Dynamic Memory Allocation In C | Layout & 4 Methods With Examples
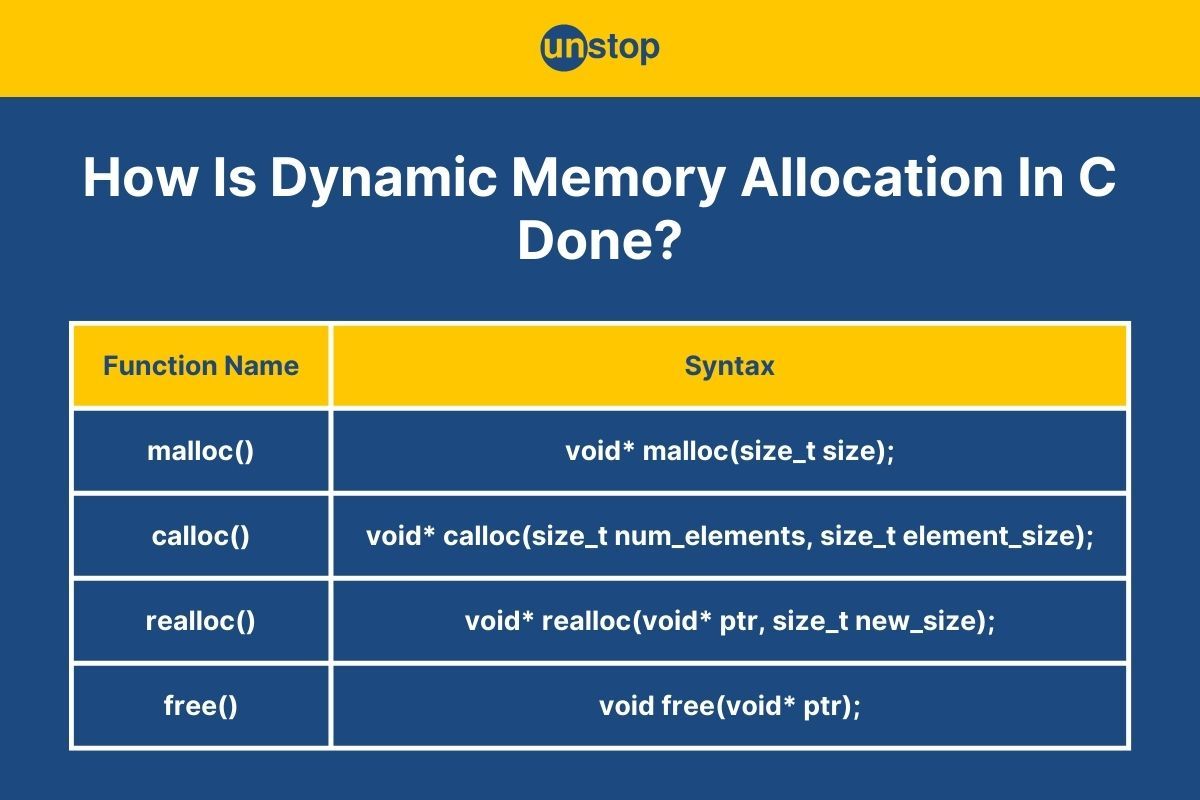
Table of content:
- How Does Memory Management Works In C Programs?
- Memory Allocation Process & Dynamic Memory Allocation In C
- What Is Dynamic Memory Allocation In C?
- Ways Of Dynamic Memory Allocation In C
- The malloc() Method For Dynamic Memory Allocation In C
- The calloc() Method For Dynamic Memory Allocation In C
- The free() Method For Dynamic Memory Allocation In C
- The realloc() Method For Dynamic Memory Allocation In C
- Pointers & Dynamic Memory Allocation In C
- Malloc() Vs Calloc() Methods Of Dynamic Memory Allocation In C
- Conclusion
- Frequently Asked Questions
In the world of computers, memory serves as the primary data storage and manipulation platform. It is categorized into two primary domains, i.e., stack memory and heap memory. To understand the concept of memory and allocation/ dynamic memory allocation in C programming, you must know about the two memory types-
- Stack Memory: This region is dedicated to storing local variables and the essential bookkeeping needed for managing function call hierarchies. It adheres to a Last-In, First-Out (LIFO) mechanism and boasts a predetermined, compile-time-set size.
- Heap Memory: In contrast to stack memory's predictability, heap memory provides dynamic freedom. This opens up a world of possibilities for designing flexible data structures of various sizes because programmers have the option to both allocate and release memory during program runtime.
The ability to manage memory, which plays a critical role in programming, will grant you the ability to construct flexible data structures and solve multiple programming problems. In this article, we will cover dynamic memory allocation in C programming language in detail. So, let’s begin the journey to explore the intricate mechanism behind dynamic memory allocation in C.
How Does Memory Management Works In C Programs?
When a C program is executed, it needs to allocate memory to store its variables, functions, instructions, and the program file itself. Additionally, there's a segment of memory that can be used dynamically during a program's runtime. Let's explore the memory components/segments used during the execution of a C program.
The system's memory is divided into four main segments:
- Stack Segment (Static Memory): This segment is allocated during compile-time. It is used to store local variables, function calls, and local statements within a function. The size of the stack is typically constant, and it is a significant part of the Static Memory.
- Global Variables Segment (Static Memory): Global variables, also known as static variables, are stored in this segment. They can be declared using the static keyword or by declaring them outside of the main() or any other function. Like the stack, the memory for global variables is allocated during compile-time.
- Instructions / Text Segment (Static Memory): Instructions and code outside of the main() function are stored in this segment. These instructions are stored during the compilation of the program. The size of this segment is fixed and cannot be reused until the program execution finishes.
- Heap Segment (Dynamic Memory): The heap is a dynamic memory segment that can be used during runtime. It can be imagined as a large pool of memory available to the program. Unlike the other segments, the space occupied by the heap is not fixed and can vary during program runtime. Functions exist for the allocation and deallocation of memory blocks in the heap, making it a flexible resource.
Key points to remember related to these memory segments:
- Instructions / Text: Instructions outside the main() function are stored in static memory during compile-time.
- Global Variables: Global (static) variables are stored in the static memory during the program's compilation.
- Stack: Stack memory is used for local variables, function calls, and local statements. It's a constant-size memory allocation made by the operating system and is part of the static memory. However, it has limitations, such as not being able to grow during runtime or allocate/deallocate memory.
- Heap: The heap is dynamic memory that can expand or contract during program runtime. It's a flexible resource, but it's important to manage it carefully to avoid exhausting the system's memory.
Memory Management Procedure | DynamicMemory Allocation In C
The complete procedure of memory management involves several steps:
Step 1- Allocation: This step involves reserving a portion of memory for a specific purpose, such as storing data or program instructions. In languages like C or C++, you can allocate memory on the heap using functions like malloc() or the new operator. In languages like Python or Java, memory allocation is typically abstracted away from the programmer.
Step 2- Tracking Memory Usage: It's essential to keep track of how memory is used to avoid issues like memory leaks (unreleased memory) or memory fragmentation (wasted memory). Tools like memory profilers or garbage collectors (in languages like Java) help manage memory usage.
Step 3- Deallocation: Once memory is no longer needed, it should be released to make it available for other purposes. In languages like C, you must manually free memory using free() when done with dynamically allocated memory.
Memory Allocation Process & Dynamic Memory Allocation In C
The memory allocation mechanism in programming involves reserving memory space for variables, data structures, or objects during the execution of a program. In C, memory allocation can occur statically, automatically (on the stack), or dynamically (on the heap). Here's a detailed explanation of each step involved in the memory allocation process:
1. Include Necessary Headers: Include the required header files for memory allocation functions.
#include <stdio.h>
#include <stdlib.h>
2. Declare Pointer: Declare a pointer variable to hold the address of the allocated memory block.
int *ptr;
3. Allocate Memory: Use a memory allocation function (malloc(), calloc(), etc.) to request memory from the heap.
ptr = (int *)malloc(sizeof(int));
4. Check for Allocation Success: Verify if the memory allocation was successful by checking if the pointer is not NULL.
if (ptr == NULL) {
printf("Memory allocation failed\n");
return 1;
}
5. Access and Use Memory: Access and use the allocated memory as needed to store data or create dynamic data structures.
*ptr = 42; // Store a value in the allocated memory
6. Deallocate Memory (Optional): If the allocated memory is no longer needed, free it using the free() function to prevent memory leaks.
free(ptr);
Let's look at a simple C program to better understand this concept.
Code Example:
Output:
Value stored at dynamically allocated memory: 98
Code Explanation:
In the C code sample-
- We include the necessary header files <stdio.h> and <stdlib.h> to access the standard input/output functions and memory allocation functions, respectively.
- Then, we declare a pointer variable ptr of data type integer (int) to hold the address of the allocated memory block.
- Using the malloc() function, we allocate memory dynamically for an integer (sizeof(int)), using the sizeof() operator to determine the size in bytes.
- Next, we use an if-statement to check if the memory allocation was successful. For this, we check if the pointer ptr is NULL using the equality relational operator.
- If the condition is true, i.e., ptr == NULL, then it means the allocation has failed; we print an error message and exit the program.
- If the condition is false, it means the allocation is successful, and the flow moves to the next line in the code.
- We then store a value (98 in this case) in the dynamically allocated memory using the pointer ptr.
- Finally, we print the value stored in the dynamically allocated memory using the printf() function and then free the memory using the free() function to avoid memory leaks.
There are two types of memory allocation in C., i.e., static and dynamic.
Static Memory Allocation In C
Static memory allocation refers to the allocation of memory for variables at compile time, where the size and type of memory required are determined before the program is executed. It involves setting aside a fixed amount of memory for variables, and this memory allocation remains constant throughout the program's execution. This method is in contrast to dynamic memory allocation in C, where memory is allocated during runtime using functions like malloc().
Process of Static Memory Allocation:
- Declaration: Variables are declared with a specific data type and name in the program code.
- Memory Allocation: Memory for these variables is allocated during the compilation phase based on their data types and sizes.
- Memory Address Assignment: Assign memory addresses to variables, and the values are stored at these addresses.
- Fixed Memory Usage: The allocated memory remains constant throughout the program's execution, and the variables retain their values until the program terminates.
Syntax:
data_type variable_name = initial_value;
Here,
- Data Type: Specify the data type of the variable (e.g., int, float, char).
- Variable Name: Give a unique name to the variable to access it in your code.
- Optional Initialization: Use = to assign an initial value (optional).
- Initial Value: If assigned, it should match the variable's data type.
Code Example:
Output:
Value of staticVar: 42
Code Explanation:
In the C code example-
- We begin by including the header file for input/ output operations, i.e., <stdio.h> and then initiate the main() function, which is the program's entry point for execution.
- In the main function, we declare an integer data type variable named staticVar and assign it the value of 42.
- This variable is statically allocated, meaning that memory for it is allocated at compile-time and is fixed throughout the program's execution.
- Next, we use a printf() statement to display the value of the staticVar variable along with a string message. Here, the %d format specifier acts as the placeholder for the integer value.
- Finally, the main function returns 0 to indicate the successful execution of the program.
Static memory allocation is suitable for scenarios where the memory requirements are known in advance and do not change during runtime. It is commonly used for global variables, constants, and other situations where memory stability is critical. However, it may not be flexible enough for cases where variable memory sizes or lifetimes need to be determined dynamically, which is where dynamic memory allocation comes into play.
What Is Dynamic Memory Allocation In C?
Dynamic memory allocation refers to the process of reserving memory for variables or data structures during program execution, typically at runtime. Unlike static memory allocation, where memory is allocated at compile time and remains constant, dynamic memory allocation in C allows programs to allocate and deallocate memory as needed during runtime. This flexibility is essential for managing memory efficiently, especially when dealing with data structures of varying sizes or lifetimes.
Key Concepts in Dynamic Memory Allocation in C:
- Heap Memory: Dynamic memory allocation in C typically involves using a region of memory known as the heap. The heap is a dynamic storage area that can grow or shrink as needed, making it suitable for allocating memory during program execution.
- Allocation Functions: Most programming languages provide functions or operators for dynamic memory allocation. In C/C++, malloc(), calloc(), and realloc() are commonly used functions for memory allocation, while free() is used for deallocation. In languages like Python and Java, memory allocation and deallocation are abstracted from the programmer.
- Lifetime Control: With dynamic memory allocation in C, the programmer has control over the lifetime of allocated memory. Memory can be allocated when needed and released when it's no longer required, reducing the risk of memory leaks.
Differences Between Static & Dynamic Memory Allocation In C
Here are the key differences between static and dynamic memory allocation in C:
Feature | Static Allocation | Dynamic Allocation |
---|---|---|
Memory Allocation | Occurs at compile time | Occurs at runtime |
Memory Management | Fixed memory size and lifetime | Variable memory size and lifetime |
Flexibility | Limited flexibility | Offers flexibility in memory management |
Memory Usage | Memory usage is fixed | Memory usage can vary during program execution |
Initialization | Memory may be initialized or uninitialized | Memory can be initialized or uninitialized |
Allocation Method | Automatic, stack-based allocation | Manual, heap-based allocation |
Deallocation | Automatic deallocation | Manual deallocation using free() function |
Examples | Static variables, global variables | Dynamically allocated memory (e.g., using malloc(), calloc()) |
In summary, static memory allocation occurs at compile time and is suitable for scenarios with fixed memory requirements. In contrast, dynamic memory allocation in C occurs at runtime, providing greater flexibility for variable-sized data structures and precise memory management. However, careful manual memory control is required to avoid memory leaks.
Ways Of Dynamic Memory Allocation In C
A flexible way of managing memory resources, dynamic memory allocation in C enables running applications to adapt to shifting data storage needs. It is facilitated by several techniques, each of which has a particular function. The most popular library functions that can be used for dynamic memory allocation in C include malloc(), calloc(), and free(), realloc(). Programmers can use these functions to change contiguous block sizes, release memory that has been allocated when it is no longer needed, and allocate memory on the heap.
The malloc() Method For Dynamic Memory Allocation In C
The core function for dynamic memory allocation in C is the malloc() method. It is a term from the C standard library that stands for "memory allocation". By enabling applications to request a certain amount of memory from the heap while they are running, this strategy enables them to manage memory resources dynamically. When working with dynamic data structures whose size is uncertain at compile time, the malloc() function enables efficient memory use for variable-sized arrays, linked lists, and other dynamic data structures.
Syntax of malloc() for Dynamic Memory Allocation in C
void* malloc(size_t size);
Here,
- The void keyword indicates that there is no fixed return type for the pointer (void*).
- This along with to the malloc() function returns a void pointer to the allocated memory. This allows you to use the allocated memory for various data types by casting it appropriately.
- The term size_t size is the size parameter that specifies the number of bytes of memory to allocate. It should typically be calculated as the product of the number of elements and the size of each element (e.g., num_elements * sizeof(element_type)).
Let's look at an example C program that demonstrates how to use malloc() for dynamic memory allocation in C.
Code Example:
Output:
Dynamic Array: 0 2 4 6 8
Code Explanation:
In the example C code-
- We first declare a pointer to an integer named dynamicArray inside the main() function. This will be used to store the memory address of the dynamically allocated array.
- Next, we define an integer variable num_elements and initialize it with the value 5, indicating the number of elements we want in the array.
- Then, we use the malloc() function to dynamically allocate memory for an integer array with 5 elements.
- The sizeof() operator determines the memory size in bytes, and the actual size of the memory block allocated is num_elements * sizeof(int) bytes. A pointer to this memory block is stored in dynamicArray.
- As mentioned in code comments, we then check if the memory allocation was successful by verifying if dynamicArray is NULL. If allocation fails, we print an error message and return with an error code (1).
- After that, we initialize the elements of the array using a for loop. Inside the loop-
- The control variable i starts with an initial value of 0, and the loop continues till i<num_elements.
- In every iteration, the element at index position i is set to the value of i multiplied by 2, using the multiplication arithmetic operator.
- After every iteration, the value of i is incremented by 1 before the next iteration begins.
- Next, we use a for loop with the printf() function to print the array elements by iterating through the array and printing each element.
- The printf() statement with the newline escape sequence (\n) is meant to shift the cursor to the next line.
- Finally, we use the free() function to release the dynamically allocated memory to prevent memory leaks.
- Lastly, the main() function terminates with a return 0 statement, indicating successful execution.
The calloc() Method For Dynamic Memory Allocation In C
The calloc() method in C is another important function for dynamic memory allocation, similar to malloc(). It stands for "contiguous allocation" and is also part of the C standard library function. This method is used to allocate memory dynamically during runtime, specifically for arrays or blocks of data. The calloc() function differs from malloc() in that it not only allocates memory but also initializes all the allocated memory to zero. This feature makes it particularly useful for initializing arrays.
Syntax of calloc() for Dynamic Memory Allocation in C
void* calloc(size_t num_elements, size_t element_size);
Here,
- void*: Like malloc(), calloc() returns a void pointer (void*) pointing to the allocated memory, allowing you to cast it for various data types.
- size_t num_elements: This parameter specifies the number of elements to allocate memory for.
- size_t element_size: This parameter specifies the size (in bytes) of each element.
Here's a sample C program that demonstrates how to use calloc() for dynamic memory allocation in C.
Code Example:
Output:
Dynamic Array (Initialized to 0): 0 0 0 0 0
Code Explanation:
In the sample C code-
- Inside the main() function, we declare a pointer to an integer named dynamicArray, which will be used to store the memory address of the dynamically allocated array.
- We then define an integer variable num_elements and set it to 5, indicating the number of elements we want in the array.
- Next, we dynamically allocate memory for the interger array with 5 elements using the calloc() function.
- Inside calloc(), we pass the number of elements and the memory size in bytes of each element (using sizeof() operator) as arguments.
- It hence allocates memory for an array of num_elements elements, each of size sizeof(int), and initializes all the elements to zero. And lastly, the pointer to this memory block is stored in dynamicArray.
- After that, we check if the memory allocation was successful by verifying if dynamicArray is NULL using an if-statement.
- If the statement condition is true, it means the allocation failed, and the program prints an error message and returns with an error code (1).
- If the condition is false, it means allocation was successful, and we move on to print the initialized array elements using a control statement for loop to iterate through the array and printf() function to display each element.
- Following this, we use the free() function to release the dynamically allocated memory to prevent memory leaks.
- Lastly, the program terminates with return 0, indicating no errors in execution.
The free() Method For Dynamic Memory Allocation In C
The free() method is a crucial function for dynamic memory allocation in C programs. It's used to deallocate memory that was previously allocated using functions like malloc(), calloc(), or realloc(). Properly freeing allocated memory is essential to prevent memory leaks, which can lead to inefficient memory usage and instability when running a C program.
Syntax of free() Function for Dynamic Memory Allocation in C
void free(void* pointer);
Here, the void* pointer is the argument taken by the free() function, which is a pointer to the memory that you want to deallocate. This pointer should point to the start of the memory block that was previously allocated dynamically.
Below is a C program example that demonstrates how to use the malloc() function to allocate and the free() function to deallocate dynamically allocated memory in C.
Code Example 1 (Using malloc() and free()):
Output:
Value stored at dynamically allocated memory: 42
Code Explanation:
In the C code sample-
- We begin by declaring an integer pointer with the identifier/ name ptr to store the memory address of the dynamically allocated memory.
- Next, we dynamically allocate memory for an integer using the malloc() function. Here, the sizeof(int) function returns the size in bytes for an integer, ensuring we allocate enough memory for one integer. The pointer to this memory block is stored in ptr.
- After that, we check if the memory allocation was successful by verifying if ptr is NULL. If allocation fails, we print an error message and return with an error code (1).
- If the allocation is successful, we assign the value 42 to the memory location pointed to by ptr using the indirection/ dereference operator (*).
- Next, we use printf() statement to print the value stored in the dynamically allocated memory space, accessing the value through the pointer ptr.
- Finally, we free the dynamically allocated memory using the free() function to prevent memory leaks.
- We can set the pointer ptr to NULL after freeing it, but this is an optional step, as mentioned in the comment.
- This is done to avoid accidental access to the memory after it has been freed. Any attempt to access ptr after freeing it would result in undefined behaviour. Setting it to NULL helps avoid such issues.
The free() function is essential for managing memory resources and preventing memory leaks in C programs. It should be used whenever dynamic memory allocation is employed to ensure efficient memory usage and program stability. Let's take a look at another C example where we use free() along with the calloc() function.
Code Example 2 (Using calloc() and free()):
Output:
Values stored at dynamically allocated memory:
1 2 3 4 5
Code Explanation:
In the example above-
- We declare a pointer to an integer named ptr, to store the memory address of the dynamically allocated memory.
- Then, we use calloc() function to dynamically allocate memory for 5 integers initialized to zero.
- Here, calloc() allocates memory for an array of 5 integers where the size in bytes for one integer is given by the sizeof() operator, i.e., (5 * sizeof(int) bytes).
- The function also initializes all elements of the array to zero and the pointer to this memory block is stored in ptr.
- After that, we use an if-statement to check if the memory allocation was successful by verifying if ptr is NULL. If the condition is true then the allocation has failed, we print an error message and return with an error code (1).
- If the ptr is not NUL (i.e., the condition is true), we move to the next part.
- We use a for loop to initialize the elements of the array by using the pointer ptr. The element at the ith place is assigned the value given by i + 1, where i represents the index of the array.
- The loop continues iteration till i<5, incrementing the value of i by 1 after every iteration.
- Once all the values are assigned, we print the values stored in the dynamically allocated memory using a printf() statement inside another for loop.
- Finally, we release the dynamically allocated memory using the free() function to prevent memory leaks.
How To Deallocate Memory Using free() Function?
Deallocating memory using the free() function is a crucial step in dynamic memory management in C. It ensures that the system releases the memory previously allocated to the heap and can reuse it. Given below is a description of the complete process of how to deallocate memory using free().
Step-by-step explanation of how to use free() function in dynamic memory allocation and deallocation in C:
- Allocate Memory: First, allocate memory on the heap using a function like malloc(), calloc(), or realloc(). This step reserves a block of memory for your data.
- Perform Operations: Use the allocated memory for your data operations. You can read from and write to this memory block as needed.
- Check for Valid Allocation: Before deallocating memory, it's essential to check whether the memory allocation was successful. If the allocation failed (e.g., if malloc() returned NULL), it's not safe to free that memory, as it might not have been allocated to begin with.
- Deallocate Memory: When you are finished using the resources that occupied the allocated memory and it's no longer needed, use the free() function to release the memory. Pass the pointer to the allocated memory as an argument to free().
- Set the Pointer to NULL (Optional): After freeing the memory, it's a good practice to set the pointer to NULL. This ensures that you don't accidentally access or modify the memory after it has been freed, which can lead to undefined behaviour.
How To Deallocate Memory Without Using free() Function?
Deallocating memory without using the free() function in C is not recommended and is generally considered a bad practice. The free() function is the standard and safe way to release dynamically allocated memory, and not using it can lead to memory leaks and program instability. However, for the sake of understanding, let's discuss how memory can be deallocated without the free() function.
Step-by-step process to Deallocate Memory Without free() function:
Deallocating memory without free() involves relying on the operating system to release memory when the program exits. Here's the procedure:
- Allocate Memory: Allocate memory using functions like malloc(), calloc(), or realloc() as usual.
- Perform Operations: Use the allocated memory for data operations.
- Exit the Program: When the program terminates, the operating system automatically reclaims all memory resources, including the dynamically allocated memory.
- Memory Cleanup: If you want to release memory explicitly without using free(), you can set the memory pointer to NULL to prevent further access to it. However, this does not actually deallocate the memory; it merely makes it inaccessible from your program. The memory will still be held by the operating system until the program exits.
Code Example:
Output:
Value allocated using malloc: 42
Code Explanation:
In the code above-
- We declare an integer pointer dynamicInt in the main() function, which we will use to store the memory address of the dynamically allocated memory.
- Then, we use malloc() to dynamically allocate memory for an integer on the heap. The sizeof(int) function returns the size of an integer in bytes, ensuring we allocate enough memory for one integer. The pointer to this memory block is stored in dynamicInt.
- We check if the memory allocation was successful by verifying if dynamicInt is NULL. If allocation failed, we print an error message and return with an error code (1).
- If the allocation was successful, we assign the value 42 to the memory location pointed to by dynamicInt using the dereference operator (*).
- Next, we print the value to whom the memory was allocated using printf() with a formatted string while accessing the value through the pointer dynamicInt.
- Then, we do not deallocate the memory using the free() function in this case. Instead, we set the pointer dynamicInt to NULL to prevent further access to the memory block.
- After that, the program exits, and the operating system automatically releases the memory allocated on the heap when the program terminates.
The realloc() Method For Dynamic Memory Allocation In C
The realloc() method is used to reallocate memory for an existing dynamically allocated block. This function allows you to resize the memory block, either making it larger or smaller, based on your requirements. It is a valuable function when you need to adjust the size of a dynamically allocated memory in C for an array or structure during program execution.
Syntax of realloc() for Dynamic Memory Allocation In C
void *realloc(void *ptr, size_t size);
Here,
- ptr: Pointer to the previously allocated memory block. It can be NULL, in which case realloc() behaves like malloc().
- size: New size (in bytes) of the memory block.
Return Value of realloc() in Dynamic Memory Allocation In C
- If reallocation is successful, realloc() returns a pointer to the newly allocated memory block.
- If reallocation fails, realloc() returns NULL, and the original memory block remains untouched.
Below is an example of a C program that demonstrates how to use realloc() to resize a dynamically allocated array.
Code Example:
Output:
Original Array: 0 10 20
Resized Array: 0 10 20 30 40
Code Explanation:
In the code example-
- In the main() function, we use the malloc() function to dynamically allocate memory for an integer array with 3 elements. The size of the memory block allocated is 3 * sizeof(int) bytes, and a pointer to this memory block is stored in dynamicArray.
- We then check if the memory allocation was successful by verifying if dynamicArray is NULL. If allocation failed, we print an error message and return with an error code (1).
- Otherwise, we use a for loop to initialize array elements setting each at the ith position element to a value calculated as i * 10.
- Next, we print the original array elements using printf(), iterating through the array with a for loop.
- After that, we resize the array to hold 5 elements using the realloc() function. This function changes the size of the memory block pointed to by dynamicArray to 5 * sizeof(int) bytes.
- Once again we use the if-statement to verify if the reallocation of memory was successful by checking if the ptr is equal to NULL.
- If the condition is true, it means the resizing failed; we print an error message and return with an error code (1).
- If the condition is false, then the resizing was successful, and we move to the next part of the program.
- Next, we initialize the additional elements in the resized array using a for a loop, starting from index 3 and setting each element to a value calculated as i * 10.
- Following that, we print the resized array elements using printf(), iterating through the array using a for loop.
- Finally, we free the dynamically allocated memory using the free() function to prevent memory leaks.
- The output shows that the realloc() function was successful in resizing the array and allocating more memory for it.
Allocation and Deallocation of memory using realloc()
Allocation and deallocation of memory using realloc() involve resizing an existing dynamically allocated memory block and managing memory resources efficiently.
1. Allocation using realloc():
When allocating memory using realloc(), you typically have an existing dynamically allocated memory block that you want to resize. The realloc() function takes two parameters: a pointer to the existing memory block and the new size you want to allocate. Here's how it works:
int *ptr = malloc(5 * sizeof(int)); // Allocate memory for 5 integers
// Reallocate memory for 10 integers
ptr = realloc(ptr, 10 * sizeof(int));
In this example, we initially allocate memory for 5 integers using malloc(). Then, we use realloc() to resize the memory block to accommodate 10 integers. If reallocation is successful, realloc() returns a pointer to the newly allocated memory block, which can be assigned back to the original pointer ptr. The original memory block is automatically freed if reallocation is successful. If reallocation fails, realloc() returns NULL, and the original memory block remains intact.
2. Deallocation using realloc():
Deallocation of memory using realloc() is similar to regular deallocation using free(). After resizing the memory block with realloc(), you can still use free() to release the memory when it's no longer needed:
free(ptr); // Free dynamically allocated memory
In the example above, we use free() to release the dynamically allocated memory pointed to by ptr. This step is crucial to prevent memory leaks and efficiently manage memory resources.
Pointers & Dynamic Memory Allocation In C
Pointers play a crucial role in dynamic memory allocation in C by providing a way to access and manage memory that is dynamically allocated from the heap space. When memory is dynamically allocated using functions like malloc, calloc, or realloc, they return a pointer to the allocated memory block. This pointer can then be used to access and manipulate the allocated memory.
Here's how pointers function in dynamic memory allocation in C:
1. Allocation: Dynamic memory allocation in C happens with the use of functions like malloc, calloc, or realloc that return a pointer to the allocated memory block. This pointer can be assigned to a pointer variable for further manipulation.
int *ptr;
ptr = (int *)malloc(sizeof(int));
2. Access and Manipulation: Once memory is allocated, pointers can be used to access and manipulate the allocated memory. For example:
*ptr = 42; // Store a value in the allocated memory
3. Deallocation: After the dynamically allocated memory is no longer needed, it should be deallocated using the free function. The pointer to the allocated memory block should be passed to free.
free(ptr); // Free dynamically allocated memory
Code Example:
Output:
Value stored at dynamically allocated memory: 42
Code Explanation:
In the code-
- Inside the main() function, we declare an integer pointer ptr to store the memory address of an integer variable.
- The memory allocation for this is done dynamically using the malloc() function. It allocates memory of size sizeof(int) (size of an integer) and returns a pointer to the allocated memory block, which we assign to ptr.
- We check if the memory allocation was successful by verifying if ptr is NULL. If allocation failed, we print an error message and return with an error code (1).
- If the allocation was successful, then the integer value 42 is assigned to the memory location pointed to by ptr using the dereference operator (*).
- We print the value stored in the dynamically allocated memory using printf() function, accessing the value through the pointer ptr.
- To prevent memory leaks, we free the dynamically allocated memory using the free() function. This deallocates the memory previously allocated by malloc, making it available for future use.
Malloc() Vs Calloc() Methods Of Dynamic Memory Allocation In C
Both malloc() and calloc() are used for dynamic memory allocation in C, but the choice between them depends on the specific requirements of the program. The table below lists the key differences between the malloc() and calloc() methods for dynamic memory allocation in C:
Aspect |
malloc() |
calloc() |
Functionality |
Allocates a specified number of bytes of memory. |
Allocates a specified number of blocks of memory, each initialized to zero. |
Initialization |
Memory content is uninitialized and contains garbage values. |
Memory content is initialized to zero (all bits are set to 0). |
Arguments |
Takes a single argument: the size (in bytes) of memory to allocate. |
Takes two arguments: the number of blocks to allocate and the size (in bytes) of each block. |
Return Type |
Returns a pointer to the allocated memory block (void pointer). |
Returns a pointer to the allocated memory block (void pointer). |
Example |
c int *arr = (int *)malloc(5 * sizeof(int)); |
c int *arr = (int *)calloc(5, sizeof(int)); |
Use Case |
Typically used when you need block memory allocation but don't require initialization. |
Used when you need memory allocation and want the allocated memory to be initialized to zero, e.g., for arrays and buffers. |
Explicit Initialization |
You must manually initialize the memory if required. |
Memory is already initialized to zero, reducing the need for explicit initialization. |
Performance |
Generally faster because it doesn't involve initializing memory. |
Slightly slower due to the initialization step, but this difference is often negligible. |
Syntax |
void *malloc(size_t size); |
void *calloc(size_t num, size_t size); |
Error Handling |
You need to check if the memory allocation failed (returned NULL) and handle the error accordingly. |
You need to check if the memory allocation failed (returned NULL) and handle it accordingly. |
Common Pitfall |
Not initializing memory, which can lead to undefined behavior if used without proper initialization. |
Assuming that the allocated memory is already initialized, which might not be the case with malloc(). Always verify the initialization when using calloc(). |
Conclusion
Dynamic memory allocation in C is a powerful feature that enables flexible and efficient memory management in programs. By allowing memory to be allocated and deallocated at runtime, dynamic memory allocation facilitates the creation of versatile data structures and improves memory utilization. The article covers essential memory allocation operations, such as malloc, calloc, and realloc, highlighting their role in creating flexible data structures and optimizing resource utilization.
Proper handling of dynamically allocated memory is essential to avoid memory leaks, undefined behaviour, and other memory-related issues. With careful management and adherence to best practices, dynamic memory allocation in C can be used effectively to develop robust and scalable C programs.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is dynamic and static memory allocation in C?
Dynamic memory allocation in C involves allocating memory at runtime from the heap using functions like malloc, calloc, realloc, and free. This allows for flexibility in managing memory as memory to variables can be allocated and deallocated as needed during program execution.
- Dynamic memory allocation in C is particularly useful when the size of data structures or the amount of contiguous memory required is not known at compile time, enabling efficient memory utilization and adaptability to changing program requirements.
- On the other hand, static memory allocation occurs at compile time and involves allocating memory on the stack or as global variables. Memory for statically allocated variables is determined during the compilation phase and remains fixed throughout the program's execution.
- While static allocation provides faster access to memory and can be more straightforward to manage, it lacks the flexibility of dynamic memory allocation in C, as the size of statically allocated memory cannot be changed during runtime.
- Additionally, static allocation may lead to memory wastage if the allocated space is not fully utilized.
Q. What are the four functions used for DMA?
DMA stands for dynamic memory allocation in C and other languages. there are four primary functions that we can use for dynamic memory allocation and deallocation in C programs. These include:
Function Name | Syntax & Purpose |
malloc() function |
|
calloc() function |
|
realloc() function |
|
free() function |
|
Q. What is the limit of malloc() function for dynamic memory allocation in C?
The limit of malloc for dynamic memory allocation in C depends on various factors such as the architecture of the system, the operating system, and available system resources like physical memory (RAM) and virtual memory. Typically, malloc() can allocate memory up to the maximum addressable memory space of the system.
- For 32-bit systems, the theoretical maximum limit is usually around 4 GB, although, in practice, it's often lower due to limitations imposed by the operating system and other system factors.
- For 64-bit systems, the limit is significantly higher, often several terabytes or more, depending on the system architecture and available resources.
Q. How do I handle memory allocation failures in C?
Memory allocation functions (malloc(), calloc(), realloc()) return NULL if memory allocation fails due to insufficient memory. It's essential to check for allocation success by verifying if the returned pointer is not NULL. If allocation fails, appropriate error handling should be performed, such as printing an error message, exiting the program, or freeing previously allocated memory before terminating the program.
Q. What are memory leaks, and how can they be avoided?
Memory leaks occur when memory allocated dynamically is not deallocated properly, leading to a gradual consumption of available memory and eventual program failure. To avoid memory leaks:
- Always free dynamically allocated memory using the free() function when it is no longer needed.
- Ensure that all code paths leading to memory allocation have corresponding deallocation.
- Use tools like valgrind to detect memory leaks during program development and testing.
Q. Can I resize dynamically allocated memory in C?
Yes, you can resize dynamically allocated memory in C using the realloc() function. It resizes a previously allocated memory block to a new size, preserving the existing data if possible. However, resizing memory using realloc() can be costly as it may involve copying data to a new location in memory. It's essential to handle reallocation failures by checking the return value of realloc() for NULL and ensuring that existing data is not lost during resizing.
Q. Why is the purpose of dynamic memory allocation in C?
Dynamic memory allocation in C programming serves several important purposes such as:
- Flexibility in Memory Allocation: Dynamic memory allocation in C enables the creation of data structures whose sizes may vary during program execution, such as linked lists, trees, and dynamic arrays.
- Efficiency in Memory Management: Memory can be allocated and deallocated as needed, optimizing memory usage and improving program performance. This is especially important for large datasets or resource-constrained environments.
- Adaptability and efficient memory sharing: With dynamic memory allocation in C programs can adjust their memory during runtime conditions, enhancing adaptability to changing workloads and resource availability.
Here are a few other C topics you must explore:
- Dangling Pointer In C Language Demystified With Code Explanations
- Pointer Arithmetic In C & Illegal Arithmetic Explained (+Examples)
- Length Of String In C | 7 Methods Explained With Detailed Examples
- Bitwise Operators In C Programming Explained With Code Examples
- Comma Operator In C | Code Examples For Both Separator & Operator
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment