Table of content:
- What Is A NULL Pointer In C?
- Declaration & Initialization Of Null Pointer In C
- Why Do We Need A NULL Pointer In C?
- How Do Null Pointers Work in C?
- Dereferencing A NULL Pointer In C
- How To Check If The Pointer Is NULL?
- What Are The Uses Of NULL Pointer In C?
- Examples Of Null Pointer In C
- Application Of NULL Pointer In C
- Best Practices For NULL Pointer Usage
- What Is The Difference Between The Void Pointer & Null Pointer In C?
- Conclusion
- Frequently Asked Questions
Null Pointer In C | Creation, Dereferencing & More (+Examples)
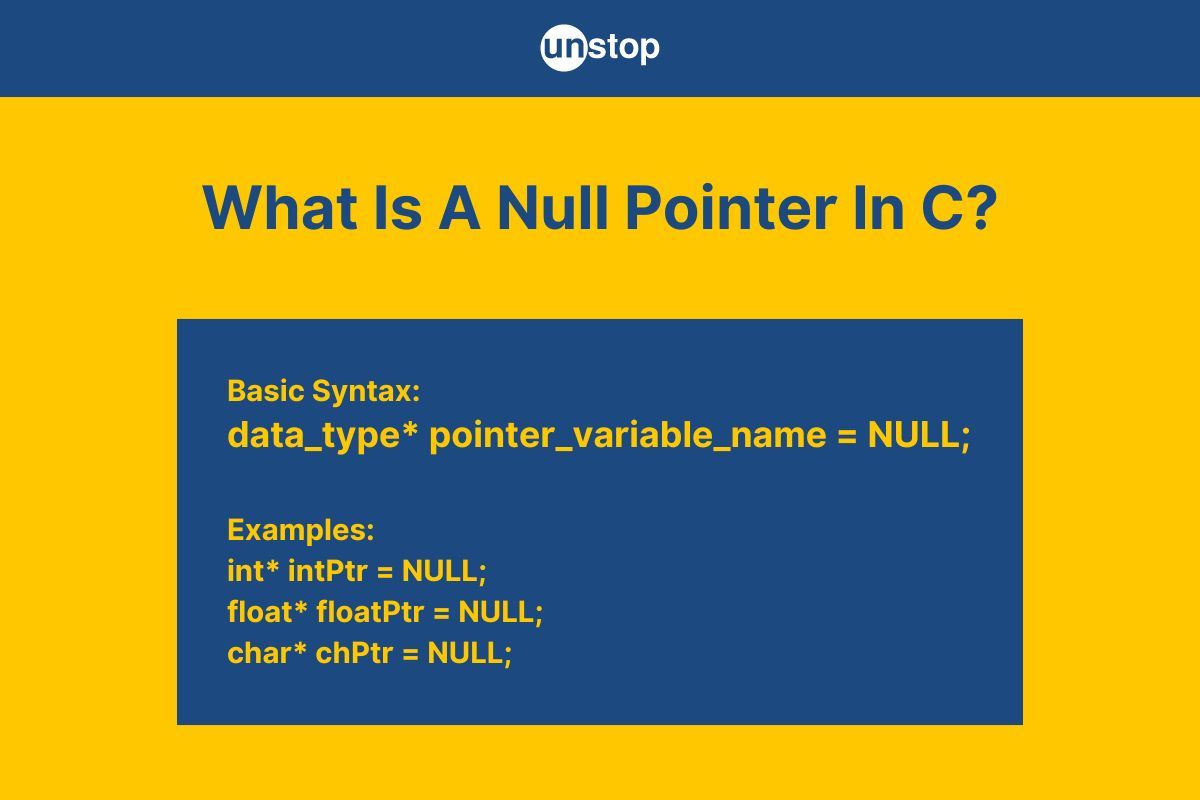
Pointers are powerful programming tools that store the memory address of variables, etc., elements of a program. They allow us to access and manipulate the data stored at these addresses, facilitating low-level memory access, dynamic memory allocation, and much more. There are four main types of pointers in the C programming language: null pointer, void pointer, wild pointer, and dangling pointer.
In this article, we will discuss the null pointer in C programming, which is misunderstood and hence dreaded by many. We'll delve into what null pointers are, why they occur, their consequences, and best practices to use or avoid them, all with the help of proper code examples.
What Is A NULL Pointer In C?
A pointer is a variable that stores the memory address of another variable. A NULL pointer is a pointer that doesn't point to any usable memory address. It is commonly represented by the constant "0" or by the macro "NULL," which is specified as 0 in the standard library. In other words, a pointer is said to be referring nowhere useful in memory when it is given the value NULL.
Causes of Null Pointer In C:
- Uninitialized Pointers: If a pointer is declared but not initialized to point to a valid memory address, it will hold a garbage value, which could be interpreted as a null pointer in C.
- Explicit Assignment: Sometimes, developers explicitly assign NULL to a pointer to signify that it doesn't currently point to anything meaningful.
- Returning NULL from Functions: Functions may return NULL to indicate failure or the absence of a valid value.
- Memory Allocation Failures: Functions like malloc(), calloc(), and realloc() return NULL if they fail to allocate the requested memory.
Rules and Regulations For Null Pointer In C:
- Initializing Pointers: Always initialize pointers to NULL when declaring them if you don't have a valid memory address to assign immediately. This ensures that the pointer starts in a safe state.
- Check for NULL Before Dereferencing: Before accessing the memory location a pointer points to (dereferencing), ensure that the pointer is not NULL. Dereferencing a NULL pointer in C leads to undefined behaviour, which can cause crashes or unexpected results.
- Assigning NULL to Pointers: You can assign NULL to a pointer to indicate that it no longer points to a valid memory location or to initialize it before using it.
- Passing NULL as Function Arguments: When passing pointers to functions, consider that a NULL pointer may be a valid input. Ensure that the function handles NULL pointers appropriately to avoid crashes.
- Returning NULL from Functions: When designing functions that return pointers, make sure that they correctly handle the possibility of returning NULL. Document the function's behaviour so users know what to expect when they receive a NULL pointer in C programs.
- Avoiding Dangling Pointers: Ensure that pointers are set to NULL or a valid address. Avoid using pointers that have been freed (dangling pointers), as accessing them can lead to undefined behaviour.
- Using calloc() for Null Initialization: When dynamically allocating memory for an array or structure, consider using calloc() instead of malloc(), as it automatically initializes the memory to zero (NULL).
Declaration & Initialization Of Null Pointer In C
The process of declaring and initializing a null pointer in C is pretty straightforward because it is also declared and initialized like any other pointer variable in C. However, the key thing to note here is that it is explicitly initialized to the special value NULL, which represents a null pointer in C.
Syntax For Null Pointer In C:
type *pointer_variable_name = NULL;
Here,
- type: The data type that the pointer will point to.
- *pointer_variable_name: The name of the pointer variable.
- NULL: Special macro representing a null pointer.
Look at the simple C program example below to better understand the concept of null pointers in C.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKCmludCogaW50UHRyID0gTlVMTDsgLy8gRGVjbGFyYXRpb24gYW5kIEluaXRpYWxpemF0aW9uIG9mIGEgbnVsbCBwb2ludGVyCmZsb2F0KiBmbG9hdFB0ciA9IE5VTEw7CgppZiAoaW50UHRyID09IE5VTEwpIHsKcHJpbnRmKCJpbnRQdHIgaXMgYSBudWxsIHBvaW50ZXIuXG4iKTsKfQoKaWYgKGZsb2F0UHRyID09IE5VTEwpIHsKcHJpbnRmKCJmbG9hdFB0ciBpcyBhIG51bGwgcG9pbnRlci5cbiIpOwp9CgpyZXR1cm4gMDsKfQ==
Output:
intPtr is a null pointer.
floatPtr is a null pointer.
Code Explanation:
We begin the C code example by including the essential header file <stdio.h> for input-output operations.
- Then, inside the main() function, which is the entry point for program execution, we declare two pointers.
- The first pointer intPtr is an integer type pointer, and floatPtr is a floating-point type pointer. We initialize them both to NULL, which indicates that neither pointer currently points to any valid memory location.
- We then use two separate if-statements to check if each pointer is equal to NULL, using the relational equality operator.
- Inside each if block, if the condition is true (i.e., the pointer is NULL), we print a string message indicating that the respective pointer is a null pointer.
- Finally, the main() function terminates with a return 0 statement, indicating successful execution to the operating system.
What Is The Difference Between An Uninitialized Pointer & Null Pointer In C?
An uninitialized pointer and a null pointer are both pointers in C programming, but they differ in their initial values and behaviour. The table given below highlights the difference between the uninitialized and null pointer in C.
Criteria | Uninitialized Pointer | Null Pointer |
---|---|---|
Initialization | Does not point to a specific memory address until explicitly assigned. | There is a need to explicitly assign the "NULL" or "0" value to indicate it does not point to any memory location. |
Value | Contains a garbage value, typically undefined. | Contains the specific value "NULL" or "0". |
Dereferencing Behavior | Dereferencing leads to undefined behaviour, often resulting in crashes or unexpected results. | Dereferencing explicitly results in a segmentation fault or access violation. |
Usage | It commonly occurs due to oversight or forgetting to initialize the pointer. | Intentionally used to represent absence of a valid memory address or to signify failure. |
Debugging | It may be harder to identify during debugging, as the pointer may hold any arbitrary value. | Typically easier to identify during debugging, as the pointer holds the specific value "NULL". |
Prevention | Best practice is to always initialize pointers before use to avoid uninitialized pointers. | Best practice is to explicitly assign NULL to pointers that are not currently pointing to valid memory locations. |
Now that we know about the distinct differentiation between the uninitialized and null pointer in C, let's look at an example C program for the former for a deeper understanding. Compare it with the null pointer in C example given above.
Code Example: (Uninitialized Pointer)
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKCgppbnQqIHVuaW5pdGlhbGl6ZWRQdHI7Cip1bmluaXRpYWxpemVkUHRyID0gNDI7CgpyZXR1cm4gMDsKfQ==
Output:
Segmentation Fault (Or blank screen)
Code Explanation:
In the example C code-
- We declare an integer pointer named uninitializedPtr without initializing it.
- This means that uninitializedPtr currently doesn't point to any valid memory location, and its value is indeterminate.
- Next, we attempt to dereference uninitializedPtr by assigning the value 42 to the memory location it points to using the indirection/ dereferencing operator (*).
- This operation is incorrect because uninitializedPtr hasn't been initialized to point to any valid memory location. Dereferencing it in this state leads to undefined behaviour, as the program tries to write to a memory location that hasn't been properly allocated.
- Finally, the main() function returns 0, indicating successful execution to the operating system.
- However, note that the program execution might encounter issues or crashes due to the attempted dereferencing of the uninitialized pointer.
Why Do We Need A NULL Pointer In C?
Null Pointers serve several important purposes in C programming, making them a crucial feature of the language. Here's why we need a null pointer in C programs:
- Indicating Absence of Data: Null pointers in C are used to represent the absence of a valid pointer or meaningful data. They serve as placeholders, indicating that a pointer does not currently point to any valid memory address.
- Error Signaling: Null pointers in C are commonly used to signal error conditions or exceptional cases, particularly in functions that perform operations like dynamic memory allocation (malloc(), calloc(), etc.). Functions may return a null pointer to indicate failure or error conditions, such as insufficient memory.
- Default Initialization: Initializing pointers to NULL provides a default and safe starting point, ensuring that pointers do not accidentally point to random memory locations. It helps prevent undefined behavior and runtime errors when pointers are used before being assigned valid memory addresses.
- Termination Conditions: In data structures like linked lists, binary trees, etc., null pointers are used to signify the end of a structure or indicate that a pointer does not point to any further elements. They allow for efficient traversal and manipulation of data structures by serving as termination conditions.
- Memory Allocation Failure Handling: Null pointers in C play a crucial role in handling memory allocation failures gracefully. Functions that allocate memory dynamically (malloc(), calloc(), etc.) return a null pointer if they fail to allocate the requested memory. Checking for null return values allows programs to detect and handle memory allocation failures appropriately.
- Prevention of Undefined Behavior: Dereferencing a null pointer in C programs leads to undefined behavior, often resulting in program crashes or unexpected behavior/ errors. Null pointers help prevent such situations by providing a clear indication that the pointer does not currently point to any valid memory address, allowing programmers to avoid dereferencing null pointers.
Now, let's take a look at a C program example that showcases the use of a null pointer to dynamically allocate memory and then access the elements it is pointing to.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdGRsaWIuaD4KCi8vIEZ1bmN0aW9uIHRvIGFsbG9jYXRlIG1lbW9yeSBmb3IgYW4gaW50ZWdlciBhcnJheQppbnQgKmFsbG9jYXRlX2FycmF5KGludCBzaXplKSB7CmludCAqYXJyYXkgPSAoaW50ICopbWFsbG9jKHNpemVvZihpbnQpICogc2l6ZSk7CmlmIChhcnJheSA9PSBOVUxMKSB7CnByaW50ZigiTWVtb3J5IGFsbG9jYXRpb24gZmFpbGVkXG4iKTsKfQpyZXR1cm4gYXJyYXk7Cn0KCmludCBtYWluKCkgewppbnQgKnB0ciA9IE5VTEw7IC8vIEluaXRpYWxpemUgcG9pbnRlciB0byBOVUxMCgovLyBBdHRlbXB0IHRvIGFsbG9jYXRlIG1lbW9yeSBmb3IgYW4gaW50ZWdlciBhcnJheQppbnQgc2l6ZSA9IDU7CnB0ciA9IGFsbG9jYXRlX2FycmF5KHNpemUpOwoKLy8gQ2hlY2sgaWYgbWVtb3J5IGFsbG9jYXRpb24gd2FzIHN1Y2Nlc3NmdWwKaWYgKHB0ciAhPSBOVUxMKSB7CnByaW50ZigiTWVtb3J5IGFsbG9jYXRpb24gc3VjY2Vzc2Z1bFxuIik7CgovLyBVc2UgdGhlIGFsbG9jYXRlZCBtZW1vcnkgKGZvciBkZW1vbnN0cmF0aW9uLCB3ZSdsbCBwcmludCB0aGUgYXJyYXkgZWxlbWVudHMpCnByaW50ZigiQXJyYXkgZWxlbWVudHM6ICIpOwpmb3IgKGludCBpID0gMDsgaSA8IHNpemU7IGkrKykgewpwcmludGYoIiVkICIsIHB0cltpXSk7Cn0KcHJpbnRmKCJcbiIpOwoKLy8gRnJlZSBhbGxvY2F0ZWQgbWVtb3J5CmZyZWUocHRyKTsKfQoKcmV0dXJuIDA7Cn0=
Output:
Memory allocation successful
Array elements: 0 0 0 0 0
Code Explanation:
In the sample C code-
- We define a function called allocate_array() to dynamically allocate memory to an array of integers and return a pointer to the memory block/ array. Inside the function-
- We create an integer pointer called array and use it with the malloc() function to allocate memory for an array with number of elements given by the size variable.
- The size in bytes for each element is determined using the sizeof() operator.
- Then, the pointer variable array is assigned the address to the memory block allocated to the integer array.
- We then check if the pointer is NULL using an if-statement. if the condition is true, then the allocation has failed and we print a string message to the same effect.
- The function then returns the array.
- Now, inside the main() function, we declare an integer pointer named ptr and initialize it to NULL. This initialization means that ptr currently doesn't point to any valid memory location.
- Next, we declare an integer variable size and assign it the value 5.
- We then call the allocate_array() function to allocate memory for an integer array of size 5 and assign the returned pointer to ptr.
- As mentioned in code comments, we then check if the memory allocation was successful by verifying if ptr is not NULL.
- Inside the if block, if the allocation was successful, we print a message indicating that memory allocation was successful.
- If the allocation is successful, we demonstrate the usage of the allocated memory by printing the elements of the array (though uninitialized) to show that the memory was indeed allocated.
- For this, we use a for loop to iterate through the array, printing each element as we go. The loop starts with loop variable i=0 and continues till i<size, incrementing the value of i by 1 at every iteration.
- We also use the pointer ptr to access the elements of the array at index position i, and the %d format specifier in the formatted string represents an integer value.
- The printf() statement with the newline escape sequence shifts the cursor to the next line.
- After using the allocated memory, we free it using free() function to release it back to the system.
How Do Null Pointers Work in C?
As mentioned before, a null pointer in C is a special pointer that does not point to any valid memory address. Here's how null pointers work in C:
- Declaration and Initialization: A null pointer in C is declared and initialized like any other pointer. It's assigned the special value NULL, typically defined as (void *)0 or 0, indicating that it currently points to no valid memory address.
- Use in Program Logic: Null pointers are used in program logic to represent the absence of a valid pointer. They can be assigned to pointer variables to indicate that they do not currently point to any valid memory location.
- Memory Allocation Failures: Functions like malloc(), calloc(), realloc(), etc., return a null pointer if they fail to allocate the requested memory. Programmers often check the return value of these functions against NULL to handle memory allocation failures gracefully.
- Error Handling: Null pointers in C are commonly used to signal errors or exceptional conditions, particularly in functions that deal with dynamic memory allocation or file operations. If a function fails to perform its task, it may return a null pointer to indicate failure.
- Termination Conditions: Null pointers in C are used in data structures like linked lists, binary trees, etc., to signify the end of a structure.
- Dereferencing: Dereferencing a null pointer in C, i.e., attempting to access the memory location it points to, leads to undefined behavior. This operation typically results in a segmentation fault or access violation, causing the program to crash.
- Prevention of Memory Leaks: Proper handling of null pointers is essential to prevent memory leaks in C programs. Memory allocated dynamically using functions like malloc() should be checked against NULL, and appropriate cleanup actions should be taken if allocation fails.
- Debugging and Testing: Null pointers in C can sometimes cause hard-to-debug issues in C programs. Debugging tools and techniques, such as runtime checks, assertions, and thorough testing, are employed to detect and fix null pointer-related errors.
Dereferencing A NULL Pointer In C
Dereferencing a pointer in C means accessing the value stored at the memory address pointed to by the pointer. It allows you to retrieve or modify the data stored at that memory location. However, attempting to dereference a NULL pointer in C, which points to no valid memory address, leads to undefined behavior and can result in program crashes or unexpected behavior.
Syntax:
*pointer;
Here,
- The dereference operator (*) is used to access the value at the memory address pointed to by the pointer.
- The term pointer is the identifier/ name of the pointer variable whose value points to a memory address.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50ICpwdHIgPSBOVUxMOyAvLyBJbml0aWFsaXplIHBvaW50ZXIgdG8gTlVMTAoKLy8gQXR0ZW1wdCB0byBkZXJlZmVyZW5jZSBhIE5VTEwgcG9pbnRlcgppbnQgdmFyID0gKnB0cjsgLy8gRGVyZWZlcmVuY2luZyBOVUxMIHBvaW50ZXIKCnByaW50ZigiVmFyaWFibGUgdmFsdWU6ICVkXG4iLCB2YXIpOyAvLyBUaGlzIGxpbmUgbWF5IGNhdXNlIHVuZGVmaW5lZCBiZWhhdmlvcgoKcmV0dXJuIDA7Cn0=
Output:
Segmentation fault (core dumped)
Code Explanation:
In the C code sample-
- We declare an integer pointer named ptr and initialize it to NULL.
- NULL is a special value that signifies a null pointer in C, meaning it doesn't point to any valid memory location.
- Next, we attempt to dereference the ptr pointer by assigning the value it points to a variable named var. Dereferencing a null pointer is illegal and results in undefined behavior.
- Afterwards, we use the printf() function to print the value of var. However, since the value was assigned by dereferencing a null pointer, this line may lead to undefined behavior, as we're trying to use an invalid memory location.
- Finally, the main() function returns 0, indicating successful execution.
How To Check If The Pointer Is NULL?
We check both the pointer's present location in memory and whether it currently carries the value NULL to determine if it is a Null pointer. When trying to dereference the pointer, this verification is essential to avoiding crashes and undefinable behavior. Let's look at how to do a pointer check for NULL along with a sample C program.
Mechanism:
- The NULL macro represents a null pointer, typically defined as (void *)0.
- To check if a pointer is NULL, we compare it against the NULL macro using the equality operator (==).
- If the pointer is NULL, the comparison evaluates to true, indicating that the pointer does not currently point to any valid memory address.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKaW50ICpwdHIgPSBOVUxMOyAvLyBJbml0aWFsaXplIHBvaW50ZXIgdG8gTlVMTAoKLy8gQ2hlY2sgaWYgcG9pbnRlciBpcyBOVUxMCmlmIChwdHIgPT0gTlVMTCkgewpwcmludGYoIlBvaW50ZXIgaXMgTlVMTFxuIik7Cn0gZWxzZSB7CnByaW50ZigiUG9pbnRlciBpcyBub3QgTlVMTFxuIik7Cn0KCnJldHVybiAwOwp9
Output:
Pointer is NULL.
Code Explanation:
In the basic C code example-
- We declare an integer pointer named ptr and initialize it to NULL. This initialization means that ptr currently doesn't point to any valid memory location.
- We then use an if-statement to check if ptr is equal to NULL. This condition verifies whether the pointer is indeed a null pointer.
- Inside the if block, if the condition is true, we print a message using printf() indicating that the pointer is NULL.
- If the condition is false, meaning the pointer is not NULL, we print a different message stating that the pointer is not NULL.
What Are The Uses Of NULL Pointer In C?
The Null pointer in C serves several essential purposes, facilitating robust and reliable programming practices. Here are the primary uses of the NULL pointer in C:
- Initialization: The NULL pointer in C is commonly used to initialize pointers before they are assigned valid memory addresses, ensuring that they start with a known safe value indicating they currently don't point to any valid memory location.
- Error Signaling: NULL pointers in C are often employed to signal errors or failure conditions, particularly in functions that dynamically allocate memory or perform file operations. Functions like malloc(), calloc(), fopen(), etc., return NULL to indicate failure.
- Termination Indication: In data structures like linked lists or binary trees, NULL pointers are used to signify the end of a structure or indicate that a pointer does not point to any further elements. For instance, in a linked list, the "next" pointer of the last node points to NULL, indicating the end of the list.
- Default or Placeholder Values: The NULL pointer in C can serve as a default or placeholder value, representing an absence of meaningful data. This usage is common in function arguments or return values where the absence of a valid value is a valid state.
- Memory Allocation Failure Handling: In applications that dynamically allocate memory using functions like malloc() or calloc(), checking for NULL return values is crucial to handle cases where memory allocation fails due to insufficient memory.
Examples Of Null Pointer In C
C Program To Check Successful Memory Allocation Using malloc()
The malloc function (short for 'memory allocation') is a standard library function used to dynamically allocate a block of memory from the heap for the course of time for which the C program is run. Data structures, arrays, and other objects requiring memory storage are frequently created via this memory allocation. The first byte of the memory block that was allocated is pointed to by the malloc function's return value.
Syntax:
void* malloc(size_t size);
Here,
- void*: A return type denoting a reference to undefined-type memory.
- size_t size: The amount of allocated bytes.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdGRsaWIuaD4KCmludCBtYWluKCkgewoKaW50IHNpemU7CmludCogZHluYW1pY0FycmF5OwoKLy8gSW5wdXQgc2l6ZSBvZiB0aGUgZHluYW1pYyBhcnJheQpwcmludGYoIkVudGVyIHRoZSBzaXplIG9mIHRoZSBkeW5hbWljIGFycmF5OiAiKTsKc2NhbmYoIiVkIiwgJnNpemUpOwoKLy8gQWxsb2NhdGUgbWVtb3J5IHVzaW5nIG1hbGxvYygpCmR5bmFtaWNBcnJheSA9IChpbnQqKW1hbGxvYyhzaXplICogc2l6ZW9mKGludCkpOwoKLy8gQ2hlY2sgaWYgbWVtb3J5IGFsbG9jYXRpb24gd2FzIHN1Y2Nlc3NmdWwKaWYgKGR5bmFtaWNBcnJheSA9PSBOVUxMKSB7CnByaW50ZigiTWVtb3J5IGFsbG9jYXRpb24gZmFpbGVkLlxuIik7CnJldHVybiAxOyAvLyBFeGl0IHdpdGggYW4gZXJyb3IgY29kZQp9CgpwcmludGYoIk1lbW9yeSBhbGxvY2F0aW9uIHN1Y2Nlc3NmdWwhXG4iKTsKCi8vIERlYWxsb2NhdGUgdGhlIGFsbG9jYXRlZCBtZW1vcnkKZnJlZShkeW5hbWljQXJyYXkpOwoKcmV0dXJuIDA7Cn0=
Output:
Enter the size of the dynamic array: 5
Memory allocation successful!
Code Explanation:
In the C program sample-
- We declare two variables, size and dynamicArray, of which the former will be used to store the size of the dynamic array provided by the user. And dynamicArray will be a pointer to the dynamically allocated array.
- Next, we use the printf() function to prompt the user to enter a value for the size of the array. We read the value and store it in the respective variable using the scanf() function with the reference/ address-of operator (&).
- Then, we allocate memory for the dynamic array using the malloc() function. The size of the allocated memory is calculated as size * sizeof(int) to accommodate size integers.
- We then check if the memory allocation was successful by verifying if the returned pointer dynamicArray is not NULL.
- If the allocation fails (i.e., dynamicArray is NULL), we print a message indicating that memory allocation failed and return 1, indicating an error.
- If the allocation succeeds, we print a message confirming that memory allocation was successful.
- Finally, we deallocate the allocated memory using free(dynamicArray) to release it back to the system.
C Program To Illustrate the Correct and Incorrect Use of NULL Pointer
When a pointer is initialized to NULL, it means it doesn't currently point to any valid memory location. In the correct usage, programmers check if a pointer is NULL before accessing the memory it points to, preventing runtime errors. Conversely, incorrect usage occurs when programmers attempt to access a NULL pointer without performing this check, leading to unpredictable behavior like program crashes. Proper handling of NULL pointers is essential for writing stable and secure C programs, ensuring memory access is controlled, and errors are minimized.
Syntax Of Null Pointer In C:
* pointer_name = NULL;
The syntax is same as the one given at the beginning of the article.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBGdW5jdGlvbiBwcm90b3R5cGVzCnZvaWQgY29ycmVjdFVzZShpbnQgKnB0cik7CnZvaWQgaW5jb3JyZWN0VXNlKGludCAqcHRyKTsKCmludCBtYWluKCkgewppbnQgKnB0ciA9IE5VTEw7IC8vIEluaXRpYWxpemUgcG9pbnRlciB0byBOVUxMCgovLyBDb3JyZWN0IHVzYWdlIG9mIE5VTEwgcG9pbnRlcgpjb3JyZWN0VXNlKHB0cik7CgovLyBJbmNvcnJlY3QgdXNhZ2Ugb2YgTlVMTCBwb2ludGVyCmluY29ycmVjdFVzZShwdHIpOwoKcmV0dXJuIDA7Cn0KCi8vIEZ1bmN0aW9uIGRlZmluaXRpb24gZGVtb25zdHJhdGluZyBjb3JyZWN0IHVzZSBvZiBOVUxMIHBvaW50ZXIKdm9pZCBjb3JyZWN0VXNlKGludCAqcHRyKSB7CmlmIChwdHIgIT0gTlVMTCkgeyAvLyBDaGVjayBpZiBwb2ludGVyIGlzIG5vdCBOVUxMIGJlZm9yZSBkZXJlZmVyZW5jaW5nCnByaW50ZigiQ29ycmVjdCB1c2FnZTogRGVyZWZlcmVuY2luZyBwb2ludGVyOiAlZFxuIiwgKnB0cik7Cn0gZWxzZSB7CnByaW50ZigiQ29ycmVjdCB1c2FnZTogUG9pbnRlciBpcyBOVUxMXG4iKTsKfQp9CgovLyBGdW5jdGlvbiBkZWZpbml0aW9uIGRlbW9uc3RyYXRpbmcgaW5jb3JyZWN0IHVzZSBvZiBOVUxMIHBvaW50ZXIKdm9pZCBpbmNvcnJlY3RVc2UoaW50ICpwdHIpIHsKcHJpbnRmKCJJbmNvcnJlY3QgdXNhZ2U6IERlcmVmZXJlbmNpbmcgcG9pbnRlcjogJWRcbiIsICpwdHIpOyAvLyBJbmNvcnJlY3RseSBkZXJlZmVyZW5jaW5nIE5VTEwgcG9pbnRlcgp9
Output:
Correct usage: Pointer is NULL
Segmentation fault
Code Explanation:
In the C example-
- We create two function prototypes, i.e., correctUse() and incorrectUse(), both taking an integer pointer as a parameter.
- Within the main() function, we declare an integer pointer ptr and initialize it to NULL.
- Then, we call the correctUse() function with ptr as an argument, demonstrating the correct usage of a NULL pointer.
- After that, we call the incorrectUse() function with ptr as an argument, demonstrating the incorrect usage of a NULL pointer.
- The main() function closes with a return of 0.
- In the function definitions, correctUse() uses an if-else statement to check if the pointer is not NULL before dereferencing it.
- If the pointer is not NULL, it prints the value it points to.
- If the pointer is NULL, it prints a message indicating that the pointer is NULL.
- The incorrectUse() function directly dereferences the pointer without checking if it's NULL. This results in undefined behavior because we're attempting to access memory that doesn't exist.
This example shows why it is important to check if the NULL pointer was successfully created in dynamic memory allocation.
C Program To Demonstrate How To Pass NULL Pointer In C To A Function
The use of a void function in this example serves to illustrate the idea of sending a NULL reference to a function. A function that returns nothing is referred to as a void function. It is frequently employed for acts or processes that don't provide a consequence.
Syntax Of Function Declaration:
void functionName(char* parameter);
Here,
- The return type of the function is given by void, indicating no fixed data type, and the functionaName refers to the name/ identifier for the function defined here.
- The expression char* parameter refers to a pointer of character type, representing a string variable.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBGdW5jdGlvbiBwcm90b3R5cGUKdm9pZCBwcm9jZXNzU3RyaW5nKGNoYXIgKnN0cik7CgppbnQgbWFpbigpIHsKY2hhciAqcHRyID0gTlVMTDsgLy8gSW5pdGlhbGl6ZSBwb2ludGVyIHRvIE5VTEwKCi8vIENhbGwgdGhlIGZ1bmN0aW9uIHdpdGggYSBOVUxMIHBvaW50ZXIKcHJvY2Vzc1N0cmluZyhwdHIpOwoKcmV0dXJuIDA7Cn0KCi8vIEZ1bmN0aW9uIGRlZmluaXRpb24gdG8gcHJvY2VzcyBhIHN0cmluZwp2b2lkIHByb2Nlc3NTdHJpbmcoY2hhciAqc3RyKSB7CmlmIChzdHIgPT0gTlVMTCkgewpwcmludGYoIlN0cmluZyBwb2ludGVyIGlzIE5VTExcbiIpOwp9IGVsc2UgewpwcmludGYoIlByb2Nlc3Npbmcgc3RyaW5nOiAlc1xuIiwgc3RyKTsKfQp9
Output:
String pointer is NULL
Code Explanation:
In the code-
- We have a function prototype processString() that takes a character pointer as a parameter. We will define it later all.
- In the main() function, we declare and initialize a character pointer ptr and initialize it to NULL value, indicating that it currently doesn't point to any valid memory location.
- We then call the processString() with ptr as an argument, demonstrating the scenario of passing a NULL pointer to the function.
- The main() function terminates after a successful execution with return 0.
- In the function definition processString(), we check if the pointer str is NULL using an if-else statement.
- If the pointer is NULL, the function uses a printf() statement to display a message indicating that the string pointer is NULL.
- If the pointer is not NULL, it assumes that it points to a valid string and prints the string using %s format specifier in the printf() function.
Important Note: There are two more methods through which we can demonstrate the usage of Null pointers. However these methods are not explicitly present in C, hence we will explain them with the help of C++ programming language:
Example To Illustrate Inappropriate Use Of NULL Constant In Overloaded Functions
Using NULL as an argument for overloaded functions is not appropriate because it leads to ambiguity for the compiler in selecting the correct function to call based on the argument's data type. It's important to provide unambiguous and properly typed arguments to overloaded functions to ensure correct function resolution.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdm9pZCBmb28oaW50IHgpIHsKc3RkOjpjb3V0IDw8ICJJbnRlZ2VyIHZlcnNpb246ICIgPDwgeCA8PCBzdGQ6OmVuZGw7Cn0KCnZvaWQgZm9vKGNoYXIqIHN0cikgewpzdGQ6OmNvdXQgPDwgIlN0cmluZyB2ZXJzaW9uOiAiIDw8IHN0ciA8PCBzdGQ6OmVuZGw7Cn0KCmludCBtYWluKCkgewpmb28oTlVMTCk7IC8vIEluYXBwcm9wcmlhdGUgdXNlIG9mIE5VTEwgd2l0aCBvdmVybG9hZGVkIGZ1bmN0aW9ucwpyZXR1cm4gMDsKfQ==
Code Explanation:
In the above code,
- We have two overloaded functions named foo(). One takes an integer parameter x, and the other takes a character pointer str.
- Within the main() function, we call the foo() function with NULL as an argument, demonstrating the inappropriate use of NULL with overloaded functions.
- C++ allows this usage due to the null pointer being implicitly convertible to any pointer type, which may lead to ambiguity in function resolution.
- In the function definitions foo(), the first version of foo() takes an integer parameter x and prints the value of x.
- The second version of foo() takes a character pointer str and prints the string pointed to by str.
Example To Illustrate Use Of NULL Pointer For Overloading
In C, the concept of nullptr does not exist as it does in C++. In C++, the nullptr keyword is used to represent a null pointer constant, and it's particularly useful for overloading functions that accept pointer arguments. Here's an example illustrating how nullptr can be used in function overloading:
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKdm9pZCBmb28oaW50IHgpIHsKc3RkOjpjb3V0IDw8ICJJbnRlZ2VyIHZlcnNpb246ICIgPDwgeCA8PCBzdGQ6OmVuZGw7Cn0KCnZvaWQgZm9vKGNoYXIqIHN0cikgewpzdGQ6OmNvdXQgPDwgIlN0cmluZyB2ZXJzaW9uOiAiIDw8IHN0ciA8PCBzdGQ6OmVuZGw7Cn0KCnZvaWQgZm9vKHN0ZDo6bnVsbHB0cl90IHB0cikgewpzdGQ6OmNvdXQgPDwgIk51bGwgcG9pbnRlciB2ZXJzaW9uIiA8PCBzdGQ6OmVuZGw7Cn0KCmludCBtYWluKCkgewpmb28oMTApOyAvLyBDYWxscyBmb28oaW50KQpmb28oIkhlbGxvIik7IC8vIENhbGxzIGZvbyhjaGFyKikKZm9vKG51bGxwdHIpOyAvLyBDYWxscyBmb28oc3RkOjpudWxscHRyX3QpCnJldHVybiAwOwp9
Output:
Integer version: 10
String version: Hello
Null pointer version
Code Explanation:
In the above code-
- We have three overloaded versions of the function foo(). One takes an integer parameter x, another takes a character pointer str, and the third takes a std::nullptr_t parameter ptr.
- Within the main() function, we call the foo() function with different types of arguments:
- foo(10) calls the version of foo() that takes an integer parameter and prints- "Integer version: 10".
- foo("Hello") calls the version of foo() that takes a character pointer parameter and prints- "String version: Hello".
- foo(nullptr) calls the version of foo() that takes a std::nullptr_t parameter and prints- "Null pointer version".
- The main() function returns 0, indicating successful execution to the operating system.
Application Of NULL Pointer In C
We have already mentioned the uses and benefits of the null pointer in C. In this section, we will explore some of the most important applications of the null pointer in C programs with the help of proper examples.
1. Null Pointer In C For Initializing Pointers:
One of the most common applications of NULL pointer in C is initializing other pointers to indicate that they are not currently pointing to any valid memory location.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKCmludCAqcHRyID0gTlVMTDsgLy8gSW5pdGlhbGl6ZSBwb2ludGVyIHRvIE5VTEwKcHJpbnRmKCJQb2ludGVyIHZhbHVlOiAlcFxuIiwgcHRyKTsgLy8gT3V0cHV0OiBQb2ludGVyIHZhbHVlOiAobmlsKQoKcmV0dXJuIDA7Cn0=
Output:
Pointer value: (nil)
Code Explanation:
In this example,
- We initialize the pointer ptr to NULL, which indicates that ptr does not currently point to any valid memory address.
- Then, we use the printf() function to print the value of ptr, which shows (nil), indicating that it is NULL.
2. Null Pointer In C For Error Handling:
The NULL pointer in C is commonly used in error handling to indicate failure to allocate memory dynamically or to access a resource.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdGRsaWIuaD4KCmludCBtYWluKCkgewppbnQgKnB0ciA9IG1hbGxvYyhzaXplb2YoaW50KSk7IC8vIEFsbG9jYXRlIG1lbW9yeSBkeW5hbWljYWxseQppZiAocHRyID09IE5VTEwpIHsKcHJpbnRmKCJNZW1vcnkgYWxsb2NhdGlvbiBmYWlsZWRcbiIpOwpyZXR1cm4gMTsgLy8gUmV0dXJuIGVycm9yIGNvZGUKfQpwcmludGYoIk1lbW9yeSBhbGxvY2F0aW9uIHN1Y2Nlc3NmdWxcbiIpOwpmcmVlKHB0cik7IC8vIEZyZWUgYWxsb2NhdGVkIG1lbW9yeQpyZXR1cm4gMDsKfQ==
Output:
Memory allocation successful
Code Explanation:
In this example,
- We use the malloc() function and sizeof() operator to dynamically allocate memory to an integer pointer ptr.
- Then, we check if the allocation was successful by verifying if ptr is NULL.
- If ptr is NULL, it means memory allocation failed, and we print an error message.
- Otherwise, if ptr is not NULL, it means memory allocation was successful, and we proceed with using the allocated memory.
3. Null Pointer In C As A Function Parameters:
Functions in C can accept NULL pointers as arguments to indicate the absence of valid data or optional parameters.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+Cgp2b2lkIHByaW50U3RyaW5nKGNoYXIgKnN0cikgewppZiAoc3RyID09IE5VTEwpIHsKcHJpbnRmKCJObyBzdHJpbmcgcHJvdmlkZWRcbiIpOwp9IGVsc2UgewpwcmludGYoIlN0cmluZzogJXNcbiIsIHN0cik7Cn0KfQoKaW50IG1haW4oKSB7CgpwcmludFN0cmluZygiSGVsbG8iKTsgLy8gT3V0cHV0OiBTdHJpbmc6IEhlbGxvCnByaW50U3RyaW5nKE5VTEwpOyAvLyBPdXRwdXQ6IE5vIHN0cmluZyBwcm92aWRlZAoKcmV0dXJuIDA7Cn0=
Output:
String: Hello
No string provided
Code Explanation:
In the above example,
- We define a printString() function, which accepts a pointer to a string (char *) as an argument. Inside the function-
- We check if the pointer is NULL to determine if a string was provided.
- If the pointer is NULL, we print a message indicating that no string was provided.
- Otherwise, if the pointer is not NULL, we print the provided string.
- In main() function, we call the printString() function twice, first with the string Hello as a parameter and then with the NULL value.
- As shown in the output the second time, we get the output that no string was provided, indicating invalid data.
4. Null Pointer In For Terminating Linked Lists:
The null pointer in C is often used to indicate the end of a list in the linked list implementations. The last node in the list typically points to NULL, indicating that there are no more nodes after it.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdGRsaWIuaD4KCi8vIERlZmluZSBhIG5vZGUgc3RydWN0dXJlIGZvciBhIGxpbmtlZCBsaXN0CnN0cnVjdCBOb2RlIHsKaW50IGRhdGE7CnN0cnVjdCBOb2RlICpuZXh0Owp9OwoKaW50IG1haW4oKSB7CnN0cnVjdCBOb2RlICpoZWFkID0gTlVMTDsgLy8gSW5pdGlhbGl6ZSBoZWFkIHBvaW50ZXIgdG8gTlVMTAoKLy8gQ29kZSB0byBjcmVhdGUgYW5kIG1hbmlwdWxhdGUgbGlua2VkIGxpc3Qgbm9kZXMuLi4KCi8vIFRyYXZlcnNlIHRoZSBsaW5rZWQgbGlzdCB1bnRpbCByZWFjaGluZyB0aGUgZW5kIChOVUxMIHBvaW50ZXIpCnN0cnVjdCBOb2RlICpjdXJyZW50ID0gaGVhZDsKCndoaWxlIChjdXJyZW50ICE9IE5VTEwpIHsKcHJpbnRmKCIlZCAiLCBjdXJyZW50LT5kYXRhKTsKY3VycmVudCA9IGN1cnJlbnQtPm5leHQ7Cn0KCnJldHVybiAwOwp9
Code Explanation:
In this example,
- We define a structure called Node using the struct keyword to represent a node in a linked list.
- Each node contains an integer type data field called data and a pointer to the next node called next, linking the nodes together.
- Then, we initialize the pointer to the structure Node called head to NULL to indicate an empty list.
- As mentioned in the comments, we must add code to create a linked list and allocate memory to it using malloc().
- As nodes are added to the linked list, each node's next pointer points to the next node in the list, and the last node's next pointer is set to NULL, indicating the end of the list.
- During traversal of the linked list using a while loop, we iterate through the nodes until reaching NULL, which signifies the end of the list.
5. Null Pointer In C For Initializating File Pointers:
File pointers in C are commonly initialized to NULL before being assigned a file stream. This ensures that the file pointer is in a known state before opening or accessing files.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgppbnQgbWFpbigpIHsKRklMRSAqZnAgPSBOVUxMOyAvLyBJbml0aWFsaXplIGZpbGUgcG9pbnRlciB0byBOVUxMCgovLyBDb2RlIHRvIG9wZW4gYW5kIG1hbmlwdWxhdGUgZmlsZXMuLi4KCnJldHVybiAwOwp9
Code Explanation:
In this example,
- We declare a file pointer fp and initialize it to NULL.
- Before opening a file or performing any file operations, we typically check if the file pointer is NULL to ensure that it's properly initialized.
- After opening a file, the file pointer will be assigned the address of the file stream, allowing us to perform read, write, or seek operations on the file.
6. Null Pointer In C For Clearing Pointers:
Assigning NULL to pointers after deallocating memory or to indicate that a pointer no longer references valid memory locations is a common practice to prevent potential bugs.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdGRsaWIuaD4KCmludCBtYWluKCkgewppbnQgKnB0ciA9IG1hbGxvYyhzaXplb2YoaW50KSk7IC8vIEFsbG9jYXRlIG1lbW9yeSBkeW5hbWljYWxseQovLyBVc2UgcHRyLi4uCmZyZWUocHRyKTsgLy8gRGVhbGxvY2F0ZSBtZW1vcnkKcHRyID0gTlVMTDsgLy8gQ2xlYXIgcG9pbnRlciB0byBhdm9pZCBhY2Nlc3NpbmcgZGVhbGxvY2F0ZWQgbWVtb3J5CnJldHVybiAwOwp9
Code Explanation:
In this example,
- We allocate memory dynamically using malloc() function.
- After using the allocated memory, we deallocate it using free() function.
- To prevent accessing deallocated memory, we assign NULL to the pointer ptr, indicating that it no longer points to valid memory.
- This practice helps avoid potential bugs caused by accessing memory that has been freed.
Best Practices For NULL Pointer Usage
Using the NULL pointer in C requires adherence to best practices to ensure program reliability and minimize the risk of runtime errors. Here are some best practices for the usage of NULL pointer in C programs:
1. Check for NULL Before Dereferencing: Before dereferencing a pointer, ensure it is not NULL to avoid segmentation faults or undefined behavior. Use conditional statements to check for NULL pointers before accessing their memory locations.
if (ptr != NULL) {
*ptr = value; // Dereference pointer only if it's not NULL
} else {
// Handle the case when the pointer is NULL
}
2. Use NULL to Indicate Failure or Absence: Use NULL to represent the absence of a valid pointer or failure conditions, such as when a function cannot return a meaningful result or when dynamic memory allocation fails.
int *ptr = allocate_memory(size);
if (ptr == NULL) {
// Handle memory allocation failure
}
3. Check Return Values of Library Functions: Many library functions in C return NULL to indicate failure or error conditions. Always check the return values of functions like malloc(), calloc(), realloc(), fopen(), etc., to ensure successful operation.
FILE *file = fopen("example.txt", "r");
if (file == NULL) {
// Handle file opening failure
}
4. Document NULL Usage: Clearly document the usage of NULL pointers in C code, especially for functions or data structures where NULL is a valid or expected value. Document the conditions under which NULL may be returned or accepted as an argument.
5. Avoid Unnecessary Comparisons with NULL: Avoid unnecessary comparisons with NULL, especially in conditional statements. Pointer variables can be evaluated directly in conditional statements without explicitly comparing them to NULL.
// Instead of this:
if (ptr != NULL) {
// Do something
}
// Prefer this:
if (ptr) {
// Do something
}
6. Use Defensive Programming Techniques: Employ defensive programming practices such as assertions or error handling to catch NULL pointer errors during development and testing. Assertions can help identify NULL dereference pointer variables early in the development process.
assert(ptr != NULL);
By following these best practices, developers can effectively use the NULL pointer in C while minimizing the risk of runtime errors and improving program reliability and robustness.
What Is The Difference Between The Void Pointer & Null Pointer In C?
A void pointer and a null pointer are two distinct concepts in C programming, each serving different purposes. The table below highlights the difference between the void pointer and the null pointer in C.
Feature | Void Pointer | Null Pointer |
---|---|---|
Syntax | void *ptr; | Type *ptr = NULL; or Type *ptr = 0; |
Definition | A pointer that can point to any data type | A pointer that does not point to any actual memory address |
Data Type | It can point to any data type. | It does not point to any data type; it is used to represent the absence of a valid memory address. |
Memory Size | Depends on the platform (typically the same as regular pointers). | Typically 4 or 8 bytes (depending on architecture). |
Use Case | Useful for implementing generic functions or data structures where the type is unknown or needs to be generic | Used to represent a pointer that does not currently point to any valid memory location, often used for error handling or initialization purposes |
Dereferencing | Dereferencing a void pointer requires explicit casting to the desired data type | Not dereferenced; used as a marker for the absence of valid memory. |
Type Safety | Not type-safe; requires explicit typecasting before use. | Type-safe; specific to pointer types (e.g., int *, char *). |
Example | c int num = 10; void *ptr = # int *ptr_int = (int *)ptr; | c int *ptr = NULL; *ptr = 10; |
Conclusion
The null pointer in C is an essential tool that represents the absence of valid memory addresses. These pointers are used for error handling, memory management, and ensuring program stability. By initializing pointers to NULL, verifying against NULL before dereferencing, and using null pointers in C code to indicate failure or exceptional conditions in functions, developers can write robust and reliable programs.
Understanding the significance, usage, and best practices associated with the null pointer in C is essential for writing clear, maintainable, and bug-free code. Leveraging null pointers effectively allows developers to handle errors gracefully, prevent crashes or segmentation faults, and ensure the stability and reliability of their C programs.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What happens if you dereference a null pointer in C?
Dereferencing a null pointer in C leads to undefined behavior. This means that the outcome of such an operation cannot be predicted or controlled by the language specification, compiler, or runtime environment.
- When you attempt to access or manipulate the data stored at the memory address pointed to by a null pointer in C, the behavior can vary widely.
- In some cases, it may result in a segmentation fault, causing the program to terminate abruptly.
- In other cases, it might lead to unpredictable behavior such as data corruption, crashes, or even seemingly correct execution despite the violation.
Q. Can we use the sizeof() operator on NULL in C?
The sizeof() operator in C is used to determine the size of a data type, variable, or expression in terms of bytes. It's evaluated at compile-time and returns the size as a constant expression of type size_t. You can use the sizeof() operator on data types, variables, or expressions in C to determine their size.
For example:
int size_of_int = sizeof(int);
int array[10];
int size_of_array = sizeof(array);
In the above examples, size_of_int refers to the size of an integer type, typically 4 bytes on most systems, and size_of_array to the size of the array array, which is 10 * sizeof(int).
However, you cannot use the sizeof() operator directly on NULL in C. The reason is that NULL is a macro that evaluates to a null pointer in C, which is not a data type or a variable. Therefore, using sizeof(NULL) is not valid in C. If you want to determine the size of a pointer type, you would use sizeof() on a pointer variable, not on the NULL macro itself.
For example:
int *ptr = NULL;
int size_of_pointer = sizeof(ptr);
In this case, size_of_pointer refers to the size of a pointer type, typically 4 or 8 bytes, depending on the system architecture.
Q. What is the size of Null In C?
In C, NULL is typically defined as a null pointer constant, representing a pointer that does not point to any valid memory location. The size of NULL in C depends on the architecture and compiler being used.
- However, NULL is commonly defined as (void *)0, which is a pointer type.
- The size of a pointer type depends on the architecture of the system.
- On most modern systems, where memory addresses are 32 or 64 bits wide, the size of a pointer is typically 4 or 8 bytes, respectively.
Therefore, in most cases, the size of NULL in C is either 4 bytes or 8 bytes, depending on the architecture. This size represents the amount of memory required to store a memory address, which is used to represent the null pointer value.
Q. Why are null pointers used?
Null pointers in C are used to represent the absence of a valid memory address. They serve several important purposes in programming:
- Error Handling: Null pointers in C are commonly used to indicate errors or exceptional conditions, particularly in functions that return pointers. For example, if a memory allocation function fails to allocate memory, it returns a null pointer to signal the failure.
- Memory Management: Null pointers in C are essential in dynamic memory allocation to indicate that memory allocation has failed. Functions like malloc, calloc, and realloc return null pointers if they are unable to allocate the requested memory.
- Pointer Initialization: Initializing pointers to null at the start of a program or before they are assigned valid memory addresses helps ensure predictable behavior. It prevents undefined behavior that can occur when dereferencing uninitialized pointer variables.
- Data Structure Terminators: Null pointers in C are often used as terminators in data structures like linked lists, trees, or arrays. For example, the last node of a linked list typically points to null to signify the end of the list.
- Default Values: In some cases, null pointers are used as default values for pointer variables. If a pointer variable is not assigned a valid memory address, it may be initialized to null to indicate that it is not currently pointing to anything.
Q. Are Null and Void the same?
Null and void are related concepts in programming but are not the same.
- Null: In programming, null typically refers to a special value that represents the absence of a valid or meaningful object or reference. For example, in C programming, NULL is a macro that represents a null pointer, which is a pointer that does not currently point to any valid memory address. Null is commonly used to signify missing or undefined data, such as when a pointer is not initialized or when a function fails to return a valid result.
- Void: Void, on the other hand, refers to a data type in some programming languages, including C. The void data type indicates the absence of any type, meaning that it does not hold any value. In function declarations, void can be used to specify that the function does not return any value or that it takes no parameters. For example, a function with a void return type does not return any value to the caller, while a function with void parameters does not accept any arguments.
Q. How do you initialize a null pointer in C?
You can initialize a null pointer in C, simply by assigning the special value NULL to a pointer variable. NULL is a macro defined in the standard C libraries, typically as ((void *)0), although its exact definition can vary across implementations. The syntax to initialize a pointer as a null pointer in C is:
int *ptr = NULL;
In this example, ptr is a pointer variable of type int *, and it's initialized to NULL. This indicates that ptr currently does not point to any valid memory address, i.e., it is a null pointer in C.
Here are a few interesting C articles you must read:
- Control Statements In C | The Beginner's Guide (With Examples)
- Pointer Arithmetic In C & Illegal Arithmetic Explained (+Examples)
- Array Of Pointers In C & Dereferencing With Detailed Examples
- Reverse A String In C | 10 Different Ways With Detailed Examples!
- Comma Operator In C | Code Examples For Both Separator & Operator
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment