Table of content:
- What Is A Structure And How To Define It?
- What Is Structure Pointer In C?
- Declaration Of Structure Pointer In C
- Initialization Of Structure Pointer In C
- Accessing Structure Member Using Structure Pointer In C
- Dynamic Memory Allocation Using Structure Pointer In C
- Uses Of Structure Pointer In C
- Structures Containing Pointer Members In C
- Conclusion
- Frequently Asked Questions
Structure Pointer In C | Declare, Initialize & Uses (+Examples)
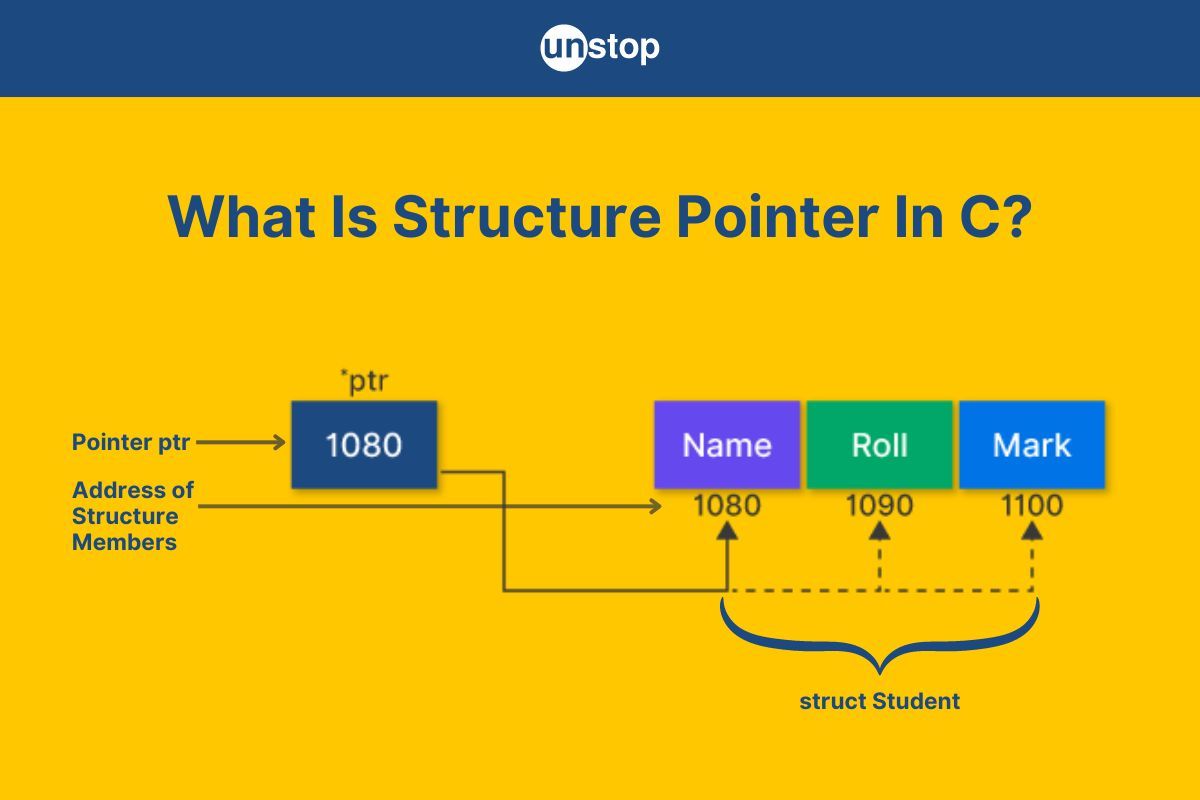
A structure pointer in C programming language is a pointer that points to a structure variable. It allows us to access and manipulate the data within the structure indirectly using the pointer. Structure pointers are particularly useful in dynamic memory management and when passing structures to functions. In this article, we will explore the concept of structure pointers in C, including their syntax, practical applications, and the benefits they provide in programming tasks.
What Is A Structure And How To Define It?
In C programming, a structure is a user-defined data type that groups variables of different types under a single name. It allows you to represent a collection of related data items as a single unit, making your development programs more organized and manageable. Each element of a structure is called a member, and each member can be of a different data type.
Syntax:
struct StructureName {
dataType member1;
dataType member2;
// Add more members as needed
};
To define a structure in C, we use the keyword struct followed by the structure name and a block of member definitions.
You can read more about structures here: Structure In C | Create, Access, Modify & More (+Code Examples)
What Is Structure Pointer In C?
In simple terms, a structure pointer in C is a pointer that specifically points to a structure. Just like you use pointers to manage variables and arrays, structure pointers allow you to handle the addresses of structure variables. It allows you to access and modify the structure's members indirectly, using the arrow operator (->) or by dereferencing the pointer.
For example, if you have a Person structure with members like name and age, a structure pointer can be used to point to an instance of Person and access or modify its members.
Declaration Of Structure Pointer In C
In C programming, declaring a structure pointer involves creating a variable that can hold the memory address of a structure. The syntax declaration for the structure pointer in C is given below.
Syntax:
struct StructureName *pointerName;
Here,
- The struct keyword is used to indicate that the type of the variable is a structure.
- StructureName: It refers to the name/ identifier of the structure type you are pointing to.
- pointerName: It represents the name of the pointer variable. The asterisk symbol(*) indicates that pointerName is a pointer variable.
Example:
Suppose we have a structure definition for a Book:
struct Book {
char title[50];
char author[50];
int year;
};
To declare a pointer to this Book structure, we can write as follows:
struct Book *bookPtr;
In this declaration, bookPtr can now hold the address of a Book structure variable. We can use bookPtr to access and modify the Book structure’s members through the pointer.
Initialization Of Structure Pointer In C
The process of initialization of a structure pointer in C involves assigning a memory address to a pointer variable so that it points to a structure instance.
Syntax:
struct StructureName *pointerName = &structureVariable;
Here,
- struct StructureName specifies the type of the structure.
- *pointerName is the pointer variable that holds the address of a structure of type struct StructureName. The asterisk symbol (*) indicates that it is a pointer.
- The assignment operator (=) is used to set pointerName to the address of the existing structure variable.
- The address-of operator (&) gets the memory address of the structureVariable, which is an instance of struct StructureName. This address is assigned to pointerName, so pointerName now points to structureVariable.
Let's look at a simple C program example to understand the initialization of structure pointers in C.
Code:
#include <stdio.h>
#include <string.h>
// Define the structure
struct Person {
char name[50];
int age;
};
int main() {
// Declare and initialize a structure variable
struct Person person1;
// Declare a structure pointer and initialize it to point to person1
struct Person *personPtr = &person1;
// Assign values to the structure members using the pointer
strcpy(personPtr->name, "Atisha");
personPtr->age = 30;
// Print the structure members using the pointer
printf("Name: %s\n", personPtr->name);
printf("Age: %d\n", personPtr->age);
return 0;
}
Output:
Name: Atisha
Age: 30
Explanation:
In the above code example-
- We start by including the necessary header files, stdio.h for standard input and output functions, and string.h for string manipulation functions.
- Next, we define a structure named Person. This structure contains two members: name, an array of size 50, and age, an integer variable. The name member is used to store the person's name, and age stores the person's age.
- In the main() function, we first declare a structure variable named person1 of type struct Person to hold the information about a person.
- Next, we declare a pointer named personPtr that points to a struct Person. We initialize this pointer to point to the address of person1 using the address-of operator (&).
- This pointer will allow us to access and modify the members of person1 indirectly.
- We then initialize the members of structure person 1, as follows:
- We use the strcpy() function (from string.h) to copy the string value "Atisha" into the name member. This sets the name of person1 to "Atisha".
- Note that we use the structure pointer personPtr with arrow operator to access the mmeber. We’ll elaborate on this in the next section.
- Similarly, we assign the value 30 to the age member of the structure pointed to by personPtr, which sets the age of person1 to 30.
- Next, we display the information of person1 to the console using the printf() function, once again accessing members using structure pointer perstonPtr.
- Here, the %s and %d format specifiers inside the formatted string are the placeholders for the string and integer values, respectively.
- Finally, the main() function returns 0 to indicate that the program has been executed successfully.
Accessing Structure Member Using Structure Pointer In C
In this section, we will discuss how to access the members of a structure using the structure pointers. These pointers allow us to indirectly access and modify the members of a structure by pointing to the structure's memory address.
There are two ways to access structure members using structure pointers in C:
- Using the indirection operator(*) and dot operator(.)
- Using the arrow ( -> ) operator, also known as the membership operator
We will discuss both of these methods in detail in the sections below.
Access Structure Member Using Indirection Operator(*),Dot Operator(.) & Structure Pointer In C
This method entails dereferencing the structure pointer in C using the asterisk (*) operator and then accessing the structure members using the dot (.) operator.
- In this method, we reference the structure whose members we want to access, using the indirection/ dereference operator (*) and structure pointer.
- We then combine it with the dot operator (.) to specify the individual member of the structure.
This way we use the structure pointer in C to get indirect access to the members of the structure it is pointing to. The exact syntax used in this method is given below, followed by an example.
Syntax:
(*pointerName).memberName;
Here,
- In the expression *pointerName, the asterisk symbol (*) is the dereference/ indirection operator used to access the value stored at the address pointed to by the pointer variable identified as pointerName.
- The dot operator (.) is used to access a specific member identified as memberName.
Code:
#include <stdio.h>
#include <string.h>
// Define the structure
struct Person {
char name[50];
int age;
};
int main() {
// Declare and initialize a structure variable
struct Person person1;
// Declare a structure pointer and initialize it to point to person1
struct Person *personPtr = &person1;
// Assign values to the structure members using the pointer and dereference operator
strcpy((*personPtr).name, "Bhaskar");
(*personPtr).age = 45;
// Print the structure members
printf("Name: %s\n", (*personPtr).name);
printf("Age: %d\n", (*personPtr).age);
return 0;
}
Output:
Name: Bhaskar
Age: 45
Explanation:
In the above code implementation-
- We start by defining a structure named Person that contains two members: a character array name with a size of 50 and an integer age.
- Inside the main() function, we declare and initialize a variable person1 of type struct Person.
- Next, we declare a structure pointer named personPtr of type struct Person. We initialize this pointer to point to the address of person1 using the address-of operator (&). This allows us to access and modify the members of person1 through the pointer.
- We then use the strcpy() function from string.h to copy the string "Bhaskar" into the name member of the structure pointed to by personPtr.
- Here, we use the dereference operator (*) to access the structure and the dot operator to specify the name member.
- Similarly, we assign the value 45 to the age member of the structure pointed to by personPtr.
- After that, we use the printf() function with formatted strings, to display the values stored in the structure, i.e., name and age members of the person1 structure using the dereference (*) and dot operators (.).
Accessing Structure Member Using The Arrow Operator(->) & Structure Pointer In C
The arrow operator (->) is also known as the ‘this’ operator/ class member access operator in C++. It allows us to access structure members using the structure pointer in C, without the need for the dot operator (.).
- This means that the pointer name references the respective structure (it is pointing to) and the arrow operator points to the individual member whose name is specified.
- The use of an arrow operator with a structure pointer is generally preferred for its simplicity and readability.
Syntax:
pointerName->memberName;
Here,
- pointerName: It is the structure pointer variable.
- The arrow operator(->): It is used to access the structure member using the structure pointer.
- memberName: It is the name of the structure member we want to access.
Code:
#include <stdio.h>
#include <string.h>
// Define the structure
struct Person {
char name[50];
int age;
};
int main() {
// Declare and initialize a struct variable
struct Person person1;
// Declare a structure pointer ptr and initialize it to point to person1
struct Person *personPtr = &person1;
// Assign values to the structure members using the pointer and arrow operator
strcpy(personPtr->name, "Dhruv");
personPtr->age = 28;
// Print the structure members
printf("Name: %s\n", personPtr->name);
printf("Age: %d\n", personPtr->age);
return 0;
}
Output:
Name: Dhruv
Age: 28
Explanation:
In the above code execution-
- We start by defining a structure named Person that consists of two members: a character array name with a size of 50 and an integer age.
- Inside the main() function, we declare and initialize a structure variable person1 of type struct Person. This variable will hold the data for a single person.
- Next, we declare a pointer named personPtr that points to a struct Person. We initialize this pointer to point to the address of person1 using the address-of operator (&). This allows us to access and modify the members of person1 through the pointer.
- We then use the pointer to assign values to the structure members:
- First, we use the arrow operator(->) to access members of the structure through the pointer.
- Next, we use strcpy() function to copy the string "Dhruv" into the name member of person1. We also set the age member of person1 to 28.
- To display the values stored in the structure, we use the printf() function. We print the name and age members of the structure using the arrow operator(->).
- Finally, the main() function returns 0 to indicate that the program has been executed successfully.
Dynamic Memory Allocation Using Structure Pointer In C
Dynamic memory allocation with structure pointers in C refers to the process of allocating memory for structures during program execution, rather than at compile time. This is achieved using functions like malloc(), which allocates memory based on the structure's size.
The allocated memory is then referenced by a structure pointer, allowing for flexible management of data that may vary in size or number. After using the allocated memory, it should be released using free() function to prevent memory leaks and ensure efficient memory use.
Here is a step-by-step explanation of how to dynamically allocate memory for a structure using its structure pointer in C programs:
- Including Necessary Headers: Start your program by including the <stdlib.h> header file to access functions like malloc() and free() for dynamic memory management.
- Defining the Structure: Next, define the structure that you want to allocate memory for. For example:
struct Person {
char name[50];
int age;
};
- Declaring a Structure Pointer: Now, declare a pointer to your structure type. This pointer will hold the address of the dynamically allocated memory block.
struct Person *personPtr;
- Allocating Memory Using malloc(): Use the malloc() function for allocating memory to your structure. The malloc() allocates a block of memory of a specified size in bytes and returns a pointer to the beginning of the block. We use the sizeof() operator to calculate the size of the structure variable.
personPtr = (struct Person *) malloc(sizeof(struct Person));
- Checking for Allocation Success: It's crucial to check if the memory allocation was successful. It is done by verifying that the returned pointer is not NULL. If malloc() fails to allocate memory or an error in the program is encountered (often due to insufficient memory available), it returns NULL.
if (personPtr == NULL) {
fprintf(stderr, "Memory allocation failed\n");
exit(1); // Exit the program or handle the error accordingly
}
- Accessing and Using the Dynamically Allocated Structure: Now that memory is allocated, you can use the structure pointer (personPtr) to access and modify the members of the structure.
strcpy(personPtr->name, "Atisha");
personPtr->age = 30;
- Freeing Dynamically Allocated Memory: When you no longer need the dynamically allocated memory, you can release it using the free() function. This deallocates the memory block and makes it available for reuse.
free(personPtr);
Uses Of Structure Pointer In C
Here are some important uses of structure pointers in C programming:
- Dynamic Memory Allocation: As discussed above, structure pointers in C are used to allocate memory dynamically for structures using functions like malloc(), calloc(), and realloc(). For example:
struct Person *ptr;
ptr = (struct Person *)malloc(sizeof(struct Person));
- Accessing Structure Members: Structure pointers in C provide a way to access structure members using the -> operator.
ptr->age = 30;
- Passing Structures to Functions: We can pass structures as an argument to functions using the structure pointers in C. This helps avoid copying the entire structure, which can be more efficient, especially for large structures.
void printPerson(struct Person *p) {
printf("Name: %s, Age: %d\n", p->name, p->age);
}
- Returning Structures from Functions: Functions can return pointers to structures, which can be useful for dynamic data creation or modification.
struct Person *createPerson(const char *name, int age) {
struct Person *newPerson = (struct Person *)malloc(sizeof(struct Person));
strcpy(newPerson->name, name);
newPerson->age = age;
return newPerson;
}
- Implementing Linked Data Structures: Structure pointers are essential for creating linked lists, trees, and other data structures that require dynamic linking between elements.
struct Node {
int data;
struct Node *next;
};
- Function Pointers in Structures: We can include function pointers within structures in C for callbacks or to implement polymorphism-like behavior.
struct Operations {
void (*print)(struct Person *);
};void printPerson(struct Person *p) {
printf("Name: %s, Age: %d\n", p->name, p->age);
}struct Operations ops = { printPerson };
ops.print(&person); // Call the function via the pointer
- Memory management: Structure pointers in C are useful for controlling the amount of memory used, especially when working with complicated data structures or numerous instances of the same structure.
- Implementing Object-Oriented Programming Concepts: While C is not inherently object-oriented, we can use pointers with structure pointers to emulate object-oriented concepts like encapsulation and polymorphism.
typedef struct {
void (*draw)(struct Shape *shape);
} Shape;typedef struct {
Shape base;
int radius;
} Circle;
Structures Containing Pointer Members In C
Structures in C can include pointer members, which means they can store the address of other data, rather than just data values. This feature is useful for managing complex data structures like linked lists or trees, where each member's pointer can dynamically connect to related data elements.
Syntax:
struct StructureName {
DataType *pointerMember; // Pointer to a data type or another structure
OtherDataType otherMember; // Other members
};
In this syntax, struct StructureName defines a structure with a pointer member (*pointerMember) that points to dynamically allocated memory or another structure, and an additional member (otherMember) of a different data type.
How To Initialize And Access Pointer Members Of A Structure In C
Here is how you can initialize and access structure pointer members:
- Declare a structure with a pointer member. Also, create an instance of the structure.
- Allocate memory for the data that the pointer member will point to. You can use functions like malloc() or calloc() to allocate memory dynamically.
- Verify that the memory allocation was successful by checking if the pointer is NULL.
- Assign a value to the pointer member.
- Access the value through the pointer member using the arrow operator (->).
- Print the value to verify.
- Don't forget to free the dynamically allocated memory when you're done.
Let's look at a code example to understand these steps.
Code:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Define the structure
struct Person {
char *name; // Pointer to a string
int age;
};
int main() {
// Declare and initialize the structure
struct Person p;
// Allocate memory for the name
p.name = malloc(50 * sizeof(char)); // Allocate space for 50 characters
// Check if memory allocation was successful
if (p.name == NULL) {
fprintf(stderr, "Memory allocation failed\n");
return 1;
}
// Initialize the structure members
strcpy(p.name, "Alia");
p.age = 30;
// Access and print the structure members
printf("Name: %s\n", p.name);
printf("Age: %d\n", p.age);
// Free the allocated memory
free(p.name);
return 0;
}
Output:
Name: Alia
Age: 30
Explanation:
In the above code example-
- We start by defining a structure named Person. This structure contains two members: name, which is a pointer to a character (a string), and age, which is an integer.
- Next, in the main() function, we declare a structure variable p of type struct Person. This variable will store data for one person.
- We then allocate memory for the name member of the structure. We use malloc() function to allocate space for 50 characters. This ensures that we have enough space to store a string of up to 49 characters plus a null terminator.
- We check if the memory allocation was successful by verifying if p.name is NULL.
- If malloc() fails to allocate memory, it returns NULL, and we print an error message to stderr(standard error) and exit the program with a non-zero status to indicate failure. The stderr stream is used in C programming for error reporting and diagnostics.
- If the memory allocation is successful, we use strcpy() function to copy the string "Alia" into the memory pointed to by p.name. We also set the age member of p to 30.
- To verify our initialization, we print the values of p.name and p.age using printf() function. This displays the name and age of the person stored in our structure.
- Finally, we release the allocated memory using the free() function. This step is crucial to prevent memory leaks by deallocating the memory that was previously allocated with malloc().
Conclusion
Structure pointers in C are pointers that hold the address of a structure. They allow us to dynamically allocate memory while the program is running, which is useful for creating complex data structures like linked lists, trees, and graphs that can change size as needed. This dynamic allocation is essential for applications where the amount of data is not known at compile time or where the data needs to be modified frequently.
One of the significant advantages of structure pointers is their ability to pass large structures to functions efficiently. By passing pointers rather than entire structures, developers can avoid the overhead of copying large amounts of data, thus improving program performance. Mastery of structure pointers is, therefore, a key skill for any C programmer aiming to write optimized and maintainable code.
Frequently Asked Questions
Q. What is the difference between a structure and a structure pointer in C?
A structure is a user-defined data type that groups together variables of different types under a single name. A structure pointer, on the other hand, is a pointer variable that holds the address of a structure.
While a structure directly contains the actual data values, a structure pointer references the location in memory where the structure is stored.
Q. Can a structure pointer point to another structure pointer?
In C programming, a structure pointer can point to another structure pointer. This capability is fundamental in creating dynamic data structures like linked lists, where each structure (or node) contains both data and a pointer to the next structure in the sequence. For example:
// Declare structure pointers
struct Node *head = NULL;
struct Node *second = NULL;
struct Node *third = NULL;// Allocate memory for each structure
head = (struct Node *) malloc(sizeof(struct Node));
second = (struct Node *) malloc(sizeof(struct Node));
third = (struct Node *) malloc(sizeof(struct Node));// Assign data and set pointers
head->data = 1;
head->next = second;second->data = 2;
second->next = third;third->data = 3;
third->next = NULL; // End of the linked list
The above code snippet demonstrates how structure pointers (head, second, third) are used to point to other structure pointers (next), forming a linked list structure in C.
Q. What is the difference between the arrow operator (->) and the dot operator (.) when accessing structure members?
The arrow operator (->) and the dot operator (.) are used to access structure members in C, but they serve different purposes:
Arrow Operator(->) | Dot Operator(->) |
It is used with a structure pointer to access members of the structure that the pointer points to. | It is used with a structure variable to directly access members of the structure. |
The arrow operator is a shorthand process. It automatically dereferences the pointer and accesses the member in one step. | We first need to dereference the pointer to get the actual structure and then use the dot operator to access its members. |
For example, ptr->member accesses member of the structure pointed to by ptr. | For example, var.member directly accesses member of the structure var. |
Q. What happens if malloc() fails to allocate memory for a structure pointer?
If malloc() fails to allocate memory for the structure, it returns NULL. This means that no memory was reserved, and the pointer intended to reference this memory will be NULL. Using this NULL pointer to access or modify data will cause the program to behave unpredictably, often resulting in crashes.
To avoid such issues, it's important to always check if the pointer is NULL after calling malloc(). If it is NULL, appropriate error handling, such as displaying an error message and stopping further execution, should be implemented to prevent further problems.
Q. What are the advantages of using structure pointers over regular structures?
Using structure pointers in C offers several advantages over regular structures. Structure pointers allow for dynamic memory allocation, which means memory can be allocated at runtime based on the program's needs rather than at compile-time with a fixed size. This flexibility is essential for handling varying amounts of data and for implementing complex data structures like linked lists, trees, and graphs.
Additionally, structure pointers enable efficient data manipulation and memory usage, as passing pointers to functions avoids the overhead of copying entire structures. This can lead to significant performance improvements, especially when dealing with large or numerous structures.
Q. What are the limitations of structure pointers in C?
Structure pointers in C, while powerful, also come with certain limitations that programmers should be aware of:
-
Dereferencing Complexity: Proper handling of dereferencing (*) and accessing structure members (->) can be error-prone, leading to bugs such as null pointer dereferencing.
-
Pointer Arithmetic Risks: Incorrect pointer arithmetic can lead to accessing invalid memory locations, causing unpredictable behavior and program crashes.
-
Static and Dynamic Confusion: Mixing static and dynamically allocated structures can lead to confusion in memory management and may complicate code maintenance.
-
Complexity in Nested Structures: Handling nested structures in memory or structures containing pointers to other structures requires careful management of memory allocation and data access.
-
Limited Type Checking: Lack of strong type checking when casting between different structure pointer types can lead to type-related errors that are difficult to debug.
-
Potential for Memory Fragmentation: Frequent allocation and deallocation of memory for structure pointers can lead to memory fragmentation, affecting overall code readability and efficiency.
-
Concurrency Issues: In concurrent programming, managing shared structure pointers across threads requires synchronization to avoid race conditions and data corruption.
-
Portability Concerns: Code using structure pointers may be less portable across different platforms or compilers due to differences in memory layout and pointer handling.
-
Debugging Challenges: Debugging programs involving structure pointers can be challenging due to difficulties in tracking memory-related issues and understanding pointer relationships.
Here are a few other interesting topics you must read:
- Difference Between Break And Continue Statements In C (+Explanation)
- Ternary (Conditional) Operator In C Explained With Code Examples
- Tokens In C | A Guide For All Types & Their Uses (With Examples)
- Bitwise Operators In C Programming Explained With Code Examples
- Decimal To Binary In C | 11 Methods (Examples + Complexity Analysis)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment