Table of content:
- What Is Hierarchical Inheritance In C++?
- Example 1: Hierarchical Inheritance In C++
- Example 2: Hierarchical Inheritance In C++
- Impact of Visibility Modes In Hierarchical Inheritance In C++
- Advantages And Disadvantages Of Hierarchical Inheritance In C++
- Use Cases Of Hierarchical Inheritance In C++
- Conclusion
- Frequently Asked Questions
Hierarchical Inheritance In C++ Explained With Real-Life Examples
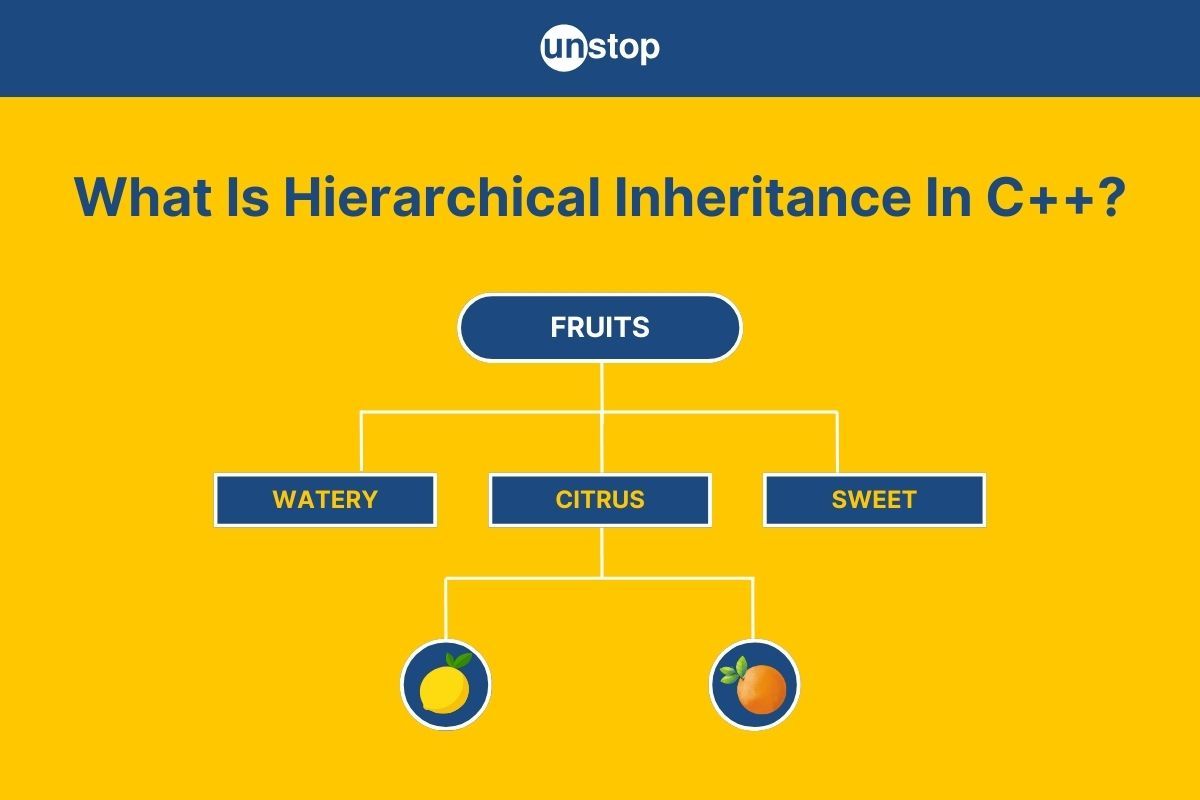
Hierarchical inheritance in C++ is a type of inheritance where multiple derived classes inherit from a single base class. This structure allows each derived class to share common functionality defined in the base class while implementing their unique features, making it an efficient approach for code reuse and organization.
In this article, we will explore the concept of hierarchical inheritance in detail, discussing its syntax, how visibility modes impact access to members and the best use cases for this inheritance model. We will also look at examples to demonstrate how hierarchical inheritance in C++ can be applied in practical scenarios, providing a clear understanding of its benefits and limitations.
What Is Hierarchical Inheritance In C++?
In hierarchical inheritance in C++ programming, a class can be derived from a single base class, and multiple derived classes can be derived from that base class. This type of inheritance creates a hierarchical relationship, where the base class is at the top, and the derived classes are at the bottom.
Characteristics Of Hierarchical Inheritance In C++
Some of the important characteristics of hierarchical inheritance in C++ are as follows:
- Tree Structure: Hierarchical inheritance creates a tree-like structure in programming. This structure allows multiple derived classes to extend from a single base class. Each derived class inherits properties and methods from the base class. This setup resembles a family tree, where the base class is at the top, and subclasses branch out below.
- Shared Functionality: The main advantage of hierarchical inheritance in C++ is the ability to share common functionality across different subclasses. A base class holds shared methods that all derived classes can use. This reduces code duplication and enhances maintainability.
- Constructors in Classes: Constructors play an essential role in hierarchical inheritance in C++. They initialize data members within each class. When creating an object of a derived class, its constructor calls the base class constructor first. This ensures that all inherited attributes are set up correctly.
- Diagram Representation: A diagram often illustrates hierarchical inheritance in C++ better than words alone. It visually represents the relationships between classes. The base class sits at the top, with lines connecting it to its derived classes below. Each line indicates an inheritance relationship.
Real-Life Analogy Of Hierarchical Inheritance In C++
Hierarchical inheritance in C++ an be compared to a family tree. In this analogy, one ancestor represents the base class. This ancestor has multiple descendants or children, which are the derived classes. Each child inherits traits from the ancestor. For example, if the ancestor is a "Fruit" class, each child could be a specific fruit like "Mango," "Apple," or "Banana." Each of these fruits shares common characteristics from the base class but also has unique features.
This structure helps visualize how traits flow down from the base class to the derived classes. The family tree shows how relationships are established. Just like in programming, where derived classes can have their own methods and attributes, children in a family can have their unique traits while still sharing a connection to their ancestors.
Syntax Of Hierarchical Inheritance In C++
class BaseClass {
// Member variables and methods
};class DerivedClass1 : public BaseClass {
// Member variables and methods specific to DerivedClass1
};class DerivedClass2 : public BaseClass {
// Member variables and methods specific to DerivedClass2
};
Here:
- Base Class is the parent class that provides common properties and methods to its derived classes. It can contain member variables and methods that are shared among all derived classes.
- Derived Classes are the child classes that inherit the properties and methods from the base class. They can have their own unique member variables and methods to specialize their functionality.
- The keyword public is used in the derived class declaration to specify public inheritance. This means that the public members of the base class become public members of the derived class.
Example 1: Hierarchical Inheritance In C++
Let's consider a vehicle hierarchy level example, where Vehicle is the base class, and Car, Truck, and Motorcycle are derived classes:
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgp1c2luZyBuYW1lc3BhY2Ugc3RkOwoKY2xhc3MgVmVoaWNsZSB7CnB1YmxpYzoKICAgIHZvaWQgc3RhcnQoKSB7CiAgICAgICAgY291dCA8PCAiVmVoaWNsZSBzdGFydGVkIiA8PCBlbmRsOwogICAgfQoKICAgIHZvaWQgc3RvcCgpIHsKICAgICAgICBjb3V0IDw8ICJWZWhpY2xlIHN0b3BwZWQiIDw8IGVuZGw7CiAgICB9Cn07CgpjbGFzcyBDYXIgOiBwdWJsaWMgVmVoaWNsZSB7CnB1YmxpYzoKICAgIHZvaWQgaG9uaygpIHsKICAgICAgICBjb3V0IDw8ICJDYXIgaG9ua2luZyIgPDwgZW5kbDsKICAgIH0KfTsKCmNsYXNzIFRydWNrIDogcHVibGljIFZlaGljbGUgewpwdWJsaWM6CiAgICB2b2lkIGxvYWQoKSB7CiAgICAgICAgY291dCA8PCAiVHJ1Y2sgbG9hZGluZyIgPDwgZW5kbDsKICAgIH0KfTsKCmNsYXNzIE1vdG9yY3ljbGUgOiBwdWJsaWMgVmVoaWNsZSB7CnB1YmxpYzoKICAgIHZvaWQgd2hlZWxpZSgpIHsKICAgICAgICBjb3V0IDw8ICJNb3RvcmN5Y2xlIGRvaW5nIGEgd2hlZWxpZSIgPDwgZW5kbDsKICAgIH0KfTsKCmludCBtYWluKCkgewogICAgQ2FyIGNhcjsKICAgIFRydWNrIHRydWNrOwogICAgTW90b3JjeWNsZSBtb3RvcmN5Y2xlOwoKICAgIGNhci5zdGFydCgpOwogICAgY2FyLmhvbmsoKTsKICAgIGNhci5zdG9wKCk7CgogICAgdHJ1Y2suc3RhcnQoKTsKICAgIHRydWNrLmxvYWQoKTsKICAgIHRydWNrLnN0b3AoKTsKCiAgICBtb3RvcmN5Y2xlLnN0YXJ0KCk7CiAgICBtb3RvcmN5Y2xlLndoZWVsaWUoKTsKICAgIG1vdG9yY3ljbGUuc3RvcCgpOwoKICAgIHJldHVybiAwOwp9Cg==
Output:
Vehicle started
Car honking
Vehicle stopped
Vehicle started
Truck loading
Vehicle stopped
Vehicle started
Motorcycle doing a wheelie
Vehicle stopped
Explanation:
In the above code example-
- We start by including the necessary header file <iostream> and use the standard namespace.
- Next, we define a base class called Vehicle, which contains functions start() and stop() to represent common behaviors for all vehicles.
- We then create three derived classes: Car, Truck, and Motorcycle, each inheriting from the Vehicle class-
- In the Car class, we implement a method honk() that represents a behavior specific to cars.
- In the Truck class, we implement a method load() that represents a behavior specific to trucks.
- In the Motorcycle class, we implement a method wheelie() that represents a behavior specific to motorcycles.
- Now in the main() function, we instantiate objects of Car, Truck, and Motorcycle.
- We call the start() and stop() methods on each object to demonstrate their inherited behaviors from the Vehicle class-
- For the Car object, we also call the honk() method to show its unique behavior.
- For the Truck object, we call the load() method to illustrate its specific functionality.
- For the Motorcycle object, we call the wheelie() method to demonstrate its unique capability.
- Finally, the main() function returns 0, indicating the successful execution of the program.
Example 2: Hierarchical Inheritance In C++
In this code example, we create a base class Animal that provides a common method for all animals and then derive two classes, Mammal and Bird, each implementing specific behaviours.
Code Example:
I2luY2x1ZGUgPGlvc3RyZWFtPgp1c2luZyBuYW1lc3BhY2Ugc3RkOwoKY2xhc3MgQW5pbWFsIHsgLy8gQmFzZSBjbGFzcwpwdWJsaWM6CiAgICB2b2lkIGVhdCgpIHsKICAgICAgICBjb3V0IDw8ICJBbmltYWwgZWF0cyBmb29kLiIgPDwgZW5kbDsKICAgIH0KfTsKCmNsYXNzIE1hbW1hbCA6IHB1YmxpYyBBbmltYWwgeyAvLyBEZXJpdmVkIGNsYXNzIDEKcHVibGljOgogICAgdm9pZCBnaXZlQmlydGgoKSB7CiAgICAgICAgY291dCA8PCAiTWFtbWFsIGdpdmVzIGJpcnRoIHRvIGxpdmUgeW91bmcuIiA8PCBlbmRsOwogICAgfQp9OwoKY2xhc3MgQmlyZCA6IHB1YmxpYyBBbmltYWwgeyAvLyBEZXJpdmVkIGNsYXNzIDIKcHVibGljOgogICAgdm9pZCBsYXlFZ2dzKCkgewogICAgICAgIGNvdXQgPDwgIkJpcmQgbGF5cyBlZ2dzLiIgPDwgZW5kbDsKICAgIH0KfTsKCmludCBtYWluKCkgewogICAgTWFtbWFsIG1hbW1hbDsKICAgIEJpcmQgYmlyZDsKCiAgICBtYW1tYWwuZWF0KCk7ICAgICAgICAvLyBJbmhlcml0ZWQgbWV0aG9kCiAgICBtYW1tYWwuZ2l2ZUJpcnRoKCk7ICAvLyBEZXJpdmVkIGNsYXNzIG1ldGhvZAoKICAgIGJpcmQuZWF0KCk7ICAgICAgICAgIC8vIEluaGVyaXRlZCBtZXRob2QKICAgIGJpcmQubGF5RWdncygpOyAgICAgIC8vIERlcml2ZWQgY2xhc3MgbWV0aG9kCgogICAgcmV0dXJuIDA7Cn0K
Output:
Animal eats food.
Mammal gives birth to live young.
Animal eats food.
Bird lays eggs.
Explanation:
In the above code example-
- We define a base class called Animal, which includes a function eat() that outputs a message indicating that an animal eats food.
- Next, we create two derived classes, Mammal and Bird, both inheriting from the Animal class-
- In the Mammal class, we add a specific method giveBirth() that outputs a message about mammals giving birth to live young.
- In the Bird class, we include a method layEggs() that outputs a message about birds laying eggs.
- Now, in the main() function, we instantiate objects of Mammal and Bird.
- We call the inherited eat() method from both objects, demonstrating that they share functionality from the Animal class.
- We also call the giveBirth() method on the Mammal object and the layEggs() method on the Bird object to show their unique behaviours.
This example illustrates hierarchical inheritance in C++ by showcasing how multiple derived classes can inherit from a single base class while implementing their specific functionalities.
Impact of Visibility Modes In Hierarchical Inheritance In C++
In hierarchical inheritance in C++, visibility modes (or access specifiers) play a crucial role in determining how base class members are inherited and accessed by derived classes. The three visibility modes are public, protected, and private, and each has a different impact on how derived classes can interact with the inherited members.
Public Visibility Mode
- Members of the base class marked as public remain public in the derived class.
- Members marked as protected remain protected in the derived class.
- Members marked as private are not accessible in the derived class.
- Impact: In public inheritance, derived classes can access and use base class public members directly, maintaining the intended accessibility.
class Base {
public:
int x; // Public member
protected:
int y; // Protected member
private:
int z; // Private member
};
class Derived : public Base {
public:
void show() {
x = 10; // Accessible
y = 20; // Accessible
// z is not accessible (private in Base)
}
};
Protected Visibility Mode
- Protected access specifiers provide a middle ground between public and private.
- Members of the base class marked as public or protected become protected in the derived class.
- Members marked as private remain inaccessible in the derived class.
- Impact: Protected inheritance limits access to base class members in further derived classes, promoting encapsulation but restricting use.
class Derived : protected Base {
public:
void show() {
x = 10; // Accessible but becomes protected
y = 20; // Accessible but protected
// z is not accessible (private in Base)
}
};
Private Visibility Mode
- All members of the base class, whether public or protected, become private in the derived class.
- Members marked as private in the base class are still inaccessible in the derived class.
- Impact: Private inheritance makes all base class members private in the derived class, so they cannot be accessed directly by further derived classes or outside code.
For Example:
class Derived : private Base {
public:
void show() {
x = 10; // Accessible but becomes private
y = 20; // Accessible but private
// z is not accessible (private in Base)
}
};
Encapsulation Effects
Visibility modes significantly influence encapsulation in hierarchical inheritance. Public and protected modes allow for more exposure of base class details. This can enhance usability but may reduce encapsulation. Private mode strengthens encapsulation by hiding implementation details from derived classes.
Effective encapsulation leads to cleaner code and easier maintenance. It allows developers to change internal implementations without affecting derived classes or external code. As a result, understanding these visibility modes is crucial for designing robust systems.
Member Exposure Control
Control over member exposure is vital in managing how derived classes interact with base class members. Public members encourage reuse but risk misuse. Protected members offer some safety while maintaining accessibility for derived classes. Private members prioritize safety but limit direct interaction.
Developers must choose visibility modes based on their design goals. They should consider how much access is necessary for derived classes while ensuring that core functionality remains intact.
Advantages And Disadvantages Of Hierarchical Inheritance In C++
Hierarchical inheritance offers benefits, but it also comes with drawbacks. Let's explore the advantages and disadvantages of hierarchical inheritance in C++.
Advantages Of Hierarchical Inheritance In C++
- Code Reusability: Hierarchical inheritance promotes code reusability. Common functionality can be defined in a base class, and multiple derived classes can inherit and reuse this code.
- Logical Structure: It creates a clear and logical structure for the program. The relationship between classes is easier to understand, making it beneficial for modelling real-world relationships.
- Easier Maintenance: Changes made in the base class can automatically propagate to derived classes, simplifying maintenance. This reduces the likelihood of code duplication and errors.
- Polymorphism: Hierarchical inheritance supports polymorphism, allowing us to call derived class methods through base class pointers or references. This enhances flexibility and dynamic behavior in programs.
- Organized Code: It helps in organizing the code effectively by grouping related classes under a common parent class, making the overall architecture more manageable.
Disadvantages Of Hierarchical Inheritance In C++
- Complexity: As the number of derived classes increases, the hierarchy can become complex and harder to navigate, potentially leading to confusion and errors in understanding the relationships between classes.
- Tight Coupling: Hierarchical inheritance can lead to tight coupling between classes. Changes in the base class can inadvertently affect all derived classes, which may introduce bugs or require additional modifications.
- Inheritance Overhead: There is some overhead associated with inheritance. Accessing members through base class pointers can be slightly slower due to the additional indirection.
- Diamond Problem: Although hierarchical inheritance itself does not cause the diamond problem, it can complicate matters if multiple inheritance is introduced in conjunction with it, potentially leading to ambiguity.
- Limited Flexibility: Once a class is established in a hierarchy, changing its place or structure may require significant refactoring, limiting design flexibility.
Understanding these limitations is vital for effective programming in C++. By recognizing the complexities and risks involved, developers can make informed decisions about using this inheritance model.
Use Cases Of Hierarchical Inheritance In C++
Here are some key use cases of hierarchical inheritance in C++:
- Hierarchical inheritance in C++ effectively models real-world relationships, such as representing entities like animals, vehicles, shapes or employees.
- Hierarchical structures allow a base class to define common properties and behaviours, which can be inherited by multiple derived classes, enhancing code reusability.
- In GUI frameworks, using hierarchical inheritance can organize different types of UI components (e.g., buttons, text boxes) under a common base class, facilitating shared functionalities like rendering and event handling.
- For payment processing systems, hierarchical inheritance enables the creation of different payment methods (e.g., credit card, PayPal) that inherit common processing logic from a base payment method class.
- In a database access layer, hierarchical inheritance helps manage various data sources (e.g., SQL, NoSQL, file) by allowing each derived class to implement specific connection and retrieval logic while reusing base class functionality.
- In game development, a hierarchical format can model different game entities (e.g., players, enemies, NPCs) with shared properties like position and movement while allowing each entity to implement its unique behaviours.
- It supports polymorphism, enabling derived classes to be used interchangeably with base class references or pointers in programs, thus enhancing flexibility.
Conclusion
Hierarchical inheritance in C++ provides a powerful mechanism for organizing and reusing code by allowing multiple derived classes to inherit from a common base class. It promotes a clear structure by sharing common functionality while enabling specialized behavior in derived classes. By effectively using visibility modes, we can control access to inherited members, ensuring encapsulation and maintaining the integrity of the class hierarchy. This inheritance model proves valuable in real-world applications where objects share fundamental traits yet exhibit distinct characteristics.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What is hierarchical inheritance in C++?
Hierarchical inheritance in C++ is a type of inheritance where a class can be derived from a single base class, and multiple derived classes can be derived from that base class. This creates a hierarchical relationship, where the base class is at the top, and the derived classes are at the bottom.
Imagine a family tree where "Vehicle" is the grandparent, "Car" and "Truck" are the parents, and "Sedan," "SUV," and "Pickup" are the children. In this analogy, "Vehicle" is the base class, "Car" and "Truck" are derived classes, and "Sedan," "SUV," and "Pickup" are further derived classes.
Q. How does hierarchical inheritance in C++ differ from other types?
Hierarchical inheritance in C++ differs from other types of inheritances in C++ in the following ways:
Feature | Hierarchical Inheritance | Single Inheritance | Multiple Inheritance | Hybrid Inheritance |
---|---|---|---|---|
Number of base classes | Multiple | One | Multiple | Combination |
Relationship | Parent-child | Parent-child | Parent-child | Combination |
Ambiguity | Potential | Less likely | More likely | Depends on the combination |
Complexity | Moderate | Simple | Complex | Can be complex |
In summary, hierarchical inheritance in C++ is characterized by a single base class and multiple derived classes, while other inheritance types involve different relationships and potential complexities.
Q. Are there any drawbacks to hierarchical inheritance in C++?
Yes, there are potential drawbacks to hierarchical inheritance:
- Complexity: Overusing hierarchical inheritance can make your code more complex and difficult to understand. If the class hierarchy becomes too deep or convoluted, it can be challenging to follow the relationships between classes and their members.
- Performance: In some cases, hierarchical inheritance can introduce performance overhead due to virtual function calls. When a derived class object calls a virtual function inherited from the base class, the compiler must determine the appropriate implementation at runtime, which can be slower than direct function calls.
- Maintenance: Changes to the base class can affect all derived classes. This means that modifying the base class can have a cascading impact on the entire class hierarchy, making it more difficult to maintain and update the code.
- Multiple inheritance ambiguity: When a derived class inherits from multiple base classes that have conflicting members (e.g., methods with the same name but different implementations), it can lead to ambiguity and compilation errors. This can be challenging to resolve, especially in a complex hierarchy among the classes.
Q. When should I use hierarchical inheritance?
Hierarchical inheritance properties are most suitable when you have a clear parent-child relationship between classes and want to:
- Promote code reusability: The base class can define common properties and methods that can be shared by multiple derived classes, reducing code duplication.
- Create a clear class hierarchy: The hierarchical structure can represent a logical relationship between classes, making the code easier to understand and maintain.
- Implement polymorphism: You can use hierarchical inheritance to create polymorphic relationships (object oriented programming), where objects of different derived classes can be treated as objects of the base class.
- Model real-world relationships: Hierarchical inheritance can be used to model real-world relationships, such as the relationship between vehicles (base class) and cars, trucks, and motorcycles (derived classes).
Q. Can hierarchical inheritance lead to ambiguity?
Yes, hierarchical inheritance can lead to ambiguity. This can occur when a derived class inherits from multiple base classes that have conflicting members (e.g., member functions with the same name but different implementations). This situation is known as multiple inheritance ambiguity. For example-
class A {
public:
void method() {
cout << "A::method()" << endl;
}
};
class B {
public:
void method() {
cout << "B::method()" << endl;
}
};
class C : public A, public B {
public:
void callMethod() {
method(); // Ambiguous call
}
};
In this example, class C inherits from both A and B, both of which have a method() function. When C calls method(), the compiler cannot determine which version to call, leading to an ambiguity error. If ambiguity arises from multiple inheritance (such as in the diamond problem), virtual inheritance can be used to ensure that only one instance of the base class is inherited.
This compiles our discussion on hierarchical inheritance in C++. You must also check out the following:
- Constructor Overloading In C++ Explained With Real Examples
- C++ If-Else | All Conditional Statements Explained With Examples
- Logical Operators In C++ | Use, Precedence & More (With Examples)
- C++ 2D Array & Multi-Dimensional Arrays Explained (+Examples)
- C++ Exception Handling | Use Try, Catch, & Throw (+Examples)
- Data Abstraction In C++ | Types, Use-Cases & More (With Examples)
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment