Fibonacci Series Using Recursion In C (With Detailed Explanation)
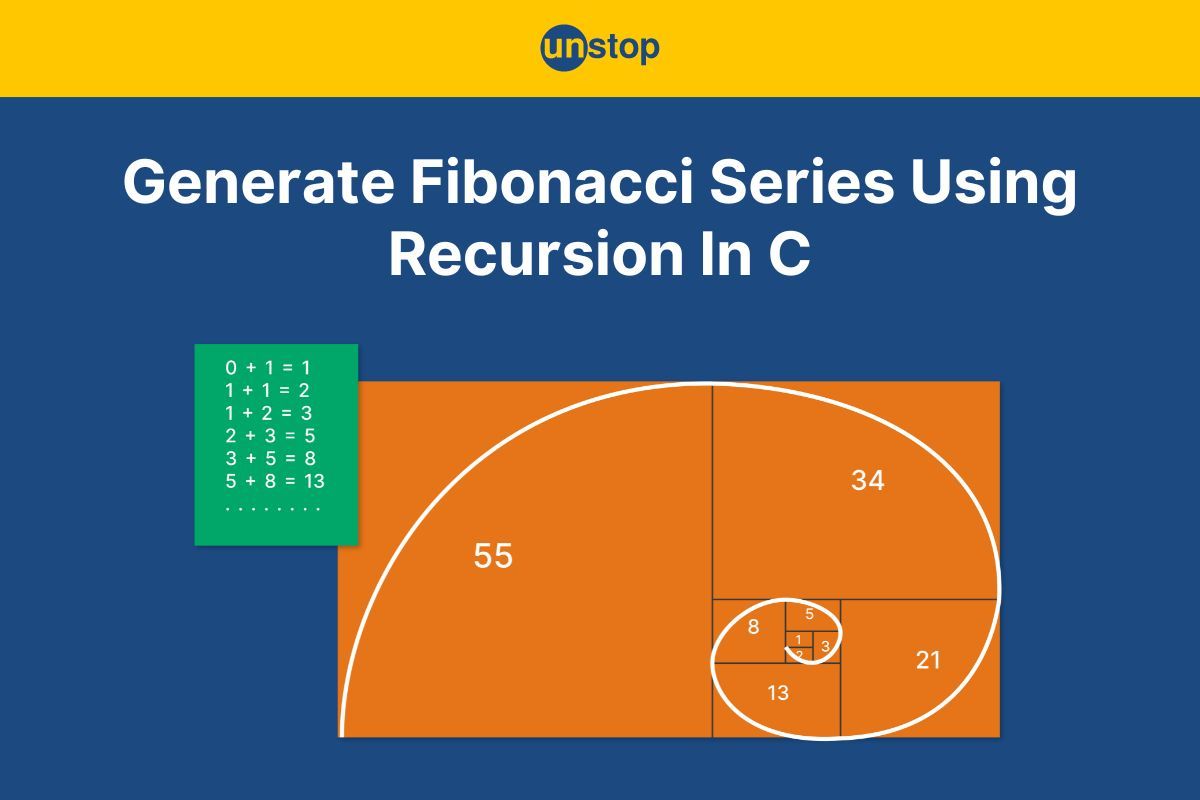
The Fibonacci series is a numerical sequence of integers where each number is the sum of the two preceding ones, usually starting with 0 and 1. Recursion, on the other hand, is a powerful concept in computer science where a function calls itself to solve smaller instances of the same problem. In this article, we will explore various ways to generate the Fibonacci series using recursion in C programming language.
By understanding the recursive approach to computing the Fibonacci series, you can gain insights into the power of recursion as a programming technique and the mathematical beauty of the Fibonacci sequence. We will use detailed explanations and illustrative examples to demystify the Fibonacci series and help you write/ run C programs that generate the series using recursion.
What Is The Fibonacci Series?
As mentioned above, the Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding ones. It typically starts with 0 and 1, and the subsequent Fibonacci sequence elements are generated by adding the two previous numbers.
- Therefore, the Fibonacci series begins as follows: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on.
- This sequence was first introduced by Leonardo of Pisa, also known as Fibonacci, in his book Liber Abaci in 1202.
- The Fibonacci series appears in various natural phenomena, such as the branching of trees, the arrangement of leaves, and spiral patterns in flowers, shells, and galaxies.
- Additionally, it has applications in mathematics, computer science, art, and finance, making it a fundamental concept in many fields.
Mathematical Representation Of Fibonacci Series Using Recursion
The Fibonacci series can be mathematically represented using a recursive definition:
-
Base Cases:
- F(0) = 0
- F(1) = 1
-
Recursive Relation:
- F(n) = F(n-1) + F(n-2) for n > 1
This recursive definition tells us that each Fibonacci number (except for the first two) is the sum of the two preceding Fibonacci numbers. This is the essence of the Fibonacci sequence and is the foundation for generating it computationally.
Example: Let's calculate the Fibonacci series using the recursive definition:
- F(0) = 0 (Base Case)
- F(1) = 1 (Base Case)
- F(2) = F(1) + F(0) = 1 + 0 = 1
- F(3) = F(2) + F(1) = 1 + 1 = 2
- F(4) = F(3) + F(2) = 2 + 1 = 3
- F(5) = F(4) + F(3) = 3 + 2 = 5
- And so on...
Using this process, we can generate the Fibonacci series indefinitely.
Properties Of Fibonacci Series
Below are some notable properties of the Fibonacci series:
- Exponential Growth: The Fibonacci series grows exponentially. The ratio of successive Fibonacci numbers converges to the golden ratio, approximately 1.6180339887..., which is a fascinating mathematical constant with various interesting properties.
- Occurrence in Nature: The Fibonacci sequence appears in various natural phenomena, such as the branching in trees, the arrangement of leaves on a stem, the flowering of artichokes, and the arrangement of a pine cone's bracts. This occurrence has intrigued scientists and mathematicians for centuries.
- Golden Ratio: The ratio of successive Fibonacci numbers converges to a value approximately equal to 1.6180339887..., known as the golden ratio (often denoted by the Greek letter phi, φ). This ratio has numerous remarkable mathematical properties and is found in various natural phenomena, art, architecture, and aesthetics.
- Lucas Numbers: Lucas numbers are a related sequence similar to the Fibonacci numbers, defined by the same recurrence relation but with different initial values. Lucas numbers exhibit properties analogous to those of the Fibonacci series and share connections with various areas of mathematics.
- Pisano Period: The Pisano period, also known as the period of the Fibonacci sequence modulo a given integer, is a periodic pattern that emerges when examining the remainders of Fibonacci numbers when divided by a fixed integer. Understanding Pisano periods is crucial in analyzing the periodic behavior of Fibonacci sequences in modular arithmetic.
- Mathematical Recurrence: The Fibonacci series serves as a fundamental example of a recursively defined sequence in mathematics. Its recursive nature allows for elegant mathematical analyses and applications in diverse fields, including number theory, combinatorics, graph theory, and computer science.
Ways To Construct Fibonacci Series With & Without Recursion In C
Constructing a Fibonacci series in the C language involves generating a sequence of numbers where each number is the sum of the two preceding ones. There are two primary approaches to constructing a Fibonacci series in C, i.e., using recursion and using iteration (without recursion).
1. Fibonacci Series In C Using Recursion
Recursion is a programming technique where a function calls itself to solve smaller instances of the same problem. In the case of the Fibonacci series, a recursive function can be used to generate each Fibonacci number based on the values of the preceding ones.
Here's how recursion works for generating a Fibonacci series in C:
- Base Cases: Define base cases for the first two Fibonacci numbers (0 and 1).
- Recursive Relation: Implement a function that calls itself with smaller inputs to compute subsequent Fibonacci numbers.
2. Fibonacci Series In C Using Iteration (Without Recursion)
An iterative approach involves using loops to generate Fibonacci numbers without the need for function calls or recursion. This method is often more efficient than recursion, especially for large values of n, as it avoids the overhead of function calls.
Here's how the iterative method works:
- Initialize variables to store the first two Fibonacci numbers.
- Use a loop to calculate subsequent Fibonacci numbers by adding the last two numbers.
How To Generate Fibonacci Series Using Recursion In C?
Recursion involves breaking a problem into smaller subproblems of the same type, solving each recursively, and combining their solutions to get the final result(i.e. recursion tree). Generating a Fibonacci series using recursion in C is an elegant way to finish the job.
In a recursive solution, a function calls itself with smaller inputs until reaching a base case, usually when the input becomes 0 or 1. In the case of the Fibonacci series, the base cases are when n is 0 or 1, where the function returns n. Otherwise, the function recursively calls itself to calculate Fibonacci(n-1) and Fibonacci(n-2) and adds their results to produce Fibonacci(n). This process continues until the base cases are reached.
Here's a step-by-step explanation of the recursive algorithm:
Step 1- Base Case Initialization: We start by defining the base cases( initial terms) of the Fibonacci sequence in the recursive function. When the input n is 0 or 1, we return n itself, as these are the first two numbers in the Fibonacci series.
Step 2- Recursion: In the recursive step, if n is greater than 1, we recursively call the fibonacci() function with n-1 and n-2. These calls continue until the base cases are reached.
Step 3- Add Results: When the base cases are reached (i.e., n is 0 or 1), we add the results of the recursive calls for n-1 and n-2 to calculate the Fibonacci number for n.
Step 4- Return Result: Finally, we return the calculated Fibonacci number for the input n.
Step 5- Output: In the main() function, we prompt users to enter the number of current terms they want in the Fibonacci series. Then, we call the recursive fibonacci() function for each term and print the series as it's generated.
Printing Fibonacci Series Using Single Recursive Function In C
In this approach, we use a single recursive function named printFibonacci() to generate and print the Fibonacci series.
- Within this function, a static variable n1, n2, and n3 are utilized to keep track of the previous two Fibonacci numbers.
- Static variables n1, n2, and n3 are used to store the previous two Fibonacci numbers and the current Fibonacci number, respectively.
- These variables retain their values across different function calls, allowing the function to remember the state of the series.
Let's look at a C code example that showcases the implementation of this method to generate and print a Fibonacci series using recursion in C language.
Code Example:
Output:
Enter the number of elements: 8
Fibonacci Series: 0 1 1 2 3 5 8 13
Explanation:
In the simple C program above, we include the header file <stdio.h> for essential input-output operations.
- We then define a function named printFibonacci to print Fibonacci numbers recursively. Inside the function-
- We have three static variables, i.e., n1 initialized to 0, n2 initialized to 1, and n3.
- Then, we have an if-statement, which first checks whether n is greater than 0.
- If the condition is met, the code inside calculates the next Fibonacci number, n3, which is equal to the sum of n1 and n2.
- The values of the existing variables are then updated, i.e., n1 to n2 and n2 to n3.
- After that, a printf() statement is used to print the calculated Fibonacci number n3.
- Following this, we call the printFibonacci function recursively with n - 1 until n becomes 0.
- In the main() function, we use a printf() statement to prompt the user to enter the number of elements for the Fibonacci series.
- Then, we use scanf() to read the value and store it in the variable n using the address-of operator/ reference, i.e., &n. The %d format specifier indicates that the value will be an integer value.
- After that, as mentioned in the code comment, we print the initial two Fibonacci numbers, 0 and 1, with space in between and after.
- Following this, we call the printFibonacci() function with n - 2 as two numbers are already printed (0 and 1).
- Finally, the main function returns 0, indicating successful execution without any errors.
Time Complexity: This approach has a linear time complexity of O(n) due to its direct computation and printing of Fibonacci numbers within a single recursive function.
Generating Fibonacci Series Using Recursion In C & Printing Seperatly
In this approach, a recursive function named fibonacci() is defined to compute the Fibonacci numbers. Here, the printing of the Fibonacci series is done separately in the main() function. This approach does not use static variables. Instead, the fibonacci() function relies on parameters and local variables to calculate Fibonacci numbers recursively.
Code Example:
Output:
Enter the number of terms: 7
Fibonacci Series: 0 1 1 2 3 5 8
Explanation:
In the sample C program-
- We define a recursive function named fibonacci() to generate Fibonacci numbers. Inside the function-
- We have two base cases, i.e., first if n is 0, we return 0. And second, if n is 1, we return 1.
- For other values of n, the else case is implemented. Here we recursively call the fibonacci() function with n - 1 and n - 2, and return the sum of the results.
- In the main() function, we prompt the user to enter the number of terms for the Fibonacci series using printf statement.
- Then, we read the input using scanf() and store it in the variable n.
- After that, we print the string message- 'Fibonacci Series: ', to indicate the start of the series.
- Following this, we use a for loop with a pintf() statement and the fibonacci() function to generate and print the series. The loop begins at i=0 and continues till i<n (or i = n - 1).
- Inside the loop, we print the Fibonacci term/ number for each value of i by calling the fibonacci() function. The value of control variable i is incremented by 1 after every iteration.
- Finally, the main function terminates with a return 0 statement.
Time Complexity: This approach has an exponential time complexity of O(2^n) due to the repeated computation of Fibonacci numbers in a recursive manner.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Fibonacci Series In C Without Recursion (Iterative Approach)
In the iterative approach for generating the Fibonacci series in C, we use loops to calculate each Fibonacci number directly without relying on function calls or recursion. This method is more efficient compared to the recursive approach, especially for larger values of n, as it avoids the overhead associated with function calls and stack manipulation.
Here's a step-by-step explanation of the iterative approach:
Step 1- Initialization: We initialize variables first and second to represent the first two terms of the Fibonacci series. Typically, term1 is set to 0, and term2 is set to 1, as these are the base cases of the Fibonacci sequence.
Step 2- Loop to Generate Fibonacci Numbers: We then use a for loop to generate subsequent Fibonacci numbers iteratively.
- In each iteration of the loop, we calculate the next Fibonacci number (next) by adding term1 and term2.
- We then update term1 to the value of term2 and term2 to the value of the next term.
- This process continues until we have generated the desired number of terms in the Fibonacci series.
Step 3- Printing Fibonacci Series: Within the loop, after calculating each Fibonacci number, we print it. This allows us to display the Fibonacci series as it is generated.
Step 4- Termination Condition: The loop runs terminate when we have generated the specified number of terms in the Fibonacci series.
Step 5- Output: Finally, we output the generated Fibonacci series to the user.
Therefore, the iterative approach eliminates the need for recursive function calls and the associated overhead by directly calculating each Fibonacci number using a loop. Given below is an implementation of the Fibonacci series in C using an iterative approach without recursion.
Code Example:
Output:
Enter the number of terms: 10
Fibonacci Series:
0 1 1 2 3 5 8 13 21 34
Explanation:
In the C code example-
- We use the void keyword to define a void function named fibonacci() to iteratively generate a Fibonacci series. Inside the function-
- We first initialize three integer type variables, i.e., term1 is assigned a value of 0, term2 is assigned 1, and next_term.
- Then, we use a printf statement to display the string message- 'Fibonacci series: ', followed by a newline escape sequence, which shifts the cursor to the next line.
- Similarly, it prints the initial two Fibonacci numbers, 0 and 1.
- Next, we use a for loop that begins iteration from the initial value i = 2 and terminates when the loop condition i<n becomes false, i.e., until i= n - 1.
- The loop calculates the Fibonacci number using the addition arithmetic operator to add the values of term1 and term2.
- The outcome is the current term which is stored in the next_term variable, and its value is subsequently printed to the console.
- After that, the value of variable term1 is updated to the value of term2 and the value of term2 is updated to next_term.
- Post this iteration, the value of i is incremented by 1, and the next iteration begins.
- In the main() function, we prompt the user to enter the number of terms for the Fibonacci series. This input is stored in the variable n, using the scanf() function and the address-of operator (&).
- Then, we call the fibonacci function with the input value n. This function iteratively computes and prints the Fibonacci series.
- Finally, the main function returns 0, indicating successful execution.
Time Complexity: The time complexity of the example C program for generating the Fibonacci series iteratively is O(n), where n is the number of terms in the Fibonacci series.
Check out this amazing course to become the best version of the C programmer you can be.
Fibonacci Series Program In C Using Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems and solving each subproblem only once, storing their solutions to avoid redundant computations and improve efficiency. It typically involves bottom-up or top-down approaches, utilizing memoization or tabulation to optimize solutions.
Here's a step-by-step explanation of the dynamic approach:
Step 1- Initialize Variables: Declare variables to store the Fibonacci series and the number of terms (n). Afte that, initialize an array to store the Fibonacci numbers.
Step 2- Handle Base Cases: Assign the first two Fibonacci numbers (0 and 1) directly without any computation.
Step 3- Calculate Fibonacci Numbers: Use a looping statement to iterate from the third position to the desired number of terms (n).
- For each position i, calculate the Fibonacci number by adding the values stored at positions i-1 and i-2.
- Store the result in the array.
Step 4- Output Fibonacci Series: Print the Fibonacci series stored in the array.
In dynamic programming, we can optimize the computation of Fibonacci numbers by storing the results of previous calculations in an array and reusing them instead of recalculating them. Below is the implementation of the Fibonacci series program in C using dynamic programming:
Code Example:
Output:
Enter the number of terms: 9
Fibonacci Series:
0 1 1 2 3 5 8 13 21 34
Explanation:
In the example C code-
- We define a function named dyn_fibonacci() to generate a Fibonacci series using dynamic programming. Inside the function-
- We declare an array fib of size n + 2 to store Fibonacci numbers. The additional two spaces accommodate the base cases.
- The base cases are explicitly set to fib[0] = 0 and fib[1] = 1, and the initial two Fibonacci numbers are displayed using the printf() statement.
- We next use a for loop to iteratively generate Fibonacci numbers for n terms starting from the third term.
- In each iteration, we compute the value of fib[i] as the sum of the previous two Fibonacci numbers, fib[i - 1] and fib[i - 2]. We print the generated Fibonacci numbers as they are computed.
- In the main() function, we prompt the user to enter the number of terms for the Fibonacci series.
- We scan the input value using scanf() and store it in the variable n using the reference operator (&).
- After that, we call the fibonacci() function with the input value n.
Time Complexity: The time complexity of the given Fibonacci series program implemented using dynamic programming is O(n), where n is the number of mathematical terms in the Fibonacci series.
Complexity Analysis
The table below provides a clear comparison of the time complexities for each approach to computing the Fibonacci series. We have two approaches where we generate the Fibonacci series using recursion in C and then the iterative and dynamic approaches.
Approach | Time Complexity | Explanation |
---|---|---|
Recursive Printing | O(n) | Each Fibonacci number is computed and printed recursively, resulting in linear time complexity. |
Recursive Computing | O(2^n) | The recursive function is called multiple times with a branching factor of 2, leading to exponential growth in time complexity without memoization. |
Iterative | O(n) | Each Fibonacci number is computed iteratively using a loop, resulting in linear time complexity. |
Dynamic Programming | O(n) | Fibonacci numbers are computed using dynamic programming, storing intermediate results to avoid redundant calculations, resulting in linear time complexity. |
Among the listed approaches, the best approach in terms of time complexity is Dynamic Programming, with a time complexity of O(n). This approach efficiently computes Fibonacci numbers by storing intermediate results and avoiding redundant calculations.
On the other hand, the worst approach in terms of time complexity is Recursive Computing. This approach has a time complexity of O(2^n), which results in exponential growth as the input size increases. Each recursive call leads to two additional recursive calls, leading to a combinatorial explosion of function calls and making it highly inefficient for larger inputs.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
Implementing the Fibonacci series using recursion in C provides a practical example of recursion and highlights the beauty of mathematics in programming. The recursion method allows us to express the recursive nature of the Fibonacci series elegantly, capturing its self-similar structure. In this article, we explored different methods to implement the Fibonacci series in C, i.e., using recursion, iteration, and dynamic programming. Each approach has its advantages and trade-offs, highlighting the versatility of programming techniques and the rich interplay between mathematics and programming.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is the Fibonacci series, and why is it important?
The Fibonacci series is an integer sequence of numbers where each number, starting from the third one, is the sum of the two preceding numbers. It typically begins with 0 and 1, resulting in the series 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on.
The Fibonacci series holds significance in various fields, including mathematics, nature, and computer science. The Fibonacci sequence appears in numerous mathematical properties and formulas, such as the golden ratio, Fibonacci numbers, and the Fibonacci identity. In nature, the Fibonacci sequence manifests in the arrangement of leaves on plants, the branching of trees, the spiral patterns in shells and galaxies, and other natural phenomena.
Q. How does recursion work in generating the Fibonacci series?
Recursion works by breaking down a problem into smaller, simpler instances of the same problem until a base case is reached, at which point the solution can be easily calculated. When we generate the Fibonacci series using recursion in C, the series is broken into smaller Fibonacci numbers until reaching the base cases, which are typically the first two Fibonacci numbers (0 and 1).
Here's how we can generate the Fibonacci series using recursion in C:
- Base Case: The base case(s) define the stopping condition for the recursion. In the case of the Fibonacci sequence, the base cases are usually the first two Fibonacci numbers: F(0) = 0 and F(1) = 1.
- Recursive Step: The recursive step involves expressing the nth Fibonacci number (F(n)) in terms of smaller Fibonacci numbers. This is typically done by summing the (n-1)th and (n-2)th Fibonacci numbers. So, F(n) = F(n-1) + F(n-2).
- Recursion: The recursive function calls itself with smaller inputs until reaching the base case(s). This process continues until the base case is reached, after which the recursion stops, and the Fibonacci number is computed using the printf() statement.
Q. How can the time complexity of the recursive approach be analyzed?
The time complexity of the recursive approach to computing the Fibonacci series can be analyzed by considering the number of function calls required. While the naive recursive approach has exponential time complexity, memoization or dynamic programming techniques can reduce it to linear time complexity.
Q. What are some practical applications of the Fibonacci series and recursion in programming?
The Fibonacci series and recursion have numerous practical applications in programming across various domains. Some of the key applications include:
- Algorithm Design: Recursion is a fundamental technique used in designing algorithms for various tasks, such as tree traversal, graph traversal (e.g., depth-first search), and divide-and-conquer strategies. The Fibonacci series can be used as a case study to understand recursion and its application in algorithm design.
- Dynamic Programming: The Fibonacci series serves as a classic example for dynamic programming, a method for solving complex problems by breaking them down into simpler subproblems. Memoization or tabulation techniques can be applied to optimize the process of generating the Fibonacci series using recursion in C, leading to improved time complexity.
- Mathematical Modeling: The Fibonacci series appears in mathematical models for describing natural phenomena and patterns, such as the growth patterns of populations, the arrangement of leaves on plants, and the spiral patterns in shells and galaxies. Recursion can be used to model and simulate these phenomena in programming.
- Numerical Analysis: Recursion and the Fibonacci series are used in numerical analysis for solving mathematical problems, such as computing factorial, exponentiation, and calculating series expansions. Recursive algorithms can be applied to implement efficient solutions for these computations.
- Financial Analysis: The Fibonacci series and related concepts, such as the golden ratio and Fibonacci retracements, are used in financial analysis and trading strategies. Recursion can be employed to model and analyze financial data, identify patterns, and make predictions in stock markets and other financial markets.
- Data Structures: Recursion is widely used in implementing and manipulating various data structures, such as trees, graphs, and linked lists. The Fibonacci series can be used to illustrate recursive algorithms for traversing and manipulating these data structures efficiently.
Q. What are the advantages and disadvantages of computing the Fibonacci series using recursion in C programming?
There are both advantages and disadvantages of computing the Fibonacci series using recursion in C language. They are listed below.
Advantages:
- Elegant Solution: Recursion provides an elegant and concise solution for computing the Fibonacci series, mirroring the mathematical definition of the sequence. The recursive function directly reflects the recursive nature of the Fibonacci sequence, making the code intuitive and easy to understand.
- Simplicity: The process of generating the Fibonacci series using recursion in C is simplistic, as it directly expresses the mathematical recurrence relation in code. This simplicity reduces the amount of code required and enhances readability.
- Ease of Understanding: Recursion closely aligns with the mathematical definition of the Fibonacci series, making it easier for programmers to grasp the underlying logic and reasoning behind the solution. This intuitive correspondence simplifies the learning and understanding of the Fibonacci sequence and recursion.
Disadvantages:
- Performance Overhead: The solutions that compute the Fibonacci series using recursion in C programs suffer from performance overhead due to repetitive function calls and redundant calculations. As the size of the input increases, the recursive approach may lead to inefficient use of memory and slower execution times compared to iterative or dynamic programming approaches.
- Stack Overflow: Other issues that may arise when computing the Fibonacci series using recursion in C include stack overflow errors for large inputs, especially when using naive recursion without memoization or tail call optimization. Each recursive call consumes stack space, and a large number of recursive calls can exceed the stack size limit, resulting in a runtime error.
- Redundant Calculations: Naive recursive implementations of the Fibonacci series involve redundant calculations, as the same Fibonacci numbers are computed multiple times. This increases with the size of the input, leading to longer execution times and decreased performance in cases where we generate the Fibonacci series using recursion in C programs.
Here are a few more interesting topics you must read:
- Compilation In C | Detail Explanation Using Diagrams & Examples
- Ternary (Conditional) Operator In C Explained With Code Examples
- Type Casting In C | Types, Cast Functions, & More (+Code Examples)
- Control Statements In C | The Beginner's Guide (With Examples)
- The Sizeof() Operator In C | A Detailed Explanation (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment