Nested Loop In C | For, While, Do-While, Break & Continue (+Codes)
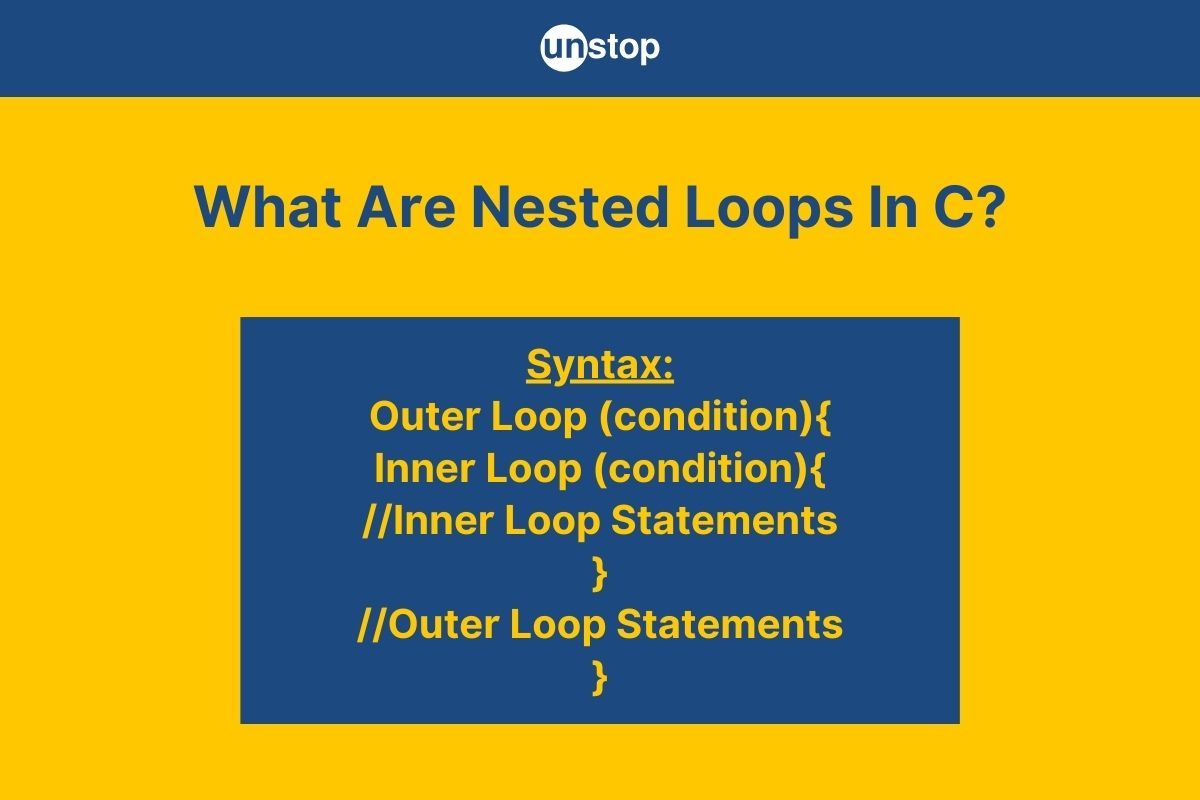
Loops are control statements in programming that iteratively repeat specific tasks without having to write the code every time. They're like your automated helpers, making your code more efficient by doing things multiple times without you having to spell it out every single time. In this article, we will discuss a special type of loop called the nested loop in C programming language.
A nested loop is when you have loops within loops, sort of like fitting a piece of code inside another. We will explore the three types of nested loops, i.e., for, while, and do-while, along with some best practices to consider when working in a nested loop in C programming. We will also discuss the use of other statements like break and continue to create looping structures that meet your specific requirements.
What Is A Nested Loop In C?
Nesting refers to enclosing a certain programming element within a similar one. For instance, you can have nested loops, nested structures, nested functions, etc.
- When we talk about a nested loop in C programming, it means having one loop statement inside another. That's why they're often referred to as loops inside loops.
- There is no limit to the number of loops you can put inside the one loop. However, it is best to avoid excessive nesting to keep things simple for code readability and maintainability.
- The level of nesting of loops can be defined at any number, denoted as 'n times.'
- You can nest any type of loop within another, i.e., for loop, while loop or the do-while loop.
- Note that the inner loop will run to completion for every iteration of the outer loop, creating a powerful mechanism for handling complex programming scenarios.
Nesting creates a hierarchical structure, enabling the execution of repetitive tasks within another loop. Nested loops in C are particularly useful when dealing with multidimensional or complicated data structures or when a certain operation needs to be repeated in a specific pattern.
Syntax Of Nested Loop In C
Outer_loop {
Inner_loop{
// inner loop statements
}
// outer loop statements
}
Here,
- As is evident, the terms outer_loop and inner_loop refer to the outer loop and the inner loop nested inside the outer, respectively. You must use keywords for, while or do (do-while) to replace these, depending on the type of loop.
- The loop statements refer to the lines of code that are a part of the outer and the inner loop body.
- Outer loop statements: The outer loop has its own set of statements enclosed within curly braces {}. It controls the execution of the entire block, representing the broader or higher-level iteration.
- Inner loop statements: The inner loop has its own set of statements enclosed within its curly braces {}. It controls a more specific or lower-level iteration, executing its statements multiple times for each iteration of the outer loop.
General Example Of Nested Loop In C
Imagine you are organizing a music festival with multiple stages, each hosting different artists. Now, you want to create a schedule that lists the performances for each stage on each festival day. In the context of programming, think of each stage as an outer loop, representing days, and each artist's performance as an inner loop, representing time slots on that particular day.
This scheduling task can be efficiently accomplished using nested loops in C as follows:
- Outer Loop (Days): Iterate through each day of the festival.
- Inner Loop (Time Slots): Iterate through the time slots for each day to schedule the performances.
Types Of Nested Loop In C
There are 3 types of loops and, hence, 3 types of nesting loops in C programming. These include:
- Nested Do-while Loop
- Nested For Loop
- Nested While Loop
The choice of which loops to use depends on the specific requirements or the task at hand. Let's explore each of these nested loop types in detail.
Check out this amazing course to become the best version of the C programmer you can be.
Nested Do-While Loop In C
A do-while loop is one where the code statements in the body are implemented first, and then the condition is checked to determine if the next iteration will happen. A do-while nested loop in C then, when one (or more) do-while loop is nested inside a main do-while loop. This type of loop is useful when you want to ensure that the loop body is executed at least once, as the condition is checked after the loop execution.
Use Cases for Do-while Nested Loop in C:
- Menu-Driven Programs: Nested do-while loops are often used in menu-driven programs where users can choose different options.
- Input Validation: When input validation is required, nested do-while loops can be employed to ensure the user provides valid input.
- Initial Execution Necessary: Situations where an action needs to be performed at least once, and then the repetition is based on a certain condition.
Syntax Of Nested Do-While Loop In C:
do {
// Outer loop statements
do {
// Inner loop statements
} while (inner_condition);
// More outer loop statements (Optional)
} while (outer_condition);
Here,
- The do keywords mark the beginning of the do-while loops, and the curly braces {} contain the loop body.
- Outer loop statements refer to the code sequence inside the main loop followed by an inner loop.
- The inner loop statements refer to the code body of the inner do-while loop.
- The while keywords mark the beginning of the condition block, where the inner_condition and the outer_condition refer to the condition for the inner and outer do-while nested loop in C.
- Note that if the inner_condition is true, the inner loop will keep iterating, and the flow will pass to the outer loop only when this condition is evaluated as false.
Working & Flowchart Of Nested Do-While Loop In C
The flowchart above represents the control flow/ logical steps the program takes when in a do-while nested loop. The working mechanism for this type of nested loop in C is as follows:
- Outer do-while Loop Body: The program enters the nested do-while loop and executes the loop body of the outer loop. This body will also contain the inner loop.
- Inner do-while Loop Body: The program enters the inner loop and executes the code statement inside the loop body.
- Inner Loop Condition Check: After the execution of statement(s) inside the inner loop body, the program moves to evaluate the inner loop condition. If the condition is true, the inner loop keeps iterating. If the condition is false, the flow moves to the next line in the outer loop.
- Outer Loop Condition Check: After moving out of the inner loop, the program evaluates the outer loop condition.
- If the condition is true, the control repeats the process of executing outer and inner loop bodies.
- The outer loop runs until the condition returns false, at which point the program exits the nested loop.
Example Of Do-While Nested Loop In C
Look at the simple C program example below to better understand the concept of nested do-while loops. Here, we will use the do-while nested loop in C to print a rectangle pattern of asterisks (*), where user input specifies the rectangle's dimensions.
Code Example:
Output:
Enter the width: 6
Enter the length: 3
* * * * * *
* * * * * *
* * * * * *
Explanation:
In the C code example, we first include the essential header files <stdio.h> for input-output (i.e., printf and scanf) functions.
- We define the main() function, which is the entry point of our program.
- Inside, we declare two integer variables, width and length, to store the dimensions of a rectangle.
- Then, we use the printf() function to prompt the user to provide variable values and read the input using the scanf() function.
- Here, the %d format specifier indicates an integer type value, and the address-of operator (&) helps store the value in the variable's location.
- Next, as mentioned in the code comments, we create a nested do-while loop where the control variable for the outer do-while loop is integer variable i, initialized to 1.
- The outer loop will run as long as i is less than or equal to the length variable, i.e., the outer loop condition is i<= length.
- Inside this outer loop, we have an inner do-while loop with the loop control variable j initialized to 1. This loop will run as long as j is less than or equal to width, i.e., j<= width.
- The inner loop body contains a printf() statement to print the star symbol, after which the value of j is incremented by 1.
- After that, the flow moves to the while-block of the inner loop where the loop checks the condition.
- If the condition j<=width is true, the flow moves back to the do-block of the inner loop.
- If the condition is false, the flow moves outside the inner loop to the next line in the outer loop.
- After that, the printf() statement with the newline escape sequence shifts the cursor to the next line, and then the value of i is incremented by 1.
- The flow moves to the while-block of the outer loop and checks the condition.
- If the condition is false, the program exits the do-while nested loop.
- If the condition is true then the outer loop iterates again, repeating the process for the inner loop as well.
- The outer loop continues to run, and for each value of i, the inner loop runs completely, printing stars for the specified width.
- After both loops complete iteration, the main() function terminates with a return 0 statement, indicating error-free execution.
Nested For Loop In C
A for loop is a control statement that is used to iterate over a range or implement a block of code repeatedly. This loop type is often used to iterate over, initialize, or access data structures like vectors, arrays, matrices, etc. Evidently, a nested for loop is when we have one (or more) for loop inside another for loop.
This type of nested loop in C is handy when we need to iterate over a set of elements, with each element iterating over another. It is often used when we have a predefined number of iterations, and it allows us to perform repetitive tasks efficiently.
Use Cases:
- Matrix Operations: When working with matrices, you might use nested loops to iterate over rows and columns.
- Multi-dimensional Arrays: Like matrices, nested loops are handy for traversing elements in multi-dimensional arrays.
- Pattern Printing: Nested loops are often used for printing patterns involving rows and columns.
Syntax For Nested For Loop In C:
for (initialization_expression1; condition1; update_expression1) {
// Outer Loop Statements
for (initialization_expression2; condition2; update_expression2) {
// Inner Loop Statements
}
// Rest of the Outer Loop Statements
}
Here,
- The for keywords mark the beginning of the for nested loops, with the outer loop statement and inner loop statements referring to the code sequence inside the inner and outer loops, respectively.
- The initialization_epsression1 and initialization_epxression2 refer to the expression that initializes the outer and inner loop control variables, respectively.
- Similarly, condition1 and condition2 are the loop conditions for the outer and inner loops, respectively.
- Boolean outcomes of these conditions determine if the respective loop will continue iterating or terminate.
- The update_expression1 and update_expression1 stipulate how we update the value of the outer and inner loop counter variables, respectively.
Working & Flowchart Of For Nested Loop In C
The above flowchart illustrates the execution flow inside a nested for loop in C programs. The steps in the working mechanism of the for nested loop in C are:
- Initialize Outer Loop Variable & Condition Check: When the program flow enters the nested for loop, the outer loop counter variable is initialized, and the outer loop condition is checked.
- Outer Loop Body Execution: If the outer loop condition is false, the program control exits the nested loop in C completely. If the condition is true, the loop statements in the outer loop are executed, and the inner loop is encountered.
- Inner Loop Variable Initialization & Condition Check: As the program enters the inner loop, the control variable is initialized, and the condition is checked. If the condition is false, the control moves back to the outer loop.
- Inner Loop Body Execution: If the inner loop condition is true, the statements inside the inner loop are executed.
- Update Inner Loop Counter Variable & Iteration: After the execution of loop statements, the inner loop variable is updated, and the inner loop reiterates until the condition becomes false.
- Update Outer Loop Variable: If and when the inner loop condition becomes false, the flow moves back to the outer loop and its counter variable is updated.
- Outer Loop Iteration: The outer loop condition is evaluated with the updated outer loop counter variable, and the whole process repeats.
- Nested Loop Termination: The program exits the nested loop if and when the outer loop condition turns false.
Note: The inner loop operates independently of the outer loop, executing its statements multiple times for each iteration of the outer loop. The combination of outer and inner loops allows for efficient handling of tasks that require nested iterations, such as working with matrices, multi-dimensional arrays, or printing patterns.
Example Of For Nested Loop In C
Let's look at a sample C program illustrating the use of a nested for loop. Here, we will initialize a matrix using a nested loop in C language, taking user input for a number of rows and columns and each element.
Code Example:
Output:
Enter the number of rows: 2
Enter the number of columns: 2
Enter element at position 1,1: 23
Enter element at position 1,2: 7
Enter element at position 2,1: 11
Enter element at position 2,2: 0
Matrix entered by the user:
23 7
11 0
Explanation:
In the sample C code-
- We declare two variables, rows and cols, of integer data type without initialization.
- Then, we use the printf() and scanf() functions to prompt the user to input values for the variables and store them in the respective variables using the address-of operator.
- Next, we declare a 2-dimensional array of integers called matrix with variables rows and cols representing the dimensions of the array, which will be a matrix.
- After that, we use a set of nested for loops with printf() and scanf() functions to take user input to initialize each element in the matrix. Here-
- The outer loop control variable i helps iterate over the rows from 0 to rows-1, with loop condition i<rows.
- The inner loop control variable j helps iterate over the columns from 0 to cols-1, with loop condition j<cols.
- Inside the inner loop, the printf() statement prompts the user to input a value for the element at position [i][j], and it is stored in the respective position.
- Once the matrix is initialized, we use another set of nested for loop with printf() statements to display the matrix on the console.
- Here, again, the outer loop iterates over the rows (from 0 to rows-1) and the inner loop iterates over the columns (from 0 to cols-1).
- The inner loop contains a printf() statement to print each element of the matrix. The outer loop prints a newline character (\n) to move to the next row.
- After the matrix is printed, the main() function returns 0.
In this example, we have generated a simple 2x2 matrix. You can generate matrices and other structures of varied dimensions using the for nested loop in C programs.
Nested While Loop In C
A while loop is a looping statement that checks a condition and iteratively executes a block of code till the condition returns True. Naturally, nested while loop is when we have one (or more) while loop inside an outer while loop.
The while nested loop in C is useful when repetitive tasks need to be performed, and the number of iterations is determined by conditions. Unlike the for nested loop in C, this type of loop is used when the number of iterations is unknown.
Use Cases:
- Menu-Driven Programs: In programs with menu-driven interfaces, nested while loops can be employed to repeatedly display the menu and execute corresponding actions until the user chooses to exit.
- Interactive Games: Games often involve repeated interactions with the player. Nested while loops can control game rounds, player turns, or specific game phases.
- Simulation and Modeling: Simulations or models involving multiple entities or processes might use nested while loops to represent the evolution of the system over time.
- File Processing: When reading or writing to files, nested while loops can be used to iterate through lines, characters, or specific sections of a file.
- Network Communication: In network programming, nested while loops can be employed to handle communication with multiple clients or manage different stages of a network protocol.
Syntax For Nested While Loop In C:
while (outer_condition) {// Outer while loop
// Outer loop statementswhile (inner_condition) { // Inner while loop
// Inner loop statements
// Update inner loop variable
}
// Update outer loop variable
}
Here,
- The while keywords mark the beginning of the loop with the outer_condition and inner_condition referring to the outer and inner loop conditions (respectively) to determine if the loops are executed or terminated.
- The comments- outer loop statements and inner loop statements refer to the body of the respective loop.
- Similarly, the update inner loop variable and outer loop variable refer to the updation expression for the respective loop counter variables.
Working & Flowchart Of While Nested Loop In C
The flowchart depicts the flow of control through a nested while loop in C (containing two while loops). The working mechanism for the same is as follows:
- Initialize Variables: In most cases, the loop counter variables are initialized before entering the nested loop in C program structure.
- Outer While Loop Condition Check: On entering the nested loop structure, the program control checks the outer loop condition. If this condition is false, then it exits the loop structure.
- Outer Loop Body Execution: If the condition is true, the code inside the outer loop is executed, and the control proceeds to the inner while loop.
- Inner While Loop Condition Check: On entering the inner loop, the program checks the inner while loop condition. If the condition is false, the program exits the inner loop and moves to the outer loop.
- Inner While Loop Body Execution: If the inner loop condition is true, then the inner loop body is executed.
- Update Inner Loop Variable: After executing the inner loop statements, the control moved back to the while block of the inner loop with an updated value of loop counter variable.
- Update Outer Loop Variable: If and when the inner loop condition turns false, the outer loop variable is updated, and the control moves back to the while block of the outer loop.
- Outer Loop Iteration & Termination: The program checks the outer condition, and the whole process repeats if it returns true. If the condition returns false, the program exists the nested loop.
Example Of While Nested Loop In C
In this section, we will look at an example C program that illustrates the while nested loop in C. Here, the outer loop iterates two times, and for each iteration, the inner loop also iterates twice, demonstrating the sequential execution of nested while loops.
Code Example:
Output:
Outer Loop Iteration 1
Inner Loop Iteration 1
Inner Loop Iteration 2
Outer Loop Iteration 2
Inner Loop Iteration 1
Inner Loop Iteration 2
Explanation:
In the example C code-
- We declare and initialize an integer variable outer to the value 1 inside the main() function. This is the counter variable for the outer loop.
- Then, we enter a nested while loop structure with the outer loop condition, outer<=2. This means that the outer loop will run as long as the outer variable is less than or equal to 2.
- Inside the outer loop, the printf() statement prints a string message indicating the current iteration number of the outer loop.
- Next, we initialize an integer variable inner with the value 1, which is the inner loop counter variable.
- The program then enters the inner while loop with the condition inner <= 2. This means that the loop will run as long as the inner variable is less than or equal to 2.
- The inner loop body contains a printf() statement, which displays a string message indicating the current iteration number of the inner loop.
- After that, the value of the inner variable is incremented by 1, and the inner loop reiterates until the loop condition inner<=2 becomes false.
- Following this, the control moves out of the inner loop, and the outer variable is incremented by 1.
- We once again evaluate the outer loop condition with the revised value of an outer variable. If the condition is true, the inner loop iterations begin again.
- If the condition is false, the nested loop is terminated, and main() closes with a return of 0.
Uses Of Nested Loop In C
1. Matrix Operations: Nested loops in C facilitate the traversal of each element in a matrix. Common operations include matrix addition, multiplication, and finding determinants. A nested loop in C is extremely useful for handling two-dimensional arrays representing matrices. For example:
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++){
// Matrix operations: addition, multiplication, etc.
} }
2. Pattern Printing: Nested loops in C are crucial for creating intricate patterns in the console. The inner loop controls the pattern's structure, determining the number of symbols or characters in each row. Common patterns include triangles, rectangles, or even more complex designs.
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
printf("* ");}
printf("\n");
}
3. Searching and Sorting: A nested loop in C can be employed for searching specific elements or sorting data in algorithms involving multidimensional arrays. Algorithms like bubble sort, selection sort, or binary search often use nested loops for array manipulation.
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
// Searching or sorting operations
} }
4. Table Generation: The nested loop in C is used for generating tables, such as multiplication tables. For example, the inner loop calculates and prints each row of the table, and the outer loop manages the repetition for different rows.
for (int i = 1; i <= 10; i++) {
for (int j = 1; j <= 10; j++) {
printf("%d * %d = %d\n", i, j, i * j);
} }
5. Image Processing: Image processing algorithms often require the use of a nested loop in C programs for iterating through pixels in two-dimensional arrays. Operations like filtering, convolution, or color adjustments are applied within nested loops for each pixel.
for (int i = 0; i < imageHeight; i++) {
for (int j = 0; j < imageWidth; j++) {
// Image processing operations
} }
6. Simulation and Modeling: The nested loop in C also helps in simulations, i.e., they help simulate different dimensions or time steps. Examples include simulating physical systems, population growth, or weather models. The inner loop may represent spatial dimensions, while the outer loop controls the simulation over time.
for (int time = 0; time < simulationTime; time++) {
for (int i = 0; i < simulationSpace; i++) {
// Simulation operations
} }
Break Inside Nested Loop In C
The break statement is a jump statement that causes the program to terminate the loop or switch statement where it appears. In other words, when encountered, it causes an immediate exit from that loop, transferring control to the statement immediately following the loop or switch.
In a nested loop in C program, the break statement will cause the program to exit the inner loop immediately when encountered. We generally use the break statement with an if conditional statement to set the condition that will lead to break being encountered.
Syntax of Break Inside a Nested Loop in C:
Outer Loop {
Inner Loop {
// Loop statements
if (condition) {
break;
}
// Loop statements
}}
Here,
- The outer loop and inner loop make the nested loop structure in C, and the loop statements refer to the lines of code that make up the loop body.
- If keyword marks the beginning of the conditional statement and the condition is the statement condition to determine where the body will be executed or not.
- The break keyword marks the break statement, which will cause the termination of the loop where it exists (in this case, the inner loop).
Let's look at a C program sample to better understand this concept.
Code Example:
Output:
i = 1, j = 1
i = 1, j = 2
Break condition encountered
i = 3, j = 1
i = 3, j = 2
Explanation:
In the C code sample-
- We create a nested for loop structure in the main() function.
- Here, the outer for loop has counter variable i, initialized to 1. This loop iterates until its value remains less than equal to 3, i.e., i<=3, and the value of i is incremented by 1 after every iteration (i++).
- Inside the outer loop, we have an inner for loop with an integer counter variable j initialized to 1.
- This loop runs till the value of j is less than or equal to 3, i.e., j<=2 and the value of j is incremented by 1.
- Next, we have an if-statement inside the inner for loop with the condition i==2 && j ==2.
- Here, we use the relational equality operator to check if the value of i is equal to 2 and j is equal to 1, with conditions connected using the logical AND operator.
- If the condition is true, the if-block is executed, and the message- Break condition encountered is displayed on the console.
- The block also contains a break statement, which causes the program to exit the inner loop immediately, skipping any remaining iterations of the inner loop for the current value of i.
- If the condition is false, the flow moves to the next line of the nested loop structure.
- Outside the if-statement, the printf() statement displays a string message with the values of counter variables i and j.
- In this example, the break statement is encountered when i==2 and j==1, resulting in the iteration of the second cycle not being executed.
Continue Inside Nested Loop In C
The continue statement is another jump statement that alters the regular flow of a program. When encountered in a loop, the continue statement causes the program to skip the rest of the code inside a loop for the current iteration and move to the next iteration. When encountered in the nested loop in C, it applies to the innermost loop enclosing it.
Syntax for Continue Inside Nested Loop in C:
Outer loop {
inner loop {
if (condition) {
continue;}
//Loop body
}
//Outer loop body
}
Here,
- The outer loop and inner loop make up the structure of the nested loop in C, with the loop bodies referring to the loop statements inside respective loops.
- We can use any type of loop to create the nested loop structure.
- The if-statement is used to implement the condition, which, when satisfied, leads us to encounter the continue statement, depicted by the continue keyword.
When the continue statement is encountered, the program jumps to the next iteration of the loop without executing the remaining code for the current iteration. Look at the C program example below, which illustrates the use of continue inside a nested for loop structure.
Code Example:
Output:
Outer loop iteration 1
Inner loop iteration 1
Inner loop iteration 3
Inner loop iteration 5
Outer loop iteration 2
Inner loop iteration 1
Inner loop iteration 3
Inner loop iteration 5
Explanation:
In the simple C code example-
- We declare two integer variables, i and j, which will be used as loop counters at the beginning of the main() function.
- Then, we create a nested for loop structure where the outer loop has counter variable i set to 1.
- The outer loop iterates till i is less than or equal to 2, i.e., i<=2 and the value of i is incremented by 1 after each iteration.
- At the beginning of every iteration, a printf() statement displays a message to the console indicating the current iteration number for the loop.
- After that, we have an inner for loop with the counter variable j set to 1.
- The inner loop iterates till the value of j is less than equal to 5, and the value of the variable is incremented by 1 after every iteration.
- This loop contains an if-statement where the condition checks if the value of variable j is even or not, using the modulo arithmetic operator.
- If this condition is true (j is even), the continue statement in the if-block is encountered which causes the program to skip the rest of the inner loop's code and move to the next iteration of the inner loop.
- If the condition is false (j is odd), then the if-block is skipped and we move to the next line of the loop.
- Outside of the if-statement, a printf() statement prints a message indicating the current iteration number of the inner loop.
- In this example, the outer loop continues to run, and for each value of i, the inner loop runs, but only prints for odd values of j (1, 3, 5).
Best Practices When Using Nested Loop In C Programs
You must consider a few points when working with a nested loop in C programs to ensure readability, maintainability, and efficiency. Here are some best practices to consider when working with nested loop in C programming:
- Use Meaningful Variable Names: Choose descriptive variable names for loop counters and other loop-related variables to enhance code readability and understanding of the code.
- Keep Nested Loops Simple: Avoid nesting too many loops deeply. Deeply nested loops in C can make code hard to understand and debug. If possible, refactor complex nested loops into separate functions or simplify the logic.
- Indentation and Formatting: Use proper indentation and formatting to visually indicate the nesting level of loops. Consistent indentation makes it easier to understand the code's structure.
- Limit Loop Scope: Declare loop variables in the narrowest scope possible to avoid unintentional variable reuse or modification outside the loop.
- Optimize Loop Conditions: Optimize loop conditions to minimize unnecessary iterations. If possible, pre-calculate loop bounds or use loop conditions that can be determined at time of compilation.
- Avoid Redundant Computations: Minimize redundant computations within nested loops in C. If a computation result remains constant within nested loops, calculate it outside the inner loop to improve performance.
- Break and Continue with Caution: Use break and continue statements judiciously within a nested loop in C. Overuse of these statements can make code harder to follow. Consider whether restructuring the loop logic can achieve the same result more clearly.
- Consider Performance Implications: Be aware of the performance implications of a nested loop in C, especially when dealing with large datasets. Profile your code to identify performance bottlenecks and optimize critical sections if necessary.
- Comment Nested Logic: Add comments to explain the purpose of the nested loop in C program, especially if the logic is complex or non-intuitive. Clear comments help other developers understand the code's intention.
- Unit Testing: Write unit tests to verify the correctness of nested loop logic, especially when dealing with edge cases or boundary conditions. Unit tests provide confidence in the behavior of nested loops in C programs during code changes or refactoring.
- Code Reviews: Conduct code reviews to gather feedback on nested loop usage. Peer reviews can help identify potential improvements, ensure adherence to coding standards, and catch logic errors.
Difference Between Single & Nested Loop In C
The table below highlights the difference between a single/ simple loop and a nested loop in C.
Criteria |
Single Loop |
Nested Loop |
Definition |
A loop that contains a set of statements |
A loop within another loop, creating a loop nest |
Iteration Control |
Controls the execution of a set of statements |
Controls the execution of a set of loops in a hierarchical manner |
Complexity |
Simpler in structure and logic |
More complex due to additional levels of iteration |
Use Cases |
Suitable for tasks that involve iterating over a single set of elements or performing a specific action multiple times |
Used when a task involves repeated operations within a set of operations, such as iterating over multiple sets of elements |
Syntax |
for (int i = 0; i < n; i++) { |
for (int i = 0; i < m; i++) { |
Indentation and Formatting |
Relatively simpler due to fewer levels of indentation |
Requires careful indentation to maintain readability, with each additional level indented further |
Readability |
Generally more readable and easier to understand |
May become harder to read and comprehend as nesting levels increase |
Execution Time |
Typically faster as there is less overhead |
May incur additional overhead, especially with deep nesting |
Scope of Variables |
Variables are scoped within the loop |
Inner loop variables can affect the outer loop, and vice versa |
Control Statements |
Common control statements like break and continue are straightforward |
The use of control statements requires careful consideration of their impact on both inner and outer loops |
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Conclusion
A nested loop in C is a control structure where we have one or more loops nested inside a main/ outer loop. There are three types of nested loops including do-while, for, and while loops. You can determine which type of loop meets your requirements depending on the syntax, structure and problem at hand.
We can also use other control/ jump statements inside a nested loop in C to alter the natural flow and meet specific requirements. For example, if you want to skip the iteration of the inner loop for specific cases, then you can use a continue statement inside the nested loop structure. Note that while there is no set limit to the number of loops you can nest, deep nesting is usually not recommended as it makes the code more complex and harder to read and maintain. We have shared a few best practices to help you make the most of a nested loop in C programs.
Also read: 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. Are there any performance considerations in nested for loops in C?
Nested for loops can impact performance, especially if the number of iterations is large. Careful consideration should be given to algorithmic efficiency. Additionally, optimizing compilers may perform loop unrolling or other optimizations to improve performance. Always benchmark and profile your code to identify performance bottlenecks.
Q. How do I avoid redundancy when using nested loop in C programming?
Here are a few points to note to avoid redundancy when working with a nested loop in C programs:
- Encapsulate Repetitive Code: Use functions to encapsulate repetitive code, promoting modularity and readability.
- Break Down Complex Logic: Divide complex logic into smaller, well-named functions, each responsible for a specific task.
- Meaningful Variable Names and Comments: Use descriptive variable names and comments to clarify the purpose and functionality of the nested loops.
- Refactor and Simplify: Regularly refactor your code to simplify and reduce redundancy.
Q. What are the factors that affect the performance of a nested loop in C?
The performance of a nested loop in C can be influenced by various factors. Whether they are slow or not depends on the specific context and how efficiently they are implemented. Here are some considerations:
- Number of Iterations: The total number of iterations in nested loops directly affects the execution time. As the number of iterations increases, the execution time generally increases proportionally.
- Algorithm Complexity: The complexity of the algorithm within the nested loop in C matters. If the algorithm has a high time complexity (e.g., O(n^2) or worse), the overall performance may be slower, especially for large inputs.
- Hardware and Compiler Optimization: Modern compilers and hardware may perform optimizations that can mitigate the overhead of nested loop in C programs. Compiler optimizations and parallel processing capabilities can significantly improve performance.
- Code Structure: Well-structured and optimized code is crucial. Redundant computations, unnecessary calculations, or inefficient code within the loops can contribute to slower performance.
- Cache Utilization: Nested loops in C can impact cache utilization. If the data accessed in the inner loop doesn't fit well in the cache, it may result in cache misses, affecting performance.
Q: What is a tightly nested loop?
A tightly nested loop in C refers to a situation where multiple loops are nested or placed inside one another with no code or statements between them. In other words, the inner loop is directly enclosed by the outer loop, with no intervening statements or operations.
Tightly nested loops in C are commonly used in algorithms and programs where multi-dimensional data structures or grids need to be traversed or processed. They are essential for tasks like matrix manipulation, searching, and sorting.
Code Example:
Output:
1 1 1
1 1 2
1 1 3
1 2 1
1 2 2
1 2 3
Explanation:
In this example, there are three tightly nested for loops. The innermost loop (k) is enclosed by the middle loop (j), and the middle loop is enclosed by the outermost loop (i). Each iteration of the outer loop triggers the entire set of iterations of the inner loops.
Q Do loops start at 0?
In many programming languages, including C, it is common for loops to start at 0, especially when iterating over arrays or other data structures. This convention aligns with the way arrays are indexed in these languages. Arrays are zero-indexed, meaning the first element of an array has an index of 0. So, starting the loop variable at 0 allows for convenient and consistent access to each element in the array.
Code Example:
Output:
1 2 3 4 5
Explanation:
In this example, we first initialized an array of integer called numbers with 5 elements. Then we use a for loop, with the counter variable set to 0 meaning the loop starts at 0. It prints the elements of the array and continues will i < 5, iterating over the elements of the numbers array.
Q. Which situation is best for a nested loop in C?
Nested loops are best suited for situations where you need to perform repetitive tasks or iterate over elements in multiple dimensions. Here are some common situations where a nested loop in C can be the most useful:
- Matrix Operations: Nested loops in C are often used for matrix operations where you must iterate over rows and columns. For example, you'll typically use nested loops when performing matrix addition, multiplication, or other operations.
- Image Processing: A nested loop in C is often used in image processing to iterate over pixels in a two-dimensional image. This allows filters, transformations, or other operations to be applied to each pixel.
- Searching and Sorting: Algorithms like bubble sort, insertion sort, or searching algorithms may involve the use of a nested loop in C programs. For example, searching for an element in a 2D array requires iterating over both rows and columns.
- Nested Data Structures: When dealing with nested data structures, such as an array of arrays or a list of lists, a nested loop in C allows you to traverse and access each element systematically.
You might also be interested in reading the following:
- Comma Operator In C | Code Examples For Both Separator & Operator
- Type Casting In C | Cast Functions, Types & More (+Code Examples)
- Decimal To Binary In C | 11 Methods (Examples + Complexity Analysis)
- Union In C | Declare, Initialize, Access Member & More (Examples)
- Identifiers In C | Types, Rules For Definition, & More (+Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment