- A Brief Intro To C++
- The Timeline Of C++
- Importance Of C++
- Versions Of C++ Language
- Comparison With Other Popular Programming Languages
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Variables In C++?
- Declaration & Definition Of Variables In C++
- Variable Initialization In C++
- Rules & Regulations For Naming Variables In C++ Language
- Different Types Of Variables In C++
- Different Types of Variable Initialization In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Primitive Data Types In C++?
- Derived Data Types In C++
- User-Defined Data Types In C++
- Abstract Data Types In C++
- Data Type Modifiers In C++
- Declaring Variables With Auto Keyword
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Structure Of C++ Program: Components
- Compilation & Execution Of C++ Programs | Step-by-Step Explanation
- Structure Of C++ Program With Example
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What is Typedef in C++?
- The Role & Applications of Typedef in C++
- Basic Syntax for typedef in C++
- How Does typedef Work in C++?
- How to Use Typedef in C++ With Examples? (Multiple Data Types)
- The Difference Between #define & Typedef in C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Strings In C++?
- Types Of Strings In C++
- How To Declare & Initialize C-Style Strings In C++ Programs?
- How To Declare & Initialize Strings In C++ Using String Keyword?
- List Of String Functions In C++
- Operations On Strings Using String Functions In C++
- Concatenation Of Strings In C++
- How To Convert Int To Strings In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is String Concatenation In C++?
- How To Concatenate Two Strings In C++ Using The ‘+' Operator?
- String Concatenation Using The strcat( ) Function
- Concatenation Of Two Strings In C++ Using Loops
- String Concatenation Using The append() Function
- C++ String Concatenation Using The Inheritance Of Class
- Concatenate Two Strings In C++ With The Friend and strcat() Functions
- Why Do We Need To Concatenate Two Strings?
- How To Reverse Concatenation Of Strings In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Find In String C++?
- What Is A Substring?
- How To Find A Substring In A String In C++?
- How To Find A Character In String C++?
- Find All Substrings From A Given String In C++
- Index Substring In String In C++ From A Specific Start To A Specific Length
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Pointers In C++?
- Pointer Declaration In C++
- How To Initialize And Use Pointers In C++?
- Different Types Of Pointers In C++
- References & Pointers In C++
- Arrays And Pointers In C++
- String Literals & Pointers In C++
- Pointers To Pointers In C++ (Double Pointers)
- Arithmetic Operation On Pointers In C++
- Advantages Of Pointers In C++
- Some Common Mistakes To Avoid With Pointers In Cpp
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Understanding Pointers In C++
- What Is Pointer To Object In C++?
- Declaration And Use Of Object Pointers In C++
- Advantages Of Pointer To Object In C++
- Pointer To Objects In C++ With Arrow Operator
- An Array Of Objects Using Pointers In C++
- Base Class Pointer For Derived Class Object In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is 'This' Pointer In C++?
- Defining ‘this’ Pointer In C++
- Example Of 'this' Pointer In C++
- Describing The Constness Of 'this' Pointer In C++
- Important Uses Of 'this' Pointer In C++
- Method Chaining Using 'this' Pointer In C++
- C++ Programs To Show Application Of 'This' Pointer
- How To Delete The ‘this’ Pointer In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What is Reference?
- What is Pointer?
- Comparison Table Of C++ Pointer Vs. Reference
- Differences Between Reference And Pointer: A Detailed Explanation
- Why Are References Less Powerful Than Pointers?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- How To Declare A 2D Array In C++?
- C++ Multi-Dimensional Arrays
- Ways To Initialize A 2D Array In C++
- Methods To Dynamically Allocate A 2D Array In C++
- Accessing/ Referencing Two-Dimensional Array Elements
- How To Initialize A Two-Dimensional Integer Array In C++?
- How To Initialize A Two-Dimensional Character Array?
- How To Enter Data In Two-Dimensional Array In C++?
- Conclusion
- Frequently Asked Questions
- What Are Arrays Of Strings In C++?
- Different Ways To Create String Arrays In C++
- How To Access The Elements Of A String Array In C++?
- How To Convert Char Array To String?
- Conclusion
- Frequently Asked Questions
- What is Memory Allocation in C++?
- The “new" Operator In C++
- The "delete" Operator In C++
- Dynamic Memory Allocation In C++ | Arrays
- Dynamic Memory Allocation In C++ | Objects
- Deallocation Of Dynamic Memory
- Dynamic Memory Allocation In C++ | Uses
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A Substring In C++ (Substr C++)?
- Example For Substr In C++
- Points To Remember For Substr In C++
- Important Applications Of substr() Function
- How to Get a Substring Before a Character?
- Print All Substrings Of A Given String
- Print Sum Of All Substrings Of A String Representing A Number
- Print Minimum Value Of All Substrings Of A String Representing A Number
- Print Maximum Value Of All Substrings Of A String Representing A Number
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Operator In C++?
- Types Of Operators In C++ With Examples
- What Are Arithmetic Operators In C++?
- What Are Assignment Operators In C++?
- What Are Relational Operators In C++?
- What Are Logical Operators In C++?
- What Are Bitwise Operators In C++?
- What Is Ternary/ Conditional Operator In C++?
- Miscellaneous Operators In C++
- Precedence & Associativity Of Operators In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is The New Operator In C++?
- Example To Understand New Operator In C++
- The Grammar Elements Of The New Operator In C++
- Storage Space Allocation
- How Does The C++ New Operator Works?
- What Happens When Enough Memory In The Program Is Not Available?
- Initializing Objects Allocated With New Operator In C++
- Lifetime Of Objects Allocated With The New Operator In C++
- What Is The Delete Operator In C++?
- Difference Between New And Delete Operator In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Types Of Overloading In C++
- What Is Operator Overloading In C++?
- How To Overload An Operator In C++?
- Overloadable & Non-overloadable Operators In C++
- Unary Operator Overloading In C++
- Binary Operator Overloading In C++
- Special Operator Overloading In C++
- Rules For Operator Overloading In C++
- Advantages And Disadvantages Of Operator Overloading In C++
- Function Overloading In C++
- What Is the Difference Between Operator Functions and Normal Functions?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Operators In C++?
- Introduction To Logical Operators In C++
- Types Of Logical Operators In C++ With Example Program
- Logical AND (&&) Operator In C++
- Logical NOT(!) Operator In C++
- Logical Operator Precedence And Associativity In C++
- Relation Between Conditional Statements And Logical Operators In C++
- C++ Relational Operators
- Conclusion
- Frequently Asked Important Interview Questions:
- Test Your Skills: Quiz Time
- Different Type Of C++ Bitwise Operators
- C++ Bitwise AND Operator
- C++ Bitwise OR Operator
- C++ Bitwise XOR Operator
- Bitwise Left Shift Operator In C++
- Bitwise Right Shift Operator In C++
- Bitwise NOT Operator
- What Is The Meaning Of Set Bit In C++?
- What Does Clear Bit Mean?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Types of Comments in C++
- Single Line Comment In C++
- Multi-Line Comment In C++
- How Do Compilers Process Comments In C++?
- C- Style Comments In C++
- How To Use Comment In C++ For Debugging Purposes?
- When To Use Comments While Writing Codes?
- Why Do We Use Comments In Codes?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Storage Classes In Cpp?
- What Is The Scope Of Variables?
- What Are Lifetime And Visibility Of Variables In C++?
- Types of Storage Classes in C++
- Automatic Storage Class In C++
- Register Storage Class In C++
- Static Storage Class In C++
- External Storage Class In C++
- Mutable Storage Class In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- Decision Making Statements In C++
- Types Of Conditional Statements In C++
- If-Else Statement In C++
- If-Else-If Ladder Statement In C++
- Nested If Statements In C++
- Alternatives To Conditional If-Else In C++
- Switch Case Statement In C++
- Jump Statements & If-Else In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A Switch Statement/ Switch Case In C++?
- Rules Of Switch Case In C++
- How Does Switch Case In C++ Work?
- The break Keyword In Switch Case C++
- The default Keyword In C++ Switch Case
- Switch Case Without Break And Default
- Advantages & Disadvantages of C++ Switch Case
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A For Loop In C++?
- Syntax Of For Loop In C++
- How Does A For Loop In C++ Work?
- Examples Of For Loop Program In C++
- Ranged Based For Loop In C++
- Nested For Loop In C++
- Infinite For Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is A While Loop In C++?
- Parts Of The While Loop In C++
- C++ While Loop Program Example
- How Does A While Loop In C++ Work?
- What Is Pre-checking Process Or Entry-controlled Loop?
- When Are While Loops In C++ Useful?
- Example C++ While Loop Program
- What Are Nested While Loops In C++?
- Infinite While Loop In C++
- Alternatives To While Loop In C++
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are Loops & Its Types In C++?
- What Is A Do-While Loop In C++?
- Do-While Loop Example In C++ To Print Numbers
- How Does A Do-While Loop In C++ Work?
- Various Components Of The Do-While Loop In C++
- Example 2: Adding User-Input Positive Numbers With Do-While Loop
- C++ Nested Do-While Loop
- C++ Infinitive Do-while Loop
- What is the Difference Between While Loop and Do While Loop in C++?
- When To Use A Do-While Loop?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Are 2D Vectors In C++?
- How To Declare 2D Vector In C++?
- How To Initialize 2D Vector In C++?
- C++ Program Examples For 2D Vectors
- How To Access & Modify 2D Vector Elements In C++?
- Methods To Traverse, Manipulate & Print 2D Vectors In C++
- Adding Elements To 2-D Vector Using push_back() Function
- Removing Elements From Vector In C++ Using pop_back() Function
- Creating 2D Vector In C++ With User Input For Size Of Column & Row
- Advantages of 2D Vectors Over Traditional Arrays
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- How To Print A Vector In C++ By Overloading Left Shift (<<) Operator?
- How To Print Vector In C++ Using Range-Based For-Loop?
- Print Vector In C++ With Comma Separator
- Printing Vector In C++ Using Indices (Square Brackets/ Double Brackets & at() Function)
- How To Print A Vector In C++ Using std::copy?
- How To Print A Vector In C++ Using for_each() Function?
- Printing C++ Vector Using The Lambda Function
- How To Print Vector In C++ Using Iterators?
- Conclusion
- Frequently Asked Questions
- Definition Of C++ Find In Vector
- Using The std::find() Function
- How Does find() In Vector C++ Function Work?
- Finding An Element By Custom Comparator Using std::find_if() Function
- Use std::find_if() With std::distance()
- Element Find In Vector C++ Using For Loop
- Using The find_if_not Function
- Find Elements With The Linear Search Approach
- Conclusion
- Frequently Asked Questions
- What Is Sort() Function In C++?
- Sort() Function In C++ From Standard Template Library
- Exceptions Of Sort() Function/ Algorithm In C++
- The Stable Sort() Function In C++
- Partial Sort() Function In C++
- Sorting In Ascending Order With Sort() Function In C++
- Sorting In Descending Order With Sort Function In C++
- Sorting In Desired Order With Custom Comparator Function & Sort Function In C++
- Sorting Elements In Desired Order Using Lambda Expression & Sort Function In C++
- Types of Sorting Algorithms In C++
- Advanced Sorting Algorithms In C++
- How Does the Sort() Function Algorithm Work In C++?
- Conclusion
- Frequently Asked Questions
- What Is Function Overloading In C++?
- Ways Of Function Overloading In C++
- Function Overloading In C++ Using Different Types Of Parameters
- Function Overloading In C++ With Different Number Of Parameters
- Function Overloading In C++ Using Different Sequence Of Parameters
- How Does Function Overloading In C++ Work?
- Rules Of Function Overloading In C++
- Why Is Function Overloading Used?
- Types Of Function Overloading Based On Time Of Resolution
- Causes Of Function Overloading In C++
- Ambiguity & Function Overloading In C++
- Advantages Of Function Overloading In C++
- Disadvantages Of Function Overloading In C++
- Operator Overloading In C++
- Function Overriding In C++
- Difference Between Function Overriding & Function Overloading In C++
- Conclusion
- Frequently Asked Questions
- What Is An Inline Function In C++?
- How To Define The Inline Function In C++?
- How Does Inline Function In C++ Work?
- The Need For An Inline Function In C++
- Can The Compiler Ignore/ Reject Inline Function In C++ Programs?
- Normal Function Vs. Inline Function In C++
- Classes & Inline Function In C++
- Understanding Inline, __inline, And __forceinline Functions In C++
- When To Use An Inline Function In C++?
- Advantages Of Inline Function In C++
- Disadvantages Of Inline Function In C++
- Why Not Use Macros Instead Of An Inline Function In C++?
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Static Data Member In C++?
- How To Declare Static Data Members In C++?
- How To Initialize/ Define Static Data Member In C++?
- Ways To Access A Static Data Member In C++
- What Are Static Member Functions In C++?
- Example Of Member Function & Static Data Member In C++
- Practical Applications Of Static Data Member In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constant In C++?
- Ways To Define Constant In C++
- What Are Literals In C++?
- Pointer To A Constant In C++
- Constant Function Arguments In C++
- Constant Member Function Of Class In C++
- Constant Data Members In C++
- Object Constant In C++
- Conclusion
- Frequently Asked Questions(FAQ)
- What Is Friend Function In C++?
- Declaration Of Friend Function In C++ With Example
- Characteristics Of Friend Function In C++
- Global Friend Function In C++ (Global Function As Friend Function )
- Member Function Of Another Class As Friend Function In C++
- Function Overloading Using Friend Function In C++
- Advantages & Disadvantages Of Friend Function in C++
- What Is A C++ Friend Class?
- A Function Friendly To Multiple Classes
- C++ Friend Class Vs. Friend Function In C++
- Some Important Points About Friend Functions And Classes In C++
- Conclusion
- Frequently Asked Questions
- What Is Function Overriding In C++?
- The Working Mechanism Of Function Overriding In C++
- Real-Life Example Of Function Overriding In C++
- Accessing Overriding Function In C++
- Accessing Overridden Function In C++
- Function Call Binding With Class Objects | Function Overriding In C++
- Function Call Binding With Base Class Pointers | Function Overriding In C++
- Advantages Of Function Overriding In C++
- Variations In Function Overriding In C++
- Function Overloading In C++
- Function Overloading Vs Function Overriding In C++
- Conclusion
- Frequently Asked Questions
- Errors In C++
- What Is Exception Handling In C++?
- Exception Handling In C++ Program Example
- C++ Exception Handling: Basic Keywords
- The Need For C++ Exception Handling
- C++ Standard Exceptions
- C++ Exception Classes
- User-Defined Exceptions In C++
- Advantages & Disadvantages Of C++ Exception Handling
- Conclusion
- Frequently Asked Questions
- What Are Templates In C++ & How Do They Work?
- Types Of Templates In C++
- What Are Function Templates In C++?
- C++ Template Functions With Multiple Parameters
- C++ Template Function Overloading
- What Are Class Templates In C++?
- Defining A Class Member Outside C++ Template Class
- C++ Template Class With Multiple Parameters
- What Is C++ Template Specialization?
- How To Specify Default Arguments For Templates In C++?
- Advantages Of C++ Templates
- Disadvantages Of C++ Templates
- Difference Between Function Overloading And Templates In C++
- Conclusion
- Frequently Asked Questions
- Structure
- Structure Declaration
- Initialization of Structure
- Copying and Comparing Structures
- Array of Structures
- Nested Structures
- Pointer to a Structure
- Structure as Function Argument
- Self Referential Structures
- Class
- Object Declaration
- Accessing Class Members
- Similarities between Structure and Class
- Which One Should You Choose?
- Key Difference Between a Structure and Class
- Summing Up
- Test Your Skills: Quiz Time
- What Is A Class And Object In C++?
- What Is An Object In C++?
- How To Create A Class & Object In C++? With Example
- Access Modifiers & Class/ Object In C++
- Member Functions Of A Class In C++
- How To Access Data Members And Member Functions?
- Significance Of Class & Object In C++
- What Are Constructors In C++ & Its Types?
- What Is A Destructor Of Class In C++?
- An Array Of Objects In C++
- Object In C++ As Function Arguments
- The this (->) Pointer & Classes In C++
- The Need For Semicolons At The End Of A Class In C++
- Difference Between Structure & Class In C++
- Conclusion
- Frequently Asked Questions
- What Are Static Members In C++?
- Static Member Functions in C++
- Ways To Call Static Member Function In C++
- Properties Of Static Member Function In C++
- Need Of Static Member Functions In C++
- Regular Member Function Vs. Static Member Function In C++
- Limitations Of Static Member Functions In C++
- Conclusion
- Frequently Asked Questions
- What Is Constructor In C++?
- Characteristics Of A Constructor In C++
- Types Of Constructors In C++
- Default Constructor In C++
- Parameterized Constructor In C++
- Copy Constructor In C++
- Dynamic Constructor In C++
- Benefits Of Using Constructor In C++
- How Does Constructor In C++ Differ From Normal Member Function?
- Constructor Overloading In C++
- Constructor For Array Of Objects In C++
- Constructor In C++ With Default Arguments
- Initializer List For Constructor In C++
- Dynamic Initialization Using Constructor In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constructor In C++?
- What Is Constructor Overloading In C++?
- Dеclaration Of Constructor Ovеrloading In C++
- Condition For Constructor Overloading In C++
- How Constructor Ovеrloading In C++ Works?
- Examples Of Constructor Overloading In C++
- Lеgal & Illеgal Constructor Ovеrloading In C++
- Types Of Constructors In C++
- Characteristics Of Constructors In C++
- Advantage Of Constructor Overloading In C++
- Disadvantage Of Constructor Overloading In C++
- Conclusion
- Frеquеntly Askеd Quеstions
- What Is A Destructor In C++?
- Rules For Defining A Destructor In C++
- When Is A Destructor in C++ Called?
- Order Of Destruction In C++
- Default Destructor & User-Defined Destructor In C++
- Virtual Destructor In C++
- Pure Virtual Destructor In C++
- Key Properties Of Destructor In C++ You Must Know
- Explicit Destructor Calls In C++
- Destructor Overloading In C++
- Difference Between Normal Member Function & Destructor In C++
- Important Uses Of Destructor In C++
- Conclusion
- Frequently Asked Questions
- What Is A Constructor In C++?
- What Is A Destructor In C++?
- Difference Between Constructor And Destructor In C++
- Constructor In C++ | A Brief Explanation
- Destructor In C++ | A Brief Explanation
- Difference Between Constructor And Destructor In C++ Explained
- Order Of Calling Constructor And Destructor In C++ Classes
- Conclusion
- Frequently Asked Questions
- Test Your Skills: Quiz Time
- What Is Type Conversion In C++?
- What Is Type Casting In C++?
- Types Of Type Conversion In C++
- Implicit Type Conversion (Coercion) In C++
- Explicit Type Conversion (Casting) In C++
- Advantages Of Type Conversion In C++
- Disadvantages Of Type Conversion In C++
- Difference Between Type Casting & Type Conversion In C++
- Application Of Type Casting In C++
- Conclusion
- Frequently Asked Questions
- What Is A Copy Constructor In C++?
- Characteristics Of Copy Constructors In C++
- Types Of Copy Constructors In C++
- When Do We Call The Copy Constructor In C++?
- When Is A User-Defined Copy Constructor Needed In C++?
- Types Of Constructor Copies In C++
- Can We Make The Copy Constructor In C++ Private?
- Assignment Operator Vs Copy Constructor In C++
- Example Of Class Where A Copy Constructor Is Essential
- Uses Of Copy Constructors In C++
- Conclusion
- Frequently Asked Questions
- Why Do You Need Object-Oriented Programming (OOP) In C++?
- OOPs Concepts In C++ With Examples
- The Class OOPs Concept In C++
- The Object OOPs Concept In C++
- The Inheritance OOPs Concept In C++
- Polymorphism OOPs Concept In C++
- Abstraction OOPs Concept In C++
- Encapsulation OOPs Concept In C++
- Other Features Of OOPs In C++
- Benefits Of OOP In C++ Over Procedural-Oriented Programming
- Disadvantages Of OOPS Concept In C++
- Why Is C++ A Partial OOP Language?
- Conclusion
- Frequently Asked Questions
- Introduction To Abstraction In C++
- Types Of Abstraction In C++
- What Is Data Abstraction In C++?
- Understanding Data Abstraction In C++ Using Real Life Example
- Ways Of Achieving Data Abstraction In C++
- What Is An Abstract Class?
- Advantages Of Data Abstraction In C++
- Use Cases Of Data Abstraction In C++
- Encapsulation Vs. Abstraction In C++
- Conclusion
- Frequently Asked Questions
- What Is Encapsulation In C++?
- How Does Encapsulation Work In C++?
- Types Of Encapsulation In C++
- Why Do We Need Encapsulation In C++?
- Implementation Of Encapsulation In C++
- Access Specifiers & Encapsulation In C++
- Role Of Access Specifiers In Encapsulation In C++
- Member Functions & Encapsulation In C++
- Data Hiding & Encapsulation In C++
- Features Of Encapsulation In C++
- Advantages & Disadvantages Of Encapsulation In C++
- Difference Between Abstraction and Encapsulation In C++
- Conclusion
- Frequently Asked Questions
- What Is Inheritance In C++?
- What Are Child And Parent Classes?
- Syntax And Structure Of Inheritance In C++
- Implementing Inheritance In C++
- Importance Of Inheritance In C++
- Types Of Inheritance In C++
- Visibility Modes Of Inheritance In C++
- Access Modifiers & Inheritance In C++
- How To Make A Private Member Inheritable?
- Member Function Overriding In Inheritance In C++
- The Diamond Problem | Inheritance In C++ & Ambiguity
- Ways To Avoid Ambiguity Inheritance In C++
- Why & When To Use Inheritance In C++?
- Advantages Of Inheritance In C++
- The Disadvantages Of Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Hybrid Inheritance In C++?
- Importance Of Hybrid Inheritance In Object Oriented Programming
- Example Of Hybrid Inheritance In C++: Using Single and Multiple Inheritance
- Example Of Hybrid Inheritance In C++: Using Multilevel and Hierarchical Inheritance
- Real-World Applications Of Hybrid Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Multiple Inheritance In C++?
- Examples Of Multiple Inheritance In C++
- Ambiguity Problem In Multiple Inheritance In C++
- Ambiguity Resolution In Multiple Inheritance In C++
- The Diamond Problem In Multiple Inheritance In C++
- Visibility Modes In Multiple Inheritance In C++
- Advantages & Disadvantages Of Multiple Inheritance In C++
- Multiple Inheritance Vs. Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Multilevel Inheritance In C++?
- Block Diagram For Multilevel Inheritance In C++
- Multilevel Inheritance In C++ Example
- Constructor & Multilevel Inheritance In C++
- Use Cases Of Multilevel Inheritance In C++
- Multiple Vs Multilevel Inheritance In C++
- Advantages & Disadvantages Of Multilevel Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Is Hierarchical Inheritance In C++?
- Example 1: Hierarchical Inheritance In C++
- Example 2: Hierarchical Inheritance In C++
- Impact of Visibility Modes In Hierarchical Inheritance In C++
- Advantages And Disadvantages Of Hierarchical Inheritance In C++
- Use Cases Of Hierarchical Inheritance In C++
- Conclusion
- Frequently Asked Questions
- What Are Access Specifiers In C++?
- Types Of Access Specifiers In C++
- Public Access Specifiers In C++
- Private Access Specifier In C++
- Protected Access Specifier In C++
- The Need For Access Specifiers In C++
- Combined Example For All Access Specifiers In C++
- Best Practices For Using Access Specifiers In C++
- Why Can't Private Members Be Accessed From Outside A Class?
- Conclusion
- Frequently Asked Questions
- What Is The Diamond Problem In C++?
- Example Of The Diamond Problem In C++
- Resolution Of The Diamond Problem In C++
- Virtual Inheritance To Resolve Diamond Problem In C++
- Scope Resolution Operator To Resolve Diamond Problem In C++
- Conclusion
- Frequently Asked Questions
Friend Function In C++ Classes | Types, Uses & More (+Examples)
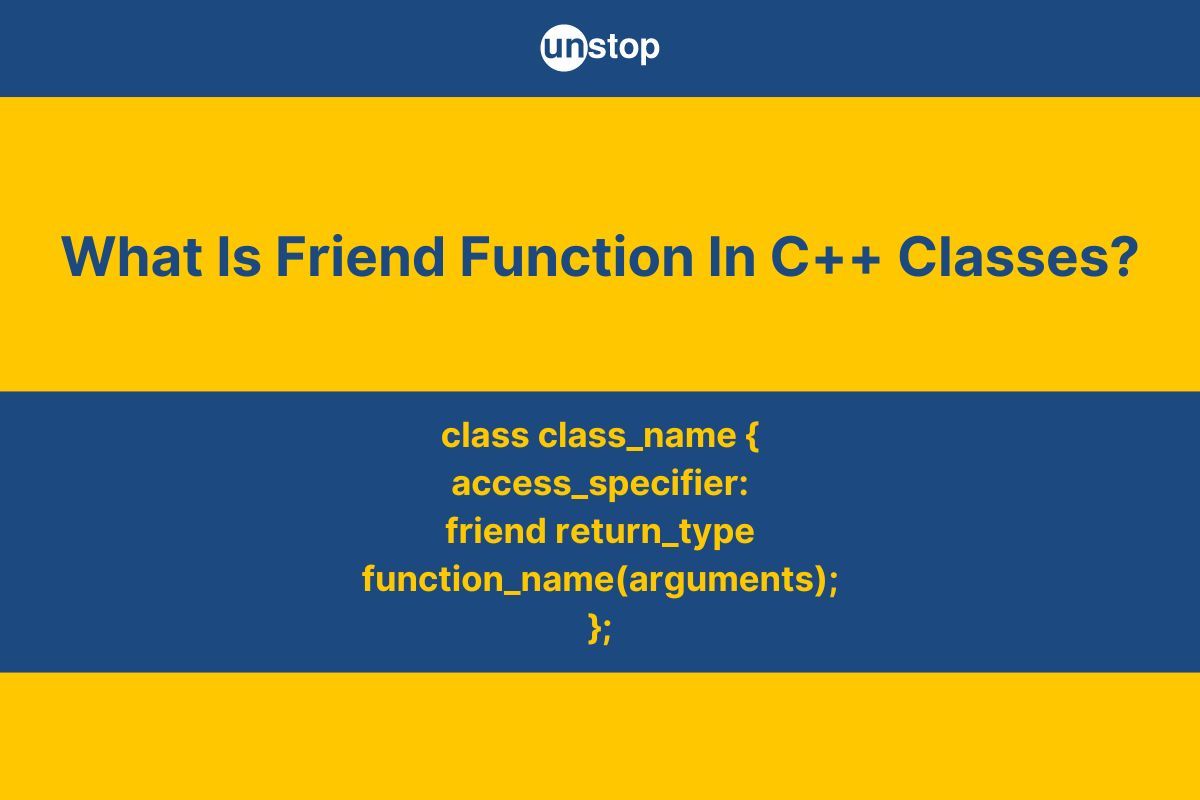
A friend function in C++ is a unique kind of function that has access to a class's private and protected members. They are defined outside the class but can be identified as friends within the class, in contrast to member functions, which are a part of the class and have direct access to its private members.
In this article, we will discuss everything about the friend function in C++ programming language, ranging from how to declare them, their characteristics, global friend functions, overloading using friend functions, and much more with the help of examples.
What Is Friend Function In C++?
It is a special type of function in C++ programming that is declared in a class but can be defined outside the class. However, it always has access to all private and protected members of a class. This allows the friend function to operate private and protected data outside the class.
- We must use the friend keyword to declare a friend function in C++ classes.
- A friend can be a member's function, function template, normal function, or class or class template, in which case the entire class and all of its members are friends.
- Understanding friend functions in C++ comes in handy when you need to give external functions access to a class's private members without making those members public.
- However, as they could weaken encapsulation and potentially make code more difficult to maintain, friend functionality should only be used selectively.
When communicating with a class's private members, it's generally preferable to use the public member functions.
Declaration Of Friend Function In C++ With Example
As mentioned before, a C++ friend function is declared by using the friend keyword. It can be declared anywhere in the class, i.e., private, protected, or public. The syntax for the same is given below, followed by an example C++ program.
Syntax Of Friend Function In C++:
class class_name {
access_specifier:
friend return_type function_name(arguments);
};
Here,
- The name/ identifier of the class is given by class_name, and the name of the friend function is given by function_name.
- The visibility mode of the function is given by access_specifier, which can be private, public or protected.
- The keyword friend signifies that the function is being declared as a friend function, which takes parameters represented by arguments.
- And return_type reflects the data type of the function's return value.
Now, let look at the implementation of the concept of friend function in C++ program given below.
Code Example:
#include <iostream>
using namespace std;
class MyClass {
private:
int privateData;
public:
MyClass(int data): privateData(data) {}
friend void friendFunction(MyClass obj);
};
// Definition of the friend function
void friendFunction(MyClass obj) {
cout << "The friend function can access privateData: " << obj.privateData << endl;}
int main() {
MyClass obj(42);
friendFunction(obj);
return 0;
}
Output:
The friend function can access privateData: 42
Explanation:
In the simple C++ program-
- We begin by including the <iostream> header to use the cout and cin commands and also use the namespace std.
- Then, we create a class called MyClass which has a private data member named privateData and a constructor to initialize it.
- We also declare a friendFunction a friend, which takes the MyClass object as an argument.
- As mentioned in code comments, we define the friendFunction outside the class. It will print the value of a private data member by using the object of MyClass and a cout statement.
- Next, in the main() function, an object of MyClass, obj, with the initial value 42, is created.
- Then obj is passed as a parameter when calling the friendFunction. The object's private member privateData is accessed inside the friendFunction and printed to the console using cout.
Note: It is evident from the example above that despite not being a member of the class itself, the friend function has access to the private member privateData of the MyClass object.
Characteristics Of Friend Function In C++
The friend function has many important characteristics that make it an integral part of C++ programming. Some important features of the friend function in C++ are as follows:
- Although friend functions are not class members, they have access to private and protected class members.
- A friend function in C++ program can be overloaded. You can declare multiple friends functions with the same name but different parameter lists.
- Friend functions are declared within the class using the friend keyword. They do not have a storage class specifier.
- They can be used to access and manipulate private data of the class without violating encapsulation.
- Friend functions in C++ can be defined inside or outside the class declaration.
- They can be regular functions or member functions of other classes declared as friends.
- Friend functions are not members of the class and do not have direct access to the class's 'this' pointer.
- A friend function in C++ cannot directly access the protected or private data members of the class. It has to make use of an object (instance of that class) either through the dot membership operator (.) or by passing a class object as an argument.
- Multiple functions or classes can be declared as friends of a single class.
- Derived classes do not inherit friend functions.
- Friendship is not mutual. That is, if class A is a friend of class B, it does not imply that class B is a friend of class A.
- The concept of friend function in C++ is typically used to provide external class member functions or classes with special access to private or protected members of a class.
Way To Implementat Friend Function In C++
A friend function in C++ can be implemented in two ways:
- As a global function
- As the member function of another class
We will discuss both of these in greater detail in the sections ahead.
Global Friend Function In C++ (Global Function As Friend Function )
The functions that are declared outside of a class or namespace and are accessible from any place in the program are known as global functions.
- The main() function can invoke these global friend functions in C++ without requiring an object.
- A global function can be attached as a friend function to one or more classes.
- Once attached, the global function has direct access to the class's private and protected members.
The syntax for the declaration of a global friend function in C++ is given below, along with an example of its implementation.
Syntax Of Global Friend Function In C++:
class class_name{
access_specifier:
friend return_type global_function(arguments);
};
return_type global_function(arguments) {
}
Here,
- The terms class_name and global_function refer to the names of the class that contains the friend function and the name of the global friend function, respectively.
- The access_specifier refers to the access controller, i.e., public, private, or protected.
- The keyword friend indicates that this is a friend function.
- arguments refer to the parameters that the friend function will take, and return_type reflects the data type of the value returned by the global_function.
Let’s understand the concept of the global friend function in C++ program with an example.
Code Example:
#include <iostream>
using namespace std;
class MyClass {
private:
int data;
public:
MyClass(int value) {
this->data=value;}
void displayData() {//function that displays the data
cout << "Data: " << data << endl;}
// Declare friend function using friend keyword
friend void multiplyByTwo(MyClass& obj);
};
// global function, which multiplies the number by two
void multiplyByTwo(MyClass& obj) {
obj.data *= 2; // it is accessing the private data of MyClass
}
int main() {
MyClass obj(5);
obj.displayData(); // Output: Data: 5
multiplyByTwo(obj);
obj.displayData(); // Output: Data: 10
return 0;
}
Output:
Data: 5
Data: 10
Explanation:
We begin the example C++ code by including essential headers and the namespace.
- Then, we create a class named MyClass with a private integer member, data, and a constructor to initialize it.
- The class includes a member function called displayData() to output the value of data using cout.
- Next, we declare is a friend function multiplyByTwo() declared inside the class, allowing it to access the private data member.
- Note that multiplyByTwo() is a global function and is a friend to MyClass.
- It uses the compound assignment operator (*=) to double the value of data for a given MyClass object, as given in the definition outside the class.
- In the main() function, a MyClass object obj is created with an initial value of 5.
- Then, we call multiplyByTwo(obj) function to double the value of data variable, and the updated value is displayed using displayData().
- As shown in the output, the friend function can access the data variable and returns the required value.
- Finally, the program terminates with a return 0 statement, indicating no errors in execution.
Note that the friend function doesn’t have access to this pointer. So, it has to use the object of a class to access the private data members.
Member Function Of Another Class As Friend Function In C++
In C++, a member function of one class may be designated as a friend function in another class. As a result, the class in which the friend function is declared has access to both its protected and private data members. Such a class can also referred to as the C++ friend class. Here is the class definition:
Syntax:
classclass_B; //Forward declaration of the class
class class_A {
public:
return_type friendFunction(arguments); //Declaring the friend function
};class class_B {
access_specifier:
friend return_type class_A::friendFunction(arguments);
};return_type class_A::friendFunction(arguments) {
}
Here,
- class_A refers to the names of the class that contains a class function that is a friend to class_B.
- class_B is the name of the class that declares the member function of class_A as a friend to it.
- access_specifier is an access controller, which could be public, private, or protected.
- The keyword friend signifies that the function is a friend function, and friendFunction refers to its name.
- The arguments are parameters that the friendFunction will take, and retun_type refers to the data type of the function's return value.
Code Example:
#include <iostream>
using namespace std;
class MyClass; // Forward declaration
class FriendClass {
public:
void friendFunction(MyClass obj); // Declaration of friend function
};
class MyClass {
private:
int privateData;
public:
MyClass(int data): privateData(data) {}
friend void FriendClass::friendFunction(MyClass obj); // Declaration of friend function from FriendClass
};
void FriendClass::friendFunction(MyClass obj) {
cout << "The friend function of FriendClass can access privateData: " << obj.privateData <<endl;}
int main() {
MyClass obj(42);
FriendClass fc;
fc.friendFunction(obj); // Output: Friend function of FriendClass can access privateData: 42
return 0;
}
Output:
The friend function of FriendClass can access privateData: 42
Explanation:
In the C++ example code-
- We first declared two classes MyClass and FriendClass.
- The FriendClass has a member function declaration named friendFunction, which will later be declared as a friend function in MyClass.
- The MyClass has a private member privateData, a constructor to initialize it, and a friend declaration, i.e., FriendClass::friendFunction.
- Note that the friendFunction is a member function of a FriendClass and a friend function to MyClass.
- The friend function is defined outside of a class using a scope resolution operator (::). The function prints the value of the privateData member of a MyClass object which is passed as an argument using cout.
- In the main() function, an object of MyClass named obj is created with an initial value of 42.
- Following this, we create an object of FriendClass named fc without any initial value.
- Next, we call friendFunction on the FriendClass object fc, passing the MyClass object obj as an argument.
- The friend function accesses and prints the private member privateData of MyClass.
Function Overloading Using Friend Function In C++
Function Overloading helps to achieve compile time polymorphism. One can declare multiple functions with the same name but different function prototypes inside a class and then make them friends by using function overloading with friend function in C++.
- These friend functions may be implemented outside of the class and may have various parameter lists.
- They can access the class's private and protected members by identifying them as friends, which enables them to behave differently depending on the function's parameters.
The use of the friend function in C++ to overload functions is an effective technique for offering several customized operations on the private data of the class. Look at overloading with friend function in C++ example below to gain a better understanding of this concept.
Code Example:
#include <bits/stdc++.h>
using namespace std;
class Bank {
private:
string account_holder;
int account_number;
int balance;
public:
Bank(int balance,string name,int account_number){
this->balance=balance;
account_holder=name;
this->account_number=account_number;}
//First overloaded execute function, which takes a reference to the bank object
friend void execute(Bank obj);
// second overloaded function, which takes two parameters and prints the account holderâs name
friend void execute(int account_num,Bank obj);
};
//defining the first overloaded function
void execute(Bank b) {
cout << "The balance in the bank is: " << b.balance << endl;
}
//defining the second overloaded function
void execute(int account_num,Bank obj) {
cout << "The account holder's name for the given account num is: " << obj.account_holder<<endl;}
int main() {
Bank b(42,"Sekhar",23561423);
execute(b);
int account_num =23561423;
execute(account_num,b);
return 0;
}
Output:
The balance in the bank is: 42
The account holder's name for the given account num is: Sekhar
Explanation:
We begin by including <bits/stdc++.h> header file and use the namespace std.
- Then, we create a class named Bank with three private data members, i.e., balance, account_number, and account_holder (string data type).
- The class also has a constructor to initialize the data members using 'this' pointer, i.e., Bank().
- Next, we define two friend functions with the same name, i.e., execute. This leads to function overloading since both have the same name but different prototypes. They are defined outside the class.
- The first execute function accepts a Bank obj (object) as an argument and prints the value using cout.
- The second execute function accepts an integer and Bank obj (object) and prints value using cout.
- Next, in the main() function, we create an object of Bank class named b and also define an integer variable account_num.
- Then, we call execute function twice, once with the obj object and once with the num variable along the Bank class object.
- The first execute function is a friend of the Bank class and can access the private data member balance, which is printed to the console.
- The second execute function accepts two parameters and prints the account holder's name.
Here, both execute functions have the same name but different prototypes, so the compiler decides which execute the function to be called based on the parameter types at compile type.
Advantages & Disadvantages Of Friend Function in C++
Listed in the table below are some of the major advantages and disadvantages of a friend function in C++.
Advantages |
Disadvantages |
Access to Private Members: Friend functions in C++ have access to a class's private and protected members, giving them the freedom to access and modify the internals of the class. Enhanced Encapsulation: A friend function in C++ can be used to hide most of the implementation details of a class while only exposing a small fraction of its interface to the outside world, which is known as enhanced encapsulation. Readability and Simplicity: The amount of code required can be decreased by using friend functions, which provide a natural and obvious way to accomplish certain activities that require access to hidden members of different classes. Greater Efficiency: The friend function in C++ improves the speed of a function call without the need for getter and setter functions. |
Access to Private Members: Friends functions in C++ have direct access to the private members of another class, which can lead to an increase in coupling between classes. If one class is changed, then all other associated classes might need to change without it actually being needed. Reduced Encapsulation: Heavy use of friend functions in C++ may reduce data hiding and data abstraction, which in turn reduces encapsulation Maintenance and Complexity: Due to the increase in the presence of the friend function in C++ programs, source code may become more complex. It is hence important to be aware of all the associations between classes before changing anything or using friend functions. Potential Security Risks: Misuse or overuse of a friend function in C++ can lead to security breaches due to unauthorized access. |
What Is A C++ Friend Class?
A friend class in C++ is similar to a friend function with special access privileges to another class. A class can identify another class as a friend to have access to the secret and protected members of that class. This promotes flexibility and cooperation across classes by allowing friend class to communicate with and alter the internal data of the other class. It is a way for classes to connect closely by building a relationship of friendship in which one class grants another class special access to its secrets.
Syntax Of C++ Friend Class
class class_name {
access_modifier:
friend class friend_class_name;
}
Here,
- class_name is the name of the class in which the friend class is declared
- access_modifier is an access controller which could be public, private, or protected.
- friend is the keyword used to declare a friend class.
- friend_class_name is the name of the class which is declared as a friend to class_name.
Code Example:
#include <iostream>
using namespace std;
class beta; // friend class
class alpha { // Class that builds a friendship with beta class
private:
int data;
public:
alpha(int data) {
this->data=data;}
friend class beta;
};
class beta {
public:
void display(const alpha& obj) {
cout<< "Private data of alpha class accessed by beta class: " << obj.data << endl;}
};
int main() {
alpha obj(42);
beta friend_Obj;
friend_Obj.display(obj);
return 0;
}
Output:
Private data of alpha class accessed by beta class: 42
Explanation:
In the example C++ program-
- We create the two classes named alpha and beta. The alpha class will give access to the friend class. Beta is the friend class that will have access to alpha's private members.
- The alpha class has a private member variable called data and a constructor to initialize it.
- Inside the alpha class, we have declared beta as a friend of alpha using the friend keyword. This grants beta access to the private members of alpha.
- In the beta class, we define a member function called display. This function takes a reference to an alpha object as a parameter and uses this object to access the private data member of the alpha class.
- The display function prints the private data member using the cout command.
- In the main function, we create an object of class alpha named obj and initialize it with a value of 42.
- Then we create an object of beta called friendObj.
- Next, we call the display function using friendObj and pass obj, the alpha class object, as an argument.
Note that display() is a member function of the beta class, which is a friend to the alpha class. It can hence access the private data member of alpha and print to the console.
A Function Friendly To Multiple Classes
In C++, a function can be declared as a friend to multiple classes. This makes it possible for the function to access the protected and private members of various classes. This will be useful when we need the classes to be collaborative and require strong communication between them. Let’s consider an example to better understand this concept.
Code Example:
#include <iostream>
using namespace std;
class ClassA; // Forward declaration
class ClassB; // Forward declaration
class FriendFunction {
public:
void doSomething(ClassA objA, ClassB objB); // Declaration of the friend function
};
class ClassA {
private:
int privateDataA;
public:
ClassA(int data): privateDataA(data) {}
friend void FriendFunction::doSomething(ClassA objA, ClassB objB);
// Declaration of friend function from FriendFunction
};
class ClassB {
private:
int privateDataB;
public:
ClassB(int data): privateDataB(data) {}
friend void FriendFunction::doSomething(ClassA objA, ClassB objB);
// Declaration of friend function from FriendFunction
};
void FriendFunction::doSomething(ClassA objA, ClassB objB) {
cout<< "Friend function can access privateDataA: " << objA.privateDataA << endl;
cout<< "Friend function can access privateDataB: " << objB.privateDataB << endl;}
int main() {
ClassA objA(42);
ClassB objB(24);
FriendFunction ff;
ff.doSomething(objA, objB); // Friend function can access privateDataB: 24
return 0;
}
Output:
Friend function can access privateDataA: 42
Friend function can access privateDataB: 24
Explanation:
In the example-
- We forward declare two classes, i.e., classA and classB, and then define a friend class called FriendFunction,
- The FriendFunction class has a member function declaration named doSomething, which will later be declared as a friend function in ClassA and ClassB.
- The ClassA has a private member named privateDataA and a constructor to initialize it.
- Similarly, ClassB has a private member named privateDataB and a constructor to initialize it.
- We have also declared a friend function, i.e., FriendFunction::doSomething, in both classes.
- Next, we define the doSomething friend function outside of the class using the scope resolution operator. It accepts objects of ClassA and ClassB as arguments.
- Inside the function, it accesses the private members privateDataA and privateDataB using corresponding objects. And prints the values of the members using the cout command.
- In the main() function, we create an object for both the classes, that for ClassA, objA and for ClassB, objB. We also initialize them with the values of 42 and 24, respectively.
- Next, we create an object of FriendFunction called ff.
- We then call the doSomething function using ff, and the objects of classA and classB are passed to it.
- The output is hence printed on the console, and the program finishes execution with a return 0.
C++ Friend Class Vs. Friend Function In C++
We have discussed the concepts of friend class and friend function in C++, in the sections above. Now let's take a look at the below, which highlights the differences between the two.
Friend function |
Friend Class |
It is a special function declared as a friend inside a class using the friend keyword. |
It is a class declared as a friend inside another class using the friend keyword. |
The friend function in C++ must fulfil a specific task using the secret information of a class. |
By default, all the functions inside a friend class are friend functions. Each one of them has a specific task to do. |
Friend functions in C++ are useful when we want to access the private and protected members of a class easily without making it more complex. |
Friend class is useful when there is a need for high communication between classes. |
Some Important Points About Friend Functions And Classes In C++
Here are some points that you must keep in mind when working with friend classes and friend functions in C++.
- Access to Private and Protected Members: Friend classes and functions have access to a class's private and protected members, including its protected members, private variables, and private methods. This enables more adaptable and effective code because some activities could need direct access to the members of the secret or protected data.
- Non-Inheritance Relationship: The use of friend classes and functions does not indicate that there is an inheritance link. They offer a way to access secret or protected members for particular functions or classes without the need for inheritance.
- Encapsulation and Information Hiding: Since friend functions and friend classes have direct access to a class's internal implementation details, they often break encapsulation and information-hiding rules. As a result, it's crucial to only use friend methods and friend classes when absolutely essential.
- Friendship is Not Mutual: By nature, friendship is not mutual. Class B is not necessarily a friend of class A just because class A is a friend of class B. If mutual access is wanted, friendship must be explicitly stated for both classes.
- Friendship is Not Inherited: If a derived class inherits from a base class, then the friend function of the base class doesn’t have access to the private data of the derived class. So, the friend function is not inherited in the derived class.
Conclusion
A friend function in C++ is a special function that is not a member of a class but has access to its protected and private members. They are often defined outside of the class but are declared inside of it using the friend keyword.
- They come in handy when you need to give particular functions or external classes access to a class's secret data without making those members publicly available.
- Since friend functions are not inherited, derived classes cannot use them.
- Friend functions in C++ are not connected to any instances of the class, and they lack this pointer.
- They are frequently used to overload operators so that non-member functions can interact with members of private classes.
- Although friend functions can increase flexibility and convenience in some situations. However, it is typically advised to use them rarely and to give priority to encapsulation.
- A friend class scope grants access to another class or multiple classes to manage its own private and protected members. This is done using a friend keyword.
- Friend classes offer a way of establishing strong relationships between classes and permit a more flexible and managed exchange of data and functionality.
- Friendship, i.e., friend relations, cannot be inherited, and it is non-commutative.
Frequently Asked Questions
Q. What is a friend function in C++ with example?
A friend function is a special type of function that is declared in a class as a friend and defined outside the class. It can access private and protected members of a class just like other member functions. Let’s understand with an example.
Code Example:
#include <iostream>
using namespace std;
class Circle {
private:
double radius;
public:
Circle(double r) : radius(r) {}
friend double calculateArea(const Circle& c);
};
// The friend function calculates the area of a Circle
double calculateArea(const Circle& c) {
double area = 3.14 * c.radius * c.radius;
return area;}
int main() {
Circle myCircle(5.0);
double area = calculateArea(myCircle);
cout << "Area of the circle: " << area << endl;
return 0;
}
Output:
Area of the circle: 78.5
Explanation:
- In this example, we created a Circle class with a private member variable named radius and a constructor to initialize it.
- Within the Circle class, the calculateArea function is listed as a friend function. This implies that it has access to the Circle objects' private member variables.
- The formula 3.14 * radius * radius is used by the calculateArea function to determine the area of a circle depending on its radius when it receives a constant reference to a Circle object as an argument.
- In the main function, we created the Circle class object myCircle, with a radius of 5.0.
- We then call the calculateArea function with the myCircle object. Since calculateArea is a friend function, it can access the private radius member of myCircle to perform the calculation.
Q. Is the friend function in C++ the same as the inheritance?
No, in C++, friend functions and inheritance are two different concepts. Friend functions (or classes) provide other functions (or other classes) access to its class's private and protected members.
- The class declares these functions as friends, granting them access to the private members while not being class members.
- The class hierarchy and inheritance do not apply to individual friend functions.
On the other hand, in object-oriented programming, inheritance is a method that enables a class (derived class) to inherit the characteristics and actions of another class (base class).
- Since the derived class inherits the base class's members (variables and functions), inheritance creates an Is-A relationship between classes.
- A class hierarchy is produced by inheritance, with derived classes inheriting and expanding the capabilities of the base class.
Q. What is the syntax of a friend class
The syntax to create a C++ friend class is given below, along with an explanation of its components.
Syntax:
class class_name {
access_modifier:
friend class friend_class_name;
}
Here,
- class_name: It is the name of the class in which the friend class is declared
- access_modifier: It is an access controller which could be a public, private, protected
- friend: It is the keyword used to declare a friend class
- friend_class_name: It is the name of the class which is declared as a friend to its class_name class.
Q. Is the friend function in C++ helpful in function overloading?
Yes, function overloading is a fundamental concept of writing multiple functions with the same name but different prototypes. A friend function in C++ helps to achieve function overloading by having multiple functions with customized behavior. Function overloading is often known as compile-time polymorphism. let’s see an example to understand the same.
Code Example:
#include <iostream>
using namespace std;
class MyClass {
private:
int data;
public:
MyClass(int d): data(d) {}
// Friend function declarations
friend void display(const MyClass& obj);
friend void display(const MyClass& obj, int value);
};
// Friend function definition for display
void display(const MyClass& obj) {
cout << "Data: " << obj.data << endl;}
// Overloaded friend function definition for display
void display(const MyClass& obj, int value) {
cout << "Data + Value: " << obj.data + value << endl;}
int main() {
MyClass myObj(42);
display(myObj); // Calls the first friend function
display(myObj, 10); // Calls the second overloaded friend function
return 0;
}
Output:
Data: 42
Data + Value: 52
Explanation:
In this example, a class called MyClass is created with a private data variable named data. The class also declares two friend functions with the same name display.
- The first display friend function takes a constant reference to a MyClass object as a parameter. It accesses the private member data of the MyClass object and prints its value to the console.
- The second display friend function is an overloaded function of the first display method. It takes a constant reference to a MyClass object and an additional integer value as parameters. It accesses the private member data of the MyClass object and adds the provided integer value to it.
- In the main function, an instance of the MyClass class named myObj is created with a value of 42.
- We then call the first friend function display with myObj as a parameter. It accesses and prints the value of the private member data using cout.
- Finally, we call the second friend function display with myObj and the value 10 as arguments. It accesses the private member data and adds 10 to it.
Q What is the difference between the friend function and an inline function in C++?
Some of the primary differences between the friend function in C++ and an inline function are as follows:
Friend function |
Inline function |
A friend function in C++ is a non-member function that has been identified as a friend of a class and has access to the class's private and protected members. |
An inline function in C++ refers to a function that is expanded at the time of its invocation rather than being called a distinct function. |
Typically, it is expressed using the friend keyword inside the friend class declaration. |
The inline keyword is used to declare and define it on a single line within the class declaration. |
Friend function in C++ may be created inside or outside of classes, and they may use the class object that has been supplied to them as a parameter to access class members. |
To increase performance by reducing the expense of a function call, inline functions are usually used for short, frequently called functions. |
Note:
When an inline function is invoked, the entire inline function's code is added or replaced at the inline function call position. The C++ compiler makes this substitution at the time of compilation.
Q. What is the need for the friend function in C++?
Friend functions play an important role in C++ programming. Listed below are some of the primary reasons why we need them.
- Accessing private members: Friend functions give certain external functions or classes controlled access to a class's private and protected members so they can work on them.
- Simplifying implementation: Friend functions can make the implementation of complicated actions involving numerous classes simpler by directly accessing and interacting with their private members.
- Operator overloading: To enable the implementation of customized behaviour for operators like +, -, *, etc., outside the class. A friend function in C++ is frequently used in operator overloading to allow access to private members.
Quiz Time!!!
You might also be interested in reading the following:
- C++ Type Conversion & Type Casting Demystified (With Examples)
- Destructor In C++ | Understanding The Key To Cleanups (+ Examples)
- C++ Exception Handling | Use Try, Catch, & Throw (+Examples)
- Constructor Overloading In C++ Explained With Real Examples
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Kartik Deshmukh 3 weeks ago
Deepa Kondapalli 1 month ago