The 'this' Pointer In C++ | Declare, Use, Code Examples & More
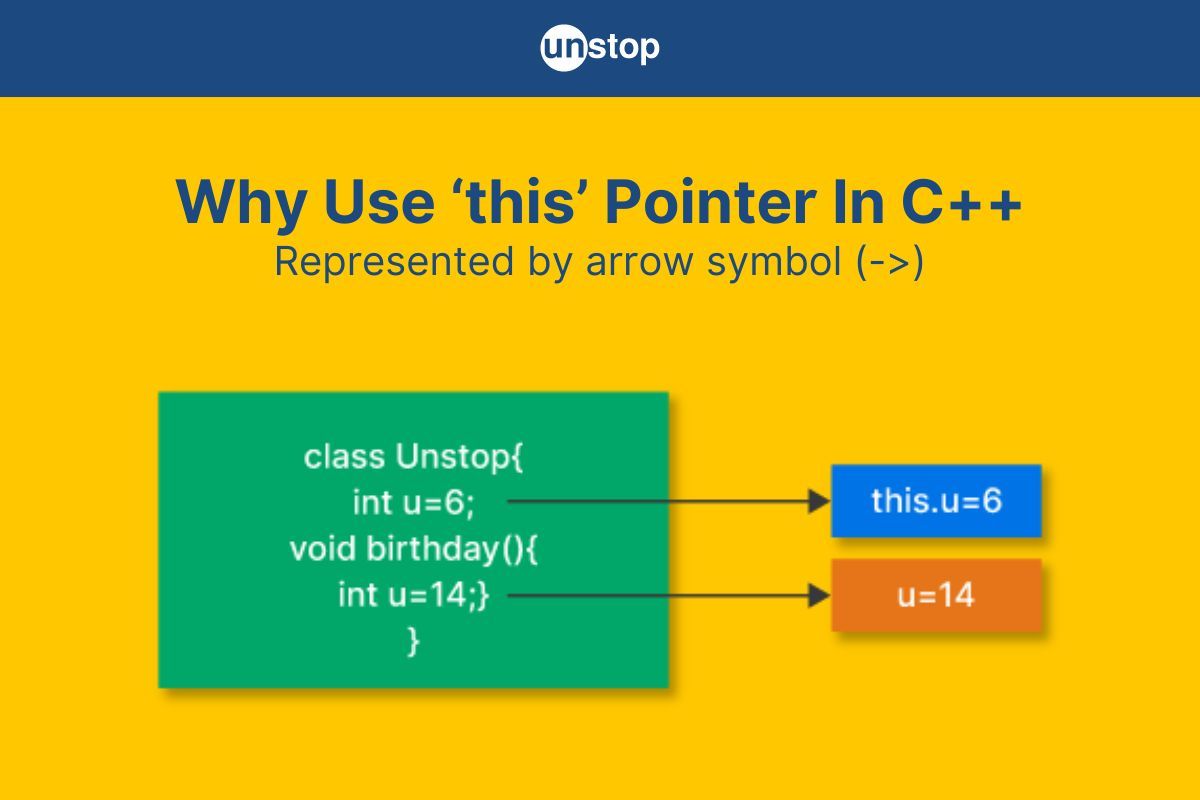
Table of content:
- What Is 'This' Pointer In C++?
- Defining ‘this’ Pointer In C++
- Example Of 'this' Pointer In C++
- Describing The Constness Of 'this' Pointer In C++
- Important Uses Of 'this' Pointer In C++
- Method Chaining Using 'this' Pointer In C++
- C++ Programs To Show Application Of 'This' Pointer
- How To Delete The ‘this’ Pointer In C++?
- Conclusion
- Frequently Asked Questions
The 'this' pointer is a unique pointer variable that is often used within classes. It holds the address of class objects and makes it easy for member function to access data members. In other words, it is a powerful concept in object-oriented programming languages that allows objects to refer to themselves within their own scope.
In this article, we will explore the purpose and behavior of 'this' pointer in C++, examine how it is used in various scenarios, and understand its significance and applications for object-oriented design.
What Is 'This' Pointer In C++?
The 'this' pointer in C++ is an implicit pointer available within non-static member functions of a class or structure. It points to the current object instance, letting the object access its own member variables and functions.
Let's have a look at the syntax of the 'this' pointer in C++ programming language and then an example for better understanding.
Syntax Of 'this' Pointer In C++
When creating or using the 'this' pointer, the 'this' keyword is used in conjunction with the arrow operator (->) along with the name of the member or method being referred to. The syntax for it is as follows:
void functionName() {
this->memberName = value;
}
Here,
- functionName refers to the identifier/ name you have given to the function.
- The term this-> represents the 'this' pointer in C++, and memberName refers to the respective member of the class you are trying to access.
An example showcasing the syntax in action is given below:
class MyClass {
public:
void myMethod() {
this->myMember = 42;
}
private:
int myMember;
};
Explanation:
In the above code snippet, we are trying to access the myMember variable/ attribute of the current object through the expression this->myMember, which is being assigned a value of 42 within the myMethod() function definition.
Defining ‘this’ Pointer In C++
In C++ programming, each object of a class has its own set of member variables/ attributes (also known as data members) and member functions.
- When a member function is called on an object, the 'this' pointer is automatically passed as a hidden argument to the member function.
- It enables the member function to have access to the object's data members (i.e., the class member variable) and call other member functions.
Note: Friend functions in C++ do not have access to this pointer.
Example Of 'this' Pointer In C++
The sample program given below demonstrates the implementation of the 'this' pointer in C++.
Code Example:
Output:
Name: Armaan
Age: 25
After updating age:
Name: Armaan
Age: 30
Explanation:
In this C++ code example, we have a Person class with private member variables/ data members: name and age. Inside the class-
- We have a class constructor that takes a reference to string variable name (string& name) and integer data type variable age as input.
- It initializes these data members with the values name and age, accessing them using the 'this' pointer.
- We also define a void member function called displayInfo(), which prints the current values of data members using the 'this' pointer (to access) and cout command to display.
- After that, we define another void member function, updateAge(), which takes an integer parameter newAge as input and updates the value of the age data member using the 'this' pointer.
- Inside the main() function, we create an object of class type Person, called person. Here, the initial values for data members, name and age are Armaan and 25, respectively.
- Then, we call the displayInfo() function on the object person to print the initial values to the console.
- Following this, we call the updateAge() function with 30 as input on the person object. This updates the value of age data member to 30.
- Once again, we call the displayInfo() function to print the updated age value to the console.
Also read: Static Member Function In C++ Explained With Proper Examples
Describing The Constness Of 'this' Pointer In C++
In C++, the constness of the 'this' pointer is an important aspect of understanding how member functions interact with class objects. We know that it is a special pointer available within nonstatic member function calls of a class, pointing to the object on which the member function is invoked.
The constness of the 'this' pointer in C++ is closely tied to whether the member function that uses it is marked as const.
- Const Member Function: When a member function is marked as const, it guarantees that it will not modify the state of the object. The 'this' pointer within such a function is treated as pointing to a constant object (const MyClass*). This means that within the function, you cannot alter any non-static member variables of the class.
- Non-Const Member Function: When a member function is not marked as const, the 'this' pointer is treated as pointing to a non-constant object (MyClass*). This allows the function to modify the state of the object.
Let's look at a code example to understand the concept better.
Code Example:
Output:
The bar() function is called on non-const object with value: 0
The value of x after calling bar(): 10
The foo() function is called on const object with value: 0
The value of x after calling foo(): 0
Explanation:
In this C++ code segment-
- We define a class called MyClass containing a private data member x and a constant foo() function, which prints a string message to the console when called.
- As mentioned in the code comments, the 'this' pointer used in this function is pointing to a constant object.
- Then, we define another function bar(), which also prints a message when called. Since this is non-constant, the 'this' pointer will also point to a non-constant object.
- Inside this function, we use the 'this' pointer to access data member x and use the compound assignment function to assign a new value that is incremented by 10.
- Lastly, in the class, we have a get () function which uses the 'this' pointer to access the value of variable x and returns the same.
- In the main() function, we create an object obj1 of type MyClass.
- Then, we call the bar() function on obj1 and use a cout command to print the value of x variable. Since x is uninitialized at this point (has a garbage value), the function increments it by 10.
- Following this, we call the getX() function on obj1 inside the cout command. This prints the value of x as updated by bar().
- Next, we create another object obj2 of type cont MyClass and call the foo() function on it.
- This function attempts to print the value of x, which is also a garbage value since it is uninitialized. Since obj2 is const, it cannot be modified. So the expression this->x += 10 results in a compilation error.
- After calling the foo() function, we call the getx() function on obj2 inside a coout command, which again accesses and prints its value.
Important Uses Of 'this' Pointer In C++
The 'this' pointer has several applications and uses. Here are some common scenarios where we use the 'this' pointer in C++ programs:
- Distinguishing between local variables and member variables: When a member variable has the same name as a local variable or parameter within a member function, the 'this' pointer in C++ allows you to explicitly refer to the member variable. This distinction helps avoid ambiguity and allows you to access or modify the intended class member variable.
- Returning the current object from a member function: Member functions can return the current object using the 'this' pointer in C++. This enables method chaining, where multiple member functions can be called on the same object in a single expression. It provides a concise and fluent style of programming.
- Accessing member variables and methods within member functions: The 'this' pointer in C++ provides a way to access and manipulate data members and member functions within other member functions. It allows for explicit reference to the single object itself, enabling operations on its state or invoking other member functions.
- Passing the current object as a parameter: The 'this' pointer in C++ can be passed as a parameter to other methods within a class. This allows those functions to operate on the current object, providing access to its state and behaviour.
- Resolving naming conflicts and accessing shadowed variables: When there is a naming conflict between member variables and local variables, the C++ 'this' pointer helps resolve the conflict by allowing explicit access to the member variables. It ensures that the intended variables are accessed and modified correctly.
- Callbacks and event handling: The 'this' pointer in C++ is often used in event-driven programming and callbacks. It allows event handlers or callback functions to access and operate on the object that registered the event or callback.
- Implementation of copy assignment and copy constructor: In the implementation of the copy assignment operator and copy constructor, the C++ 'this' pointer is used to reference the object being assigned or copied from. This helps in correctly copying the object's state.
These are some of the common applications of the 'this' pointer in C++. It provides a way to refer to the current object within member functions, enabling proper access and manipulation of member variables and methods.
Method Chaining Using 'this' Pointer In C++
Method chaining in C++ involves returning the object itself from each method so that subsequent methods can be called on the same object.
- It is a programming technique that allows multiple methods to be called sequentially on the same object instance without the need for intermediate variables or separate function calls.
- The result is a concise and readable code structure that promotes fluent interfaces.
- In method chaining, each method call modifies the object's state and returns a reference to the modified object.
- This allows subsequent methods to be called directly on the returned object, creating a chain of method invocations.
Let's look at an example showcasing the method chaining concept using the 'this' pointer in C++.
Code Example:
Output:
Result: 6
Explanation:
- In this example, we have a Calculator class with member functions that perform various arithmetic operations on the input value.
- Each method modifies the value member variable and returns a reference to the current object using the *this pointer.
- Inside the main() function, we create an object of class Calculator called calc and initialize it with the value of 10.
- We then chain functions, i.e., chain multiple method calls, applying arithmetic operations to the object and store the outcome in the result variable.
- Here we use the dot operator to chain and access the functions on the object calc. Lastly, we retrieve the result by calling getResult() function.
- By using method chaining, we can perform a series of arithmetic operations on the Calculator object in a single line of code, making the code more readable and concise.
- Finally, we print the value of the result variable using the std::cout statement.
Method chaining is a powerful technique that can be applied to various programming scenarios, such as configuration builders, query builders, and fluent interfaces. It enhances code expressiveness, improves readability, and reduces the need for temporary variables, resulting in more maintainable and efficient code.
C++ Programs To Show Application Of 'This' Pointer
Returning An Object Using The 'this' Pointer In C++
In C++, an object is returned to the caller in the form of a copy whenever a function does so. This might be helpful whenever the caller has to save the function's result for later use or utilize it to do further tasks in a C++ program.
Syntax:
Classname FunctionName() {
// Code to create and initialize an object
return object;
}
Here,
- Classname refers to the name of the current class whose object is being returned.
- FunctionName is the name of the function that returns the object, and object is the class instance in question.
Code Example:
Output:
x = 15, y = 25
Explanation:
In the sample C++ program-
- We create a Point class, which represents a point in a two-dimensional space. It has two private member variables, x, and y, representing the coordinates of the point.
- Then, we define a constructor that initializes these variables with 0, facilitating the creation of a point without any coordinates.
- Next, we define an overloaded function operator+() to add two Point objects. It takes constant reference to an object as input and returns a new point object after adding two points.
- Lastly, inside the class, we define the display() function, which uses cout commands to print the values of both data members.
- Inside the main() function-
- We create two objects of Point objects, p1 and p2, with coordinates (10, 15) and (5, 10), respectively.
- Then, we call the operator+() function on the object to add the two points thus creating a new point p3.
- Next, we call the display() function on the new point p3 to display its value.
- Lastly, the program terminates with a return 0 statement, indicating a successful execution.
Distinguishing Between Local Variables & Member Variables
In C++, the 'this' pointer is an implicit pointer that is essential for differentiating between member variables and local variables, especially when they have the same name. This distinction is crucial when assigning values to member variables or when retrieving their current state.
-
Local Variables are variables declared within a function body or a block of code. They are only accessible within the scope of that function or block.
-
Class Member Variables are variables declared within a class. They are accessible by all member functions of the class and represent the state of an object.
When local variables and member variables have the same name, you can use the 'this' pointer to refer to the member variables explicitly. The 'this' pointer points to the current object instance, allowing you to access its member variables.
Code Example:
Output:
Local value: 20
Member value: 10
Local value: 5
Member value: 10
Explanation:
In this example, the MyClass has a private member variable/ data member called value. The member function setValue() sets the value of the member variable using the parameter passed to it.
- The printValue() member function demonstrates the distinction between the local variable and the member variable with the same name. It takes an integer parameter named value.
- Within the function, we use the 'this' pointer to access the member variable explicitly and print its value.
- In the main() function, we declare a local variable named value and initialize with the value 5.
- Then, we call the setValue() function on the object obj, setting the member variable to 10.
- Subsequently, the printValue() member function is called twice, once with the value 20 as a parameter and once with the local variable value as a parameter.
Resolve Shadowing Issue Using 'this' Keyword In C++
The 'this' pointer in C++ can be used to resolve ambiguity whenever there is a shadowing issue in C++. That is, whenever a local variable or parameter has the same name as a member variable, the 'this' pointer can be used to explicitly refer to the member variable.
For example, the expression this->memberVariable, clearly indicates that we are trying to access a class data member called memberVariable. It helps differentiate between the local variable and the member variable. Let's take a look at an example of how that's done.
Code Example:
Output:
Local count: 10
Member count: 5
Explanation:
In this example, the MyClass has a member variable called count, and the constructor takes an integer parameter with the same name.
- Here, we use the 'this' pointer to resolve the shadowing issue and differentiate between the local parameter and the member variable.
- Inside the constructor, the expression this->count refers to the member variable, while the term count refers to the local parameter.
- We ensure that the member variable is correctly initialized by assigning the value of the parameter to the member variable using the 'this' pointer.
- Now, in the printCount() member function, we have both a local variable count and a member variable count.
- So, we resolve the shadowing issue by using the 'this' pointer (i.e., this->count) and explicitly accessing the member variable.
Using the 'this' pointer to resolve shadowing issues allows for explicit access to the member variables of a class, even when local variables or parameters have the same names. It ensures that the intended variables are accessed, avoiding ambiguity and ensuring correct behavior within the class methods.
Constructor & 'this' Pointer In C++
In C++, a constructor is a special member function that is automatically called when an object of a class is instantiated. It is responsible for initializing the object's member variables and setting up the initial state of the object. The 'this' pointer, which is implicitly available within non-static member functions, plays a crucial role during this initialization process.
Specifically, within a constructor, 'this' points to the object being created, allowing the constructor to distinguish between member variables and parameters with the same name. By using the 'this' pointer, a constructor can assign values to member variables, ensuring that the object is correctly initialized with the desired state. We have discussed a similar example in the section 'Resolve Shadowing Issue Using 'this' Keyword In C++' above.
Access Currently Executing Object Using 'this' Keyword In C++
The 'this' keyword in C++ allows you to navigate to the object that is currently running inside a member function of a class or struct. This enables you to call the member functions and variables of the object from a function.
Code Example:
Output:
Value of the current object: 10
Value of the current object: 20
The values are not equal.
Explanation:
In this example, the MyClass has a member variable called value. The constructor initializes the member variable using the parameter passed to it.
- The printValue() member function uses the 'this' pointer to access the member variable of the current object explicitly. It then prints the value of the member variable.
- The compareValues() member function demonstrates the use of the 'this' pointer to access the member variables of the current object and another object passed as a pointer. It compares the values of the member variables and prints a message accordingly.
- In the main() function, we create two objects of the MyClass: obj1 with value 10 and obj2 with value 20.
- Then, we call the printValue() member function is called on each object, printing their respective values.
- After that, we call the compareValues() member function on obj1, passing &obj2 as the argument to compare the values of both objects.
Calling Member Functions Using 'this' Keyword In C++
In C++, the 'this' pointer is used to call member functions within other member functions of a class. The 'this' pointer represents the current object, and using it to call member functions allows you to perform operations on the same object from within its own member functions. An example of the same is given below.
Code Example:
Output:
Calling bar()...
Calling foo()...
Explanation:
In the above C++ program, the MyClass has two member functions: foo() and bar().
- Here, the bar() function calls the foo() function using the 'this' pointer.
- Inside the bar() function, this->foo() is used to call the foo() member function on the current object.
- The 'this' pointer represents the object on which the bar() function is called.
- In the main() function, an object of the MyClass is created, and its bar() member function is called.
How To Delete The ‘this’ Pointer In C++?
It is important to note that one cannot explicitly delete the 'this' operator in C++. That is, the 'this' pointer is automatically managed by the language and does not need to be explicitly deleted.
- We know that the lifetime and deallocation of objects are handled by the language's memory management mechanisms.
- For example, stack allocation, automatic destruction, or manual deallocation through the 'delete' operator for dynamically allocated objects.
- The 'this' pointer is implicitly available within the scope of nonstatic functions of a class. It is a hidden pointer that points to the current object for which the member function is called.
- The program execution environment manages an object's memory, and the memory is released when the object goes out of scope or is explicitly deallocated.
The example below showcases the working mechanism behind this process.
Code Example:
Output:
Constructor called. this = 0x12345678 (Example address)
Object address: 0x12345678 (Example address)
Destructor called. this = 0x12345678 (Example address)
Explanation:
In this example, the MyClass has a constructor and a destructor.
- The constructor is called when the object is created, and the destructor is called when the object is destroyed or explicitly deallocated.
- In the main() function, a dynamic object of MyClass is created using the new operator, and its address is assigned to the obj pointer.
- The delete operator is then used to deallocate the object.
Conclusion
The 'this' pointer is a fundamental feature of C++ that plays a crucial role in object-oriented programming. It provides a way for member functions to access the address of the current object. It induces a range of functionalities from chaining method calls to implementing operator overloading. By understanding how to effectively use the 'this' pointer, we can write more intuitive and advanced-level development programs that help us enhance the power of C++'s object-oriented principles.
Frequently Asked Questions
Q. When is the this pointer used?
The this pointer is used in non-static member functions to refer to the current object instance. It is particularly useful for distinguishing between member variables and parameters with the same name, and for returning the current object from methods for method chaining.
Q. How many types of pointers are there in C++?
In C++, pointers can be classified into several types based on their usage and the kind of data they point to. Here are the main types:
-
Null Pointer: A pointer that is explicitly initialized to nullptr (or NULL in older code) to indicate that it is not pointing to any valid memory location.
-
Void Pointer: A generic pointer that can point to any data type. It must be cast to another pointer type before dereferencing. It is used when the data type of the pointer is unknown or not important.
-
Pointer to Object: Points to an object of a specific class or structure. It allows access to the object's members and methods.
-
Pointer to Member: Points to a member of a class. It is used to access or modify the members of a class through a pointer. This type of pointer requires special syntax and is less commonly used.
-
Pointer to Function: Points to a function, allowing dynamic invocation of functions. It can be used to pass functions as arguments or to implement callbacks.
Q. How does the 'this' pointer assist in method chaining?
The 'this' pointer facilitates method chaining by allowing member functions to return a reference to the current object (*this). This enables calling multiple methods on the same object in a single statement.
Q. What is a pointer to a derived class in C++?
A pointer that points to an object type of a derived class is referred to as a pointer to a derived class in C++.
- In other words, when you create a pointer that points to a derived class object, it can be referred to as a pointer to a derived class.
- The pointer to a derived class is especially useful when using a base class pointer to access the members or functions of a derived class (i.e., polymorphism).
Q. What is 'this' pointer function in CPP?
The keyword 'this' in C++ indicates a pointer to the currently selected object. The 'this' pointer is a hidden pointer that is implicitly passed to an object member function as the first function argument when it is called on an object.
- In a class member function, the current object can be specifically referred to using the 'this' pointer in C++.
- The member variable of the current object is, for instance, denoted by "this->memberVariable."
You might also be interested in reading:
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- Inline Function In C++ | Declare, Working, Examples & More!
- C++ Templates | Class, Function, & Specialization (With Examples)
- C++ Type Conversion & Type Casting Demystified (With Examples)
- Bitwise Operators In C++ Explained In Detail With Examples
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment