The Sizeof() Operator In C | A Detailed Explanation (+Examples)
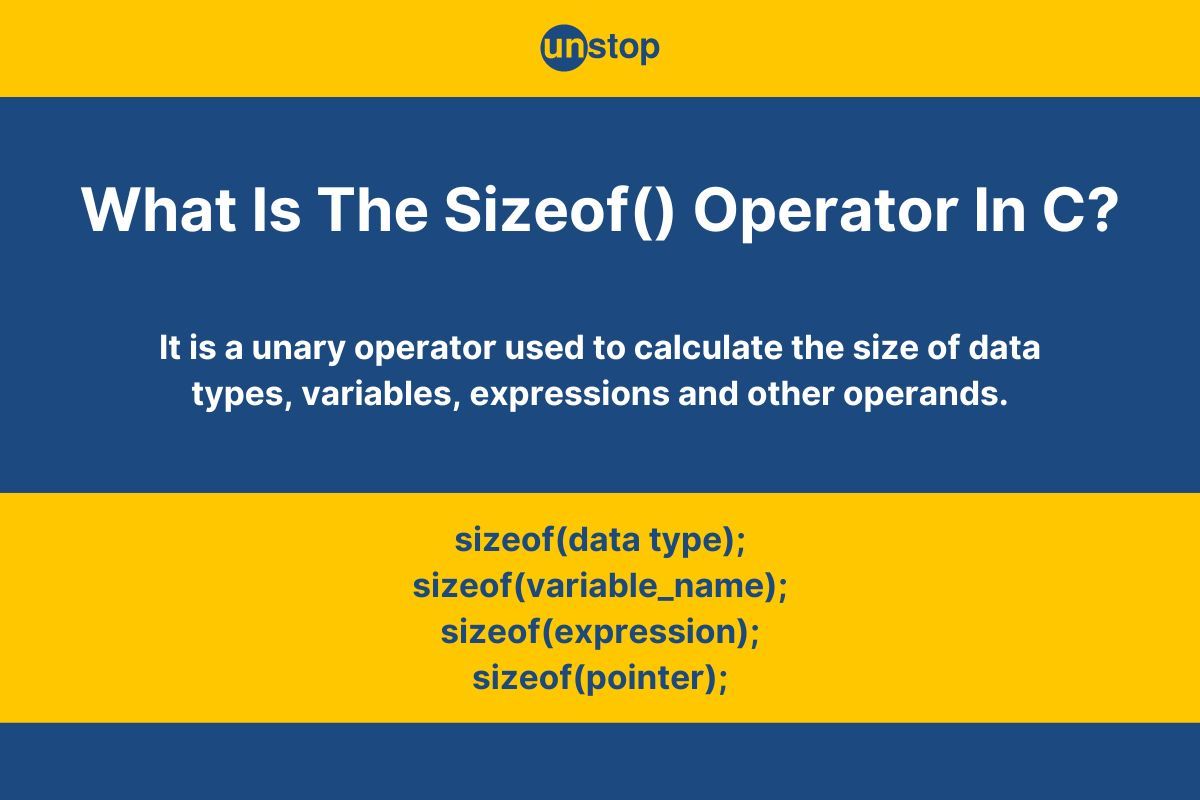
In C programming, operators are fundamental symbols used to perform various operations on operands. These operations include arithmetic calculations, relational comparisons, assignments, logical evaluations, bitwise manipulations, etc. Another operator that is often used to determine the memory size occupied by operands/ elements in bytes is the sizeof() operator in C language.
This operator not only provides us with a straightforward means to ascertain the storage size of data types, variables, or expressions but also aids in efficient memory allocation and resource management within C programs. In this article, we will cover all the basics of the sizeof() operator like its syntax, operand type, return type, applications, and more, with easy-to-understand and detailed examples.
What Is The Sizeof() Operator In C?
As mentioned above, the sizeof() operator in C returns the size of its operand in bytes. The operand could be a data type, a variable, expressions, or other elements used when writing a C program.
- For example, when working with expressions, the sizeof() operator does not evaluate the expression it operates on. Instead, it returns the size of the operand's data type in bytes.
- This value is crucial for understanding the memory requirements of variables and data structures, especially in environments where memory optimization is paramount.
- The return value of the operator depends on the type of operand. The operator returns the memory space (storage size) of a data type in bytes.
- The return type also depends on the machine, as different machines may allocate different memory space to the same operand. The size of a data type or object is not fixed and can vary from one machine to another.
- That is, different computers and processors may allocate different amounts of memory to store the same data type or object. Hence, when we use the sizeof operator in C, the return type (size_t) and the actual size of the operand being evaluated depend on the machine's architecture.
It is important to note that the sizeof() operator in C is a compile-time unary operator. This means it calculates the amount of memory space required by the operand at compile time and operates on only one input.
Syntax Of sizeof() In C
The syntax for using the sizeof operator in C programming language takes different forms depending on the type of operand it works with.
General Syntax Of Sizeof() Operator In C:
sizeof(operand)
Here,
- The keyword sizeof marks the operator's name. It is used to specify the size taken in memory by the operand.
- The operand refers to the input that sizeof will analyze.
This is the general syntax that changes as per the operand, which we will discuss further in this section. But before that, let's take a look at a basic C code example showing the implementation of the sizeof() operator.
Code Example:
Output:
The size of integer variable is: 4 bytes
The size of the string variable is: 7 bytes
Explanation:
In this simple C program, we begin by including the <stdio.h> header file essential for input/ output operations.
- Inside the main() function, we initialize two variables of different data types, i.e., an integer variable num1 with value 23 and a string variable/ character array called string with the literal value Unstop.
- Next, we use a set of printf() statements to display the size of blocks of memory occupied by these variables. The %lu format specifier in the formatted string of printf() indicates an integer value, and the newline escape sequence (\n) shifts the cursor to the next line.
- For this, we use sizeof() operator inside printf() and pass the respective variables to it as operands.
As mentioned above, there are various applications of the sizeof() operator or the forms it takes based on the type of operand it uses. Let us look at each one of them in detail one by one.
Sizeof() Operator In C When Operand Is A Data Type
We can use the sizeof() operator to find the amount of memory allocated to the primitive data types in C, such as int, float, char, double, short, and long. In addition, it can also be used to determine the size of compound data types, like structures (struct), union, or pointer data types.
SizeOf() Operator In C Syntax When Operand Is A Datatype:
sizeof(datatype)
Here, the datatype (i.e., the type of the data stored in the variable/ element) is the operand, which could be integer, string, character data type, etc.
Code Example:
Output:
The size of a char type is: 1 bytes
The size of an int type is: 4 bytes
The size of a float type is: 4 bytes
The size of a long type is: 8 bytes
The size of a short type is: 2 bytes
The size of a double type is: 8 bytes
Size of int array (5 elements): 20 bytes
Size of struct MyStructure: 8 bytes
Explanation:
In the sample C code-
- As mentioned in the code comment, we use a set of printf() statements with the built-in sizeof operator to determine and print the size of the block of memory occupied by various data types.
- These include the integer data type, char data type, float data type, long type, short type, and double data type. For this, we pass each data type as a parameter to the sizeof() operator.
- Next, we declare an integer array arr containing 5 elements. Then again, we use the sizeof() operator inside the printf() statement to display the size occupied by this array to the console.
- After that, we create a structure MyStructure using the struct keyword. Since a structure can contain multiple types of elements, we have an integer member element, x, and a character type member element, y.
- Then, we use the sizeof() operator to determine the size of this struct and print it to the console using a printf() statement.
- Finally, the program terminates with a return 0 statement.
Sizeof() Operator In C When Operand Is A Variable Name
We can use the sizeof() operator to find the amount of memory allocated to different variables which were previously declared in the program. The memory used by these variables is independent of the value they hold.
SizeOf() Operator In C Syntax When Operand Is A Variable:
sizeof(variable_name)
Here, the variable_name refers to the variable, and the sizeof operator will return the amount of memory it will occupy.
Code Example:
Output:
The size of num variable is: 4
The size of character variable is: 1
The size of floating_point variable is: 4
Explanation:
In the example C program-
- We declare three variables of different data types, i.e., integer variable num, char variable character, and float type variable floating_point. We assign values 10, a, and 1.23 to them, respectively.
- Then, we use the built-in sizeof operator to determine the memory size occupied by each variable by passing the variable name/ identifier as the parameter. This operator evaluates the size based on the data type of the operand passed to it.
- Once the size is determined, the printf() statements display the same on the console.
Sizeof() Operator In C When Operand Is An Expression
We can use the sizeof() operator to find the amount of memory that will be allocated to store the resultant of the expression. The operator first evaluates the final datatype of the resultant value of the expression and returns the size it will take up in the memory.
SizeOf() Operator In C Syntax When Operand Is An Expression:
sizeof(expression)
Here, the operand is an expression, and the sizeof() operator determines the storage size/ memory storage space the output of this expression will occupy.
Code Example:
Output:
The size of the result is: 4
The size of the outcome value is: 8
Explanation:
In the example C code-
- We first declare and initialize two integer variables, num1 and num2, with the values 10 and 5, respectively.
- Then, we use the syntax of sizeof when the operand is an expression and print the result to the console using a printf() statement.
- In the expression, we simply perform an addition operation on the two variables and calculate the size occupied by the outcome, i.e., sizeof( num1 + num2). here, since both operands are of type int, the resultant expression is also of type int with a size of 4 bytes.
- We then declare another variable dub of double type and assign the value 11.56 to it.
- Next, we have another printf() statement with the sizeof() operator to calculate the size of a second expression, i.e., sizeof(num1 + dub).
- In this expression, we use the arithmetic addition operator to add the values of num1 and dub. Here, the operands are of different types, i.e., int and double, so the result is of type double, and its size is 8 bytes.
Sizeof() Operator In C When Operand Is A Pointer
We can use the sizeof() operator to find the amount of memory that will be allocated to store the pointer to some variable. The sizeof() operator will basically return the size of the address held by the pointer to a variable.
SizeOf() Operator In C Syntax When Operand Is A Pointer:
sizeof(ptr)
Here, ptr represents the pointer type operand, and the sizeof operator will return the amount/ bytes of memory the pointer will occupy.
Code Example:
Output:
The size of integer pointer is: 8
The size of character pointer is: 8
Explanation:
In this example-
- First, we declare an integer variable num1 and assign the value 10 to it.
- Then we create an integer pointer, numptr, and assign the memory address of num1 variable to it using the addressof/ reference operator (&).
- We then initialize a new character variable, c, and assign the character 's' to it. Then, we create a character pointer, charptr, and assign the address of the c variable to it.
- Next, we create two pointer variables, one of integer type and the other of character type.
- After that, we use two printf() statements with the sizeof() operator to determine the amount of space these pointers will take and print the same to the console.
- Lastly, the program terminates with a return 0 statement indicating successful execution without any errors.
- Notably, in the provided code, both pointer variables numptr and charptr have a size of 8 bytes. This is because pointers store memory addresses and, on a 64-bit system, are implemented as 64-bit addresses, hence requiring 8 bytes of memory.
In this section, we have seen The C code example showcases the use of the sizeof() operator in C language with a variety of operands, including data type, variables, expressions, and pointers.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Return Type Of sizeof() Operator In C
The return type of the sizeof() operator in C is an unsigned integer type defined in the standard C libraries, typically in <stddef.h> or <stdlib.h>. It is basically the amount of memory in bytes occupied by the operand. It is specifically designed to represent the sizes of objects in memory and guarantees that it can hold the size of the largest object that can exist in memory on the current platform. So when we use the printf() function to display the size of the operand, we can use the %lu format specifier, which is used for unsigned long integers.
Code Example:
Output:
The size of x is: 4
The size of x is: 4
The size of d is: 8
Explanation:
In the C example program-
- We initialize an integer variable x with the value 56 and then use the sizeof() operator inside a printf() statement to display the space occupied by the variable.
- Here, we use the %lu format specifier to indicate a long unsigned integer value. An integer usually occupies 4 bytes of space, as shown in the output.
- Next, we reassign the value 10 to the integer variable x and again print the size occupied by it using the sizeof() operator inside printf().
- The size occupied remains 4 bytes since the value is still an integer. This shows that the sizeof() operator is dependent on the data type and not affected by the actual value of the variable.
- Taking it one step further, we declare a double data type variable d and assign it the value 10.23.
- Again, we use the sizeof() operator inside a printf() statement to calculate and display the size it occupies. A double type commonly occupies 8 bytes of memory on many systems, which is reflected in the output.
How Does The sizeof() Operator In C Work?
As mentioned before, the size of() operator is a compile-time operator, i.e., its result is determined by the compiler during the compilation phase, and the actual size information is embedded into the generated machine code. Here's how the sizeof() operator works in C:
1. Compile-Time Evaluation: The sizeof() operator is evaluated by the compiler during the compilation process of a C program. It is not executed at runtime; instead, the compiler determines the size of the specified type or object while translating the source code into machine code.
2. Determine Size of Data Type or Object: When you use sizeof(type) or sizeof(expression), the compiler calculates the size of the specified data type or object. For example, sizeof(int) would give you the size of an int data type in bytes.
3. Replace with Constant Value: The compiler replaces the sizeof() expression with a constant value representing the size in bytes. This constant is determined based on the target platform and the data type or object being evaluated.
4. Usage in Memory Allocation and Operations: The result of sizeof() is often used in memory-related operations. For instance, when dynamically allocating memory using malloc or calloc, the size to allocate is often specified using sizeof(type) * number_of_elements. It is also used when calculating offsets in structures or arrays.
Let's look at the example below to understand it better:
Example:
Output:
Size of int: 4 bytes
Size of float: 4 bytes
Size of double: 8 bytes
Size of char: 1 bytes
Size of int array (5 elements): 20 bytes
Size of ExampleStruct: 16 bytes
Explanation:
In the code above-
- In the main() function, we use several printf() statements, each using the sizeof() operator to determine and print the sizes of various data types. These data types include int, float, double, and char.
- We then use %lu format specifier in printf() to correctly display the size obtained from sizeof() as an unsigned long integer.
- Additionally, within the code, we demonstrate the use of sizeof() with an array and a structure. An integer array arr of size 5 is declared. We print the size of this array using sizeof(arr).
- Next, a structure named ExampleStruct is defined with three members: int x, char y, and double z.
- We then print the size of this structure using sizeof(struct ExampleStruct).
- Finally, the program returns 0, indicating successful execution.
Need Of The sizeof() Operator In C Programming
The sizeof() operator in C plays a crucial role in various programming scenarios, particularly in the context of dynamic memory allocation. Its significance stems from its ability to determine the size of data types, providing a versatile tool for effective memory management during runtime.
Dynamic Memory Allocation
Dynamic memory allocation is a memory management mechanism by which memory can be allocated to variables during run time. In C programming, there are several functions available to facilitate dynamic memory allocation, such as the malloc() function. The need for sizeof() becomes apparent in scenarios involving dynamic memory allocation because when allocating memory dynamically, it's often crucial to ascertain the size of a particular data type.
For instance, if we wish to allocate space for 10 units of an unknown data type, sizeof() helps determine the size of that data type, allowing precise memory allocation using the malloc() function. In essence, the sizeof() operator empowers programmers with a flexible and machine-independent means of obtaining data type sizes, ensuring accurate dynamic memory allocation tailored to the requirements of the program.
Code Example:
Explanation:
In this C code example-
- We begin by declaring an integer pointer ptr to point to an integer variable.
- We assign the pointer the address of an integer variable by dynamically allocating a bloc of memory to it.
- For this, we first use the sizeof() operator to determine the size of the integer type and multiply it by 10. This ensures that the memory for 10 integers is reserved on the heap.
- Then, the malloc() function dynamically allocates that memory space to ptr, and we use the typecast operator to convert the pointer returned by malloc() to an integer pointer type variable. That is (int*)malloc().
- It's important to note that the allocated memory is not initialized and may contain arbitrary values. So, there is no real output unless we assign some value to the pointer.
Find Number Of Elements In An Array
We can also use the sizeof() operator to find the number of elements in an array. Since all the elements in an array are of the same data type, they occupy the same amount of memory space. Therefore, if we find out the total amount of memory occupied by an array and the amount of memory a single element of that datatype occupies, we can find the number of elements present in the array.
To get this done, we use the sizeof() operator by passing the parameters name of the array and the first element of the array, respectively. Let us look at an example to see how it is implemented.
Code Example:
Output:
Number of elements in the array is 12
Explanation:
In the sample C code-
- We initialize an integer array, arr, in the main() function, with 12 integer values separated by the comma operator.
- Then, we use a printf() statement to display the number of elements in the array to the console. For this-
- We use the sizeof() operator to calculate the size of a single element of the array, i.e., sizeof(arr[0]), and the size of the array as a whole, i.e., sizeof(arr).
- Next, we divide the size of the whole array by the size of a single element and get the number of elements. This calculation is done to make the code adaptable to changes in the array size.
What Do We Mean By sizeof() Being A Compile-time Operator?
The sizeof operator in C is considered a compile-time operator because its functionality is determined and resolved during the compilation phase of the program, not at runtime. This means that the size of a data type or object, which is obtained using sizeof(), is known to the compiler before the program is executed.
- When you use sizeof in your C code, the compiler evaluates the size of the specified type or object and replaces the sizeof expression with a constant value representing the size in bytes.
- This value is then used by the compiler to allocate memory, determine offsets, and perform other compile-time operations.
For example, in the code given below, the sizeof(int) expression is evaluated by the compiler during compilation. The result is replaced with the actual size of an int on the target platform, and the program prints this information. The entire process happens at compile time, and the compiled program already "knows" the size of an int before it runs.
Code Example:
Output:
Size of int: 4 bytes
Explanation:
In this example in C language-
- Inside the main() function, we declare a variable named size_of_int and assign the result of the sizeof(int) expression to it. This expression determines the size (in bytes) of the int data type.
- The printf() function is then used to print the size of the int data type to the console. The format specifier %d is used to print an integer, and the value of size_of_int is provided as an argument.
- The printed message includes information about the size of the int data type in bytes.
- The return 0 statement indicates that the program has been executed successfully and returns a status code of 0 to the operating system.
Advantages Of The sizeof() Operator In C
The sizeof() operator in C is a very useful operator used while programming. Some of the advantages are listed below:
- We can find the size of memory used by an operand using it, which can be a variable, datatype, pointer, or expression.
- We can use the sizeof() to calculate and find the number of elements present in the array.
- The sizeof() operator can also be used to determine the type of system on which the program is being executed, as it is a compiler-dependent operator.
- We can also use the sizeof() to dynamically allocate memory when we don’t know the size of a datatype on a particular compiler.
Conclusion
In this article, we learned all about the sizeof() operator in C. It primarily helps us determine the amount of memory space allocated depending on the operand passed to it. The operand can be a variable, datatype, pointer, or expression, and the return type is dependent on the type of operand and the compiler.
The applications of this unary compile-time operator are diverse, such as dynamic memory allocation, assessing the number of elements in an array, size of various elements, and more. We discussed the different syntaxes of the sizeof() operator in C based on operand, the return type, the working mechanism, and advantages in great detail with a clear and easy understanding.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. Why do we use the sizeof() operator in the C language?
The sizeof () operator in C is a common operator used to determine the size of memory occupied by an operand.
- The sizeof() operator consists of just one operand, meaning it is a unary operator that can take a data type, variable, pointer, or expression enclosed within the parenthesis as a parameter.
- The result given by the sizeof() operator depends on the operand it uses and is also dependent on the compiler.
- It has other applications, such as finding the number of elements present in the array and dynamic memory allocation using the malloc() function.
Q. What are the different data types and the sizes they occupy in C?
There are many data types in C, with each requiring a certain amount of memory. The table below lists the most common types and the memory in bytes occupied by them.
Data Type |
Size In Bytes |
Integer data type (int) |
4 |
Short int |
2 |
Unsigned int |
4 |
Unsigned short int |
2 |
Long int |
4 |
Unsigned long int |
4 |
Signed char data type |
1 |
Unsigned char data type |
1 |
Float data type |
4 |
Double type |
8 |
Q. What are the basic types of operators in C?
Operators are elements in programming that help us perform various manipulations on operands. Some common operations are mathematical computations, comparisons/ relational comparisons, logical operations, and operations on but level. There are 6 primary types of operators, as listed in the table below.
Operator Type/ Name |
Description |
Arithmetic Operators (+, -, *, /, %) |
They help us perform mathematical computations like addition, subtraction, division, etc. |
Relational Operators (<, >, ==, !=, <=, >=) |
They help compare two operands for equality and or inequality. |
They are used to compare the values of two or more operands and draw inferences based on that. |
|
These operators help us compare the values of operands on the bit level. |
|
There are simple and compound operators that are used to assign values to variables/ elements. |
|
Special Operators |
The sizeof() operator determines the size, and the addressof operator (&) references a variable. |
Some other operators include the ternary/ conditional operator and the increment/ decrement operator.
Q. What is the size of a variable?
A variable is an identifier of a memory location that can store different types of data/ values. The size of the variable depends on the data type it stores, which can be int, char, float, double, etc.
Code Example:
Output:
Size of int: 4 bytes
Size of float: 4 bytes
Size of char: 1 bytes
Explanation:
This example C program declares variables of different data types (int, float, char) and uses the sizeof operator to print their respective sizes in bytes. The output provides information about the memory occupied by each variable type.
Q. Which type of format specifier does the sizeof() operator use?
The sizeof() operator in C is employed to determine the size of a data type or a variable in terms of bytes. It returns the size as an unsigned integer. When presenting this size using the printf function, the format specifier %lu is typically used to denote an unsigned long integer. This format specifier is suitable for printing the result of sizeof() because the size is represented as an unsigned long integer value.
Q. How to calculate the size of an array in C?
We can use the sizeof() operator in C to find the size of an array. For this, we first determine the size of the whole array, i.e., the entire array space in bytes, and the the size of a single element of the array. Then, we divide the size of the whole array by the size of a single element, which results in the number of elements in the array.
Syntax:
data_type size = sizeof(Array_name) / sizeof(Array_name[index]);
Here,
- The data_type refers to the type of variables/ data we want to store the size of the array.(like int, size_t, etc.).
- The name/ identifier of the array is given by the term Array_name.
- The sizeof(Array_name) determines the size of the entire array in bytes, and sizeof(Array_name[index]) returns the size of a single element in the array in bytes.
- The term index indicates the position of the element in the array.
Code Example:
Output:
The length of the array is: 8
Time Complexity: O(1)
Space Complexity: O(1)
We are at the end of our discussion on the sizeof() operator in C programming. Here are a few other amazing reads you will enjoy:
- Length Of String In C | 7 Methods Explained With Detailed Examples
- Conditional/ If-Else Statements In C | The Ultimate Guide
- Entry Control Loop And Exit Control Loop In C Explained (With Codes)
- Difference Between Break And Continue Statements In C (+Explanation)
- Control Statements In C | The Beginner's Guide (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment