Break Statement In C | Loops, Switch, Nested Loops (Code Examples)
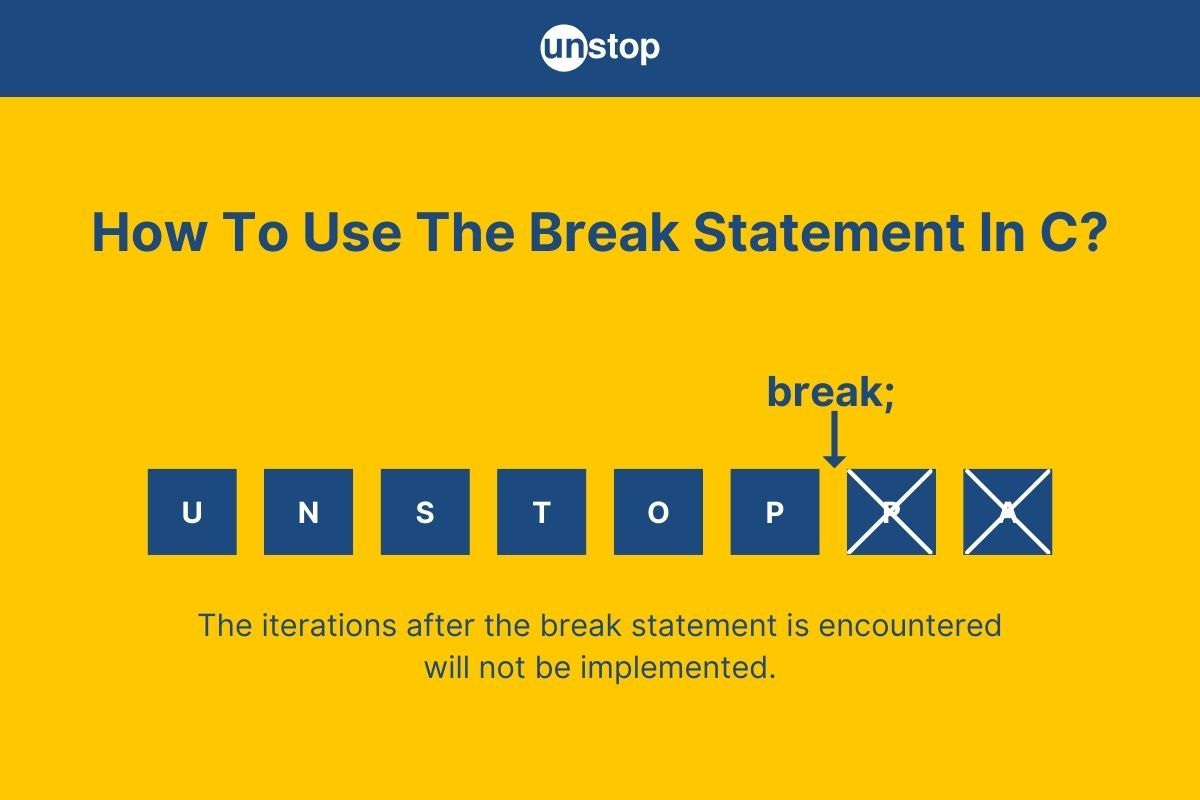
Control statements play a crucial role in determining the program control/ flow in C programming language. We use a variety of tools to write code that meets our purpose. One tool that, though understated, is an important part of coding is the break statement in C programming. Often found within loops and switch statements, the break statement allows programmers to alter the execution flow dynamically, providing flexibility and control over program behaviour.
In this comprehensive guide, we delve deep into the intricacies of the break statement in C, its syntax, applications, uses with loops and decision-making statements, best practices, and potential pitfalls.
What Is Break Statement In C?
The break statement in C programming language is a powerful tool for controlling program flow within loops and switch statements. It is one of the four jump statements in programming. Its primary function is to terminate the nearest enclosing loop control statement or switch statement immediately upon encounter. This capability allows programmers to introduce conditional exits, enhancing the flexibility and efficiency of their code.
Syntax Of Break Statement In C
break;
The statement syntax is simple, comprising the reserved keyword break followed by the terminator semi-colon. When encountered during program execution, it triggers the immediate termination of the nearest enclosing loop or switch expression.
How Does Break Statement Work In C?
The break statement in C is a control flow mechanism primarily used within loops (for, while, do-while) and switch statements. Its fundamental function is to prematurely terminate the nearest enclosing loop or switch statement when encountered. Here's how it works:
- Encounter/ Immediate Termination: When the program execution encounters a break statement within a loop or switch statement, the action associated with the break is triggered. That is, the execution of the nearest enclosing loop condition or switch statement is immediately terminated.
- Exit Control: The termination caused by the break statement bypasses any remaining iterations of the loop or switch statement. And the control exits the respective loop/ statement.
- Control Transfer: Once the loop or switch case block is terminated by break, control is transferred to the statement immediately following the terminated construct.
- Loop Iteration Control: In the context of loops, the break statement allows for conditional exits based on specific runtime conditions. It effectively skips any further iterations of the loop and continues executing the statement after the loop.
- Switch Case Management: Within a switch statement, break ensures that execution halts upon encountering a matching case, preventing the flow from falling through to subsequent cases.
- Flexibility and Adaptability: The behaviour of the break statement in C is dynamic and responsive to runtime conditions. It provides programmers with flexibility in controlling program flow based on changing circumstances.
- Code Readability and Maintainability: While break offers powerful control flow capabilities, its excessive or improper use can lead to code complexity and reduced readability. Thus, using break judiciously is essential, focusing on enhancing code clarity and maintainability.
Switch Case & Break Statement In C
Ever played a game where you get multiple choices at each step, and your actions shape the outcome? That's precisely what the switch statement does- it lets your program decide based on different options. Combining a break statement with this is like having a quick exit button whenever needed.
In other words, the break statement in C inside the switch case stops the switch statement as soon as it appears in a switch block and avoids running any more case statements afterwards.
Syntax:
switch (expression) {
case constant1:
// Code block
break;
case constant2:
// Code block
break;
// Additional cases
default:
// Default code block
}
Here,
- The switch keyword marks the beginning of the switch statement, and the (expression) is a statement condition whose evaluated value is compared with the values of various case labels inside the switch statement.
- The case keyword marks the various cases against which statement value is compared, and the constant1 refers to the constant value for that case.
- //Code block refers to the block of code to be executed is a case matches the switch expression.
- The break keyword marks the break statement in C, which terminates the execution of the switch statement.
- It is placed inside every case to ensure that the statement terminates as soon as a case match is found. Without a break statement, execution would continue to the next case label, leading to unintended behaviour.
- The term default is used to specify a block of code to be executed if none of the case labels match the expression. It serves as a catch-all mechanism.
Let's look at a simple C program that showcases how a break statement works inside a switch case statement.
Code Example:
Output:
Choose an option (1-3):
1
Option 1 selected.
Explanation:
In the C code example, we first include the standard header file for input/output functions, i.e., <stdio.h>.
- In the main() function, we declare an integer variable choice to store the user's selection.
- Next, we prompt the user to choose an option by displaying the string message- Choose an option (1-3), using a printf() statement.
- Once done, we use the scanf() function to read user input and store it in the choice variable using the address-of operator (&).
- Then, as mentioned in code comments, we employ a switch-case statement to check the value of choice, i.e., what the user picked.
- If case 1 is met, then it prints the message- "Option 1 selected." and the break statement in that case causes the program to break out of the switch statement.
- If case 2 is met, the message "Option 2 selected." is printed, and the statement terminates.
- If case 3 is met, then the message "Option 3 selected." is printed before the statement terminates.
- If none of the cases are matched, then the default code block prints the message- "Invalid choice.".
- Lastly, the main() function closes with a return 0 statement indicating the successful execution of the program.
- The %d format specifier used in the code represents an integer value, and the newline escape sequence (\n) shifts the cursor to the next line in the output window.
Loops & Break Statement In C
Loops are control flow structures that allow a set of statements to be executed repeatedly based on a condition. They are essential for performing repetitive tasks efficiently in a program. There are three main types of loops in C:
- The For Loop: Used when the number of iterations is known beforehand.
- The While Loop: Executes a block of code repeatedly as long as a specified condition is true.
- The Do-while Loop: Similar to a while loop, but the condition is checked after the loop body is executed, ensuring that the loop body is executed at least once.
The break statement in C is used to prematurely exit a loop when a certain condition is met, regardless of whether the loop's condition has been fulfilled. It allows for conditional termination of loop execution.
Syntax of For Loop with Break Statement in C:
for (initialization; condition; updation) {
// loop body
if (if_condition) {
break;
}
}
Here,
- For keyword marks beginning of the for loop.
- The expressions- initialization, condition, and updation define the beginning value for loop variable, the condition that needs to be satisfied for iterations to continue, and the increment/ decrement stipulations for the loop control variable for every iteration, respectively.
- If keyword marks the if-statement with the if_condition referring to the condition that must be met for the if-block to be executed.
- The if-block contains the break statement, which will cause the program to exit the loop immediately.
Syntax of While Loop with Break Statement in C:
while (condition) {
// loop body
if (condition_to_exit_loop) {
break;
}
}
Just like above, here-
- The while loop begins with the while keyword, with the term condition referring to the loop condition.
- When the if_condition in the if-statement is met, the program encounters the break statement and immediately terminates loop iterations.
- In case the if_condition is false, the loop executes normally.
Syntax of Do-While Loop with Break Statement in C:
do {
// loop body
if (if_condition) {
break;
}
} while (condition);
Here,
- The loop begins with the do keyword, followed by the loop body enclosed in curly braces {}.
- In case the if_condition, i.e. the condition for the if-statement, is true, the break statement is encountered, and the loop terminates prematurely.
- If the if_condition is false, the control moves to the while keyword and the condition closed is evaluated to determine whether the iterations will continue or not.
The example C program below illustrates how you can the break statement with the three types of loops.
Code Example:
Output:
For loop with break:
1 2 3 4 5
While loop with break:
1 2 3 4 5
Do-while loop with break:
1 2 3 4 5
Explanation:
In the example C code-
- In the main() function, we declare a variable i of integer data type, without initialization.
- We first employ a for loop to iterate through numbers from 1 to 10 and print them to the console.
- The loop control variable i is initially set to 1, and iterations continue till it is less than or equal to 10. Its value is incremented by 1 after every iteration.
- Inside the loop, we have an if-statement that checks if i equals 5 using the equality relational operator.
- If this condition is true, the break statement is encountered, and the loop does not iterate any further despite the loop condition.
- Once the for loop terminates, the printf() statement with the newline escape sequence shifts the cursor to the next line.
- Then, we set the value of variable i to 1 and create a while loop to continue iterations till the condition i <=10 remains true.
- This loop contains a printf() statement to display the value of i and then an if-statement to check if condition i==5 is true.
- If this condition is true, the break statement is encountered, which terminates the loop, and the control moves to the next line after the loop.
- After the while loop completes execution, we reassign variable i the value 1 and start a do-while loop.
- This loop runs at least once and continues as long as i is less than or equal to 10.
- The printf() statement inside the loop displays the value of i, and the if-statement checks if i equals 5.
- If true, then the break statement is executed, causing the program to exit the loop.
- Finally, the main() function returns 0 to indicate the successful execution of the program.
This example shows how the break statement in C can cause premature termination of a loop construct, irrespective of whether the loop condition is true.
Nested Loop & Break Statement In C
Nested loops are like Russian nesting dolls, i.e., where one loop snugly fits inside another. We often use this technique when dealing with data grids or when we need to repeat a task within another task. They could be likened to a maze of loops, where the break statement will be the hidden exit sign. Break is like a secret passageway to break out of multiple layers of looping whenever a certain condition is met.
Syntax:
for (initialization; condition; update) {
for (inner_initialization; inner_condition; inner_update) {
// Inner loop body
if (condition_to_exit_inner_loop) {
break;}
}// Outer loop body
if (condition_to_exit_outer_loop) {
break;}
}
Here,
- The for keyword marks the beginning of the loops. And the terms initialization, condition and update set the initial condition, continuation condition and updating condition for the loop variable of the outer for loop, respectively.
- The expressions inner_initialization, inner_condition, inner_update play the same role in the inner for loop.
- // Inner loop body refers to the block of code executed in each iteration of the inner loop while the inner_condition remains true. It can contain one or more statements.
- if (condition_to_exit_inner_loop): This is an if statement nested within the inner loop. It checks a condition that, if true, will cause the inner loop to exit prematurely.
- // Outer loop body refers to the block of code executed in each outer loop iteration while the condition remains true. It can contain one or more statements.
- if (condition_to_exit_outer_loop): This is an if statement outside both loops. It checks a condition that, if true, will cause the outer loop to exit prematurely.
The C program sample given below implements the concept of break statement inside nested for loops.
Code Example:
Output:
Example of nested loops with break:
(1, 1) (1, 2) (1, 3)
(2, 1) (2, 2)
(3, 1) (3, 2) (3, 3)
Explanation:
In the C code sample-
- We have created a nested for loop structure, where the outer loop is controlled by loop variable i, and it runs from 1 to 3.
- Inside the outer loop, we have another for loop that also runs from 1 to 3 and is controlled by loop variable j.
- The inner loop contains a printf() statement to display the values of i and j enclosed in parentheses.
- It also has an if-statement, which checks if the value of variables i and j is equal to 2. We use the AND logical operator to connect the two expressions.
- If the condition (i==2 && j==2) is false, the inner loop keeps iterating until the loop condition j <=3 becomes false.
- If the condition (i ==2 && j==2) is true, the program encounters the break statement, and the inner loop terminates immediately.
- The flow then moves back to the outer loop, and the inner loop iterations start again with the incremented value of variable i.
- The outer loop continues until i reaches 3, but if the inner loop encounters the condition specified, it breaks out of the loop.
- Finally, we return 0 to indicate the successful execution of the program.
Infinite Loop & Break Statement In C
Infinite loops are iterative constructs that continue indefinitely, executing the loop body repeatedly without an explicit exit condition. While these loops can be useful in certain scenarios, they often require a mechanism to break out of the loop to prevent infinite execution. The break statement in C provides a means to exit an infinite loop prematurely, thereby controlling the execution flow.
Syntax:
while (1) {
// Loop body
if (if_condition) {
break;}
}
Here,
- The while keyword indicates that it is a while loop, and the loop condition is (1), making it an infinite loop because the condition will always remain true.
- // Loop body is the placeholder for the block of code executed in each iteration of the loop.
- The if() is the if-statement with the condition being specified by if_condition.
- The break keyword indicates the break statement, which will be executed if the if_condition is true. That is, once break is encountered, the loop is terminated, and control moves to the statement following the loop.
The sample C program given below illustrates the concept of using a break statement inside an infinite loop for termination.
Code Example:
Output:
Example of an infinite loop with break:
1 2 3 4
Explanation:
In the sample C code-
- We initialize an integer variable i with the value 1 inside the main() function.
- Then, we enter an infinite while loop with the condition (1). This loop contains a printf() statement to print the current value of loop control variable i.
- The value of i is incremented by 1 after every iteration, and then we enter an if-statement.
- The if-statement checks if the value of i after incrementation equals 5. The loop continues iterations till the time this condition is false.
- If the if_condition is true, then the break statement is executed, which terminates the loop.
- Since herein this example, the if condition is true when i==5, the loop continues indefinitely until i reaches 5.
- Once the loop terminates, the main() function closes with return 0.
Uses Of Break Statement In C
The break statement in C is a versatile tool that finds its utility in various scenarios, offering flexibility and control over program execution. Let's explore some of its primary use cases:
- Exiting Loops Prematurely: One of the most common uses of the break statement in C is to exit a loop prematurely based on a certain condition. When the condition is met, the break statement terminates the loop, allowing the program to proceed to the next statement outside the loop's scope.
- Breaking Out of Switch Statements: In switch statements, the break statement is used to exit the switch block once a specific case has been executed. Without the break statement in C programs, control would fall through to subsequent case statements, potentially leading to unintended behaviour. By including a break after each case, you ensure that only the desired case is executed and the control exits the switch block afterwards.
- Implementing Menu-Driven Programs: The break statement in C is also used in menu-driven programs where users are presented with a list of options. It is employed to exit the loop after executing the selected option, allowing the running C program to resume its main execution flow.
- Error Handling: Break statement in C can be employed for error handling purposes, allowing you to gracefully exit a loop or switch block in case of unexpected errors or invalid conditions. By strategically placing break statements within error-checking code, you can efficiently handle exceptional situations and maintain the stability of your program.
- Terminating Infinite Loops: The break statement in C offers a convenient means of breaking out of an infinite loop if it is intentional. This prevents the program from getting trapped in a never-ending loop.
- Implementing Search Algorithms: Break statements are often used in search algorithms such as linear search or binary search. Once the target element is found, a break statement in C is employed to exit the loop early, as further iterations would be unnecessary.
Advantages & Disadvantages Of Break Statement In C
Here are some of the most important advantages and disadvantages of the break statement in C that you must be aware of.
Advantages Of Break Statement In C
- Loop Termination Flexibility: The break statement in C provides you with the flexibility to end loops prematurely if the required condition is met (i.e., a set purpose is met). This means you don't waste time looping through unnecessary steps.
- Clearer Code Structure: By using break statements wisely, we make our code easier to follow. They act as signposts, marking where the loop exits, making it simpler for others (and our future selves) to understand what's happening.
- Quick Error Response: When something goes wrong inside a loop, the break statement in C gives us a way to bail out (and fast). This allows us to swiftly handle errors without getting bogged down in unnecessary iterations.
- Tailored Control: The use of a break statement in C programs provides an alternative way of exiting a loop. We can leave based on specific conditions, whether it's hitting a certain count, encountering a particular value, or meeting our custom criteria. It's like having a key to unlock the loop when we need it.
Disadvantages Of Break Statement In C
- Readability Concerns: While the break statement in C can enhance code clarity when used appropriately, excessive or improper usage can negatively impact code readability. For example, nesting multiple break statements or using them in complex loop structures may obscure the logic of the code, making it harder to understand and maintain.
- Potential Bugs: Incorrect usage of the break statement in C can introduce bugs or unintended behaviour into the code. Careless placement of break statements may cause loops to exit prematurely or skip essential processing steps, resulting in logic errors or unexpected program behaviour.
- Flow Disruption: The break statement in C disrupts the natural flow of control within loops, potentially complicating program logic. In some cases, excessive reliance on break statements may indicate suboptimal loop design or the need for refactoring to improve code structure and maintainability.
- Overuse Concerns: Overusing the break statement in C can indicate a code smell and may signal underlying design issues. While they offer a convenient means of loop termination, excessive reliance on them may indicate a lack of clarity in the program's control flow or an opportunity for refactoring to simplify the logic.
Conclusion
In summary, the break statement in C is a vital tool for controlling program flow within loops, switch statements, and other control statements. It allows for the premature termination of loops or switch blocks when specific conditions are met.
In other words, the break statement in C saves us from endless iterations and helps us make at least a bit of sense of complex logic. It's like having a reliable buddy to help while coding iterative problems, always ready to lend a hand when things get tricky. But like any tool, the break comes with its own set of cons. While it can simplify our code and make it more readable, we need to use it wisely to avoid creating tangled knots of logic that are hard to untangle.
So, you must strategically integrate the break statement in C to enhance code efficiency and adaptability. Mastering its usage ensures precise control over loop iterations and switch evaluations, fostering clearer and more expressive code.
Frequently Asked Questions
Q. What is a switch in C?
In C programming, a switch statement is a control statement that allows you to select one of many possible blocks of code to be executed. If a match is found, the corresponding block of code is executed. It provides an efficient and readable alternative to using multiple if-else statements.
Syntax for Switch Case in C:
switch (expression) {
case label 1:
//code block 1
break;
case label 2:
//code block 2
break;
default:
//default_code block
break;}
Q. Can break statements in C be used outside of loops or switch statements?
No, the break statement in C cannot be used outside of loops or switch statements. It's designed specifically to control the flow within these constructs. Attempting to use break outside of loops or switch statements will result in a compilation error.
Q. What happens if the break is used within nested loops?
When break is used within nested loops in C, it only terminates the loop inside which it is placed. For example, if it is placed inside the inner loop, it will terminate the subsequent iterations of that loop when encountered. The control then passes to the statement immediately following the terminated loop. However, the outer loops continue their normal execution unaffected by the break statement. This behaviour allows you to selectively exit from nested loops while leaving the outer loops intact and continuing their iterations.
Q. How to use the break statement with an if-statement in C?
The break statement in C is typically used within loops or switch statements to exit the loop or block when a certain condition is met. To use a break with an if statement, you would first check the condition using the if statement and then place the break statement inside the if block to exit the loop or block if the condition is true.
Code Example:
Output:
Enter a number: 5
Enter a number: 0
Enter a number: -5
Sum = 5.00
Explanation:
- The program prompts the user for a number and checks if the number entered is negative using an if-statement.
- If a negative number is entered, the loop exits immediately.
- Otherwise, it adds the non-negative number to the running total.
- This process continues until a negative number is entered.
- After the loop ends, it displays the sum of all non-negative numbers entered.
Q. Is there any way to skip the remaining iterations of the current loop and continue with the next iteration without exiting the loop entirely?
Yes, you can use the continue statement to skip the remaining code within the current iteration of a loop and proceed directly to the next iteration. This allows you to bypass certain iterations while continuing to loop through the rest of the elements. The continue statement in C is particularly useful when you want to skip specific iterations based on certain conditions without exiting the loop entirely.
Code Example:
Output:
1 2 4 5
Explanation:
- In this example, we have a for loop that iterates from 1 to 5 using the loop variable i. Inside the loop, we check if the value of i is equal to 3 using the if statement.
- If i is indeed equal to 3, the continue statement is executed, causing the current iteration to be skipped.
- As a result, the value of i is not printed for that iteration.
- For all other iterations where i is not equal to 3, the printf statement prints the value of i. Therefore, the output of the program excludes the number 3 from the sequence.
Q. Is break a function in C?
No, the break statement in C is not a function. It is a control statement used to exit from a loop or switch block prematurely. When encountered within a loop or switch statement, the break statement in C immediately terminates the loop or block and transfers control to the following line.
Here are a few more topics you must explore:
- C Program To Reverse A Number - 6 Ways Explained With Examples
- Convert Binary To Decimal In C | 4 Ways Explained (+Code Examples)
- Transpose Of A Matrix In C | Properties, Algorithm, Examples & More!
- Swapping Of Two Numbers In C | 5 Ways Explained (With Examples)
- Operators In C Programming: Explained With Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment