Table of content:
- Explanation Of Call By Value And Call By Reference
- Differences Between Call By Value And Call By Reference
- What Is Call By Value?
- What Is Call By Reference?
- When To Use Call By Value And Call By Reference In C++/ C/ Other Languages?
- Conclusion
- Frequently Asked Questions
Difference Between Call By Value And Call By Reference (+Examples)
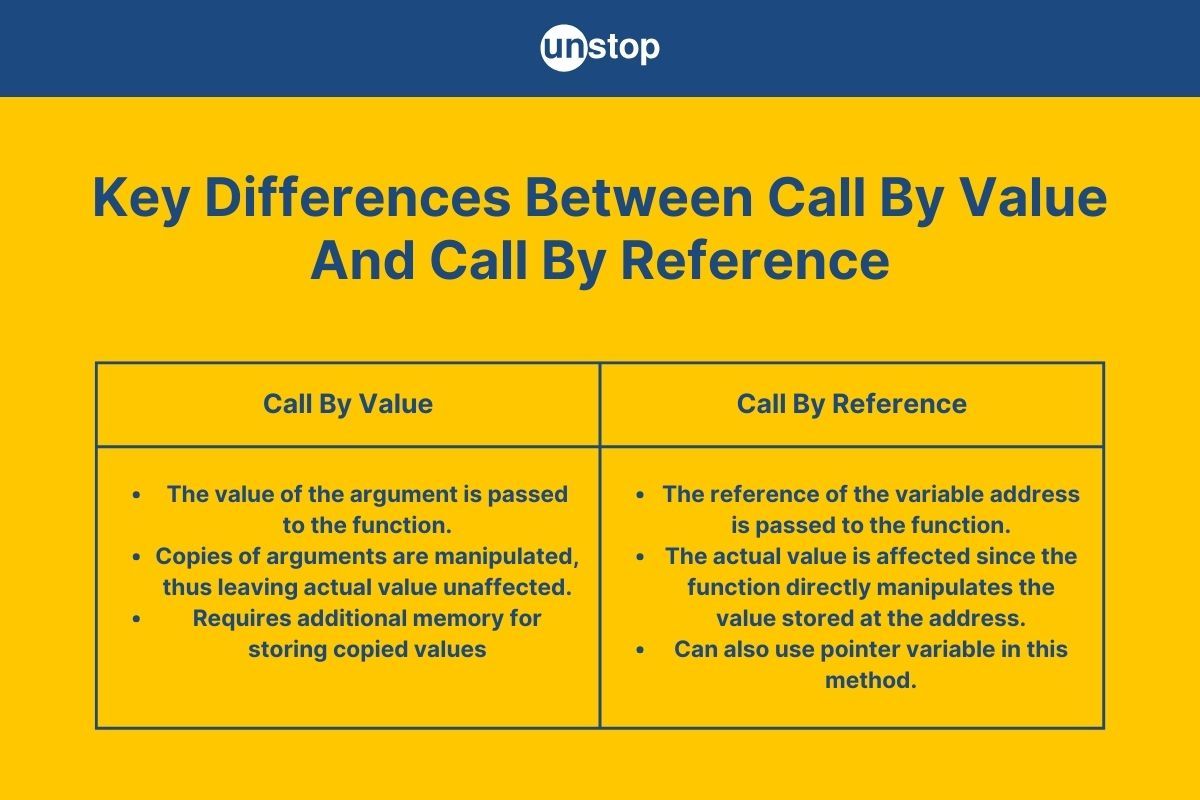
In programming, functions are blocks of code that enclose statements to perform specific tasks. We call these functions to repetitively perform this task without having to write the statements again and again. There are two primary ways of calling functions in most programming languages, i.e., one by value and one by reference. While both of these fulfil the same purpose, they are not the same in every sense. In this article, we will explore the difference between call by value and call by reference.
Explanation Of Call By Value And Call By Reference
To begin with, let us understand what defining and calling a function means.
- When you define a function, you enclose the code statements that stipulate its behaviour on the input values.
- We use function parameters/ dummy variables in the definition and not actual values.
- Then, when you call a function, you provide it with data to work with. This data/ actual value is referred to as actual arguments
- In other words, we provide the actual variable values. The function takes these values, does its job and returns a result (when a return statement is defined).
In short, when we define a function (function definition), we use formal parameters. When we call it, we use formal arguments/ actual variable values, which the function works with. The methods mentioned above are how we provide the function with input or data (values of variables) to work on.
Call by Value- This is a straightforward method in which we pass the value of the parameters by mentioning argument names. In effect, a copy of the actual parameter value (i.e., formal argument) is passed to the function.
Call by Reference- In the reference method, instead of passing the value directly, we pass a reference to the argument variables. That is, we pass the address of variables/ arguments to functions when making function calls.
Differences Between Call By Value And Call By Reference
Understanding the differences between Call-by-value and Call-by-Reference is crucial for designing efficient and reliable software systems. The table below highlights the key difference between call-by-value and call-by-reference methods of calling a function.
Aspect | Call by Value | Call by Reference |
---|---|---|
Parameter passing | Copies the actual value of an argument into the formal parameter | Passes the address of the actual parameter into the formal parameter |
Effect on actual parameters | Actual parameters remain unchanged | Actual parameters can be changed within the function |
Convention of Naming | Typically uses descriptive names for formal parameters | May use prefixes like "ptr_" or "&" to denote references |
Manipulation of Variables | Cannot directly modify variables outside the function | Allows direct modification of variables outside the function |
Memory allocation | Requires additional memory for storing copied values | Requires minimal memory for storing addresses |
Performance | Generally faster due to direct value access | Slightly slower due to indirection when accessing values |
Access to original data | Cannot directly modify the original data | Can directly modify the original data through references |
Scope of changes | Changes made to formal parameters do not affect actual parameters outside the function | Changes made to formal parameters affect actual parameters outside the function |
Example | int x = 10; foo(x); // x remains 10 | int x = 10; foo(&x); // x can be modified within foo |
What Is Call By Value?
As mentioned before, in this simple technique, we pass the values of variables to the function. This is the default method of calling functions in most programming languages. When we pass the value of variables through function calls, we are essentially creating a copy of the value/ function argument value. This means that any changes made to the variable value inside the function do not affect the actual values of variables. Let's discuss the working mechanism to understand this concept better.
How Does The Call By Value Method Work?
As the name suggests, pass the actual value to the function by using the variable name directly. The process for this is as follows-
- When we call a function, we add the actual parameter name to the function call syntax.
- As a result, a copy of the value of the actual argument is created in the function's memory space.
- The function uses this copy to perform argument operations, and any changes made to the value hereon out are limited to this local copy.
- Once the function finishes execution, the local copies of function arguments are destroyed. The original/ actual argument value remains unchanged in the memory space of the calling function.
- This is why any modification made to the actual argument value within the function does not affect the actual parameter value.
Advantages & Disadvantages Of The Call By Value Method
Advantages:
- Predictability: Call by Value ensures that the original data remains unchanged since only a copy of the value is passed to the function. This makes the behavior of the program more predictable, as functions cannot inadvertently modify data outside their scope.
- Simplicity: Call by Value is straightforward to understand and implement. There's no need to manage memory addresses or worry about unintended side effects caused by modifying the original data.
- Safety: Since Call by Value doesn't allow functions to modify the original data, it reduces the risk of accidental data corruption or unintended consequences in the program. This is particularly beneficial in large codebases where tracking data mutations can be challenging.
- Parallel Execution: Call by Value facilitates parallel execution since each function operates on its own copy of the data. This can potentially improve performance in concurrent programming environments by reducing the need for synchronization mechanisms.
- Efficiency for Primitive Types: For primitive data types like integers or floating-point numbers, Call by Value is efficient as it involves simple copying operations, which are typically fast.
Disadvantages:
- Overhead for Large Objects: When dealing with large data structures or objects, Call by Value can incur performance overhead due to the need to copy the entire data. This overhead can become significant, especially in cases where the data size is substantial.
- Memory Consumption: Since Call by Value involves copying data, it can lead to higher memory consumption, especially in scenarios where functions are called frequently with large parameters.
- Limited Mutability: Call by Value restricts the ability to modify data within functions. While this is advantageous for safety and predictability, it can be a limitation in situations where in-place modifications are necessary for performance optimization or algorithm implementation.
- Inefficient for Complex Objects: For complex objects with nested structures or dynamic memory allocation, Call by Value can be inefficient as it recursively copies all nested data. This can result in excessive memory usage and slower execution times.
- Copying Overhead: The process of copying data in Call by Value can introduce overhead, especially for custom data types or objects with complex constructors and destructors. This can impact performance, particularly in time-sensitive applications.
Examples Of Call By Value
In this section, we will look at examples of the call-by-value method in various languages, i.e., C/C++, Java and Python.
Code Example (C/C++):
#include <stdio.h>
void modifyValue(int x) {
x = x + 10;
printf("Value inside function: %d\n", x);
}
int main() {
int a = 5;
modifyValue(a);
printf("Value outside function: %d\n", a);
return 0;
}
Output:
Value inside function: 15
Value outside function: 5
Explanation:
We begin the simple C program example by including the essential header file <stdio.h> for input-output operations.
- Then, we define a function called modifyValue, which takes an integer variable/ parameter called x. We use the variable name in the function definition and not the reference, which you will see in the call-by-reference case.
- Inside the function, we add a value 10 to x and reassign the modified value back to the variable x.
- We then use a printf() statement to display the variable's value after the arithmetic operation is performed. The %d format specifier indicates an integer value, and the newline escape sequence (\n) shifts the cursor to the next line.
- In the main() function, we declare and initialize variable a of an integer data type with the value 5.
- We then call the modifyValue function by passing with value. This essentially passes a copy of the value of a (the argument) to x (the parameter).
- After that, we use another printf() statement to display the value of the variable a outside of the function. As seen in the output, the function operation does not affect the initial value of the variable/ argument.
- Finally, the main() function terminates with a return 0 statement indicating successful execution without any errors.
Also read: Functions In C | Uses, Types, Components & More (+Code Examples)
Code Example (Java):
public class Main {
public static void modifyValue(int x) {
x = x + 10;
System.out.println("Value inside function: " + x);
}
public static void main(String[] args) {
int a = 5;
modifyValue(a);
System.out.println("Value outside function: " + a);
}
}
Output:
Value inside function: 15
Value outside function: 5
Explanation:
In the above code,
- We define a static method called modifyValue in the main class, which takes an integer x as a parameter.
- Inside the modifyValue method, we increment the value of x by 10.
- We then print the modified value of x inside the modifyValue method.
- In the main method, we declare and initialize an integer a with the value 5.
- We call the modifyValue method with the value of a as an argument.
- We print the value of a outside the modifyValue method.
Code Example (Python):
def modify_value(x):
x = x + 10
print("Value inside function:", x)
a = 5
modify_value(a)
print("Value outside function:", a)
Output:
Value inside function: 15
Value outside function: 5
Explanation:
In the simple Python program-
- We have a function named modify_value, which takes a parameter x.
- Inside modify_value, we increment the value of x by 10.
- We then print the modified value of x inside the modify_value function.
- Next, we declare variable a and assign it the value 5.
- We call the modify_value function with the value of a as an argument.
- We print the value of a outside the modify_value function.
What Is Call By Reference?
Call by reference is a method of passing arguments to a function where a reference to the actual parameter is passed, allowing the function to modify the original value.
Call by reference provides the function with a more direct path to the original argument. Instead of copying the value, it passes the memory address of the original variable. This memory address location acts like a reference, allowing the function to access and modify the value stored at that location. We can also use pointer variables to pass the reference memory address to the function call.
How Does The Call By Reference Method Work?
Here is how the call-by-reference method works-
- You must first create a pointer variable, which stores the memory address of the arguments.
- Then, you pass this reference address to the function. In effect, the function receives a pointer variable containing the memory address of the original argument. (Note: This point marks a major difference between call by value and call by reference methods of calling a function.)
- With the help of the dereference operator (* in most programming languages), the function can then access the actual content/ value stored at that memory location.
- Any modifications made using the pointer variable directly affect the original argument's value in the calling program's memory.
Advantages & Disadvantages Of The Call By Reference Method
Advantages:
- Efficiency: Call by Reference avoids the overhead of copying large data structures or objects since only the memory address (reference) of the original data is passed to the function. This can lead to significant performance improvements, especially when dealing with large or complex data.
- Memory Conservation: Since Call by Reference passes only the address of the original data, it conserves memory compared to Call by Value, which requires storing copies of data. This can be particularly beneficial in memory-constrained environments or when dealing with large datasets.
- Mutable Function Parameters: Call by Reference allows functions to modify the original data directly. This is useful when implementing algorithms that require in-place modifications or when the goal is to update variables outside the function's scope.
- Ability to Return Multiple Values: Call by Reference enables functions to return multiple values by modifying the values of reference parameters. This can simplify code and improve readability by eliminating the need for complex return structures or the out parameters.
- Flexibility: Call by Reference offers flexibility in handling data, as functions can access and modify variables defined outside their scope. This allows for more dynamic and interactive programming patterns, especially in scenarios where shared state or global variables are used.
Disadvantages:
- Unintended Side Effects: Call by Reference can lead to unintended side effects if functions modify the original data unexpectedly. This can make code harder to understand, debug, and maintain, especially in large codebases or collaborative projects.
- Mutability Concerns: The direct modification of data within functions can make it challenging to track changes and reason about program behavior. This can introduce complexity and increase the risk of bugs, particularly in multi-threaded or concurrent environments.
- Security Risks: Call by Reference can pose security risks, such as potential data corruption or unauthorized access, if not used carefully. Modifying data directly via references can open up vulnerabilities to malicious attacks, such as pointer manipulation or buffer overflows.
- Dependency on External State: Functions using Call by Reference rely on external variables, which can lead to dependencies and coupling between different parts of the codebase. This can hinder code modularity and make it harder to isolate and test individual components.
- Potential for Dangling References: If the referenced data is deallocated or goes out of scope before the reference is used, it can result in dangling references or memory access errors. Proper memory management and lifetime management are essential to mitigate this risk.
Examples Of Call By Reference
In this section, we will look at examples of the call-by-reference method in various languages, i.e., C/C++, Java and Python.
Code Example (C/C++):
#include <iostream>
using namespace std;
void modifyValue(int &x) {
x = x + 10;
cout << "Value inside function: " << x << endl;
}
int main() {
int a = 5;
modifyValue(a);
cout << "Value outside function: " << a << endl;
return 0;
}
Output:
Value inside function: 15
Value outside function: 15
Explanation:
In this C++ program, we include the standard header file for input/ output operations <iostream>.
- We define a function called modifyValue, which takes the reference to variable x (integer data type) as a parameter.
- Inside the function, we add 10 to the value of x and reassign that back to it. Then, we use cout commands to print this value to the output console.
- In the main() function, we declare an integer variable a and assign value 5 to it.
- Then, we call the modifyValue function, passing variable a as an argument. This means a is passed by reference to modifyValue since, by definition, the function takes reference to the input variable. This allows the function to directly manipulate its value.
-
After the modifyValue function call, we use cout to print the current value of a to the console. Since variable a was passed by reference to modifyValue, any changes made to it within the function persist outside of it as well.
-
Finally, the program returns 0, indicating successful execution.
Also read: C++ Function | A Comprehensive Guide (With Code Examples)
Code Example (Java):
class Main {
public static void modifyValue(int[] arr) {
arr[0] = arr[0] + 10;
System.out.println("Value inside function: " + arr[0]);
}
public static void main(String[] args) {
int[] a = {5};
modifyValue(a);
System.out.println("Value outside function: " + a[0]);
}
}
Output:
Value inside function: 15
Value outside function: 15
Explanation:
In the above code,
- We define a Java class named Main.
- We have a static method named modifyValue, taking an integer array arr.
- Inside modifyValue, we increment the first element of arr by 10. We print the modified value of the first element of arr.
- In the main method, we declare and initialize an array a with a single element, 5. We call modifyValue with the array a as an argument.
- We print the value of the first element of array a.
Code Example (Python):
def modify_value(lst):
lst[0] = lst[0] + 10
print("Value inside function:", lst[0])
a = [5]
modify_value(a)
print("Value outside function:", a[0])
Output:
Value inside function: 15
Value outside function: 15
Explanation:
In the Python code example-
- We have a function named modify_value, which takes a list lst as a parameter.
- Inside modify_value, we increment the first element of lst by 10. We print the modified value of the first element of lst.
- Then, we declare a list a with a single element, 5.
- After that, we call the modify_value function with list a as an argument.
- Finally, we print the value of the first element of list a.
When To Use Call By Value And Call By Reference In C++/ C/ Other Languages?
In programming languages like C++, C, and many others, choosing between Call by Value and Call by Reference is a crucial decision that impacts the behavior, performance, and maintainability of your code. Each method has its own strengths and weaknesses, making it important to understand when to use each one.
Call by Value is often preferred when you need to ensure that the original data remains unchanged.
- This method is suitable for passing immutable data types or when you want to avoid unintended side effects caused by function modifications.
- It provides predictability and safety by guaranteeing that functions cannot inadvertently alter external data.
- Call by Value is particularly useful for passing primitive data types like integers or floating-point numbers, where copying overhead is minimal, and for scenarios where data integrity and security are paramount.
Call by Reference, on the other hand, shines when dealing with large or complex data structures.
- By passing the memory address of the original data, Call by Reference eliminates the need to copy large data, thus improving performance and conserving memory.
- This method is ideal for mutable function parameters, enabling functions to directly modify the original data.
- It's commonly used for passing arrays, vectors, or custom objects, where copying overhead can be significant and in-place modifications are necessary.
Ultimately, the decision between Call by Value and Call by Reference should be based on careful consideration of factors such as data mutability, performance requirements, code clarity, and design goals.
Conclusion
Understanding the difference between call by value and call by reference is crucial for effective programming. These are both methods to call a function in various programming languages.
- In the call-by-value method, we pass the actual argument value to the function, which creates a local copy. Any changes made to the value inside the function do not affect the actual value. The call-by-value method ensures safety and simplicity, making it ideal when you do not want to alter the original data.
- Conversely, in the call-by-reference method, we pass a reference to the variable, i.e., its address to the function. It can then access and modify this value, meaning any changes to the value inside the function affect the initial value. The call-by-reference method offers efficiency and functionality, allowing functions to modify the original values.
It is important to know when to use each method if you want to write more efficient and safer code tailored to your specific needs.
Frequently Asked Questions
Q. What is Call By Value and Call By Reference in C?
Call by Value involves passing a copy of the data to a function, where modifications made within the function do not affect the original data. In contrast, Call by Reference passes the memory address (reference) of the original data, allowing functions to directly modify the original data.
Q. When should I use Call By Value in C?
Call by Value is preferred when you want to ensure that the original data remains unchanged. It's suitable for passing immutable data types or when you need predictable behavior without unintended side effects caused by function modifications.
Q. When should I use Call By Reference in C?
Call by Reference is ideal for passing large or complex data structures where copying overhead is significant. It's used when functions need to modify the original data directly or when in-place modifications are necessary. Call by Reference is commonly employed for passing arrays, vectors, or custom objects.
Q. What are the performance considerations for Call By Value and Call By Reference in C?
Call by Value incurs copying overhead, which can be negligible for primitive data types but significant for large data structures. Call by Reference avoids copying overhead by passing memory addresses, making it more efficient, especially for large data. However, Call by Reference may have slight indirection costs compared to Call by Value.
Q. How do I ensure data safety and security when using Call By Reference in C?
While Call by Reference offers efficiency and flexibility, it's essential to ensure data integrity and prevent unintended side effects. Proper documentation and adherence to coding conventions can help clarify which functions modify data via references. Additionally, implementing safeguards such as const qualifiers for non-mutable references can enhance code safety and readability.
By now, you must be clear about the difference between call by value and call by reference methods. Here are a few other interesting topics you'd like:
- Pointers in C++ | A Roadmap To All Pointer Types (With Examples)
- Difference Between Pointer And Reference In C++ (With Examples)
- Difference Between Java And JavaScript Explained In Detail!
- Top 15+ Difference Between C++ And Java Explained! (+Similarities)
- Comma Operator In C | Code Examples For Both Separator & Operator
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment