Destructor In C++ | Syntax, Rules, Properties & More (+Examples)
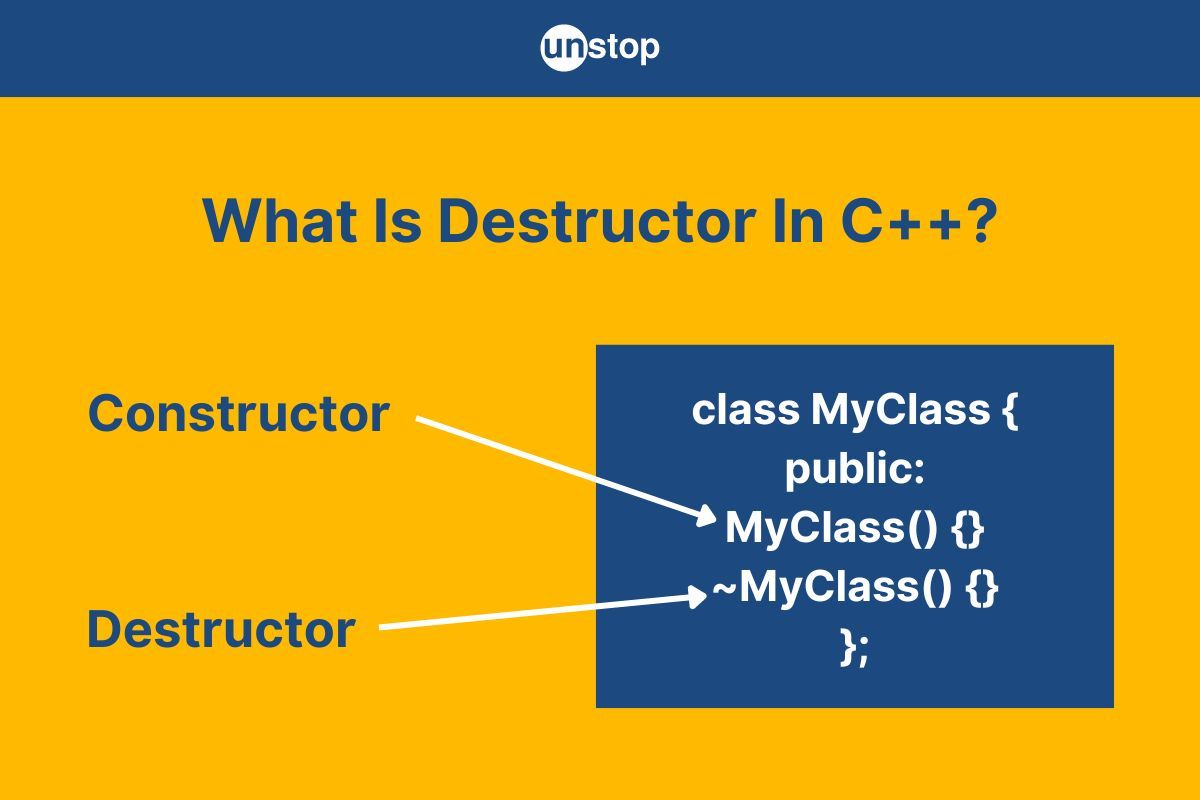
Table of content:
- What Is A Destructor In C++?
- Rules For Defining A Destructor In C++
- When Is A Destructor in C++ Called?
- Order Of Destruction In C++
- Default Destructor & User-Defined Destructor In C++
- Virtual Destructor In C++
- Pure Virtual Destructor In C++
- Key Properties Of Destructor In C++ You Must Know
- Explicit Destructor Calls In C++
- Destructor Overloading In C++
- Difference Between Normal Member Function & Destructor In C++
- Important Uses Of Destructor In C++
- Conclusion
- Frequently Asked Questions
A destructor in C++ programming is a special member function of a class that is automatically called when an object of that class goes out of scope or is explicitly deleted. The primary purpose of a destructor is to perform clean-up operations, such as releasing resources, closing files, or freeing dynamically allocated memory, ensuring that the object’s termination is handled gracefully.
This article will explore destructors in C++ in detail, covering their syntax, how they work, and best practices for their usage. We will also discuss scenarios where destructors are particularly useful and common pitfalls to avoid when implementing them in your classes.
What Is A Destructor In C++?
As mentioned earlier, a destructor in C++ language is a special member function that is automatically called when an object is about to be destroyed or exits its scope. Its primary purpose is to perform cleanup tasks and release resources that the object has accumulated throughout its lifetime. The name of a destructor in C++ is the same as the class name, prefixed by a tilde (~) symbol.
- The C++ runtime automatically calls the proper destructor to release any resources retained by an object when its lifetime ends.
- This could be either because the object exits scope or if it is explicitly removed using the delete keyword (in the case of objects created dynamically).
- This ensures appropriate dynamic memory management to prevent memory leaks and resource wastage.
Syntax Of Destructor In C++
class MyClass {
public:
// Constructor
MyClass() {
}
// Destructor
~MyClass() {
// Destructor code here
}
// Other member functions and data members
};
Here,
- MyClass is the name of the class being defined using the class keyword.
- MyClass() represents the constructor, which is called when a local variable of MyClass is defined and initialized.
- The term ~MyClass() refers to the destructor, whose code is executed when an object is destroyed.
Both the constructor and the destructor in C++ play key responsibilities in effectively handling object creation and destruction, ensuring correct resource management and cleaning throughout the object's lifespan.
Example Of Destructor In C++
Now, let's look at an example of a destructor in C++ to better understand the same.
Code Example:
Output:
The file closed successfully.
Explanation:
In the above code example-
- We begin by including two header files: <iostream> for input and output operations and <fstream> for file handling operations.
- Then, we define the FileHandler class, which is responsible for managing file operations. It has a private data member file of type std::ofstream, which represents an output file stream.
- Next, we define a constructor that takes a string parameter named filename and specifies the name of the file we want to open.
-
Inside the constructor:
- We first attempt to open the file using the file.open(filename) function.
- After trying to open the file, we check if the file was successfully opened by using the if-else statement.
- If the file fails to open, we output an error message to indicate the problem.
- We then define a destructor given by ~FileHandler() responsible for closing the file if it is still open. Inside-
- It uses an if-statement to check if the file is open using the file. is_open() function.
- If the condition is true, the destructor closes the file with file.close() and prints a message indicating successful closure to the console.
- In the main() function, we create an instance of the FileHandler class named fileHandler and pass example.txt as the filename.
- This triggers the FileHandler constructor, which attempts to open example.txt.
- After creating the fileHandler object, we can perform any file operations as needed.
- Once the main() function reaches its end and fileHandler goes out of scope, its destructor is automatically called.
- The destructor ensures the file is properly closed and any associated resources are released.
Just like a constructor, a destructor can also be declared both inside and outside of a class when writing a C++ program. Let's consider the changes in syntax when a destructor in C++ is declared outside of the class.
Syntax Of Destructor In C++ Outside Class
The following syntax can be used to specify the destructor for a C++ class outside of the class:
class MyClass {
public:
~MyClass(); // Destructor declaration
// Other member functions and data members
};// Destructor definition outside of the class
MyClass::~MyClass() {
// Destructor code here
}
Here,
- class MyClass is the name of the class being defined using the class keyword.
- ~MyClass(); is the declaration of the destructor within the class, indicating that a destructor exists but not providing its implementation.
- MyClass::~MyClass() is the definition of the destructor outside the class, using the scope resolution operator (::) to specify that this destructor belongs to MyClass.
Now, let's discuss an example of the destructor in C++ when it is defined outside the class.
Code Example:
Explanation:
- In this code, we define a FileHandler class to manage file operations.
- The constructor FileHandler() opens a file for writing and checks if the file was successfully opened.
- The destructor ~FileHandler() is defined outside the class to ensure the file is closed properly when the FileHandler object goes out of scope.
Rules For Defining A Destructor In C++
When defining a destructor in C++, there are several important rules to follow to ensure it functions correctly and efficiently:
- Name and Syntax Of The Destructor In C++: The destructor must have the same name as the class, prefixed with a tilde (~). Also, it cannot have any return type, not even void, and it cannot take any arguments. For Example-
class MyClass {
public:
~MyClass() {
// Destructor code here
}
};
- Only One Destructor: A class can have only one destructor in C++. You cannot overload a destructor, meaning you cannot have multiple destructors with different parameters.
- No Explicit Call: You should not explicitly call a destructor on an object. Instead, let the compiler manage the destruction process. Manually calling a destructor can lead to undefined behavior, especially if the object is later deleted again. For Example-
MyClass obj;
// obj.~MyClass(); // Not recommended, let the compiler handle it
- No Exception Throwing: Destructors should not throw exceptions. If an exception is thrown during a destructor’s execution and another exception is already active, the program will terminate. To handle exceptions, use a try-catch block within the destructor. For Example-
~MyClass() {
try {
// Code that might throw an exception
} catch (...) {
// Handle exceptions
}
}
- Destructor for Non-Resource Classes: If a class does not acquire resources that need explicit release (e.g., memory, file handles), it may not require a custom destructor. The compiler will provide a default destructor if none is defined.
- Destructor Order in Inheritance: In a class hierarchy, destructors are called in the reverse order of the constructor calls. The derived class’s destructor is called first, followed by the base class destructor.
These guidelines serve as the basis for the definition of a destructor in C++. They contribute to effective object decomposition and resource management, which results in more dependable and maintainable code.
When Is A Destructor in C++ Called?
The destructor in C++ may be called automatically in any of the following situations:
- When an object exits scope: The destructor of the object is automatically invoked before it is destroyed when the block of code in which it is declared ends. This guarantees that all cleaning and resource release procedures are carried out.
- When an object is explicitly deleted: After an object is created dynamically with the new operator, it may be deliberately destroyed by calling its destructor in C++ with the delete keyword. This deals with memory deallocation.
- When an object belongs to a different class: The destructors of all the objects that are members of the outer class are automatically invoked in the reverse order of their declarations when the destructor of an object that is a member of that class is called.
- When a container is cleaned or destroyed while an object is inside: The destructors of all the objects included in a container, such as a vector or list, are automatically called when the container is cleared or destroyed.
- When a smart pointer is used with an object: When a smart pointer itself exits its scope or is explicitly reset, it automatically calls the destructor of the object to which it is addressed. Examples of such smart pointers are std::shared_ptr and std::unique_ptr.
- When an item of a derived class is destroyed: If both a base class and a derived class have destructors, the destructor of the base class is called first when an object of the derived class is destroyed.
It is important to note that in C++, the runtime environment automatically handles destructor invocation in these situations, which helps in managing resources and avoiding memory leaks. This automatic management supports effective resource handling and simplifies the programmer's task.
Order Of Destruction In C++
The order of destruction in C++ mirrors the order of construction but in reverse. When an object is created, its base classes and member objects are constructed before the object itself.
Conversely, when an object is destroyed, the destructor of the member object is called first, followed by the destructors of its base classes and the object itself, in the reverse order of their declaration. Think of it like taking apart a toy. You'd remove the smaller parts (member objects) first, then the bigger parts (base classes), and finally the main piece (the object itself).
Here’s the sequence of destruction in C++:
-
Member Objects: The destructors of a class's member objects are called first when the object is destroyed. This includes every member object explicitly defined within the class.
-
Destructors of Base Classes: If the class is derived from a base class, the destructor of the base class is called next. The destructors are called from the most derived class to the base class along the entire inheritance chain.
-
Object Itself: Finally, the destructor of the object itself is executed once all member objects and base class destructors have been invoked.
Let us look at an example that showcases this sequence of constructor and destructor calls in C++ programs.
Code Example:
Output:
Base constructor
Member constructor
Derived constructor
Derived destructor
Member destructor
Base destructor
Explanation:
In the above code example-
- We start by defining three classes: Base, Member, and Derived.
- The Base class has a public constructor and destructor. When an object of this class is created, the constructor prints "Base constructor" to the console. When the object is destroyed, the destructor prints "Base destructor".
- Similarly, the Member class also has a public constructor and destructor. The constructor prints "Member constructor", and the destructor prints "Member destructor".
- The Derived class inherits from the Base class publicly. It contains a private member variable of type Member.
- The Derived class also has its own constructor and destructor. The constructor prints "Derived constructor", and the destructor prints "Derived destructor".
- In the main() function, we create an instance of the Derived class named obj. When obj is created, the constructors of its base class (Base) and its member (Member) are called first. This results in the output:
- Base constructor (from the Base class constructor)
- Member constructor (from the Member class constructor)
- Derived constructor (from the Derived class constructor)
- When obj goes out of scope at the end of the main() function, its destructor is called. This triggers the following sequence of destructor calls:
- Derived destructor (from the Derived class destructor)
- Member destructor (from the Member class destructor)
- Base destructor (from the Base class destructor)
- This sequence ensures that resources are properly released and destructor messages are printed in the reverse order of construction.
Default Destructor & User-Defined Destructor In C++
Default Destructor In C++
If you do not explicitly define a destructor in C++ classes, the compiler automatically generates a default destructor. It performs basic cleanup tasks by invoking the destructors of member objects and base classes. It is suitable for classes that do not manage dynamic memory or other resources needing explicit cleanup.
Characteristics Of Default Destructor In C++:
- Automatic Creation: If no destructor is explicitly defined, the compiler generates a default destructor in C++ programs.
- Basic Cleanup: It does not handle complex resource management or custom cleanup beyond calling destructors of member objects and base classes.
- Implicit: You don't see it in the class definition unless you specifically declare it, but it's always there if you don't provide one.
Let's look at an example that illustrates the use of a default constructor in C++ code.
Code Example:
Output:
Creating an object.
Constructor called.
Object created.
Explanation:
In the above code example-
- We start by defining a class called DefaultDestructorDemo with an explicit public constructor.
- In the constructor, we use std::cout to print the message to the console indicating when the constructor is being executed.
- In this class, we don’t explicitly define a destructor. Since we don’t provide one, the C++ compiler automatically generates a default destructor.
- The default destructor will be called when an object of DefaultDestructorDemo goes out of scope, but it doesn’t perform any special cleanup beyond the basic tasks provided by the compiler.
- In the main() function, we start by printing a message to the console indicating that we are about to create an object of the DefaultDestructorDemo class.
- We then declare an object named obj of type DefaultDestructorDemo. This triggers the constructor, and the corresponding message is printed to the console.
- After the object is created, we print another message to the console to signal that the object creation process is complete.
- As the main() function reaches its end, the obj goes out of scope. Here, the default destructor automatically generated by the compiler is called.
- Finally, the main() function returns 0, which indicates that the program has been executed successfully.
User-Defined Destructor In C++
A user-defined destructor in C++ is explicitly declared and defined by the programmer. It allows for custom cleanup and resource management when an object is destroyed. It is necessary for classes that manage dynamic resources, like memory allocated with new operator, file handles, or network connections.
Characteristics Of User-Defined Destructor In C++:
- Explicit Definition: You define it in your class to handle specific resource management tasks, such as releasing memory or closing files.
- Custom Cleanup: Allows for complex resource management and custom actions to be performed when the object is destroyed.
- Overriding: If a user-defined destructor is provided, it overrides the default destructor in C++ classes.
Code Example:
Output:
Creating an object.
Constructor called.
Object created.
Exiting the scope.
User-defined destructor called.
End of program.
Explanation:
In the above C++ code example-
- We define a class called UserDefinedDestructorDemo containing a public constructor and a user-defined destructor.
- The public constructor UserDefinedDestructorDemo() is a special member function that is called automatically when a class object is created. It uses std::cout to print a message to the console, letting us know when the constructor is being executed.
- We also define a user-defined destructor ~UserDefinedDestructorDemo(), which is called automatically when an object of class goes out of scope or is explicitly deleted. When called, it prints a message indicating the same.
- In the main() function, we first print a message to the console to indicate that we are about to create an object of the UserDefinedDestructorDemo class.
- We then declare an object named obj of type UserDefinedDestructorDemo. This action triggers the constructor, so a message is printed to the console, confirming that the object has been created.
- After the object is created, we print a message to the console to signal that the object creation process is complete.
- We then print another message to indicate that we are approaching the end of the main() function, where the object obj will go out of scope.
- As the main() function reaches its end, the object obj goes out of scope, which automatically triggers the user-defined destructor. Therefore a message is printed to the console, confirming that the destructor is being executed.
Virtual Destructor In C++
A destructor that is defined using the virtual keyword in a base class is known as a virtual destructor in C++. This technique is especially useful when a class is meant to serve as a base class, and pointers to the base class may be used to remove objects of derived classes. It is the virtual destructor that makes sure that the right destructor for a derived class is invoked when destroying an object via a base class pointer.
Syntax Of Virtual Destructor In C++
class Base {
public:
virtual ~Base() {
// Destructor code
}
};
The syntax for virtual destructor in C++ remains similar to that of regular destructor. The only difference is the use of the virtual keyword indicating its type.
Use Cases For The Virtual Destructor In C++
- Inheritance Hierarchies: When there is an inheritance structure in place, base class pointers are used to generate and manage instances of derived classes. To prevent resource leaks, it is essential to make sure the proper derived class destructor in C++ is executed after deletion.
- Dynamic binding and polymorphism: Polymorphic behavior is made possible via virtual destructors. The proper destructor in C++ is selected at runtime based on the type of the actual object when you use a base class reference to remove an object of a derived class.
Code Example:
Output:
Derived destructor
Base destructor
Explanation:
In the C++ code example-
- We define a Base class with a virtual destructor. The virtual destructor ensures that the correct destructor is called for derived objects when deleting a base class pointer.
- Next, we define a Derived class that inherits from Base. The Derived class has its own destructor that prints output to the console when called.
- In the main() function-
- We first create an object of the Derived class, which is dynamically allocated using the new keyword, and is given a pointer ptr of type Base. This allows us to use polymorphism, where the base class pointer can point to a derived class object.
- Then, the item referred to by ptr is destroyed using the delete operator. As a result, a series of destructors are invoked.
- The Derived class's destructor is called first, printing "Derived destructor".
- After that, the Base class destructor is invoked next as a result of the virtual destructor, printing "Base destructor".
- This ensures that both the derived and base class resources are properly cleaned up.
Pure Virtual Destructor In C++
In C++, a pure virtual destructor is one that is declared as pure virtual (just like pure virtual functions). Meaning it has no implementation in the base class and must be overridden in derived classes. This is achieved by assigning 0 to the destructor's declaration.
Pure virtual destructors in C++ are used in abstract base classes to prevent the base class from being instantiated directly and ensure that all derived classes provide their own implementation of the destructor.
Code Example:
Output:
Derived show function
Derived destructor called
Base destructor called
Explanation:
In the above code example-
- We define a class named Base containing a pure virtual destructor. It makes the Base class abstract, meaning it cannot be instantiated directly and that derived class must implement its own destructor to be instantiated.
- The Base class also has a pure virtual function, show(), that must be implemented by any derived class.
- Then, we define the pure virtual destructor outside the class. Even though the destructor is pure virtual, we must give a definition to ensure proper destruction of derived objects. Here, the destructor prints "Base destructor called" to indicate when it is invoked.
- Next, we define the Derived class, which publically inherits from Base. It provides its own implementation of the show() function, printing "Derived show function", and the destructor that prints "Derived destructor called".
- In the main() function, we create a Base class pointer b and initialize it with an object of Derived class, using new operator.
- Then, we call the show() function using base class pointer b and the 'this' pointer.
- This invokes the Derived class's implementation of the virtual function as shown my the message printing in the output console.
- Next, we use the delete operator to remove/ release the b pointer. This invokes the Derived destructor first, followed by the Base destructor, thus ensuring proper cleanup of resources for both classes.
Key Properties Of Destructor In C++ You Must Know
Let's look at some of the important characteristics of a destructor in C++:
- Automatic Invocation: When an automatic object leaves its scope or when a dynamically created object is deallocated using the delete operator, the destructor in C++ is automatically executed. The C++ runtime handles the destructor, i.e., there is no need to call it explicitly.
- Name: The destructor in C++ has the same name as the class, but it is preceded by the tilde symbol (~). For instance, the destructor will be called ~MyClass() if the class is called MyClass.
- No Return Type Or Arguments: Destructors don't accept any arguments and don't even have a void return type. They can't have too many distinct signatures on them.
- Number Of Destructors: Each class may only have one destructor in C++, which may be known as a single destructor. The compiler will build a default destructor if a class doesn't declare one. However, it's crucial to create a unique destructor if the class handles resource management or cleaning tasks.
- Derived Classes Inherit From Base Classes: When an object belonging to a derived class is destroyed, the base class's destructor will be immediately invoked. The destructor of the derived class is called after the destructor of the base class.
- Virtual Destructors For Polymorphic Behavior: It is frequently required to specify the base class destructor in C++ as virtual when promoting inheritance and polymorphism. This avoids memory leaks and unexpected behavior by ensuring that the appropriate destructor of the derived class is invoked when removing an object using a base class reference.
- Order Of Destruction: In the reverse order of their declarations, the member variables' destructors are invoked first when an object exits its scope. After that, the class's destructor is used.
- Implicitly Declared If Not Defined: If a class doesn't explicitly declare a destructor in C++, the compiler will construct one for it. To guarantee appropriate cleanup, it's recommended to create a custom destructor if the class maintains resources like dynamic memory allocations or file handles.
The destructor in C++ is crucial for resource management and stopping resource leaks. It is essential to preserve the program's integrity and make sure that allotted resources are released in the right way when they are no longer required.
Explicit Destructor Calls In C++
Explicit destructor calls in C++ refer to the manual invocation of a destructor for an object, rather than relying on the automatic destructor call that happens when an object goes out of scope or is deleted. Normally, destructors are automatically invoked when an object’s lifetime ends, but in certain cases, we might want to call the destructor explicitly. This can occur in scenarios like:
- Manual Memory Management: When dynamically allocating objects in C++ using a new operator (which allows constructing objects in pre-allocated memory), it becomes necessary to manually call the destructor to ensure proper resource cleanup. This is because the delete operator won’t be used, and thus the destructor won’t be automatically called.
- Resource Management in Custom Allocators: If you're implementing custom memory management or object pools, you might need to call destructors explicitly to ensure that objects release their resources before being returned to the pool or deallocated.
- Explicit Destructor Calls: In rare cases, you might explicitly need to call a destructor. This can be done by using the following syntax:
obj.~ClassName();
Here, obj is an instance of ClassName. Although this is technically possible, it is generally discouraged because it can lead to undefined or unexpected behavior if not handled carefully.
Potential Issues with Explicit Destructor Calls In C++
Explicitly invoking a destructor can lead to the following issues:
-
Destructed State: If you call a destructor explicitly and then attempt to use the object again, the object will be in a destructed state. This can lead to unpredictable behavior and bugs.
-
Inheritance Issues: In a class hierarchy, explicitly calling a destructor might not correctly follow the inheritance chain. This could result in base class destructors not being called, leading to incomplete cleanup and potential resource leaks.
-
Placement New and Manual Memory Management: In scenarios involving placement new, where you construct an object in a pre-allocated memory block, you might need to call the destructor explicitly before reusing or freeing that memory. This is one of the few cases where explicit destructor calls are justified.
In short, we discourage explicit calls to destructor in C++ programs because they run the risk of causing unexpected behavior and making manual memory management more difficult. The code will be more predictable and manageable if destructors are called automatically and correct memory management techniques are used.
Destructor Overloading In C++
Destructors in C++ cannot be overloaded. This is because destructors have a fixed signature: they take no parameters and do not return any value. A class can only have one destructor, which is automatically called when an object of the class is destroyed.
The purpose of a destructor is to perform clean-up tasks, such as releasing resources or freeing memory. Since the language does not allow destructors to have parameters, there is no scope for having multiple destructors with different parameter lists, which is the essence of function overloading. Below is an example illustrating why a class cannot have multiple destructors.
Code Example:
Explanation:
In the above code example,
We are attempting to define a second destructor ~MyClass(int x). This would result in a compilation error because C++ does not support destructor overloading. Only one destructor without parameters is allowed per class definition.
Difference Between Normal Member Function & Destructor In C++
To begin with, normal member functions refer to regular functions declared inside a class. Given below is a table outlining the key differences between a normal member function and a destructor in C++:
Aspect | Destructor | Normal Member Function |
---|---|---|
Name | Same as the class name, prefixed with a tilde character (~). | Can have any valid identifier as its name. |
Return Type | No return type (not even void). | Can have any return type, including void. |
Parameters | Cannot take any parameters (no arguments allowed). | Can take zero or more parameters, as needed. |
Overloading | Cannot be overloaded (only one destructor per class). | Can be overloaded with different parameter lists. |
Purpose | Used to perform cleanup tasks like releasing resources. | Can perform any task, depending on the function's purpose. |
Virtual Keyword | Often declared as virtual in base classes for polymorphism. | Can be declared virtual to allow overriding in derived classes. |
Order of Execution | Executed in reverse order of constructor calls in inheritance hierarchies. | Execution order depends on when and how it is called within the code. |
Explicit Call | Should not be called explicitly (handled by the compiler). | Must be explicitly called when needed. |
Exception Handling | Should generally not throw exceptions (to avoid program termination). | Can throw exceptions, and can use exception-handling mechanisms. |
Important Uses Of Destructor In C++
Destructors in C++ play a crucial role in resource management and cleanup when objects go out of scope or are explicitly deleted. Below are some of the most important uses of destructors in C++ programming:
-
Resource Deallocation: Destructors are commonly used to release resources that the object has acquired during its lifetime. This includes releasing system-level resources like file handles, network connections, and dynamically allocated memory.
-
Memory Management: To prevent memory leaks, objects that manage dynamically allocated memory, such as those using pointers to arrays or other dynamic data structures, should release that memory in their destructors.
-
File Handling: When an object is responsible for opening files or managing other external resources, its destructor should ensure that these resources are properly closed or released, preventing issues like file locks or resource exhaustion.
-
Database Connections: Destructors in classes that encapsulate database connections are used to close the connection and release associated resources when the object is no longer needed.
-
Network Sockets: Objects representing network connections or sockets can use destructors to close the socket and release any related resources when the communication is finished or the object goes out of scope.
-
Custom Cleanup Operations: In some cases, objects may manage complex data structures or resources that require more than just memory deallocation. For instance, releasing semaphores, resetting hardware states, or other specific cleanup tasks can be performed in the destructor.
-
Preventing Resource Leaks: Without a properly defined destructor, resources held by objects may not be released when the objects are no longer needed, leading to resource leaks and potential performance issues.
-
Base Class Polymorphism: When using polymorphism and inheritance, defining a virtual destructor in the base class ensures that the correct destructor of the derived class is invoked when an object is deleted through a base class pointer. This is essential for preventing memory leaks and ensuring proper cleanup.
-
Clean-up for Smart Pointers: Smart pointers like std::shared_ptr and std::unique_ptr use their destructors to automatically deallocate memory or release resources when the last reference to the pointed object is no longer in use.
Conclusion
A destructor in C++ is essential for appropriate resource management and cleaning in object-oriented programming. They control memory deallocation, guarantee automatic resource release, and enable customized cleanup actions. Destructor order follows the reverse order of object generation, and virtual destructors facilitate inheritance scenarios by ensuring that the relevant derived class destructors are called. Effective destructor techniques help developers maintain efficient and robust software systems while improving the stability of their code and reducing memory leaks.
Also read: 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What is the difference between a constructor and a destructor in C++?
Feature | Constructor | Destructor |
---|---|---|
Name | It has the same name as the class. | It has the same name as the class, preceded by a tilde (~). |
Parameters | It can have any number of parameters. | It cannot have any parameters. |
Return type | It does not have a return type. | It does not have a return type. |
Scope | It can be declared in any section of the class. | It must be declared in the public section of the class. |
Access specifier | Can have any access specifier | It is typically declared as public. |
Overloading | Can be overloaded | Cannot be overloaded |
Throwing exceptions | Can throw exceptions (temporary object goes out of scope). | Cannot throw exceptions |
Purpose | Initializes an object | Destroys an object |
When called | When an object is created | When an object is destroyed |
Q. How many arguments does a destructor in C++ have?
Destructors in C++ have no arguments since its primary function is to destroy objects that have either been explicitly deleted or have gone out of scope. Also, the name of the destructor is the same as the class name, preceded by a tilde (~).
Here is an example of a simple destructor program in C++:
class MyObject {
public:
~MyObject() {
// do some cleanup
}
};
As you can see, the destructor has no arguments. This is because destructors are not supposed to take any input from the object that they are destroying. They are simply supposed to clean up any resources that were allocated by the object. The compiler will generate an error if you try to define a destructor with arguments.
Q. What is a deconstructor in OOP?
In object-oriented programming, a destructor is a particular special function of a class that is called automatically whenever an object of that class is destroyed, either because it is being removed directly or going out of scope. It handles the cleaning chores related to an object's lifespan, releases resources, and carries out cleanup procedures using the destructor. A destructor in C++ is specified using the class name followed by a tilde sign (~).
Q. Does the destructor in C++ free memory?
Yes, a destructor is in charge of executing cleaning tasks, which frequently include releasing memory that was created during the lifetime of the object. However, the destructor itself does not immediately free the heap memory. When an object is destroyed in C++, either because it exits scope or is purposefully deleted, its destructor is immediately executed. You are in charge of making sure that any dynamically allocated memory or resources linked to the object are correctly freed in the destructor. This stops unnecessary resource utlization and memory leaks.
Q. When do we need to write a user-defined destructor in C++?
A destructor is a special member function used in object-oriented programming(OOPS) to clear up resources or carry out activities before an object is destroyed or deallocated. When your class maintains resources that are not automatically taken care of by the language's trash collection or memory management systems, you often need to create a user-defined destructor.
Here are several circumstances when creating a user-defined destructor in C++ may be necessary:
- Dynamic Memory Allocation: If your class dynamically allocates memory using C++'s new or C's malloc function, you should write a destructor that deallocates the memory location using the appropriate C++ or C normal function, delete expression or free. Failure to do so can cause memory leaks.
- File or Resource Handling: If your class controls files, network connections, or other external resources, you should create a destructor function to make sure that these resources are correctly closed or relinquished prior to the static object being destroyed. By doing this, resource leaks are avoided.
- Custom Resource Management: A destructor in C++ is necessary to appropriately release any custom resources that your class wraps around, such as database connections, graphics contexts, or hardware interfaces, when the object leaves its scope.
- Cleanup Tasks: A destructor for class can be used to gracefully carry out cleaning activities for your class, such as releasing locks, semaphores, or other synchronization mechanisms.
- Logging or Reporting: In some circumstances, you may want your class to log or report its status before being destroyed. Such last reporting responsibilities can be carried out by a destructor in C++.
Here are a few other interesting topics that you'll love reading:
- Friend Function In C++ | Class, Global Use, & More! (+Examples)
- Array Of Objects In C++ | A Complete Guide To Creation (Using Examples)
- C++ Templates | Class, Function, & Specialization (With Examples)
- Static Data Member In C++ | An Easy Explanation (With Examples)
- Pointers in C++ | A Roadmap To All Pointer Types (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment