Table of content:
- What Are Access Specifiers In C++?
- Types Of Access Specifiers In C++
- Public Access Specifiers In C++
- Private Access Specifier In C++
- Protected Access Specifier In C++
- The Need For Access Specifiers In C++
- Combined Example For All Access Specifiers In C++
- Best Practices For Using Access Specifiers In C++
- Why Can't Private Members Be Accessed From Outside A Class?
- Conclusion
- Frequently Asked Questions
Access Specifiers In C++ | Types & Usage Explained (+Code Examples)
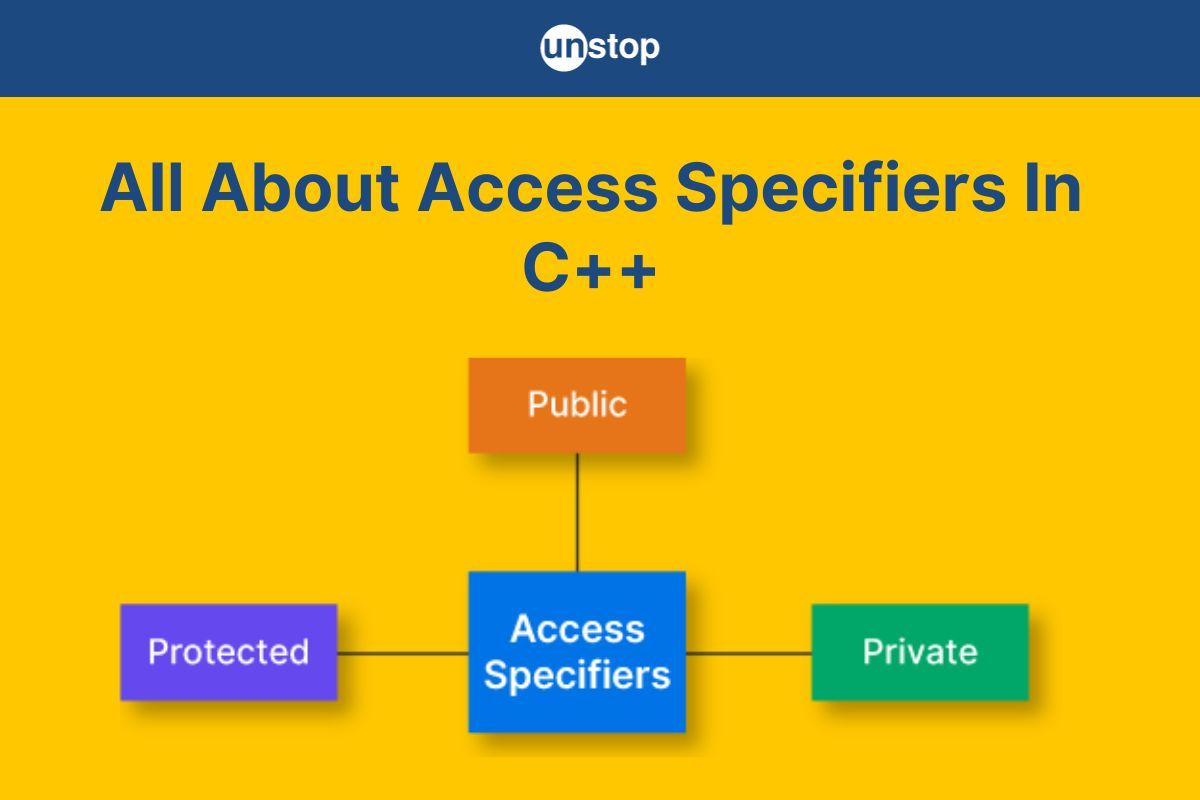
Access specifiers in C++ programming are keywords used to define the accessibility of members (attributes and methods) within a class. These specifiers—public, private, and protected—determine how and where the members of a class can be accessed, ensuring encapsulation and data hiding, which are crucial principles of object-oriented programming.
In this article, we'll explore the three primary access specifiers in C++ in detail. We'll discuss how each one affects the visibility and accessibility of class members, provide examples to illustrate their usage and examine common scenarios where each specifier is most appropriate.
What Are Access Specifiers In C++?
Access specifiers in C++ language are keywords that control who can access or use different components or members of a class. They decide if something is:
- Public: Open for everyone to use, like general information you share with everyone.
- Private: Hidden but accessible to anyone in a specific class, like personal information you share with family or a few trusted friends.
- Protected: Shared with only certain parts of the code, like secrets that you keep to yourself or maybe 1-2 confidants.
In other words, these access specifiers in C++ are a way to control who can access and interact with different pieces of a program. To understand this concept better, consider a class in your code as a house. Then-
- The public members of the class are like the front door, accessible to anyone (the public).
- Private members are like the inner rooms or personal belongings, i.e., you can't access them from outside.
- Protected members are like rooms accessible to family members (derived classes) but not to the public.
Using access specifiers in C++ provides multiple benefits:
- Enabling encapsulation: Protects the internal state of the object by controlling access to its data and methods.
- Data Hiding: Keeps implementation details private and exposes only what is necessary.
- Security: Ensures that critical parts of the code are not accidentally modified or accessed improperly.
Access specifiers in C++ programs promote better code organization, maintain data integrity, and enhance software reliability by designating members as public, private, or protected. Now, let's look at the syntax for these specifiers and an example to illustrate their usage.
Syntax Of Access Specifiers In C++
class MyClass {
public:
// Public members
int publicVar;private:
// Private members
int privateVar;protected:
// Protected members
int protectedVar;
};
Here,
- MyClass refers to the name of the class you have created. It contains all three types of access modifiers with integer variables declared.
- The public, private, and protected are access specifiers, which further contain publicVar, privateVar, and protectedVar variables of integer data type, respectively.
We will discuss each access specifier in detail individually, but first, let's look at a basic example illustrating their usage.
Example Of Access Specifiers In C++
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgTXlDbGFzcyB7CnB1YmxpYzoKaW50IHB1YmxpY1ZhcjsgLy8gUHVibGljIG1lbWJlcgoKLy8gQ29uc3RydWN0b3IKTXlDbGFzcyhpbnQgcHViVmFyLCBpbnQgcHJpVmFyLCBpbnQgcHJvVmFyKQo6IHB1YmxpY1ZhcihwdWJWYXIpLCBwcml2YXRlVmFyKHByaVZhciksIHByb3RlY3RlZFZhcihwcm9WYXIpIHt9Cgp2b2lkIFB1YmxpY0Z1bmN0aW9uKCkgewpzdGQ6OmNvdXQgPDwgIlB1YmxpYyBGdW5jdGlvbjogcHVibGljVmFyID0gIiA8PCBwdWJsaWNWYXIgPDwgc3RkOjplbmRsOwpQcml2YXRlRnVuY3Rpb24oKTsgLy8gQWNjZXNzaW5nIGEgcHJpdmF0ZSBmdW5jdGlvbiBmcm9tIGEgcHVibGljIG9uZQpQcm90ZWN0ZWRGdW5jdGlvbigpOyAvLyBBY2Nlc3NpbmcgYSBwcm90ZWN0ZWQgZnVuY3Rpb24gZnJvbSBhIHB1YmxpYyBvbmUKfQoKcHJpdmF0ZToKaW50IHByaXZhdGVWYXI7IC8vIFByaXZhdGUgbWVtYmVyCgp2b2lkIFByaXZhdGVGdW5jdGlvbigpIHsKc3RkOjpjb3V0IDw8ICJQcml2YXRlIEZ1bmN0aW9uOiBwcml2YXRlVmFyID0gIiA8PCBwcml2YXRlVmFyIDw8IHN0ZDo6ZW5kbDsKfQoKcHJvdGVjdGVkOgppbnQgcHJvdGVjdGVkVmFyOyAvLyBQcm90ZWN0ZWQgbWVtYmVyCgp2b2lkIFByb3RlY3RlZEZ1bmN0aW9uKCkgewpzdGQ6OmNvdXQgPDwgIlByb3RlY3RlZCBGdW5jdGlvbjogcHJvdGVjdGVkVmFyID0gIiA8PCBwcm90ZWN0ZWRWYXIgPDwgc3RkOjplbmRsO30KfTsKCmNsYXNzIERlcml2ZWRDbGFzcyA6IHB1YmxpYyBNeUNsYXNzIHsKcHVibGljOgpEZXJpdmVkQ2xhc3MoaW50IHB1YlZhciwgaW50IHByaVZhciwgaW50IHByb1ZhcikgOiBNeUNsYXNzKHB1YlZhciwgcHJpVmFyLCBwcm9WYXIpIHt9Cgp2b2lkIEFjY2Vzc0Jhc2VNZW1iZXJzKCkgewpzdGQ6OmNvdXQgPDwgIkRlcml2ZWQgQ2xhc3MgQWNjZXNzOiIgPDwgc3RkOjplbmRsOwovLyBBY2Nlc3NpbmcgcHVibGljIG1lbWJlciBmcm9tIHRoZSBiYXNlIGNsYXNzCnN0ZDo6Y291dCA8PCAicHVibGljVmFyIChmcm9tIGJhc2UgY2xhc3MpID0gIiA8PCBwdWJsaWNWYXIgPDwgc3RkOjplbmRsOwoKLy8gQXR0ZW1wdGluZyB0byBhY2Nlc3MgcHJpdmF0ZSBhbmQgcHJvdGVjdGVkIG1lbWJlcnMgKGNvbXBpbGUtdGltZSBlcnJvcnMpCi8vIHN0ZDo6Y291dCA8PCAicHJpdmF0ZVZhciAoZnJvbSBiYXNlIGNsYXNzKSA9ICIgPDwgcHJpdmF0ZVZhciA8PCBzdGQ6OmVuZGw7Ci8vIHN0ZDo6Y291dCA8PCAicHJvdGVjdGVkVmFyIChmcm9tIGJhc2UgY2xhc3MpID0gIiA8PCBwcm90ZWN0ZWRWYXIgPDwgc3RkOjplbmRsOwp9Cn07CgppbnQgbWFpbigpIHsKLy8gQ3JlYXRpbmcgaW5zdGFuY2VzIG9mIE15Q2xhc3MgYW5kIERlcml2ZWRDbGFzcwpNeUNsYXNzIG9iajEoMSwgMiwgMyk7CkRlcml2ZWRDbGFzcyBvYmoyKDQsIDUsIDYpOwoKLy8gQWNjZXNzaW5nIHB1YmxpYyBtZW1iZXIgYW5kIGZ1bmN0aW9uCm9iajEuUHVibGljRnVuY3Rpb24oKTsKCi8vIEFjY2Vzc2luZyBwcm90ZWN0ZWQgbWVtYmVyIGFuZCBmdW5jdGlvbiBmcm9tIHRoZSBkZXJpdmVkIGNsYXNzCm9iajIuQWNjZXNzQmFzZU1lbWJlcnMoKTsKCnJldHVybiAwOwp9
Output:
Public Function: publicVar = 1
Private Function: privateVar = 2
Protected Function: protectedVar = 3
Derived Class Access: publicVar (from base class) = 4
Explanation:
We begin the code above by creating a base class called MyClass.
- Inside the class, we declare a public member variable publicVar, private data member, privateVar, and protected data member, protectedVar, all of the int type.
- We also define a public constructor that initializes the values of all these members when an instance of the class is created.
- Next, we also define three functions-
- A public function, PublicFunction(), accesses the public variable and the private and protected functions. It prints a phrase to the console using std::cout.
- The second function is PrivateFunction(), which accesses the privateVar and prints its value.
- Similarly, the third function, ProtectedFunction(), accesses the protectedVar and prints its value to the console.
- Next, we create a derived class called DerivedClass, which inherits from MyClass with the public specifier.
- Inside the derived class-
- We have a public constructor to initialize the base class members.
- A public member function called AccessBaseMembers() accesses the base class public members and prints a phrase to the console.
- Next, as mentioned in the comments, if we try to access the private and protected members of the base class, we will run into a compile-time error.
- In the main() function-
- We create an instance of MyClass named obj1, with the initial values of its data members set to 1 (publicVar), 2 (privateVar), and 3 (protectedVar).
- Next, we create an instance of DerivedClass named obj2, with initial values for its members set to 4, 5, and 6.
- Then, we use obj1 and dot operator to call the PublicFunction() function, i.e., obj1.PublicFunction().
- The function thus accesses and prints the values of public, private, and protected members and functions from MyClass.
- Next, we use the derived class object to call the AccessBaseMembers() function, which demonstrates the access to public members from the base class but results in compile-time errors when attempting to access private and protected members from the base class within the derived class.
Types Of Access Specifiers In C++
There are three main types of access specifiers in C++ (or access modifiers), which control the visibility and accessibility of class members. These access specifiers are pivotal in defining how different parts of a class can be accessed and utilized within a program. In this section, we will discuss these three types of access specifiers in greater detail.
Public Access Specifiers In C++
The public access specifier in C++ is a keyword used to define class members that are accessible from anywhere in the program, including external code. In simple words, they are publicly available to access from all program sections.
- Members declared as public form the interface of the class, providing a means for external code to interact with the class's functionality. They are the most visible and accessible components of a class.
- Think of public members like books on a library’s open shelves intended for public use. Anyone can access and borrow these books without restrictions, just as anyone can access and use public class members (using a direct member access operator).
Syntax Of Public Access Specifier In C++
class MyClass {
public:
// Public members
int publicVar;
};
Here,
- MyClass is the name of the C++ class you have created.
- The public keyword indicates that entities contained will be publicly accessible, even from outside the class.
- publicVar is the name of the variable in MyClass that can be accessed and modified from anywhere in the program.
Example Of Public Access Specifiers In C++
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgQ2FyIHsKcHVibGljOgpzdGQ6OnN0cmluZyBtYWtlOwpzdGQ6OnN0cmluZyBtb2RlbDsKCi8vIENvbnN0cnVjdG9yCkNhcihzdGQ6OnN0cmluZyBjYXJNYWtlLCBzdGQ6OnN0cmluZyBjYXJNb2RlbCkgOiBtYWtlKGNhck1ha2UpLCBtb2RlbChjYXJNb2RlbCkge30KCi8vIFB1YmxpYyBmdW5jdGlvbgp2b2lkIFN0YXJ0RW5naW5lKCkgewpzdGQ6OmNvdXQgPDwgIlN0YXJ0aW5nIHRoZSAiIDw8IG1ha2UgPDwgIiAiIDw8IG1vZGVsIDw8ICIncyBlbmdpbmUuIiA8PCBzdGQ6OmVuZGw7fQp9OwoKaW50IG1haW4oKSB7Ci8vIENyZWF0ZSBhbiBpbnN0YW5jZSBvZiB0aGUgQ2FyIGNsYXNzCkNhciBteUNhcigiVG95b3RhIiwgIkNhbXJ5Iik7CgovLyBBY2Nlc3MgYW5kIG1vZGlmeSBwdWJsaWMgbWVtYmVycwpteUNhci5tYWtlID0gIkZvcmQiOwpteUNhci5tb2RlbCA9ICJGb2N1cyI7CgovLyBDYWxsIGEgcHVibGljIGZ1bmN0aW9uCm15Q2FyLlN0YXJ0RW5naW5lKCk7CgpyZXR1cm4gMDsKCn0=
Output:
Starting the Ford Focus's engine.
Explanation:
In the above C++ program -
- We define a class named Car that has two public member variables: make and model, both of which are of string type.
- Next, we define a constructor for the Car class that takes two string parameters: carMake and carModel. It initializes the make and model member variables using an initialization list.
- Then we create a public member function named StartEngine(), which uses std::cout to print a message indicating the start of the car's engine, including its make and model.
- Inside the main() function-
- We first create an instance of the Car class named myCar and initialize it with the values Toyota (make) and Camry (model).
- Next, we access the variables using the dot operator in the code and modify the values to Ford (make) and Focus (model).
- Then, we call the StartEngine() function on myCar object, which prints a message to the console indicating the engine start with the updated make and model.
Private Access Specifier In C++
The private access specifier in C++ serves as a keyword to designate class members that can only be accessed within the class where they are defined.
- Members declared as private are hidden from external code and cannot be accessed or modified directly from outside the class. This encapsulation safeguards the class's internal workings and confidential data.
- To understand this, think of a class as a company's internal office space. Private members are like confidential documents stored in a secure, restricted-access room within the office.
- Only employees with the highest clearance levels (i.e., methods within the class) can access and modify these documents. They are hidden from visitors and lower-level employees (i.e., external code).
Syntax Of Private Access Specifier In C++
class MyClass {
private:
// Private members
int privateVar;
};
Here,
- The private keyword indicates that the entities after the colon (:) are only accessible from within the class. Or it forbids members from being accessed from outside the class.
- MyClass and privateVar are the names of the class and the data member inside the class, respectively.
Example Of Private Access Specifiers in C++
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgQmFua0FjY291bnQgewpwcml2YXRlOgpkb3VibGUgYmFsYW5jZTsgLy8gUHJpdmF0ZSBtZW1iZXIKCnB1YmxpYzoKLy8gQ29uc3RydWN0b3IKQmFua0FjY291bnQoZG91YmxlIGluaXRpYWxCYWxhbmNlKSA6IGJhbGFuY2UoaW5pdGlhbEJhbGFuY2UpIHt9CgovLyBQdWJsaWMgbWV0aG9kIHRvIGNoZWNrIGJhbGFuY2UKdm9pZCBDaGVja0JhbGFuY2UoKSB7CnN0ZDo6Y291dCA8PCAiWW91ciBiYWxhbmNlIGlzIElOUiAiIDw8IGJhbGFuY2UgPDwgc3RkOjplbmRsO30KCi8vIFB1YmxpYyBtZXRob2QgdG8gZGVwb3NpdCBtb25leQp2b2lkIERlcG9zaXQoZG91YmxlIGFtb3VudCkgewppZiAoYW1vdW50ID4gMCkgewpiYWxhbmNlICs9IGFtb3VudDsKc3RkOjpjb3V0IDw8ICJEZXBvc2l0ZWQgSU5SICIgPDwgYW1vdW50IDw8ICIgaW50byB5b3VyIGFjY291bnQuIiA8PCBzdGQ6OmVuZGw7fQp9Cn07CgppbnQgbWFpbigpIHsKLy8gQ3JlYXRlIGFuIGluc3RhbmNlIG9mIHRoZSBCYW5rQWNjb3VudCBjbGFzcwpCYW5rQWNjb3VudCBteUFjY291bnQoMTAwMC4wKTsKCi8vIEF0dGVtcHRpbmcgdG8gYWNjZXNzIHRoZSBwcml2YXRlIG1lbWJlciAoY29tcGlsZS10aW1lIGVycm9yKQovLyBteUFjY291bnQuYmFsYW5jZSA9IDIwMDAuMDsKCi8vIEFjY2Vzc2luZyBwdWJsaWMgbWV0aG9kcyB0byBjaGVjayBiYWxhbmNlIGFuZCBkZXBvc2l0IG1vbmV5Cm15QWNjb3VudC5DaGVja0JhbGFuY2UoKTsKbXlBY2NvdW50LkRlcG9zaXQoNTAwLjApOwpteUFjY291bnQuQ2hlY2tCYWxhbmNlKCk7CgpyZXR1cm4gMDsKfQ==
Output:
Your balance is INR 1000
Deposited INR 500 into your account.
Your balance is INR 1500
Explanation:
In the C++ example above,
- We define a class named BankAccount that contains a private member variable balance of type double. This variable stores the balance of the bank account, which is hidden/ private, meaning it cannot be accessed directly from outside the class.
- Next, we define a constructor for the BankAccount class that takes one parameter, initialBalance, which initializes the balance variable using an initialization list.
- We then create a public method CheckBalance() in the BankAccount class. This method outputs the current balance by accessing the private balance variable and displaying it.
- We also define a public method Deposit(double amount) in the BankAccount class. This method allows us to deposit money into the account. It uses if-else statement to check if the amount is greater than zero before adding it to the balance and then outputs a confirmation message.
- Inside the main() function-
- We first create an instance of the BankAccount class named myAccount, initializing it with an initial balance of 1000.0.
- Next, we attempt to access the private member balance directly in a commented-out line, which would result in a compile-time error because balance is private and cannot be accessed directly from outside the class.
- Then we call the CheckBalance() method on myAccount, which displays the current balance, i.e., INR 1000.0.
- We also call the Deposit(500.0) method on myAccount, which adds INR 500.0 to the current balance and outputs a message confirming the deposit.
- After depositing, we call the CheckBalance() method again to verify the new balance, which now shows INR 1500.0.
- Note that here, we can access the private members through the public methods, which are a part of the class.
- Finally, the program terminates successfully by returning 0.
Protected Access Specifier In C++
The protected access specifier in C++ is a keyword used to designate class members that can be accessed within the class where they are defined and within classes that derive from this base class.
- Protected members remain hidden from external code but can be inherited and employed by classes deriving from the base class.
- This allows the controlled sharing of features between a base class and its derived classes, promoting code reuse while maintaining encapsulation.
- Think of a class as a family. In this sense, the protected members are like family secrets or possessions shared among family members (base and derived classes). These secrets are not known to outsiders (external code) but can be inherited and used by family members (derived classes).
Syntax Of Protected Access Specifier In C++
class MyClass {
protected:
// Protected members
int protectedVar;
};
Here,
- The protected keyword indicates that access to the members it contains is restricted to the class itself and its derived classes only.
- The class keyword is used for the creation of a class with the name MyClass.
- protectedVar is the name of the data member (of type integer) that can be accessed and modified within the MyClass class and any classes derived from MyClass.
Example Of Protected Access Specifiers In C++
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgTXlCYXNlQ2xhc3Mgewpwcm90ZWN0ZWQ6CmludCBwcm90ZWN0ZWRWYXI7IC8vIFByb3RlY3RlZCBtZW1iZXIKCnB1YmxpYzoKTXlCYXNlQ2xhc3MoaW50IHZhbHVlKSA6IHByb3RlY3RlZFZhcih2YWx1ZSkge30KCnZvaWQgQWNjZXNzUHJvdGVjdGVkVmFyKCkgewpzdGQ6OmNvdXQgPDwgIlByb3RlY3RlZFZhciBmcm9tIE15QmFzZUNsYXNzOiAiIDw8IHByb3RlY3RlZFZhciA8PCBzdGQ6OmVuZGw7fQp9OwoKY2xhc3MgTXlEZXJpdmVkQ2xhc3M6IHB1YmxpYyBNeUJhc2VDbGFzcyB7CnB1YmxpYzoKTXlEZXJpdmVkQ2xhc3MoaW50IHZhbHVlKSA6IE15QmFzZUNsYXNzKHZhbHVlKSB7fQoKdm9pZCBBY2Nlc3NQcm90ZWN0ZWRWYXJGcm9tRGVyaXZlZCgpIHsKc3RkOjpjb3V0IDw8ICJQcm90ZWN0ZWRWYXIgZnJvbSBNeURlcml2ZWRDbGFzczogIiA8PCBwcm90ZWN0ZWRWYXIgPDwgc3RkOjplbmRsO30KfTsKCmludCBtYWluKCkgewpNeUJhc2VDbGFzcyBiYXNlT2JqZWN0KDQyKTsKTXlEZXJpdmVkQ2xhc3MgZGVyaXZlZE9iamVjdCg3Nyk7CgpiYXNlT2JqZWN0LkFjY2Vzc1Byb3RlY3RlZFZhcigpOyAvLyBBY2Nlc3MgZnJvbSB0aGUgYmFzZSBjbGFzcwpkZXJpdmVkT2JqZWN0LkFjY2Vzc1Byb3RlY3RlZFZhcigpOyAvLyBBY2Nlc3MgZnJvbSB0aGUgZGVyaXZlZCBjbGFzcwpkZXJpdmVkT2JqZWN0LkFjY2Vzc1Byb3RlY3RlZFZhckZyb21EZXJpdmVkKCk7IC8vIEFjY2VzcyBmcm9tIHRoZSBkZXJpdmVkIGNsYXNzCgpyZXR1cm4gMDsKfQ==
Output:
ProtectedVar from MyBaseClass: 42
ProtectedVar from MyBaseClass: 77
ProtectedVar from MyDerivedClass: 77
Explanation:
In this code example-
- We define a class named MyBaseClass that contains a protected member variable protectedVar of integer type. This variable is protected, meaning it can be accessed by derived classes but not outside the class hierarchy.
- Next, we define a constructor for MyBaseClass that takes an integer parameter value. This parameter is used to initialize the protectedVar using an initialization list.
- Then, we create a public method AccessProtectedVar(), which accesses the member variable and prints its value using std::cout.
- We then define a derived class named MyDerivedClass that publicly inherits from MyBaseClass. This means that all public and protected members of MyBaseClass are accessible within MyDerivedClass. Inside-
- We have a constructor, MyDerivedClass, that calls the base class's constructor to initialize protectedVar.
- In MyDerivedClass, we define a public method AccessProtectedVarFromDerived(). This method outputs the value of protectedVar using the access granted by inheritance. The method allows us to see the value of protectedVar from within the derived class.
- Inside the main() function-
- We create an instance of MyBaseClass named baseObject with the initial value of protectedVar set to 42.
- Next, we create an instance of MyDerivedClass named derivedObject with protectedVar initialized to 77.
- Then, we call the AccessProtectedVar() function on baseObject using the dot operator. It accesses and prints the value of protectedVar as initialized in MyBaseClass constructor to the console.
- After that, we again call the AccessProtectedVar() function, but on derivedObject, which correctly accesses and prints the value of protectedVar from the derived class, which is 77.
- Lastly, we call the AccessProtectedVarFromDerived() on the derived class object, derivedObject. It also accesses protectedVar and displays the value 77, but it does so from within the derived class, demonstrating that the derived class can access protected members of its base class.
The Need For Access Specifiers In C++
Access specifiers in C++ serve vital purposes, such as the ones mentioned below.
- Encapsulation: They enable encapsulation, a core principle of object-oriented programming (OOP). This bundles data and methods into classes, controlling what's visible outside, preserving data integrity, and averting unintended interference.
- Data Hiding: Private members shield internal data, enhancing security by restricting access to critical information.
- Code Organization: Access specifiers in C++ categorize class members (public, private, protected), improving code clarity and aiding developers in understanding and working with classes.
- Security: By hiding private members, access specifiers in C++ effectively prevent unauthorized access, modifications and misuse thus bolstering software security.
- API Design: Public members define external interfaces, guiding proper usage and ensuring predictable interactions.
- Inheritance: Access specifiers in C++ regulate which base class members are inherited, upholding the 'base class invariance' principle.
- Code Maintenance: Access specifiers also facilitate evolving code while preserving external interfaces, minimizing disruptions.
- Debugging: Clearly defined specifiers assist in debugging and troubleshooting by distinguishing internal and external components.
In essence, access specifiers are crucial for structured, secure, and maintainable C++ code.
Combined Example For All Access Specifiers In C++
We have already discussed the three access specifiers individually with examples. Now, let's look at an example showcasing the implementation of all specifiers together.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgoKY2xhc3MgQ2FyIHsKcHVibGljOgovLyBQdWJsaWMgc3BlY2lmaWVyIG1lbWJlcnMgYWNjZXNzaWJsZSBmcm9tIGFueXdoZXJlCnN0ZDo6c3RyaW5nIG1ha2U7CnN0ZDo6c3RyaW5nIG1vZGVsOwoKLy8gQ29uc3RydWN0b3IKQ2FyKHN0ZDo6c3RyaW5nIGNhck1ha2UsIHN0ZDo6c3RyaW5nIGNhck1vZGVsKSA6IG1ha2UoY2FyTWFrZSksIG1vZGVsKGNhck1vZGVsKSB7fQoKLy8gUHVibGljIGZ1bmN0aW9uCnZvaWQgU3RhcnRFbmdpbmUoKSB7CnN0ZDo6Y291dCA8PCAiU3RhcnRpbmcgdGhlICIgPDwgbWFrZSA8PCAiICIgPDwgbW9kZWwgPDwgIidzIGVuZ2luZS4iIDw8IHN0ZDo6ZW5kbDsKQ2hlY2tPaWxMZXZlbCgpOyAvLyBBY2Nlc3NpbmcgYSBwcml2YXRlIGZ1bmN0aW9uIGZyb20gYSBwdWJsaWMgb25lLgp9Cgpwcml2YXRlOgovLyBQcml2YXRlIG1lbWJlcnMgYXJlIGhpZGRlbiBmcm9tIG91dHNpZGUgdGhlIGNsYXNzCmludCBmdWVsTGV2ZWw7IC8vIEhpZGRlbiBkYXRhCmJvb2wgZW5naW5lUnVubmluZzsgLy8gSGlkZGVuIGRhdGEKCi8vIFByaXZhdGUgZnVuY3Rpb24Kdm9pZCBDaGVja09pbExldmVsKCkgewpzdGQ6OmNvdXQgPDwgIkNoZWNraW5nIG9pbCBsZXZlbC4uLiIgPDwgc3RkOjplbmRsOwovLyBTaW11bGF0ZSBjaGVja2luZyBvaWwgbGV2ZWwgbG9naWMKfQoKcHJvdGVjdGVkOgovLyBQcm90ZWN0ZWQgbWVtYmVycyBhcmUgYWNjZXNzaWJsZSB3aXRoaW4gZGVyaXZlZCBjbGFzc2VzCmludCBzcGVlZDsgLy8gQWNjZXNzaWJsZSB0byBkZXJpdmVkIGNsYXNzZXMKCi8vIFByb3RlY3RlZCBmdW5jdGlvbgp2b2lkIEFjY2VsZXJhdGUoKSB7CnN0ZDo6Y291dCA8PCAiQWNjZWxlcmF0aW5nLi4uIiA8PCBzdGQ6OmVuZGw7CnNwZWVkICs9IDEwOwp9Cn07CgpjbGFzcyBTcG9ydHNDYXIgOiBwdWJsaWMgQ2FyIHsKcHVibGljOgpTcG9ydHNDYXIoc3RkOjpzdHJpbmcgY2FyTWFrZSwgc3RkOjpzdHJpbmcgY2FyTW9kZWwpIDogQ2FyKGNhck1ha2UsIGNhck1vZGVsKSB7fQoKLy8gUHVibGljIGZ1bmN0aW9uIGluIHRoZSBkZXJpdmVkIGNsYXNzCnZvaWQgU3BlZWRVcCgpIHsKQWNjZWxlcmF0ZSgpOyAvLyBBY2Nlc3NpbmcgYSBwcm90ZWN0ZWQgZnVuY3Rpb24gZnJvbSBhIGRlcml2ZWQgY2xhc3MKc3RkOjpjb3V0IDw8ICJTcGVlZGluZyB1cCB0aGUgIiA8PCBtYWtlIDw8ICIgIiA8PCBtb2RlbCA8PCAiIHRvICIgPDwgc3BlZWQgPDwgIiBtcGguIiA8PCBzdGQ6OmVuZGw7Cn0KfTsKCmludCBtYWluKCkgewpDYXIgbXlDYXIoIlRveW90YSIsICJDYW1yeSIpOwpteUNhci5tYWtlID0gIkZvcmQiOyAvLyBBY2Nlc3NpbmcgcHVibGljIG1lbWJlcnMKbXlDYXIubW9kZWwgPSAiRm9jdXMiOwpteUNhci5TdGFydEVuZ2luZSgpOwovLyBteUNhci5DaGVja09pbExldmVsKCk7IC8vIEVycm9yOiBDYW5ub3QgYWNjZXNzIHByaXZhdGUgbWVtYmVyCgpTcG9ydHNDYXIgbXlTcG9ydHNDYXIoIkZlcnJhcmkiLCAiNDg4IEdUQiIpOwpteVNwb3J0c0Nhci5TcGVlZFVwKCk7IC8vIEFjY2Vzc2luZyBwcm90ZWN0ZWQgZnVuY3Rpb24gdGhyb3VnaCB0aGUgZGVyaXZlZCBjbGFzcwoKcmV0dXJuIDA7Cn0=
Output:
Starting the Ford Focus's engine.
Checking oil level...
Accelerating...
Speeding up the Ferrari 488 GTB to 137183658 mph.
Explanation:
- We begin the code by including the iostream header file and then create a class called Car. The Car class contains public, private, and protected members.
- The public specifier in the Car class contains-
- Two public data members/ variables make and model of string data type.
- A public constructor that initializes the variables when an object of the Car class is created.
- A StartEngine() function which uses the std::cout to print a message and calls a private function in code, CheckOilLevel().
- With the private specifier of the class-
- We declare two data members, fuelLevel (int type) and engineRunning (bool type), that are hidden from external access.
- A private function CheckOilLevel(), which prints a statement using std::cout.
- Finally, using the protected specifier in the class-
- We declare an integer type variable/ data member called speed.
- And define an Accelerate() function, which prints a phrase and updates the speed variable's value by 10.
- Both of these can be used within derived classes.
- Next, we create a derived class called SportsCar, which inherits properties from Car with the public specifier. Inside the derived class-
- We declare two data members carMake and carModel, inherited from the base class.
- Then, we define a SpeedUp() function that calls the protected Accelerate function and prints a phrase using std::cout.
- Inside the main() function-
- We create an instance of the Car class called myCar, with initial values for data members to Toyota and Camry.
- Then, we access the public data members using the dot operator and modify their values to Ford and Focus, respectively.
- Next, we call the StartEngine() function using the myCar object and dot operator.
- Note that, as mentioned in the code comments, an attempt to call the CheckOilLevel() function would result in an error since it is restricted by private access.
- After that, we create an instance of the SportsCar class, called mySportsCar, and call the SpeedUp() function on it.
- The program prints messages for various actions and terminates successfully.
Best Practices For Using Access Specifiers In C++
To effectively use access specifiers in your C++ code, consider the following best practices:
-
Keep Data Members Private: Data members should generally be declared private or protected to maintain data integrity. One should only expose data through controlled public member functions. For example-
class Employee {
private:
std::string name; // Private data member
int age;
};
-
Use Public Member Functions: Provide well-defined public member functions that allow external code to interact with the class. These functions should encapsulate the necessary operations on the class's data. For example-
class Calculator {
public:
void Add(int value); // Public interface method
};
-
Minimize Use Of Protected: While protected members can be helpful in some cases, it's generally a good practice to minimize their use and prefer private members when possible.
-
Document Your Classes: Clearly document the public interface of your classes, including the purpose and usage of each public member function and the format of data that should be passed. This makes your code easier to understand, maintain, and use correctly by others (or by yourself in the future). For example-
// Class to represent a simple bank account
class BankAccount {
public:
// Deposits a specified amount into the account
void Deposit(double amount);// Retrieves the current account balance
double GetBalance() const;
};
-
Follow The Principle Of Least Privilege: Only expose what is necessary. Limit the visibility of members to the smallest scope required for their functionality. This reduces the risk of unintended interactions and simplifies the code. For example-
class Logger {
private:
void WriteLog(const std::string& message); // Private helper methodpublic:
void LogInfo(const std::string& message); // Public method for logging
};
-
Avoid Global Data: Minimize the use of global data, as it goes against the principles of encapsulation and can lead to code that is difficult to maintain. Instead, encapsulate data within classes and provide controlled access through member functions.
Why Can't Private Members Be Accessed From Outside A Class?
In C++, private variables cannot be accessed directly from outside the class they are declared in, and this is by design. The concept of access control in C++ is an important part of the language's encapsulation and data-hiding features. We know that in the Derived class, private members are not accessible, but public and protected members are accessible. And only public members are accessible through an object.
To better understand why this limitation happens, let's dive into some real-life examples explaining concepts of public, protected, and private access specifiers in object-oriented programming (OOP).
Example: A Bank Account
Imagine you're designing a class for a bank account in an OOP context.
Public Access Specifier: You would define the account balance as a public member, allowing users of your class to easily check their balance or deposit money. This is like the public interface of the bank account.
class BankAccount {
public:
double balance; // Public member for balance
};
In this case, anyone can access the balance directly:
BankAccount myAccount;
myAccount.balance = 1000.0; // Direct access to balance
The idea is to provide convenient and open access to information that should be readily available to users.
Private Access Specifier: Now, consider the PIN associated with the bank account. It's something sensitive that should not be directly accessible or modifiable by external code. You'd define it as a private member, hidden from external access.
class BankAccount {
private:
double balance; // Private member for balance
int pin; // Private member for PIN
};
With this setup, external code cannot directly access or modify the PIN.
BankAccount myAccount;
// myAccount.pin = 1234; // Compile-time error - PIN is private
The idea is to protect sensitive data and ensure it can only be accessed or modified through controlled methods.
Protected Access Specifier: Now, consider a scenario where you want to create specialized account types like SavingsAccount or CheckingAccount that inherit from the base BankAccount class. These specialized accounts need access to some of the base class's members, like the balance, but they shouldn't have access to the PIN. This is where the protected access specifier comes in.
class BankAccount {
private:
double balance; // Private member for balance
int pin; // Private member for PINprotected:
// Protected member for overdraft protection
double overdraftLimit;
};
Now, specialized accounts can access the balance and overdraftLimit but not the PIN. As seen in the example below.
class CheckingAccount : public BankAccount {
public:
void CheckBalance() {
// Accessing balance and overdraftLimit (protected)
double currentBalance = balance;
double overdraft = overdraftLimit;
// Cannot access the private member pin
// int myPin = pin; // Compile-time error
}
};
The idea behind protected access is to allow derived classes to access certain members for inheritance while still maintaining privacy and encapsulation.
In summary, the public, private, and protected access specifiers in OOP help you control the visibility and accessibility of class members, just like how a bank exposes your balance publicly, keeps your PIN private, and allows limited access (overdraft protection) for specialized account types. These rules promote data security, encapsulation, and maintainable code.
Conclusion
Access specifiers in C++ and programming are tools that let developers control how information in classes can be used. They keep data safe and make sure that the code is organized and information is being used correctly.
- There are three types of specifiers, namely, public, private, and protected. They determine which parts of a class can be seen by outside code.
- Each member type (like a variable or function) has a specific job. That is, public members act as a gateway for outside code, private members keep sensitive information hidden, and protected members allow controlled sharing within class groups.
- Together, they help keep code secure, predictable, and simple to handle.
Every developer needs to understand access specifiers in C++/ programming to write high-quality code. This is because it improves code maintainability and helps to lessen unwelcome intervention.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. When should I use private over protected or public?
Use private when you want to restrict access to class members so that they can only be accessed within the class itself. This is ideal for internal implementation details that should not be exposed or modified from outside the class.
Q. What is the default access specifier in C++?
In C++, unless specified otherwise while declaring members within a class, the members are by default considered private. That is, the default access specifier in C++ is private. Even though public and private aren't the same in C++, a member will always be considered private by default unless told otherwise. To call a member public, one must specify it with the correct access specifier.
Q. What is a protected class in C++?
The protected class in C++ differs from those in programming languages like Java and Python. A protected class refers to one of the three access modifiers/ specifiers, namely Public, Protected, and Private. The primary purpose of a class being declared as protected is that protected class members cannot be accessed by members from outside the class. However, members from the inherited class can access the members of a protected class.
Q. What is a friend class function in C++?
A friend function in C++ can be thought of as a privileged member who, despite not being a member of a class, has access to its members. It emphasizes mutual trust between functions and is especially useful when dealing with operation overloading. While it is helpful in specific cases and can access multiple classes, it should be used sparingly and only when required.
Q. How does protected differ from private in terms of inheritance?
Protected members can be accessed by derived classes, whereas private members cannot. This makes protected useful for creating a base class that shares certain implementation details with its derived classes without exposing them to the outside world.
Q. Can derived classes access private members of a base class?
No, derived classes cannot directly access private members of a base child class. However, derived classes can access protected members, which allows for controlled inheritance while maintaining encapsulation.
You might also be interested in reading the following:
- Pointer To Object In C++ | Simplified Explanation & Examples!
- Pure Virtual Function In C++ & Abstract Classes ( With Examples)
- Dynamic Memory Allocation In C++ Explained In Detail (With Examples)
- Function Prototype In C++ | Definition, Purpose & More (+Examples)
- C++ Type Conversion & Type Casting Demystified (With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment