- What Is Return Statement In C?
- How Does The Return Statement Work In C Programs?
- Uses Of Return Statements In C Programming
- Methods Not Returning A Value & Return Statements In C (Void Functions)
- Method Returning A Value & Return Statement In C
- If-Statements & Return Statement In C
- Different Forms Of Return Statements In C Based On Return Type
- Best Practices For Using Return Statements in C
- Conclusion
- Frequently Asked Questions
Return Statement In C | Uses, Types, Working & More! (+Examples)
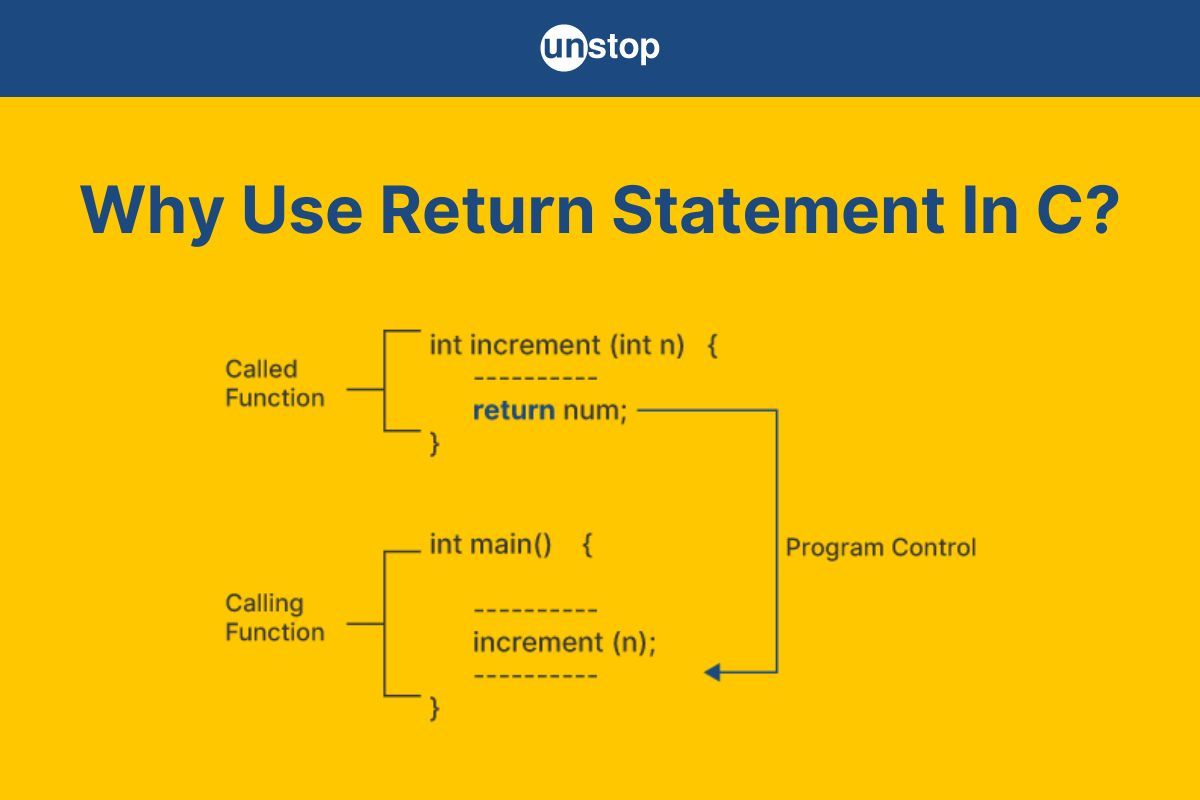
Renowned for its efficiency and control, the C language is a cornerstone of software development. Within this venerable language lies a fundamental feature essential to program flow control, i.e., the return statement. This seemingly modest command plays a pivotal role in shaping the flow of a C program, allowing developers to design elegant and organized code.
In this article, we explore the significance and intricacies of the return statement in C programming, including its syntax, role in program flow control, its broader implications for code organization, and more.
What Is Return Statement In C?
The return statement in C programming language is a fundamental construct used within functions to control the execution flow and send a value back to the calling program or current function. It is employed to signify the end of a function's execution and return a specific result or signal to the calling code.
Syntax Of Return Statement In C:
The syntax of the return statement is concise, yet its implications are profound. Here are the three primary forms of the return statement in C programming:
1. Return with an Expression:
return expression;
In this form, the expression represents the value that the C function returns to the calling code. This value could be a constant, a variable, or the result of a computation.
2. Return with a Value:
return;
When a function has no return value or is used to perform a task without providing any output, the return statement can be used without an expression. This form simply indicates the end of the function's execution.
3. No Return Statement:
void myFunction() {
// Function logic without a return statement
}
If a function does not require a return statement, it can be defined with a return type of void, indicating that the function does not return any value.
Now that you know what the return statement in C entails and the various forms it can take, let's look at an example showcasing its implementation. Below is an example where we are using a return statement without an expression.
Code Example:
#include <stdio.h>
// Function with no return value
void greeting() {
printf("Hello, Unstoppable!\n");
return; // Return without expression
}
int main() {
greeting();
return 0;
}
Output:
Hello, Unstoppable!
Explanation:
In the code above,
- We start by defining a function named greeting() with a return type of void, indicating that we don't return any value.
- The function uses a printf() statement to print the string message- Hello, Unstoppable!
- We invoke the greeting() function inside the main() function using the statement greeting();.
- This call runs the code within the greeting() function, thus printing the respective message to the console.
- We use the return; statement in the greeting() function without an expression. Because the return type of greet is void, it means the function doesn't return any value.
- After running the code within the greeting() function, the control comes back to the main() function.
- To indicate a successful program execution, we use the return 0 statement in the main() function. In C, a return value of 0 usually means the program ran without any errors.
Purpose Of Return Statements in C
The return statement in C serves several crucial purposes, contributing to the functionality and structure of programs.
- It acts as the bridge between a function and the calling code, transmitting values, indicating errors, and facilitating modular and structured programming.
- When a function executes a task, the return statement in C marks its conclusion, providing a specific value or status to the calling code.
- This enables the program to handle diverse scenarios, such as error conditions, calculated results, or specific outcomes, enhancing code clarity, modularity, and the ability to manage different aspects of program execution effectively.
How Does The Return Statement Work In C Programs?
The return statement in C programs is a fundamental mechanism that facilitates the transfer of control and data between functions and the calling code. Let us now understand how the return statement operates in C programs:
Step 1- Function Call: When a function is called from the main program or anywhere else in the program, the control is transferred to the beginning of the called function.
Step 2- Execution of Function Code: The function's code is executed sequentially, carrying out the tasks specified within the function body. This can include variable declarations, calculations, conditional statements, and other operations.
Step 3- Encounter of Return Statement: The return statement in C programs is typically the last statement in a function. When the return statement is encountered, the function execution is halted, and the control is handed back to the calling code.
Step 4- Expression Evaluation (Optional): If the return statement in C includes an expression (e.g., return exp;), the value of that expression is computed. This value serves as the result that the function will pass back to the calling code.
Step 5- Transfer of Control: The control is transferred back to the point in the calling code from where the function was invoked. If the calling code is another function, the execution resumes from the point immediately after the function call.
Step 6- Handling the Return Value: If the calling code expects a return value, it can capture the value returned by the function and use it in subsequent operations. This allows functions to communicate results, data, or error conditions to the rest of the program.
Step 7- Function Termination: After the return statement in C is executed, the function is considered terminated, and any local variables within the function are typically deallocated. The program then continues with the next set of instructions in the calling code.
Step 8- Void Functions: In the case of functions with a return type of void, the return statement may still be used to terminate the function, but it does not include an expression. This is simply a signal that the function has completed its task without returning a specific value.
Uses Of Return Statements In C Programming
The return statements in C play a crucial role in shaping the flow of a program, facilitating communication between functions, and providing a means to handle errors. Let's delve into specific uses of return statements in C from a programming point of view:
- Value Transmission: Functions often perform calculations or operations, and return statements are how these functions transmit the result or output back to the calling code. For example:
int calculateSum(int a, int b) {
return a + b; // Returns the sum of a and b
}
- Function Termination: The return statement in C signifies the end of a function's execution. The function terminates once a return statement is encountered, and control is handed back to the calling code. For example:
void displayMessage() {
printf("Function execution complete.\n");
return; // Function termination
}
- Error Handling: Return statements in C are pivotal for conveying error conditions. Functions can use specific return values to signal errors, enabling the calling code to detect and respond to exceptional situations. For example:
int divide(int a, int b) {
if (b == 0) {
printf("Error: Division by zero.\n");
return -1; // Returns -1 to indicate an error
}
return a / b; // Returns the result of division
}
- Conditional Execution: By incorporating return statements within conditional statements (if-else), functions can dynamically alter the value they return based on specific conditions. For example:
int absoluteValue(int num) {
if (num < 0) {
return -num; // Returns the negative of num if it's negative
}
return num; // Returns num if it's non-negative
}
- Exiting Main Function: In the main function of a C program, the return statement indicates the program's completion status. Conventionally, a return value of 0 signifies successful execution, and non-zero values represent different error codes. For example:
int main() {
// Program logic
return 0; // Indicates successful program execution
}
- Handling Dynamic Memory: Functions returning pointers play a key role in managing dynamic memory. Return statements in C programs allow functions to provide the calling code with pointers to dynamically allocated memory.
int* createArray(int size) {
int* arr = (int*)malloc(size * sizeof(int));
// ... (populate array)
return arr; // Returns a pointer to the dynamically allocated array
}
Methods Not Returning A Value & Return Statements In C (Void Functions)
In C programming, a void function is a function that does not return a value. It is used when the function's primary purpose is to perform a task or operation without producing a result that needs to be sent back to the calling code. Unlike functions with a return type, void functions utilize the void keyword in their declaration to indicate that they do not return any value. There are two forms of the void function depending upon the use of the return statement in C.
1. Not Using Return Statement In Void Function
In this approach, a void function is defined without an explicit return statement in C program. The absence of a return statement is acceptable for void functions since they are inherently designed not to return a value. The completion of the function is implicit, marked by reaching the end of the function's block. This approach is straightforward and suitable for functions that perform a task without the need for result transmission.
Syntax:
void functionName(parameters) {
// Function logic
// No explicit return statement needed
}
Here,
- Return Type: void indicates that the function does not return a value.
- Function Name: functionName is the identifier for the function definition.
- Parameters: (parameters) represent any input values the function may take.
- Function Logic: The block { // Function logic } contains the operations or tasks the function performs.
- No Explicit Return Statement: In this case, there is no explicit return statement, and the function concludes by reaching the end of its block.
Code Example:
#include <stdio.h>
// Void function without a return statement
void greet() {
printf("Hello, World!\n");
// No explicit return statement needed
}
int main() {
// Calling the void function
greet();
return 0;
}
Output:
Hello, World!
Explanation:
We begin the C code example above by including the <stdio.h> header file for input/ output operations. Then-
- As mentioned in the code comment, we define a void function named greet(), which uses a printf() statement to print the string message- Hello, World!
- There is no explicit return statement within the greet function, as void functions don't require a return statement.
- In the main() function, we call the greet() function, which prints- Hello, World! to the console on execution.
- Finally, the program terminates with a return 0 statement. Note that the return statement is a part of the main() function and not the void greet() function.
2. Using Return Statement In Void Function
Contrary to the conventional expectation for void functions, this approach involves including a return statement with no associated expression (also used as a jump statement). While void functions naturally lack a return value, adding a return statement in C programs serves a stylistic purpose by explicitly marking the end of the function.
Although not mandatory, this practice contributes to code readability and consistency, making it clear to developers that the function is intentionally concluding at that point.
Syntax:
void functionName(parameters) {
// Function logic
return; // Explicit return keyword statement without an expression
}
Here,
- Return Type: void indicates that the function does not return a value.
- Function Name: functionName is the identifier for the function.
- Parameters: (parameters) represent any input values the function may take.
- Function Logic: The block { // Function logic } contains the operations or tasks the function performs.
- Explicit Return Statement: return; is included without an expression, explicitly marking the end of the function.
Code Example:
#include <stdio.h>
// Void function with a return statement
void showMessage() {
printf("This is a message.\n");
return; // Explicit return statement without an expression
}
int main() {
// Calling the void function
showMessage();
return 0;
}
Output:
This is a message.
Explanation:
In the sample C program above-
- We first define a void function named showMessage(), which displays a string message "This is a message." to the console using a printf() statement.
- An explicit return statement without an expression (return;) is used at the end of the showMessage() function.
- In the main() function, we call the showMessage() function, whose execution prints the respective message to the console.
Method Returning A Value & Return Statement In C
In C programming, functions can be designed to return a value to the calling code by including a return statement. This is particularly useful when the function performs a calculation or a task and needs to communicate the result back to the part of the program that invoked it.
Approach To Using A Return Statement In C Functions:
- Define Function: Start by defining a function with a specific return type, indicating the type of value the function will return.
- Use Return Statement: Inside the function, use the return statement to specify the value to be returned.
- Invoke Function: In the calling code, invoke the function and capture the returned value.
Syntax:
return_type function_name(parameters) {
// Function logic
return value; // Value to be returned
}
Here,
- The term return_type indicates the data type of the value that the function will return to the calling code.
- The term function_name is the identifier for or the name given to the function.
- Parameters: represent any input values the function may take.
- The block {// Function logic } contains the operations or tasks the function performs.
- The return value; is the return statement used to send a specific value back to the calling code.
Code Example:
#include <stdio.h>
// Function to calculate the square of a number
int calculateSquare(int num) {
int square = num * num;
return square;
}
int main() {
// Calling the function and capturing the returned value
int result = calculateSquare(5);
// Displaying the result
printf("Square of 5: %d\n", result);
return 0;
}
Output:
Square of 5: 25
Explanation:
In the example C code-
- We define a function named calculateSquare() to calculate the square of a given integer. The function takes an integer parameter num.
- Inside the function, we use the multiplication arithmetic operator to calculate the square of the num variable, which is stored in the variable square.
- The calculated square value is returned using the return statement, i.e., return square.
- In the main() function, we declare a variable named result to capture the returned value from calling calculateSquare(5).
- The function calculateSquare is invoked with the argument 5, and the result is stored in the result variable.
- Next, we use the printf() function to display the result, showing the square of 5. The %d format specifier in the formatted string indicates an integer value, and the newline escape sequence shifts the cursor to the next line.
- The program concludes with a return 0 statement in the main function, indicating successful program execution.
If-Statements & Return Statement In C
The synergy between if-statements and return statements in C programming allows for efficient conditional execution and early termination within functions. The if-statement serves as a decision-making mechanism, enabling the function to check specific conditions. When combined with return statements, it facilitates the immediate return of a value or termination of the function based on the evaluated conditions. This further enhances code readability, simplifies control flow, and promotes the creation of concise and expressive functions. Let's explore how if-statements and return statements work together in C.
If-Statements in C: If-statements are used for conditional branching, allowing the program to execute different blocks of code based on whether a specified condition is true or false.
Syntax:
if (condition) {
// Code to be executed if the condition is true
} else {
// Code to be executed if the condition is false
}
Here:
- The condition is a boolean expression or a comparison expression that determines whether the code inside the if block or the else block should be executed.
- The code within the if-block, i.e., curly braces after the if keyword, is executed if the condition evaluates to true.
- The code within the else-block is executed if the condition is evaluated to be false.
Return Statements in C: The return statement is used to terminate the execution of a function and return a value to the calling function or program.
Syntax:
return expression;
Here:
- The return keyword indicates that the function will return a value.
- The expression determines the value being returned by the function.
Now, let's combine if-statements and return statements in a simple example to understand their working.
Code Example:
#include <stdio.h>
// Function to determine the absolute value of a number
int absoluteValue(int num) {
if (num < 0) {
return -num; // Return the negation if the number is negative
} else {
return num; // Return the number itself if it's non-negative
}
}
int main() {
int num1 = -7;
int num2 = 10;
// Using the absoluteValue function with if-statements and return statements
int result1 = absoluteValue(num1);
int result2 = absoluteValue(num2);
// Displaying the results
printf("The absolute value of %d is: %d\n", num1, result1);
printf("The absolute value of %d is: %d\n", num2, result2);
return 0;
}
Output:
The absolute value of -7 is: 7
The absolute value of 10 is: 10
Explanation:
In the sample C code-
- We define a function absoluteValue() that takes an integer parameter num and calculates its absolute value.
- Inside the function, we use an if-statement to check if the input number (num) is less than 0 using the less than (<) relational operator.
- If the condition is true (the number is negative), we return the negation of the number (-num).
- Else if the condition is false (the number is non-negative), we return the number itself (num).
- Then, we initiate the main() function, which is the entry point for the program's execution.
- Inside main(), we declare two integer variables num1 and num2 and assign them with values -7 and 10, respectively.
- We then call the absoluteValue() twice with num1 and num2 as arguments, and the results are stored in result1 and result2.
- After that, we use two printf() statements to display the values of the variables num1 and num2 and their absolute values, i.e., result1 and result 2.
- Finally, the main function returns 0, indicating successful program execution.
The program demonstrates the use of if-statements and the return statement in C within the absoluteValue function to calculate absolute values based on conditions.
Different Forms Of Return Statements In C Based On Return Type
In C, the return statement can take different forms based on the return type of the function. Here are the common forms:
1. Integer Return Type:
Functions with an integer return type (int) are designed to calculate or produce integer values. The return statement is used to send back an integer result from the function to the calling code.
Syntax:
return expression;
Code Example:
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(3, 4);
printf("Sum: %d\n", result);
return 0;
}
Output:
Sum: 7
Explanation:
The add() function returns the sum of two integers (a and b), and the main() function prints the result.
2. Void Return Type:
Functions with a void return type indicate that they do not return any value. The return statement is used to terminate the function without returning a specific value. Void functions are often used for tasks that involve printing, input/output, or other actions without a direct result.
Syntax:
return;
Code Example:
#include <stdio.h>
void greet() {
printf("Hello, World!\n");
return;
}
int main() {
greet();
return 0;
}
Output:
Hello, World!
Explanation:
The greet() function prints a greeting, and since it has a void return type, it doesn't return any value.
3. Floating-point Return Type:
Functions with a floating-point return type (float) are meant to calculate or produce decimal values. The return statement in such C functions sends a floating-point result back to the calling code.
Syntax:
return expression;
Code Example:
#include <stdio.h>
float divide(float a, float b) {
return a / b;
}
int main() {
float result = divide(10.0, 2.0);
printf("Division Result: %f\n", result);
return 0;
}
Output:
Division Result: 5.000000
Explanation:
The divide() function returns the result of dividing two floating-point numbers, and the main function prints the result.
4. Character Return Type:
Functions with a character return type (char) return a single character. Such a return statement in C function either returns a specific character, or the result of some character manipulation within the function.
Syntax:
return expression;
Example:
#include <stdio.h>
char firstChar(char* str) {
return str[0];
}
int main() {
char result = firstChar("Hello");
printf("First Character: %c\n", result);
return 0;
}
Output:
First Character: H
Explanation:
The firstChar() function returns the first character of a string literal, and the main() function prints the result.
5. Pointer Return Type:
Functions with a pointer return type (int*, char*, etc.) returns a memory address pointing to a specific type of data. Such a return statement in C programs returns the memory address, often obtained through dynamic memory allocation (malloc, calloc, etc.). This is useful for returning arrays, strings, or dynamically allocated data structures.
Syntax:
return pointer_expression;
Code Example:
#include <stdio.h>
#include <stdlib.h>
int* createArray(int size) {
int* arr = (int*)malloc(size * sizeof(int));
// Populate the array (not shown for brevity)
return arr;
}
int main() {
int* newArray = createArray(5);
printf("Array Created at Address: %p\n", (void*)newArray);
free(newArray); // Don't forget to free dynamically allocated memory
return 0;
}
Output:
Array Created at Address: 0x23fc2a0
Explanation:
The createArray() function dynamically allocates an integer array and returns its address. The main() function prints the address of the created array (address will vary). Don't forget to free the memory using free to avoid memory leaks.
Best Practices For Using Return Statements in C
Using the return statement in C effectively is crucial for writing clear, efficient, and maintainable programs. Here are some best practices for using return statements in C:
- Consistency in Return Types: Ensure consistency in return types across the program. If a function is declared to return a specific type, make sure that all return statements within that function adhere to the declared type.
- Explicitly Define Return Types: Clearly specify the return type of each function. Whether it's an integer, floating-point number, pointer, or void, explicitly declare the return type in the function prototype and definition.
- Use Descriptive Variable Names: When using a return statement in C programs with an expression, use descriptive variable names. This enhances code readability and makes it easier for others (or yourself) to understand the purpose of the returned value.
- Consistent Naming Conventions: Follow consistent naming conventions for functions and their return values. This promotes code uniformity and helps maintain a clean and readable codebase.
- Single Return Point: Adopt the practice of having a single point of return in a function. While multiple return statements in C functions are valid, consolidating them into a single exit point often improves code readability and maintainability.
- Avoid Unreachable Code: Be cautious about placing code after a return statement in C programs. Code that follows a return statement will never be executed, so avoid introducing unnecessary or unreachable code.
- Error Handling with Return Values: Use meaningful return values to indicate success or failure. Establish a convention for error handling and communicate it clearly in the function's documentation. Commonly, 0 indicates success, while non-zero values signify errors.
- Avoid Returning Pointers to Local Variables: Avoid returning pointers to local variables within a function. Once the function exits, local variables are deallocated, and returning a pointer to them can lead to undefined behavior.
- Document Return Values: Provide clear documentation for the expected return values of your functions. Documenting the purpose and potential values of return statements in C helps other developers understand how to use your functions correctly.
- Handle Void Functions Appropriately: For functions with a return type of void, use the return statement without an expression (i.e., return;) to signify the end of the function. Avoid using this type of return statement in C functions with a non-void return type.
- Considerations for Main Function: It is ideal to use the return 0 statement in C program's main() function to indicate successful program execution. Although some systems interpret any non-zero return value as an error, explicitly using return 0; is a good practice.
- Avoid Overusing Return Statements for Flow Control: While return statements in C are crucial for function exit and result communication, avoid overusing them for general flow control. Instead, use other control flow statements like if, else, and looping statements, which are more appropriate.
Conclusion
The return statement in C is not merely a syntactic element; it is a powerful tool that influences the structure and behavior of programs. By allowing functions to communicate results and potential errors to the calling code, the return statement enhances the clarity, modularity, and maintainability of C programs.
Understanding the nuances of the return statement empowers C developers to design robust and efficient code, creating a seamless interplay between functions and the calling program. As we continue to navigate the vast landscape of C programming, let the return statement be our guiding beacon, steering us towards code that is both elegant and functional.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is the exit and return statement in C?
In C, both exit and return are statements used to control program flow, but they serve different purposes.
- The return statement in C is used within functions to specify a value to be sent back to the calling code. It marks the end of a function's execution, and the specified value is returned to the calling function or the operating system if it's the main function.
- The exit function is part of the <stdlib.h> library, and it is used to terminate the program. It takes an integer argument that serves as the exit status of the program. A status of 0 typically indicates successful execution, while non-zero values may represent errors or other conditions.
Q. What is the purpose of the return statement in C?
In C programming, the return statement serves a pivotal role in controlling program flow and facilitating communication between functions and the calling code.
- Its primary purpose is to signify the conclusion of a function's execution and to return a specific value or signal to the part of the program that invoked the function.
- By using the return statement in C, functions can provide results, handle errors, or convey information back to the calling code.
- This mechanism allows for the creation of modular and reusable code, as functions encapsulate specific tasks and can be easily integrated into various parts of a program.
- The return statement in C programs also plays a crucial role in error handling, where different return values can indicate success or failure conditions, allowing the calling code to respond appropriately.
Q. How to return from the main function in C?
The main function in C is typically defined to return an integer value. A return statement in C's main() is used to specify the exit status of the program. By convention, a return value of 0 indicates successful execution, while non-zero values may represent errors or specific conditions.
int main() {
// Program logic
return 0; // Indicates successful execution
}
It's important to note that if the main() function does not explicitly contain a return statement, the compiler assumes a default return 0; at the end of the main function.
Q. Can we have 2 return statements in C?
Yes, we can have multiple return statements in C functions. The execution of a function will terminate when any return statement is encountered. Each return statement can return a different value, and the choice of which one to execute depends on the conditions specified in the function.
Code Example:
#include <stdio.h>
int absoluteValue(int num) {
if (num < 0) {
return -num; // Return the negative of the number if it's negative
} else {
return num; // Return the number if it's non-negative
}
}
int main() {
int result1 = absoluteValue(-5);
int result2 = absoluteValue(10);
printf("Absolute value of -5: %d\n", result1); // Output: 5
printf("Absolute value of 10: %d\n", result2); // Output: 10
return 0;
}
Output:
Absolute value of -5: 5
Absolute value of 10: 10
Explanation:
In this example, the absoluteValue() function has two return statements, i.e., one for the case when the input is negative (return -num;) and another for the case when the input is non-negative (return num;). Depending on the input, the function will execute the corresponding return statement.
Q. Is the return statement mandatory in the main function of a C program?
No, the return statement is not mandatory in the main function of a C program. If the main function reaches the end without encountering a return statement, the compiler assumes a default return 0;, indicating successful program execution. However, it's a good practice to explicitly use the return statement in C program's main() function, i.e., return 0; to enhance code clarity.
Here are a few articles you might be interested in reading:
- Logical Operators In C Explained (Types With Examples)
- Compilation In C | A Step-By-Step Explanation & More (+Examples)
- Type Casting In C | Types, Cast Functions, & More (+Code Examples)
- Comma Operator In C | Code Examples For Both Separator & Operator
- Void Pointer In C | Referencing, Dereferencing & More (+Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment