C Program To Find Factorial Of A Number (Multiple Ways + Examples)
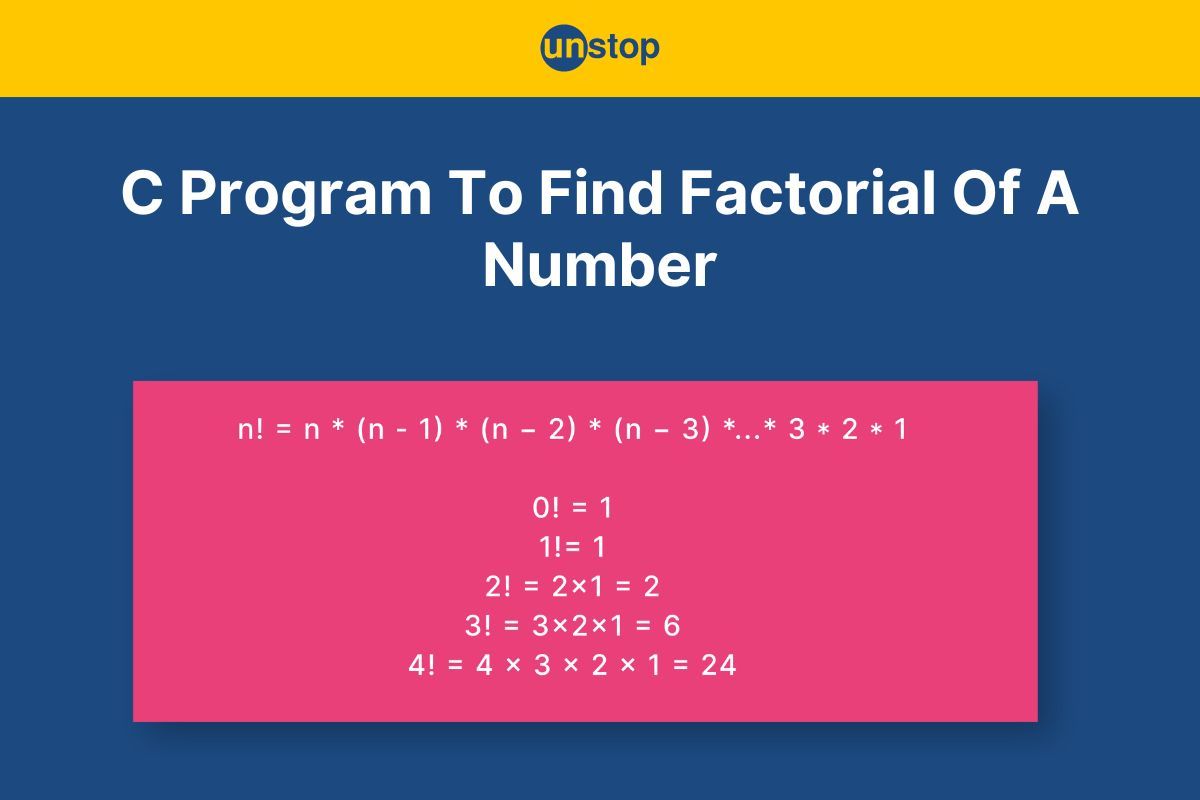
In the world of programming, understanding how to calculate factorials is akin to mastering the ABCs. It's one of the fundamental programming concepts that teaches the basics of loops and arithmetic operations and serves as a gateway to more complex algorithms and mathematical problems. In this article, we will explore how to write a C program to find factorial of a number using different techniques. Let's start with the basics and gradually build our understanding how to solve this in the C programming language.
What Is Factorial?
The factorial of a non-negative integer n is a mathematical operation denoted by the exclamation mark (!). It is defined by the product of all consecutive positive integers smaller than or equal to n. For example, the factorial of 3 is 6. Also, the factorial of 0 is considered to be 1. Another way to calculate the factorial of n is defined as the factorial of n equals the product of n with the next smaller factorial, i.e., n-1. It is mathematically represented as:
n!=n×(n−1)×(n−2)×…×3×2×1
We are all familiar with the mathematical calculation of factorials. But, in this article, we will discuss ways to write code/ C programs to find factorials of a number using different methods.
Why Do We Need Factorial?
Some of the most common applications of factorials of a number are-
- Factorials are important when one wants to count permutations, i.e., the number of ways to arrange n distinct objects is n!.
- They are widely used in combinatorics formulae involving binomial coefficients.
- In Algebra, they are used in binomial theorems, which involve computing powers of sum.
- Frequently appear in denominators of power series like e^x and many more.
- It is used as a basic example to understand loops and recursion.
How To Find The Factorial Of Any Number?
As mentioned before, the factorial of a number n is the product of all positive integers smaller than or equal to n. Here is a step-by-step description of how we generally calculate the factorial of a number:
- To find the factorial of n, we have to multiply all the positive numbers by smaller than equal to n. So, we start from n, then n-1,n-2,n-3 and so on until 1.
- So, the factorial of n is-
n! = n*(n-1)*(n-2)*....*3*2*1 = 1*2*3*....*(n-2)*(n-1)*n
n!=n*(n-1)!=n*(n-1)*(n-2)!=n*(n-1)*(n-2)*(n-3)*(n-4)*.... *1 - Note that 0!=1.
- For n=0, the definition of factorial involves the product of no numbers at all. So it is an example of the broader convention that there is only one permutation of zero items. So 0! Is considered to be 1.
Let's look at a proper mathematical example to better understand the concept. Say we want to calculate the factorial of number 5.
We know that, n! = n*(n-1)!, where, n!=n*(n-1)*(n-2)*(n-3)*..... *2*1, 1!=1, and 0!=1. So, to compute 5! We need to multiply all the positive numbers smaller than equal to 5, i.e., (5,4,3,2,1).
5!= 5*4*3*2*1 = 5*4! = 5*4*3! = 5*4*3*2! = 120
So, the result is 5!=120
Prerequisites To Write C Program To Find Factorial Of A Number
Before we move on to discussing how to write a C program to find the factorial of a number, let's rehash a few basic programming concepts that are essential for writing and running such C program.
- Programming Basics: You should understand basic programming concepts like variables, data types, functions, loops, conditional statements, etc.
- C Language Syntax: Familiarize yourself with C syntax, including its rules for declaring variables, writing conditions for control statements (loops, etc.), various data structures, defining functions and various operators to carry out manipulations on data.
- Functions: It's important to understand how to break down tasks into functions and how to call them.
- Looping statements: Factorial calculation typically involves loops that allow us to run the same code again and again. You will need to know about for and while loops.
- Arithmetic Operations: While you must be familiar with most operator types, factorial calculations involve multiplication arithmetic operators.
- Basic Input/Output Operations: You should be familiar with reading input from the user (using scanf()) and printing output (using printf()).
Algorithm Of C Program To Find The Factorial Of A Number
In this section, we will discuss the general algorithm or the working mechanism behind a C program to find the factorial of a number.
Step 1- Start: Begin the algorithm/ program. It can either be the main() function or a function you define to find the factorial down the line.
Step 2- Input: Prompt the user to input the number whose factorial you will calculate. Read and store the input number.
Step 3- Check for Non-Negativity: Verify if the input number is non-negative.
- If the number is negative, the program generates an error message indicating that the factorial is not defined for negative numbers. And the program will terminate.
- If the number is non-negative, then move to the next step.
Step 4- Initialize Variables: Declare and initialize a variable to hold the factorial value, for example fact initialized to 1. Declare a variable (control variable) to iterate through the numbers from 1 to the input number and initialize it to 1.
Step 5- Factorial Calculation Loop: Start a loop that iterates from 1 to the input number. Within each iteration:
- Multiply the current value of fact by the current iteration number.
- Update the value of fact with the result of the multiplication.
- Move to the next iteration.
Step 6- Output Result & Terminate: After the loop terminates, print the calculated factorial value and use a return statement to terminate the program.
Pseudocode:
Start
Input num
If num < 0
Print "Factorial is not defined for negative numbers."
Else
Initialize fact to 1
Initialize i to 1
While i <= num
fact = fact * i
Increment i
End While
Print "Factorial of num is fact."
End
Explanation:
- This pseudocode outlines a simple algorithm to compute the factorial of a non-negative integer num.
- It begins by prompting the user to input a number.
- If the input is negative, it prints an error message; otherwise, it initializes a variable fact to 1 and a counter variable i to 1.
- It then enters a loop, multiplying fact by i in each iteration until i exceeds num, updating fact accordingly.
- Finally, it prints the calculated factorial value.
- This algorithm provides a clear and concise approach to finding factorials in C
C Program To Find Factorial Of A Number Using Recursion
Finding the factorial of a non-negative integer using recursion (recursive approach) involves defining a function that calls itself with a decreasing value until a base condition is met. The base condition is typically when the input number reaches 0 or 1, at which point the factorial value is 1. The function then returns the product of the current number and the factorial of the number minus one. Below is a sample C program to find the factorial of a number using recursion.
Code Example:
Output:
Enter the number: 9
362880
Explanation:
In the simple C program, we begin by including the <stdio.h> header file for input-output operations.
- Then, we define a function factorial(int n), which recursively calculates the factorial of a given integer n. Inside the function-
- We have a base case check/ condition to see if the number is 0 or 1, using the relational equality and logical OR operators.
- If n is either 0 or 1, the factorial is 1. This condition prevents infinite recursion and serves as the base case for the recursive function.
- For other values of n, the function recursively calls itself with n - 1 until it reaches the base case. It multiplies n with the result of factorial(n - 1) to calculate the factorial.
- In the main() function, we prompt the user to enter a number using printf().
- The program then reads the user input using scanf() and stores it in the variable n using the reference/ address-of operator. The %d format specifier is the placeholder for the integer value.
- After that, we call the factorial() function, which calculates the factorial of the input number n and stores the result in the variable res.
- Finally, we print the calculated factorial value res using the printf() function.
- At last, the return 0 statement signifies the successful completion of the main() function, leading to the termination of our program.
Time Complexity: O(n)
Space Complexity: O(n) recursion call stack
How To Write C Program For Factorial Of A Number Without Recursion?
We can employ an iterative approach to calculate the factorial of a number without using recursion (recursive method). This involves using a loop structure, such as a for loop or a while loop. With the for loop approach, we initialize a loop control variable (typically denoted i) to 1, and then iterate until it reaches the given number n. On the other hand, the while loop approach starts with an initialization outside the loop, typically initializing a loop control variable (i) to 1 before entering the loop.
These iterative solutions efficiently compute the factorial of a number without relying on recursive function calls, making them suitable for scenarios where recursion might be less desirable due to potential stack overflow issues or performance concerns. We have discussed both of these iterative methods in detail in the sections below.
C Program To Find Factorial Of A Number Using For Loop
The program to find factorial using a for loop in C is a concise implementation that leverages the looping mechanism to calculate the factorial of a given non-negative integer. It begins by prompting the user to input a number. If the input is negative, it displays an error message. Otherwise, it initializes a variable fact to 1 and enters a for loop, iterating from 1 to the input number. Within each iteration, it multiplies fact by the current loop index, effectively calculating the factorial. After completing the loop, it outputs the computed factorial.
Given below is a sample factorial program in C language that employs the for loop.
Code Example:
Output:
Enter a non-negative integer to find its factorial: 8
Factorial of 8 is 40320.
Explanation:
In the example C program-
- In the main() function, we declare an integer data type variable num to store the user input and an unsigned long long integer fact and assign the value 1 to it. This initialization is done to handle large factorial values.
- We then use printf() to prompt the user to input a non-negative integer, which we will find the factorial of.
- With the help of the scanf() function, we read the user input and store it in the variable num.
- Then, we use an if-else statement to check if the input number is non-negative. If num is negative, an error message is printed to the console stating that factorial calculation is not defined for negative numbers.
- If the condition is false, i.e., num is non-negative the else block is executed.
- The block contains a for loop that iterates from 1 to num, allowing us to multiply each value by fact.
- Within the loop, we update the value of fact by multiplying its current value with the loop index i. After every iteration, the value of control variable i is incremented by 1.
- After the loop completes its iterations, we use the printf() function to output the computed factorial value. Here, we display both the input number num and its corresponding factorial fact.
- The %d and %llu format specifiers stand for integer and long long unsigned. The newline escape sequence shifts the cursor to the next line.
- Finally, the program terminates successfully with a return 0 statement.
Time Complexity: O(n) as we run the loop for n iterations
Space Complexity: O(1) as we don't require any extra space
C Program To Find Factorial Using While Loop in C
A program to find the factorial of a number using a while loop typically involves initializing variables, utilizing a while loop to iterate through the numbers from 1 to the given number, and multiplying them to calculate the factorial. The loop continues until the counter reaches the given number. Here's a simple implementation:
Code Example:
Output:
Enter a positive integer: 7
Factorial of 7 = 5040
Explanation:
In the example C code-
- In the main() function, we declare three integer variables, i.e., number to store user input, factorial to handle multiplication process, and loop control variable i. We assign the value 1 to variables factorial and i.
- We then prompt the user to input a positive integer using printf().
- Using scanf(), we read the user input and store it in the variable number with the reference/ address-of operator (&).
- Following that, we create a while loop to calculate the factorial. The loop begins with i=1 and continues till i is less than or equal to the number variable.
- Inside the loop, we update the factorial by multiplying its current value with i using the compound multiplication assignment operator.
- Then we increment the value of i to move to the next number in the sequence.
- Once the loop finishes, we use printf() to output the computed factorial value along with the input number.
- Lastly, the program terminates with return 0 statement.
Time Complexity: O(n) as we run the loop for n iterations
Space Complexity: O(1) as we don't require any extra space
C Program To Find Factorial Of A Number Using A Ternary Operator
The ternary operator, also known as the conditional operator, is a shorthand way of writing an if-else statement. It takes three operands, i.e., a condition followed by a question mark, a value to be returned if the condition is true, and a value to be returned if the condition is false, separated by a colon (:).
Syntax:
Condition ? Statement 1: Statement 2;
Here,
- The condition refers to the condition for decision-making.
- The question mark/ symbol (?) is the ternary operator, which leads to the execution of a statement based on whether the condition is true or false.
- Statement 1 is executed if the condition is true.
- Statement 2 is executed if the condition is false.
Let's look at an example C program for factorial using a ternary operator in C.
Code Example:
Output:
Enter the number: 5
The factorial of 5 is 120
Explanation:
In the C code example-
- We start by defining a function factorial(int n), which recursively computes the factorial of a given integer n.
- Inside the factorial function, we utilize a ternary conditional operator to succinctly express the factorial calculation logic.
- If n is either 0 or 1, the factorial is 1.
- Otherwise, it multiplies n with the factorial of n - 1 recursively.
- Inside the main() function, we prompt the user to input a number using printf().
- We then use scanf() to read the input and store it in the variable n using reference.
- Next, we call the factorial() function inside a printf() statement.
- The factorial() function calculates the factorial of the input number n, and the printf() statement directly prints the result with the input number.
- Finally, the main() function returns a 0, indicating successful execution without any errors.
Time Complexity: O(n)
Space Complexity: O(n)
C Program To Find Factorial Of A Number Using The tgamma() Function
The tgamma() function is a mathematical function provided in the C standard library, specifically from the <math.h> header file. It calculates the gamma function, denoted by Γ(x), for a given real number x. For non-negative integer values of x, Γ(x) is equivalent to the factorial of (x-1), i.e., Γ(n) = (n-1)! for n ≥ 1. This property makes the gamma function useful for calculating factorials of non-integer values, and it's commonly used in statistical and mathematical computations.
Syntax:
In the <math.h> header, the prototype of the tgamma() function is:
double tgamma(double x);
Here,
- x is the real number for which you want to calculate the gamma function.
- The tgamma() function returns the value of Γ(x).
Code Example:
Output:
The factorial of 5 is 120
Explanation:
In the sample C code-
- We initialize an integer variable num with the value 5 inside the main() function. This is the number whose factorial we want to compute.
- We then use the tgamma() function from the math.h library to calculate the gamma function of num + 1.
- Since the gamma function of an integer n is equal to (n - 1)! adding 1 to num allows us to obtain num factorial directly.
- The result of the tgamma() function is stored in a double variable res.
- Finally, we print the calculated factorial result along with the input number using the printf() function.
- The %.0f specifier in the formatted string ensures that the result is displayed as a whole number since factorials are integers.
- Lastly, the return 0 statement signifies the successful completion of the main() function, leading to the termination of our program.
Time Complexity: O(n)
Space Complexity: O(1)
C Program To Find Factorial Of A Number With User-Defined Function
We can calculate the factorial of a number by creating our own function, which we can use every time we need to calculate the factorial of any number. By doing this we can simply call our factorial function for the required result instead of writing the code for finding the factorial numerous times. Below is a sample C program for factorial of a number where we define our own function.
Code Example:
Output:
Enter a number to get factorial: 6
The factorial of 6 is: 720
Explanation:
In the C program example-
- We first forward declare a function fact(int) to calculate the factorial of a number, i.e., given integer n.
- Inside the main() function, we prompt the user to enter a number for which they want to find the factorial using printf().
- The program then reads the user input and stores it in the variable n via scanf().
- After that, we call the fact() function to calculate the factorial of the input number n and store the result in the variable res.
- We then print the calculated factorial result along with the input number using printf() statement.
- The main() function terminates with return 0.
- Lastly, we define the fact() function iteratively calculates the factorial using a for loop.
- We declare loop control variable x and initialize the res variable with the value of 1.
- The loop begins with x=0 and continues till it is less than equal to n. In every iteration, the loop multiplies res with consecutive integers from 1 to n to compute the factorial.
- The function returns the value of res (which is the factorial) after the loop is done with all iterations.
Time Complexity: O(n)
Space Complexity: O(1)
C Program To Find Factorial Of A Number Using Pointers in C
We can also compute the factorial of a number using pointers. A pointer in C is defined as a derived data type that can store the address of other C variables or a memory location. We can access and manipulate the data stored in that memory location using pointers directly.
Code Example:
Output:
Enter a number to get factorial: 7
The factorial of 7 is: 5040
Explanation:
In the sample C code-
- As mentioned in code comments, we forward declare a function fact(int, int *) responsible for computing the factorial of a given integer n and storing the result in an integer pointer res.
- Inside the main() function, we prompt the user to input a number for which they want to find the factorial using printf().
- The program reads the user input and stores it in the variable n via scanf() function.
- Then, we call the fact() function, passing the input number n and the address of the variable res as arguments. This allows the function to directly modify the value of res to store the factorial result.
- After calling the function, we print the calculated factorial result along with the input number using the printf() function.
- The main() function then terminates with a return 0 statement indicating successful execution.
- Finally, we define the fact() function, which iteratively calculates the factorial using a for loop.
- Inside the function, we declare an integer variable x and initialize a pointer variable res to 1.
- Then, the for loop multiplies the value of the res variable with consecutive integers from 1 to n to compute the factorial. The result is stored at the memory location pointed to by the pointer res.
Time Complexity: O(n)
Space Complexity: O(1)
C Program To Find Factorial Of All Numbers In A Given Range
We can use nested loops to create a C program to find the factorial of all numbers in a given range. The outer loop will iterate through each number in the specified range, and an inner loop will calculate its factorial for each number.
Code Example:
Output:
Enter the first number of the range:
4
Enter the last number of the range:
8
Factorials of all numbers in the given range are:
24 120 720 5040 40320
Explanation:
In this example code in C language-
- We declare variables res as a long integer initialized to 1, and x, n, s, and e as integers inside the main(). Here, the variables s and e represent the start and end of the range, respectively.
- Then, we use printf() to prompt the user to enter the values for variables s and e, one by one.
- When the user input values, we read it using scanf() and store them in the respective variables.
- We then print a message indicating that we will display the factorials of all numbers within the given range using printf().
- After that we use a set of nested for loop to iterate through each number in the range from s to e.
- In the outer loop, we initialize res to 1 for each new number. And the inner loop multiplies res with consecutive integers from 1 to n and reassigns the value back to res.
- Once the loop is done calculating the factorial, we print the result using printf().
- Finally, the return 0 statement signifies the successful completion of the main() function.
Time Complexity: O((e-s)*n)
Space Complexity: O(1)
Conclusion
Understanding how to calculate factorials in C is an essential skill for any budding programmer. In this article, we have learned varioustechniques we can use to write a C program to find factorial of a number. These include the recursive approach (recursion), iterative solutions (for loop and while loop), ternary operator, tgamma() function, user-defined function, and pointers.
Factorials have many applications in real life, and understanding how to write such C programs will solidify your grasp of basic programming topics and lay the groundwork for tackling more advanced mathematical problems.
Frequently Asked Questions
Q. What is the significance of factorial programs in programming?
We use factorials to solve a variety of mathematical and computational problems. They help in calculating permutations, combinations, power series, probabilities and binomial theorems. Additionally, factorials have applications in fields like mathematics, statistics, and physics. Also it acts as a basic example in C programming to understand loops and recursion easily. We can write a C program to find factorial of a number using various methods such as loops, recursion, ternary operators, pointers, functions, etc.
Q. What is the value of 5!?
The factorial of a number is defined by the product of all positive integers smaller than equal to n. The mathematical formula for this is-
n!=n*(n-1)*(n-2)*(n-3)*_ _ _ _ _ *2*1
Here, n! = n*(n-1)!
1!=1 and 0!=1
Now we have n = 5. So, to compute 5! We need to multiply all the positive numbers smaller than equal to 5 i,e(5,4,3,2,1)
5!= 5*4*3*2*1 = 5*4! = 5*4*3! = 5*4*3*2! = 120
Q. Can we calculate factorials for negative numbers?
No, we cannot calculate factorials for negative integers. Negative numbers and non-integer values do not have factorial representations. Factorials are only defined for negative integers like 0, 1, 2, and so on.
n! = n*(n-1)! = n*(n-1)*(n-2)! And so on
0! = 1, 1! = 1, 2! = 2, 3! = 6 and so on…
Q. Is there a factorial function in the math library of C?
The tgamma() function is a library function defined in math.h library in C programming language. It computes the gamma function of the passed argument.
For a number n, we should call tgamma(n + 1) for finding the factorial of n. We are finding n! by passing n + 1 to the function because the gamma function is evaluated over a value lesser than the original value by 1 which means that calling tgamma(n) would give us the factorial of n – 1.
Q. What happens if a large number is entered for factorial calculation?
Factorials grow exponentially as the input increases. If a large number is an input to find a factorial, it may result in an overflow as the calculated factorial may exceed the maximum representable value by an integer in C, which will lead to the result being inaccurate.
For example, if the input is greater than 12, the result of the required factorial crosses the maximum value of int in C, i.e. 2,147,483,647 as 13! = 6,227,020,800, and further factorial results will be much greater than this, due to which we will get to see undefined behaviour with respect to our results. As a result we would have to use data types such as long long in that case so that the required result does not fall out of range.
Q. Can the factorial program be optimized for efficiency?
Yes, we can optimize the program to find the factorial using techniques like memoization (dynamic programming), where previously calculated factorials are stored for reuse. This optimizes the program, which uses normal loops or recursion. Additionally, algorithms like Stirling’s approximation can be used for faster approximations for large factorials.
Q. Write a C program to find the factorial of a number without using recursion.
Here, we have to write a C program to find the factorial of the number without using recursion. We can do the same by using loops (for or while) easily.
Code Example:
Output:
Enter a non-negative integer: 9
Factorial of 9 = 362880
Explanation:
This C program calculates the factorial of a non-negative integer entered by the user without using recursion. It prompts the user to input a number and then calculates its factorial using a for loop, multiplying the current factorial value by each successive integer until reaching the input number. It handles negative inputs by displaying an error message, as factorials are not defined for negative numbers. To accommodate larger factorial values, it uses an unsigned long long variable type. Finally, it prints the calculated factorial value.
We are sure that now you can easily write a C program to find factorial of a number by choosing the technique that best suits your needs. Here are a few other articles to expand your understanding of the language:
- Type Casting In C | Types, Cast Functions, & More (+Code Examples)
- Reverse A String In C | 10 Different Ways With Detailed Examples!
- Compilation In C | Detail Explanation Using Diagrams & Examples
- Bitwise Operators In C Programming Explained With Code Examples
- Control Statements In C | The Beginner's Guide (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment