Pointer Arithmetic In C & Illegal Arithmetic Explained (+Examples)
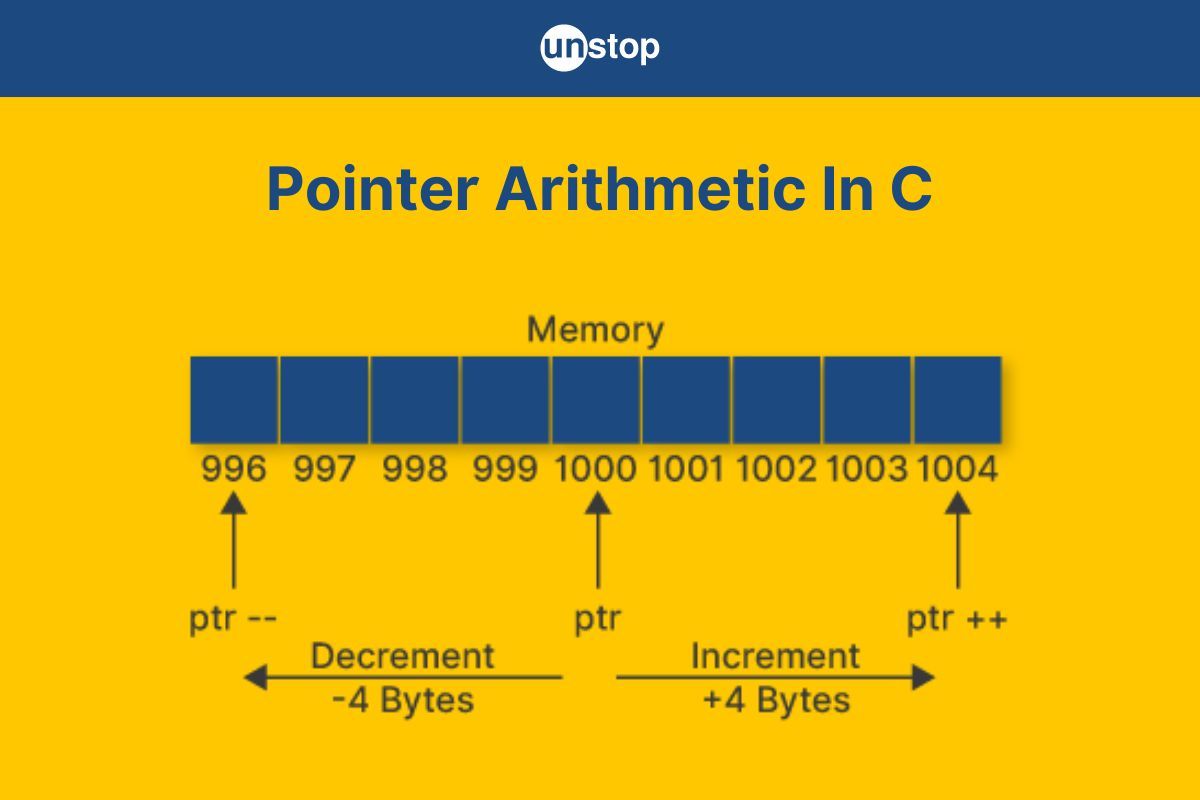
Table of content:
- What Is Pointer Arithmetic In C?
- Incrementing Pointer Arithmetic In C
- Pointer Arithmetic In C | Decrementing Pointers
- Addition Pointer Arithmetic In C
- Subtraction Pointer Arithmetic In C
- Float Pointer & Pointer Arithmetic In C
- Character Pointer & Pointer Arithmetic In C
- Comparison Of Pointers (Same Type) With Pointer Arithmetic In C
- Comparison Pointer Arithmetic In C On Arrays
- NULL Pointers & Comparison Pointer Arithmetic In C
- Rules For Performing Pointer Arithmetic In C
- Illegal Pointer Arithmetic In C
- Pointer Conversions & Pointer Arithmetic In C
- Conclusion
- Frequently Asked Questions
Pointer variables are a fundamental feature in the C programming language, which offers extensive capabilities for enhanced memory management and data manipulation. These variables are designed to store memory addresses of other variables, as opposed to storing their values directly. By utilizing the concept of pointers in programming, you can increase the flexibility and efficiency of your C programs. In this article, we will discuss pointer arithmetic in C language and see how we can perform various arithmetic manipulations on data by using pointers to variables.
What Is Pointer Arithmetic In C?
Pointer arithmetic involves performing arithmetic operations on pointers to navigate through memory. In C, when you perform arithmetic operations on pointers, they are scaled according to the size of the data type to which the pointer points.
For example, consider an array of integers:
int arr[5] = {10, 20, 30, 40, 50};
int *ptr = arr; // Pointer ptr points to the first element of the array
Here, ptr points to the first element of the array arr. When you perform arithmetic operations/ actions on pointers, the result is based on the size of the data type being pointed to or the pointer data type.
Pointer arithmetic in C language allows you to perform multiple mathematical manipulations on pointers, such as addition, subtraction, and comparisons. We will explore all of these one by one in the sections ahead.
Also read: Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
Incrementing Pointer Arithmetic In C
In C, the process of pointer increment involves advancing the memory blocks or address that the pointer stores to the next location of the same data type. The increment operation is accomplished using the increment operator (++) to add an offset to the current memory address.
- The amount of increment performed on a pointer is determined by the size of the data type it is pointing to.
- For instance, incrementing a pointer to an integer (int* ptr) will cause it to advance to the next memory location that can hold an integer value.
- Similarly, incrementing a pointer to a character (char* ptr) will move it to the subsequent memory location intended for characters.
For Example: When incrementing a pointer in C, the amount of increment depends on the size of the data type the pointer is pointing to. For instance, if an integer pointer holds the memory address 2050 and is incremented, it will move forward by the size of an integer (typically 4 bytes on most systems). Consequently, the new address it points to will be 2054. Similarly, if a float type pointer is incremented, it will also advance by the size of a float (usually 4 bytes) and result in the new address being 2054.
Syntax Of Incremental Pointer Arithmetic In C
ptr++;
Here, the pointer variable is given by ptr, and the double plus sign (++) represents the increment operator. Let's look at an example showcasing the implementation of incremental pointer arithmetic in C.
Code Example:
Output:
Elements of the array: 10 20 30 40 50
Explanation:
In the simple C program, we first include the essential header files, here <stdio.h> for I/O operations.
- In the main() function, which is the entry point for the program's execution, we declare an integer array called numbers and initialize it with 5 elements (10, 20, 30, 40, 50).
- Next, we create an integer pointer ptr and initialize it with the array numbers. This leads to the pointer pointing to the 1st element of the array.
- We then use a for loop to iterate through the array and print all elements using increment pointer arithmetic operation. The loop starts with the control variable i set to 0 and goes on till i<5.
- In every iteration, the printf() statement inside the loop prints the value being pointed to by the pointer. We use the dereference operator to access the locations via pointers, and the %d format specifier refers to the integer value.
- After the value is printed, the pointer is incremented by 1 to move it to the next element. That is, we perform increment arithmetic on the pointer so that it points to the next element in the array.
- This way, the loop prints all the elements of the array before terminating.
- Lastly, the main() function completes execution successfully, without any errors by returning a value of 0.
Pointer Arithmetic In C | Decrementing Pointers
In C, when we decrement pointers, we are basically shifting the pointer to the preceding memory location, which holds a value of the same data type. The decrement operation is performed by subtracting an offset from the current memory address stored in the pointer variable using the decrement operator.
- The decremented amount applied to a pointer depends on the size of the data type it is pointing to.
- For instance, if you have an integer pointer (int* ptr), decrementing it (ptr--) will shift it to the previous address or memory location designed to hold an integer.
For Example: If an integer pointer with the memory address 2050 is decremented, then the address will be decremented by 4 which is the size of an int type. So, this results in a new address pointing to 2046. Similarly, if a float pointer is decremented, it will also decrement by 4 (the size of a float), leading to a new address of 2046.
Syntax Of Decrementing Pointer Arithmetic In C
ptr--;
Here, ptr is the identifier/ name for the pointer variable, and the double minus sign (--) signifies the decrement operator. Below is an example showcasing the implementation of the decrement pointer arithmetic in C.
Code Example:
Output:
Elements of the array in reverse order: 50 40 30 20 10
Code Explanation:
In the sample C program-
- We first declare an integer array numbers containing five elements that are separated by a comma inside the initializer list.
- Next, we initialize a pointer ptr to point to the last element of the array using the address of operator (&) and the index value 4, i.e., int* ptr = &numbers[4];
- Then, we use a for loop to iterate through the array starting at index value 4 until i>=0.
- In every iteration, the printf() function inside the loop displays the value at the respective index value using the dereference operator to get access to memory addresses.
- After printing the value, we decrement the pointer, i.e., ptr--, to move it to the previous element of the array. That is, the addresses of the pointer are iteratively decremented by 1 until we reach the element at index 0.
- As a result, the loop prints the elements of the array in reverse order.
- Lastly, the return 0 statement then signals that the program has run its course successfully by returning a value of 0.
Addition Pointer Arithmetic In C
Pointer addition refers to the process of adding an offset value to a pointer, which shifts the pointer ahead/ forward. In other words, it shifts the pointer to point to the address of memory location, which is ahead of the previous location, by the value of the offset. This operation, known as addition pointer arithmetic in C, enables efficient navigation through memory by taking into account the size of the data type the pointer points to.
- By adding an integer value to a pointer, the pointer is incremented by the appropriate number of bytes in memory based on the size of the underlying data type.
- Consequently, you can access elements within an array or traverse a data structure in a sequential manner by manipulating pointers.
Syntax Of Addition Pointer Arithmetic In C
pointer_variable + integer_value
Here, the pointer_variable refers to the pointer we declared, and the integer_value is the offset/ integer value that we want to add to the pointer.
Code Example:
Output:
Value at index 2: 30
Code Explanation:
In the C code example-
- We have an array of integers array and a pointer ptr that initially points to the first element of the array.
- We then define an integer variable offset and set it to 2. This value represents the number of elements to skip in the array.
- By adding the offset to the pointer ptr using the addition arithmetic operator (pointer + offset), we obtain a new pointer newPointer that points to the element at the adjusted index position in the array.
- We can then dereference newPointer (*newPointer) to retrieve the value at the adjusted position. In this example, it will be the 3rd element, i.e., element at index 2 of the array.
- Finally, we print the result using the printf() function, which displays the value at the adjusted index position in the array. The newline escape sequence in the formatted string shifts the cursor to the next line.
- The main() terminates with a return 0 statement.
Important Note: Keep in mind that an integer variable occupies 2-byte memory space in 32-bit OS. So, in this case, offsetting the pointer by 1 will lead to an actual memory address change of 2 bytes. In the 64-bit OS, an integer value occupies 4-bytes, so an offset by 1 value will shift the pointer to adjacent memory location, but the shift of space in bytes will be 4. This applies to both addition and subtraction pointer arithmetic in C.
Subtraction Pointer Arithmetic In C
Pointer subtraction in C involves subtracting two pointers to calculate the difference in memory addresses between them. This operation is a form of pointer arithmetic in C that enables you to determine the distance or offset between two memory locations.
When you subtract one pointer from another, the resulting value represents the number of elements or objects between the two pointers, accounting for the size of the data type associated with the pointers.
Syntax For Subtraction Pointer Arithmetic In C:
pointer1 - pointer2
Here, pointer1 and pointer 2 refer to the two pointer variables we have, and the minus sign (-) represents the subtraction arithmetic operator.
Code Example:
Output:
Pointer difference: 2
Explanation:
In the example C code-
- We first declare an initialize and integer of arrays, array with 5 elements.
- Then, we create two pointers, pointer1 and pointer2, that point to the 1st element (at index 0) and the 3rd element (at index 2) of the array.
- As mentioned in the code comments, we then calculate the difference between the two pointers by performing subtraction pointer arithmetic.
- We subtract pointer2 from pointer1 (pointer1 - pointer2), and we obtain the difference the two pointers (or distances between elements at those positions) taking into account the size of the pointer data type.
- In other words, the outcome represents the number of elements between the two pointers, and it is stored in the pointerDifference variable.
- We display the value to the console using printf(). The %td format specifier corresponds to the ptrdiff_t type, which is the signed integer type suitable for representing pointer differences.
- Lastly, the program terminates with return 0, indicating successful execution.
Subtracting Integer From Pointer | Subtraction Pointer Arithmetic In C
Subtraction of integer value from a pointer is also a form of subtraction pointer arithmetic in C. This operation involves adjusting the memory address stored in the pointer variable by subtracting a calculated offset based on the size of the data type the pointer points to.
When you subtract an integer value from a pointer, the resulting memory address is determined by the size of the data type associated with the pointer. The integer value is scaled by the size of the data type, and the resulting offset is subtracted from the original memory address.
Syntax For Subtracting Integer From Pointer
pointer_variable - integer_value
Here, the pointer_variable represents the pointer that we declared, and integer_value is the offset value that is to be subtracted from the pointer variable.
Code Example:
Output:
Value at index 4: 30
Explanation:
In the sample C code-
- We create an array of integers called array with 5 elements.
- Then, we create an integer pointer variable pointer initialized to point to the 5th element (last) of the array using the address-of/ reference operator.
- Next, we declare an integer variable offset, which we will subtract from the variable pointer and initialize it with the value 2.
- After that, we subtract an integer value from the pointer using the subtraction operator, i.e., pointer - offset. The outcome of this subtraction is stored in the newPointer pointer variable that points to the element at the adjusted position in the array.
- We then dereference newPointer using the dereference operator (*newPointer) to retrieve the value at the adjusted position. In this case, this is the element at the 2nd index, i.e., the 3rd element.
- Following that, we display the element at the adjusted position and the index value using the printf() function. To determine the index of the adjusted position relative to the start of the array, we use the expression (pointer - array) in the formatted string.
Float Pointer & Pointer Arithmetic In C
A float pointer is a variable that can hold the memory address of a float type variable. It enables indirect access and manipulation of the float variable by providing a means to reference its memory location. By using a float type pointer, you can perform operations on the float variable through its address rather than directly accessing its value.
- Pointer arithmetic in C on a float pointer allows you to perform mathematical operations on the pointer itself, taking into account the size of the data type it points to (in this case, float).
- When performing pointer arithmetic on a float pointer, the result is adjusted according to the size of a float, typically 4 bytes.
- For example, adding 1 to the original pointer of float type will move it forward by the size of a float (4 bytes). Similarly, subtraction 1 will move it backwards by the same size.
- This feature is particularly useful when working with arrays or blocks of memory allocated dynamically, as it allows you to access and manipulate elements in a contiguous sequence.
Syntax For Pointer Arithmetic In C On Float Type Pointer
ptr = ptr + N; // Move the pointer N elements forward
ptr = ptr - N; // Move the pointer N elements backward
Here, the identifier ptr refers to the pointer variable we will perform the pointer arithmetic on and the term N refers to the integer value representing the number of elements by which we will move the pointer. Look at the example below to understand how to perform pointer arithmetic in C on a float pointer.
Code Example:
Output:
Value at initial pointer position: 1.1
Value after moving pointer forward: 3.3
Value after moving pointer backward: 2.2
Code Explanation:
In the C code sample-
- We declare a float array called arr with five elements, i.e., float values: 1.1, 2.2, 3.3, 4.4, and 5.5.
- Then, we declare a float pointer ptr and initialize with the array arr, effectively the address of the first element of arr array.
- Next, we use the printf() function with a formatted string to display the initial being pointed to by the float pointer. This is achieved by dereferencing the pointer using *ptr.
- After that, we perform pointer arithmetic to move the pointer two elements forward by adding integer value 2 to it, i.e., ptr = ptr + 2. This shits the pointer to the 3rd element of arr array.
- Once again, using printf(), we display the value being pointed to after moving it forward.
- Continuing the pointer arithmetic, we move the pointer one element backward by subtracting 1 from it, i.e., ptr = ptr - 1. This brings the pointer backward to the 2nd element of the array.
- Again, we print the value at the new pointer position after moving it backwards.
Character Pointer & Pointer Arithmetic In C
In C, a character pointer is a variable that is specifically designed to store the memory address of a character or a sequence of characters, also known as a string. It allows us to indirectly access and manipulate textual data by holding the address where the character(s) are stored in memory.
Character pointers are commonly used to work with strings, as strings in C are represented as arrays of characters. By assigning the address of the first character of a string to a character pointer, we can easily traverse the string and perform operations on its individual characters. The declaration of a character pointer in C uses the asterisk (*) symbol to indicate that the variable is a pointer.
Syntax For Pointer Arithmetic In C On Character Pointer
ptr = ptr + N; // Move the pointer N bytes forward
ptr = ptr - N; // Move the pointer N bytes backward
The syntax is the same as that in the case of a float pointer, where-
- The term ptr represents the pointer, but here, the pointer type is character.
- N represents the integer value that we use to offset the pointer.
- The addition (+) and subtraction (-) operators determine if we will deduct or add the offset to the pointer.
Code Example:
Output:
Initial pointer position: 0x7ffd6b8be6ac
Pointer position after moving forward: 0x7ffd6b8be6ae
Pointer position after moving backward: 0x7ffd6b8be6ad
Explanation:
In the C program sample-
- We start by declaring a character array called str with the string value- "Hello" using the double quotes method.
- Next, we declare a character pointer named ptr and assign the character array str to it. As a result, the ptr points to the address of the first character of the string.
- Then, using the printf() function, we display the initial position of the pointer ptr. Here, the %p format specifier represents the memory address of a variable.
- After that, we perform addition pointer arithmetic by adding 2 to the pointer, i.e., ptr = ptr + 2. This moves the pointer two characters forward in the string.
- Again, using printf(), we display the new position of the pointer after moving it ahead by 2.
- Continuing with pointer arithmetic, we subtract 1 from the pointer, i.e., ptr = ptr - 1. This moves the pointer one character backwards in the string.
- Similar to before, we print the address stored in the pointer after adjustment to the console using printf().
Comparison Of Pointers (Same Type) With Pointer Arithmetic In C
We can perform pointer comparisons on the pointers of the same data type in programming languages like C or C++. This part usually involves comparing the memory addresses stored in the pointers. Here are a few key factors to bear in mind when comparing pointers using principles of pointer arithmetic in C:
- Memory addresses: Pointers store memory addresses and the comparison revolves around determining if they point to the same memory location.
- Equality (==): If two pointers hold the same memory address, they are considered equal, indicating that they refer to the same object or memory location.
- Inequality (!=): Pointers are deemed unequal if their memory addresses differ, suggesting that they point to different objects or memory locations.
- Relative ordering: Pointers can be compared for relative ordering using greater-than (>) or less-than (<) relational operators. This comparison relies on the memory addresses rather than the values stored at those addresses.
- Pointer arithmetic: Pointers of the same type can undergo arithmetic operations like addition and subtraction, adjusting the pointer based on the size of the underlying type.
These comparison operations and arithmetic capabilities have significant implications in a wide range of programming scenarios. They are particularly crucial in areas such as memory management features, data manipulation, sorting algorithms, linked data structures, and low-level system programming.
- By utilizing these features, developers gain control over and comprehension of how pointers interact with memory.
- This understanding enables them to achieve efficient and precise memory allocation, deallocation, and data traversal.
- These capabilities are pivotal for optimizing resource utilization, enhancing program performance, and implementing complex data structures and algorithms.
The example below shows how to run a C program that performs comparison operations on pointers.
Code Example:
Output:
ptr1 and ptr3 are equal.
ptr1 and ptr2 are not equal.
ptr1 is less than ptr2.
Code Explanation:
In the C example-
- We initialize two variables of integer data type, num1 and num2, with values 10 and 20, respectively.
- Next, we declare three integer pointer variables, ptr1, ptr2, and ptr3 and assign them the addresses of variables num1, num2, and num1, respectively.
- After that, we use three if-else conditional statements with relational operators to compare these pointers.
- First, we check if ptr1 is equal to ptr3, i.e., ptr1 == ptr3. Since both of these point to the same variable num1, this comparison evaluates to true. So, the printf() statement inside the if-block is executed.
- Second, we check if ptr1 is not equal to ptr2, i.e., ptr1 != ptr2. Since they point to different variables, this comparison evaluates to true. As a result, the printf() statement inside the if block executes.
- Finally, we compare the relative ordering of ptr1 and ptr2, i.e., ptr1 < ptr2. Since ptr1 points to num1, which comes before num2 in the context of memory addresses/ space, this comparison evaluates to true. So, the code inside the if-block is executed.
- Lastly, the program terminates with return 0, indicating successful execution to the OS.
Comparison Pointer Arithmetic In C On Arrays
An array is a fundamental data structure in computer programming, providing a systematic way to organize and access a set of elements of the same data type. It stores these elements in contiguous memory locations, assigning each element a unique index representing its position within the array.
In programming languages like C or C++, comparison operators can be used on pointers to compare array elements. Here's how comparison pointer arithmetic works in reference to pointers to arrays:
- Equality (==): You can compare two pointers to determine if they point to the same location in memory, indicating they refer to the same array or element within the array.
- Inequality (!=): The inequality operator can be used to check if two pointers point to different memory locations.
- Relative ordering: Pointers to elements of an array can be compared using the less-than (<) and greater-than (>) operators to determine their relative positions in the array. The comparison is based on the memory addresses of the elements.
Code Example:
Output:
The second element comes before the fourth element in the array.
Explanation:
In the C code-
- We create an integer array named arr with four integer values, i.e., {5, 2, 8, 3}.
- Then, we declare two integer type pointer variables, ptr1 and ptr2, and assign them the addresses of the 2nd element (index 1) and the 4th element (index 3) of the array.
- After that, we use an if-else statement to perform a relative ordering comparison on them. We check if the address in ptr1 is less than the address in ptr2.
- If the condition is true, then the if block is executed, otherwise the code inside else-block is executed.
- Since the address of the second element (ptr1) is lower than the address of the fourth element (ptr2) in memory, the condition ptr1 < ptr2 evaluates to true.
- Therefore, the printf() statement inside the if block prints the string message- 'The second element comes before the fourth element in the array.' to the console.
NULL Pointers & Comparison Pointer Arithmetic In C
A Null pointer is one that does not point to anything. They can also be referred to as uninitialized pointers. In C or C++, comparing pointers to NULL is a common approach to verify whether a pointer is referencing a null address. It is commonly used to represent a null pointer.
- Equality (==): The equality operator (==) is employed to carry out this comparison. If the pointer is indeed pointing to a null address, the comparison will yield a true result.
- Inequality (!=): The inequality operator (!=) can be used to compare a pointer to NULL to check if it is not null. If the pointer is pointing to a non-null address, the comparison will evaluate to true.
Comparing pointers to NULL serves the purpose of assessing the validity of a pointer, indicating whether it points to a valid memory address or if it is a null pointer (pointing to no memory address/ invalid memory location). This comparison is crucial in error checking, proper memory utilization/ allocation, and ensuring safe pointer dereferencing.
Code Example:
Output:
Pointer is null.
Code Explanation:
In the code above-
- We declare a pointer variable named ptr that can hold the address of an integer variable. Initially, we set it to NULL, meaning it doesn't point to any valid memory location.
- Next, we use an if-else statement to check if the pointer ptr is NULL, i.e., ptr == NULL. If the condition is true, the if-blockis executed, if not then else-block is executed.
- Since ptr is indeed NULL, the printf() statement inside the if block prints the message- 'Pointer is null.' to the console.
Rules For Performing Pointer Arithmetic In C
Performing pointer arithmetic in C involves adhering to several rules to ensure correct behaviour and avoid undefined behaviour. Here are the key rules for performing pointer arithmetic in C:
- Pointer Arithmetic is based on the size of the Pointed-to Type: When you perform pointer arithmetic in C, the pointer is incremented or decremented by a value equivalent to the size of the data type it points to. For example, if a pointer points to an integer, incrementing the pointer by one moves it to the next integer memory location.
- Valid Operations on Pointers: You can perform the following operations on pointers:
- Incrementing (ptr++ or ptr += n): Moves the pointer forward by n elements (where n is the size of the pointed-to type).
- Decrementing (ptr-- or ptr -= n): Moves the pointer backwards by n elements.
- Addition (ptr + n): Moves the pointer forward by n elements without modifying the original pointer.
- Subtraction (ptr - n): Moves the pointer backwards by n elements without modifying the original pointer.
- Pointer difference (ptr2 - ptr1): Calculates the difference between two pointers, giving the number of elements between them.
- Pointer Arithmetic is Limited to Elements within the Same Array or Allocation: Pointer arithmetic in C should only be performed within the bounds of the memory block allocated to the array or dynamically allocated memory. Attempting to access memory beyond these bounds leads to undefined behaviour.
- Arithmetic with Void Pointers is Invalid: Void pointers (void*) cannot be used for pointer arithmetic directly. They need to be cast to a specific type of pointer before performing arithmetic.
- Pointer Arithmetic with Function Pointers: Arithmetic with function pointers is invalid because there's no concept of incrementing or decrementing addresses of functions in C.
- Overflow and Underflow: Pointer arithmetic in C must account for potential overflow or underflow situations, especially when dealing with large data structures or dynamic memory allocation. Overflowing or underflowing a pointer leads to undefined behaviour.
- Alignment Considerations: Pointer arithmetic in C should consider the alignment requirements of the pointed-to data type, especially when dealing with structures or dynamically allocated memory. Misaligned accesses may lead to performance penalties or undefined behaviour on some architectures.
- Portability: Pointer arithmetic in C should be done with consideration for portability across different systems and compilers. Different architectures may have different alignment requirements and behaviour regarding pointer arithmetic.
Illegal Pointer Arithmetic In C
There a few operations/ manipulations which cannot be performed on pointers for because these variables hold memory addresses and not actual data values. These operations are collectively referred to as illegal/ improper pointer arithmetic in C. They violate the rules and principles of pointer arithmetic, leading to undefined behaviour. These illegal operations can result in program crashes, memory corruption, or unpredictable outcomes. Here are some examples of illegal pointer arithmetic in C:
Operation |
Legality |
Example |
Adding two addresses together |
Illegal |
ptr1 + ptr2 |
Multiplying two addresses |
Illegal |
ptr1 * ptr2 |
Taking the modulus of two addresses |
Illegal |
ptr1 % ptr2 |
Dividing two addresses |
Illegal |
ptr1 / ptr2 |
Performing a bitwise AND operation on two addresses |
Illegal |
ptr1 & ptr2 |
Performing a bitwise XOR operation on two addresses |
Illegal |
ptr1 ^ ptr2 |
Performing a bitwise OR operation on two addresses |
Illegal |
ptr1 | ptr2 |
Applying the bitwise complement operator (~) to an address |
Illegal |
~ ptr1 |
Now, let's try to implement one of these operations and check what is the result of the manipulation of the pointer value.
Code Example:
Output:
error: invalid operands to binary * (have 'int *' and 'int')
Code Explanation:
In the C program example-
- We first declare and initialize an integer array arr with five elements: 10, 20, 30, 40, and 50.
- Next, we declare a pointer variable ptr of type integer pointer (int*) and initialize it with the array. This results in the pointer pointing to the first element of the array (at index 0).
- After that, we attempt illegal arithmetic by multiplying the pointer ptr by 2, which is not allowed in C.
- The compiler detects the illegal operation and raises a compilation error, i.e., error: invalid operands to binary * (have 'int *' and 'int'), as shown in the output.
Note: This error occurs because pointer arithmetic in C only permits addition, subtraction increment, decrement and comparisons. Other arithmetic operations like multiplication are not allowed.
Pointer Conversions & Pointer Arithmetic In C
When performing pointer arithmetic in C, you might come across two pointer variables of different data types. In such a case, you have to convert both pointers to the same data type before performing manipulations on them.
This is where type conversions come into play. It refers to the process of converting one type of pointer into another type. C allows various conversions between pointers, which can be implicit or explicit, depending on the situation. These conversions are governed by certain rules to ensure type safety and compatibility.
Implicit Conversions
Implicit conversions occur automatically by the compiler when it encounters expressions involving different data types. These conversions are performed to ensure compatibility and maintain type safety.
For example, in arithmetic expressions involving different data types, the operands are implicitly converted to a common type.
int num = 10;
float fnum = 3.5;
float result;
result = num + fnum; // Implicit conversion of 'num' to float
Explicit Conversions
Explicit conversions, also known as typecasts, are performed explicitly by the programmer using the cast operator (type). These conversions override the default behaviour of the compiler and are sometimes necessary when dealing with incompatible data types.
For example, we can explicitly convert a function pointer from one type to another as follows:
int add(int a, int b) {
return a + b;
}
float (*fptr)(float, float);
fptr = (float (*)(float, float))add; // Explicit conversion from int(*)(int, int) to float(*)(float, float)
Conclusion
In conclusion, pointer arithmetic in C is a powerful feature that allows developers to manipulate memory addresses efficiently. Throughout this article, we have explored various aspects of pointer arithmetic, including incrementing and decrementing pointers, pointer addition and subtraction, comparison of pointers, and arithmetic on different pointer types, such as float and character pointers.
Understanding pointer arithmetic is crucial for efficient memory management and advanced programming techniques in C. Developers can optimize their code for better performance and more concise logic by mastering these concepts.
However, it's important to note that pointer arithmetic should be used with caution. Illegal arithmetic operations with pointers can lead to undefined behaviour and potentially serious bugs in the code. Developers must adhere to the rules for performing pointer arithmetic and avoid illegal conversions to maintain program correctness and stability.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. Why is pointer arithmetic useful?
Pointer arithmetic in C/ C++ is a fundamental concept that has numerous advantages and serves various purposes, making it an invaluable asset for developers. Here are key reasons why we need to use pointer arithmetic in C:
- Efficient array manipulation: Pointer arithmetic in C facilitates efficient memory traversal, and it is a flexible memory manipulation tool, especially for data structures like arrays.
- Dynamic memory allocation: Pointer arithmetic is crucial in part of dynamic memory management.
- Efficient data structures: Pointer arithmetic in C enables the creation and management of complex/ complicated data structures like linked lists, trees, and graphs.
- Working with complex data types: Pointer arithmetic in C simplifies the process of accessing and manipulating individual elements.
- File handling: When reading from or writing to files, pointer arithmetic can efficiently manage data buffers and process large datasets without the need for intermediate storage.
Q. What are the different types of pointers in C?
In C, there are several types of pointers that serve various purposes and allow programmers to manipulate data efficiently. The different types of pointers in C include:
- Null Pointer
- Wild Pointer
- Dangling Pointer
- Void Pointer (void*)
- Integer Pointer, i.e., pointer to integer type (int*)
- Character Pointer, i.e., pointer to char type (char*)
- Float Type Pointer, i.e., pointer to float type (float*)
- Pointer to double type (double*)
- Pointer Constant
- Pointer to array (int (*arr)[SIZE])
- Function Pointer, i.e, a pointer to function (int (*func)())
- Double pointer, i.e., pointer to pointer (int** or int* *)
Q. What is the size of a pointer arithmetic in C?
The size of a pointer arithmetic operation in C depends on the data type of the pointer (effectively, the type of data it is pointing to) and the specific architecture of the system.
In C, when you perform pointer arithmetic, the compiler automatically scales the offset based on the size of the data type that the pointer points to. For example, if you have an int* pointer, incrementing or decrementing it by 1 would move it by the size of an integer. The actual size of the pointer arithmetic operation is determined by the sizeof() operator, which gives the size of the data type the pointer points to.
Q. What are the limitations of pointer arithmetic in C?
Pointer arithmetic in C provides powerful capabilities, but it also comes with certain limitations and potential pitfalls that programmers need to be aware of.
- Pointer arithmetic does not perform automatic bound checks.
- You cannot directly perform pointer arithmetic with void pointers because they lack a specific data type size.
- Incorrectly using pointer arithmetic in C, with pointers of different types, can lead to unintended results or memory access errors such as unauthorized memory access, incorrect memory access, etc.
- Pointer comparison is only valid within the array elements of the same array or allocated block of memory.
- The behaviour of pointer arithmetic might vary between different platforms or compilers, especially in cases of pointer overflow or underflow.
Q. What is the pointer structure in C?
In C, a pointer to a structure, also known as a pointer to struct, is a special type of pointer that stores the memory address of a structure variable. It allows you to indirectly access and manipulate the members of the structure using the pointer. The syntax to declare a pointer to a structure is similar to declaring a pointer to any other data type.
Syntax:
struct MyStruct {
// Members of the structure
};
// Declare a pointer to the struct
struct MyStruct* ptr;
Here,
- MyStruct: This is the structure we declared
- Ptr: This is the pointer we declared
Q. What is the difference between ++ ptr and ptr ++ in C?
Feature | ++ptr | ptr++ |
---|---|---|
Operation | Pre-increment | Post-increment |
Meaning | Increments ptr by 1 | Increments ptr by 1 |
Result | Value of ptr after increment | Value of ptr before increment |
Side Effect | ptr is incremented before its value is used | ptr is incremented after its value is used |
Expression | ++ptr returns the value of ptr after the increment | ptr++ returns the value of ptr before increment |
Usage | Commonly used in expressions where the incremented value is immediately required. | Commonly used when the original value of the pointer is required before incrementing. |
Q. What are the contents of the pointer variable in C?
Unlike regular variables that store data values, a pointer variable is designed to store the address of another variable data, more specifically, its location. This characteristic makes pointers a potent tool for indirect access and manipulation of data.
In other words, rather than directly holding the actual value, a pointer serves as a reference to the memory location where the data resides. The memory address represented by the pointer is crucial as it enables the program to locate and interact with the data indirectly. Pointer arithmetic in C is an essential concept that defines how we can perform manipulations on pointers.
Read the following to enhance your understanding of other key C concepts:
- Array Of Pointers In C & Dereferencing With Detailed Examples
- Control Statements In C | The Beginner's Guide (With Examples)
- Logical Operators In C (AND, OR, NOT, XOR) With Code Examples
- Keywords In C Language | Properties, Usage, Examples & Detailed Explanation!
- Ternary (Conditional) Operator In C Explained With Code Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment