Bool In C Programming | A Comprehensive Guide (+Detailed Examples)
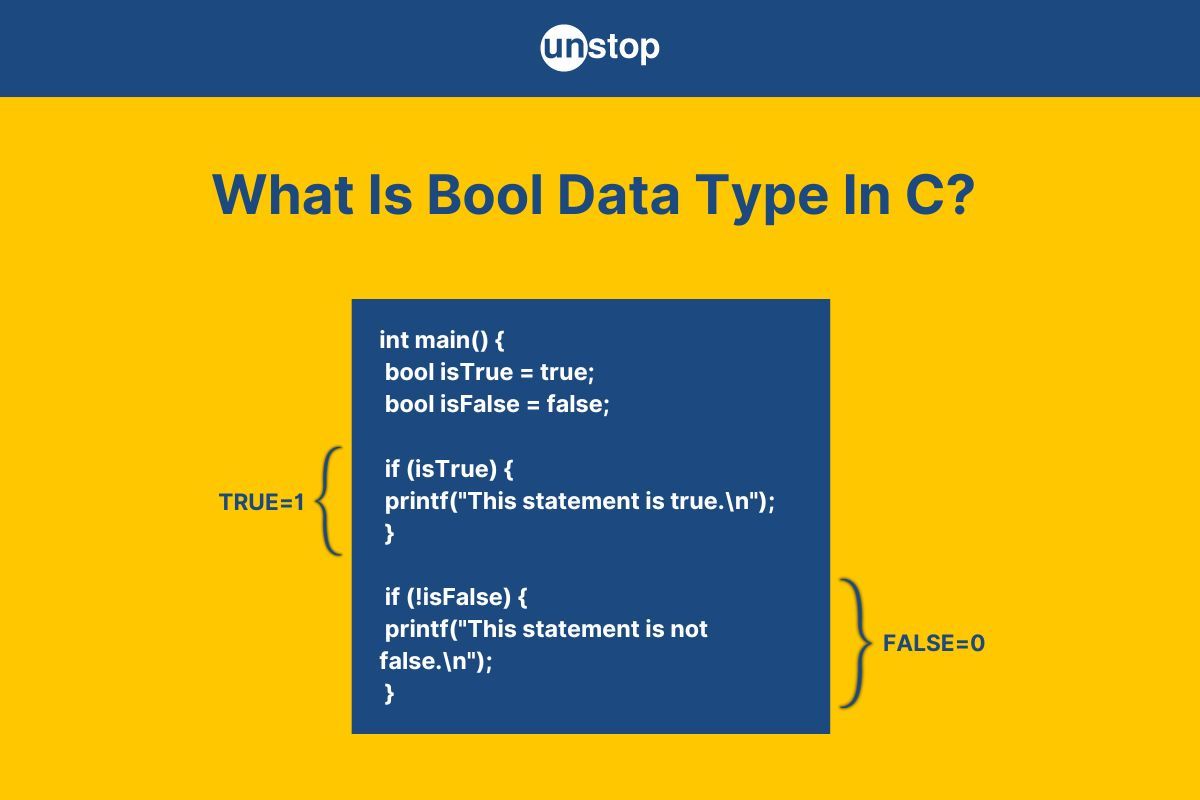
Table of content:
- What Is Bool In C?
- How To Declare Boolean In C?
- Boolean Arrays In C
- Logical Operators & Bool In C
- Conditional Statements & Bool In C
- Loops & Bool In C
- Bool In C As Function Return Type
- What Is The Need For Bool In C?
- Conclusion
- Frequently Asked Questions
Bool in C programming, also known as boolean, is a fundamental data type used to determine true or false values. It plays a central role in decision-making and control flow in programming. Historically, C used integers for boolean logic, where 1 represents true and 0 represents false. The term boolean algebra is named after the mathematician George Boole. Therefore, any kind of logic, expressions, or work theories given by him is considered Boolean.
In this article, we will explore the evolution of bool in C, including how to declare and use boolean variables. We will also discuss their importance in modern C standards.
What Is Bool In C?
In C programming language, a bool is a variable that can have one of two values, which are either true or false. These values are typically represented using integers with 0 representing false and 1 as true.
- Bool in C is a part of the C99 standard version which was introduced in 1999, and all the latter versions support this type of variable.
- It is defined in the <stdbool.h> header file.
- There is no difference between boolean and bool in C as C doesn’t have a built-in data type for boolean.
- Instead, it relies on the convention of using numbers to denote boolean values.
- Bool was introduced to provide a more natural and readable way to work with true or false conditions in C.
- Bool prevents logical errors that might occur when using integers to represent boolean values.
- It also makes the code more portable across different systems and compilers.
What Is The Size Of A Bool In C?
Bool in C has the size of 1 byte as it needs only two values 0 and 1. It actually needs only 1 bit of memory but takes up 8 bits due to the structure of the computing system, making it equal to 1 byte.
How To Declare Boolean In C?
Bool in C is generally declared by using the keyword bool followed by a variable name. However, in C programming, boolean values can be declared in various ways depending on the version of the language and the level of compatibility required.
There are three common methods for declaring boolean values/ bool in C, which we will discuss in this section.
The <stdbool.h> Header & Bool Keyword To Declare Boolean In C
The stdbool.h header, introduced in C99, defines a bool type, along with true and false constants values. This introduction of the built-in bool data type simplified boolean operations and improved code readability.
Syntax:
#include <stdbool.h>
bool variableName;
Here,
- bool is the keyword used for boolean values, as defined in stdbool.h header file.
- The variableName defines the name of the variable we are declaring. It will hold a boolean value (true or false).
Code:
Output:
The connection is established.
Explanation:
In the above code example-
- We start by including two header files: stdbool.h and stdio.h to use the bool type and the constants true and false, and input/output operations, respectively.
- In the main() function, we declare a variable named isConnected of type bool and initialize it with the value true. This means that isConnected is set to indicate that a connection is established.
- Next, we use if-else statements to check the value of the variable isConnected.
- Since the value of isConnected is true, the condition in the if statement evaluates to true. Therefore, the code inside the if block is executed, and we use printf() to output the message "The connection is established." to the console.
- If isConnected were false, the condition would evaluate to false, and the code inside the else block would run instead, outputting the message "The connection is not established."
- Finally, the main function returns 0, which signals that the program has completed successfully.
Using Define To Declare Boolean In C
The #define preprocessor directive can be used to create boolean constants in C, with 1 representing true and 0 representing false. This method is compatible with older versions of C.
Syntax:
#define TRUE 1
#define FALSE 0int variableName;
Here,
- #define TRUE 1: This preprocessor directive creates a macro that replaces every occurrence of TRUE with 1 in your code.
- #define FALSE 0: This preprocessor directive creates a macro that replaces every occurrence of FALSE with 0 in your code.
- int variableName: This declares an integer variable that will hold boolean-like values (1 or 0).
Code:
Output:
Task is completed.
Explanation:
In the above code example-
- We start by defining two macros: TRUE and FALSE using the #define processor directive. The macro TRUE is set to 1, and the macro FALSE is set to 0.
- These macros are used as symbolic constants to represent boolean values.
- Inside the main() function, we declare an integer variable named isCompleted and initialize it with the value of the TRUE macro, which is 1. This means that isCompleted is set to indicate that a task is completed.
- We then use an if-else statement to check the value of isCompleted.
- Since isCompleted is 1, which is non-zero, the condition in the if statement evaluates to true. Thus, the code inside the if block executes, and printf() outputs the message "Task is completed." to the console.
- If isCompleted were 0 (representing FALSE), the condition would be false, and the code inside the else block would run instead, outputting "Task is not completed."
- Finally, the main() function returns 0, indicating that the program has been completed successfully.
Using The Enumeration Type To Declare Bool In C
Before the introduction of the bool type in C99, the enumeration type (enum) was commonly used to simulate boolean values.
Syntax:
enum { FALSE, TRUE } variableName;
Here,
- enum: It denotes the enumeration data type, which defines two named constants, FALSE and TRUE. By default, FALSE is assigned the value 0, and TRUE is assigned the value 1.
- variableName: This is the name of the variable declared as part of this enumeration type. The variable can hold either FALSE or TRUE.
Code:
Explanation:
- In the above code snippet, we define an enumeration with values FALSE and TRUE, as constants to represent boolean values.
- In the main() function, we initialize status to TRUE and use an if statement to check the value of status, printing "The status is TRUE." if status equals TRUE, otherwise printing "The status is FALSE."
Alternatively, we can combine the typedef keyword and enum to create a custom boolean type in C, allowing us to use descriptive names like true and false for boolean values.
Syntax:
typedef enum{
false,
true
}bool;
Note: The enum type in C lacks strong type safety, which can lead to unintentional errors when used with other integer types. This limitation, along with the availability of more modern and type-safe alternatives, has made enum a less commonly used method in modern C practices.
Boolean Arrays In C
An array in C is a simple data structure that allows us to store a collection of elements of the same data type. Arrays are used to group related data items and each element in the array is accessed by its position or index.
- A boolean array is an array of elements where each element is of boolean type i.e. it can have one of two values which are either true or false.
- These arrays are typically implemented using a data type that can be represented as boolean values such as a bool or int.
- Boolean arrays are often used to store and manipulate sets of binary data flags or for conditions.
Syntax:
bool myBoolArray[size];
Here:
- bool: It signifies the data type used to represent boolean values.
- myBoolArray[ ]: It denotes the chosen name for the array with the array notation [].
- size: It corresponds to the number of elements within the array, which must be a positive integer.
Code:
Output:
Element 0: true
Element 1: false
Element 2: true
Element 3: false
Element 4: true
Explanation:
In the above code example-
- We start by declaring an array named myBoolArray that can hold 5 boolean values. Each element in this array is of type bool, which can store either true or false.
- We then initialize the elements of myBoolArray with specific values:
- The first element (i.e., element at index 0, myBoolArray[0]) is set to true.
- The second element (i.e., myBoolArray[1]) is set to false.
- The third element (i.e., myBoolArray[2]) is set to true.
- The fourth element (i.e., myBoolArray[3]) is set to false.
- The fifth element (i.e., myBoolArray[4]) is set to true.
- After initializing the array, we use a for loop to iterate over each element of myBoolArray.
- The loop variable i starts at 0 and increments up to 4, covering all 5 elements of the array.
- In each iteration, we use printf() to print the index of the element (i) and its value.
- The ternary/conditional operator (? :) is used to inside the formatted string in printf() to determine whether to print "true" or "false" based on the value of the current array element.
- If the element is true, it prints "true"; otherwise, it prints "false".
- Finally, the main() function returns 0, indicating that the program has finished executing successfully.
Alternatively, we can use int data type to declare an integer array and use specific values to indicate true or false conditions. This approach is useful for older C standards or when stdbool.h is not available but requires careful handling to distinguish between true and false.
Syntax:
int myBoolArray[size];
// Declare an integer array with size elements to represent boolean values
Code:
Explanation:
In the above code example, we declare an integer array where 1 is used to represent true and 0 represents false. We print each value, showing how integer arrays can be used to simulate boolean arrays.
Logical Operators & Bool In C
Logical operators in C are used to perform logical operations on boolean values, which are typically represented as integers. These operators in C allow you to create compound conditions and make decisions. The three main logical operators in C are Logical AND (&&) OR (||) NOT (!).
The bool data type in C is closely related to logical operators because it represents true or false conditions. When you use comparison operators like logical operators with bool you can combine and manipulate these conditions to make decisions in your code.
Logical AND (&&) & Bool In C
The double ampersand symbol (&&) represents the AND operator is used to perform logical AND operations. This means, it combines the expression on the lefthand and righthand side, and returns a boolean value.
- Simply stated, the operator returns 1 if both the operands are true and 0 when it is false.
- In boolean terms, if both the operands are ‘true’ then the result is ‘true’ otherwise it gives the result as ‘false’.
Truth Table:
Expression |
Is |
True && True |
True |
True && False |
False |
False && True |
False |
False && False |
False |
Code:
Output:
a && b = 0
Explanation:
In the above code example-
- We start by declaring three boolean variables: a, b, and result.
- Of these, we initialize a with the value true and b with the value false.
- Next, we use the logical AND operator (&&) to compute the value of the variable result. The logical AND operator evaluates to true only if both operands are true.
- Since a is true and b is false, the expression a && b evaluates to false. Therefore, the variable result is assigned the value false.
- We use the printf() statement to print the value of result. Since result is false, printf() outputs 0, which represents false in the context of the %d format specifier, which is used for printing integers.
- Finally, the main function returns 0, indicating that the program has been executed successfully.
Logical OR (||) & Bool In C
The double pipe symbol (||) is used to represent the OR operator and perform a logical OR operation. This operator also logically compares the two operands and returns a boolean value true if at least one of the operands is true. It returns false if both operands are false.
When working with boolean values if at least one operand is true then the result is true and false when both the operands are false.
Truth Table:
Expression |
Is |
True || True |
True |
True || False |
True |
False || True |
True |
False || False |
False |
Code:
Output:
a || b = 1
Explanation:
In the above code example-
- We start by declaring three boolean variables: a, b, and result. We initialize a with the value true and b with the value false.
- Then we compute the value of the variable result using the logical OR operator (||).
- The logical OR operator evaluates to true if at least one of its operands is true. In this case, a is true, and b is false.
- Since a is true, the expression a || b evaluates to true. Therefore, the result is assigned the value true.
- Next, we use printf() statement to print the value of the result. Since True is represented as 1 in an integer value, the output is 1.
Logical NOT (!) & Bool In C
The exclamation symbol (!) represents the Logical NOT operator which performs a negation operation.
- It reverses the logical value of its operand, i.e., if the operand is true it returns as false and vice versa.
- When working with boolean values Logical NOT inverts the value i.e. if the operand is true, it makes it false, and if the operand is false! , it makes it true.
Truth Table:
Expression |
Is |
!True |
False |
!False |
True |
Code:
Output:
!a = 0
Explanation:
In the above code example-
- We start by declaring two boolean variables: a and result. We will initialize a with the value true.
- Next, we calculate the value of result using the logical NOT operator (!).
- The logical NOT operator inverts the boolean value of its operand. If the operand is true, the result is false; if the operand is false, the result is true.
- Since a is true, on applying the NOT operator (!a) the value becomes false which is assigned to variable result.
- We then use printf() statement to display the value of result, i.e., false (bool) or 0 (integer).
Short-Circuiting
Short-circuiting is a behavior of logical operators in C where the evaluation of an expression stops as soon as the outcome is determined. This means that if the result of a logical operation can be determined on basis of a single bool value without evaluating all the operands, the evaluation process will be halted early. Short-circuiting can thus improve code performance by avoiding unnecessary computations and errors in code.
Relationship with Logical Operators & bool in C
Logical AND (&&): If the first operand evaluates to bool value false, the overall expression will be false regardless of the second operand. Therefore, the second operand is not evaluated because the result is already known.
Logical OR (||): If the first operand evaluates to bool value true, the overall expression will be true regardless of the second operand. Hence, the second operand is not evaluated because the result is already determined.
Let's look at a simple C program example, to understand the fundamental concept of short-circuiting in logical operators & bool:
Code:
Output:
Evaluating first condition.
Evaluating second condition.
At least one condition is false.
Explanation:
In the above code example-
- We first define two functions, checkFirstCondition() and checkSecondCondition(), each returning a boolean value.
- The checkFirstCondition() function prints the string message- "Evaluating first condition." and return false. This simulates a condition that evaluates to false.
- Similarly, checkSecondCondition() prints the message- "Evaluating second condition." and returns true, simulating a condition that evaluates to true.
- Now in the main() function, we use an if statement to evaluate whether both conditions are true using the logical AND operator (&&).
- We know that this operator requires both conditions to be true for the entire expression to be true. Since the first function call checkFirstCondition() returns false, the evaluation can stop here due to short-circuiting with the logical AND operator.
- Since the first condition is false, the overall result will be false regardless of the second condition. Hence, checkSecondCondition() is not called.
- As an outcome, the else block is executed, and printf() statement is used to output "At least one condition is false." to the console.
Conditional Statements & Bool In C
Conditional statements in C are used to make decisions in a program based on specific cases and conditions being met. That is, they execute different blocks of code depending on whether a condition evaluates to true or false.
The different types of conditional statements in C are:
- If statement: Executes a block of code if the specified condition is true.
- If-else statement: Executes one block of code if the condition is true, and another block if the condition is false.
- Switch statement: Selects and executes a block of code among many options based on the value of an expression.
We use boolean variables or expressions within conditional statements to control the flow of the program. The bool in C (or integers representing boolean values) is used to evaluate these conditions. A condition that evaluates to true (non-zero) will trigger the execution of the associated code block, while a condition that evaluates to false (zero) will not.
Code:
Output:
It's a sunny day!
It's a weekday.
You have plans for today.
Explanation:
In the above code example-
- We start by declaring three boolean variables: isSunny, isWeekend, and hasPlans.
- We first initialize isSunny to true, indicating that it is sunny.
- Next, we set isWeekend to false, indicating that it is not the weekend.
- Finally, we set hasPlans to true, indicating that there are plans for the day.
- Then, we use an if statement to check if variable isSunny is true. Since it is true, the condition is also true, and the printf() statement inside if-block is executed. It display the message- "It's a sunny day!" to the console.
- Next, we use an if-else statement to check the value of isWeekend.
- If isWeekend is true, i.e., the if-condition is true, then the if-block is executed printing the message-"It's the weekend!" to the console.
- If the condition is false, then the else-block is executed. Since isWeekend is false, the else block prints- "It's a weekday."
- Lastly, we use a switch statement to evaluate the value of hasPlans. The switch statement checks the value of hasPlans against the cases provided.
- If the value matches case true, then the respective block executes, printing "You have plans for today." to the console.
- If value matches case false, then that block executes, printing "You don't have any plans for today."
- In both cases, as soon as the block is executed, the break statement is encountered, and the control exits the switch statement.
- Since here hasPlans is true, the case true is executed.
- Finally, the program terminates with a return of 0.
Loops & Bool In C
Loops are control flow statements that allow the user to run a block of code that will be executed repeatedly until a specific condition is met.
- Loops rely on boolean conditions to decide if they should continue running or stop.
- Boolean values act as traffic lights for loops, guiding when the loop's code executes or ends. Instead of using integers (0 and non-zero values), bool values (true and false) make the intent of the code more understandable.
- This practice is especially useful for complex logic and to improve the flow of the code.
The three main types of loops are for, while, and do-while. We will explore all these types and their connection with bool in C in the sections ahead.
While Loop & Bool In C
The while loop executes a block of code as long as the loop condition of the loop returns a bool value true. Using a bool variable for the condition allows us to control the while loop's execution more explicitly by setting the condition to true or false based on specific criteria.
Code:
Output:
While loop count: 0
While loop count: 1
While loop count: 2
Explanation:
In the above code example-
- We start by declaring an integer variable count and initialize it to 0. We also declare a boolean variable continueLoop and initialize it to true.
- Next, we use a while loop that continues executing as long as continueLoop is true. Inside theloop:
- We use printf() to display the current value of count and then increment it by 1 (count++).
- Then, we have an if-statement to check the loop condition, i.e., whether count is greater than or equal to 3.
- If the condition is false, the if-block is ignored and the loop continues iterations.
- If this condition is true, we set continueLoop to false, which turns the loop condition false, thus terminating the loop (after the current iteration).
- In this example, the loop continues iterations until count reaches 3 after which continueLoop is set to false, and the loop terminates.
For Loop & Bool In C
The for loop runs a block of code a specific number of times based on an initial condition, a boolean condition for continuation, and an increment/decrement operation. A bool value can be used in the evaluation of a condition to clearly define when the for loop should continue or stop.
Code:
Output:
For loop iteration: 0
For loop iteration: 1
For loop iteration: 2
Explanation:
In the above code example-
- We begin by declaring a boolean variable named keepRunning and initialize it to true. We use this variable to control the execution of the loop.
- We then enter a for loop and initialize the loop variable i to 0. The loop will continue as long as keepRunning is true. Inside-
- In every iteration, we use printf() statement to print the current iteration number i.e. value of i to the console.
- After that, the value of i is incremented automatically by 1.
- Then we check if i is greater than or equal to 2 using an if statement.
- If this condition is true, we set keepRunning to false. This change results in the loop condition keepRunning becoming false (not true) terminating the loop after the current iteration.
- In this example, the loop executes, printing the iteration number for each loop cycle, until i reaches 2. At that point, keepRunning is set to false, and the loop ends.
Do-While Loop & Bool In C
A do-while loop guarantees the execution of a block of code at least once, regardless of the initial condition. After that it checks the condition, and continues iteration and as long as the condition returns bool value true. A bool variable can be used to determine whether the do-while loop should continue running or terminate after each iteration.
Code:
Output:
Do-while loop count: 0
Do-while loop count: 1
Do-while loop count: 2
Explanation:
In the above code example-
- We begin by initializing an integer variable count with 0 and a boolean variable continueLoop with value true.
- Then, we enter a do-while loop which guarantees that the loop body will be executed at least once before checking the condition. Inside the loop-
- We first print the current value of variable count using the printf() statement.
- Next, we increment count by 1 using the count++ statement.
- We then update the continueLoop variable with the result of the expression (count < 3). This expression checks whether count is less than 3. If it is, continueLoop is set to true; otherwise, it is set to false.
- In this example, the loop continues to execute as long as continueLoop is true. When count reaches 3, the expression (count < 3) evaluates to false, so continueLoop becomes false, and the loop terminates.
Bool In C As Function Return Type
The bool in C can be used as a function return type to indicate that a function returns a boolean value (true or false).
- Using the bool return type is useful especially when a function aims to perform a true or false test or condition evaluation.
- This practice enhances code readability and allows you to write self-documenting code.
Functions can return any data type, including bool in C, which can be useful for writing functions that perform logical checks and return the check result.
Approach: To use bool in C as the return type of a function, follow these steps-
- Include the <stdbool.h> header file in your program.
- Declare the function return type as bool.
- Implement the function body and perform the necessary logical checks.
- Return a bool value indicating the result of the check.
Code:
Output:
6 is even.
Explanation:
In the above code example-
- We include the <stdbool.h> header file to enable the use of the bool type and the boolean values true and false.
- Next, we declare a function prototype for isEven(), which takes an integer as an argument and returns a boolean value. This tells the compiler that we will define this function later in the code.
- In the main() function, we declare an integer variable num and initialize it with the value 6.
- Next, we use an if statement to check whether the number is even by calling the isEven() function with num as the argument.
- The isEven() function returns true if num is even and false if num is odd.
- Inside the isEven() function, we calculate the remainder of the number divided by 2 using the modulo operator (%):
- If the remainder is 0, the function returns true, indicating that the number is even. Otherwise, it returns false.
- Since num is even(6), and 6 divided by 2 has no remainder, isEven returns true. Therefore, the condition in the if statement evaluates to true, and the printf() statement outputs "6 is even." to the console.
- Note that if num were an odd number, the isEven function would return false and the if-condition would evaluate to false. As a result the else block would execute, printing the message that the number is not even.
What Is The Need For Bool In C?
Bool in C helps developers control program flow, make decisions, and handle errors. Here are a few reasons why the bool type is essential in C programming:
- Improved Readability: Using bool in C makes the code more readable and intuitive. When you see a variable declared as a bool, it's immediately clear that it is intended to store a boolean value (true or false). For example-
int main() {
bool isFinished = false;if (!isFinished) {
printf("The task is not finished yet.\n");
}
-
Decision making: When it comes to programming we need to make decisions based on certain conditions and in accordance, one part of the code is executed and the other part of the code reaches the other condition. Bool in C helps you evaluate these conditions with ease. For example-
bool isUserLoggedIn = true;
if (isUserLoggedIn) {
// Execute code for logged-in users
printf("Welcome back, user!\n");
} else {
// Execute code for guests
printf("Please log in to continue.\n");
}
- Loop control: Loops are used to execute a block of code repeatedly until a certain condition is met. Boolean determines when the loop should continue or stop. The loop continues when the condition keeps on becoming true and terminates the loop when the condition values become false. For example-
bool continueLoop = true;
while (continueLoop) {
// Code here
// Update the condition
continueLoop = (/* some condition */);
}
- Error handling: Boolean values are often used in error handling to indicate whether a function or operation was successful (true) or not (false). This helps us write robust and reliable code that can respond to errors. For example-
bool processData(int data) {
if (data < 0) {
return false; // Error: Invalid data
}
// Process data here
return true; // Success
}int main() {
bool result = processData(-1);if (!result) {
// Handle error
printf("Error processing data.\n");
} else {
// Continue with successful operation
printf("Data processed successfully.\n");
}
- Type Safety: Using bool in C helps us to ensure type safety. When boolean values are represented by integers, it is easy to accidentally misuse them in arithmetic operations. The bool type restricts the values to true and false, reducing the risk of errors. For example-
void checkFlag(bool flag) {
if (flag) {
printf("Flag is set.\n");
} else {
printf("Flag is not set.\n");
}
}int main() {
checkFlag(true);
- Semantic Meaning: Declaring a variable as bool in C programs adds semantic meaning to the code, making it easier to understand the programmer’s intent. This is especially useful in complex conditions and logical operations.
- Compatibility with Other Languages: Many modern programming languages have a dedicated boolean type. Using bool in C aligns with these languages, making it easier for developers to switch between languages and understand each other's code.
- Memory Efficiency: While bool typically occupies 1 byte, it is more memory-efficient compared to using larger data types like int for boolean values, especially when used in structures or arrays. For example-
bool flags[3] = {true, false, true};
for (int i = 0; i < 3; i++) {
if (flags[i]) {
// Code here
}
}
Conclusion
Bool in C was introduced with the C99 standard through the <stdbool.h> header file. It was an enhancement that provides a more user-friendly and widely accepted way to handle boolean values. By using true and false instead of integers, we can significantly improve code readability and maintainability.
The bool type is commonly employed in control statements, function return types, and logical expressions, which simplifies decision-making and streamlines program flow based on conditions being true or false. Therefore, utilizing bool in C can lead to more effective and error-free programming practices.
Frequently Asked Questions
Q. What is the stdbool.h header, and why is it necessary?
The stdbool.h header is a standard header file in C that defines the bool data type and the macros true and false respectively. Before its introduction in the C99 standard, C programmers often used integers to represent boolean values, which could lead to less readable and more error-prone code. Including stdbool.h simplifies and clarifies the use of boolean values in C programming.
Q. What is the size of a bool in C, and how is it stored in memory?
The size of a bool in C is typically 1 byte (8 bits), even though it only needs 1 bit to store true or false. This is because the smallest addressable unit of memory in most systems is a byte. Thus, a bool occupies 1 byte to align with the system's memory structure. This size is sufficient to represent the two possible boolean values (true or false).
Q. What is bool in C and how is it different from integer types?
The bool in C is a data type introduced through the stdbool.h header, representing boolean values true and false. Unlike integers, which can represent a wide range of values, bool is specifically designed to hold one of two values: true (1) or false (0).
Q. Can I use bool in conditional statements and loops?
Yes, bool in C is commonly used in conditional statements (if, if-else, else-if, switch) and loops (while, for) to control the flow of the program based on boolean conditions. Using bool makes the code more readable by explicitly indicating that the variable holds a boolean value. For example-
#include <stdbool.h>
bool isRunning = true;
if (isRunning) {
printf("The system is running.\n");
}
Q. What is the benefit of using bool in C?
Some of the key benefits of using bool in C are as follows:
- Improved Code Clarity: The bool in C provides a clear and straightforward way to represent Boolean values, which are at the heart of decision-making in programming. When you use bool type variables and boolean expressions, your code becomes more self-explanatory. It's easier for you and other developers to understand the intent behind your code.
- Self-Documenting Code: By using bool in C you create self-documenting code. When someone reads your code, the presence of bool variables and functions with `bool` return types immediately signals that you're dealing with true-or-false conditions. This reduces the need for extensive comments in your code to explain its purpose.
- Reduced Risk of Errors: Traditional C programming often relied on integers (0 for false, non-zero for true) to represent Boolean conditions. This practice could lead to errors when programmers accidentally assign incorrect values. With bool, the risk of such errors is significantly reduced because it explicitly enforces true-or-false semantics.
- Enhanced Debugging: When debugging code that uses bool, identifying and isolating issues related to conditional logic becomes more straightforward. You can focus on the correctness of the logic itself without getting distracted by integer-to-boolean conversions or misinterpretations.
- Standardization: The introduction of bool in the C99 standard brought a standardized approach to working with boolean data types. This means that code using `bool` is more portable across different C compilers and platforms, ensuring consistency in behavior.
- Readability and Maintenance: In larger codebases or team projects, code maintainability is crucial. bool makes it easier for developers to modify and extend code because the logical flow is more transparent. Changes and updates can be made with confidence, knowing that the intent of the code remains clear.
You might also be interested in reading the following:
- Compilation In C | Detail Explanation Using Diagrams & Examples
- The Sizeof() Operator In C | A Detailed Explanation (+Examples)
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Comma Operator In C | Code Examples For Both Separator & Operator
- Type Casting In C | Types, Cast Functions, & More (+Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment