Entry Control Loop And Exit Control Loop In C Explained (With Codes)
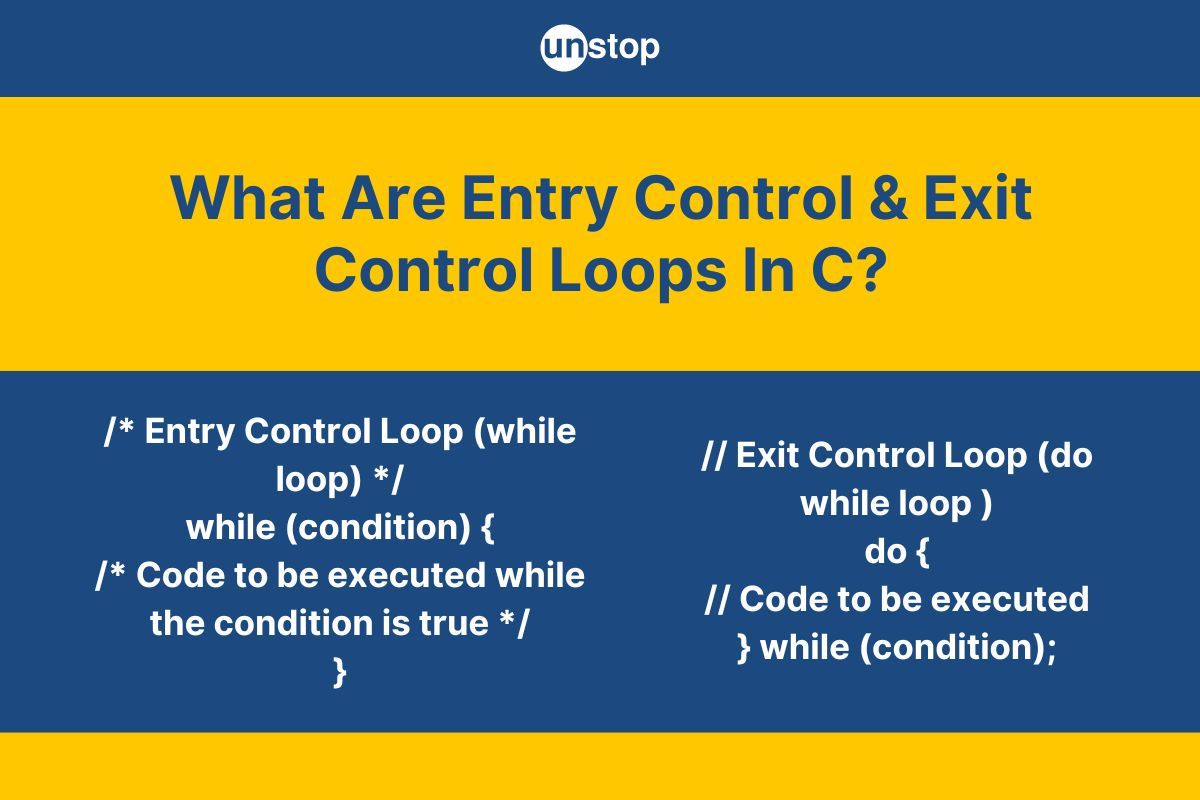
A loop is a type of control flow structure/ control statement used in computer programming. It enables a set of instructions to be carried out repeatedly or periodically depending on a condition. It may also run for a predetermined number of repetitions. There are two major kinds of loops, i.e., entry control loop and exit control loop in C programming.
These loops allow for the efficient handling of processes that must be repeated several times, as well as the automation of repetitive jobs. You may make your programs shorter and simpler to maintain by using loops to prevent repeatedly writing the same code. Each type of loop has its specific use cases and can be chosen based on the requirements of the program and the iteration control needed. It's essential to use loops wisely to ensure efficient and effective program execution.
What Are Entry Control Loops And Exit Control Loops?
The terms entry control loop and exit control loop are frequently used in the world of computer programming and software development. They refer to particular control structures that specify how a program's execution will proceed.
- Entry Controlled Loop: It is a control structure that regulates how an object enters a loop. At the very beginning, the control loop checks the condition, and the control flows through the loop if the entry control loop condition is true. If the loop condition is false, then the loop is skipped, and the program moves on to the sentence that follows the loop. There are two types of entry-controlled loops, i.e., the for loop and the while loop.
- Exit Controlled Loop: An exit control loop is a control statement that is based on regulating how a loop is terminated. That is, the code inside the loop will be executed at least once, and the control loop condition expression is checked at the exit of the iteration. After each repetitive execution of the loop, a condition is checked, and the loop is ended if the condition is false. The loop continues to run if the condition is true. The do-while is an example of an exit control statement/ loop.
Differences Between Entry Control Loop and Exit Control Loop In C
The difference between entry control loop and exit control loop in C programming or general programming is evident in the name. While the former type of loop structure must meet the loop condition for the current loop execution to be realized, the former checks the condition after the first loop execution is done. Therefore, the key contrast between entry control loop and exit control loop in C language is the implementation and exit control mechanisms.
The table below highlights the difference between entry control loop and exit control loop in C.
Entry Control Loop |
Exit Control Loop |
The loop condition is checked before entering the loop. |
The condition check occurs at the end of each iteration. |
The loop may not execute at all if the condition is false initially. |
The loop executes at least once before checking the condition. |
Examples: while loop, for loop |
Example: do while loop |
Suitable when it is uncertain whether the loop body needs to be executed. It may or may not run based on the initial condition. |
Suitable when the loop body must be executed at least once, regardless of the initial condition. It ensures that the loop body is executed before checking the condition. |
Syntax: // Entry Control Loop (while loop) |
Syntax: // Exit Control Loop (do while loop ) |
Entry Control Loop In C
As mentioned before, an entry control loop in C is a type of loop in which the loop condition is examined prior to entering the body of the loop. The body of the loop is executed if the condition is true and will be skipped if the condition is false. The while loop and the for loop are a few examples of entry control loops in C.
Execution Flow of Entry Control Loop In C
- Initialization: The loop control variable/s are initialized before entering the loop. This step is mostly done outside the loop; however, in the case of for loops, we can initialize inside the loop itself.
- Condition Evaluation: Next, the condition of the loop is evaluated. If the condition is true, the lines of code inside the loop are executed. If the condition is false, the program moves on to the next statement after the loop.
- Code Block Execution: The code block inside the loop is executed if the condition in the previous step is true. The repetitive execution continues as long as the condition remains true.
- Iteration: After execution of the piece of code inside the loop, the program returns to the beginning of the loop and re-evaluates the condition.
- Condition Check: The condition is checked again. If the condition is true, the execution returns to step 3, and the code block is executed again. If the condition is false, the program exits the loop and continues with the next statement after the loop.
Steps 3 through 5 of the execution flows are repeated until the condition is no longer true, at which time the program ends the loop and moves on to the statement that follows it.
Also read- How To Run C Program | Step-by-Step Explanation (With Examples)
While Loop In C
While a given condition is true, the while loop continually runs a block of code. Since the while loop is an entrance control loop in C, the loop condition is assessed before the body of the loop is entered. The body of the loop is executed, and the loop iterates as long as the condition is true. The loop ends when the condition is false, and the program continues on to the statement that follows.
Syntax
while (condition) {
// Code to be executed
}
Here,
- The while keyword marks the beginning of the loop.
- The condition represents the test expression that must return true or the loop to run.
- The curly braces contain the code statements.
Code Example:
Output
1 2 3 4 5 6 7 8 9 10
Explanation:
In this simple C program, we begin by importing the <stdio.h> header file for input/ output operations.
- We then declare a variable i, of integer data type and assign the value 1 to it.
- Then, as mentioned in the code comments, we create a while loop to print the numbers from 1 to 10.
- The while loop's condition is i <= 10, which means the loop will continue executing as long as the value of i is less than or equal to 10.
- We have a printf() statement inside the loop body execution, which displays the current value of variable i. The %d format specifier here represents an integer value.
- After that, the increment operator increases the value of i by 1 before we again move to check the loop condition.
- The loop continues to execute until i becomes 11. When i is 11, the condition i <= 10 becomes false, and the loop exits.
- Finally, the program terminates with a return 0 statement, signifying successful execution without errors.
For Loop In C
The for loop is another entry-controlled loop, i.e. the control loop checks condition before loop body execution. If the condition is true, the code block inside the loop is executed and terminates if the condition is false. There is also an updating statement which updates the value of the control variable in each iteration.
Syntax
for (initialization; condition; update) {
// Code to be executed
// ...
}
Here,
- The for keyword marks the beginning of the loop, and the curly braces contain the code that will be executed if the loop condition is true.
- The terms initialization, condition, and update refer to the expressions that make variable initialization, loop condition and the updation expression.
Code Example:
Output:
1 2 3 4 5 6 7 8 9 10
Explanation:
In this C code example-
- We create the main() function, which is the entry of the program's execution.
- Inside main(), we create a for loop to 10 print numbers. The variable initialization statement sets the initial value of control variable i to 1.
- Then, the program checks the loop condition, i.e., i <= 10. If the condition is true, the code block inside the loop is executed.
- Inside the loop, we have a print() statement which displays the current value of variable i.
- Then, the updation expression increases the value of the variable by 1.
- The loop continues as long as the condition i <= 10 is true. When i becomes 11, the condition becomes false, and the loop terminates.
The for loop provides a concise way to control the loop initialization, condition, and increment, making it easier to handle sequences and repetitions with a clear structure. In this example, it allows us to print numbers from 1 to 10 in a straightforward and organized manner.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Exit Control Loop In C
As mentioned before, an exit control loop is one where the loop body execution is done first, and then the loop condition is checked. That is, flow is controlled by an exit condition that is evaluated at the end of each loop iteration. So, the loop body will definitely be executed at least once before evaluating the exit condition.
In the case of exit control loops (like do-while), the loop condition can also be considered as the condition for termination. That is, the loop is terminated if the condition is false, and the flow of control directly moves to the next line of the program.
Execution Flow of Exit Control Loop In C
- Initialization: The loop begins with the execution of the statements inside the do block. This block contains the code that you want to repeat.
- Loop Body Execution: As soon as the loop begins, the body of the loop is executed. The statements inside the do block are executed. This ensures that the loop code block is executed at least once.
- Condition Check: After the execution of the do block, the condition specified in the while statement is evaluated. If the condition is true, the loop body will be executed again; otherwise, the loop will be terminated.
- Termination: If the condition is true, the control goes back to the do block, and the loop body is executed again. If the condition is false, the loop terminates, and the program continues with the next statement after the while loop.
Do-while Loop
In a do-while loop, the body is executed at least once before assessing the loop condition. When the loop's body has finished running, it checks to see if the condition is true, and if it is, it runs the body again. For as long as the loop condition is still true, the loop iterates. The program moves on to the following statement if the condition turns out to be false, ending the loop.
The do-while loop is useful when you need to execute a block of code at least once and then decide whether to continue or stop the loop based on a condition.
Syntax
do {
// Code to be executed
} while (condition);
Here,
- Do and while are the keywords marking the two stages of the loop, and the curly braces contain the code snippet that is executed.
- The condition is the loop condition, which determines if the loop is repeated or skipped.
Code Example:
Output:
1 2 3 4 5
Explanation:
In the sample C program above-
- We declare and initialize an integer variable i to the value of 1 inside the main() function.
- Next, we create a do-while loop to print numbers from 1 to 5. Since this is an exit control loop, the code inside the loop is first executed.
- It contains a printf() statement to display the value of control variable i. Then, the value of i increases by 1 (i.e., i++), which means it will take the next value in the sequence.
- Next, the program determines if the loop condition is true or false, i.e., i<=5.
- The loop continues to execute as long as the condition (i <= 5) is true. As a result, the output consists of numbers 1 to 5.
- Once i becomes 6, the condition (i <= 5) becomes false, and the loop terminates.
Conclusion
In conclusion, entry control loops and exit control loops in C provide different ways to control the repetition of code in a program. Entry control loops check the loop condition before entering the loop, while exit control loops ensure the loop body is executed at least once before evaluating the exit condition. Both types of loops have their use cases and are valuable tools for managing the flow of execution and achieving specific programming tasks efficiently. Programmers can choose between entry control loop and exit control loop based on the requirements of their program and the desired behaviour for loop execution.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. What are the 3 types of loops in C?
Loop constructs are structures inside a program that allow repetitive execution of the same piece of code without the need to write it again and again. The consists of the loop and updating conditions to determine the number of times or situations in which the execution happens. The 3 primary types of loops are:
For Loop: It is mostly used when you know the number of iterations in advance. It consists of three parts, i.e., initialization, condition, and increment/decrement. The loop repeatedly executes a block of code as long as the condition remains true.
for (initialization; condition; increment/decrement) {
// Code to be executed in each iteration
}
While Loop: The while loop is used when the number of iterations is not known in advance and is determined by a condition. It consists of a condition, and the loop continues as long as the condition is true.
while (condition) {
// Code to be executed in each iteration
}
Do-while: This loop ensures that the code block will run at least once. Since the loop is exit-controlled, the condition is verified after each iteration. If the condition is true, the loop keeps running; if it is false, it is stopped.
do {
// Code to be executed in each iteration
} while (condition);
Q. What is another name for a control loop?
In programming, an iterative loop is another term for a control loop. The phrase iterative describes the repetitive execution of a set of instructions till a predetermined condition is satisfied or a particular result is obtained.
A loop offers a mechanism to cycle around a block of code, enabling repetition and proper management of program execution. Each iteration ends with a check for the condition. If the condition is true, the loop keeps running; if it is false, it is stopped.
Q. Which loop should I use to calculate the factorial of a number?
It is ideal to use an entry control loop to calculate the factorial of a number. The for loop is commonly used to calculate the factorial of a number because it allows you to control the number of iterations precisely, which is crucial for calculating factorials.
Example:
Output:
Enter a number: 6
Factorial of 6 = 720
Explanation:
In this example, the for loop initializes i to 1, iterates as long as i is less than or equal to the input number (num), and increments i in each iteration. Inside the loop, the factorial is updated by multiplying it with the current value of i. This loop structure is concise and commonly used for tasks with a known number of iterations, such as factorial calculations.
Q. What is the entry control loop in C?
In C programming, an entry control loop refers to a loop construct where the condition is checked before the loop body is executed. In other words, the loop body execution is dependent on the condition being true. The while loop and the for loop are examples of entry control loops.
In other words, in an entry control loop, first the loop condition is evaluated. If it is true, the loop body is executed. If the condition is false initially, the loop is not entered, and the loop body is skipped entirely. The loop continues executing as long as the condition remains true.
Here's an example of an entry control while loop:
int i = 0;
while (i < 5) {
printf("%d ", i);
i++;}
Output:
0 1 2 3 4
Explanation
In this example, the condition i < 5 is checked before each iteration. As long as i is less than 5, the loop body is executed, which prints the value of i. The loop continues until i reach 5, at which point the condition becomes false, and the loop is exited.
Q. What are the 3 parts of loop control?
The three parts of loop control in programming are typically associated with the for loop. These parts are
- Initialization: This part is responsible for initializing the loop control variable before the loop begins. It sets the initial value of the variable and is executed only once at the beginning of the loop.
- Condition: The condition is evaluated before each iteration of the loop. It determines whether the loop should continue executing or terminate. If the condition is true, the loop body is executed. If the condition is false, the loop is exited, and the program flow continues to the next statement after the loop.
- Increment/Decrement: This part specifies how the loop variable is modified after each iteration. It updates the loop variable so that the loop moves toward its termination condition. It is executed at the end of each iteration immediately before the condition is checked again.
Q. Can I use break in both entry control loops and exit control loops in C?
Yes, you can use the break statement in both entry-controlled loops (like while and for loops) and exit-controlled loops (like do-while loops) in C. The break statement is used to terminate the loop prematurely, regardless of the loop condition. It is commonly used to exit a loop based on a certain condition without waiting for the loop's normal termination condition.
This compiles our discussion on entry control loop and exit control loop in C. Here are a few other topics you must read:
- Compilation In C | A Step-By-Step Explanation & More (+Examples)
- Transpose Of A Matrix In C | Properties, Algorithm, Examples & More!
- Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
- Conditional/ If-Else Statements In C | The Ultimate Guide
- Tokens In C | A Complete Guide To 7 Token Types (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment