6 Relational Operators In C & Precedence Explained (+Examples)
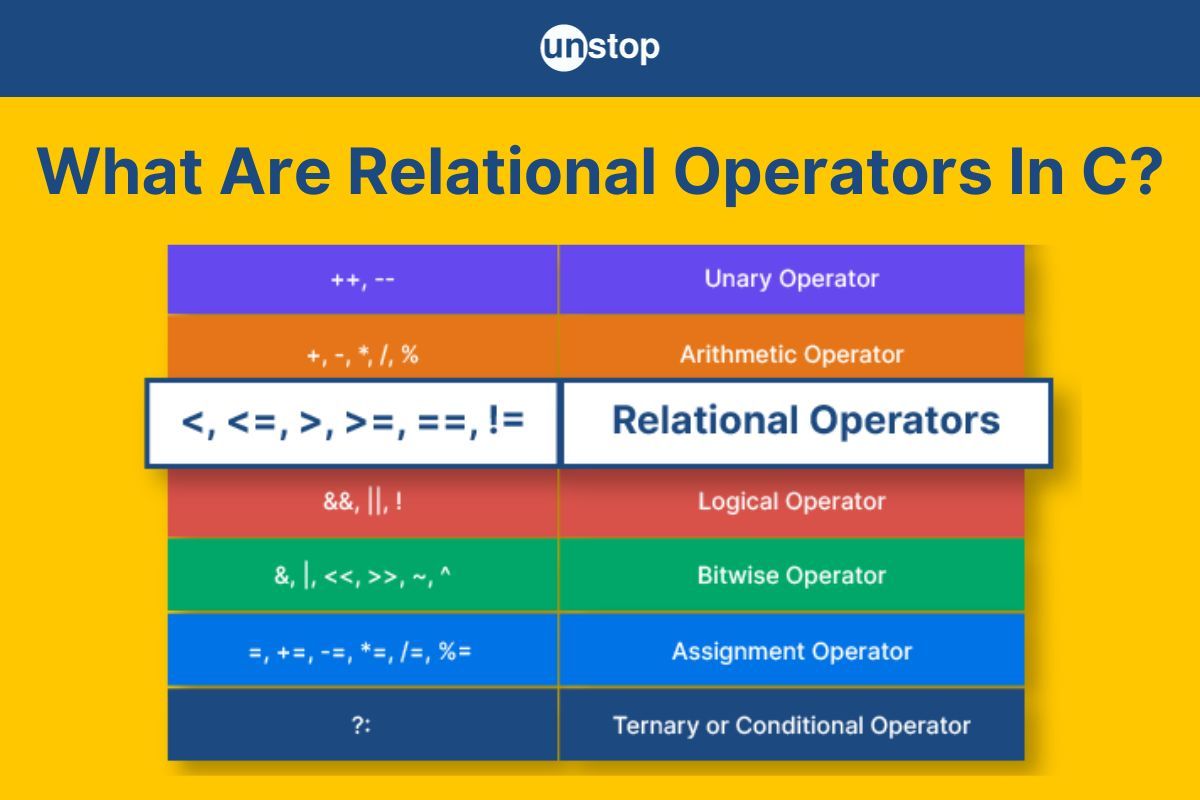
Operators are symbols or special characters that perform specific operations on one or more operands. These operands can be variables, constants, or expressions. There are multiple types of operators, such as arithmetic operators, logical operators, ternary operators, and relational operators in C. These operators enable various computations and manipulations of data within C programs.
Understanding and utilizing these operators effectively is essential for writing efficient and expressive C code. They enable complex computations, comparisons, and control flow within programs, allowing developers to manipulate data in a variety of ways. In this article, we will focus our discussion on relational operators in the C programming language, their purpose, types, and more.
What Are Relational Operators In C?
Relational operators in C language are symbols that are used to compare two values and determine the relationship between them. Also known as evaluation operators, they are binary operators, i.e., they compare two operands and always return the output in Boolean type, i.e., true or false. These operators are commonly used in conditional statements and loops to control program flow.
- Relational operators are essential in decision-making processes in programming languages.
- They allow you to create logical expressions that can be evaluated as true or false, which can be used to control the flow of your program.
- It is important to note that relational operators in C are case-sensitive and cannot be used to compare strings.
- Also, their usage can vary depending on the data type of the operands being compared.
In short, understanding the use and functionality of relational operators is an essential part of becoming proficient in C programming. We can perform powerful comparisons and make more informed decisions in their code by utilizing these binary operators.
Table Of Relational Operators In C
Operator Symbol |
Operator Name |
Description |
Relational Operator Examples |
== |
Equal to |
It checks whether the values of two operands are equal. If they are equal, then it returns true; if not, then false. |
if (x == y) |
!= |
Not equal to |
It checks whether the values of two operands are not equal. If not equal, then returns true; if not, then false. |
if (x != y) |
> |
Greater than |
It checks whether the left operand is greater than the right operand. If greater than, then returns true; otherwise, it returns false. |
if (x > y) |
< |
Less than |
It checks whether the left operand is less than the right operand. If less than, then it returns true; otherwise, it returns false. |
if (x < y) |
>= |
Greater than or equal to |
It checks whether the left operand is greater than or equal to the right operand. If greater than or equal, then it returns true; otherwise, it returns false. |
if (x >= y) |
<= |
Less than or equal to |
It checks whether the left operand is less than or equal to the right operand. If less than or equal, then it returns true; otherwise, it returns false. |
if (x <= y) |
How Do Relational Operators in C Work?
As mentioned before, relational operators compare two operands and infer their relationship as per the given condition. The working mechanism of relational operators is depicted in the flow diagram below.
Here is how one can go about using relational operators in their C programs-
- The first step is to enter the values of the operands to be compared.
- Next, the relational operator is applied between the operands, and the values are compared.
- The result is a Boolean value that is either true or false, depending on the outcome of the comparison.
- A programmer can then use this result in a conditional statement or other control structure to perform further operations.
- For example, if the result is true, the program can execute one block of code, and if the result is false, it can execute a different block of code.
Types Of Relational Operators In C
Let us discuss different types of relational operators in C language through examples and code implementation.
Equal To Relational Operator In C ( == )
The equal to operator is used to compare two values or expressions and check if they are equal. It can also be referred to as the equality operator and is denoted by two equal signs (==). The syntax for this relational operator is-
A==B
Now, let's look at an example where we want to check if two variables, x and y, are equal or not.
Sample Code:
Output:
a == b: 0
a == c: 1
Code Explanation:
We begin the C program above by including the essential header file <stdio.h> for input/output operations.
- Then, we define the main() function, which serves as the entry point of the program.
- Inside the main, we declare three integer variables, a, b, and c, and assign them the values 5, 7, and 5, respectively.
- Next, as mentioned in code comments, we use the equal to operator in expression a==b to compare a and b and the printf() function to print the comparison result.
- We also use the %d format specifier to specify that the value must be replaced with an integer and the newline escape sequence to shift the cursor to the next line once the output is printed. The output is a == b: 0 because 5 is not equal to 7.
- Similarly, we compare a to c and print the result of the comparison, which is a == c: 1 since 5 is equal to 5.
- The program returns 0 at the end of the main function to indicate successful execution and ends with a closing curly brace marking the end of the main function.
Not Equal To Relational Operator In C ( != )
The not equal to operator is used to compare two values or expressions to check if they are not equal. In other words, this relational operator checks for inequality between the two operands. It is denoted by an exclamation mark followed by an equal sign (!=). The syntax for this operator is thus as follows-
A!=B
For example, we want to check if two variables, say, a and b, are not equal. Then, the following simple C program example shows how to make this comparison.
Sample Code:
Output:
a != b: 1
a != c: 0
Code Explanation:
This example C program is similar to the one for equal to, with the same variables and values. The only difference is here, we use the not equal to operator.
- There are three integer variables, a, b, and c, with values 5, 7, and 5, respectively.
- We use the not equal to operator inside the printf() function to compare a to b and print the comparison result. The output is a != b: 1 because 5 is not equal to 7, and the != operator checks for inequality, resulting in a true condition (1).
- Similarly, we use the relational operator in the expression a!=c and the printf() function to compare and display the result. The output is a != c: 0 since 5 is equal to 5, and the != operator checks for inequality and returns false, i.e. 0.
- The program culminates execution with a return 0 statement.
Greater Than Relational Operator In C (>)
The greater than operator is used to compare two values or expressions to check if the first value is greater than the second value. It is denoted by a greater than sign (>). The syntax for this relational operator is-
A>B
For example, say you want to check whether a variable x is greater than a variable y. Then, the C program for this comparison will be given below.
Sample Code:
Output:
a > b: 0
b > a: 1
Code Explanation:
We begin the example C program by including the necessary headers and defining the main() function.
- Inside the main, we define two integer variables, a and b, and initialize them with values 5 and 7, respectively.
- Then, we use the greater than relational operator inside the printf() function to compare a and b and print the comparison result. The output is a > b: 0 because 5 is not greater than 7, and the > operator checks for greater-than condition, resulting in a false condition (0).
- Similarly, we compare b to a and print the comparison result, i.e., b > a: 1 since 7 is greater than 5, and the > operator checks for greater-than condition, resulting in a true condition (1).
Check out this amazing course to become the best version of the C programmer you can be.
Less Than Relational Operator In C (<)
The less than operator compares two values or expressions to check if the first value is less than the second value. In other words, it checks the less than condition and is denoted by a less than sign (<). The syntax for this, where A and B are the two operands, is-
A<B
For example, if we want to check if variable a is less than variable b, the C program sample below shows how it can be done.
Sample Code:
Output:
a < b: 1
b < a: 0
Code Explanation:
Like the example for the greater than relational operator, here we include the essential header and define the main() function.
- Inside, we declare two integer variables, a and b, and initialize them with the values of 5 and 7, respectively.
- Next, we use the less than relational operator in expression a<b and use the printf() function to print the comparison result. It prints a < b: 1 because 5 is less than 7, and the < operator checks for a less-than condition, resulting in a true condition (1).
- Similarly, we compare b<a and print the result, which is b < a: 0 since 7 is not less than 5, and the < operator checks for a less-than condition, resulting in a false condition (0).
- The program finally completes the execution with a return 0, indicating successful completion.
Greater Than Or Equal To Relational Operator In C (>=)
The greater than or equal to operator is used to compare two values or expressions to check if the first value is greater than or equal to the second value. It is denoted by a greater than sign followed by an equal sign (>=). The syntax is-
A>=B
For example, we want to check if a variable x is greater than or equal to a variable y. The sample C program given ahead, illustrates this comparison.
Sample Code:
Output Window:
a >= b: 0
a >= c: 1
Code Explanation:
We begin the program by including the <stdio.h> header and define the int main() function.
- Inside the main, we declare three integer variables, a, b, and c, and assign values 5, 7, and 5 to them, respectively.
- Next, we use the greater than equal to operator in the expression a>=b to compare a and b. We also use the printf() function to display the result, which is a >= b: 0 because 5 is not greater than or equal to 7, and the >= operator checks for greater than or equal to condition, resulting in a false condition (0).
- Similarly, we compare a to c inside printf() to get the result, i.e., a >= c: 1 since 5 is greater than or equal to 5, and the >= operator checks for greater than or equal to condition, resulting in a true condition (1).
- The program finished execution successfully with a return 0, signifying no errors.
Less Than Or Equal To Relational Operator In C ( <= )
The less than or equal to operator is used to compare two values or expressions to check if the first value is less than or equal to the second or not. The syntax to be used to conduct this comparison is-
A<=B
For example, say we want to check if variable a is less than or equal to a variable b. The comparison condition will be a<=b, and the code will be like the example C code below.
Code Snippet:
Output Window:
a <= b: 1
a <= c: 1
Code Explanation:
We begin the C code example by including the stdio.h header file.
- Next, we define the main() function, inside which we declare three integer variables, a, b, and c, and assign them values of 5, 7, and 5, respectively.
- Then, we use the less than equal to operator to compare a and b and print the output using the printf() function. Here, a is the left-side operand, and b is the right-side operand.
- The result of this comparison is a <= b: 1 because 5 is less than or equal to 7, and the <= operator checks for less than or equal to condition, resulting in a true condition (1).
- Similarly, we compare a to c, and since the operand values are 5 for both and the <= operator checks for less or equal to condition, the operator returns true (1), i.e., a<=c:1.
- After both the results are printed to the console, the program terminates execution successfully (i.e., return 0).
Hone your coding skills with the 100-Day Coding Sprint at Unstop and claim bragging rights now!
Relational Operator Precedence and Relationship With Other Operators
The precedence of operators plays a vital role in determining the order in which operations must be carried out. In general, relational operators in C have higher precedence than most other operators, except for arithmetic and shift right/ left operators. This means relational operators are evaluated or applied before other operators (with lower precedence) in an expression. The precedence table of operators in C lists the precedence of these operators amongst them. Look at the image below for the image to understand the precedence value of different operators present in C.
Consider the example that highlights what precedence between operators means. Say we have three variables, i.e., a, b, and c. Then, for the expression a > b + c, the addition arithmetic operator (+) will be evaluated first, followed by the relational operator greater than (>). This is because the addition operator has a higher precedence value than the relational operator.
It's important to note that the logical operators (&&, ||, and !) have lower precedence than the relational operators. This means that relational expressions are evaluated before logical expressions in an expression. For example, in the expression a && b > c, the logical operator (&&) is evaluated after the relational operator (>).
Besides knowing the precedence of relational operators compared to other operator types, we must also consider precedence within the six relational operators in C. The precedence table for the same is given below, with relational operators listed from highest to lowest in terms of precedence/ applicable importance.
Relational Operator In C | Symbol |
Less than | < |
Less than or equal to | <= |
Greater than | > |
Greater than or equal to | >= |
Equal to | == |
Not equal to | != |
In addition to precedence, relational operators also have a relationship with other operators in C. For example, they are often used in combination with arithmetic operators (+, -, *, /, and %) to perform complex mathematical operations. They can also be used in control/ conditional statements (if, else, if, switch) to compare values and control program flow.
Overall, the relationship between relational operators and other operators in C is complex, and understanding their precedence and interaction with other operators is critical for writing efficient and effective code. By mastering these concepts, programmers can write better code that performs complex operations quickly and accurately.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Other Operators Vs. Relational Operators In C
Some points highlighting the differences between relational operators and other operators in c language are-
Purpose:
Relational Operators: Relational operators are used to compare values or expressions and determine the relationship between them. They evaluate the equality, inequality, and ordering of operands. So they are also known as comparison operators.
Other Operators: Other operators in C perform various tasks such as arithmetic calculations, logical operations, bitwise operations, assignment of values, and control flow operations.
Syntax:
Relational Operators: Relational operators are represented by symbols, including equality operators (==, !=), relational operators (<, >, <=, >=), etc.
Other Operators: Other operators include arithmetic operators (+, -, *, /), logical operators (&&, ||, !), bitwise operators (&, |, ^), assignment operators (=, +=, -=, =, etc.), and more.
Operation:
Relational Operators: Relational operators compare the values of two operands and produce a Boolean result (true or false) based on the comparison. They determine the relationship between the operands.
Other Operators: Other operators perform specific operations, such as arithmetic calculations (addition, subtraction, multiplication, division), logical operations (conjunction, disjunction, negation), bitwise operations (AND, OR, XOR), assignment of values, control flow operations, etc.
Result Type:
Relational Operators: The result of a relational operator is a Boolean value (true or false) indicating the outcome of the comparison.
Other Operators: The result of other operators depends on the specific operator being used. For example, arithmetic operators produce numeric values, logical operators produce Boolean values, bitwise operators produce bitwise results, etc.
Usage:
Relational Operators: Relational operators are commonly used in conditional statements (if, while, for) and expressions that require comparisons between variables or values to control program flow.
Other Operators: Other operators are used in a wide range of scenarios, including mathematical calculations, logical evaluations, bitwise manipulations, variable assignments, and controlling program behavior.
Precedence:
Relational Operators: Relational operators have higher precedence than logical operators but lower precedence than arithmetic operators. They are evaluated before logical operators and assignment operators.
Other Operators: Operators in C have different levels of precedence, which determines the order in which they are evaluated within an expression. Understanding operator precedence is crucial for writing accurate and predictable code.
Comparing Characters With Relational Operators In C
Comparing one character to another might sound like an odd concept. But is it entirely possible to do so since characters are represented as integer values in C, and relational operators can compare integer values.
In other words, to compare characters using relational operators, the characters must first be converted to their corresponding integer values. This is done using the int data type, which can hold integer values ranging from -2147483648 to 2147483647.
Below is a code showcasing an example of comparing characters using the greater than operator (>):
Output:
a is not greater than b
Explanation:
In the above example, two characters (c1 and c2) are compared using the greater than operator (>).
- First, we declare two character variables, c1 and c2, which are initialized with values a and b, respectively.
- The characters are then converted to their corresponding integer values using the int data type.
- The resulting integer values (i1 and i2) are then compared using the greater-than operator and an if-else statement.
- As per the statement, if i1 is greater than i2, the program prints a message indicating that c1 is greater than c2. Otherwise, it prints a message indicating that c1 is not greater than c2.
- We use the printf() function inside the statement to display the result to the console.
Relational operators in C can also be used to compare characters in string literals. In this case, the characters in the strings are compared based on their ASCII values. For example:
Output:
hello is less than world
Explanation:
In the above example, the first characters of two string literals (str1 and str2) are compared using the less than operator (<). The characters are compared based on their ASCII values, and the result is used to print a message indicating whether str1 is less than str2. Here, where the program is a twist on the classic Hello World program, the output is 'hello is less than world'.
In conclusion, characters can be compared using relational operators in C by first converting them to their corresponding integer values. This capability allows programmers to compare characters in various contexts, including string literals and individual character variables.
Conclusion
Relational operators in C play a pivotal role in controlling program logic based on conditions. They allow developers to make decisions, define branching paths, and respond to specific situations, making programs more intelligent and adaptive. By understanding and effectively using relational operators, C programmers can create robust and responsive applications tailored to specific requirements.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. What are relational operators in C?
Relational operators in C are used to compare two values or expressions. They help determine the relationship between the values being compared. Relational operators return a boolean result, which is either true or false, based on the outcome of the comparison.
Q. Which relational operator is used for comparison in a C Program?
The basic purpose of the relational operators, as evident from the name, is to compare two operands to figure out the relationship between them. The different types of relational operators in C are:
- The equal to operator represented by (==)
- The not equal to operator represented by (!=)
- The greater than to operator represented by (>)
- The less than to operator represented by (<)
- The greater than equal to operator represented by (>=)
- The less than to operator represented by (<=)
Q. What is the output of relational operators in C?
The output/ result of a relational operator in C is a boolean value. A boolean value represents a condition as either true or false. In C, boolean values are typically represented using integers, where 0 represents false, and any non-zero integer represents true.
Here's a general rule:
- When a relational expression is evaluated to be true, it returns the integer value 1.
- When a relational expression is evaluated to be false, it returns the integer value 0.
This means that the result of a relational operator can be used in conditional statements, like if statements, to control the flow of a program. For example, you can use relational operators to compare values and make decisions in your code based on whether the comparison is true or false.
Q. Can relational operators be chained in C?
Yes, it is possible to chain relational operators in C using logical operators such as && (logical AND) and || (logical OR). For example, a < b && b < c checks if a is less than b and b is less than c.
Q. How do you compare strings in C using relational operators?
Strings cannot be compared directly using relational operators in C. Instead, you need to use string comparison functions such as strcmp() or strncmp() from the <string.h> library.
Q. What is the result of comparing floating-point numbers using relational operators in C?
Comparing floating-point numbers using relational operators in C can lead to unexpected results due to rounding errors. It is recommended to use an epsilon value and compare the difference between two floating-point numbers to determine if they are close enough.
Q. Can relational operators be used with non-numeric data types in C?
Generally, relational operators cannot be used with non-numeric data types in C. They are most commonly used with numeric data types such as int, float, and double. For example, in the expression a < b < c, the expression a < b is evaluated first, and then the result of that comparison is compared to the value of c.
However, it is possible to use relational operators to compare characters. But to do this, the characters must first be converted to integral values, which are then compared, like any other numerical values, using relational operators in C.
You might also be interested in reading the following:
- Ternary (Conditional) Operator In C Explained With Code Examples
- Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
- Increment And Decrement Operators In C With Precedence (+Examples)
- Structure In C | Create, Access, Modify & More (+Code Examples)
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment