Table of content:
- What Is A Decimal Number?
- What Is A Binary Number?
- Representation Of Decimal & Binary Numbers
- Algorithm For Converting Decimal To Binary In C
- Convert Decimal To Binary In C Using Naive Approach/ Division Optimization (Without Arrays)
- Convert Decimal To Binary In C Using While Loop (User Input)
- Converting Decimal To Binary In C Using Stack
- Converting Decimal To Binary In C Using Bitwise Operators
- C Program to Convert Decimal to Binary Using Functions
- Converting Decimal To Binary In C Using For Loop
- Converting Decimal To Binary In C Using Math Module
- Converting Decimal To Binary In C Using Right Shift With AND Operator
- Converting Decimal To Binary In C Using Recursion
- Converting Decimal To Binary In C For Negative Number
- Converting Floating-Point Decimal Numbers To Binary In C
- Time & Space Complexity Comparison For Programs To Convert Decimal To Binary In C
- Conclusion
Decimal To Binary In C | 11 Methods (Examples + Complexity Analysis)
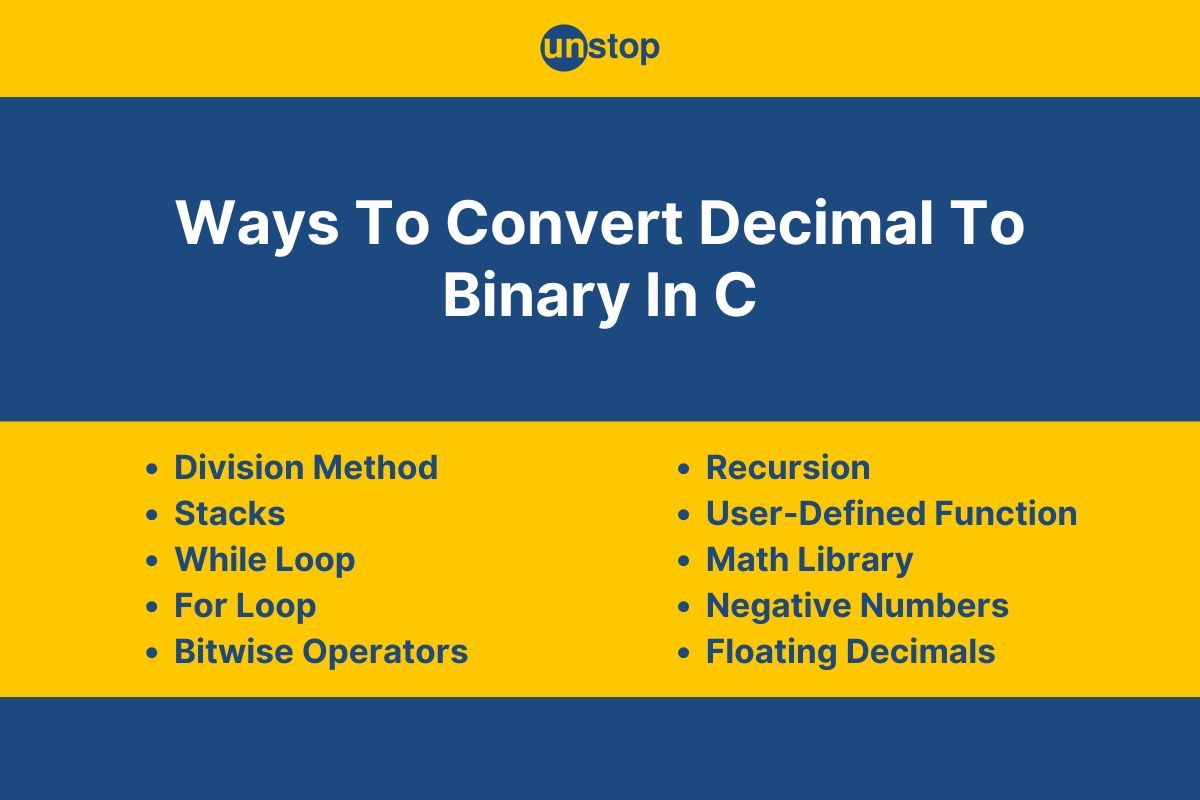
The number system is a way of representing and expressing quantities in a structured format. The two most commonly used number systems are the decimal (base-10) system and the binary (base-2) system. The decimal system is a base-10 number system used in everyday life, while the binary system is a base-2 number system used in computers.
In this article, we'll look at the process of converting a number from decimal to binary in C programming. We will discuss multiple approaches that can be used to accomplish this goal. Let's look at the fundamentals of number systems, binary numbers, and decimal representations before exploring the various techniques we can use to write and run C programs for decimal to binary conversion.
What Is A Decimal Number?
Decimal numbers belong to the decimal system and are symbolized by digits ranging from 0 to 9. The concept of a base is crucial for representing numbers, and decimal numbers are established on the foundation of base 10, a widely recognized system worldwide. This base system also forms the basis for converting a number from decimal to binary in C programming and other fields.
In programming, decimal numbers are represented using the 64-bit floating data type or double type variable, which is chosen based on the required level of precision. The type float consumes 4 bytes and ensures accuracy within the range of 6-7 decimal digits, while the double type occupies 8 bytes and offers precision spanning 15-16 decimal digits.
What Is A Binary Number?
Binary numbers belong to the binary system and are represented using only two digits 0 and 1. The binary system is built upon the base 2, which is used whenever converting a number from decimal to binary in C or vice versa.
In the context of C programming, binary numbers find their representation using the int or long int data types, which respectively allocate memory space in the sizes of 32 or 64 bits. Binary numbers are denoted by the prefix "0b" in C, followed by a sequence of 0s and 1s, a distinctive feature streamlining their identification and utilization.
Representation Of Decimal & Binary Numbers
The decimal system uses 10 digits: 0, 1, 2, 3, 4, 5, 6, 7, 8, and 9. Each position in a decimal number represents a power of 10. The rightmost position represents 10^0 (which is 1), the next position to the left represents 10^1 (which is 10), then 10^2 (which is 100), and so on.
For example, the decimal number 321 is interpreted as: 3 * 10^2 + 2 * 10^1 + 1 * 10^0 = 300 + 20 + 1 = 321.
In the binary system, we have only 2 digits: 0 and 1. Each position in a binary number represents a power of 2. The rightmost position represents 2^0 (which is 1), the next position to the left represents 2^1 (which is 2), then 2^2 (which is 4), and so on.
For example, the binary number 1011 can be interpreted as follows:
The rightmost digit, 1, represents 1 * 2^0 = 1.
The second rightmost digit, 1, represents 1 * 2^1 = 2.
The third rightmost digit, 0, represents 0 * 2^2 = 0.
The leftmost digit, 1, represents 1 * 2^3 = 8. So, 1011 in binary is equal to: 1 * 8 + 0 * 4 + 1 * 2 + 1 * 1 = 8 + 0 + 2 + 1 = 11.
Algorithm For Converting Decimal To Binary In C
A straightforward method for converting a number from decimal to a binary in C involves the implementation of the following algorithm:
- Begin by dividing the given number by 2 to obtain both the quotient and the remainder.
- If the remainder is found to be 0, the corresponding digit in the binary number is also 0.
- Similarly, if the remainder is 1, then the associated binary digit becomes 1.
- Proceed by applying the same process iteratively to the quotient and the new remainder obtained.
- Throughout each step, consistently attach the acquired remainder to the binary number representation.
For Example, the conversion of a decimal number 17 to binary can be done as follows:
Step 1: Remainder when 17 is divided by 2 is 1. Therefore, arr[0] = 1.
Step 2: Divide 17 by 2. The new number is 17 / 2 = 8.
Step 3: Remainder when 8 is divided by 2 is 0. Therefore, arr[1] = 0.
Step 4: Divide 8 by 2. The new number is 8 / 2 = 4.
Step 5: Remainder when 4 is divided by 2 is 0. Therefore, arr[2] = 0.
Step 6: Divide 4 by 2. The new number is 4 / 2 = 2.
Step 7: Remainder when 2 is divided by 2 is 0. Therefore, arr[3] = 0.
Step 8: Divide 2 by 2. The new number is 2 / 2 = 1.
Step 9: Remainder when 1 is divided by 2 is 1. Therefore, arr[4] = 1.
Step 10: Divide 1 by 2. The new number is 1 / 2 = 0.
Step 11: Since the number becomes 0, print the array in reverse order.
Therefore, the equivalent binary form for decimal number 17 is 10001. Let's look at the various techniques, control statements/ contrasts, operators, etc., we can use to write and run a C program for decimal to binary conversion.
Convert Decimal To Binary In C Using Naive Approach/ Division Optimization (Without Arrays)
The naive approach is the most simplistic approach to solving a problem in programming. When writing a program to convert numbers from decimal to binary in C language, this approach involves the division operations/ division method. We carry out the conversion by iteratively dividing the decimal number by 2 and accumulating the remainder to construct the binary representation.
The simple C program example below showcases how to implement this approach to successfully carry out the conversion from decimal to binary.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+Cgpsb25nIGRlY2ltYWxUb0JpbmFyeShsb25nIG4pIHsKbG9uZyBhbnMgPSAwOwpsb25nIHJlID0gMCwgcHYgPSAxOwoKd2hpbGUgKG4gPiAwKSB7CnJlID0gbiAlIDI7CmFucyA9IGFucyArIHJlICogcHY7CnB2ID0gcHYgKiAxMDsKbiA9IG4gLyAyOwp9CnJldHVybiBhbnM7Cn0KCmludCBtYWluKCkgewoKbG9uZyBuOwpwcmludGYoIkVudGVyIGEgZGVjaW1hbCBudW1iZXIgeW91IHdhbnQgdG8gY29udmVydDogIik7CnNjYW5mKCIlbGQiLCAmbik7CgpwcmludGYoIlRoZSBudW1iZXIgaW4gYmluYXJ5IGlzOiAlbGQiLCBkZWNpbWFsVG9CaW5hcnkobikpOwoKcmV0dXJuIDA7Cn0=
Output:
Enter a decimal number you want to convert: 17
The number in binary is: 10001
Explanation:
In the example C code, we begin by including the essential header file <stdio.h> for input/ output operations.
- We then define a function decimalToBinary() that takes a single parameter n of type long, representing the decimal number.
- The function returns a long integer representing its binary equivalent of decimal input. Inside the function-
- We first initialize a long data type variable ans with the value 0, to accumulate the binary representation.
- Then, we initialize two more long type variables in the same line using the comma operator. The variable re to store the remainder of n divided by 2 and variable pv to keep track of place value of binary digits, initialized with values 0 and 1, respectively.
- Next, we employ a while loop to convert n to its binary representation through the iterative process till the condition n>0 is satisfied.
- In every iteration, we first use the modulus operator (arithmetic operator) to calculate the remainder of n divided by 2 and store it in the variable re.
- Then, we update ans by adding re * pv, where pv represents the current place value of the binary digit.
- We then multiply pv by 10 to move to the next place value and update n by dividing it by 2.
- This process repeats until n becomes 0, and the loop continues to accumulate the binary digits of the number in variable ans.
- Once n becomes 0, the entire decimal number will be converted to binary, and the function returns this value stored in the variable ans.
- In the main() function, we declare a variable n (long type) and then prompt the user to enter a decimal number using the printf() function.
- We then use the scanf() function to read the value and store it in the corresponding variable using the address-of operator (&). The %ld format specifier is the placeholder for the long type value.
- We call the decimalToBinary() function with the input decimal number n as a parameter and print the result with the original number using the printf() function.
- Finally, the main() function terminates with a return 0 statement indicating successful execution.
The time complexity of this code is O(log n).
The space complexity is O(1).
Convert Decimal To Binary In C Using While Loop (User Input)
In this approach, we use a while loop to repeatedly divide the input number (decimal) by 2 and accumulate the remainder (0 or 1) in an array of binary digits. The conditions are set such that on the completion of loop iterations, the array will contain the binary equivalent of the input decimal value.
Please note that this decimal conversion code doesn't handle negative decimal numbers or the two's complement form. That is, it focuses solely on converting positive numbers from decimal to binary. The example C program below showcases the implementation of this approach to convert decimal to binary in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovL0RlZmluaW5nIGEgZnVuY3Rpb24gdG8gY29udmVydCBkZWNpbWFsIHRvIGJpbmFyeSB1c2luZyB3aGlsZSBsb29wCnZvaWQgZGVjaW1hbFRvQmluYXJ5KGludCBuKSB7CmlmIChuID09IDApIHsKcHJpbnRmKCJCaW5hcnk6IDBcbiIpOwpyZXR1cm47fQoKaW50IGJpbmFyeVszMl07IC8vIEFzc3VtaW5nIDMyLWJpdCBpbnRlZ2VycwppbnQgaW5kZXggPSAwOwp3aGlsZSAobiA+IDApIHsKYmluYXJ5W2luZGV4XSA9IG4gJSAyOwpuIC89IDI7CmluZGV4Kys7Cn0KCnByaW50ZigiVGhlIGJpbmFyeSByZXByZXNlbnRhdGlvbiBvZiBkZWNpbWFsIGlucHV0IGlzOiIpOwpmb3IgKGludCBpID0gaW5kZXggLSAxOyBpID49IDA7IGktLSkgewpwcmludGYoIiVkIiwgYmluYXJ5W2ldKTt9CnByaW50ZigiXG4iKTsKfQoKaW50IG1haW4oKSB7CgovL0RlY2xhcmluZyB2YXJpYWJsZSB3aGljaCB3ZSB3aWxsIGNvbnZlcnQgdG8gYmluYXJ5IGFuZCBhc2tpbmcgZm9yIHVzZXIgaW5wdXQKaW50IG47CnByaW50ZigiRW50ZXIgYSBkZWNpbWFsIG51bWJlciB5b3Ugd2FudCB0byBjb252ZXJ0OiAiKTsKc2NhbmYoIiVkIiwgJm4pOwoKaWYgKG4gPCAwKSB7CnByaW50ZigiQmluYXJ5IHJlcHJlc2VudGF0aW9uIG9mIG5lZ2F0aXZlIG51bWJlcnMgaXMgbm90IHN1cHBvcnRlZC5cbiIpOwp9IGVsc2UgewpkZWNpbWFsVG9CaW5hcnkobik7Cn0KCnJldHVybiAwOwp9
Output:
Enter a decimal number you want to convert: 23
The binary representation of decimal input is:10111
Explanation:
In the C code example-
- We define a function decimalToBinary() that takes a single parameter n, representing the decimal number to be converted to binary.
- The function type is void since it will not return a value of a specific data type. Inside the function-
- We first check if the input value n equals 0, using an if-statement and equality relational operator. If n==0 is true, the printf() statement displays the string message- "Binary: 0" as 0 in binary is just 0. And the function terminates.
- If n==0 is false, we move to the next part of the function and declare an integer array called binary to store the binary representation of the number. As mentioned in the code comment, we assume a size of 32 bits since it's common for integers (32-bit binary representation).
- Next, we initialize an integer variable index to keep track of the current position in the binary array with the value 0.
- We then create a while loop to carry out the binary conversion of input decimal n.
- In every iteration of the loop, we store the remainder of n divided by 2 in the binary array element at the position given by index variable.
- We then update n by dividing it by 2 and increment the index variable by 1 to move to the next position in the binary array.
- The while loop continues iterating until n>0, after which it temrinates, and the binary array will contain the binary representation of decimal input.
- After that, we use a for loop to print the binary representation by iterating through the binary array in reverse order and printing each element as we go.
- In the main() function, we declare an integer variable n and store user-generated value in it using printf() and scanf() functions.
- Following this, we use an if-else statement to first check if the entered number is negative.
- If n<0 is true, then the if-block is executed, printing a message stating that the binary representation of negative numbers is not supported.
- Otherwise, the else-block is executed, calling the decimalToBinary() function with the entered decimal number (n).
- The function prints the binary representation of the input decimal number.
The time complexity of this code is O(log n).
The space complexity is O(1).
Converting Decimal To Binary In C Using Stack
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. This means that the last element added to the stack is the first one to be removed. It's similar to a stack of plates or books, where you can only add or remove items from the top.
In this approach to convert a number from decimal to binary in C, we implement a simple stack data structure using the struct keyword. We then define a function that converts a decimal number to binary using that stack to hold the binary digits in reverse order. After pushing all the digits onto the stack, we pop them one by one to print the binary representation correctly. The C program example below provides the implementation of this approach.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdGRib29sLmg+CgovLyBEZWZpbmUgYSBzaW1wbGUgc3RhY2sgc3RydWN0dXJlCnN0cnVjdCBTdGFjayB7CmludCBkYXRhWzMyXTsgLy8gQXNzdW1pbmcgMzItYml0IGludGVnZXJzCmludCB0b3A7Cn07CgovLyBGdW5jdGlvbiB0byBpbml0aWFsaXplIHRoZSBzdGFjawp2b2lkIGluaXRpYWxpemUoc3RydWN0IFN0YWNrICpzdGFjaykgewpzdGFjay0+dG9wID0gLTE7Cn0KCi8vIEZ1bmN0aW9uIHRvIGNoZWNrIGlmIHRoZSBzdGFjayBpcyBlbXB0eQpib29sIGlzRW1wdHkoc3RydWN0IFN0YWNrICpzdGFjaykgewpyZXR1cm4gc3RhY2stPnRvcCA9PSAtMTsKfQoKLy8gRnVuY3Rpb24gdG8gcHVzaCBhbiBlbGVtZW50IG9udG8gdGhlIHN0YWNrCnZvaWQgcHVzaChzdHJ1Y3QgU3RhY2sgKnN0YWNrLCBpbnQgdmFsdWUpIHsKc3RhY2stPnRvcCsrOwpzdGFjay0+ZGF0YVtzdGFjay0+dG9wXSA9IHZhbHVlOwp9CgovLyBGdW5jdGlvbiB0byBwb3AgYW4gZWxlbWVudCBmcm9tIHRoZSBzdGFjawppbnQgcG9wKHN0cnVjdCBTdGFjayAqc3RhY2spIHsKaW50IHZhbHVlID0gc3RhY2stPmRhdGFbc3RhY2stPnRvcF07CnN0YWNrLT50b3AtLTsKcmV0dXJuIHZhbHVlOwp9CgovLyBGdW5jdGlvbiB0byBjb252ZXJ0IGRlY2ltYWwgdG8gYmluYXJ5IHVzaW5nIGEgc3RhY2sKdm9pZCBkZWNpbWFsVG9CaW5hcnkoaW50IG4pIHsKaWYgKG4gPT0gMCkgewpwcmludGYoIkJpbmFyeTogMFxuIik7CnJldHVybjsKfQoKc3RydWN0IFN0YWNrIHN0YWNrOwppbml0aWFsaXplKCZzdGFjayk7CndoaWxlIChuID4gMCkgewpwdXNoKCZzdGFjaywgbiAlIDIpOwpuIC89IDI7Cn0KCnByaW50ZigiVGhlIGJpbmFyeSByZXByZXNlbnRhaW9uIG9mIGRlY2ltYWwgaW5wdXQgaXM6ICIpOwp3aGlsZSAoIWlzRW1wdHkoJnN0YWNrKSkgewpwcmludGYoIiVkIiwgcG9wKCZzdGFjaykpOwp9CnByaW50ZigiXG4iKTsKfQoKaW50IG1haW4oKSB7CgppbnQgbjsKcHJpbnRmKCJFbnRlciBhIGRlY2ltYWwgbnVtYmVyIHlvdSB3YW50IHRvIGNvbnZlcnQ6ICIpOwpzY2FuZigiJWQiLCAmbik7CgppZiAobiA8IDApIHsKcHJpbnRmKCJCaW5hcnkgcmVwcmVzZW50YXRpb24gb2YgbmVnYXRpdmUgbnVtYmVycyBpcyBub3Qgc3VwcG9ydGVkLlxuIik7Cn0gZWxzZSB7CmRlY2ltYWxUb0JpbmFyeShuKTsKfQoKcmV0dXJuIDA7Cn0=
Output:
Enter a decimal number you want to convert: 12
The binary representaion of decimal input is: 1100
Explanation:
In the sample C code, we include the <stidio.h> and <stdbool.h> header for input-output and boolean data type support, respectively.
- We then define a simple stack structure named Stack, which consists of an integer array named data to store elements and an integer top to keep track of the top element's index.
- Then, we define four functions to work with the stack as follows:
- The initialize() function takes the pointer to the structure Stack as input and initializes the stack by setting the top index to -1. We use the arrow operator (this pointer) to access the elements.
- Then, the isEmpty() function is defined to take pointer to Stack as input and check if the top element is -1 to determine if the structure is empty. It returns a boolean value true (indicating it is empty) and false (indicating it is not empty).
- Next, we define the push() function to add/ push an element onto the stack by incrementing the top index and storing the value at that index. It takes the pointer to Stack and an integer value to be added to the structure as parameters.
- We then define the pop() function to pop an element from the stack by retrieving the value at the top index and decrementing the top index.
- After that, we define a function called decimalToBinary() that takes a single parameter n, representing the decimal number to be converted to binary.
- In this function, we first check if the input n equals 0 using an if statement. If n==0 is true, the function prints the string- "Binary: 0" to the console, as 0 in binary is just 0.
- if the condition n==0 is false, we initialize a stack by calling the initialize function and entering a while loop to convert n to its binary representation.
- In every iteration of the loop, we call the push() function to add/ push the remainder of n divided by 2 onto the stack and update n by dividing it by 2.
- After converting n to binary, we use the printf() function inside a while loop to print the binary representation.
- For this, we call the pop function by passing reference to the stack as an argument, which pops elements from the stack until it is empty.
- The function closes with a printf() statement using a newline escape sequence to shift the cursor to the next line.
- Moving onto the main() function, we declare an integer variable n and store the user provided decimal value in it.
- After that, we check if the entered number is negative using an if-else statement.
- If the statement condition n<0 is true, the if-block prints the message stating that the binary representation of negative numbers is not supported.
- If the condition n<0 is false, the else-block calls the decimalToBinary() function with the entered decimal number (n).
The time complexity of this code is O(log n).
The space complexity is O(1).
Converting Decimal To Binary In C Using Bitwise Operators
Bitwise operators are used to carry out manipulations on numbers, variables or elements at the bit-level. We can use these operators to convert a number from decimal to binary in C programs. For this, you must define a function/ condition that iterates through each bit position of the number from the most significant bit (31) to the least significant bit (0). The mask is used to isolate each bit, and the result is printed as 1 or 0 to represent the binary number.
The sample C program below gives an implementation of how to use bitwise operators to convert a number from deciaml to binary in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+Cgp2b2lkIGRlY2ltYWxUb0JpbmFyeShpbnQgbnVtKSB7CmludCBsZWFkaW5nWmVyb3MgPSAxOyAvLyBGbGFnIHRvIHRyYWNrIGxlYWRpbmcgemVyb3MKLy8gYXNzdW1pbmcgMzItYml0IGludGVnZXIKCnByaW50ZigiVGhlIGJpbmFyeSBlcXVpdmFsZW50IG9mIGRlY2ltYWwgaW5wdXQgaXM6Iik7CmZvciAoaW50IGkgPSAzMTsgaSA+PSAwOyBpLS0pIHsKaW50IG1hc2sgPSAoMSA8PCBpKTsKaWYgKG51bSAmIG1hc2spIHsKbGVhZGluZ1plcm9zID0gMDsgLy8gU2V0IHRoZSBmbGFnIHRvIGluZGljYXRlIG5vIG1vcmUgbGVhZGluZyB6ZXJvcwpwcmludGYoIjEiKTsKfSBlbHNlIGlmICghbGVhZGluZ1plcm9zKSB7CnByaW50ZigiMCIpO30KfQoKaWYgKGxlYWRpbmdaZXJvcykgewpwcmludGYoIjAiKTsgLy8gSWYgdGhlIG51bWJlciBpcyB6ZXJvLCBwcmludCBhIHNpbmdsZSB6ZXJvCn0KcHJpbnRmKCJcbiIpOwp9CgppbnQgbWFpbigpIHsKCmludCBudW07CnByaW50ZigiRW50ZXIgYSBkZWNpbWFsIG51bWJlciB5b3Ugd2FudCB0byBjb252ZXJ0OiAiKTsKc2NhbmYoIiVkIiwgJm51bSk7CgpkZWNpbWFsVG9CaW5hcnkobnVtKTsKCnJldHVybiAwOwp9
Output:
Enter a decimal number you want to convert: 14
The binary equivalent of decimal input is:1110
Explanation:
In the C code sample-
- We define a function called decimalToBinary() that takes a single parameter, num, representing the decimal number to be converted to binary.
- In this function, we initialize an integer variable leadingZeros to 1, which will act as a flag to track whether the leading zeros are in the binary representation.
- Then we enter a for loop to iterate over each bit position from the most significant bit (31) to the least significant bit (0), assuming a 32-bit integer
- In the loop, we create a variable mask, which will be the bitmask, by left shifting 1 by i bits (Using the left shift bitwise operator).
- We then perform a bitwise AND operation between num and mask to check if the bit at position i is 1, using an if-else statement
- If the bit is 1 and there are no leading zeros, we print "1". Else, if the bit is 0 and there are no leading zeros, we print "0". This approach ensures that we skip leading zeros in the binary representation.
- After that, we check if the number is zero, and in case there are leading zeros, we print a single "0" to represent the binary zero.
- Finally, the decimalToBinary() function ends with a newline character in the printf() statement to end the output.
- In the main() function, we declare an integer variable num and ask the user to provide a decimal number to store it in. This is the number we will convert from decimal to binary.
- Following that, we call the decimalToBinary() function with the decimal number num as an argument.
- The invoked function calculates and prints the binary equivalent of the num variable to the console and the program terminates.
The time complexity of this code is O(1).
The space complexity is O(1).
C Program to Convert Decimal to Binary Using Functions
In this approach, we convert a number from decimal to binary form using a custom function. The function takes an integer number, a character buffer, and the desired base as input. It converts the integer to its ASCII representation in the specified base and stores the result in the buffer. The C program sample below gives an implementation of this approach to convert a number from decimal to binary in C language.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxtYXRoLmg+CiNpbmNsdWRlIDxzdGRsaWIuaD4KCmNoYXIqIGl0b2EoaW50IG51bSwgY2hhciogYnVmZmVyLCBpbnQgYmFzZSkgewppbnQgY3VycmVudCA9IDA7CgppZiAobnVtID09IDApIHsKYnVmZmVyW2N1cnJlbnQrK10gPSAnMCc7CmJ1ZmZlcltjdXJyZW50XSA9ICdcMCc7CnJldHVybiBidWZmZXI7Cn0KCmludCBudW1fZGlnaXRzID0gMDsKaWYgKG51bSA8IDApIHsKaWYgKGJhc2UgPT0gMTApIHsKbnVtX2RpZ2l0cysrOwpidWZmZXJbY3VycmVudF0gPSAnLSc7CmN1cnJlbnQrKzsKbnVtICo9IC0xOwp9IGVsc2UKcmV0dXJuIE5VTEw7Cn0KCm51bV9kaWdpdHMgKz0gKGludClmbG9vcihsb2cobnVtKSAvIGxvZyhiYXNlKSkgKyAxOwp3aGlsZSAoY3VycmVudCA8IG51bV9kaWdpdHMpIHsKaW50IGJhc2VfdmFsID0gKGludCkgcG93KGJhc2UsIG51bV9kaWdpdHMgLSAxIC0gY3VycmVudCk7CmludCBudW1fdmFsID0gbnVtIC8gYmFzZV92YWw7CmNoYXIgdmFsdWUgPSBudW1fdmFsICsgJzAnOwpidWZmZXJbY3VycmVudF0gPSB2YWx1ZTsKY3VycmVudCsrOwpudW0gLT0gYmFzZV92YWwgKiBudW1fdmFsOwp9CmJ1ZmZlcltjdXJyZW50XSA9ICdcMCc7CnJldHVybiBidWZmZXI7Cn0KCmludCBtYWluKCkgewoKaW50IG47CnByaW50ZigiRW50ZXIgYSBkZWNpbWFsIG51bWJlciB5b3Ugd2FudCB0byBjb252ZXJ0OiAiKTsKc2NhbmYoIiVkIiwgJm4pOwoKY2hhciBidWZmZXJbMjU2XTsKCmlmIChpdG9hKG4sIGJ1ZmZlciwgMikgIT0gTlVMTCkgewpwcmludGYoIlRoZSBiaW5hcnkgcmVwcmVzZW50YXRpb24gb2YgaW5wdXQgZGVjaW1hbCBudW1iZXIgaXM6ICVzXG4iLGJ1ZmZlcik7Cn0gZWxzZSB7CnByaW50ZigiQ29udmVyc2lvbiBub3Qgc3VwcG9ydGVkLlxuIik7Cn0KCnJldHVybiAwOwp9
Output:
Enter a decimal number you want to convert: 13
The binary representation of input decimal number is: 1101
Explanation:
In the C code-
- We include the necessary header files, i.e., <stdio.h> for input/output operations, <math.h> for mathematical functions, and <stdlib.h> for memory allocation.
- Then, we define a function itoa(), that takes three parameters: num (representing the decimal number to be converted), buffer (representing the character buffer to store the resulting string), and base (representing the base of the desired output which in this case is binary).
- In this function, we declare a variable current to keep track of the current position in the buffer and initialize it to 0.
- After that, we use an if-statement to verify if the input variable num equals 0. If the condition num==0 is true, we handle this special case by storing '0' in the buffer and returning it.
- If the condition is false, we move to calculate the number of digits required to represent the number in the specified base. For this, we first initialize an integer variable num_digits to 0.
- Next, we check if the value of num is negative (i.e., num<0) and the base is 10 (i.e., base==10).
- If both conditions are true, we increment the num_digits variable to accommodate the negative sign. Otherwise, the statement returns NULL.
- We then enter a while loop to convert num to its binary representation.
- Within the loop, we calculate the value of the most significant digit using the base and subtract it from num.
- We repeat this process until we have converted all digits.
- Finally, we add the null terminator '\0' to the buffer to mark the end of the string and return the buffer.
- In the main() function, we prompt the user to enter a decimal number, read it using scanf, and store it in the variable n.
- After that, we declare a character variable, buffer to store the resulting binary string.
- Next, we call the itoa() function inside an if-else statement, with the decimal value in n, buffer, and the base 2 (for binary conversion) as arguments.
- If the conversion is successful (the function does not return NULL), we print the binary representation stored in the buffer.
- Else, if the conversion is not supported (for example, if the base is not 10 for negative numbers), we print a message stating that the conversion is not supported.
The time complexity of this code is O(log n).
The space complexity is O(1).
Converting Decimal To Binary In C Using For Loop
The for loop is a popular control statement that is used to resolve many programming issues through iteration. In this, you must use the loop to iteratively calculate the remainder of the decimal number divided by 2 and use it to construct the binary representation. Look at the program example below to understand how to use this approach to convert a number from decimal to binary in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+Cgp2b2lkIGRlY2ltYWxUb0JpbmFyeShpbnQgbikgewppZiAobiA9PSAwKSB7CnByaW50ZigiQmluYXJ5OiAwXG4iKTsKcmV0dXJuOwp9CmludCBiaW5hcnkgPSAwOwppbnQgcGxhY2UgPSAxOwpmb3IgKGludCB0ZW1wID0gbjsgdGVtcCA+IDA7IHRlbXAgLz0gMikgewppbnQgYml0ID0gdGVtcCAlIDI7CmJpbmFyeSArPSBiaXQgKiBwbGFjZTsKcGxhY2UgKj0gMTA7Cn0KcHJpbnRmKCJUaGUgbnVtYmVyIGluIGJpbmFyeSByZXByZXNlbnRhdGlvbiBpczolZFxuIiwgYmluYXJ5KTsKfQoKaW50IG1haW4oKSB7CgppbnQgbjsKcHJpbnRmKCJFbnRlciBhIGRlY2ltYWwgbnVtYmVyIHlvdSB3YW50IHRvIGNvbnZlcnQ6ICIpOwpzY2FuZigiJWQiLCAmbik7CgppZiAobiA8IDApIHsKcHJpbnRmKCJCaW5hcnkgcmVwcmVzZW50YXRpb24gb2YgbmVnYXRpdmUgbnVtYmVycyBpcyBub3Qgc3VwcG9ydGVkLlxuIik7Cn0gZWxzZSB7CmRlY2ltYWxUb0JpbmFyeShuKTsKfQoKcmV0dXJuIDA7Cn0=
Output:
Enter a decimal number you want to convert: 9
The number in binary representation is:1001
Explanation:
In the code-
- We define a function called decimalToBinary() that takes a single parameter n, representing the decimal number to be converted to binary.
- In the function, we first check if the input n equals 0. If it is, then we print "Binary: 0" and return, as 0 in binary is just 0.
- Otherwise, we declare two variables: binary to accumulate the binary representation and place to keep track of the place value of the binary digits. We initialize these variables with values 0 and 1, respectively.
- After that, we create a for loop where we initialize a temporary variable temp with the value of n. The loop continues as long as temp is greater than 0, and its value is updated by dividing it by 2 after every iteration.
- In the loop, we calculate the least significant bit of temp by storing the remainder of temp divided by 2 in the variable bit.
- We then update the binary variable by adding bit * place, where place represents the current place value of the binary digit.
- We also update the place variable by multiplying its value by 10 (to move to the next place value) and reassigning that value using a compound assignment operator.
- Once the loop completes execution, it has converted the entire decimal number to binary form stored in the variable binary.
- We print this value to the console using a printf() statement, and the function closes.
- In the main() function, we prompt the user to enter a decimal number, read it using scanf, and store it in the variable n.
- Next, we use an if-else statement to check if the entered number is negative. If it is, we print a message stating that the binary representation of negative numbers is not supported.
- Otherwise, we call the decimalToBinary() function (contained in the else-block) with the decimal value in variable n as an argument.
- The function calculates and prints the binary representation of the input number to the console.
The time complexity of this code is O(log n).
The space complexity is O(1).
Converting Decimal To Binary In C Using Math Module
There are many library functions in the <math.h> header defined especially to carry out mathematical operations on inputs. For example, we can use the power function, i.e., the pow() function from this library, to calculate the powers of 10 for positions of the binary digits of a number.
Given below is an example program in C that shows how we can use the pow() function with the while loop to repeatedly divide the decimal number by 2 and build up the binary representation of the same.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxtYXRoLmg+Cgpsb25nIGRlY2ltYWxUb0JpbmFyeShpbnQgbikgewpsb25nIGJpbmFyeSA9IDA7CmludCByZW1haW5kZXIsIGNvdW50ID0gMDsKd2hpbGUgKG4gPiAwKSB7CnJlbWFpbmRlciA9IG4gJSAyOwpiaW5hcnkgKz0gcmVtYWluZGVyICogcG93KDEwLCBjb3VudCk7Cm4gLz0gMjsKY291bnQrKzsKfQpyZXR1cm4gYmluYXJ5Owp9CgppbnQgbWFpbigpIHsKCmludCBuOwpwcmludGYoIkVudGVyIGEgZGVjaW1hbCBudW1iZXIgeW91IHdhbnQgdG8gY29udmVydDogIik7CnNjYW5mKCIlZCIsICZuKTsKCmlmIChuIDwgMCkgewpwcmludGYoIkJpbmFyeSByZXByZXNlbnRhdGlvbiBvZiBuZWdhdGl2ZSBudW1iZXJzIGlzIG5vdCBzdXBwb3J0ZWQuXG4iKTsKfSBlbHNlIHsKbG9uZyBiaW5hcnkgPSBkZWNpbWFsVG9CaW5hcnkobik7CnByaW50ZigiVGhlIGJpbmFyeSBmb3JtIG9mIHRoZSBudW1iZXIgaXM6ICVsZFxuIixiaW5hcnkpOwp9CgpyZXR1cm4gMDsKfQ==
Output:
Enter a decimal number you want to convert: 67
The binary form of the number is: 1000011
Explanation:
In the code-
- We begin by defining a function decimalToBinary() that takes a single parameter n, representing the decimal number to be converted to binary, and returns a long integer representing its binary equivalent.
- In the function, we initialize two variables, i.e., binary (to accumulate the binary representation) with the value 0 and count (to keep track of the position of each binary digit) with the value 1.
- Then, we enter a while loop where we repeatedly divide n by 2 and store the remainder in the variable remainder.
- We then update the binary variable by adding remainder * pow(10, count), where pow(10, count) calculates the appropriate place value of the binary digit.
- After that, we increment count variable to move to the next place value.
- The loop terminates iteration once n becomes 0, at which point we have converted the entire decimal number to binary.
- The function returns the binary variable, which stores the binary form of the input decimal number.
- In the main() function, we prompt the user to enter a decimal number, read it using scanf, and store it in the int variable n.
- After that, we employ an if-else statement, which first checks if the input value is negative. If the condition n<0 is true, we print a message stating that the binary representation of negative numbers is not supported.
- Otherwise, the else-block calls the decimalToBinary() function with variable n as an argument.
- The outcome of the function's execution is stored in a long type variable binary.
- Lastly, we use a printf() statement to display this binary representation of the entered decimal number.
The time complexity of this code is O(log n).
The space complexity is O(1).
Check out this amazing course to become the best version of the C programmer you can be.
Converting Decimal To Binary In C Using Right Shift With AND Operator
In this code, we use bitwise operators to extract the least significant bit (bit) of the decimal number in each iteration of the loop. Then, we use the bitwise AND operator (&) and the right shift operator (>>) for right shifting to remove the least significant bit. This approach is efficient and uses the inherent binary nature of numbers to perform the conversion.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+Cgp2b2lkIGRlY2ltYWxUb0JpbmFyeShpbnQgbikgewppZiAobiA9PSAwKSB7CnByaW50ZigiQmluYXJ5OiAwXG4iKTsKcmV0dXJuOwp9CmludCBiaW5hcnkgPSAwOwppbnQgcGxhY2UgPSAxOwp3aGlsZSAobiA+IDApIHsKaW50IGJpdCA9IG4gJiAxOyAvLyBHZXQgdGhlIGxlYXN0IHNpZ25pZmljYW50IGJpdCB1c2luZyBiaXR3aXNlIEFORApiaW5hcnkgKz0gYml0ICogcGxhY2U7CnBsYWNlICo9IDEwOwpuID4+PSAxOyAvLyBSaWdodCBzaGlmdCBuIGJ5IDEgdG8gcmVtb3ZlIHRoZSBsZWFzdCBzaWduaWZpY2FudCBiaXQKfQpwcmludGYoIlRoZSBiaW5hcnkgZXF1aXZhbGVudCBvZiB0aGUgaW5wdXQgbnVtYmVyIGlzOiAlZFxuIiwgYmluYXJ5KTsKfQoKaW50IG1haW4oKSB7CgppbnQgbjsKcHJpbnRmKCJFbnRlciBhIGRlY2ltYWwgbnVtYmVyIHlvdSB3YW50IHRvIGNvbnZlcnQ6ICIpOwpzY2FuZigiJWQiLCAmbik7CgppZiAobiA8IDApIHsKcHJpbnRmKCJCaW5hcnkgcmVwcmVzZW50YXRpb24gb2YgbmVnYXRpdmUgbnVtYmVycyBpcyBub3Qgc3VwcG9ydGVkLlxuIik7Cn0gZWxzZSB7CmRlY2ltYWxUb0JpbmFyeShuKTsKfQoKcmV0dXJuIDA7Cn0=
Output:
Enter a decimal number you want to convert: 12
The binary equivalent of the input number is: 1100
Explanation:
In the above code-
- We define a function called decimalToBinary() that takes a single parameter n, representing the decimal number to be converted to binary.
- Within the decimalToBinary function, if the input n equals 0, we print "Binary: 0" and return, as 0 in binary is just 0.
- Otherwise, we initialize two variables: binary (to accumulate the binary representation) with value 0 and place (to keep track of the place value of the binary digits) with value 1.
- We then enter a while loop where we extract the least significant bit of n using bitwise AND (n & 1) and store it in the variable bit.
- After that, we update binary variable by adding bit * place and multiply the place variable by 10 to move to the next place value.
- After each iteration, we right-shift n by 1 (n >>= 1) to remove the least significant bit.
- Once n becomes 0, we have converted the entire decimal number to binary, and we print the result.
- In the main() function, we prompt the user to enter a decimal number, read it using scanf, and store it in the variable n.
- We check if the value of input n is negative. If it is, we print a message stating that the binary representation of negative numbers is not supported.
- Otherwise, we call the decimalToBinary() function with the entered decimal number (n).
- Finally, the program returns 0, indicating successful execution.
The time complexity of this code is O(log n).
The space complexity is O(1).
Converting Decimal To Binary In C Using Recursion
Recursion is a programming concept where a function calls itself in order to solve a problem. In other words, a function in a recursive solution is defined in terms of itself. Recursion is a powerful technique used to solve problems that can be broken down into smaller instances of the same problem.
In the code below, we convert a number from decimal to binary in C by defining a recursive function. The decimalToBinary function repeatedly divides the decimal number by 2, appending the remainder (binary digit) to the binary representation. This process continues until the decimal number becomes zero.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBGdW5jdGlvbiB0byBjb252ZXJ0IGRlY2ltYWwgdG8gYmluYXJ5IHJlY3Vyc2l2ZWx5CmxvbmcgZGVjaW1hbFRvQmluYXJ5KGxvbmcgbiwgbG9uZyBhbnMsIGxvbmcgcHYpIHsKaWYgKG4gPT0gMCkgewpyZXR1cm4gYW5zOwp9CmxvbmcgcmUgPSBuICUgMjsKYW5zID0gYW5zICsgcmUgKiBwdjsKcHYgPSBwdiAqIDEwOwpuID0gbiAvIDI7CnJldHVybiBkZWNpbWFsVG9CaW5hcnkobiwgYW5zLCBwdik7Cn0KCmludCBtYWluKCkgewpsb25nIG47CnByaW50ZigiRW50ZXIgYSBkZWNpbWFsIG51bWJlciB5b3Ugd2FudCB0byBjb252ZXJ0OiAiKTsKc2NhbmYoIiVsZCIsICZuKTsKaWYgKG4gPCAwKSB7CnByaW50ZigiTmVnYXRpdmUgbnVtYmVycyBhcmUgbm90IHN1cHBvcnRlZC5cbiIpOwpyZXR1cm4gMTsgLy8gRXhpdCB3aXRoIGVycm9yIGNvZGUKfQpwcmludGYoIlRoZSBudW1iZXIgaW4gYmluYXJ5IGlzOiAlbGRcbiIsIGRlY2ltYWxUb0JpbmFyeShuLCAwLCAxKSk7CnJldHVybiAwOwp9
Output:
Enter a decimal number you want to convert: 78.43
The number in binary is: 1001110
Explanation:
In the above code,
- We define a recursive function named decimalToBinary() that takes three parameters: n, representing the decimal number to be converted, ans, representing the accumulator for the binary conversion, and pv, representing the place value for the binary digits.
- The function first uses an if-statement to check if the input n is 0. If so, then it returns the accumulated binary result ans.
- Otherwise, if n==0 is false, the function calculates the remainder of n divided by 2 and stores it in the variable re.
- Then it updates the accumulator variable ans by adding the product of re and pv, then multiplies variable pv by 10 to update the place value, and updates n by dividing it by 2.
- After that, the function recursively calls decimalToBinary() with the updated n, ans, and pv.
- In the main() function, we prompt the user to enter a decimal number, read it using scanf, and store it in the variable n.
- Then, we check if the entered number n is negative. If it is, we print a message stating that negative numbers are not supported and return 1, indicating an error.
- Otherwise, if n is not negative, we call the decimalToBinary function with the entered decimal number (n), initial accumulator value (0), and initial place value (1).
- Finally, we use the printf() function to display the binary equivalent of the entered decimal number to the console.
The time complexity of this code is O(log n).
The space complexity is O(log n).
Converting Decimal To Binary In C For Negative Number
Converting negative decimal to binary in C involves handling the sign bit and then converting the absolute value of the number to binary using the standard conversion method.
- The solution to this is to use the two's complement approach to handle negative numbers, converting the absolute value of the number to binary and appending a negative (-) sign if the original number is negative.
- For non-negative numbers, print the binary representation directly.
The example code below shows how we can convert a negative decimal to binary in C language.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBGdW5jdGlvbiB0byBjb252ZXJ0IGRlY2ltYWwgdG8gYmluYXJ5IHVzaW5nIHR3bydzIGNvbXBsZW1lbnQKdm9pZCBkZWNpbWFsVG9CaW5hcnkoaW50IG4pIHsKLy8gQ2hlY2sgaWYgdGhlIG51bWJlciBpcyBuZWdhdGl2ZQppZiAobiA8IDApIHsKcHJpbnRmKCJCaW5hcnk6IC0iKTsKCi8vIENvbnZlcnQgdGhlIGFic29sdXRlIHZhbHVlIG9mIG4gdG8gYmluYXJ5Cm4gPSAtbjsKZm9yIChpbnQgaSA9IDMxOyBpID49IDA7IGktLSkgewppZiAobiAmICgxIDw8IGkpKSB7CnByaW50ZigiMSIpOwp9IGVsc2UgewpwcmludGYoIjAiKTsKfQp9Cn0gZWxzZSB7IC8vIElmIHRoZSBudW1iZXIgaXMgbm9uLW5lZ2F0aXZlCnByaW50ZigiQmluYXJ5OiAiKTsKaWYgKG4gPT0gMCkgewpwcmludGYoIjAiKTsKfSBlbHNlIHsKaW50IGxlYWRpbmdaZXJvcyA9IDE7CmZvciAoaW50IGkgPSAzMTsgaSA+PSAwOyBpLS0pIHsKaW50IGJpdCA9IChuID4+IGkpICYgMTsKaWYgKGJpdCkgewpsZWFkaW5nWmVyb3MgPSAwOwpwcmludGYoIjEiKTsKfSBlbHNlIGlmICghbGVhZGluZ1plcm9zKSB7CnByaW50ZigiMCIpOwp9Cn0KfQp9CnByaW50ZigiXG4iKTsKfQoKaW50IG1haW4oKSB7CmludCBudW07CnByaW50ZigiRW50ZXIgYSBkZWNpbWFsIG51bWJlciB5b3Ugd2FudCB0byBjb252ZXJ0OiAiKTsKc2NhbmYoIiVkIiwgJm51bSk7CgpkZWNpbWFsVG9CaW5hcnkobnVtKTsKCnJldHVybiAwOwp9
Output:
Enter a decimal number you want to convert: -65
Binary: -00000000000000000000000001000001
Explanation:
In the above code,
- We define a function called decimalToBinary() that takes a single parameter n, representing the decimal number to be converted to binary using two's complement representation.
- in the function, we first check if the number n is negative, i.e., n<0. If this condition is true, we print "Binary: -" to indicate that the number is negative.
- If the condition n<0 is false, we convert the absolute value of n to binary by iterating over each bit position from the most significant bit (31) to the least significant bit (0) using a for loop.
- Within the loop, we check if the corresponding bit in n is 1 using bitwise AND with a bitmask (1 << i). If it is, we print "1"; otherwise, we print "0".
- If the number n is non-negative, we print "Binary: " to indicate a positive number. If n equals 0, we simply print "0".
- Otherwise, we iterate over each bit position from the most significant bit (31) to the least significant bit (0).
- Within the loop, we shift n to the right by i bits and then perform a bitwise AND operation with 1 to extract the bit at position i. If the bit is 1, we print "1"; otherwise, we print "0". This approach ensures that we skip leading zeros in the binary representation.
- Finally, we print a newline character to end the output.
- In the main() function, we prompt the user to enter a decimal number, read it using scanf, and store it in the variable num.
- After that, we call the decimalToBinary() function with the variable num as a n argument.
- The function calculates and prints the binary equivalent of the decimal input to the console.
The time complexity of this code is O(1)
The space complexity is O(1).
Converting Floating-Point Decimal Numbers To Binary In C
Converting floating point decimal to binary in C involves separating the integer and fractional parts and handling them individually. The process is as follows:
- For the integer part, the simple decimal number is repeatedly divided by 2 until it becomes zero, and the remainders are stored to form the binary representation.
- The fractional part is converted by iteratively multiplying it by 2 and extracting the integer part of the result, representing each binary digit.
- Finally, the integer and fractional binary representations are combined to form the complete binary representation of the decimal number.
It is important to handle the zero fractional parts correctly and to determine the precision of the conversion to ensure accurate results. Look at th example below to understand this approach of converting a floating-point decimal to binary in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxtYXRoLmg+CgovLyBGdW5jdGlvbiB0byBjb252ZXJ0IGZyYWN0aW9uYWwgcGFydCB0byBiaW5hcnkKdm9pZCBjb252ZXJ0RnJhY3Rpb24oZG91YmxlIGZyYWN0aW9uKSB7CnByaW50ZigiLiIpOwoKd2hpbGUgKGZyYWN0aW9uID4gMCkgewovLyBNdWx0aXBseSBmcmFjdGlvbiBieSAyCmZyYWN0aW9uICo9IDI7CgovLyBJZiB0aGUgcmVzdWx0IGlzID49IDEsIG91dHB1dCAxIGFuZCBzdWJ0cmFjdCAxCmlmIChmcmFjdGlvbiA+PSAxKSB7CnByaW50ZigiMSIpOwpmcmFjdGlvbiAtPSAxOwp9IGVsc2UgewpwcmludGYoIjAiKTsKfQp9Cn0KCi8vIEZ1bmN0aW9uIHRvIGNvbnZlcnQgZGVjaW1hbCB0byBiaW5hcnkKdm9pZCBkZWNpbWFsVG9CaW5hcnkoZG91YmxlIGRlY2ltYWwpIHsKLy8gSGFuZGxlIG5lZ2F0aXZlIG51bWJlcnMKaWYgKGRlY2ltYWwgPCAwKSB7CnByaW50ZigiLSIpOwpkZWNpbWFsID0gZmFicyhkZWNpbWFsKTsKfQoKLy8gRXh0cmFjdCBpbnRlZ2VyIHBhcnQKaW50IGludGVnZXJQYXJ0ID0gKGludCkgZGVjaW1hbDsKCi8vIEV4dHJhY3QgZnJhY3Rpb25hbCBwYXJ0CmRvdWJsZSBmcmFjdGlvbmFsUGFydCA9IGRlY2ltYWwgLSBpbnRlZ2VyUGFydDsKCi8vIENvbnZlcnQgaW50ZWdlciBwYXJ0IHRvIGJpbmFyeQpwcmludGYoIkludGVnZXIgcGFydDogJWRcbkJpbmFyeSBlcXVpdmFsZW50OiAiLCBpbnRlZ2VyUGFydCk7CmlmIChpbnRlZ2VyUGFydCA9PSAwKSB7CnByaW50ZigiMCIpOwp9IGVsc2UgewppbnQgYmluYXJ5WzMyXTsgLy8gQXJyYXkgdG8gc3RvcmUgYmluYXJ5IGRpZ2l0cwppbnQgaSA9IDA7Cgp3aGlsZSAoaW50ZWdlclBhcnQgPiAwKSB7CmJpbmFyeVtpXSA9IGludGVnZXJQYXJ0ICUgMjsKaW50ZWdlclBhcnQgLz0gMjsKaSsrOwp9CgovLyBQcmludGluZyBiaW5hcnkgZGlnaXRzIGluIHJldmVyc2Ugb3JkZXIKZm9yIChpbnQgaiA9IGkgLSAxOyBqID49IDA7IGotLSkgewpwcmludGYoIiVkIiwgYmluYXJ5W2pdKTsKfQp9CgovLyBDb252ZXJ0IGZyYWN0aW9uYWwgcGFydCB0byBiaW5hcnkKaWYgKGZyYWN0aW9uYWxQYXJ0ID4gMCkgewpjb252ZXJ0RnJhY3Rpb24oZnJhY3Rpb25hbFBhcnQpOwp9Cn0KCmludCBtYWluKCkgewpkb3VibGUgZGVjaW1hbDsKcHJpbnRmKCJFbnRlciBhIGRlY2ltYWwgbnVtYmVyOiAiKTsKc2NhbmYoIiVsZiIsICZkZWNpbWFsKTsKCmRlY2ltYWxUb0JpbmFyeShkZWNpbWFsKTsKCnJldHVybiAwOwp9
Output:
Enter a decimal number: 17.17
Integer part: 17
Binary equivalent: 10001.0010101110000101000111101011100001010001111011
Explanation:
In the code-
- We begin by defining two functions, namely, convertFraction() and decimalToBinary().
- The convertFraction() function takes a double parameter fraction, representing the fractional part of a decimal number, and converts it to binary.
- It starts by printing a decimal point (.) and then enters a loop.
- Within the loop, it multiplies the fraction by 2 and checks if the result is greater than or equal to 1.
- If so, it prints "1" and subtracts 1 from the fraction; otherwise, it prints "0". This process continues until the fraction becomes 0.
- The decimalToBinary() function takes a double parameter decimal, representing the entire decimal number to be converted to binary.
- It first handles negative numbers by printing (-) if the input is negative and then taking the absolute value of the decimal.
- Then, it separates the integer part and the fractional part from the decimal number.
- It then converts the integer part to binary by repeatedly dividing it by 2 and storing the remainder in an array.
- The binary digits are then printed in reverse order.
- After that, if the fractional part exists (i.e., is greater than 0), the convertFraction function is called to convert it to binary.
- In the main() function, we prompt the user to enter a decimal number, read it using scanf, and store it in the variable decimal.
- We pass this variable as an argument to call the decimalToBinary() function which then prints the integral part and binary form of the input number to the console.
Time complexity is O(log(integerPart)) + O(precision)
Space complexity is O(1).
Time & Space Complexity Comparison For Programs To Convert Decimal To Binary In C
Given below is a complete analysis of the time and space complexity of the different approaches that we have discussed above:
Approach | Time Complexity | Space Complexity |
---|---|---|
Converting Decimal To Binary In C | Naive Approach (Division Op) | O(log n) | O(1) |
Converting Decimal To Binary In C Using While Loop (User Input) | O(log n) | O(1) |
Converting Decimal To Binary In C Using Stack | O(log n) | O(n) |
Converting Decimal To Binary In C Using Bitwise Operators | O(1) | O(1) |
C Program to Convert Decimal to Binary Using Functions | O(log n) | O(1) |
Decimal To Binary In C Using For loop | O(log n) | O(1) |
Decimal To Binary In C Using Math.h | O(log n) | O(1) |
Decimal To Binary In C Using Right shift With AND Operator | O(1) | O(1) |
Converting Decimal To Binary In C Using Recursion | O(log n) | O(log n) |
Converting Negative Decimal To Binary In C | O(log(integerPart)) + O(precision) | O(1) |
Converting Decimal To Binary In C For Decimal With Floating Points | O(n) | O(1) |
Note that the time complexity is primarily determined by the number of digits in the decimal number, which is log(N) where N is the decimal number itself. The space complexity depends on the use of additional data structures like stacks or buffers for intermediate calculations.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
Converting numbers into binary form is a task in computer science and programming. We have explored methods to accomplish the conversion of numbers from decimal to binary in C language. These include using the naive approach, for loop, while loop, math library, stack, bitwise operators, and functions. Each of these approaches has its own pros and cons. Iterative methods like bitwise operators offer simplicity and efficiency, while array-based solutions provide flexibility for handling negative and fractional components. Recursive approaches and specialized techniques, such as binary search, offer elegance and efficiency in certain scenarios.
Overall, the choice of approach to convert a number from decimal to binary in C depends on the specific requirements of the application, including considerations such as input range, precision, and performance constraints.
Also Read: 100+ Top C Interview Questions With Answers (2024)
Q. How do you convert 0.75 to binary?
To convert a decimal number to binary, you can use the fractional part conversion method. Multiply the fractional part by 2 and take the integer part as the next binary digit. Repeat this process until the fractional part becomes 0 or until you've obtained the desired number of binary digits. For example-
0.75 * 2 = 1.5, so the first binary digit is 1
0.5 * 2 = 1.0, so the second binary digit is 1
0.0 * 2 = 0.0, so the conversion is complete
Therefore, 0.75 in binary is 0.11
Q. What is the binary representation of 0.8125 in decimal?
To transform a decimal number into binary, the fractional part conversion technique can be employed. Multiply the fractional portion by 2 and assign the integer portion as the subsequent binary digit. Continue this procedure until the fractional component reaches 0 or until the required count of binary digits is achieved. For example-
0.8125 * 2 = 1.625, so the first binary digit is 1
0.625 * 2 = 1.25, so the second binary digit is 1
0.25 * 2 = 0.5, so the third binary digit is 0
0.5 * 2 = 1.0, so the fourth binary digit is 1
0.0 * 2 = 0.0, so the conversion is complete
Therefore, 0.8125 in binary is 0.1101
Q. What is the purpose of the "\b" escape sequence in C programming?
In C, the expression \b represents the backspace character. When used in a string or character literal, it moves the cursor or output position one character back, effectively erasing the character before the cursor.
Q. How can the decimal value 0.625 be expressed in binary form?
To convert a decimal number to its binary representation, you can utilize the fractional part conversion approach. This involves multiplying the decimal's fractional portion by 2 and recording the integer part as the next binary digit. Repeat this process iteratively until the fractional part becomes zero or until the desired number of binary digits is attained.
0.625 * 2 = 1.25, so the first binary digit is 1
0.25 * 2 = 0.5, so the second binary digit is 0
0.5 * 2 = 1.0, so the third binary digit is 1
0.0 * 2 = 0.0, so the conversion is complete
Therefore, 0.625 in binary is 0.101
Q. Convert the binary number 1110101100110 into hexadecimal format.
To convert a binary number to hexadecimal you must begin by grouping the binary digits, starting from the rightmost digit, grouping them in sets of four. If the total number of digits is not a multiple of four, you can add leading zeros to the leftmost group. For example-
Binary: 1110 1011 0011 0
Convert Each Group to Hexadecimal:
- Binary 1110 is equivalent to hexadecimal E.
- Binary 1011 is equivalent to hexadecimal B.
- Binary 0011 is equivalent to hexadecimal 3.
- Binary 0000 is equivalent to hexadecimal 0.
Combine the Hexadecimal Digits: Combine the hexadecimal digits obtained from each group to form the final hexadecimal representation.
Hexadecimal: EB30
Q. What is the decimal equivalent of the binary number 10101010?
To convert a binary number to its decimal equivalent, you can use the powers of 2. Each digit in the binary number represents a power of 2 based on its position, where the rightmost digit represents 2^0, the next digit to the left represents 2^1, then 2^2, and so on.
For example, the binary number 10101010 can be converted to decimal as follows:
- The rightmost digit (0) represents 0 * 2^0 = 0.
- The next digit (1) to the left represents 1 * 2^1 = 2.
- The next digit (0) represents 0 * 2^2 = 0.
- The next digit (1) represents 1 * 2^3 = 8.
- The next digit (0) represents 0 * 2^4 = 0.
- The next digit (1) represents 1 * 2^5 = 32.
- The next digit (0) represents 0 * 2^6 = 0.
- The leftmost digit (1) represents 1 * 2^7 = 128.
Now add up these values: 0 + 2 + 0 + 8 + 0 + 32 + 0 + 128 = 170. So, the decimal equivalent of the binary number 10101010 is 170.
Q. What is the concept of binary search in the C programming language?
Binary search is a fundamental algorithm used to efficiently locate a target value within a sorted array or list. The concept of binary search revolves around the idea of repeatedly dividing the search interval in half until the target element is found or the interval becomes empty. Here's how binary search works:
- Begin with the entire sorted array or list.
- Compare the target value with the middle element of the array.
- If the target matches the middle element, the search is complete.
- If the target is less than the middle element, continue the search on the left half of the array.
- If the target is greater than the middle element, continue the search on the right half of the array.
- Repeat steps 2-5 until the target is found or the search interval becomes empty.
Binary search is highly efficient, with a time complexity of O(log n), where n is the number of elements in the array. This makes it significantly faster than linear search, especially for large arrays.
Q. How do you perform the conversion of the decimal number 12 into binary notation?
To convert a decimal number to binary notation, you can use the process of repeated division by 2. Here's how you can convert the decimal number 12 into binary:
- Divide 12 by 2, get quotient 6 and remainder 0.
- Divide 6 by 2, get quotient 3 and remainder 0.
- Divide 3 by 2, get quotient 1 and remainder 1.
- Divide 1 by 2, get quotient 0 and remainder 1.
Reading the remainders from bottom to top gives you the binary representation: 1100. So, the decimal number 12 in binary notation is 1100.
This compiles our discussion on how to convert a number from decimal to binary in C. Here are a few more interesting topics you must explore:
- Swapping Of Two Numbers In C | 5 Ways Explained (With Examples)
- Compilation In C | Detail Explanation Using Diagrams & Examples
- Logical Operators In C (AND, OR, NOT, XOR) With Code Examples
- Understanding Constant In C: How To Define, Syntax, and Types
- Dangling Pointer In C Language Demystified With Code Explanations
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment