Pure Virtual Function In C++ & Abstract Classes ( With Examples)
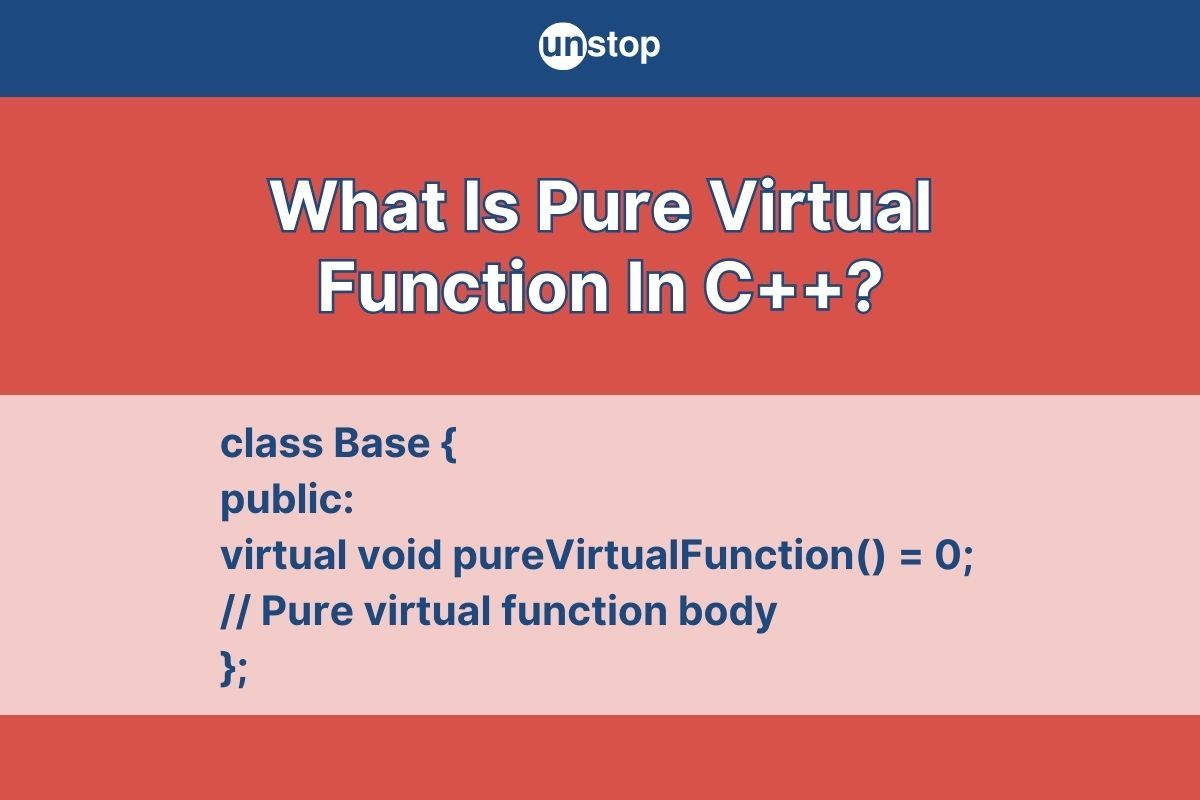
Table of content:
- What Is A Virtual Function In C++?
- Concept Of Pure Virtual Function In C++
- Characteristics Of Pure Virtual Function in C++
- Abstract Class In C++
- Polymorphism, Late Binding & Virtual Functions
- Points To Remember About Pure Virtual Function in C++
- Advantages Of Pure Virtual Function In C++
- Disadvantages of Pure Virtual Functions in C++
- Similarities Between Virtual And Pure Virtual Function In C++
- Difference Between Virtual And Pure Virtual Function In C++
- Conclusion
- Frequently Asked Questions
C++ is a versatile and powerful programming language known for its support of object-oriented programming (OOP) principles. One of the key features that make OOP in C++ so robust is the concept of pure virtual functions. A pure virtual function in C++ provides a mechanism for creating abstract classes and enabling polymorphism, which plays a pivotal role in designing flexible and extensible software. In this article, we will delve into the world of pure virtual functions and abstract classes in C++, exploring what they are, how they work, and why they are essential in object-oriented programming. We'll also examine practical examples to illustrate their usage and benefits and understand how they differ from virtual functions.
What Is A Virtual Function In C++?
In C++, a virtual function is a member function declared within a base class and is marked with the virtual keyword. These functions enable the implementation of a fundamental concept in object-oriented programming (OOP) known as polymorphism. Polymorphism allows objects of different classes to be treated as objects of a common base class, providing a high level of abstraction and flexibility in software design.
Let's use a real-life analogy to explain virtual functions in C++ programming. Consider a scenario involving various electronic devices, such as smartphones, tablets, and laptops, and a common interface for handling power management.
Imagine you are designing software to manage these devices, and you want to create a hierarchy of classes to represent them. Each device has a different way of powering on and off, but they all share some common behaviors, like battery status checks, etc. This situation is analogous to the need for polymorphism and virtual functions. The code given below shows how you can model this scenario in C++.
Code:
Output:
Smartphone is booting up.
Smartphone battery status: 70%
Smartphone is shutting down.
Tablet is starting.
Tablet battery status: 50%
Tablet is powering off.
Explanation:
The C++ program above defines a hierarchy of classes to represent electronic devices, including a base class called ElectronicDevice and two derived classes, Smartphone and Tablet.
-
In the base class, we have three virtual member functions, i.e., powerOn(), powerOff(), and checkBatteryStatus(). These base class functions are defined using the virtual keyword and are overridden by the derived classes with their custom implementations.
- All the functions and their overriding implementations print a message to the console using std::cout commands.
-
In the main() function, we create two pointers to the object of base class ElectronicDevice, called myDevice1 and myDevice2.
-
These base class pointers are used to demonstrate polymorphism, as they can reference objects of derived classes (Smartphone and Tablet). Here, myDevice1 is assigned to a Smartphone object, and myDevice2 is assigned to a Tablet object, using the new keyword.
-
We then call the virtual functions to manage the power states and check the battery status of the electronic devices using the 'this' pointer.
-
When these functions are called through the pointers myDevice1 and myDevice2, the program dynamically determines which version of the function to execute based on the actual type of the object being referenced.
-
For myDevice1, calling powerOn() results in the execution of the Smartphone class's powerOn() function, and the same applies to checkBatteryStatus() and powerOff().
-
The same dynamic binding occurs for myDevice2 but with the Tablet class's functions.
-
-
The program then uses the delete keyword to clean up and delete the dynamically allocated objects pointed to by myDevice1 and myDevice2 to free memory.
The output of the program demonstrates that the correct implementations of the functions are called based on the actual types of the devices. For example, 'Smartphone is booting up.' is printed when powerOn() is called for myDevice1, and 'Tablet battery status: 50%' is printed when checkBatteryStatus() is called for myDevice2. This code illustrates how virtual functions enable polymorphism and dynamic binding in C++, allowing a common interface to be used for different derived classes with distinct behaviors.
Concept Of Pure Virtual Function In C++
In C++, a pure virtual function (or an abstract function) is a special type of virtual function that is declared in a base class but does not have a definition (i.e., it has no implementation) in the base class. Instead, it is intended to be overridden and implemented by derived classes. A pure virtual function in C++ is used to define an interface or an abstract base class, which cannot be instantiated on its own but can be used as a blueprint for creating concrete derived classes.
Pure Virtual Function In C++ Declaration
Given below is the syntax of how to declare/ define a pure virtual function in C++:
class Base {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function body
};
Here,
- Base is the name of the class which contains the function.
- The virtual keyword indicates that the function with the name pureVirtualFunction is virtual.
- The equal to zero (= 0) in the function definition indicates that it is a pure virtual function. This is what primarily differentiates a virtual function from a pure virtual function. This makes the class an abstract base class, and you cannot create objects of this class directly.
Here's a code example that demonstrates the use of pure virtual functions in C++. We'll create a simple hierarchy of shapes, including a base class Shape with pure virtual functions for calculating area and perimeter. We'll also provide implementations for these functions in derived classes Circle and Rectangle. Finally, we'll demonstrate how polymorphism allows us to use these shapes interchangeably in a collection.
Pure Virtual Function In C++ Example
Output:
Area: 78.5
Perimeter: 31.4
------------------------
Area: 24
Perimeter: 20
------------------------
Explanation:
The code demonstrates the concept of pure virtual functions in C++, which allows us to create abstract classes and achieve polymorphism.
- We first create an abstract base class named Shape, with two pure virtual functions, i.e., area() and perimeter(). This class defines an interface for calculating the area and perimeter of shapes but does not provide implementations.
-
Then, we create two derived classes, Circle and Rectangle, that inherit from the Shape class. They override the pure virtual functions and provide their own implementations for calculating the area and perimeter specific to their shapes.
- The Circle class also contains of a private data member radius (of data type double) and a constructor. The Rectangle class also contains two private data members, width and height.
-
In the main() function-
- We create two instances of Circle and Rectangle classes, called circle and rectangle, respectively. These objects are initialized with specific dimensions (e.g., radius and width/height).
- Then, we create a vector (using std::vector) named shapes to store pointers to Shape objects. This allows us to store objects of different shapes in the same collection due to polymorphism.
- Using the push_back() function, we push the circle and rectangle objects into the vector by using object reference.
- Next, we initiate a for loop to iterate over each element in the shapes vector, treating them as Shape pointers.
- Inside the loop, we call the area() and perimeter() functions, using the arrow operator, on each shape.
- The output demonstrates that we can calculate the area and perimeter of different shapes (e.g., a circle and a rectangle) using the same code structure. The code adapts dynamically based on the type of shape being processed.
Characteristics Of Pure Virtual Function in C++
Pure virtual functions in C++ possess several distinctive characteristics that set them apart from regular virtual functions. These characteristics make them a powerful tool for enforcing a common interface across a hierarchy of classes while allowing derived classes to provide their own implementations. Here are the key characteristics of pure virtual functions:
-
No Implementation in the Base Class: A pure virtual function in C++ is declared in the base class using the virtual keyword followed by equal to zero (=0). This notation indicates that no implementation is provided in the base class.
-
Forcing Derivation: Classes containing one or more pure virtual functions are often referred to as abstract classes. Abstract classes cannot be instantiated directly, as they serve as blueprints for derived classes.
Any attempt to create an instance of an abstract class results in a compilation error. This enforces the rule that derived classes must provide implementations for the pure virtual functions.
-
Common Interface: A pure virtual function in C++ defines a common interface that all derived classes must adhere to. This ensures that specific methods are implemented consistently across the hierarchy of classes.
-
Dynamic Binding: Like regular virtual functions, pure virtual functions enable dynamic binding or late binding. This means that when a derived class object is accessed through a pointer or reference to the base class, the correct implementation of the pure virtual function is determined at runtime based on the actual type of the object.
-
Customized Behavior in Derived Classes: Each derived class must provide its own implementation for a pure virtual function in C++. This allows derived classes to customize the behavior of the function according to their specific requirements.
-
Incomplete Base Class: A class that contains one or more pure virtual functions is considered incomplete because it lacks complete implementations for all of its member functions. Consequently, an incomplete base class cannot be used to create objects directly.
-
Interface Contracts: Pure virtual functions define an implicit contract between the base class and its derived classes. The contract specifies that any class inheriting from the base class must provide concrete definitions for all pure virtual functions, thus ensuring that the derived classes fulfill the interface requirements.
-
Runtime Error Prevention: The use of pure virtual functions helps prevent runtime errors that might occur if a derived class fails to provide implementations for essential methods. This is especially important in large codebases where maintaining consistency and correctness is crucial.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Abstract Class In C++
An abstract class in C++ is a class that cannot be instantiated directly and is often used as a base class for other classes. Abstract classes are designed to provide a common interface or contract for derived classes while leaving the implementation details to those subclasses. They contain pure virtual functions, which are virtual functions with no implementation in the base class. The presence of pure virtual functions in an abstract class ensures that a derived class provides an implementation for those functions.
Syntax:
Here's the syntax for declaring an abstract class in C++:
class AbstractClass {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
// Other members and methods (can be both virtual and non-virtual)
};
- AbstractClass is the name of the abstract class.
- pureVirtualFunction() is a pure virtual function, which means it has no implementation in the base class.
Code:
Output:
Derived class implementation of pureVirtualFunction
Concrete function in Derived class
Derived class implementation of pureVirtualFunction
Concrete function in AbstractClass
Explanation:
In the code above,
- We begin by including the iostream header to enable input and output operations.
- Then, we define an abstract base class called AbstractClass. Abstract classes are classes that cannot be instantiated (objects of these classes cannot be created) and are used to provide a common interface for derived classes.
- Inside the AbstractClass class, we declare a pure virtual function, pureVirtualFunction(). A pure virtual function is declared with "= 0" and must be implemented by any derived class that inherits from this abstract class.
- The AbstractClass also contains a non-virtual function called concreteFunction(), which has a default implementation. This function can be inherited by derived classes but is not mandatory to override.
- Next, we define a derived class named Derived, which publicly inherits from AbstractClass. This means that Derived inherits both the pure virtual function and the concrete function from AbstractClass.
- The Derived class provides its own implementation of the pureVirtualFunction() and concreteFunction().
- Here, the pureVirtualFunction is overridden with its own implementation. And since concreteFunction is not marked as virtual but is just a regular member function. This means that it hides the concreteFunction() function of the base class but does not override it.
-
In the main() function, we-
- Create an object of the abstract class (AbstractClass obj;), but this results in a compilation error because abstract classes cannot be instantiated directly.
- We then create an object d of the derived class. Using this object, we call the pureVirtualFunction(), which invokes the overridden pureVirtualFunction() in the Derived class.
- Similarly, when we call the concreteFunction() using object d, it invokes the non-virtual concreteFunction() from the Derived class.
- Then, we create a pointer to AbstractClass, ptr, and initialize it with the address of the Derived object d, using reference.
- Next, we use the arrow operator with the ptr to call the virtual function (i.e., ptr->pureVirtualFunction()). This invokes the overridden pureVirtualFunction() in the Derived class. Thus, demonstrates polymorphism, as the function called is determined at runtime based on the actual object type.
- We also call the concreteFunction() using ptr, which invokes the non-virtual concreteFunction() from the AbstractClass. This function is not polymorphic, and the function called is determined at compile-time based on the pointer type.
Polymorphism, Late Binding & Virtual Functions
One of the primary motivations for using a pure virtual function in C++ is to enable polymorphism. Polymorphism allows different objects to respond differently to the same function call based on their actual types. This feature is crucial for creating flexible and reusable code.
In C++, polymorphism is achieved through virtual functions, including pure virtual functions. When a base class declares a virtual function, and a derived class provides its implementation, the actual function to be called is determined at runtime. This is known as late binding or dynamic binding, and it ensures that the correct function is invoked, depending on the object's type.
Points To Remember About Pure Virtual Function in C++
We already know what a pure virtual function is, how to use it, and the reasons for its use, etc. In this section, we will look at a few important points that anyone working with pure virtual functions must remember.
1. Removal of Pure Virtual Function in C++
Once a pure virtual function is declared in a base class, it cannot be removed or undefined in any derived class. Attempting to do so will result in a compilation error. The purpose of pure virtual functions is to define a contract that derived classes must adhere to. Removing or altering the contract in derived classes would violate the principles of object-oriented design.
Code:
Output:
Compilation Error
Explanation:
- The Base class defines a pure virtual function pureVirtualFunction() using = 0.
- In the Derived class, we attempt to remove the pure virtual function by providing an empty implementation. This is not allowed.
- The error message generated during compilation will indicate that the class Derived is still abstract due to the presence of pure virtual functions in its base class.
2. Creating Objects of Base Class (Pure Virtual Function case):
You cannot create objects of a class that contains pure virtual functions. Attempting to do so will result in a compilation error. Since pure virtual functions do not provide complete implementations, objects of the base class are considered incomplete. Consequently, they cannot be instantiated directly.
Code:
Output:
Compilation Error
Explanation:
- In this code, we attempt to create an object of the Base class, which contains a pure virtual function.
- Since pure virtual functions do not provide complete implementations, objects of the base class are considered incomplete and cannot be instantiated directly.
- The error message indicates that objects of abstract classes cannot be created
3. Overriding Pure Virtual Function of Base Class:
Derived classes must override pure virtual functions from the base class to provide their own implementations. Failure to do so will also result in a compilation error. Pure virtual functions define an interface that derived classes must adhere to. Overriding these functions ensures that each derived class provides a concrete implementation, fulfilling the interface contract.
Code:
Output:
Derived class implementation
Explanation:
- The Base class defines a pure virtual function pureVirtualFunction() with no implementation.
- The Derived class inherits from Base and overrides the pure virtual function with its own implementation.
- In the main function, we create an object of the Derived class and call pureVirtualFunction(), which invokes the overridden function in the Derived class.
4. Base Class Constructor:
A base class with pure virtual functions can indeed have its constructor. The constructor of the base class is executed when objects of derived classes are created. The base class constructor can perform necessary initialization tasks common to all derived classes. It does not violate the concept of pure virtual functions because constructors are not part of the interface contract.
Code:
Output:
Base class constructor
Derived class implementation
Explanation:
- In this code, the Base class contains a constructor, and it prints a message when objects of the Base class are constructed.
- The Derived class inherits from Base and overrides the pure virtual function with its implementation.
- When we create an object of the Derived class, both the constructor of the Base class and the overridden function in the Derived class are executed.
5. Illegal Definition of Pure Virtual Function:
Attempting to provide a definition (implementation) for a pure virtual function within the base class is not allowed and will result in a compilation error. Also, providing an implementation in the base class would be contradictory to their purpose, which is to leave the implementation details to derived classes.
Code:
Output:
Compilation Error
Explanation:
- The Base class defines a pure virtual function pureVirtualFunction() using = 0.
- In this code, we attempt to define an implementation for the pure virtual function within the Base class, which is not allowed.
- The error message generated during compilation indicates that it is illegal to provide an implementation for a pure virtual function in the base class.
Advantages Of Pure Virtual Function In C++
Pure virtual functions in C++ offer several advantages that make them a valuable tool in object-oriented programming. These advantages contribute to cleaner, more maintainable, and flexible code designs. Here are the key advantages of using pure virtual function in C++:
-
Common Interface: They enforce a common interface that derived classes must implement, ensuring consistency.
-
Polymorphism: A pure virtual function in C++ enables dynamic binding for runtime flexibility and polymorphic behavior.
-
Customization: They allow each derived class to provide its unique implementation.
-
Prevent Instantiation: Pure virtual functions ensure abstract classes (with pure virtual functions) can't be instantiated directly, reducing errors.
-
Modularity: A pure virtual function in C++ helps create modular and extensible code by separating interface from implementation.
-
Improved Documentation: They clearly indicate which functions should be overridden, enhancing code readability.
-
Runtime Error Prevention: Catch missing implementations at compile time, reducing runtime errors.
-
Design Patterns: Support design patterns like Template Method and Factory Method for flexible object creation and behavior.
Disadvantages of Pure Virtual Functions in C++
While pure virtual functions offer several advantages in C++, they also come with certain disadvantages and considerations. They are:
-
Complexity: Pure virtual functions can add complexity to the codebase. Developers need to manage and maintain the hierarchy of derived classes carefully, ensuring that each class provides the required implementations.
-
Inflexibility: Once a pure virtual function in C++ program is declared in a base class, it becomes part of the class's interface. Changing or removing it can be challenging without potentially breaking existing derived classes.
-
Limited Default Behavior: Unlike regular virtual functions, a pure virtual function in C++ cannot provide default implementations in the base class. This means that every derived class must provide its implementation, even if the behavior is the same across most subclasses, potentially leading to code duplication.
-
Runtime Overhead: The dynamic binding mechanism used for virtual functions, including pure virtual functions, can introduce a slight runtime overhead due to the need to determine the correct function to call at runtime. While this overhead is generally minimal, it may be a concern in performance-critical applications.
-
Compile-Time Dependency: The requirement for derived classes to implement a pure virtual function in C++ introduces compile-time dependencies. Any changes to the base class's interface may necessitate updates in all derived classes, potentially leading to a cascade of changes throughout the codebase.
-
Learning Curve: For developers new to C++ or object-oriented programming, the concept of pure virtual functions and abstract classes may introduce additional complexity and lead to a steeper learning curve.
-
Limited to Class Hierarchies: Pure virtual functions are most useful in class hierarchies where multiple classes share a common interface. In situations where polymorphism or a shared interface is not necessary, they may be unnecessary and add complexity.
-
Potential Design Pitfalls: Overusing pure virtual functions inappropriately can lead to overly complex class hierarchies and designs that are harder to understand and maintain.
Similarities Between Virtual And Pure Virtual Function In C++
Virtual functions and pure virtual functions in C++ share several similarities, as they are both integral to object-oriented programming and polymorphism. Here are the key similarities between the two:
-
Declared in Base Class: Both virtual functions and pure virtual functions are declared in the base class. This allows for the creation of a common interface or contract that derived classes must adhere to.
-
Use of the virtual Keyword: Both types of functions use the virtual keyword in their declarations within the base class. This keyword signifies that these functions support dynamic binding and can be overridden by derived classes.
-
Dynamic Binding: Virtual functions and pure virtual functions both support dynamic binding (also known as late binding). This means that the decision about which function implementation to execute is determined at runtime based on the actual type of the object being referenced.
-
Overriding Capability: In both cases, derived classes can override these functions to provide their own implementations. This allows for the customization of behavior in derived classes while adhering to the common interface defined in the base class.
-
Polymorphism: Both virtual functions and pure virtual functions are essential for achieving Run-time polymorphism, which enables objects of different classes to be treated as objects of a common base class. This is a fundamental concept in object-oriented programming.
-
Interface Enforcement: Both types of functions contribute to enforcing a common interface or contract among derived classes. This ensures that specific virtual methods are implemented consistently across the class hierarchy.
Difference Between Virtual And Pure Virtual Function In C++
Here's a table highlighting the differences between virtual and pure virtual functions in C++:
Characteristic | Virtual Function | Pure Virtual Function |
---|---|---|
Provides Default Implementation | Yes (optional) | No (required) |
Allows Direct Instantiation | Yes (unless all functions are purely virtual) | No (always prevents direct instantiation) |
Abstract Class | No (unless all functions are purely virtual) | Yes (always creates an abstract class) |
Enforces a Common Interface | Yes (if used consistently) | Yes (mandates a common interface) |
Customization in Derived Classes | Yes | Yes (requires customization) |
Must Be Overridden in Derived Classes | No (optional to override) | Yes (mandatory to provide implementation) |
The key differences between virtual functions and pure virtual functions lie in whether they provide default implementations, whether direct instantiation is allowed, and whether they create abstract classes.
Virtual functions may have default implementations, do not prevent direct instantiation, and do not always create abstract classes. In contrast, pure virtual functions do not provide default implementations, always prevent direct instantiation, and always create abstract classes. Pure virtual functions also mandate that derived classes provide their implementations.
Conclusion
Pure virtual functions in C++ are a powerful mechanism for creating abstract classes and enabling polymorphism. They allow you to define an interface that derived classes must follow while ensuring that objects of the abstract class cannot be instantiated. This promotes clean code design, encapsulation, and code reuse.
By leveraging pure virtual functions, you can create flexible class hierarchies and design software systems that are easy to maintain and extend. Their ability to facilitate late binding and dynamic dispatch makes them a fundamental feature of C++ for achieving polymorphism, a cornerstone of object-oriented programming. As you continue to explore C++ and its object-oriented capabilities, embracing pure virtual functions will undoubtedly be an essential part of your toolkit for designing elegant and efficient software solutions.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. Can a pure virtual function be called using an object?
No, you cannot directly call a pure virtual function using an object of an abstract class that declares the pure virtual function. The primary purpose of a pure virtual function is to serve as a placeholder for functions that must be implemented in derived classes. Since an abstract class cannot be instantiated, you cannot create an object of the abstract class and directly call its pure virtual functions.
If you attempt to create a direct object of an abstract class or call a pure virtual function on such an object, you will encounter a compilation error. The compiler will prevent you from doing so to enforce the rule that pure virtual functions must be overridden in derived classes before they can be called.
Here's an example:
class Base {
public:
virtual void pureVirtualFunction() = 0; // Pure virtual function
};int main() {
// Attempting to create an object of an abstract class type will result in a compilation error.
// Base obj; // Error: cannot declare variable 'obj' to be of abstract type 'Base'// Attempting to call a pure virtual function on an object will also result in an error.
// Base* ptr = new Base(); // Error: cannot allocate an object of abstract type 'Base'
// ptr->pureVirtualFunction();return 0;
}
Explanation:
In the code snippet above, attempting to create an object of the Base class or call the pureVirtualFunction() function on an object of type Base results in compilation errors, as mentioned in code comments. Instead, you should create objects of concrete derived classes that override the pure virtual function.
Q. Why pure virtual function is necessary in C++?
Pure virtual functions are necessary for several reasons. Listed below are a few uses of pure virtual function in C++, that make it an integral part of programming.
-
Abstraction and Interface Definition: Pure virtual functions allow you to define an abstract base class that serves as an interface or contract for derived classes. This abstraction enforces a common interface that derived classes must adhere to, ensuring that they provide specific functionality.
-
Polymorphism: They are a fundamental component of polymorphism in C++. They enable dynamic binding or late binding, where the actual function to be called is determined at runtime based on the type of object. This allows you to write more flexible and extensible code.
-
Code Organization: It helps organize code by separating the interface (defined in the abstract base class) from the implementation (provided by derived classes). This promotes a cleaner and more modular design, making it easier to maintain and extend the codebase.
- Creating Abstract Base Classes: A pure virtual function in C++ makes it possible to create abstract base classes, which cannot be instantiated on their own but can be used as a blueprint for creating concrete-derived classes. Abstract base classes are essential for defining common behavior and establishing a hierarchy of related classes.
-
Enforcing Derived Class Implementations: By declaring functions as pure virtual in the base class, you ensure that all derived classes must provide an implementation for those functions. This helps avoid incomplete or incorrect implementations in derived classes.
-
Frameworks and APIs: In the context of software frameworks and APIs, a pure virtual function in C++ enables framework designers to define interfaces that users of the framework must implement. This allows developers to extend and customize the behavior of the framework to suit their specific needs.
Q. What is the size of a virtual function in C++?
In C++, the size of a virtual function itself is typically implementation-dependent and not explicitly defined by the C++ language standard. The size of a virtual function pointer, which is used to manage virtual function calls, is what contributes to the overall size of a class.
The size of a virtual function pointer is usually the same as the size of a regular function pointer on the platform you are using. On many modern systems, this size is typically 4 bytes (32 bits) or 8 bytes (64 bits), depending on whether you are working with a 32-bit or 64-bit architecture.
Q. Can a virtual function be declared as a static function?
No, in C++, a virtual function cannot be declared as a static function. Virtual functions and static functions serve different purposes and have different behaviors, and they are not meant to be used together.
Here are the key differences between virtual functions and static functions:
Virtual Function:
- Virtual functions are used in the context of inheritance and polymorphism.
- They are declared in a base class and can be overridden in derived classes.
- The function to be called is determined at runtime based on the actual type of the object.
- Virtual functions are non-static and are associated with instances of classes (objects).
Syntax:
class Base {
public:
virtual void myFunction() {
// Virtual function implementation
}
};
Static Function:
- Static functions are not associated with class instances; they are class-level functions.
- They are declared using the static keyword and can be called using the class name without creating an object.
- Static functions do not participate in inheritance or polymorphism and are not overridden in derived classes.
Syntax:
class MyClass {
public:
static void myStaticFunction() {
// Static function implementation
}
};
Attempting to declare a virtual function as static or vice versa will result in a compilation error because these concepts are fundamentally different and cannot be combined. If you want to use a function in a class hierarchy with inheritance and polymorphism, you should declare it as a virtual function. If you want a function to be associated with a class itself (rather than instances of the class) and not participate in inheritance, you can declare it as a static function.
Q. Is a pure virtual function the same as an abstract class?
A pure virtual function in C++ and an abstract class are closely related concepts, but they are not exactly the same. They are often used together to achieve the goal of creating abstract classes, but there are some differences between the two, as mentioned in the table below.
Pure Virtual Function | Abstract Class |
|
|
So, while a pure virtual function in C++ is used to define an abstract class, an abstract class may contain more than just pure virtual functions. It may also have regular functions with implementations that can be inherited by derived classes.
Here are a few more fun and comprehensive reads for you:
- C++ Type Conversion & Type Casting Demystified (With Examples)
- Function Overloading In C++ With Code Examples & Explanation
- Destructor In C++ | Understanding The Key To Cleanups (+ Examples)
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- Classification Of Embedded Systems In 5 Ways (With Applications)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment