Difference Between Break And Continue Statements In C (+Explanation)
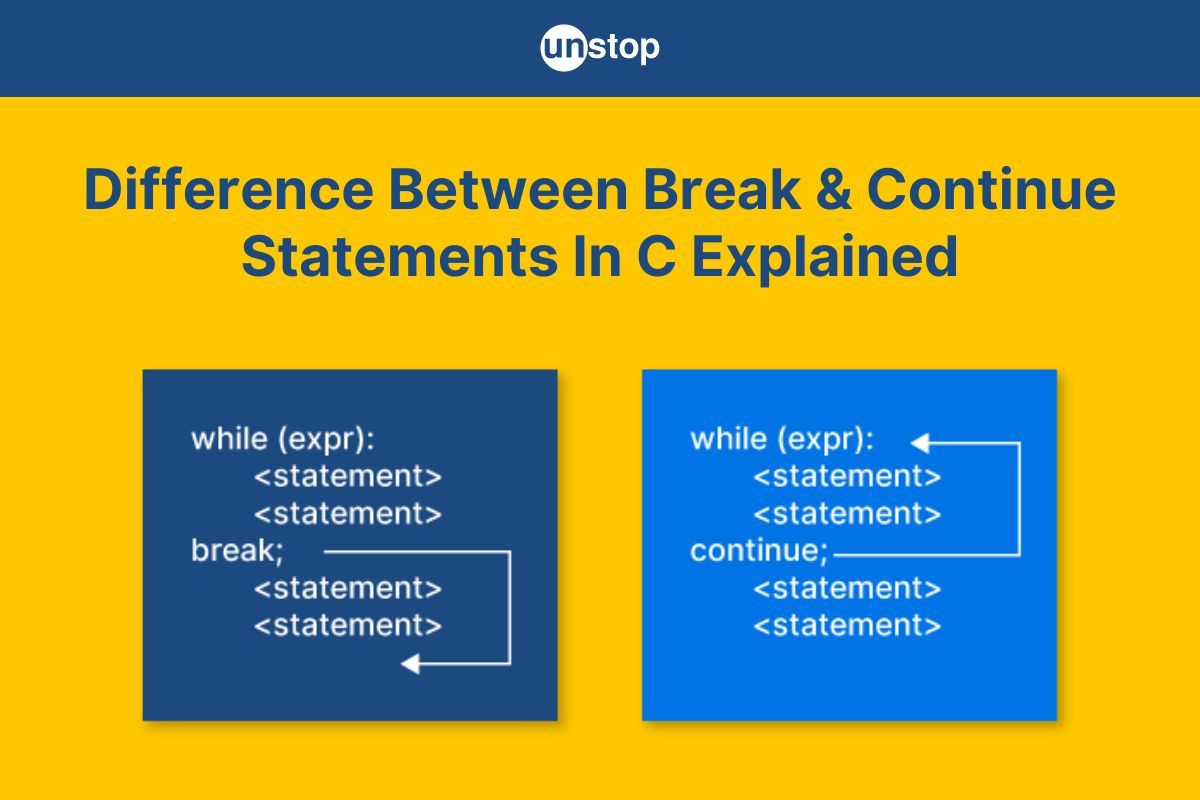
The C programming language provides two essential flow control statements, i.e., break and continue, which are used within loops to manipulate the control flow of the program. Both the break and continue statements are also known as jump statements. While both statements influence the iteration process, they serve distinct purposes. This article will delve into the key differences between the break and continue statements in C.
Key Differences Between Break And Continue Statements
The break statement is used within a loop or switch statement to terminate the loop or exit the switch. It is typically used within conditional statements. The continue statement is used within a loop to skip the rest of the code in the current iteration and begin the next iteration. It is also used within conditional statements.
Given in the table below are the primary differences between break and continue statements in C language.
Category |
Break Statement |
Continue Statement |
Basic purpose |
The break statement has the ability to halt the entire execution flow within a loop. |
The continue statement has the ability to skip/ stop the ongoing iteration in the loop. |
Iterations |
It is particularly useful when a specific condition is satisfied, allowing for early termination of the loop without the need to iterate through any remaining iterations. |
It allows the loop to smoothly move on to the next iteration, effectively bypassing the remaining code in the current iteration. |
Applicability |
It can be utilized to enhance the code efficiency and flexibility of control flow structures. Can be used in switch, for, while, and do-while loop statements. |
It is not used for switch statements but primarily used in for, while, and do-while loops |
Execution & Exit |
It provides the ability to swiftly exit the loop as soon as it is encountered. |
By skipping the remaining code within the current iteration, it seamlessly proceeds to the next iteration, ensuring a smooth and efficient execution flow. |
Flow |
In this, the flow control of the loop is interrupted entirely. |
In this, only the current iteration’s flow is interrupted. |
Syntax |
break; |
continue; |
Example |
for(int i = 0; i < 10; i++) { if(i == 5) break; } |
for(int i = 0; i < 10; i++) { if(i == 5) continue; } |
Now that we know about the key differences between break and continue statements, let's discuss each of these in greater detail for a better understanding of the same.
What Is Break Statement In C?
The break statement plays a crucial role in controlling the flow of a loop. It provides a means to exit the loop prematurely, regardless of whether the loop's termination condition has been satisfied.
In other words, by placing a break statement within the loop body and specifying a condition, you can determine the exact moment when the loop should be terminated. Once this condition is met, the break statement immediately halts the execution of the loop, allowing the program to proceed to the next statement outside the current loop iteration.
The syntax for the break statement:
for (initialization; condition; update) {
// Code block
if (break_condition) {
break;}
// More code
}
Here,
- The for keyword marks the beginning of the for loop, where the initialization condition initializes the loop control variable.
- The condition refers to the test expression, which is checked for the loop.
- The update condition is what dictates how to update the value of the control variable after every iteration.
- The if keyword marks the if statement with the break_condition, which must be met for the break statement to be executed.
- The code block contains the code to be executed during each iteration of the loop.
Below is the flowchart, which shows how the control flows through a loop to reach a break statement.
How Does The Break Statement Work?
- Iteration Check: During each iteration of a loop, the program checks a specific condition.
- Encounter break: If the condition for the break statement is met, the program encounters the break statement.
- Immediate Exit: Upon encountering a break, the control immediately exits the loop or switch statement, regardless of whether the loop's conditions are satisfied.
- Jump to Next Statement: The control then jumps to the statement immediately following the terminated loop or switch.
- End of Execution: The loop is effectively terminated, and the program continues with the rest of the code after the loop or switch.
Given below is an example C program of the break statement to help clarify the flow and working mechanism.
Code Example:
Output:
0 1 2 3 4 5 6 7 8
Exited the loop after i=8
Explanation:
In the sample C code, we first include the <stdio.h> header file so that we can use the input/ output functions.
- We then declare a variable i of integer data type inside the main() function without initialization.
- Then, we create a for loop to iteratively print the value of integers from 0 to 20. Inside the loop-
- The initial value of the loop variable is set to 0, and the condition is that the value is below 20.
- As long as the condition is met, the printf() function displays the value to the console, where the %d format specifier indicates an integer value.
- Also, the value is incremented by 1 after every iteration.
- The loop also contains an if-statement where the condition uses the relational equality operator to check whether the value of i equals 8.
- As mentioned in code comments, if the condition true, the break statement inside it is executed, and the loop is terminated prematurely.
- After the loop terminates, we use another printf() statement to display a string message indicating that the loop exited after a specific value of i.
- The newline escape sequence ensures that this message is printed in the next line.
- Finally, the return 0 statement then signals that the program has run its course successfully by returning a value of 0 without any errors.
- In this example, as you can see from the output, none of the values after 8 are printed because the break statement is encountered when i==8. The values before this are printed as there was no break statement before i==8.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
What Is Continue Statement In C?
The continue statement allows us to skip a specific iteration in a loop without stopping the entire loop. If a condition is met, the iteration is skipped, and the loop proceeds to the next iteration. If the condition is not true, the remaining code within the iteration is executed. This provides a way to control the flow of execution within a loop based on specific conditions.
The syntax for the continue statement:
for (initialization; condition; update) {
// Code block
if (continue_condition) {
continue;}
// More code
}
Here;
- Initialization: Typically initializes a loop control variable.
- Condition: Checks a condition for each iteration. When continue_condition is met, the continue statement is executed.
- Update: Modifies the loop control variable after each iteration.
- Code Block: Contains the code to be executed during each iteration of the loop.
The flowchart below shows how the flow moves in a loop where we encounter a continue statement.
How Does The Continue Statement Work?
- Iteration Check: Similar to break, during each iteration of a loop, the program checks a loop condition to see if the iteration will continue.
- Encounter continue statement: Nested inside the loop is the condition for the continue statement, which when met, the continue statement is executed.
- Skip Remaining Code: Upon encountering continue, the control skips the remaining code in the current iteration.
- Jump to Next Iteration: The control then jumps to the next iteration of the loop, bypassing the remaining code for the current iteration.
- Repeat Iterations: The loop continues its iterations as long as the main loop condition is satisfied.
- End of Execution: The loop completes its iterations, and the program continues with the rest of the code after the loop.
To further elucidate the flow and functioning of the continue statement, an illustrative C program example is presented below.
Code Example:
Output:
1 2 3 4 5 6 7 9 10
Explanation:
In the simple code example-
- We include the input/ output header file and initiate the main() function.
- Then, we declare an integer variable i without assigning it any initial value.
- Next, we create a for loop to iteratively print the values of integers. Inside the loop-
- The initial value of i is set to 1, and the loop condition checks if its value is less than 10, i.e., i<10.
- If this is met, the loop moves to the if-statement, which checks whether the value of i equals 8.
- If not, then the printf() function inside the loop displays the value of i to the console. After which, the increment operator increases the value by 1, before the next iteration.
- If i==8 is met, then the continue statement is executed, and the program will skip the remaining statements within the loop and proceed to the next iteration.
- As a result, the program prints numbers from 1 to 10, excluding the number 8.
- Finally, the return 0 statement serves as an indication that the program has been executed successfully, as it returns the value of 0 to the operating system.
Also read- Compilation In C | A Step-By-Step Explanation & More (+Examples)
Conclusion
Understanding the distinctions between the break and continue statements in C is crucial for effective loop control. While break terminates the entire loop or switch, continue selectively skips the code within a specific iteration. These statements provide programmers with powerful tools for controlling the flow of their programs and handling various scenarios within loops. Choosing between break and continue depends on the specific requirements and logic of the program being developed.
Also read- 100+ Top C Interview Questions With Answers (2023)
Frequently Asked Questions
Q. What is the difference between break, return, and continue statements?
The break statement is used to halt the whole process of the loop and switch statement and move to the code for further implementation. The continue statement will terminate only the current iteration of the loop and move to the next iteration for execution. The return statement halts the execution of the current function and transfers control back to the calling function.
Q. What is the main purpose of the break statement in C?
The main purpose of the break statement in C is to prematurely terminate the execution of the innermost loop or exit a switch statement. When encountered, the break statement immediately exits the loop or switch, transferring control to the statement following the loop or switch. It is commonly used when a specific condition is met, and there is no need to continue with the remaining iterations of the loop or cases in the switch.
Q. How does the continue statement differ from the break in terms of control flow?
The continue statement and break statement serve different purposes in terms of control flow.
- The break statement is used to exit the entire loop or innermost switch block when a specific condition is met. Control moves to the next statement after the loop or switch.
- In contrast, the continue statement is used to skip the remaining code within the current iteration of a loop and immediately jump to the next iteration. It does not exit the loop entirely.
Q. Can the break and continue statements be used interchangeably?
No, the break and continue statements cannot be used interchangeably. They have distinct functionalities. The break statement is used to terminate a loop or switch prematurely. The continue statement is used to skip the remaining code in the current iteration of a loop and move to the next iteration.
Q. In which loop constructs can the break and continue statements be applied?
Both the break and continue statements can be applied in various types of loop constructs, including:
- For loops
- While loops
- Do-while loops
However, the continue statement is not applicable to switch statements; it is designed specifically for loop constructs.
Q. How does the continue statement impact loop execution in nested loops?
The continue statement in C has a specific impact on loop execution within nested loops. Here's how the continue statement behaves in the context of nested loops:
-
Innermost Loop Impact: When a continue statement is encountered within the body of an innermost loop, it affects only that particular loop block. The continue statement skips the remaining code in the current block iteration of the innermost loop and moves on to the next iteration of that same loop.
-
Outer Loops Remain Unaffected: Importantly, the continue statement does not affect the execution of outermost loops in a nested structure. Outer loops continue their normal iterations, and the continue statement has no influence on them.
-
Control Limited to Innermost Loop: The impact of the continue statement is limited to the loop in which it is placed. If there are multiple nested loops, each with its continue statement, the control flow will be influenced only within the loop where the continue is encountered in the entire loop process.
By now, you must have a clear idea about the difference between break and continue statements in C. Here are a few other interesting topics you must read:
- Logical Operators In C Explained (Types With Examples)
- Bitwise Operators In C Programming Explained With Code Examples
- Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
- Constant In C | How To Define & Its Types Explained With Examples
- Ternary (Conditional) Operator In C Explained With Code Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment