Continue Statement In C | Working & Use Explained (+Code Examples)
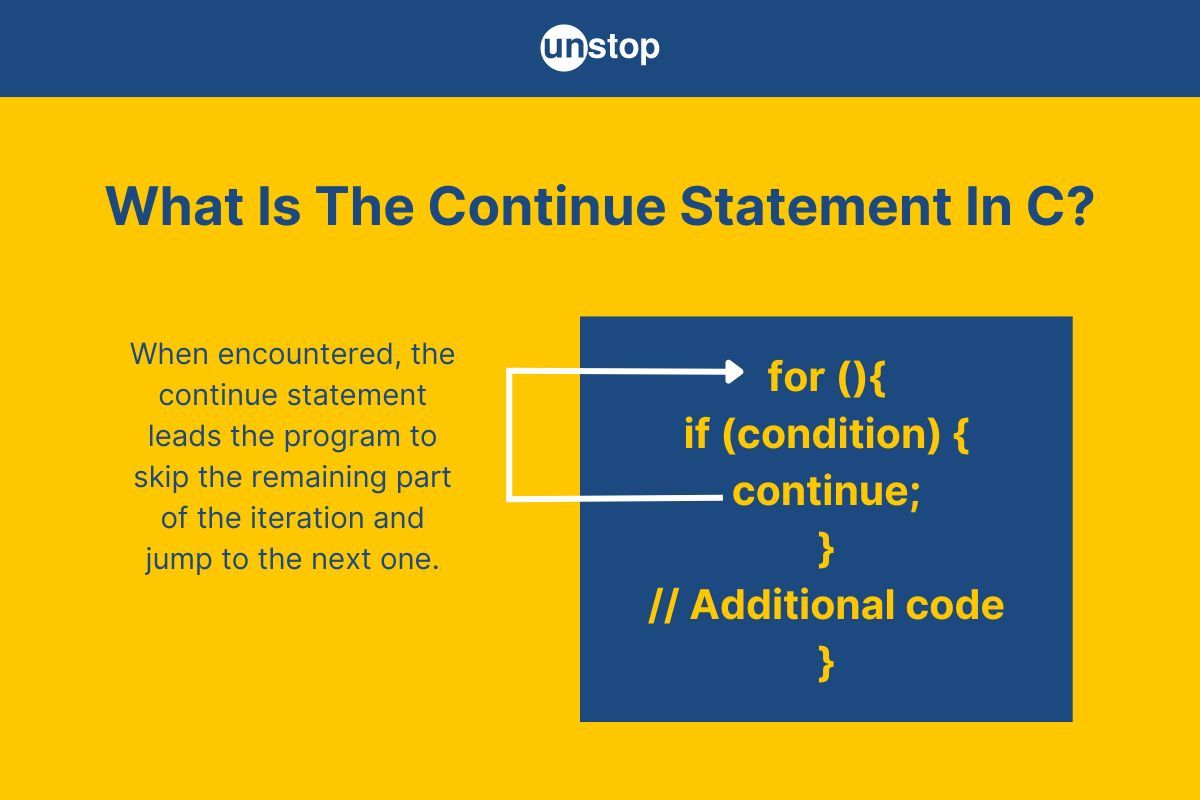
Control statements in programming languages are constructs that alter the flow of program execution based on certain conditions or criteria. They allow programmers to alter the natural program control flow, i.e., alter the sequence in which instructions are executed. The continue statement in C programming language is one construct that is used to control loop flow. When encountered, it makes the program skip the correct iteration and jump to a certain place in the loop (usually the new iteration).
In this article, we delve into the intricacies of the continue statement in C, exploring its syntax, functionality, capabilities, and practical applications.
What Is Continue Statement In C?
The continue statement is a loop control statement, which, when used within loops, causes the program to skip the remaining statements in the current loop iteration and proceed to the next one. It is especially useful when a programmer wants to skip a specified section of the loop body depending on predetermined conditions without exiting the entire loop structure.
Key Points:
- The continue statement is primarily used within loop constructs.
- It skips the remaining statements in the current iteration and proceeds to the next iteration of the loop.
- The continue statement in C can be useful for skipping certain iterations based on specific conditions, thus controlling the normal flow of the loop.
- It does not terminate the loop but only affects the current iteration.
Syntax Of Continue Statement In C:
continue;
Here, the continue keyword is used to signal the continuation of the loop. It is followed by a semicolon (;) to terminate the statement. Below is a simple C program that illustrates the continue statement and its use.
Code Example:
Output:
2
4
6
8
10
Explanation:
In the simple C code, we first include the essential header file for input-output operations (i.e., <stdio.h>).
- Inside the main() function, we declare a variable i, of integer data type without initialization.
- We then create a for loop to iterate over numbers from 1 to 10. The loop control variable i begins from, and the loop runs until i<=10.
- The loop update expression increments the value of i by 1 after every iteration.
- Within this loop, we have an if-statement that checks if the current value of i is odd using the modulus arithmetic operator and the not equal to relational operator.
- If the statement condition i % 2 != 0 is true, then the number is odd. In this case, the continue statement is executed, which skips the rest of the loop's code and moves to the next iteration.
- If the statement condition is false, meaning the value of i is even, then the if-block is skipped. And the printf() statement after that is executed, which displays the value of i.
- Here, the %d format specifier indicates an integer value, and the newline escape sequence (\n) shifts the cursor to the next line so that the next output is printed in a new line.
- As shown in the output for the example of the continue statement in C, this loop continues printing even numbers to the console, skipping odd numbers.
- Once the loop completes its iterations, the main() function closes with a return 0 statement, indicating the successful execution of the program.
Working Of Continue Statement In C
The continue statement in C alters the flow of execution within loops. When encountered, it immediately transfers control to the next iteration of the loop, effectively skipping any remaining code within the loop's body for the current iteration. Here's a detailed explanation of how the continue statement works in C:
- Encounter within a Loop: The continue statement is typically used within loops such as for, while, or do-while.
- Evaluation of Loop Condition: When the loop begins its iteration, the loop condition is evaluated. If the condition is true, the loop body is executed.
- Condition Check: Inside the loop body, if a condition is encountered that triggers the need to skip the remaining code for the current iteration, the continue statement is used.
- Execution of Continue: Upon encountering the continue statement, the program immediately jumps to the next iteration of the loop, skipping any code that follows the continue statement within the loop body.
- Increment/Decrement (for loops): If the loop is a for loop, the increment or decrement part of the loop header is executed after the continue statement, preparing the loop for the next iteration.
- Loop Condition Check: After the increment or decrement operation (if applicable), the loop condition is evaluated again to determine whether to continue or exit the loop.
- Loop Continues: If the loop condition evaluates to true after the continue statement, the loop continues with the next iteration. If the condition is false, the loop terminates.
When To Use Continue Statement In C?
The purpose of the continue statement in C programming is to modify the control flow inside loops, namely in the for, while, and do-while loops. Its main purpose is to skip the execution of the statements following it for that iteration and move to the next iteration when a certain condition is satisfied.
The main purposes of the continue statement in C are:
- Skipping Certain Iterations: It allows skipping iteration of a loop, completely or partially (depending on where it is placed), based on a specific condition without terminating the loop altogether. This is useful when we want to skip the execution of certain statements inside a loop for specific cases/ iterations.
- Conditional Execution: It facilitates the execution of only specific statements of the loop body and skips others when the condition calls for it.
- Code Optimization: It helps in improving code readability and efficiency by avoiding unnecessary code execution. In other words, loop efficiency can be maximized by using the continue statement in C to skip iterations that aren't essential, especially when working with big datasets.
- Streamlining Logic: The use of the continue statement in C programs helps streamline the logic within loops by avoiding unnecessary computations or operations for certain iterations.
- Error Handling: When running a C program, the continue statement can also be used for error handling within loops. In such cases, you can set conditions to indicate that further processing for the current iteration is unnecessary or invalid.
Overall, the continue statement in C is used to fine-tune the behavior of loops and improve code clarity, efficiency, and maintainability in various programming scenarios. However, it should be used judiciously to ensure that the code remains understandable and maintainable.
If-Statements & Continue Statement In C
If-statements are conditional statements that allow you to execute a block of code based on whether a condition is true or false. The continue statement is used within if-statements, where when the condition is met, the program encounters the continue statement. It ultimately causes the program to skip the remaining code inside the loop for the current iteration if a certain condition is met.
Here's how the continue statement in C works inside if-statements:
- The program evaluates the condition specified in the if-statement.
- If the condition is false, the program continues to the next statement after the if statement.
- If the condition is true, the program executes the code block inside the if-statement.
- The if-block must contain a continue statement in this case. As a result, the program skips the remaining statements (after the if-statement) within the current loop and directly jumps to the next iteration.
Syntax:
if (condition) {
continue;
}
// Additional code
}
Here,
- The if keyword marks the beginning of the if-statment and condition refers to the statement condition to determine whether the code body enclosed in the curly braces {} will be executed or not.
- The continue keyword inside the if-block is the continue statement, which will cause the program to skip the lines following it and jump to the beginning of the if statement (or loop in other cases).
Let's look at another C program example to see how this works.
Code Example:
Output:
Executing iteration 1
Skipping iteration 2
Executing iteration 3
Skipping iteration 4
Executing iteration 5
Explanation:
In the C code example-
- We first declare an integer variable, i, inside the main() function, without assigning an initial value.
- As mentioned in the code comment, we then create a for loop to iterate from numbers 1 to 5 (inclusive).
- The loop contains an if-statement which first checks if the current value of loop control variable i is even (i % 2 == 0).
- If i is even, the printf() statement inside the if block prints a message indicating that the iteration is being skipped.
- The continue statement then causes the flow to skip the rest of the loop's code and move to the next iteration.
- If i is not even, the printf() statement outside the if block prints a message indicating that the current iteration is being executed.
- After the loop, we return 0 to indicate the successful execution of the program.
Simple Loops & Continue Statement In C
Loops are control structures that allow you to repeat a block of code multiple times until a certain condition is met or for a specified number of iterations. The three types of loops in C are:
The continue statement in C is usually used inside loops to skip the rest of the code block for the current iteration and move to the next iteration of the loop based on specific conditions.
The working mechanism of the continue statement in C inside loops:
- The loop begins its iteration.
- Upon encountering the continue statement, the program immediately jumps to the next iteration of the loop, skipping any remaining code within the loop's body for the current iteration.
- The loop continues its execution based on the loop condition.
- This process repeats until the loop condition evaluates to false or until the specified number of iterations is reached.
For Loop & Continue Statement In C
A common type of loop in programming where a block of code is repeatedly executed for a specified number of iterations, determined by a control variable or expression.
Syntax:
for (initialization; condition; updation) {
// code block
if (condition_to_continue) {
continue;}
// additional code
}
Here,
- The for and if keywords mark the beginning of the for loop and the if-statement respectively.
- In the loop, initiaization, condition, and updation set the initial value of loop variable, the condition for the loop to run, and the updation expression, respectively.
- The curly braces {} enclose the code body/ statements.
- The continue keyword refers to the continue statement.
The examples given in the sections above illustrate how for loop with continue statements work.
While Loop & Continue Statement In C
Within a while loop, the continue statement skips the remaining code for the current iteration. The program then immediately jumps to the next iteration. This allows for selective execution based on the while loop's condition. Programmers use this to enhance efficiency and logic in their code.
Syntax:
while (condition) {
// code block
if (condition_to_continue) {
continue;}
// additional code
}
Here,
- The while keyword marks the beginning of the while loop and the loop condition enclosed in brackets().
- // code block: This is the block of code that gets executed each time the loop iterates while the condition remains true.
- if (condition_to_continue) { continue; }: This conditional statement checks if a specific condition is met. If it is, the continue statement is executed, causing the loop to skip the remaining code in the current iteration and proceed to the next iteration.
- // additional code: This refers to the additional code within the loop that will only execute if the condition_to_continue evaluated to false, or if there's no such condition present.
Look at the example C program given below, which illustrates the execution of a continue statement inside a while loop.
Code Example:
Output:
1 2 4 5
Explanation:
In the example C code-
- Inside the main() function, we declare an integer data type variable i and assign the value 0 to it.
- Then, we use a while loop that continues as long as i is less than 5.
- As the loop body is executed, the value of the loop variable i is incremented by 1 (i++) in each iteration.
- After that, we have an if-statement that checks if the current value of i is equal to 3.
- If i is equal to 3, the continue statement is executed, which causes the program to skip the rest of the loop's code for this iteration and moves to the next iteration.
- If i is not equal to 3, then the if-block is skipped, and the following printf() statement prints the value of i to the console.
- The loop continues iterations, printing or skipping values as per the continue, until variable i becomes 5. At this point, the loop condition (i < 5) becomes false, and the loop terminates.
- Finally, we return 0 to indicate the successful execution of the program.
- As you can see in the output section, the loop prints numbers 1, 2, 4, and 5, skipping 3 due to the continue statement's existence and if-statement condition.
Do-While Loop & Continue Statement In C
The do-while loop is a unique loop where the code body is implemented first, and then the loop condition is checked. This means that the loop body will be executed at least once. When using the continue statement inside a while loop, it is possible for continue to keep pushing to new iterations before checking the loop condition even once.
Syntax:
do {
// code block
if (condition_to_continue) {
continue;}
// additional code
} while (condition);
Here,
- The do and while keywords mark the two sections of the do-while loop.
- Loop body/ block of code is contained inside the curly braces along with an if statement marked by the if keyword.
- The expression condition_to_continue refers to the if-condition, which, when true, leads to the execution of the if-block.
- The continue keyword refers to the continue statement, and the additional code refers to any other statement that forms a part of the loop body.
- Lastly, the condition following while is the loop condition to determine whether the next iteration will happen or not.
Here is a sample C program that illustrates the use of the continue statement with the do-while loop.
Code Example:
Output:
1 2 4 5
Explanation:
In the sample C code-
- In the main() function, we initialize an integer variable i with the value 0.
- We then have a do-while loop. where the value of loop variable i is incremented by 1, and then we move to an if-statement.
- The if condition checks if the value of i is equal to 3, i==3. If the condition is true, the continue statement is executed, which skips the rest of the loop's code for this iteration and moves to the next iteration.
- If this condition is false, i.e., i is not equal to 3, the flow moves to the next line outside the if-statement. The following printf() statement dislays the value of i to the console.
- After printing or skipping, the loop continues to the next iteration and continues until i is less than 5.
- Once i reaches 5, the condition of the do-while loop (i < 5) becomes false, and the loop terminates.
- Note that in the output of this example, the program did not print 3 as the statement was skipped.
Check out this amazing course to become the best version of the C programmer you can be.
Nested Loops & Continue Statement In C
Nested loops refer to the situation where one loop is nested inside another loop. In these, the program first checks the outer condition, and the flow moves to the inner loop if the outer condition is met.
The inner loop keeps iterating until its condition becomes false. After this, the flow moves back to the outer loop. When placed inside the inner loop (of a nested loop), the continue statement causes the program to skip the rest of the current iteration of the innermost loop and jump to the next iteration.
Working Mechanism of Continue in Nested Loops:
- When encountered within a nested loop, the continue statement skips the remaining code inside the innermost loop for the current iteration and proceeds to the next iteration of that loop.
- The outer loop(s) continue to iterate normally, unaffected by the continue statement in the inner loop.
- This behavior allows skipping specific iterations within the inner loop while continuing the overall iteration process of the outer loop(s).
Syntax:
Outer loop (condition) {
Inner loop (condition) {
// Code statements
if (condition) {
continue;
}
}
// Code block for outer loop
}
Here,
- Outer loop: Controls the repetition of the inner loop and executes its code block based on a condition.
- Inner loop: Executes its code block repeatedly within the outer loop based on its own condition.
- Code statements: Instructions executed within the inner loop's code block.
- Condition check: Evaluates a condition within the inner loop, allowing for conditional execution.
- Continue statement: Skips the rest of the current iteration of the inner loop and proceeds to the next iteration.
The C program sample given below illustrates the function of the continue statement inside a nested for loop.
Code Example:
Output:
1 3 4
1 3 4
1 3 4
Explanation:
In the C code sample-
- Inside the main() function, we create a nested for loop where the control variable for the outer loop is integer variable i, and for the inner loop is integer variable j.
- The outer for loop iterates from 1 to 3, i.e., the initial value of i is 1, the loop continues till i<=3, and the value is incremented by 1 after every iteration.
- For every iteration of the outer loop, the inner loop iterates from 1 to 4. In the inner loop-
- We have an if-statement that checks if the value of j is equal to 2.
- If the condition is false, the if-block is skipped, and the printf() statement following it displays the values of variables i and j.
- If the condition is true, the continue statement is executed, and the following printf() statement is skipped.
- After the inner loop completes a set of iterations, the printf() statement with a newline escape sequence shifts the cursor to the next line.
- Then, the flow moves back to the outer loop and the process begins all over again.
- As seen in the output window, for every iteration of the outer loop, the inner loop prints only numbers 1, 3, and 4. It skips the number 2 due to the execution of the continue statement.
Looking for mentors? Find the perfect mentor for select experienced coding & software experts here.
Example Of Difference Between Break & Continue Statements In C
The break and continue statements in C are both control flow statements used within loops. They are also referred to as jump statements, but they serve different purposes. The break statement is used to exit the current loop prematurely. On the other hand, the continue statement in C is used to skip the current iteration of a loop and proceed to the next iteration.
Let's illustrate the difference between the break and continue statements in C with an example:
Code Example:
Output:
For loop with break:
Iteration 1
Iteration 2
For loop with continue:
Iteration 1
Iteration 2
Iteration 4
Explanation:
In the basic C code example-
- Inside the main() function, we first use a printf() statement to display a string message- For loop with break.
- Then, as indicated, we create a for loop that iterates from 1 to 5. Within this loop, we have an if-statement whose condition checks if the current value of i is equal to 3.
- If i is equal to 3, the break statement is executed, which exits the loop immediately.
- If i is not equal to 3, we print a message indicating the current iteration number.
- After the first loop, we print another message- For loop with continue. Then, we create a second for loop that iterates from 1 to 4.
- Within this loop, we have an if-statement that checks if the current value of j is equal to 3.
- If j is equal to 3, the continue statement is executed, which skips the rest of the loop's code and moves to the next iteration.
- If j is not equal to 3, we print a message indicating the current iteration number.
- This example shows, how the continue statement in C makes the program jump out of one iteration of the loop and begin the next one. While the break statement terminates the loop altogether.
For more detailed differences, read: Difference Between Break And Continue Statements In C (+Explanation)
Conclusion
In conclusion, the continue statement in C programming serves as a valuable tool for controlling the flow of execution within loops. Its ability to selectively skip iterations or jump out of an iteration based on specific conditions provides developers with flexibility and control over loop execution.
By strategically using the continue statement in C, programmers can optimize code efficiency, streamline processing, and enhance program readability. However, it is essential to use the continue statement judiciously in your C programs, as excessive use or misuse can lead to code complexity and decreased readability.
Also read- 100+ Top C Interview Questions With Answers (2024)
Frequently Asked Questions
Q. What is the purpose of the continue statement in C?
The purpose of the continue statement in C is to selectively skip the remaining code within the body of a loop for the current iteration and directly jump to the next iteration. This allows programmers to control the normal flow of execution within loops based on specific conditions, optimizing loop performance and efficiency. In other words, by using the continue statement in C, developers can exclude certain iterations from executing particular code blocks within loops, streamlining processing and enhancing code readability.
Q. What is the break statement with an example?
The break statement in C is a control statement used within loops to immediately terminate their execution, allowing the program to exit the loop prematurely. When encountered, it causes the control flow to exit the innermost loop it is contained in.
Code Example:
Output:
0 1 2 3 4
Q. Can the continue statement in C be used in switch-case statements?
No, the continue statement cannot be used directly within a switch-case construct in languages like C, C++, Java, and similar languages.
- The continue statement is typically used within loops like for or while to skip the rest of the current iteration and move on to the next iteration.
- It is not applicable within a switch-case construct because switch-case is used to select one of many code blocks to execute based on the value of an expression.
If you want to skip the execution of certain case blocks in a switch-case construct, you can simply omit the break statement at the end of those case blocks. This will cause the execution to fall through to the next case or the end of the switch block, effectively skipping the code in the omitted case block(s). However, this technique should be used judiciously and documented well, as it might make the code less readable and more error-prone.
Q. What is a real-life example of a continue statement?
A real-life example of a situation where you might use the continue statement can be found in a task list or project management scenario.
Let's say you're managing a team, and you have a list of tasks to be completed. Each task has its own set of requirements and dependencies. You're iterating over this list to check the status of each task and perform some actions based on their status.
Here's how you might use continue in this scenario:
- Checking Task Status: You're iterating over the list of tasks. For each task, you check its status:
- If the task is completed, you log it and move to the next task.
- If the task is not completed yet, you perform some actions related to ongoing tasks.
- Skipping Certain Tasks: Some tasks might not require any action at the moment. For example, tasks that are on hold or waiting for external dependencies. Instead of executing actions for these tasks, you want to skip them and move to the next task. In this scenario, you would use the continue statement:
- When encountering a task that doesn't require any immediate action, you can use continue to skip the remaining actions related to that task and move to the next task in the list.
- This allows you to efficiently manage your workflow by focusing only on the tasks that require attention at the current time without getting bogged down by tasks that can be deferred or don't need immediate action.
Q. Are there any potential pitfalls or drawbacks to using the continue statement?
Yes, there are potential pitfalls or drawbacks to using the continue statement in C programming:
- Complexity and Readability: Excessive use of the continue statement in C within loops can make code harder to understand and maintain. It may obscure the logic of the loop and make it challenging for other developers to follow.
- Potential Bugs: Incorrect placement or usage of the continue statement in C can lead to unintended behavior or bugs in the program. Misinterpreting loop conditions or overlooking edge cases may result in logic errors.
- Performance Impact: While the continue statement in C can optimize loop execution by skipping unnecessary iterations, it may also introduce overhead, especially in nested loops or loops with complex conditions. Profiling tools can help identify performance bottlenecks caused by excessive use of continue.
- Code Smells: In some cases, frequent use of continue may indicate that the loop structure or conditionals could be simplified or refactored for improved clarity and efficiency. It's essential to review the use of continue and assess whether it aligns with best practices and code quality standards.
- Potential for Infinite Loops: Incorrect placement of the continue statement may result in infinite loops, further causing program crashes or freezes. Hence, you must be careful when using the continue statement in C while ensuring the validation of loop logic.
Suggested reads:
- Comma Operator In C | Code Examples For Both Separator & Operator
- Identifiers In C | Types, Rules For Definition, & More (+Examples)
- Pointers In C | Ultimate Guide With Easy Explanations (+Examples)
- Union In C | Declare, Initialize, Access Member & More (Examples)
- Difference Between Call By Value And Call By Reference (+Examples)
- Functions In C | Uses, Types, Components & More (+Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment