Dangling Pointer In C Language Demystified With Code Explanations
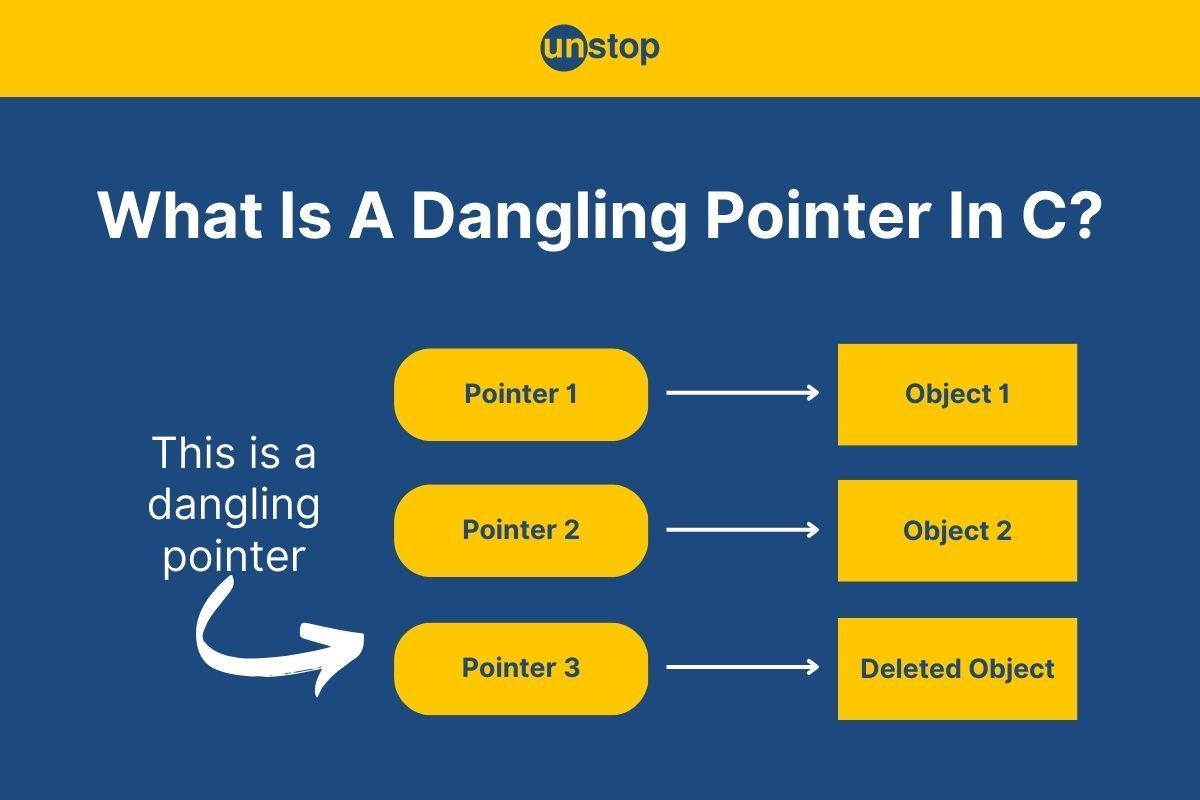
Table of content:
- What Is A Dangling Pointer In C?
- How Does A Dangling Pointer In C Work?
- Reasons For Occurance Of Dangling Pointers In C
- Methods To Avoid Dangling Pointer In C
- How Can Dangling Pointers In C Be Security Holes?
- Conclusion
- Frequently Asked Questions
Pointers in programming are variables/ elements that point to the memory address/ location in the storage of another variable. They allow us to access and manipulate the variable stored in that memory addresses directly. This enables programmers to perform efficient memory management and low-level programming tasks. When a pointer points to a deallocated memory block (or invalid memory location), it is called a dangling pointer in C programming.
The issues that often arise due to dangling pointers are called the Dangling Pointer Problem. These errors occur when a pointer points to a variable that has gone out of scope or when the memory of an object/variable has been deallocated. In this article, we will discuss the concept of dangling pointers in C programming language.
What Is A Dangling Pointer In C?
Dangling pointers in C can be likened to objects that hang freely in the air. As their name suggests, these pointers refer to memory locations that have been freed or deleted from the program's memory, making them invalid memory locations. In other words, they point to memory locations that are no longer in use.
The concept of dynamic memory allocation is closely associated with the concept of dangling pointers. When we dynamically allocate memory using functions like malloc() and calloc(), we acquire memory blocks for our program's use. However, once we no longer need that memory, we must deallocate it using the free() function.
The critical point to understand is that once we free a memory block using free(), any pointers pointing to that memory location become dangling pointers. These pointers now hold addresses to memory that are no longer reserved for our program, which can lead to unpredictable and erroneous behavior if accessed. It is crucial to handle memory allocation and deallocation carefully to avoid such issues and ensure we don't end up with dangling pointers lingering in our code!
Here are some rules and guidelines to avoid and handle dangling pointers in C:
- Avoid Dereferencing Freed Pointers: After using the free() function to deallocate memory, refrain from accessing or dereferencing the pointer.
- NULL Initialization: Always initialize pointers to NULL when declaring them.
- Assigning Valid Addresses: Ensure that pointers point to valid memory addresses before dereferencing them. Assign them the address of an existing variable or a valid memory block obtained from functions like malloc() or calloc().
- Limited Pointer Lifetime: Be cautious with pointers that point to local variables within a function. When the function ends, the local variables go out of scope, creating dangling pointers in C programs.
- Scope Management: Minimize the scope of pointers as much as possible.
- Copy Pointers with Caution: Be mindful when copying pointers. If you copy a pointer, both the original pointer and the copy will point to the same memory location.
- Avoid Pointer Arithmetic: Be cautious when performing pointer arithmetic, as it can lead to accessing unintended or invalid memory.
Let’s consider a real-life example: Imagine you have a sticky note (pointer) with the address of a friend's house written on it (memory location). You use this note to find your friend's house easily.
However, your friend decides to move to a different house, and you forget to update the address on the sticky note. Now, when you try to use the same note to visit your friend's house, you end up at the old address, now someone else's new residence.
In this scenario, the sticky note becomes a dangling pointer since it points to an outdated memory location (your friend's old house) that is no longer valid for you. Similarly, in C programming, a dangling pointer points to a memory location that has been deallocated or is no longer in use, causing potential errors when accessed.
How Does A Dangling Pointer In C Work?
In C, a dangling pointer refers to a pointer that points to a memory location that has been deallocated or freed. Accessing or dereferencing a dangling pointer can lead to undefined behavior, as the memory it points to may have been reallocated to some other part of the program or released back to the system. Here's an explanation of how a dangling pointer works:
- Memory Allocation: When we create a variable or any other elements, memory is allocated to store that variable or the respective data.
- Two Types of Allocation: Static allocation occurs during compile-time for fixed-size variables, while dynamic allocation occurs during runtime for variables with sizes determined at runtime.
- Dynamic Memory Allocation: Achieved using functions like malloc(), calloc(), or realloc().
- Pointer Assignment: A pointer stores the memory address of the dynamically allocated block, allowing access for manipulation of data.
- Memory Deallocation: After use, memory must be deallocated using the free() function to prevent memory leaks.
- Dangling Pointer Generation: When memory is deallocated using free(), the pointer still holds the address of the now-freed memory block, becoming a dangling pointer.
- Undefined Behavior: Attempting to access or dereference a dangling pointer leads to accessing memory no longer reserved for the program, resulting in unexpected program behavior.
Now, let's look at a C code example that demonstrates the working of dangling pointers.
Code Example:
Output:
Dangling Pointer Value: 42
free(): invalid pointer
The output of this code is unpredictable and can vary from system to system. It may produce incorrect results or even crash the program. This is because the dangling pointer, danglingPtr, points to memory that has been deallocated, and accessing it leads to undefined behavior.
Explanation:
We begin the simple C program by including the <stdio.h> and <stdlib.h> header files for input/output functions and dynamic memory allocation functions (malloc() and free()).
- Then, we define a function createAndReturnPointer(), which creates and returns the pointer for a variable of integer data type. Inside the function-
- We have a local variable num, which is assigned the value 42.
- Next, we create a pointer variable numptr and assign the value of num variable to it using the address-of()/ reference operator.
- The function then returns the pointer numptr.
- We then initiate the main() function, which is the entry point of the program's execution.
- Inside main(), we declare a pointer ptr and call the createAndReturnPointer() function to initialize the pointer.
- As a result, ptr points to the memory location of the num variable. Ideally, since num is a local variable, the pointer ptr will become a dangling pointer as soon as the createAndReturnPointer() function finishes execution.
- So when we use a printf() statement to display the value pointed to by the pointer ptr, accessing it using the indirection operator (*). This should throw an error since the memory it points to is no longer reserved for our program.
- However, the value 42 might still be present in that memory location. The behavior is not guaranteed and can vary based on the compiler, optimization settings, and other factors.
- Inside printf(), the %d format specifier is the placeholder for integer value and the newline escape sequence shift the cursor to the next line.
Also read- How To Run C Program | Step-by-Step Explanation (With Examples)
Reasons For Occurance Of Dangling Pointers In C
Dangling pointers are created when a pointer continues to point to a memory location that has been deallocated or freed. This situation can arise due to various programming errors or mismanagement of memory. In this section, we will discuss various ways/ scenarios that lead to the creation of dangling pointers in C and how to prevent them from occurring.
Deallocation Of Memory Using free() & Dangling Pointers In C
When a pointer points to a memory block that has been deallocated using a function like the free() library function, the pointer becomes a dangling pointer. That is, the memory is no longer reserved for the program, but the pointer still holds the address of that memory location. Below is a code example showcasing how this happens, followed by the corrected code showing how we can prevent it from happening.
Code Example:
Output:
Dangling Pointer Value: [undefined behavior]
Dangling Pointer Value: 1617726173
Explanation:
In the sample C code-
- We create an integer pointer ptr and dynamically allocate memory to it using the malloc() method.
- Here, malloc() reserves a memory block of the size of an integer variable on the heap, given by the sizeof(int).
- We also use the type cast operator to indicate that the result of malloc() is an integer pointer, i.e., (int*).
- Next, we assign the value 42 to be stored in the location pointed to by the ptr pointer. At this pointer, ptr is a valid pointer pointing to a dynamically allocated integer on the heap.
- Then, we use the free() method to deallocate the memory we had previously blocked using the malloc() function.
- As a result of this, while the memory space has been deallocated, the pointer ptr is still pointing to the location. Thus, making ptr a dangling pointer.
- We then use a printf() statement to display the value stored at the memory location pointed to by ptr. But since the memory has been freed, accessing ptr will lead to undefined behavior.
- As shown above, the output will be unpredictable and could lead to a runtime error or may print a seemingly valid but wrong value.
Prevention Of Dangling Pointers In C Due To Deallocation Using free():
To prevent the creation of dangling pointers in C due to the deallocation of memory using free(), it is essential to adopt disciplined memory management practices. That is, immediately after freeing memory with free(), you must set the pointer to NULL to avoid accidental use of the deallocated memory. Then, you must check for NULL before freeing to prevent double freeing and ensure that you only free valid memory. The code given below showcases how this can be implemented in practice.
Corrected Code:
Output:
The pointer is now NULL. It is safe to access it.
Explanation:
This code example is a continuation of the example above, where we dynamically allocate memory using malloc() and then deallocate Iit using free(). However, as we saw, if we try to access the pointer after using free(), it leads to undefined behavior. To avoid this danlging pointer error, we have set the pointer to NUL after dellocating the memory. For this-
- We assign the value NULL to the pointer ptr after using free().
- Next, we use an if-else statement to check if it is safe to access the pointer. The statement checks if the value of ptr is not equal to NULL using the relational operator.
- If the condition is true, we try to print the value using a printf() statement. This is the same as the previous example, which leads to an error.
- If the condition is false (which is the case here), then the ptinrf() statement in the else bloc is executed, displaying the string message- The pointer is now NULL. It is safe to access it.
Creation Of Dangling Pointer In C When Variable Goes Out Of Scope
Local variables are those that are either created locally inside a function or inside a specific code block. This means they are out of scope for the rest of the program, i.e., they cannot be accessed from other parts of the program. When we have a pointer that points to a local variable, it can lead to the creation of dangling pointers in C programs after that specific block/ function is done executing.
Code Example:
Output:
ERROR!
gcc /tmp/pgq7v5o9tH.c -lm
/tmp/pgq7v5o9tH.c: In function 'main':
/tmp/pgq7v5o9tH.c:12:27: error: 'x' undeclared (first use in this function)
12 | printf("%d %d", *ptr, x);
| ^
/tmp/pgq7v5o9tH.c:12:27: note: each undeclared identifier is reported only once for each function it appears in
Explanation:
In this example C code-
- We start by declaring a pointer variable named ptr, capable of holding the memory address of an integer. This pointer is uninitialized initially.
- Next, we enter a new block using curly braces {}, inside which we declare a local variable x and initialize it with the integer value 54.
- Then, we assign the memory address of the local variable x to the pointer variable ptr using the address-of operator (&). At this point, ptr holds the address of x, effectively pointing to x.
- As the block scope ends, the local variable x goes out of scope, and its memory is no longer valid. However, our pointer ptr still holds the address of x, making it a dangling pointer.
- We then attempt to print the value pointed to by ptr and the value of x, using a printf() statement and dereference operator. This operation is unreliable as x is out of scope, and accessing its value through a dangling pointer leads to undefined behavior.
- Similarly, we try to print the value pointed to by the dangling pointer ptr. Again, this operation is unreliable and may result in printing garbage values or causing program crashes due to undefined behavior.
- Finally, the program returns 0, indicating successful execution, but its reliability is compromised due to the use of dangling pointers.
Prevention Of Dangling Pointer In C Due To Out Of Scope Variables:
To prevent the creation of dangling pointers in C when a variable goes out of scope, we can use the pointer scope management. It involves ensuring that pointers are used only within the scope where the pointed-to variable is valid. By restricting the usage of pointers to the block or function where the variable was declared, you can minimize the chances of creating dangling pointers.
Corrected Code:
Output:
54
Explanation:
In this corrected code-
- We begin by declaring an integer variable x and initialize it with the value of 54. Note that the variable is declared inside the scope of the main() function.
- Next, we declare a pointer variable named ptr which is also within the scope of the main() function.
- We start by assigning the memory address of the integer variable x to the pointer ptr, using the address-of operator.
- We then use the pointer to print the value of the integer variable it points to using a printf() statement.
- As the variable x is still within the scope of the main() function, the pointer ptr is not a dangling pointer.
- We continue using the pointer safely within the block.
- The program returns 0, indicating successful execution.
An alternative approach is to use static variable with global scope instead of local variables. If a variable is declared globally, it can be accessed from anywhere in the program and will not go out of scope until the program ends. This eliminates the possibility of creation of dangling pointers. We will discuss this in a later section.
Creation Of Dandling Pointers In C & Function Call
When a function returns a pointer that points to a local variable within the function, the returned pointer becomes a dangling pointer. After the function completes its execution, the local variable goes out of scope, making the pointer invalid and pointing to memory that is no longer accessible.
Code Example:
Output:
No output due to undefined behavior caused by dangling pointer
Code Explanation:
In this C program example-
- We begin by declaring a function returnDanglingPointer() to return an integer pointer (int*).
- Inside the function, an integer variable num is declared and initialized with the value 20.
- Next, a pointer variable ptr is declared and assigned the address of the local variable num. The function returns the pointer ptr.
- ,Then in the main() function, we declare a pointer variable named danglingPtr.
- We then call the returnDanglingPointer() function from main, and assign the returned pointer to danglingPtr.
- At this point, danglingPtr points to a memory location that was occupied by the local variable num in the returnDanglingPointer function.
- However, num goes out of scope as the function returnDanglingPointer() ends, making the pointer danglingPtr a dangling pointer.
- Attempting to dereference the dangling pointer danglingPtr and access the value it points to results in undefined behavior.
- The output is unpredictable and may lead to a program crash or unexpected values.
Prevention Of Dangling Pointer In C Due To Scope Of Function Call & Return:
To prevent the situation of returning a dangling pointer, you should avoid returning pointers that point to local variables with automatic storage duration (local variables declared within a function). Instead, consider using dynamic memory allocation (e.g., malloc, calloc) for creating objects whose lifetime extends beyond the function's scope. Below is the correct code example continuation to showcase how this is implemented.
Corrected Code:
Output:
Value: 20
Code Explanation:
The code example is a correction of the previous C code, where we have made a change to the function definition to eliminate the possibility of creating dangling pointers in the C program. Here-
- We dynamically allocate memory for the integer variable inside the definition of the createValidPointer() function using malloc().
- Then, as mentioned in code comments, we use an if-statement to check if the allocation was successful before proceeding.
- If the condition is true, it means allocation failed, and the function returns an error.
- If not, then the function initializes the allocated memory with a value and returns the dynamically allocated pointer.
- In the main() function, we create a pointer validPtr and call the createvaidPointer() function to initialize it.
- The returned pointer (validPtr) points to dynamically allocated memory, and it can be used safely.
- Note that after using the dynamically allocated memory, it is essential to free it using free to avoid memory leaks.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Methods To Avoid Dangling Pointer In C
To steer clear of issues stemming from dangling pointers in C, programmers can implement a range of methods and best practices in their code. As mentioned before, dangling pointers arise when a pointer points to memory that has been deallocated or is no longer accessible. These errors can trigger unpredictable behavior, cause program crashes, and even result in data corruption.
Here are a few methods to effectively minimize the risks linked to dangling pointers in C programming:
- Nullify Pointers After Deallocation: After using functions like free() to deallocate memory, immediately set the pointer to NULL. This prevents the pointer from holding the address of the deallocated memory and reduces the chances of it becoming a dangling pointer in C.
- Avoid Returning Pointers to Local Variables: Be cautious when returning pointers from functions, especially if they point to local variables within the function. Instead, consider returning dynamically allocated memory that the caller must later deallocate responsibly.
- Use Dynamic Memory Allocation Carefully: Use dynamic memory allocation functions like malloc(), calloc(), and realloc() judiciously. Avoid accessing the memory after deallocation to prevent dangling pointers in C programs.
- Limit Pointer Scope: Minimize the scope of pointers whenever possible. That is, declare pointers in the smallest scope necessary to reduce the chances of them becoming dangling pointers later in the program's execution.
- Avoid Pointer Arithmetic with Dangling Pointers: Using pointer arithmetic with dangling pointers in C programs can lead to serious memory-related issues. To avoid this, always ensure that the pointers point to valid memory locations before performing any pointer arithmetic operations.
- Use Static Variables for Persistent Storage: For data that needs to persist beyond a function's scope, consider using static variables or dynamically allocating memory that will be managed carefully throughout the program's lifetime.
- Testing and Code Review: Conduct thorough testing, including both positive and negative test cases, to identify potential issues related to dangling pointers.
- Code reviews by peers can help catch potential pitfalls and ensure adherence to best practices.
- Use static analysis tools and memory debugging tools to detect and address potential dangling pointer issues during development.
- Memory Management Techniques: Employ memory management techniques like RAII (Resource Acquisition Is Initialization) or smart pointers in C++ to automate memory management and minimize the risk of dangling pointers.
Assigning NULL After De-allocation Of Memory To Avoid Dangling Pointers In C
Assigning NULL after deallocating memory is a critical technique used to prevent dangling pointers in C. It involves setting a pointer to the value NULL immediately after freeing the memory it was pointing to using the free() function. This practice helps to mitigate the risk of the pointer becoming a dangling pointer, where it continues to hold an invalid memory address after the memory has been deallocated.
Here's a detailed explanation of the process:
- Dynamic Memory Allocation: In C, dynamic memory allocation is performed using functions like malloc, calloc, and realloc. These functions request memory from the operating system to store data at runtime. The allocated memory exists until it is explicitly deallocated using the free() function.
- Deallocating Memory: After the dynamically allocated memory is no longer needed, it must be returned to the operating system to avoid memory leaks. This is achieved by calling the free() function and passing the pointer to the beginning of the allocated memory block as an argument. the free() function marks the memory as available for reuse.
- Setting Pointers to NULL: After deallocating the memory, it is crucial to set the pointer that was pointing to the deallocated memory to NULL. Assigning NULL to the pointer ensures that it no longer holds the address of the deallocated memory block, effectively preventing it from becoming a dangling pointer.
Example of Assigning NULL after deallocating memory:
Output:
Before deallocation - Value: 42
The pointer is NULL. Cannot access deallocated memory.
Code Explanation:
In the above code,
- We declare and initialize a pointer ptr with the address of a dynamically allocated integer using malloc() inside the main() function. This integer is intended to store the value 42.
- Next, we perform a check to ensure that the memory allocation was successful using an if-statement.
- If ptr is NULL, the program prints an error message, exits, and returns a non-zero value (1) to indicate failure.
- If the memory allocation is successful, the value 42 is assigned to the memory location pointed to by ptr.
- The program then prints the value of the integer using a printf() statement before deallocating the dynamically allocated memory using the free() function.
- After freeing the memory, the pointer ptr is set to NULL to avoid it becoming a dangling pointer.
- Another check is performed to determine whether ptr is NULL using another if-statement.
- If not, the program attempts to print the value at the memory location pointed to by ptr.
- However, since ptr is now NULL, the program prints a message indicating that it cannot access deallocated memory.
- The program concludes by returning 0 to the operating system, indicating successful execution.
Static Variables With Global Scope To Avoid Dangling Pointers In C
In C programming, a static variable with global scope is a variable declared using the keyword static outside any function or block. This grants it accessibility throughout the entire program's execution. The static variable retains its value between function calls, offering persistence beyond individual function invocations. However, its visibility is confined to the file in which it is defined. Consequently, static global variables remain hidden from other files in a multi-file program, promoting encapsulation and preventing conflicts with identically named variables in other source files.
A static variable with global scope in C possesses several noteworthy characteristics and behaviors:
- Accessible within Source File: Such a variable can be accessed from any function within the same source file where it is declared. This feature facilitates the sharing of data among functions within that specific file.
- Limited to File Scope: Being declared outside any function or block, the static variable has file scope, confining its visibility solely to the file where it is defined. Consequently, it remains inaccessible from other files within the same program.
- Persistent Throughout Program Execution: The static variable endures throughout the entire runtime of the program. It is created when the program commences and remains in memory until the program terminates. As a result, its value persists between function calls, retaining the last assigned value across different function invocations.
- Automatic Default Initialization: If not explicitly initialized, the static variable is automatically set to zero (0) for numeric data types (e.g., int, float) or a null pointer for pointer types.
- Internal Linkage by Default: By default, static variables with global scope possess internal linkage, preventing them from being visible and accessed from other files in multi-file programs. This quality aids in encapsulating data within a single source file and prevents naming conflicts with variables of the same name in other files.
- Promotes Data Hiding and Encapsulation: Limited to file scope, the static variable enables data hiding and encapsulation within the file it is declared. This enhances code organization and reduces the risk of naming conflicts with variables in other parts of the program.
- Initialization Occurs Only Once: If a static variable with global scope is initialized within a function, it undergoes initialization only once, regardless of how many times the function is called during program execution. This feature proves beneficial when initialization is resource-intensive and needs to be executed only once.
Code Example:
Output:
Global variable value: 15
Explanation:
In the C code sample-
- We start by defining a global variable named globalVar and initializing it to 10 using the static keyword.
- We then define a function called function1(), which doesn't take any parameters. It increments the value of the global variable globalVar by 5.
- Then, we define a second function, function2(), which also doesn't take any parameters. It utilizes printf() to print the value of globalVar along with a message.
- Inside the main() function, we call function1(), which modifies the global variable's value, adding 5 to it.
- Subsequently, we call function2(), which prints the updated value of globalVar to the console.
- The program concludes by returning 0 from the main function, indicating successful execution to the operating system.
How Can Dangling Pointers In C Be Security Holes?
Dangling pointers in C can be serious security vulnerabilities because they can lead to unpredictable behavior, crashes, or unauthorized access to memory. Exploiting these vulnerabilities can allow attackers to manipulate the program's behavior in unintended ways, potentially leading to security breaches. Here are several ways in which dangling pointers can pose security risks:
- Unauthorized Memory Access: If a dangling pointer in C is dereferenced, it may point to a memory location that has been freed or reallocated for a different purpose. This can result in reading or modifying data that the attacker is not supposed to have access to, potentially revealing sensitive information or causing data corruption.
int *ptr = (int *)malloc(sizeof(int));
free(ptr);
// At this point, 'ptr' is a dangling pointer.
*ptr = 42; // Dereferencing the dangling pointer may lead to unauthorized memory access.
- Control Flow Manipulation: Exploiting dangling pointers in C programs may allow an attacker to manipulate the program's control flow, leading to unexpected execution paths or code injection. This can be a stepping stone for more sophisticated attacks, such as code execution exploits.
int *ptr = (int *)malloc(sizeof(int));
free(ptr);
// At this point, 'ptr' is a dangling pointer.
// An attacker may manipulate the pointer to redirect the program's control flow.
- Crashes and Denial of Service: Dereferencing a dangling pointer in C programs can result in undefined behavior, including crashes. While this may not always lead to a security breach, it can be exploited by attackers to cause denial-of-service (DoS) attacks by intentionally crashing the program.
int *ptr = (int *)malloc(sizeof(int));
free(ptr);
// At this point, 'ptr' is a dangling pointer.
int value = *ptr; // Dereferencing the dangling pointer may lead to a crash.
- Code Injection: In certain scenarios, attackers may manipulate dangling pointers to overwrite function pointers or other critical data structures, leading to code injection attacks. This can potentially result in arbitrary code execution with the privileges of the compromised process.
int *ptr = (int *)malloc(sizeof(int));
free(ptr);
// At this point, 'ptr' is a dangling pointer.
// An attacker may exploit this to overwrite critical data structures.
Conclusion
A dangling pointer in C is a pointer that points to an invalid memory address or one that is not in use anymore. These pointers arise from memory deallocation or when variables go out of scope, leading to a potential hazard in program execution known as the Dangling Pointer Problem.
Such issues can cause errors in program behavior. It is hence crucial to handle the issues with dangling pointers in C properly. One of the ways to get this done is to assig NULL to the pointer immediately after memory deallocation thus mitigating dangling pointers. Additionally, utilizing static variables ensures the variable persists in memory until program execution concludes, preventing premature deallocation and associated hazards. Employing these approaches enhances program stability and helps steer clear of dangling pointers in C and other related errors.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is a dangling pointer in C? Give an example.
A dangling pointer is a pointer that points to a memory location that has been deallocated, freed, or is no longer valid. Dereferencing a dangling pointer in C programs can lead to undefined behavior since the memory it points to may have been reallocated for other purposes, or it may no longer be accessible by the program.
Code Example:
Output:
Segmentation fault (core dumped)
Explanation:
The above code demonstrates a common issue known as a use-after-free error. Once the memory is freed using free(ptr), the pointer ptr becomes a dangling pointer. Accessing or dereferencing a dangling pointer leads to undefined behavior.
Q. How to fix a dangling pointer in C?
Here are a few ways in which you can fix dangling pointers in C,:
- After freeing a pointer with free() or delete, immediately set it to NULL to prevent accidental dereferencing.
- Avoid using freed pointers and perform checks before accessing them to ensure their validity.
- Never free a pointer more than once (double free).
- Prefer automatic variables over dynamic memory allocation (heap-based) whenever possible.
- In C++, consider using smart pointers like std::unique_ptr and std::shared_ptr for automatic memory management.
Q. What are the different types of pointers in C?
There are several types of pointers in C and C++, based on their properties and usage. Here are the common types of pointers:
- Null Pointer: A pointer that does not point to any memory address. It is represented as NULL or null ptr (in C++11 and later).
- Wild Pointer: A wild pointer in C is a pointer that has not been properly initialized or points to an arbitrary memory location. Unlike null pointers, which are intentionally set to NULL or 0, wild pointers contain unpredictable memory addresses.
- Void Pointer (Generic Pointer): A pointer without any specific data type. Used to store the address of any data type, requiring explicit type casting before dereferencing.
- Pointer to Data Types: Pointers specifically designed to store the addresses of variables of specific data types. Examples include int* (pointer to an integer), char* (pointer to a character), etc.
- Function Pointer: A pointer that points to the address of a function. Allows dynamic function invocation, enabling the selection and execution of functions at runtime.
- Array Pointer: A pointer that points to the first element of an array. Arrays in C can be accessed using pointers and vice versa.
- Pointer to Pointer (Double Pointer): A pointer that holds the address of another pointer. Used in cases where multiple levels of indirection are required.
- Constant Pointer: A pointer whose value (address it points to) cannot be changed after initialization. Example: int* const ptr; declares a constant pointer to an integer.
- Pointer to Constant: A pointer that can change the address it points to but cannot modify the value at that address. Example: const int* ptr; declares a pointer to a constant integer.
- Constant Pointer to Constant: A pointer that cannot modify the value at the address it points to and cannot change the address it points to. Example: const int* const ptr; declares a constant pointer to a constant integer.
Q. Is the dangling pointer in C a memory leak?
A dangling pointer in C is not the same as a memory leak, but both issues are related to memory management and can cause problems in a program.
- A memory leak occurs when a program allocates memory dynamically (using functions like malloc, calloc, or new in C or C++) but fails to release or deallocate that memory before the program terminates.
- This results in a gradual accumulation of unreleased memory, which can lead to increased memory usage over time.
- On the other hand, a dangling pointer occurs when a pointer continues to hold the address of a memory location after that memory has been deallocated or freed.
- This can happen, for example, when a pointer is not set to NULL after the corresponding memory is freed.
- Accessing or modifying the memory through a dangling pointer leads to undefined behavior and can cause crashes, unexpected results, or security vulnerabilities.
Q. What is a dangling reference in storage allocation?
In the context of storage allocation, a dangling reference refers to a reference or pointer that points to a memory location that is no longer valid or has been deallocated. Dangling references are similar to dangling pointers but specifically refer to references in languages that use reference semantics, such as C++.
- In languages with reference semantics, like C++, references are aliases or alternative names for existing variables.
- Unlike pointers, references cannot be reassigned to point to a different object or memory location after initialization.
- However, references can still become dangling if they refer to objects that are destroyed or go out of scope.
To avoid dangling references, it's essential to ensure that references do not outlive the objects they refer to. This can be achieved by using references only within the scope of the objects they point to and avoiding the return of references to local variables from functions.
Q. What are the 4 types of memory in C?
In C programming, there are typically four types of memory:
- Stack Memory: The stack is a region of memory used for storing local variables, function call data and control information. It operates in a Last-In-First-Out (LIFO) manner, and memory allocation and deallocation are managed automatically by the compiler as functions are called and returned. When a function is called, its local variables and other data are pushed onto the stack, and when the function returns, the memory for those variables is automatically deallocated.
- Heap Memory (Dynamic Memory): The heap is a region of memory used for dynamic memory allocation during runtime. It is managed manually by the programmer using functions like malloc, calloc, realloc, and free. The memory allocated on the heap persists until explicitly deallocated, providing more flexibility for creating and managing data structures of varying sizes and lifetimes.
- Global Memory (Static Memory): Global memory is used for storing global variables, which are accessible throughout the entire program's execution. Global variables are initialized before the program starts and remain in memory until the program terminates. They have a static lifetime and are typically located in a separate region of memory from the stack and heap.
- Constants Memory: Constants memory stores constants like string literals, numeric constants, and other data that should not be modified during program execution. These constants are typically stored in read-only memory (ROM) or a separate region of memory, depending on the specific implementation and platform.
Q. What are the disadvantages of dangling pointers?
Dangling pointers in a C program presents several disadvantages and potential risks, including the following:
- Undefined Behavior: Dereferencing a dangling pointer in C leads to undefined behavior. It causes program crashes, unpredictable results, or seemingly correct behavior, making it challenging to debug and maintain the code.
- Data Corruption: Dangling pointers in C may inadvertently modify unrelated data or overwrite valid memory. This leads to data corruption and introduces elusive bugs that are difficult to detect.
- Security Vulnerabilities: Dangling pointers in C can become security vulnerabilities. Allows attackers to exploit undefined behavior for arbitrary code execution or unauthorized access to sensitive data.
- Debugging Complexity: Identifying and tracing bugs caused by dangling pointers can be arduous. Especially challenging when the problem arises in a different part of the code from where the pointer was dereferenced.
- Code Reliability Reduction: Existence of dangling pointers in C programs make them less reliable and robust. Increases the likelihood of unexpected failures, impacting overall code reliability.
Here are a few more amazing topics you must read up on:
-
- Control Statements In C | The Beginner's Guide (With Examples)
- Array Of Pointers In C & Dereferencing With Detailed Examples
- Comma Operator In C | Code Examples For Both Separator & Operator
- Ternary (Conditional) Operator In C Explained With Code Examples
- Tokens In C | A Complete Guide To 7 Token Types (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment