Table of content:
- What Is A Structure In C Programming?
- Syntax For Defining Structure In C
- How To Declare A Member/ Variable Of Structure In C?
- How To Initialize Members Of Structure In C?
- Accessing Members Of Structure In C
- Copying Member Values Of Structure In C
- Modifying Variable Values Of Structure In C
- Memory Allocation For Structure In C
- Structure In C As Function Arguments
- Typedef & Structure In C
- What Is An Array Of Structures In C?
- Nested Structures In C
- What Is Pointer To Structure In C (Structure Pointer)?
- Self-Referential Structure In C
- What Is Member Alignment For Structure In C?
- Padding & Packing For Structure In C
- Bit Fields & Structure In C
- Why Use Structure?
- Disadvantages of Structure in C Programming
- Conclusion
- Frequently Asked Questions
Structure In C | Create, Access, Modify & More (+Code Examples)
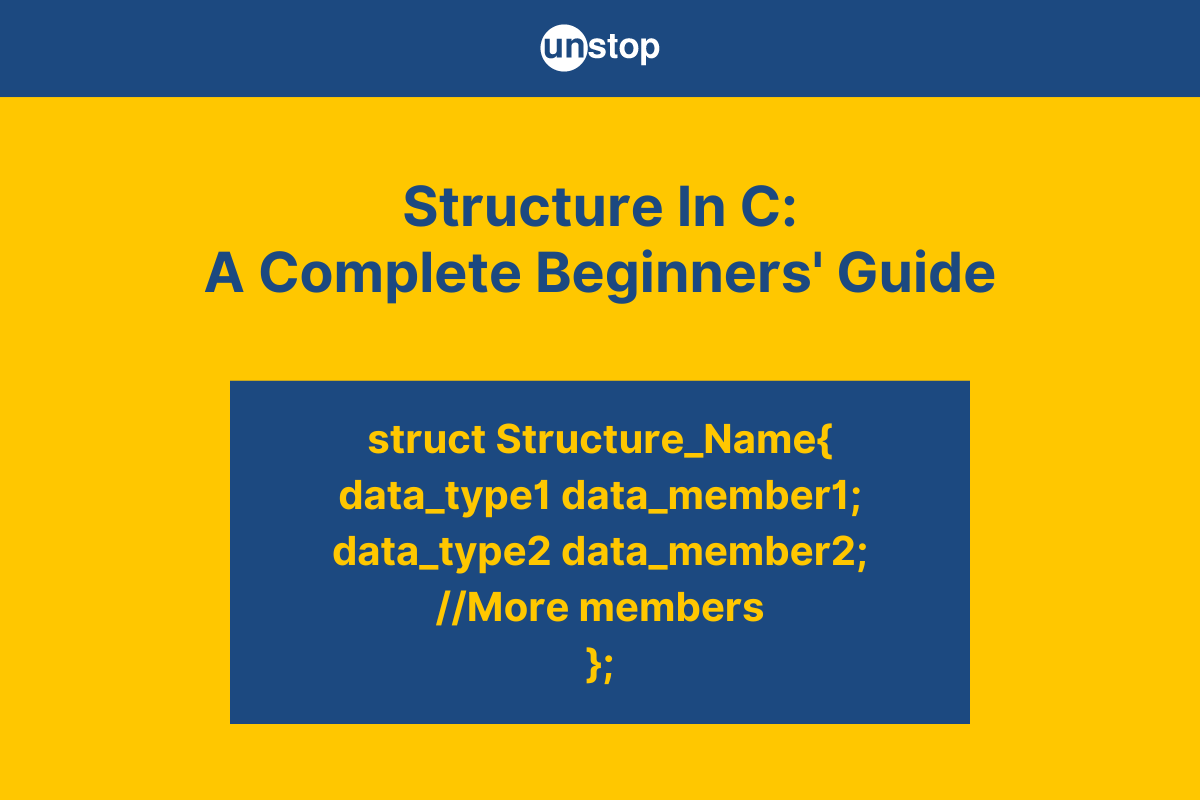
As the name suggests, a structure in C programming is a type of data structure or a user-defined type used to store data. It is a powerful tool that, unlike other data structures, permits you to store data of different data types in a single name.
In this article, we'll deep dive into the concept of structures in C, exploring their syntax, usage, applications and best practices.
What Is A Structure In C Programming?
A structure in C is a user-defined data type that allows programmers to group together variables of different data types under a single name. The structure template is used for creating composite data types that represent entities with multiple attributes.
A structure in C is defined using the struct keyword followed by the structure's name. Inside curly braces {}, member variables, also known as fields, are declared. These fields can be of different data types and represent the attributes of the structure.
Real-life Analogy: We can explain the concept of structure by considering a real-life scenario of representing a student's information. A structure named can store this information, where each information head will be a structure member, including name, roll number, age, and GPA.
Syntax For Defining Structure In C
The syntax of structures in C language consists of the struct keyword followed by the structure's name, enclosed in curly braces {}. Inside the braces, member variables, also known as fields, are declared, each with its own data type.
struct StructureName {
dataType1 member1;
dataType2 member2;
// Additional members
};
Here,
- The struct keyword defines a structure with the name/ identifier given by StrcutureName.
- The terms dataType and member refer to the data type and the name of a structure member.
- Curly braces {} enclose the components of a structure in C.
Below is a simple C program example showcasing how to create a structure and initialize a member for access. We will discuss the ways of declaring, initialising and accessing the members of a structure in C in the later sections.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgdG8gcmVwcmVzZW50IGEgc3R1ZGVudApzdHJ1Y3QgU3R1ZGVudCB7CmNoYXIgbmFtZVs1MF07CmludCByb2xsTnVtYmVyOwpmbG9hdCBtYXJrczsKfTsKCmludCBtYWluKCkgewoKLy8gRGVjbGFyaW5nIGFuZCBpbml0aWFsaXppbmcgYSBzdHJ1Y3R1cmUgdmFyaWFibGUgb2YgdHlwZSBTdHVkZW50CnN0cnVjdCBTdHVkZW50IHN0dWRlbnQxID0geyJBa2Fua3NoYSIsIDEwMSwgOTUuNX07CgovLyBEaXNwbGF5aW5nIHRoZSBzdHVkZW50J3MgaW5mb3JtYXRpb24KcHJpbnRmKCIlcyB3aXRoIHJvbGwgbnVtYmVyICVkIHNjb3JlZCAlLjJmIG1hcmtzLiIsIHN0dWRlbnQxLm5hbWUsIHN0dWRlbnQxLnJvbGxOdW1iZXIsIHN0dWRlbnQxLm1hcmtzKTsKCnJldHVybiAwOwp9
Output:
Akanksha, with roll number 101, scored 95.50 marks.
Explanation:
In the simple C code example, we first include the header file <stdio.h> for input/ output operations.
- We then define a structure named Student using the keyword struct. This structure contains three members-
- We have a character array/ string variable called name representing the student's name. This array can hold up to 50 characters.
- Then we have rollNumber (integer data type) and marks (floating-point), representing the roll number and marks of a student, respectively.
- In the main() function, as mentioned in the code comments, we declare and initialize a variable student1 of type Student, effectively creating an instance of the Student structure.
- We initialize the name member with string Akansha, rollNumber with 101, and marks with 95.5.
- Next, we use a printf() statement to display the information with a descriptive formatted string.
- Here, the %s, %d, and %.2f format specifiers indicate string, integer and floating-point values, respectively.
- Finally, the main function terminates with a return 0 statement indicating successful execution.
How To Declare A Member/ Variable Of Structure In C?
There are primarily two ways in which we can declare a structure variable in C programming. We have discussed both in this section.
Method 1: Declare Structure Variable With Structure Definition
In this method, you define the structure and declare structure variables simultaneously. The structure definition is placed before the main function or at a global scope, and structure variables are declared immediately after the structure definition.
Syntax:
struct StructureName {
dataType1 member1;
// Additional members
} structureVariable1, structureVariable2, ...;
Here, the syntax of structure declaration remains the same. The only addition is that we have declared two structure variables with the name/ identifier structureVariable after the structure definition.
Method 2: Declare Structure Variable Separately
In this method, the structure is defined separately from the declaration of structure variables. First, the structure is defined at a global scope or before the main function. Then, structure variables are declared separately using the structure name.
Syntax:
struct StructureName {
// members
};
int main(){
struct StructureName variable1, variable2;}
Here, the syntax to declare the structure in C remains the same. For declaration of the structure variables-
- The declaration is done inside the main() function, which is the entry point of the program's execution.
- We use the struct keyword with the name of the structure StructureName whose variable you want to create and the name you want to give to the variable, i.e., variabe1 and variable2.
- Also, we use the comma operator/ separator to declare two or more variables in a single line.
Below is a C program example showcasing both of these methods of declaring a structure variable.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgbmFtZWQgUG9pbnQKc3RydWN0IFBvaW50IHsKaW50IHg7CmludCB5Owp9IHAxOyAvLyBEZWNsYXJlIHN0cnVjdHVyZSB2YXJpYWJsZSBwMQoKaW50IG1haW4oKSB7CgovL0RlY2xhcmluZyBhIHN0cnVjdHVyZSB2YXJpYWJsZSBpbnNpZGUgbWFpbgpzdHJ1Y3QgUG9pbnQgcDI7CgovLyBBc3NpZ24gdmFsdWVzIHRvIHN0cnVjdHVyZSB2YXJpYWJsZXMKcDEueCA9IDEwOwpwMS55ID0gMjA7CnAyLnggPSAzMDsKcDIueSA9IDQwOwoKLy8gUHJpbnQgdmFsdWVzIG9mIHN0cnVjdHVyZSB2YXJpYWJsZXMKcHJpbnRmKCJQb2ludCBwMTogKCVkLCAlZClcbiIsIHAxLngsIHAxLnkpOwpwcmludGYoIlBvaW50IHAyOiAoJWQsICVkKVxuIiwgcDIueCwgcDIueSk7CgpyZXR1cm4gMDsKfQ==
Output:
Point p1: (10, 20)
Point p2: (30, 40)
Explanation:
In the C code example-
- We define a structure named Point, with two integer type members, x and y, representing the coordinates of a point.
- Right after the structure declaration, we declare a structure variable called p1.
- Note that since we declare the variable here, we do not need to use the struct keyword or the structure name.
- Moving onto the main() function, we declare another structure variable called p2. Note that here, we use the struct keyword followed by the structure name to create an instance of the structure.
- Next, we assign values to the members of structure variables p1 and p2, i.e., initialize the structure variables. For p1, we set x to 10 and y to 20. For p2, we set x to 30 and y to 40.
- Then, we print the values of p1 and p2 using printf() statements. We format the output to display the coordinates of each point. The newline escape sequence shifts the cursor to the next line.
Which Approach Is Better For Declaring Variables Of Structure In C?
Both methods have their advantages and use cases. The choice between them depends on the specific requirements of the program and personal preference:
Declare Structure Variable With Structure Definition | Declare Structure Variable Separately |
|
|
Ultimately, the choice between the two methods depends on factors such as code organization, readability, and maintainability requirements. It's essential to consider the specific needs of the program and choose the approach that best suits those needs.
How To Initialize Members Of Structure In C?
Initializing structure members allows you to set initial values for the variable member thus ensuring that the structure's data is properly initialized before it is used in the program. Therearee threewayss of initializing the members of a structure in C, which we will discuss in this section with the help of examples.
- Individually With Dot Operator
- Initializer List
- Designated Initializer List
Initialize Members Of Structure In C Using Dot Operator
As per this method, you can use the dot operator with the assignment operator to initialize structure members. Each member is initialized individually and is assigned a specific value. For this, you must use the structure variable name with the dot operator, as shown in the syntax below.
Syntax:
structVar.memberName = value;
Here,
- The structVar is the structure variable/ instance whose members we want to initialize, and memberName is the respective member that we want to initialize.
- The assignment operator is denoted by the equals to (=) symbol, and the dot notation (.) signifies the dot operator.
In the example C program below, we have illustrated this concept for a better understanding.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgbmFtZWQgUG9pbnQKc3RydWN0IFBvaW50IHsKaW50IHg7CmludCB5Owp9OwoKaW50IG1haW4oKSB7Ci8vIERlY2xhcmUgYSBzdHJ1Y3R1cmUgdmFyaWFibGUKc3RydWN0IFBvaW50IHAxOwoKLy8gSW5pdGlhbGl6ZSBzdHJ1Y3R1cmUgbWVtYmVycyB1c2luZyB0aGUgYXNzaWdubWVudCBvcGVyYXRvcgpwMS54ID0gMjM7CnAxLnkgPSAxMTsKCi8vIFByaW50IHRoZSBpbml0aWFsaXplZCB2YWx1ZXMKcHJpbnRmKCJDb29yZGluYXRlcyBvZiBQb2ludCBwMTogKCVkLCAlZClcbiIsIHAxLngsIHAxLnkpOwoKcmV0dXJuIDA7Cn0=
Output:
Coordinates of Point p1: (23, 11)
Explanation:
In the example C code-
- We first define a structure named Point, containing two members, x and y, representing the coordinates of a point.
- In the main() function, we declare a structure variable p1 of type Point. This declaration creates an instance of the Point structure named p1.
- Then, we initialize the members of the structure variable p1 individually using the assignment operator (=).
- We assign the value 10 to member x and the value 20 to member y.
- After that, we print these values using the printf() statement.
Initialize Members Of Structure In C Using Initializer List
Initializer lists are one of the most only used method to initialize the members of a data structure. Here, the values that you want to assign to structure variable members are enclosed inside curly braces {}. We use this method to initialize the structure members at the time of variable declaration itself.
It is important to note that the sequence of values within the initializer list must be the same as the sequence of members within the structure. That is, the values, separated by commas, must correspond to the members' order in the structure declaration. This approach becomes particularly advantageous when we need to initialize all the data members simultaneously.
Syntax:
struct StructureName variableName = {value1, value2, ...};
Here,
- The structureName and variableName refer to the name of the structure and the structure variable respectively.
- The curly braces {} represent the initializer list and the value1, value2, .... are the initial values you want to assign.
The sample C program below illustrates this approach to initialize the structure variable members.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgbmFtZWQgUmVjdGFuZ2xlCnN0cnVjdCBSZWN0YW5nbGUgewppbnQgbGVuZ3RoOwppbnQgd2lkdGg7Cn07CgppbnQgbWFpbigpIHsKLy8gRGVjbGFyZSBhbmQgaW5pdGlhbGl6ZSBhIHN0cnVjdHVyZSB2YXJpYWJsZSB1c2luZyBpbml0aWFsaXplciBsaXN0CnN0cnVjdCBSZWN0YW5nbGUgcjEgPSB7MTAsIDV9OwoKLy8gUHJpbnQgdGhlIGluaXRpYWxpemVkIHZhbHVlcwpwcmludGYoIkxlbmd0aCBvZiBSZWN0YW5nbGUgcjE6ICVkXG4iLCByMS5sZW5ndGgpOwpwcmludGYoIldpZHRoIG9mIFJlY3RhbmdsZSByMTogJWRcbiIsIHIxLndpZHRoKTsKCnJldHVybiAwOwp9
Output:
Length of Rectangle r1: 10
Width of Rectangle r1: 5
Explanation:
In the sample C code-
- We define a structure named Rectangle, containing two members, length and width, representing the dimensions of a rectangle.
- Inside main(), we declare and initialize a structure variable r1 of type Rectangle using an initializer list.
- Note that in the initializer list, we assign values 10 to length and 5 to width, which adheres with the sequence in structure declaration.
- Next, we use a set of printf() statement to display the values of these members to the console.
Initialize Members Of Structure In C Using Designated Initializer List
A designated initializer list allows you to specify values for specific members regardless of their order of declaration. Here, you must mention the name of the member along with the value inside the curly braces.
- The method may seem similar to the initializer method since we use curly braces to enclose the values.
- However, there is a major difference, i.e., we do not have to follow the sequence given in the structure declaration.
- This method provides more flexibility in initializing structure members.
Syntax:
struct StructureName variableName = {.member1 = value1, .member2 = value2, ...};
Here,
- The member1, member2, ... refer to the names of the members you want to initialize and the values are given by value1, value2....
- The dot operator (.) is used before the member name for access.
- You can follow any sequence since you are providing member name, separated by commas.
Look at the C program sample below to better understand this approach.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgbmFtZWQgUG9pbnQKc3RydWN0IFBvaW50IHsKaW50IHg7CmludCB5Owp9OwoKaW50IG1haW4oKSB7Ci8vIERlY2xhcmUgYW5kIGluaXRpYWxpemUgYSBzdHJ1Y3R1cmUgdmFyaWFibGUgdXNpbmcgdGhlIGRlc2lnbmF0ZWQgaW5pdGlhbGl6ZXIgbGlzdApzdHJ1Y3QgUG9pbnQgcDEgPSB7LnkgPSAxMCwgLnggPSAyMH07CgovLyBQcmludCB0aGUgaW5pdGlhbGl6ZWQgdmFsdWVzCnByaW50ZigiQ29vcmRpbmF0ZXMgb2YgUG9pbnQgcDE6ICglZCwgJWQpXG4iLCBwMS54LCBwMS55KTsKCnJldHVybiAwOwp9
Output:
Coordinates of Point p1: (20, 10)
Explanation:
In the C code sample-
- We define a structure named Point with two members, x and y, representing the coordinates of a point.
- In the main() function, we declare and initialize a structure variable p1 of type Point using a designated initializer list.
- Note that we first initialize y (with 10) and then x (with 20) since we provide values for the members by specifying the member names.
- Then, we print the values of its members (p1.x and p1.y) using printf() statements.
Accessing Members Of Structure In C
There are two ways of accessing the members of a stricture in C. These include the dot operator(.) and the arrow/ this operator (-->). The dot operator is used when working with member names, and the arrow operator is used when working with structure pointers. Let's discuss these methods in more detail.
The Member/ Dot Operator To Access Members of Structure In C
The member/dot operator (.) is used to access members of a structure variable, just like we used when initializing the members. It allows you to directly access the members using the variable's name followed by a dot and the member name. The syntax for the same is given below.
Syntax:
structureVariable.member
Look at the initialization example in the previous section to see how this works.
The Arrow/ This Operator To Access Members of Structure In C
In C, the arrow (->) operator is used to access members of a structure through a pointer to that structure. This operator is crucial when working with pointers to structures, as it allows us to directly access and modify the members of the structure that the pointer points to.
Syntax:
structurePointer->member
Here,
- structurePointer is a pointer to the structure.
- member is the name of the member variable within the structure.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgbmFtZWQgUG9pbnQKc3RydWN0IFBvaW50IHsKaW50IHg7CmludCB5Owp9OwoKaW50IG1haW4oKSB7Ci8vIERlY2xhcmUgYSBwb2ludGVyIHRvIGEgc3RydWN0dXJlCnN0cnVjdCBQb2ludCAqcHRyOwoKLy8gQWxsb2NhdGUgbWVtb3J5IGZvciB0aGUgc3RydWN0dXJlCnB0ciA9IChzdHJ1Y3QgUG9pbnQgKiltYWxsb2Moc2l6ZW9mKHN0cnVjdCBQb2ludCkpOwoKLy8gQWNjZXNzIGFuZCBhc3NpZ24gdmFsdWVzIHRvIHN0cnVjdHVyZSBtZW1iZXJzIHVzaW5nIHRoZSBhcnJvdyBvcGVyYXRvcgpwdHItPnggPSAxMDsKcHRyLT55ID0gMjA7CgovLyBQcmludCB0aGUgdmFsdWVzIG9mIHN0cnVjdHVyZSBtZW1iZXJzCnByaW50ZigiQ29vcmRpbmF0ZXMgb2YgUG9pbnQ6ICglZCwgJWQpXG4iLCBwdHItPngsIHB0ci0+eSk7CgovLyBGcmVlIGFsbG9jYXRlZCBtZW1vcnkKZnJlZShwdHIpOwoKcmV0dXJuIDA7Cn0=
Output:
Coordinates of Point: (10, 20)
Explanation:
In the C code-
- We define a structure named Point containing two members, x and y, representing the coordinates of a point.
- Then, in the main() function, we declare a pointer to a structure of type Point named ptr.
- We then allocate memory for the structure using the malloc() function and sizeof() operator.
- The sizeof(struct Point) calculates the size of the Point structure in bytes, and malloc() function allocates memory for this size.
- We then cast the result of malloc to (struct Point *) to ensure compatibility with the pointer type.
- After allocating memory, we access and assign values to the members of the structure using the arrow operator (->). This operator is used with pointers to structures to access members.
- We assign the value 10 to the member x and the value 20 to the member y using ptr->x and ptr->y, respectively.
- Next, we print the values of ptr->x and ptr->y using printf() statements. We format the output to display the coordinates of the point stored in the dynamically allocated memory.
- Finally, we free the allocated memory using free(ptr) to prevent memory leaks, and we return 0 to indicate the successful execution of the program.
Copying Member Values Of Structure In C
Copying structure member values involves taking the values stored in the members of one structure and assigning them to another structure. This can be done using the assignment operator (=) and simply assigning one member to another.
Syntax:
structure2 = structure1;
Here, structure1 and structure2 are variables of the same structure type.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCi8vIERlZmluZSBhIHN0cnVjdHVyZSBuYW1lZCBQZXJzb24Kc3RydWN0IFBlcnNvbiB7CmNoYXIgbmFtZVs1MF07CmludCBhZ2U7Cn07CgppbnQgbWFpbigpIHsKLy8gRGVjbGFyZSBhbmQgaW5pdGlhbGl6aW5nIGEgc3RydWN0dXJlIHZhcmlhYmxlCnN0cnVjdCBQZXJzb24gcGVyc29uMSA9IHsiQWthYXNoIiwgMjV9OwoKLy9EZWNsYXJpbmcgYW5vdGhlciBzdHJ1Y3R1cmUgdmFyaWFibGUKc3RydWN0IFBlcnNvbiBwZXJzb24yOwovLyBDb3B5IHZhbHVlcyBmcm9tIHBlcnNvbjEgdG8gcGVyc29uMiB1c2luZyBhc3NpZ25tZW50IG9wZXJhdG9yCnBlcnNvbjIgPSBwZXJzb24xOwoKLy8gUHJpbnQgdGhlIHZhbHVlcyBvZiBwZXJzb24yCnByaW50ZigiUGVyc29uIDI6IE5hbWUgLSAlcywgQWdlIC0gJWRcbiIsIHBlcnNvbjIubmFtZSwgcGVyc29uMi5hZ2UpOwoKcmV0dXJuIDA7Cn0=
Output:
Person 2: Name - Akaash, Age - 25
Explanation:
In the basic C code example-
- We define a structure Person with two members, name (char array/ string) and age (integer), representing the name and age of a person, respectively.
- In main() function, we first declare and initialize a structure variable of type Person, called person1. Here, we initialize the member name with Akaash and age with 25.
- Then, we declare another structure variable person2, without initialization.
- Next, we copy the values from person1 to person2 using the assignment operator (=). This operation copies each member of person1 to the corresponding member of person2.
- After that, we print the values of members of person2 structure variable using the printf() function.
Important Note- Alternatively, you can copy individual members using the dot (.) or arrow (->) operator, depending on whether you're working with structure variables or pointers to structures.
Modifying Variable Values Of Structure In C
There will most likely be cases where you'd want to update or modify the information contained within structure variables. To modify structure variable values, you directly assign new values to the members of the structure just like you initialized them using assignment statements.
Syntax:
structureVariable.member = newValue;
Here, we write the structure variable name followed by a dot operator and the member name. And then assign the new value.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCi8vIERlZmluZSBhIHN0cnVjdHVyZSBuYW1lZCBQZXJzb24Kc3RydWN0IFBlcnNvbiB7CmNoYXIgbmFtZVs1MF07CmludCBhZ2U7Cn07CgppbnQgbWFpbigpIHsKLy8gRGVjbGFyZSBhIHN0cnVjdHVyZSB2YXJpYWJsZQpzdHJ1Y3QgUGVyc29uIHBlcnNvbjEgPSB7IkFzaGlzaCIsIDMxfTsKcHJpbnRmKCJUaGUgYWdlIG9mIFBlcnNvbiAxOiAlZFxuIiwgcGVyc29uMS5hZ2UpOwoKLy8gTW9kaWZ5IHRoZSBhZ2Ugb2YgcGVyc29uMQpwZXJzb24xLmFnZSA9IDM1OwoKLy8gUHJpbnQgdGhlIG1vZGlmaWVkIHZhbHVlIG9mIGFnZQpwcmludGYoIlRoZSBtb2RpZmllZCBhZ2Ugb2YgUGVyc29uIDE6ICVkXG4iLCBwZXJzb24xLmFnZSk7CgpyZXR1cm4gMDsKfQ==
Output:
The age of Person 1: 31
The modified age of Person 1: 35
Explanation:
In the code example-
- We first define a structure named Person with two members, i.e., name and age, representing the information of a person.
- In the main() function, we declare and initialize a structure variable person1 of type Person, with member name set to Ashish and age set to 31.
- Next, we use a printf() statement to display this information to the console.
- After that, we modify the age by assigning a new value of 35 to the age member of the person variable. Here, we use the dot operator to access the member.
- We then print the modified value using the printf() statement.
Memory Allocation For Structure In C
Memory allocation refers to the process of allocating memory space for structure variables and their member variables.
- When we define a structure in C programs, the compiler calculates the total size of the structure based on the sizes of its individual member variables and any padding added for memory alignment.
- Memory blocks for structure variables can be allocated statically, dynamically, or as part of other data structures like arrays or linked lists.
- For static allocation, memory consumption for structure variables is allocated at compile time and remains fixed throughout the program's execution.
- Dynamic allocation, on the other hand, allows memory to be allocated at runtime using functions like malloc() or calloc(). This enables flexible memory management and is particularly useful when the number of structure instances or their sizes vary during program execution.
Also read: The Difference Between Malloc() And Calloc() Simplified (+Examples)
Operations On Structure Variables In C
In the sections above, we have covered a variety of operations that can be performed on structure variables. The table given below summarizes the basic operations, actions, and manipulations that can be performed on a structure in C.
Operation |
Description |
Syntax |
Initialization |
Creating a new structure variable and assigning values. |
struct StructureType variable_name = {value}; |
Modification |
Changing the values of structure members. |
variable.member_name = new_value; |
Copying (Assignment) |
Creating a copy of a structure variable. |
destination_structure = source_structure; |
Printing (Displaying) |
Outputting the values of structure members. |
printf("Member: %s\n", variable.member_name); |
Check out this amazing course to become the best version of the C programmer you can be.
Structure In C As Function Arguments
A structure in C can be used as a function argument, especially when working with complex data structures or passing multiple related data items to a function. Structures provide a convenient way to encapsulate and organize data, making functions more modular and easier to understand.
We can pass a structure in C as a function argument in one of two ways, i.e., passing by value and passing by reference (using pointers). Each approach has its advantages and use cases, depending on factors such as performance, memory usage, and the need to modify the original structure.
Passing-By-Value | Structure In C As Function Argument
The process of passing a structure in C by value as a function argument involves passing a copy of the entire structure to the function. This means any modifications made to the structure within the function do not affect the original structure.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUKc3RydWN0IEVtcGxveWVlIHsKY2hhciBuYW1lWzUwXTsKaW50IGFnZTsKfTsKCi8vIEZ1bmN0aW9uIHRvIG1vZGlmeSB0aGUgc3R1ZGVudCdzIGFnZQp2b2lkIG1vZGlmeUFnZShzdHJ1Y3QgRW1wbG95ZWUgZW1wKSB7CmVtcC5hZ2UgPSAyNTsKcHJpbnRmKCJJbnNpZGUgRnVuY3Rpb246IE5hbWUgPSAlcywgQWdlID0gJWRcbiIsIGVtcC5uYW1lLCBlbXAuYWdlKTsKfQoKaW50IG1haW4oKSB7CgpzdHJ1Y3QgRW1wbG95ZWUgZW1wMSA9IHsiQXJhZGh5YSIsIDIwfTsKcHJpbnRmKCJCZWZvcmUgRnVuY3Rpb24gQ2FsbDogTmFtZSA9ICVzLCBBZ2UgPSAlZFxuIiwgZW1wMS5uYW1lLCBlbXAxLmFnZSk7CgovLyBDYWxsIHRoZSBmdW5jdGlvbgptb2RpZnlBZ2UoZW1wMSk7CnByaW50ZigiQWZ0ZXIgRnVuY3Rpb24gQ2FsbDogTmFtZSA9ICVzLCBBZ2UgPSAlZFxuIiwgZW1wMS5uYW1lLCBlbXAxLmFnZSk7CgpyZXR1cm4gMDsKfQ==
Output:
Before Function Call: Name = Aradhya, Age = 20
Inside Function: Name = Aradhya, Age = 25
After Function Call: Name = Aradhya, Age = 20
Explanation:
In the code-
- We define a structure Employee with tw members name and age to store the employee information.
- Next, we define a function called modifyAge() that takes a structure variable (emp) of type Employee as its parameter.
- Inside the function, we modify the value of the age member of the structure emp to 25, and then print values of name and age.
- Moving to the main() function, we variable emp1 of type Employee structure and initialize its members name with Aradhya and age with 20.
- Following this, we print the member value of the emp1 structure variable with a string message stating that this is before calling the modifying function.
- Next, we call the modifyAge() function, passing emp1 as an argument. The function modifies the age member and prints the values to the console.
- We then use a printf() statement to display the values of the structure members of emp1 to show what happens after the variable is passed to a modifying function.
- As shown in the output, the changes made inside the modifyAge function do not affect the original student1.
- This is because the modifyAge function receives a copy of the student1 structure, not a reference to it. Therefore, modifications made inside the function are made to the copy, not the original structure.
Passing-By-Reference | Structure In C As Function Argument
When we say passing a structure in C to a function by reference, we mean that a pointer to the structure is passed to the function. This allows the function to directly modify the original structure, as it operates on the memory location of the structure.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUKc3RydWN0IEVtcGxveWVlIHsKY2hhciBuYW1lWzUwXTsKaW50IGFnZTsKfTsKCi8vIEZ1bmN0aW9uIHRvIG1vZGlmeSB0aGUgc3R1ZGVudCdzIGFnZQp2b2lkIG1vZGlmeUFnZShzdHJ1Y3QgRW1wbG95ZWUgKmVtcCkgewplbXAtPmFnZSA9IDI1OwpwcmludGYoIkluc2lkZSBGdW5jdGlvbjogTmFtZSA9ICVzLCBBZ2UgPSAlZFxuIiwgZW1wLT5uYW1lLCBlbXAtPmFnZSk7Cn0KCmludCBtYWluKCkgewoKc3RydWN0IEVtcGxveWVlIGVtcDEgPSB7IlRhYXZpIiwgMjB9OwpwcmludGYoIkJlZm9yZSBGdW5jdGlvbiBDYWxsOiBOYW1lID0gJXMsIEFnZSA9ICVkXG4iLCBlbXAxLm5hbWUsIGVtcDEuYWdlKTsKCi8vIENhbGwgdGhlIGZ1bmN0aW9uIHdpdGggYSBwb2ludGVyIHRvIHRoZSBzdHJ1Y3R1cmUKbW9kaWZ5QWdlKCZlbXAxKTsKcHJpbnRmKCJBZnRlciBGdW5jdGlvbiBDYWxsOiBOYW1lID0gJXMsIEFnZSA9ICVkXG4iLCBlbXAxLm5hbWUsIGVtcDEuYWdlKTsKCnJldHVybiAwOwp9
Output:
Before Function Call: Name = Taavi, Age = 20
Inside Function: Name = Taavi, Age = 25
After Function Call: Name = Taavi, Age = 25
Explanation:
In the above code-
- Just like the previous example, we have a structure named Employee with two members, name and age, to record employee information.
- Then, we define a function modifyAge() that takes a pointer to the Employee structure as its parameter.
- Inside the function, we modify the value of the age member of the structure pointed to by emp to 25 and print the member values to the console.
- We then declare and initialize a structure variable emp1, with the name member set to Taavi and age to 20, inside the main() function.
- Next, we print the initial values of members of emp1 to the console using the printf() statement.
- After that, we call the modifyAge() function, passing emp1 by reference (using address-of operator) as an argument.
- The function modifies the value of the age member and prints revised values to the console.
- Next, we again print the member's values of the emp1 struct variable and see the changes made by the modifying () function.
- As shown in the output, the changes made inside the function also alter the original value. This was not the case when we passed the struct variable to the function by value in the previous example.
Also read: Difference Between Call By Value And Call By Reference (+Examples)
Typedef & Structure In C
The typedef keyword is used to create an alias or a new name for an existing data type. This applies to all primitive types and also user-defined types like structure in C.
- It provides a way to define custom names for complex data types, making code more readable and maintainable.
- Using typedef for structures in C is a valuable technique for creating custom data types with more meaningful and readable names.
- It simplifies the declaration of variables and enhances code clarity, especially when working with complex data structures.
Given below is the syntax to use typedef on structure in C followed by a basic C program example showacing its application.
Syntax:
typedef struct {
data_type1 member1;
data_type2 member2;
...
} new_type_name;
Here,
- The typedef keyword implies that we are defining a new type.
- The term new_type_name represents the new name or alias we assign to this structure type.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgd2l0aG91dCB0eXBlZGVmCnN0cnVjdCBQb2ludCB7CmludCB4OwppbnQgeTsKfTsKCi8vIERlZmluZSBhIHN0cnVjdHVyZSB3aXRoIHR5cGVkZWYKdHlwZWRlZiBzdHJ1Y3QgewppbnQgeDsKaW50IHk7Cn0gUHQyRDsKCmludCBtYWluKCkgewovLyBVc2luZyB0aGUgc3RydWN0dXJlIHdpdGhvdXQgdHlwZWRlZgpzdHJ1Y3QgUG9pbnQgcDEgPSB7NSwgMTB9OwoKLy8gVXNpbmcgdGhlIHN0cnVjdHVyZSB3aXRoIHR5cGVkZWYKUHQyRCBwMjsKcDIueCA9IDM7CnAyLnkgPSA4OwoKcHJpbnRmKCJQb2ludCBwMTogeCA9ICVkLCB5ID0gJWRcbiIsIHAxLngsIHAxLnkpOwpwcmludGYoIlBvaW50IHAyOiB4ID0gJWQsIHkgPSAlZFxuIiwgcDIueCwgcDIueSk7CgpyZXR1cm4gMDsKfQ==
Output:
Point p1: x = 5, y = 10
Point p2: x = 3, y = 8
Explanation:
In the example-
- We define a structure named Point with two members, x and y, representing the coordinates of a point.
- Then, we define another structure using typedef. The typedef keyword allows us to define a new name (Pt2D) for an existing data type (struct {int x; int y;}).
- This new name can then be used to declare variables of this structure type without using the struct keyword.
- In the main() function, we declare and initialize a variable p1 of type struct Point with x set to 5 and y to 10.
- Then, we declare another variable p2 of type Pt2D, which is a typedef for the structure with members x and y.
- Next, we initialize its members x and y of the p2 struct variable with the values 3 and 8, respectively.
- After that, we use a set of printf() statements to display the member values of both structure variables p1 and p2.
- As shown in the output, the typedef-ed alias works just like the original type name for the structure Point.
What Is An Array Of Structures In C?
An array of structure in C is a data structure that consists of multiple instances of a structure type arranged sequentially in memory. It allows you to store and manipulate collections of related data, where each element of the array represents a single instance of the structure.
In simpler terms, an array of structures is like a table where each row represents an individual structure, and each column represents a member variable within the structure.
Syntax:
struct StructureType arrayName[numberOfElements];
Here,
- The struct keyword indicates structures with the type name StructureType.
- The arrayName refers to the name of the array containing structures and numberOfElements refers to the instances of structure inside the array.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCi8vIERlZmluZSBhIHN0cnVjdHVyZSBuYW1lZCBTdHVkZW50CnN0cnVjdCBTdHVkZW50IHsKY2hhciBuYW1lWzUwXTsKaW50IGFnZTsKfTsKCmludCBtYWluKCkgewovLyBEZWNsYXJlIGFuIGFycmF5IG9mIHN0cnVjdHVyZXMgKGFycmF5IG9mIFN0dWRlbnRzKQpzdHJ1Y3QgU3R1ZGVudCBzdHVkZW50c1szXTsKCi8vIEluaXRpYWxpemUgdmFsdWVzIGZvciBlYWNoIHN0dWRlbnQgaW4gdGhlIGFycmF5CnN0cmNweShzdHVkZW50c1swXS5uYW1lLCAiQXRoYXJ2YSIpOwpzdHVkZW50c1swXS5hZ2UgPSAyMDsKCnN0cmNweShzdHVkZW50c1sxXS5uYW1lLCAiQWRhaCIpOwpzdHVkZW50c1sxXS5hZ2UgPSAyMjsKCnN0cmNweShzdHVkZW50c1syXS5uYW1lLCAiQW5hbnQiKTsKc3R1ZGVudHNbMl0uYWdlID0gMjE7CgovLyBQcmludCBpbmZvcm1hdGlvbiBhYm91dCBlYWNoIHN0dWRlbnQgaW4gdGhlIGFycmF5CmZvciAoaW50IGkgPSAwOyBpIDwgMzsgaSsrKSB7CnByaW50ZigiU3R1ZGVudCAlZDogTmFtZSAtICVzLCBBZ2UgLSAlZFxuIiwgaSArIDEsIHN0dWRlbnRzW2ldLm5hbWUsIHN0dWRlbnRzW2ldLmFnZSk7Cn0KCnJldHVybiAwOwp9
Output:
Student 1: Name - Atharva, Age - 20
Student 2: Name - Adah, Age - 22
Student 3: Name - Anant, Age - 21
Explanation:
In this code-
- We define a structure named Student (using the struct keyword) with two members, name and age. The name is a character array/ string that can have up to 50 characters.
- In the main function, we declare an array of structures (array of Student structures) named students with a size of 3. That is, this array will hold information about three students.
- Next, we use the strcpy() function from the <string.h> library and the index number to initialize each name member of the 3 structure variables.
- We also initialize the age members of the structure variables in the array using the dot and assignment operators.
- After that, we use a for loop to iterate over each element of the students array, starting from index value i=0 till i is less than 3.
- In every iteration, the loop prints the information of each student, incrementing the value of control variable i by 1 afterwards.
- We access the name and age members of each Student structure using array indexing (students[i].name and students[i].age). We also include the index i + 1 to indicate the student number.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Nested Structures In C
Structure nesting refers to the practice of defining one structure inside another structure in C programs. This allows you to create complex data structures where a structure can have members that are themselves structures. This concept is useful for organizing and representing hierarchical or composite data. It provides a way to model real-world entities with multiple attributes and subattributes.
Syntax:
struct OuterStructure {
// Outer structure members
struct InnerStructure {
// Inner structure members
} inner_member;
};
Here,
- The terms OuterStructure and InnerStructure are names of the outer and inner structures, respectively.
- The curly braces {} contain the structure members for the respective structure.
- The inner_member is a member of the outer structure that has a type of the inner structure.
There are two different types of nesting that can be done with structures in C.
Embedded Nesting Of Structure In C
Embedded structure nesting, also known as structure composition, involves defining an inner structure as a direct member of the outer structure. In this type of nesting, the inner structure is a part of the outer structure, and it's included directly within the outer structure's definition.
The syntax for this is similar to that of nested structure in C. Let’s take a look at an example to better understand the same.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KCnN0cnVjdCBQZXJzb24gewpjaGFyIG5hbWVbNTBdOwpzdHJ1Y3QgRGF0ZU9mQmlydGggewppbnQgZGF5OwppbnQgbW9udGg7CmludCB5ZWFyOwp9IGRvYjsKaW50IGFnZTsKfTsKCmludCBtYWluKCkgewoKc3RydWN0IFBlcnNvbiBwZXJzb247CgovLyBJbml0aWFsaXplIG91dGVyIHN0cnVjdHVyZSBtZW1iZXJzCnN0cmNweShwZXJzb24ubmFtZSwgIkhhcnNoYSIpOwpwZXJzb24uYWdlID0gMjU7CgovLyBJbml0aWFsaXplIGlubmVyIHN0cnVjdHVyZSBtZW1iZXJzCnBlcnNvbi5kb2IuZGF5ID0gMTU7CnBlcnNvbi5kb2IubW9udGggPSA3OwpwZXJzb24uZG9iLnllYXIgPSAxOTk4OwoKcHJpbnRmKCJOYW1lOiAlc1xuIiwgcGVyc29uLm5hbWUpOwpwcmludGYoIkRhdGUgb2YgQmlydGg6ICVkLyVkLyVkXG4iLCBwZXJzb24uZG9iLmRheSwgcGVyc29uLmRvYi5tb250aCwgcGVyc29uLmRvYi55ZWFyKTsKcHJpbnRmKCJBZ2U6ICVkXG4iLCBwZXJzb24uYWdlKTsKCnJldHVybiAwOwp9
Output:
Name: Harsh
Date of Birth: 15/7/1998
Age: 25
Explanation:
In the code-
- We define a structure Person with three members, i.e., name (string), age (integer) and date of birth, which itself is a structure.
- The dob structure contains three members, i.e., day, month, and year, each representing the corresponding components of the date of birth. We also declare a structure variable dob after the inner structure definition.
- Then, we create a structure variable person of type Person in the main() function.
- We use the strcpy() function to initialize the name member with the string value Harsha and the normal dot operator assignment method to initialize the age member with 25.
- For the members of the dob structure, we use the dot operator direct assignment method and initialize each member individually.
- Note that we use the dot operator twice here since they are members of the embedded inner structure.
- After that, we use three printf() statements to display all the information of the person variable.
Separate Nesting Of Structure In C
In separate structure nesting, you define an inner structure independently of the outer structure and then include it as a member within the outer structure. The inner structure is not directly defined within the outer structure but rather referenced by the outer structure.
Syntax:
struct InnerStructure {
// Inner structure members
};
struct OuterStructure {
// Outer structure members
struct InnerStructure inner_member;
};
Here, the InnerStructure definition is given first, and then one of its members, i.e., inner_member, is created inside the OuterStructure definition.
Code Example:
I2luY2x1ZGUgPHN0cmluZy5oPgojaW5jbHVkZSA8c3RkaW8uaD4KCi8vIERlZmluZSBhbiBpbm5lciBzdHJ1Y3R1cmUgc2VwYXJhdGVseQoKc3RydWN0IEFkZHJlc3MgewpjaGFyIHN0cmVldFs1MF07CmNoYXIgY2l0eVszMF07CmNoYXIgc3RhdGVbMjBdOwp9OwoKLy8gRGVmaW5lIGFuIG91dGVyIHN0cnVjdHVyZSB0aGF0IHJlZmVyZW5jZXMgdGhlIGlubmVyIHN0cnVjdHVyZQpzdHJ1Y3QgRW1wbG95ZWUgewpjaGFyIG5hbWVbNTBdOwpzdHJ1Y3QgQWRkcmVzcyBhZGRyZXNzOyAvLyBSZWZlcmVuY2UgdG8gQWRkcmVzcyBzdHJ1Y3R1cmUKaW50IGVtcGxveWVlSWQ7Cn07CgppbnQgbWFpbigpIHsKCi8vIERlY2xhcmUgYSB2YXJpYWJsZSBvZiB0aGUgb3V0ZXIgc3RydWN0dXJlCnN0cnVjdCBFbXBsb3llZSBlbXA7Ci8vIEluaXRpYWxpemUgb3V0ZXIgc3RydWN0dXJlIG1lbWJlcnMKc3RyY3B5KGVtcC5uYW1lLCAiRGhydXYiKTsKZW1wLmVtcGxveWVlSWQgPSAxMjM0NTsKCi8vIEluaXRpYWxpemUgaW5uZXIgc3RydWN0dXJlIG1lbWJlcnMKc3RyY3B5KGVtcC5hZGRyZXNzLnN0cmVldCwgIjEyMyBSSyBQdXJhbSIpOwpzdHJjcHkoZW1wLmFkZHJlc3MuY2l0eSwgIlNvdXRoIERlbGhpIik7CnN0cmNweShlbXAuYWRkcmVzcy5zdGF0ZSwgIkRlbGhpIik7CnByaW50ZigiTmFtZTogJXNcbiIsIGVtcC5uYW1lKTsKcHJpbnRmKCJFbXBsb3llZSBJRDogJWRcbiIsIGVtcC5lbXBsb3llZUlkKTsKcHJpbnRmKCJBZGRyZXNzOiAlcywgJXMsICVzXG4iLCBlbXAuYWRkcmVzcy5zdHJlZXQsIGVtcC5hZGRyZXNzLmNpdHksIGVtcC5hZGRyZXNzLnN0YXRlKTsKCnJldHVybiAwOwp9
Output:
Name: Dhruv
Employee ID: 12345
Address: 123 Main St, Cityville, State
Explanation:
In the above code-
- We first define an inner structure named Address containing three members, i.e., street, city, and state, each representing parts of an address.
- Then, we define an outer structure named Employee, with three members, i.e., name (string/ char array), address (a structure variable of type Address) and employeeId (integer).
- Note that the structure Address becomes the inner structure as a variable of its type is a member of the Employee structure
- In the main() function, we declare a variable emp of type Employee and initialize the name and employeeID members with values Dhruv and 12345, respectively.
- Then, we use the double dot operator with strcpy() function to initialize the members of the inner structure, i.e., street, city, and state.
- After that, we use a set of printf() statements to display the name, employee ID, and address information of the emp structure variable on the console.
Accessing Nested Members
In both embedded and separate structure nesting, you access nested members using the dot (.) operator. For example, in the code examples provided earlier, we accessed members like person.dob.day or emp.address.city. This notation allows you to access the individual members of nested structures and manipulate their data.
What Is Pointer To Structure In C (Structure Pointer)?
Pointers are variables that store memory addresses of other variables and point to those values. They enable direct access to the memory location where data is stored, allowing for efficient manipulation of data and dynamic memory allocation.
A pointer to a structure in C is a variable that holds the memory address of a structure. It allows indirect access to the members of the structure, enabling efficient manipulation of structure data and dynamic memory allocation for structures.
Syntax:
struct StructureType *ptr;
Here,
- The struct keyword indicates that the component is a structure whose name is given by StructureType.
- The asterisk symbol (*) indicates the pointer variable, and ptr is the name given to the pointer.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CiNpbmNsdWRlIDxzdHJpbmcuaD4KI2luY2x1ZGUgPHN0ZGxpYi5oPgoKLy8gRGVmaW5lIGEgc3RydWN0dXJlCnN0cnVjdCBTdHVkZW50IHsKY2hhciBuYW1lWzUwXTsKaW50IGFnZTsKfTsKCmludCBtYWluKCkgewoKLy8gRGVjbGFyZSBhIHBvaW50ZXIgdG8gYSBzdHJ1Y3R1cmUKc3RydWN0IFN0dWRlbnQgKnN0dWRlbnRQdHI7CgovLyBBbGxvY2F0ZSBtZW1vcnkgZm9yIHRoZSBzdHJ1Y3R1cmUgYW5kIGFzc2lnbiBpdHMgYWRkcmVzcyB0byB0aGUgcG9pbnRlcgpzdHVkZW50UHRyID0gKHN0cnVjdCBTdHVkZW50ICopbWFsbG9jKHNpemVvZihzdHJ1Y3QgU3R1ZGVudCkpOwoKLy8gQ2hlY2sgaWYgbWVtb3J5IGFsbG9jYXRpb24gd2FzIHN1Y2Nlc3NmdWwKaWYgKHN0dWRlbnRQdHIgPT0gTlVMTCkgewpwcmludGYoIk1lbW9yeSBhbGxvY2F0aW9uIGZhaWxlZC5cbiIpOwpyZXR1cm4gMTsKfQoKLy8gQWNjZXNzIGFuZCBtb2RpZnkgc3RydWN0dXJlIG1lbWJlcnMgdXNpbmcgdGhlIHBvaW50ZXIKc3RyY3B5KHN0dWRlbnRQdHItPm5hbWUsICJTaGl2YW5pIik7CnN0dWRlbnRQdHItPmFnZSA9IDI1OwovLyBEaXNwbGF5IHRoZSBzdHJ1Y3R1cmUgbWVtYmVycyB0aHJvdWdoIHRoZSBwb2ludGVyCnByaW50ZigiTmFtZTogJXNcbiIsIHN0dWRlbnRQdHItPm5hbWUpOwpwcmludGYoIkFnZTogJWRcbiIsIHN0dWRlbnRQdHItPmFnZSk7CgovLyBGcmVlIHRoZSBhbGxvY2F0ZWQgbWVtb3J5CmZyZWUoc3R1ZGVudFB0cik7CgpyZXR1cm4gMDsKfQ==
Output:
Name: Shivani
Age: 25
Explanation:
In the example code-
- We first define a structure named Student, with two members, i.e., name and age, to store the student's information.
- In the main() function, we declare a pointer variable studentPtr, that points to a Student structure.
- Then, we dynamically allocate memory for a Student structure using the malloc() function and sizeof() operator.
- Here, the sizeof() operator determines the size of the structure in bytes which the malloc() function assigns to the pointer after typecasting the value.
- Next, we use an if-statement and NULL pointer to check if the memory was successfully allocated.
- If the condition studentPtr equals NULL, i.e., studentPtr == NULL is true, it means the memory allocation failed. The if block executes, prints an error message and returns 1 to indicate failure.
- If the condition is false, then the memory allocation is successful, and we move to the next part of the code.
- After that, we use the arrow/ this pointer (->) to access the members of the structure variable through studentPtr. We initialize the name and age members of the variable.
- Then, we use a printf() statement to display these values to the console along with a message.
- Once the pointer variable is no longer in use, we free the dynamically allocated memory using the free() function. This prevents memory leaks and deallocates the memory previously allocated with malloc.
- Finally, the main returns 0 to indicate the successful execution of the program.
Self-Referential Structure In C
Self-referential structure in C is a special type of structure that contains a member that is a pointer to the same type of structure.
- This self-referential capability allows you to create data structures that are linked together, forming complex hierarchical or recursive data structures like linked lists, trees, graphs, and more.
- Each structure instance points to another structure of the same type, enabling the creation of dynamic and interconnected data structures.
- The ideal approach involves defining a structure that contains a member, typically a pointer, to the same structure type.
- This creates a linked structure where each instance of the structure can be connected to another instance, allowing for the creation of various data structures like linked lists.
- You allocate memory dynamically as needed and use pointers to traverse and manipulate the linked structures.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzZWxmLXJlZmVyZW50aWFsIHN0cnVjdHVyZSByZXByZXNlbnRpbmcgYSBub2RlIGluIGEgbGlua2VkIGxpc3QKc3RydWN0IE5vZGUgewppbnQgZGF0YTsKc3RydWN0IE5vZGUgKm5leHQ7Cn07CgppbnQgbWFpbigpIHsKLy8gRGVjbGFyZSB0aHJlZSBub2RlcwpzdHJ1Y3QgTm9kZSBub2RlMSwgbm9kZTIsIG5vZGUzOwoKLy8gQXNzaWduIGRhdGEgdmFsdWVzIHRvIHRoZSBub2Rlcwpub2RlMS5kYXRhID0gMTA7Cm5vZGUyLmRhdGEgPSAyMDsKbm9kZTMuZGF0YSA9IDMwOwoKLy8gTGluayB0aGUgbm9kZXMgdG9nZXRoZXIKbm9kZTEubmV4dCA9ICZub2RlMjsKbm9kZTIubmV4dCA9ICZub2RlMzsKbm9kZTMubmV4dCA9IE5VTEw7IC8vIEVuZCBvZiB0aGUgbGlua2VkIGxpc3QKCi8vIFRyYXZlcnNlIHRoZSBsaW5rZWQgbGlzdCBhbmQgcHJpbnQgdGhlIGRhdGEgdmFsdWVzCnN0cnVjdCBOb2RlICpjdXJyZW50ID0gJm5vZGUxOyAvLyBTdGFydCBmcm9tIHRoZSBoZWFkIG9mIHRoZSBsaXN0CndoaWxlIChjdXJyZW50ICE9IE5VTEwpIHsKcHJpbnRmKCIlZCAiLCBjdXJyZW50LT5kYXRhKTsKY3VycmVudCA9IGN1cnJlbnQtPm5leHQ7IC8vIE1vdmUgdG8gdGhlIG5leHQgbm9kZQp9CnByaW50ZigiXG4iKTsKCnJldHVybiAwOwp9
Output:
10 20 30
Explanation:
In the above code,
- We define a self-referential structure named Node. This structure represents a node in a linked list.
- It contains two members, i.e., data, an integer to store the data of the node, and next, a pointer to another Node structure, representing the next node in the linked list.
- In the main() function, we declare three node variables node1, node2, and node3, each representing individual nodes in the linked list.
- We then initialize the data members of the node variables using the dot notation and assignment operator.
- Then, we link the next members together by initializing them with the address of the next node in the sequence.
- That is, we assign the address-of node 2 to next member of node1, node3 to next member of node2 and NULL value to next member of node3.
- This creates a linked list where node1 points to node2, node2 points to node3, and node3 points to NULL, indicating the end of the list.
- Next, we declare a pointer variable called current of type struct Node* and initialize it with the address of node1. Here, the first node (node1) serves as the head of the linked list.
- After that, we use a while loop to traverse the linked list starting from the head (node1). The loop condition checks if the value of current pointer is NULL. If the condition is false, we move outside the loop.
- If the condition is true, the loop body is executed. Inside the controlling loop, the data value of the current node (current->data) is printed using the arrow operator (->).
- Then, the next pointer of the current node is assigned to current, effectively moving to the next node in the list.
- The loop continues until current becomes NULL, indicating the end of the linked list.
- Finally, a newline character is printed to format the output, and 0 is returned to indicate successful execution of the program.
What Is Member Alignment For Structure In C?
Structure member alignment refers to the way in which the compiler arranges the member variables within a structure in memory. It ensures that each member starts at a memory address that is aligned according to its data type's alignment requirements. Proper alignment is essential for optimizing memory access and ensuring efficient data storage and retrieval for structure in C.
-
Data Alignment: Data types in C have specific alignment requirements, meaning they should be stored at memory addresses that are multiples of their size in bytes.
- For example, on many systems, an int typically requires alignment to a 4-byte boundary, meaning it should start at a memory address that is divisible by 4.
- Similarly, a double may require alignment to an 8-byte boundary, and so on.
-
Structure Member Alignment: When you define a structure in C, the compiler arranges its member variables in memory based on their alignment requirements.
- The compiler tries to optimize memory usage and access speed by inserting padding bytes between structure members if necessary.
- Padding bytes ensure that each member starts at an appropriate memory address according to its alignment requirements.
-
Padding: Padding refers to the insertion of additional bytes between structure members to ensure proper alignment.
- Padding bytes are not part of the structure's logical data but are used to maintain alignment.
- The amount of padding added between members depends on the alignment requirements of the members themselves and the compiler's specific padding rules.
Padding & Packing For Structure In C
Structure padding and packing are techniques used to control the alignment and layout of data members within C structures. They are particularly important when dealing with low-level programming, data serialization, and interfacing with external systems where memory layout matters.
What Is Padding In Structure In C?
Structure padding refers to the insertion of additional bytes between structure members to ensure proper alignment. The compiler adds padding bytes to ensure that each member starts at a memory address that is aligned according to its data type's alignment requirements. This alignment is necessary for efficient memory access, as misaligned memory access can lead to performance penalties or even program crashes on some architectures.
What Is Packing In Structure In C?
Structure packing, also known as packing or structure pragma packing, is the process of minimizing the amount of padding added between structure members. By default, most compilers add padding to structures for alignment purposes. However, in some cases, you may want to minimize or eliminate this padding to reduce the memory footprint of the structure, especially in embedded systems or when dealing with data that needs to be stored or transmitted efficiently.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgd2l0aG91dCBwYWNraW5nCnN0cnVjdCBFeGFtcGxlV2l0aG91dFBhY2tpbmcgewpjaGFyIGE7CmludCBiOwpjaGFyIGM7Cn07CgovLyBEZWZpbmUgYSBzdHJ1Y3R1cmUgd2l0aCBwYWNraW5nCnN0cnVjdCBFeGFtcGxlV2l0aFBhY2tpbmcgewpjaGFyIGE7CmludCBiOwpjaGFyIGM7Cn0gX19hdHRyaWJ1dGVfXygocGFja2VkKSk7CgppbnQgbWFpbigpIHsKLy8gUHJpbnQgc2l6ZSBvZiB0aGUgc3RydWN0dXJlIHdpdGhvdXQgcGFja2luZwpwcmludGYoIlNpemUgb2Ygc3RydWN0IEV4YW1wbGVXaXRob3V0UGFja2luZzogJXp1IGJ5dGVzXG4iLCBzaXplb2Yoc3RydWN0IEV4YW1wbGVXaXRob3V0UGFja2luZykpOwoKLy8gUHJpbnQgc2l6ZSBvZiB0aGUgc3RydWN0dXJlIHdpdGggcGFja2luZwpwcmludGYoIlNpemUgb2Ygc3RydWN0IEV4YW1wbGVXaXRoUGFja2luZzogJXp1IGJ5dGVzXG4iLCBzaXplb2Yoc3RydWN0IEV4YW1wbGVXaXRoUGFja2luZykpOwoKcmV0dXJuIDA7Cn0=
Output:
Size of struct ExampleWithoutPacking: 12 bytes
Size of struct ExampleWithPacking: 6 bytes
Explanation:
In the above code,
- We begin by defining two structures ExampleWithoutPacking and ExampleWithPacking.
- ExampleWithoutPacking represents a structure without any packing directive, so the compiler adds padding between members for alignment. It contains three members: a (a character), b (an integer), and c (a character).
- ExampleWithPacking is defined with the GCC-specific __attribute__((packed)) attribute, indicating that it should be packed without any padding. It has the same members as ExampleWithoutPacking, but it includes an attribute __attribute__((packed)).
- This attribute tells the compiler to pack the structure members without adding any padding between them, resulting in a smaller memory footprint.
- In the main() function, we use the sizeof() operator to determine the size of each structure and print the same to the console.
- As seen in the output, the size without packing is more than with parking, indicating that packing is useful in optimizing space allocation.
Bit Fields & Structure In C
In computing, a bit (short for binary digit) is the basic unit of information, representing either a 0 or a 1. It is the smallest unit of data in a computer and is used to store and transmit information in digital systems.
- Bits are the building blocks of digital representation and manipulation, forming the foundation of binary arithmetic and data storage.
- Bit fields in C allow you to specify the number of bits a particular data member of a structure should occupy.
- They provide a way to compactly represent data in structures, which can be useful for saving memory and optimizing storage of values that don't require a full byte (8 bits) for storage.
Syntax for Defining Bit Fields:
struct BitFieldStruct {
data_type member_name : number_of_bits;
};
Here,
- The struct keyword begins the definition of the structure, the name of which is given by BitFieldStruct.
- The terms data_type, member_name, and number_of_bits refer to the data type of the structure member, its identifier/ name, and the number of bits to be allocated to that member, respectively.
Code Example:
I2luY2x1ZGUgPHN0ZGlvLmg+CgpzdHJ1Y3QgQml0RmllbGRFeGFtcGxlIHsKdW5zaWduZWQgaW50IGZsYWcgOiAxOwp1bnNpZ25lZCBpbnQgdmFsdWUgOiAzOwp9OwoKaW50IG1haW4oKSB7CgpzdHJ1Y3QgQml0RmllbGRFeGFtcGxlIGJmOwoKYmYuZmxhZyA9IDE7CmJmLnZhbHVlID0gNTsgLy8gNSBpbiBiaW5hcnkgaXMgMTAxLCBzbyBvbmx5IHRoZSBsYXN0IDMgYml0cyBhcmUgc3RvcmVkCgpwcmludGYoIkZsYWc6ICVkXG4iLCBiZi5mbGFnKTsKcHJpbnRmKCJWYWx1ZTogJWRcbiIsIGJmLnZhbHVlKTsKCnJldHVybiAwOwp9
Output:
Flag: 1
Value: 5
Explanation:
In the above code-
- We define a structure named BitFieldExample containing two bit-field members, i.e., flag and value. Bit-fields are used to specify the number of bits to allocate for each member.
- In the main() function, we declare a structure variable bf of type BitFieldExample, representing an instance of the BitFieldExample structure.
- Then, we set the flag member of the bf variable to 1 and the value member to 5.
- When assigning 5 to value, only the last 3 bits of the binary representation of 5 (which is 101) will be stored because we've specified 3 for the value member, meaning it can only hold 3 bits.
- Next, we use a set of printf() statements to display the values of the flag and value members.
Why Use Structure?
Structures in C serve several important purposes, making them essential for organizing and managing data effectively. Here are the key reasons why structures are used in C:
- Grouping Related Data: Structures in C allow the grouping of variables of different data types under a single name. This enables the organization of related data items into a cohesive unit, making it easier to manage and manipulate data.
- Encapsulation: Structures encapsulate data and operations related to a specific entity or concept. By bundling related data together, structures promote modular and organized code, enhancing readability and maintainability.
- Real-world Modeling: Structures in C facilitate the representation of real-world entities, such as employees, students, or geometric shapes, by organizing their attributes into a structured format. This simplifies the modeling of complex systems and entities in software applications.
- Complex Data Structures: Structures in C allow the creation of complex data structures, such as linked lists, trees, and graphs, by combining multiple instances of structured data. These data structures are fundamental for implementing various algorithms and data processing techniques.
- Improved Code Readability: Using structures improves code readability by providing meaningful names for data members. This makes the code self-documenting and easier to understand, especially for other programmers who may work on the code later.
- Code Reusability: Using structure in C programs promotes code reusability by encapsulating data and operations within a single unit. Once defined, a structure can be used in multiple parts of the program without the need to redefine its structure or members.
- Passing Complex Data to Functions: Structures in C enable the passing of complex data to functions as a single argument. This simplifies function interfaces and parameter lists, making it easier to work with functions that require multiple pieces of related data.
- Memory Allocation and Efficiency: Structures in C provide a convenient way to allocate memory for related data items, either statically or dynamically. This helps optimize memory usage and improve program efficiency by organizing data in a structured manner.
- Hierarchical Data Representation: Structures support nested structures, allowing the representation of hierarchical data relationships. This is useful for modelling complex entities with multiple levels of attributes or components.
- Flexibility and Extensibility: Structures offer flexibility and extensibility, allowing developers to add or modify data members as needed to accommodate changing requirements. This flexibility makes structures adaptable to evolving software needs.
Overall, structures play a crucial role in C programming by providing a flexible and efficient mechanism for organizing, encapsulating, and manipulating data in a structured manner. Their usage enhances code organization, readability, and maintainability, making them indispensable in software development.
Disadvantages of Structure in C Programming
While structures offer numerous benefits in C programming, they also come with certain disadvantages. Some common disadvantages of a structure in C are:
- Memory Overhead: Structures in C can introduce memory overhead due to padding and alignment requirements. This overhead can become significant when dealing with large structures or when memory usage needs to be optimized.
- Limited Abstraction: Structures in C provide a basic form of abstraction, but they may not be as flexible or powerful as other data abstraction mechanisms available in modern programming languages. For example, they lack features like inheritance and polymorphism found in object-oriented languages.
- No Built-in Encapsulation: C does not provide built-in support for encapsulation, so there's no inherent protection mechanism for structure members. This can lead to accidental modification of structure members by external code, potentially causing unexpected behavior.
- No Built-in Methods: C does not support methods or functions directly associated with structures. While it's possible to define functions that operate on a structure in C programming, they are not inherently linked to the structure itself, unlike in object-oriented languages where methods are part of classes.
- Limited Type Safety: C structures do not enforce type safety at compile-time. This means that it's possible to perform operations on structures that may lead to undefined behavior or memory corruption if not handled carefully.
- Complexity in Nested Structures: Handling a nested structure in C can become cumbersome, especially when dealing with deeply nested structures or complex data relationships. This complexity can make code harder to understand and maintain.
- No Direct Support for Inheritance: C does not provide native support for inheritance, making it more challenging to implement complex inheritance hierarchies or reuse code across different structure types.
- Limited Runtime Checking: C compilers typically perform minimal runtime checking on structure operations, such as boundary checks or type validation. This can lead to runtime errors or memory corruption if structures are accessed improperly.
Conclusion
A structure in C is a user-defined data type that allows developers to organize and manage data efficiently. Through structures, C programmers can group related data elements under a single name, making code more readable, organized, and maintainable. Structures in C facilitate the creation of complex data structures such as linked lists, trees, and graphs, enabling the implementation of various algorithms and data processing tasks.
- Structures in C also offer flexibility by supporting nested structures, pointers to structures, and dynamic memory allocation, allowing for the creation of complex data structures and dynamic data manipulation.
- They also play a crucial role in interfacing with hardware and external data formats, making them indispensable in system-level programming and embedded systems development.
Programmers can write clearer and more modular code that is easy to understand, as well as maintain and extend it by using the concept of structure in C programming.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. List some differences between the c variable, c array, and c structure.
Here are some key differences between C variables, arrays, and structures:
Aspect | C Variable | C Array | C Structure |
---|---|---|---|
Definition | Represents a single data item | Represents a collection of multiple data items of the same type | Represents a collection of related data elements of different types |
Data Storage | Single value | Multiple values | Multiple data elements |
Accessing Elements | Accessed directly by name | Accessed using indices | Accessed using member names |
Memory Allocation | Fixed-size memory location | Contiguous memory locations | Memory for each member |
Homogeneity/ Heterogeneity | Homogeneous (single data type) | Homogeneous (same data type) | Heterogeneous (different data types) |
Declaration | Name and data type | Name and size | Name and member names/types |
Initialization | Single value | List of values | Initialization per member |
Memory Access/ Manipulation | Direct manipulation and access | Element access, iteration, manipulation | Member-wise access, manipulation, traversal |
Usage | Storing individual data items | Storing and processing collections of data items | Representing complex data structures, organizing related data elements |
Q. What are some common uses of structures in C?
Common uses of structures include representing records (e.g., employee records), managing data for applications, creating data structures (e.g., linked lists), and defining packet or protocol formats in networking.
Q. Can a structure in C contain a class?
In C programming, structures (structs) and classes are distinct concepts. While structures are used to group related data elements under a single name, classes are fundamental to object-oriented programming in languages like C++. While C++ allows for structures (structs) to be nested within classes, enabling the creation of complex data structures within class definitions, C itself does not support this feature. In C, structures are standalone entities used for organizing and managing data, and they cannot contain classes or other types of user-defined types within their definition.
Q. What is the difference between a union and a structure in C?
Aspect |
Structure |
Union |
Purpose |
A structure is used to group related data members into a single unit. |
A union in C is used to store multiple data members in a single memory location. |
Memory Allocation |
Allocates memory for all members simultaneously, taking up space for each member. |
Allocates memory for only one member at a time, sharing memory space among members. |
Data Members |
Contains data members (variables) of various data types. |
Contains data members (variables) of various data types, but only one member can be accessed at a time. |
Memory Usage |
May have padding between members for alignment, leading to potentially larger memory usage. |
Typically uses less memory as it shares the same memory location for different members. |
Member Access |
Members are accessed individually using the dot (.) operator. |
Only one member can be accessed at a time using the union name. |
Size Calculation |
The size of a structure is the sum of the sizes of its members, plus any padding. |
The size of a union is equal to the size of its largest member. |
Initialization |
Each member of a structure can be initialized individually. |
Only one member of a union can be initialized at a time. |
Use Cases |
Suitable for representing a collection of related data fields where all members are relevant simultaneously. |
Suitable for scenarios where you want to save memory by sharing memory space among multiple members, and only one member is relevant at a time. |
Access to Members |
All members can be accessed independently. |
Only one member can be accessed at a time, and changing one member affects the value of other members sharing the same memory location. |
Example |
c struct Point { int x; int y; }; struct Point p; p.x = 10; p.y = 20; |
c union Color { int red; float green; char blue; }; union Color c; c.red = 255; |
Q. Can structures be used for data serialization and deserialization in C?
Yes, structures can be used for data serialization and deserialization in C. Serialization refers to the process of converting data structures or objects into a format suitable for storage or transmission, while deserialization is the reverse process of reconstructing the original data structures from the serialized format.
In C, you can define structures to represent the data you want to serialize. You can then write functions to convert these structures into a serialized format, such as a byte stream or a formatted text file, and vice versa.
For serialization:
- You can iterate through the structure members and write them to a file or buffer in a specified format.
- This format could be binary, where the raw bytes of each member are written sequentially, or it could be text-based, where members are formatted as strings separated by delimiters.
For deserialization:
- You read data from a file or buffer in the serialized format.
- You parse the serialized data and populate the corresponding structure members accordingly.
It's essential to ensure proper handling of data types, byte order (endianness), and any necessary error checking during serialization and deserialization to ensure data integrity and compatibility across different platforms.
Q. What are the advantages of using structures in C programming?
Using structures in C programming offers several advantages:
- Organization: Structures in C help organize related data elements under a single name, enhancing code clarity and organization.
- Readability: They improve code readability by encapsulating data elements and providing meaningful names for easy understanding.
- Encapsulation: Structures in C support data encapsulation, hiding internal details and exposing only necessary interfaces, promoting modularity and reusability.
- Complex Data Handling: Structures in C facilitate the creation of complex data structures like linked lists and trees, allowing for efficient handling of intricate data relationships.
- Dynamic Memory Allocation: They enable dynamic memory allocation, useful for managing variable-sized data structures in C and dynamic data manipulation.
- Functionality: Structures in C can be passed as function arguments and returned as function results, simplifying program logic and enabling efficient data processing.
- Efficient Access: Once defined, the members of a structure in C can be accessed directly, facilitating efficient data access and manipulation.
Here are a few other topics you must explore:
- Pointer Arithmetic In C & Illegal Arithmetic Explained (+Examples)
- Bitwise Operators In C Programming Explained With Code Examples
- Nested Loop In C | For, While, Do-While, Break & Continue (+Codes)
- Jump Statement In C | Types, Best Practices & More (+Examples)
- Break Statement In C | Loops, Switch, Nested Loops (Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment