Typedef In C | Syntax & Use With All Data Types (+ Code Examples)
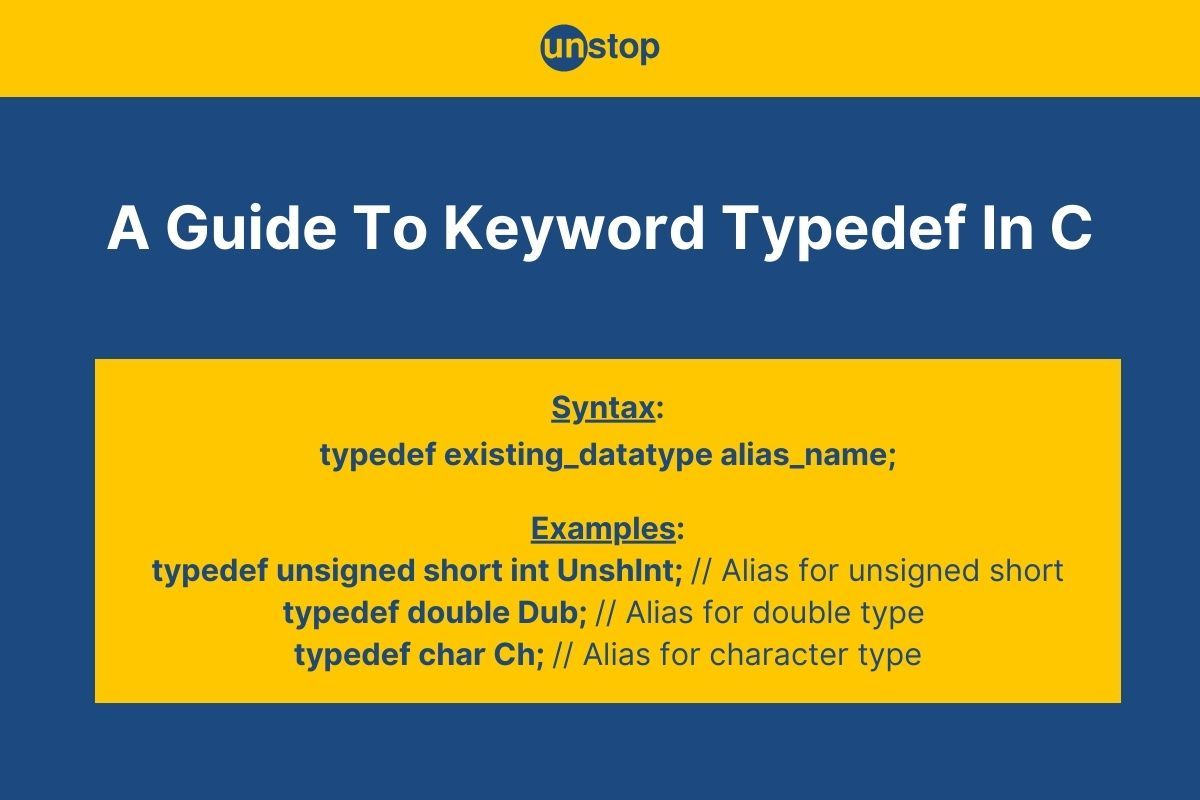
Data types play a crucial role in programming by indicating which type of data is stored in a variable and other components of the program. But what if the symbolic name for a respective data type is too complex to be used repeatedly when writing code? Well, this is where the typedef in C programming comes into play.
It is a powerful tool that helps us develop clear and concise code to maintain readability and improves collaboration among developers. In this article, we'll delve into the intricacies of typedef in C, including its syntax, working, applications, and more, to demonstrate how it can enhance code quality and maintainability.
What Is typedef In C?
The typedef in C is a reserved keyword that helps us give new names to existing data types, essentially redefining their nomenclature. This is useful when the original names of data types become too long or cumbersome in program usage.
- When used with user-defined data types, typedef in C program structure acts as an alias for commands.
- It allows users to create aliases for established data types, offering the advantage of using a custom name instead of the original data type name.
By using the typedef keyword when writing and running C programs, you can assign names to built-in data types such as int, float, char, and double. These temporary names can then be used as aliases when declaring variables, simplifying the code and making it easier to understand.
Syntax for Typedef in C:
typedef existing_data_type new_name;
Here,
- typedef: The keyword that indicates the creation of a new type.
- existing_data_type: The data type for which a new name is being created.
- new_name: The alias or new name assigned to the existing data type.
Look at the simple C program below to understand how typedef can be used to define an alias name for the unsigned short int data type and use it to declare variables of this type.
Code Example:
Output:
The sum of 10 and 20 is 30
Explanation:
In the simple C code, we begin by including the essential header file for input/output functions, i.e., <stdio.h>.
- We then use the keyword typedef to create an alias for the data type unsigned short integer, naming it UnshInt.
- Inside the main() function, we declare three unsigned short int type variables, x, y, and sum, using the alias name for unsigned short int, i.e., UnshInt.
- We also assign values of 10 and 20 to variables x and y, respectively. For variable sum, we define it as the sum of x and y (using the addition arithmetic operator).
- Next, we use the printf() function to display the values of x and y, as well as their sum to the console inside a formatted string.
- Here, we use the %d and %hu format specifiers to indicate integer value and unsigned short integer, respectively. Also, the newline escape sequence (\n) shifts the cursor to the next line.
- Finally, the main() function terminates with a return 0 statement, indicating successful execution.
Working Of typedef In C
Using typedef in C language involves creating an alias or a new name for an existing data type, which can be especially beneficial for managing complex declarations. Here are the steps and guidelines to use typedef in C:
- Declaration: The typedef keyword begins a declaration that creates an alias for an existing data type. It is typically used at the global scope or within a function definition or structure definition.
- Existing Data Type: Identify the existing data type for which you want to create an alias. This can be any valid data type, including primitive types (int, float, char), derived types (arrays, structures, pointers), or user-defined types (enums).
- New Name (Alias): Choose a new name or alias that you want to assign to the existing data type. The alias can be any valid C identifier, following the same rules as variable names. However, note that it cannot be any other reserved keyword.
- Apply typedef Syntax: Write the typedef keyword followed by the existing data type and the new name (alias), separated by a space.
- Usage: Once you've defined the alias using typedef, you can use it anywhere you would use the original data type. This includes variable declarations, function parameters, return types, structures, unions, enums, and more.
- Scope: The scope of the typedef declaration depends on where it's placed in the code.
- If declared globally (outside of any function or structure), the alias is available throughout the entire program.
- If declared within a function or structure, the alias is limited to the scope of that function or structure.
- Documentation: It's good practice to document typedefs and their intended usage, especially in larger codebases or when collaborating with other developers. This helps other programmers understand the purpose and meaning of each typedef, improving overall code comprehension and maintainability.
Look at the C example program below to understand how typedef enhances code readability and reduces complexity without damaging the original meaning of data types.
Code Example:
Output:
Enter 2 values: 7 9
Sum value is: 16
Explanation:
In the C example code-
- We use typedef to create an alias name for int data type, i.e., myint. This allows us to use myint as an alias for int throughout our code.
- Inside the main() function, we declare an integer variable x using int and an integer variable y using myint. Since myint is an alias for int, y is an integer variable.
- Then, as mentioned in the code comments, we create an alias for type myint called smallint, using the typedef in C.
- Next, we declare a variable z of type smallint. Because smallint is typedef-ed to myint, which in turn is typedef-ed to int, z is essentially an integer variable.
- After that, we use the printf() function to prompt the user to input two int values and read them using the scanf() function.
- We store the value inputted by the user to the respective variables using the address-of operator (&).
- Following this, we define variable z to contain the sum of variables x and y, which we then print to the console using the printf() function.
This example clearly shows how we can create aliases for predefined data types and use them for the same purpose without any difficulty.
How To Use typedef In C With Basic Types
The fundamental data types include int for integers, char for characters, float for floating-point numbers, double for double-precision floating-point numbers, and more. To use typedef with basic types, we need to provide an alias for the type followed by the original type name. Look at the C program example below to see the implementation of the same.
Code Example:
Output:
Integer: 10
Unsigned Integer: 20
Long Integer: 1234567890
Unsigned Long Integer: 1234567890
Float: 3.140000
Double: 3.141593
Character: A
Unsigned Character: B
Void Pointer: (nil)
Explanation:
In the C code example-
- We use typedef several times to create aliases of many basic data types. Each time we use the keyword, we create an alias as follows-
- It for int, UnsIt for unsigned integer, LgInt for long integer, UnsLInt for unsigned long integer, Float for float, Dub for double, Ch for char, UnsCh for unsigned char, and Vd for void.
- Inside the main() function, we declare variables using the alias/ symbolic names we created using typedef for each data type and initialize them with specific values.
- After that, we use a set of printf() statements to display the values of these variables. For each variable, we use the corresponding format specifier (%d, %u, %ld, %lu, %f, %lf, %c, %p) to ensure correct output.
- Finally, the main() function returns 0, indicating successful execution.
Structure & typedef In C
A structure is a user-defined composite data type that allows you to group together variables of different data types under a single name. In other words, it enables you to create complex data structures by defining your own data types, each consisting of multiple fields or members.
We can use the typedef in C to create aliases for structure types and define shorter, more descriptive names for these structures. Using typedef with structures promotes clearer, more concise code, making it easier for developers to understand and work with complex data structures in C programs.
Syntax:
// Define a structure
struct structure_name {
// Member variables
data_type member1;
data_type member2;
// ...
};// Create an alias (typedef) for the structure
typedef struct structure_name alias_name;
Here,
- struct structure_name: This is the name of the structure being defined.
- { ... }: Within the curly braces, you list the member variables of the structure.
- data_type member: Each member variable consists of a data type followed by a member name.
- typedef: This keyword is used to create an alias for the structure.
- struct structure_name alias_name: Here, alias_name is the alias that you're creating for the structure.
Let's look at an example C program where we use the typedef keyword to create an alias for the structure. We will then use the lais to declare, initialize and access structure elements with ease.
Code Example:
Output:
Coordinates of point p1: (3, 5)
Explanation:
In the example C code-
- We define a structure named Point to represent a point in 2D space. This structure has two members: x and y, both of type int, representing the coordinates of the point.
- Then, we create an alias for the Point structure using the typedef in C and name it Point2D. This alias allows us to refer to the Point structure simply as Point2D.
- Inside the main() function, we declare a variable p1 of type Point2D, which is essentially a Point structure. We initialize p1 with coordinates (3, 5) using the syntax {3, 5}.
- We access and print the coordinates of the point p1 using printf(). We use dot notation (p1.x and p1.y) to access the x and y coordinates respectively.
- Finally, the main() function returns 0, indicating successful execution.
Pointers & typedef In C
A pointer is a variable that holds the memory address of another variable. It allows indirect access to the value stored in memory. The typedef in C allows developers to create aliases for pointer types, providing more descriptive names for pointers and enhancing code readability. By using typedef with pointers, developers can simplify complex pointer declarations, abstract away implementation details, and improve code portability.
Syntax:
// Define a typedef for a pointer type
typedef existing_type* alias_name;
Here,
- typedef: This keyword is used to create an alias for the pointer type.
- existing_type*: This represents the existing pointer type that you want to alias. It could be any valid pointer type.
- alias_name: This is the alias you're creating for the pointer type. It can be any valid C identifier.
Below is a sample C program that showcases how to use typedef to create an alias for an integer pointer so that we don't have to use the lengthy syntax everywhere in the code.
Code Example:
Output:
Value stored at memory location 0xa002a0: 42
Explanation:
In the sample C code-
- We define a typedef for a pointer to an integer called IntPtr. This typedef allows us to refer to a pointer to an integer as IntPtr.
- Inside the main() function, we declare a variable ptr of type IntPtr, which is essentially a pointer to an integer.
- We allocate memory for an integer using the malloc() function. The sizeof(int) expression determines the memory size to allocate for an integer.
- Then, we use an if-statement to check if the memory allocation was successful by verifying if ptr is NULL (i.e., is a null pointer), using an equality relational operator.
- If allocation fails, we print an error message and return a non-zero error code (1).
- If not, then we move to the next line of the code.
- Next, we assign a value of 42 to the memory location pointed to by ptr using the dereference operator *ptr.
- After that, we use a printf() statement to display the value stored at the memory location pointed to by ptr, along with the memory address.
- Then, we use the free() function to deallocate the dynamically allocated memory to prevent any memory leaks.
Arrays & typedef In C
An array is a collection of elements of the same data type stored in contiguous memory locations. They are widely used for storing and manipulating multiple values of the same type efficiently. The typedef in C allows developers to create aliases for array types, enabling the definition of more descriptive and concise names for these arrays. Below is a C program sample that showcases the implementation of typedef with arrays.
Syntax:
// Define a typedef for an array type
typedef existing_type alias_name[array_size];
Here,
- typedef: This keyword is used to create an alias for the array type.
- existing_type: This represents the existing data type of the elements in the array.
- array_size: This specifies the size of the array.
- alias_name: This is the alias you're creating for the array type. It can be any valid C identifier.
Code Example:
Output:
Elements of the array: 1 2 3 4 5
Explanation:
In the C code sample-
- We define a typedef for an array of integers called Array5. This typedef allows us to refer to an array of five integers as Array5.
- Inside the main() function, we create an array called numbers of type Array5, which is essentially an array of five integers.
- We initialize the elements of the numbers array with the values {1, 2, 3, 4, 5}.
- After that, we use a for loop with a printf() statement inside to display the elements of the array to the console.
- The loop begin from i= 0, accessing the element of the array using array subscript notation (numbers[i]).
- We increment the value of the loop variable i, after every iteration and continue to access subsequent element, while printing them to the console.
- Finally, we print a newline character to improve the readability of the output.
Purpose Of typedef In C
Here are some of the primary reasons for using typedef in C programs to create aliases of existing data types:
1. Enhancing Readability: The primary purpose of typedef in C is to improve code readability by providing more meaningful and descriptive names for data types. This makes the code easier to understand and maintain, especially when dealing with complex or custom data types.
typedef struct {
int day;
int month;
int year;
} Date;
Here, Date becomes an alias data type name for the structure and can be used just like the structure.
2. Abstracting Implementation Details: The typedef in C can abstract away implementation details, allowing developers to change underlying data types without affecting the rest of the code.
typedef unsigned int ID;
3. Simplifying Complex Declarations: The typedef in C simplifies the declaration of complex data types, especially those involving pointers or function pointers.
typedef int (*CompareFunc)(const void*, const void*);
4. Creating Modular Interfaces: We can use typedef in C to define clear and concise interfaces for libraries or modules, hiding implementation details from users.
typedef struct {
float x;
float y;
} Point;
5. Improving Portability: The typedef in C can also enhance code portability by abstracting away platform-specific details or implementation-specific data types. By using typedefs for common data types, you can write code that is more resilient to changes in underlying architectures or compilers.
6. Defining Custom Data Types: typedef enables the creation of custom data types tailored to specific application requirements.
typedef enum {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
} Day;
Overall, typedef is a versatile tool in C programming that promotes code clarity, modularity, and portability. By providing meaningful aliases for data types, typedef in C simplifies code maintenance and enhances developer productivity.
Difference Between #define & typedef In C
It is important for everyone to understand the distinction between #define and typedef. While #define performs text substitution, enabling straightforward preprocessor directives typedef focuses on creating aliases for data types, enhancing code readability and maintainability. These differences, presented in tabular form below, underscore the importance of choosing the appropriate tool for specific programming needs.
Basis | #define | typedef |
---|---|---|
Purpose | It is a preprocessor directive primarily used for creating macros for constant values or code snippets. | It is a keyword used for creating aliases for existing data types or defining new data types. |
Syntax | #define identifier value | typedef existing_type new_type; |
Preprocessor | Resolved by the preprocessor before compilation. Macros are essentially textual substitutions. | Resolved by the compiler during compilation. Typedefs create new type names based on existing types. |
Type Safety | Macros are not type-safe. They substitute text without understanding the underlying data type. | Typedefs are type-safe. They create new type names based on existing types, ensuring type safety. |
Scope | Macros have a global scope and apply throughout the entire program unless explicitly undefined. | Typedefs have a limited scope and are typically confined within a specific code block or module. |
Example | #define PI 3.14159<br>printf("The value of PI is: %f\n", PI); | typedef double Distance;<br>Distance d = 10.5; |
Code Example: #define
Output:
The value of PI is: 3.141590
Explanation:
In the example code in C-
- We define a macro for the mathematical constant PI using the #define directive. The macro PI is assigned the value 3.14159.
- Inside the main() function, we use the printf() function to print the value of PI.
- We include the macro PI in the formatted string, specifying %f to indicate that the value will be printed as a floating-point number.
- The value of PI is substituted into the format string during compilation, so when the program runs, the output will display the value of PI as 3.14159.
- Finally, the main() function returns 0, indicating successful execution.
Code Example: typedef in C
Output:
Distance: 10.500000
Explanation:
In the above code,
- We use typedef to create an alias for the double data type named Dist. This means we can use it to create and access double-type variables anywhere in the program.
- Inside the main() function, we declare a variable d of type Dist, which is essentially a double.
- We initialize d with the value 10.5, which is allowed because Dist is defined as a double.
- Next, using the printf() function, we print the value of the variable d. We specify %f in the format string to indicate that the value will be printed as a floating-point number.
Applications Of typedef In C
Here are some common applications of typedef in C:
- Platform Portability: The typedef in C can help in writing code that is more portable across different platforms by abstracting away platform-specific data types.
- Code Maintenance: By defining typedefs for commonly used data types or complex declarations, code maintenance becomes easier as changes can be made in one place.
- Interface Design: When designing interfaces for libraries or modules, using typedef in C can provide clear and concise abstractions, making the interface more intuitive for users.
- Documentation: Using typedef in C can improve code documentation by making the intent of certain data types or structures clearer to other developers.
- Error Prevention: Typedef in C can help prevent errors caused by incorrect type usage, as developers can use the typedef name instead of the actual type, reducing the likelihood of mistakes.
- Code Refactoring: When refactoring code, typedef in C can make the process smoother by providing a centralized location for type changes, minimizing the risk of introducing bugs.
- Enhancing Readability: We can use typedef in C to create more meaningful names for data types, making the code easier to understand.
- Abstraction of Implementation: It allows hiding implementation details by providing a high-level name for a complex type, thus simplifying its usage.
- Simplifying Pointer Declarations: Typedef in C can simplify complex pointer declarations, especially those involving pointers to functions or pointers to arrays.
- Standardization: In large codebases or projects involving multiple developers, typedefs can standardize naming conventions for data types, leading to more consistent and maintainable code.
Conclusion
In conclusion, typedef in C programming language is a versatile tool that enhances code readability, maintainability, and portability. By allowing developers to create aliases for data types, the keyword typedef provides a means to define more descriptive names for types, abstract away implementation details, and simplify complex declarations.
Whether used with basic types, structure type, pointer variables, or arrays, typedef in C empowers developers to write clearer and more concise code, making it easier to understand and maintain. Moreover, it fosters modularity and abstraction, enabling the creation of portable and reusable code components. Overall, understanding and leveraging the power of typedef in C can greatly contribute to developing efficient and maintainable software solutions.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What does typedef enum mean in C?
The typedef enum statement is used to create a named enumeration type. This syntax combines the typedef keyword with the enum keyword to define a new type name for a set of named integer constants. It allows you to create more meaningful and self-explanatory names for the enumeration type. For example,
typedef enum { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY } Days;
Q. Differentiate between typedef, enum, typedef enum, and #define in C.
Basis |
typedef in C |
enum |
typedef enum |
#define |
Purpose |
Creates an alias for an existing data type, improving code readability. |
Defines named integer constants, improving code readability, and maintainability. |
Combines keyword typedef with enum to create named integer constants with an alias for improved readability. |
Performs text substitution at compile-time, replacing defined names with specified values. |
Usage/ Example |
typedef int Integer; |
enum Days {MON, |
typedef enum {MON, TUE, WED} Days; |
#define PI 3.14 |
Defining Named Constants |
No |
Yes |
Yes |
Yes |
Scoping |
Limited to the scope where it is defined. |
Limited to the scope where it is defined. |
Limited to the scope where it is defined. |
Global scope (unless defined within a specific scope). |
Type Safety |
Yes |
No |
Yes |
No |
Supports Initialization |
Yes |
Yes |
Yes |
No |
Size and Type |
Depends on the existing data type. |
The enum creates integers, and the size is compiler-dependent. |
Depends on the existing data type. |
No specific type or size (text substitution). |
Compile-time Execution |
No |
No |
No |
Yes (text substitution). |
Q. Expand the abbreviation typedef in C.
As the nomenclature implies, typedef is short for "type definition." In the realm of C, typedef serves as a mechanism for assigning a fresh moniker to an established data type. Essentially, it is a reserved keyword employed to generate an alternative name, or alias, for a particular data type. Importantly, this does not alter the function or purpose fulfilled by the original data type.
Q. Is typedef considered a storage class in C?
Storage class specifiers in C are keywords that indicate the storage duration and/or linkage of a variable. Yes, typedef in C is considered a storage class specifier, which also includes:
- auto
- register
- static
- extern
- typedef
The classification of typedef in C as a storage class specifier is rooted more in syntactic resemblance than functional alignment, primarily because a typedef declaration doesn't involve the allocation of storage.
Q. Define data type in C.
In C programming, a data type is a classification that specifies which type of value a variable can hold. It defines the operations that can be performed on the data, the meaning of the data, and the way values of that type can be stored. C provides several basic data types, which can be broadly categorized into the following:
- Basic Data Types:
- int: Integer data type is used for storing whole numbers.
- float: Floating-point data type used for storing real numbers with decimal points.
- double: Double-precision floating-point data type used for storing larger real numbers with decimal points.
- char: Character data type used for storing a single character.
- Derived Data Types:
- array: A collection of elements of the same data type.
- Pointer: A variable that stores the memory address of another variable.
- structure: A user-defined data type that groups related variables under a single name.
- union: A user-defined data type that allows storing different data types in the same memory location.
- Enumeration Data Type: A user-defined data type that consists of named integer constants.
- Void Data Type: Represents the absence of a data type. It is often used as the return type of function that does not return a value.
We can use typedef in C to create aliases for any of these data types, as and when needed.
Here are a few other topics you must explore:
-
- Comma Operator In C | Code Examples For Both Separator & Operator
- Control Statements In C | The Beginner's Guide (With Examples)
- Type Casting In C | Cast Functions, Types & More (+Code Examples)
- Tokens In C | A Complete Guide To 7 Token Types (With Examples)
- Bitwise Operators In C Programming Explained With Code Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment