see if the number is even.
- Here, the modulo operator and the equality relational operator determine if the value of the number is completely divisible by 2. i.e., number % 2 ==0.
- If the condition returns true, then the if-code block is executed, and the printf() function inside prints the string message- The number is even.
- If the condition is false, then the program moves to the else-code block, and the message- The number is odd, is printed.
- In this if-else example, the value of the number variable 5 is not divisible by 2, which is why the else-code block is executed.
- Finally, the program finishes with a return statement 0, indicating error-free execution.
Time Complexity: The time complexity is O(1).
Space Complexity: The space complexity is O(1).
Advantages & Disadvantages Of If-Else Statement In C
The if and if-else statements are two of the most commonly used condition statements. Let's look at some of the advantages and disadvantages of using the if-else statement in C.
Advantages Of If-Else Statement In C
- Decision Making: The if-else statements provide a straightforward way to implement decision-making in a program. They allow the execution of different code blocks based on whether a particular condition is true or false.
- Readability: A well-structured if-else statement in C code can enhance readability. They make it clear which block of code will be executed based on the condition, improving the understanding of the code/ programming logic.
- Flexibility: If-else statement in C offers flexibility in handling multiple conditions. You can also chain multiple if-else statements or use nested if-else statements to handle complex decision structures.
- Ease of Use: The syntax of if-else statement is relatively simple, making it easy to use and understand. This simplicity is especially beneficial for beginners and contributes to the overall maintainability of the code.
- Logical Flow: Using the if-else statement in C helps maintain a logical flow of control in the program. Conditions can be structured to represent the program's logical progression, making it easier to follow.
Disadvantages Of If-Else Statements In C
- Code Duplication: In some cases, the use of if-else statements may lead to code duplication, especially when handling similar conditions in different parts of the program. This can make the code harder to maintain and increase the risk of introducing errors.
- Nested Complexity: Excessive nesting of if-else statements in C programs can lead to complex and hard-to-read code. Deeply nested structures can make it challenging to understand the logic and debug the code effectively.
- Maintenance Challenges: As the number of conditions and branches increases, maintaining if-else statements in C programs can become challenging. Modifying one part of the code may require careful consideration of its impact on other parts of the program.
- Limited Expressiveness: While if-else statements in C are powerful for basic decision-making, more complex scenarios may be better handled with other control structures or by using switch statements. This limitation becomes apparent in scenarios involving multiple conditions.
- Potential for Error: Human error may lead to mistakes in writing conditions or in the logic of the if-else statement in C. This is especially true in complex conditions or when dealing with a large number of conditions.
Nested If-Else Statement In C
When we stack one conditional statement inside another conditional statement, it is known as a nested statement.
- So, a nested if-else statement is when one if or if-else statement is contained inside of another.
- These nested conditional statements in C enable more complex decision-making by assessing a number of conditions and running various blocks of code in response to the findings.
- This is how a nested if-else statement allows for the conditional execution of code based on multiple conditions.
Depending on how complicated the circumstances are, nested if-else statements can be expanded to numerous levels. However, note that it is important to maintain the code's structure and readability to prevent confusion or undefined behaviour.
Nested If-Else Syntax:
if (condition 1) {
// If-code 1 to execute if condition1 is true
if (condition 2) {
// If-code 2 to execute when both condition1 & condition2 are true
} else {
// Else-code 1 to execute if condition1 is true but condition2 is false
}
} else {
// Else-code 2 to execute if condition1 is false
}
Here,
- Condition 1 and Condition 2 are the conditions we are checking to be true or false for the inner and outer if-else statements, respectively.
- If condition 1 is true, if-code 1 will be executed, and if-code 2 will be implemented if both conditions are true.
- Else-code 1 is what is executed when condition 1 is true but condition 2 is false.
- And Else-code 2 is what is executed when condition 1 returns false.
Working Mechanism Of Nested If-Else Conditional Statement In C
Here is the working mechanism of the nested conditional statement in C is as follows:
- If (condition 1) marks the beginning of the nested if-else statement. This is the main conditional statement, also referred to as the outer if-else statement.
- In case the outer condition is false, then the code inside the curly braces following the outer else block is executed.
- If the outer if-condition is true, then the flow of the program moves to the if-else statement nested inside the curly brackets inside the outer statement.
- The program then moves to analyze the inner if-condition (i.e., condition 2), and based on that, the further course of execution is determined.
- If the second if-condition (condition2) is true within the context of the first condition being true, the code inside the nested if block is executed, i.e., if block 2.
- Otherwise, if condition 2 is false within the context of condition 1 being true, the code inside the nested else block is executed, i.e., else block 2.
Look at the example C program below to understand the implementation of this conditional statement in C.
Code Example:
Output:
You are eligible for the ride.
Explanation:
We begin the example C code above by including the essential headers and then define the main() function.
- We declare two integer variables, age and height, and initialize them with the values 40 and 185, respectively.
- Next, we create a nested if-else statement to check the eligibility of the rider based on their age and height.
- The outer if-condition checks if the age of the person is greater than equal to 18.
- If the condition is false, we move to the outer else block, which prints a message- Sorry, you must be at least 18 years old to go on the ride.
- If the condition is true, we move to the inner if-statement, where the condition checks if the height is greater than or equal to 160.
- If the inner condition also returns true, then the if-block is executed. It contains a printf() function which displays the message- You are eligible for the ride.
- In case the inner if-condition is false (i.e., height is less than 160), we move to the inner else-block. It prints the message- Sorry, you must be taller to go on the ride.
- In this example, the values assigned to both the variables result in true for both conditions. As a result, the inner else block is executed, and the corresponding message is printed to the console.
- Lastly, the program has a return statement of 0, indicating that the main() function has been completed and the program exits. The value 0 is commonly used to indicate a successful execution of the program.
Time Complexity: The time complexity of a program refers to the amount of time it takes to run based on the input size. In this specific program, there are no loops or recursive functions, so the time complexity is constant (O(1)).
Space Complexity: The space complexity of a program refers to the amount of memory space required by the program to run based on the input size. In this program, the space complexity is also constant (O(1)).
If-Else-If Ladder Conditional Statement In C
The if-else-if ladder, also known as a chain of if-else statements, allows you to evaluate multiple conditions sequentially and execute the corresponding code block based on the first condition that evaluates to true. Each condition is evaluated in order until a true condition is found or until the last else statement is reached.
In short, the program checks each condition one by one, and if a condition is true, the associated code block is executed. If none of the conditions are true, the code block within the final else statement is executed.
If-else-if Syntax:
if (condition 1) {
// If-code 1 to execute if condition1 is true
}else if (condition 2) {
// If-code 2 to execute if condition 2 is true and condition1 is false
}else if (condition 3) {
// If-code 3 to execute if condition 3 is true and both condition1 and condition2 are false
}else {
// Else-code to execute if all conditions are false
}
Here,
- Condition 1, condition 2, and condition 3 refer to the conditions we are testing to be true or false.
- The codes in the curly brackets after every statement refer to the block of statements that will be executed if the respective condition is true, conditional upon the previous condition being false.
- The else {code} refers to the code that will be executed if all of the initial conditions are false.
The Working Mechanism Of If-Else-If Ladder Statement In C
Here is a step-by-step explanation of the working mechanism of this conditional statement in C-
- The first if-condition (condition 1) is evaluated.
- If condition1 is true, the block of statements inside the curly brackets associated with condition1 is executed, and the rest of the ladder is skipped.
- If condition 1 is false, the next condition (condition 2) is evaluated.
- If condition 2 is true, the code block associated with condition 2 is executed, and the rest of the ladder is skipped.
- This process continues for each subsequent condition until a true condition is found or until the last else statement is reached.
- If all conditions are false, the code block associated with the else statement is executed.
Look at the C program sample below to understand how this conditional statement is implemented inside the code.
Code Example:
Output:
Tuesday
Explanation:
In the C code sample-
- We begin the example by initializing an integer variable day with the value 3 inside the main() function.
- Then, we initiate an if-else-if ladder statement to determine the day of the week based on the value of the day. Here-
- The first condition checks if the day is equal to 1. If the condition is true, then the printf() statement inside the if-block prints the message- Sunday to the console.
- If the 1st condition is false, the program moves to the next else if condition. The next if-condition checks if the value of the day is equal to 2.
- If it is true, the if-block is executed, and the rest of the statement is skipped. If the condition is false, then the program moves to the next else-if ladder block.
- The program repeats this process for each day of the week, checking for values from 3 to 7.
- If all the conditions are false (i.e., day is not 1 to 7), the program prints the default message- Invalid day.
- In this example, the value assigned to the variable day is 3. As a result, the 3rd if-block is executed, and the word Tuesday is printed to the console.
- Finally, the program terminates with a return 0 statement.
Time Complexity: The time complexity is constant, or O(1), as the number of comparisons and executions does not depend on the input size.
Space Complexity: The space complexity is O(1).
Nested Else-If Statement In C
Earlier, we discussed nested if-else statements, where one if-else statement was nested inside the other. In that case, the inner if-else statement was nested inside the if-block of the outer statement, and so on. And this is most commonly used concept.
- But you might come across a nested else-if statements, where the inner if-else statement is nested inside the else-code block.
- These conditional statements in C may also be referred to as else-if ladder or cascading if-else statements.
Just like nested if-else statements, these conditional statements in C also offer a way to handle multiple conditional branches within conditional statements. They provide a hierarchical structure for evaluating multiple conditions and executing corresponding blocks of code based on the results.
As shown in the flowchart, with nested else-if statements, you can create a series of condition checks that are executed sequentially. Each condition is evaluated one by one, and the corresponding block of code associated with the first matching condition is executed. If none of the conditions match, an optional else block can be used to provide a default action.
Nested else-if statement Syntax:
if (condition1) {
// Code to execute if condition1 is true
}else if (condition2) {
// Code to execute if condition1 is false and condition2 is true
}else if (condition3) {
// Code to execute if both condition1 and condition2 are false, and condition3 is true
}
// ...
else {
// Code to execute if all conditions are false
}
As is evident from the syntax, the nested else-if statements are similar to the ladder if-else-if statements. Let's take a look at a sample C program to illustrate the use of this statement type.
Code Example:
Output:
Grade: C
Explanation:
- We begin the sample C code by including the standard input-output library (<stdio.h>) and then initialize an integer variable mark with the value of 70 inside the main() function.
- Then, we create a nested else-if statement to determine the grade based on the value of the mark variable-
- The program checks the first if-condition, i.e., if the mark is greater than or equal to 90. If this is true, then the printf() function inside the if-block prints- Grade: A.
- If the condition is false, the value of mark is not greater than or equal to 90, the program moves to the next else if condition.
- The program repeats this process for each grade, checking for values from 80 to 60.
- If all the conditions are false (i.e., the mark is less than 60), the program prints- Grade: F.
- In this example, the value of the grade variable is 70, so the third if-block prints the message- Grade: C to the console.
Time Complexity: The time complexity remains constant, or O(1), as the number of operations executed remains the same regardless of the input value.
Space Complexity: The space complexity is O(1).
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Need of Conditional Statements In C
Programmers generally make decisions by evaluating conditions and then running various pieces of code in response to the results of those assessments. Conditional control statements are quite important in this context as they help make software more dynamic and responsive.
The following describes the necessity of conditional statements in C programs-
- Making decisions: Conditional statements let programmers decide what to do in response to certain circumstances. For instance, certain features of a program may be accessible if a user is signed in, whereas such functions may be restricted if the user is not. The program can modify its behavior in response to changes in the system state or user input by using conditional/ if-else statements in C.
- Code branching: These statements make it possible to branch code execution. This means that the program can take alternative turns and carry out various sets of instructions depending on how a logical condition turns out. This branching feature is crucial for managing dynamic scenarios and creating diverse behaviors based on specific conditions.
- Input validation: Conditional statements in C are frequently used to authenticate user input or verify the accuracy of data. If a user is asked to enter a number, for instance, a conditional/ if-else statement can be used to determine whether the input is legitimate and to take the proper action if it is not.
- Loop Termination: Loop termination is governed by conditional if-else statements in C programs. By assessing a condition at the start or end of a loop, the program can choose whether to keep iterating or exit the loop entirely. This enables effective code repetition until a particular condition is met.
Switch Conditional Statements In C
The if-statements/ conditional statements in C we have discussed so far are one of the types of control statements. The control statement, as the name suggests, helps control the flow of program execution to meet the needs of the programmer and the project at hand.
One other such conditional statement that is closely related to the if-statements is the switch-case statement. It allows us to switch from one segment of the code to another based on specified conditions.
- The program can guide execution to certain code segments by evaluating the switch expression and comparing it to various constants.
- When it matches a certain case, the program control jumps to that block. This is why they are also referred to as jump statements.
- The switch block is terminated when the break statement is encountered or when the optional default case acts as a fallback when no specific case matches.
The switch statement serves as an alternative to nested if-else statements when dealing with a discrete set of possible values for an expression.
Switch Case Statement Syntax:
switch (expression) {
case label 1:
// Code to execute when expression matches label 1
break;
case label 2:
// Code to execute when expression matches label 2
break;
// ...
default:
// Code to execute when the expression does not match any case
break;}
Here,
- The expression refers to the main condition that will be compared against the other labels or cases.
- The case label is a common keyword that marks the values against which the expression is compared. These values can be constants, integers, etc.
- The codes of blocks inside the curly brackets are executed if the respective case label matches the switch expression.
- The break keyword marks the break statement, signalling the program to break execution and exit the statement.
- The default keyword marks the default case label code to be executed if none of the other cases matches the switch expression.
Now, let's take a look at an example of the switch-case statement in C to understand how the statements really work.
Code Example:
Output:
Menu:
Option 1
Option 2
Option 3
Enter your choice: 1
You selected Option 1.
Explanation:
- Inside the main() function, we declare an integer variable named choice to store the user's menu selection.
- Then, using a series of printf() statements, we display the menu options to the user.
- We then use another printf() statement to prompt the user to feed in their choice, which is read using the scanf() function and store it in the choice variable.
- Next, we create a switch-case statement to compare the user-input choice against menu options. Here-
- If the value of choice is 1, it matches case 1 and the program prints- You selected Option 1.
- If the value of choice is 2, it matches case 2, and the program prints- You selected Option 2.
- If the value of choice is 3, it matches case 3, and the program prints- You selected Option 3.
- If none of the above cases match (i.e., the value of choice is not 1, 2, or 3), the default case is executed, and the program prints- Invalid choice.
- Each case is followed by a break statement, which exits the switch statement.
- In this example, the user-input provided is 1, which si why the coe block following case 1 is executed and corresponding message is printed to the console.
- Finally, the program terminates with a return 0 statement.
In summary, this program displays a menu of options, prompts the user to make a choice, and then uses a switch statement to determine the user's selection and provide the corresponding output.
Read for more: Jump Statement In C | Types, Best Practices & More (+Examples)
Relational Operators In C
Relational operators are often used in the conditional expression of the conditional statements in C as well as other programming languages.
- We use relational operators, such as <, >, <=, >=, ==, and !=, to evaluate the relationship between two values.
- This evaluation returns a Boolean value (true or false) based on the comparison result.
- Relational operators are closely related to conditional statements, such as if statements and loop control statements.
The outcome of a relational operator comparison determines whether the condition is true or false, and the subsequent code block or statement is executed accordingly.
Code Example:
Output:
a1 is greater than b1
a1 is greater than or equal to b1
a1 is not equal to b1
Explanation:
- In the main() function, we initialize two integer variables, a and b, with values 10 and 5, respectively.
- We then use a series of if-statements with different relational operators to compare the values of the variables.
- Depending upon the outcome of the if-conditions inside these statements, the printf() statement contained in the if-code block is executed, and the corresponding message is printed to the console.
- The main function ends, and the program returns 0 to the operating system, indicating successful completion.
Time Complexity: Since the number of if statements is fixed and does not depend on the input size, the time complexity remains constant, or O(1).
Space Complexity: The code only uses a fixed amount of memory to store the integer variables a and b. It does not dynamically allocate any additional memory or scale with the input size. Therefore, the space complexity is O(1).
For more, read: 6 Relational Operators In C & Precedence Explained (+Examples)
Conditional Expressions: An Alternative To Conditional Statements In C
A discussion on conditional statements in C is incomplete without the mention of the conditional/ ternary operator. This operator lets you pick between two values or expressions based on a condition.
They offer a succinct approach to expressing straightforward conditional statements in a single line of code. That is, a single statement can sometimes meet the same purpose as a conditional statement.
Basic Syntax:
condition ? expression_if_true : expression_if_false;
Here,
- The condition is an expression that evaluates to either true or false.
- If the condition is true, expression1 is executed and becomes the result of the entire conditional expression.
- If the condition is false, expression 2 is executed and becomes the result.
Code Example:
Output:
The maximum value is: 11
Explanation:
- We begin the main() function by declaring three variables, i.e., a, b, and max. Of these, we initialize a and b with the values 11 and 6, respectively.
- Then, we initialize max using the ternary/ conditional operator. Here-
- The condition is to check if a is greater than b (i.e., a>b), and if the condition is true, assign the value of a to max.
- If the condition a > b is false, assign the value of b to max.
- In other words, the result of the ternary expression is assigned to the variable max.
- We then use a printf() function to display the calculated maximum value. We use a formatted string with the format specifier %d to represent the integer value.
Time Complexity: The code consists of a single conditional expression (a > b) ? a : b. The evaluation of the conditional expression takes constant time, regardless of the input size. Therefore, the time complexity is constant, or O(1).
Space Complexity: The code uses a fixed amount of memory to store the integer variables a, b, and max. It does not dynamically allocate any additional memory or scale with the input size. Hence, the space complexity is O(1).
Conclusion
Conditional statements in C allow you to control the flow of a program based on certain conditions. They include the if statement, if-else statement, nested if-else statement, and if-else-if ladder.
- The if statement executes a block of code if a condition is true. Or else the program exits the statement.
- It can be extended with the else clause to handle the case when the condition is false. This is referred to as the if-else statement in C.
- The nested if-else statement allows for the nesting of if-else statements within another if or else block, enabling more complex decision-making.
- The if-else-if ladder structure is used when there are multiple conditions to be evaluated sequentially. It provides a series of if and else-if statements to choose from among multiple options.
- The switch statement evaluates an expression and matches it with different cases, providing an alternative to using multiple if-else statements.
- Relational operators and relational expression play a crucial role in conditional statements by allowing comparisons and determining the truth or falsity of conditions.
By using conditional statements effectively, you can control program behavior, execute specific code blocks, and handle different scenarios based on various conditions, resulting in more robust and flexible programs.
Frequently Asked Questions
Q. What is called an if-else statement in C?
In C programming, an if-else statement is a control statement that allows for conditional execution of code. It provides the ability to specify different blocks of code to be executed based on the evaluation of a condition.
The syntax of the if-else statement in C is as follows:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
In this syntax, the condition is an expression that is evaluated to be either true or false. If the condition is true, the code block within the first set of curly braces is executed. If the condition is false, the code block within the second set of curly braces (after the else keyword) is executed.
The if-else statement provides a way to handle mutually exclusive scenarios, allowing different actions to be taken based on the outcome of the condition evaluation.
Q. What is an example of a conditional statement in programming?
The condition statement example is an if-else statement in the C programming language. Programmers use the if-else statement as a fundamental control structure to decide which blocks of code to run in response to a given situation. It gives your program the ability to decide amongst many options based on whether the provided condition is true or not.
Code Example:
Output:
The number is positive.
Explanation:
- In this code, we have a variable num initialized with the value 10. The if-else statement is used to check whether the value of num is positive or not.
- If the condition num > 0 is evaluated as true, meaning that the num is greater than 0, the code block within the first set of curly braces is executed, and it prints the number as positive.
- If the condition num > 0 evaluates to false, indicating that `num` is not greater than 0, the code block within the second set of curly braces (the else block) is executed, and it prints. The number is not positive.
In this example, since num is 10, which is indeed greater than 0, the output will be The number is positive.
Q. What is the difference between conditional and if-else?
The if-else statement is a type of conditional statement in C. However, it does not describe every type of conditional statement. The table below lists the primary differences between these two terms:
Aspect |
Conditional |
If-Else Statement |
Definition |
A general term referring to any construct based on conditions. |
A specific type of conditional construct. |
Purpose |
Enables making decisions in code based on conditions |
Controls flow by executing different code blocks. |
Execution |
Executes code block(s) if the condition is true. |
Executes one code block if true and another if false. |
Conditions |
Can involve multiple conditions, logical operators, etc. |
Involves a single condition often based on true/false |
Code Blocks |
May involve multiple code blocks. |
Involves a primary block and an optional else block. |
Alternative Actions |
Does not always require an alternative action. |
Provides an alternative action for false condition. |
Flexibility |
More versatile due to various types and combinations. |
Limited to true/false branching with if-else. |
Use Cases |
Suitable for a wide range of decision-making scenarios. |
Effective for binary choices or alternate outcomes. |
Q. What are the types of conditional operators in C?
Generally, the term conditional operator is used to refer to the ternary operator, which provides a compact way of writing if-statements in a single line. However, there are multiple other operators that we use inside the conditions/ expressions of conditional statements.
Collectively, these could also be sometimes referred to as conditional operators since they help determine the result of conditional expressions. There are various sorts of conditional operators available in C programming that can be applied to judgment calls and comparisons. The most popular conditional operators in C are shown below:
Comparison Operators:
Operator |
Description |
== |
Equal to |
!= |
Not equal to |
> |
Greater than |
< |
Less than |
>= |
Greater than or equal to |
<= |
Less than or equal to |
Operator |
Description |
&& |
AND (returns true if both operands are true) |
|| |
OR (returns true if either operand is true) |
! |
NOT (negates the boolean value) |
With the help of these conditional operators, judgments can be made based on the evaluation of conditions and the creation of conditions. In C programming, they are frequently used in if default statements, while loops, for loops, and other control structures. These operators and conditions can be combined to produce strong decision-making logic in your programs.
Q. What is the condition in an if-else statement?
The condition in an if-else construct statement is an expression or a condition that is evaluated to be either true or false. It is used to determine which code block should be executed based on the result of the evaluation. The condition can be any expression that returns a boolean value (true or false). Typically, it involves the use of relational operators or logical operators to compare values or combine multiple conditions.
Here's the general syntax of an if-else statement:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
The condition in an if-else statement allows you to control the flow of execution based on the outcome of the evaluation, enabling different code paths to be taken depending on the condition's result.
Q. Is it possible to do a nesting of conditional statements?
Yes, it is possible to nest conditional statements, including ternary operators. Nesting means placing one conditional statement inside another. This allows you to create more complex decision-making statements. However, it's crucial to maintain code readability to avoid confusion, which is why one must proceed with caution when nesting conditional statements in C.
Here's an example of nested ternary operators in C:
Output:
x is greater than y
Explanation:
In this C example, the result variable is assigned a string based on the comparisons between x and y. The %s format specifier is used to print the string.
As mentioned earlier, while ternary operators can be handy for concise conditional expressions, nesting them extensively may reduce code readability. In practice, it's often more readable to use if-else statements for complex conditions.
Q. What does a ternary operator mean?
A ternary operator, also known as the conditional operator, takes three operands and evaluates a boolean expression. The syntax of the ternary operator is:
Syntax:
condition ? expression_if_true : expression_if_false;
Here's how it works:
- The condition is evaluated.
- If it is true, the value of the entire expression is the result of expression_if_true.
- If the condition is false, the value is the result of expression_if_false.
This operator is a shorthand way of expressing an if-else conditional statement in C in a single line of code.
You might also be interested in reading the following:
- Identifiers In C | Rules For Definition, Types, And More!
- Tokens In C | A Complete Guide To 7 Token Types (With Examples)
- 5 Types Of Literals In C & More Explained With Examples!
- Increment And Decrement Operators In C With Precedence (+Examples)
- Difference Between C And C++ Explained With Code Example
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment