Advantages And Disadvantages Of SQL Simplified (With Examples)
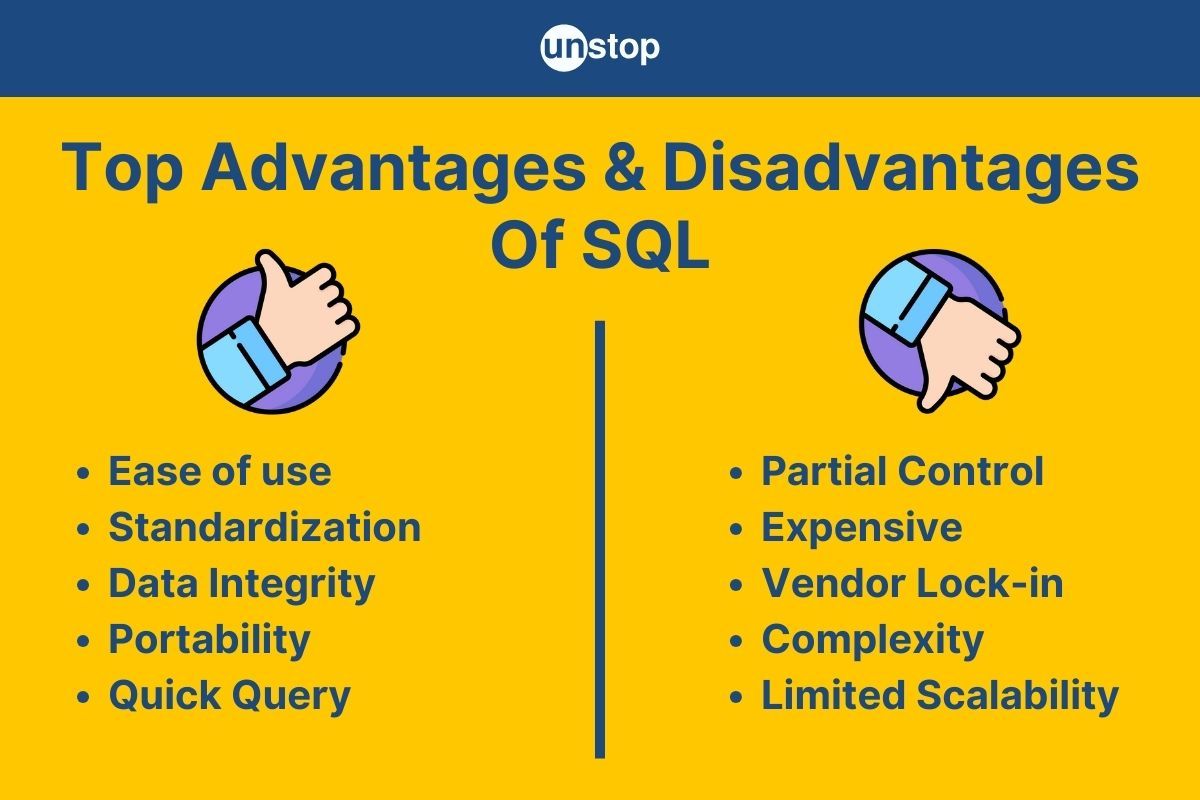
SQL stands for Structured Query Language. It is a domain-specific programming language that is designed for managing and manipulating data, such as insertion, deletion, and updation, held in a relational database management system (RDBMS) or for stream processing in a relational data stream management system. According to the American National Standards Institute, it is the standard language for relational database management systems. Moreover, It is also approved by the ISO (International Standard Organization). In this article, we will discuss the advantages and disadvantages of SQL programming language, how it works, and its applications for a variety of tasks.
How Does SQL Work?
Structured Query Language (SQL) is a domain-specific language designed for the management, manipulation, and dynamic modification of relational databases. It serves as a standard interface for interacting with relational database management systems (RDBMS), allowing users to perform a variety of routine tasks and operations on data, such as querying, updating, inserting, and deleting records.
Here's an overview of how SQL works:
Database Creation:
SQL starts with the creation of a relational database. A database is a structured collection of data organized in tables.
CREATE DATABASE mydatabase;
Within the databases, tables define the structure of the data, with each table consisting of rows and columns.
Database Table Creation:
You use SQL to define tables within the database. Each table has a name and a set of columns with specified data types.
CREATE TABLE employees (
employee_id INT,
first_name VARCHAR(50),
last_name VARCHAR(50),
hire_date DATE
);
Inserting Data:
Once tables are created, you can insert data into them using the INSERT statement.
INSERT INTO employees VALUES (1, 'John', 'Doe', '2023-01-01');
Querying Data:
The core function of SQL is to query data. The SELECT statement is used for this purpose.
SELECT first_name, last_name FROM employees WHERE employee_id = 1;
In this example, the query retrieves the first name and last name of the employee with an ID of 1.
Updating Data:
SQL provides the UPDATE statement to modify existing data in a table.
UPDATE employees SET first_name = 'Jane' WHERE employee_id = 1;
This statement changes the first name of the employee with ID 1 to "Jane."
Deleting Data:
The DELETE statement is used to remove data from a table.
DELETE FROM employees WHERE employee_id = 1;
This example shows how to remove the employee with ID 1 from the 'employees' table.
Data Integrity:
SQL enforces data integrity using constraints. Common constraints include PRIMARY KEY, FOREIGN KEY, UNIQUE, and CHECK.
ALTER TABLE employees
ADD CONSTRAINT pk_employee PRIMARY KEY (employee_id);
These lines of code allow us to add a primary key constraint to ensure the uniqueness of the "employee_id" column.
Joining Tables:
SQL allows you to combine data from multiple tables using JOIN operations.
SELECT employees.first_name, departments.department_name
FROM employees
JOIN departments ON employees.department_id = departments.department_id;
This query retrieves the first name of employees along with their corresponding department names by joining the 'employees' and 'departments' tables.
Transactions:
SQL supports transactions, which allow you to group multiple SQL statements into a single atomic operation. This ensures that either all the statements in the transaction are executed or none of them are.
BEGIN TRANSACTION;
UPDATE accounts SET balance = balance - 100 WHERE account_id = 1;
UPDATE accounts SET balance = balance + 100 WHERE account_id = 2;
COMMIT;
These lines of code showcase how to begin a transaction that transfers 100 units from one account to another.
Security:
SQL databases implement security measures to control access to data. This includes user authentication, authorization, and role-based access control.
GRANT SELECT ON employees TO user1;
Important Features Of SQL
Structured Query Language (SQL) is a domain language that offers a variety of features that make it a powerful and versatile tool for managing and manipulating relational databases. Here are some key features of SQL:
- Data Query Language (DQL): SQL provides a Data Query Language (DQL) that allows users to retrieve data from numerous tables. The SELECT statement is used for querying data. It supports filtering, sorting, and grouping of data as per your specific requirements.
SELECT column1, column2 FROM table WHERE condition;
- Data Definition Language (DDL): DDL statements in SQL are used to define, modify, and delete the structure of a database and its objects. Common DDL statements include CREATE, ALTER, and DROP.
CREATE TABLE table_name (column1 datatype, column2 datatype, ...);
Also read- What Is The Difference Between DELETE, DROP And TRUNCATE?
- Data Manipulation Language (DML): DML statements in SQL are used to manipulate data stored in the database. Common DML statements include INSERT, UPDATE, and DELETE.
INSERT INTO table_name (column1, column2, ...) VALUES (value1, value2, ...);
- Data Control Language (DCL): DCL statements control access to data within the database. For example, the GRANT statement can be used to provide specific privileges to users, while REVOKE is used to remove those privileges.
GRANT SELECT, INSERT ON table_name TO user_name;
- Transaction Control: SQL supports transactions, which are sequences of one or more SQL statements treated as a single unit of work. The COMMIT statement is used to save changes, and ROLLBACK is used to undo changes if an error occurs.
BEGIN TRANSACTION;
-- SQL statements
COMMIT;
-- or ROLLBACK if needed
- Data Integrity Constraints: SQL allows the definition of various constraints to maintain data integrity, such as foreign keys, primary keys, unique constraints, and check constraints. For example-
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
department_id INT,
FOREIGN KEY (department_id) REFERENCES departments(department_id)
);
- Joins: SQL supports different types of joins to combine rows from two or more tables based on related columns. Common join types include INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN. For example-
SELECT employees.first_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
- Aggregation Functions: SQL provides aggregate functions like SUM, AVG, MIN, MAX, and COUNT for performing calculations on sets of values. For example-
SELECT AVG(salary) FROM employees WHERE department_id = 1;
Advantages And Disadvantages Of SQL
Advantages of SQL | Disadvantages of SQL |
---|---|
1. Ease of Use: SQL is a simple and easy-to-learn language with no complicated queries as such, making it accessible to both beginners and experienced developers. | 1. Limited Scalability: SQL databases are enabled with vertical scaling by enhancing hardware investment. Hence, they may face challenges when it comes to horizontal scaling, especially with large datasets and high traffic. |
2. Standardization: SQL is a standardized language that ensures consistency and compatibility across various database management systems (DBMS). | 2. Complexity: Complex queries and database designs can be challenging to optimize and maintain. |
3. Flexibility: SQL provides flexibility in querying and manipulating database components, allowing for a wide range of operations on database schemas and systems. | 3. Lack of Real-time Processing: SQL databases might not be the preferred choice for businesses and real-time applications due to potential latency in data processing. |
4. Data Integrity: SQL databases enforce data integrity constraints (e.g., primary keys, foreign keys) to maintain accurate and reliable data relationships between tables. | 4. Cost: Some commercial SQL databases can be expensive, especially for large enterprises requiring advanced features and support. |
5. Support for Transactions: SQL supports ACID properties (Atomicity, Consistency, Isolation, Durability), ensuring the reliability of database transactions. | 5. Limited Support for Unstructured Database: SQL is primarily designed for structured data, making it less suitable for handling unstructured database types like images or videos. |
6. Security: SQL databases offer robust security features, including user authentication, authorization, and encryption. | 6. Learning Curve: While the basics are easy to grasp, mastering advanced SQL concepts and optimization techniques can be challenging for some users. |
7. Powerful Query Language: SQL's declarative nature allows users to express what they want to achieve without specifying how to achieve it, making queries powerful and expressive. | 7. Single Point of Failure: If a central SQL database fails, it can lead to a complete system failure, impacting all applications relying on it. |
8. Community Support: SQL has a large and active community, providing resources, forums, and documentation for developers. | 8. Vendor Lock-in: Switching from one SQL database vendor to another may be challenging due to differences in implementations and features. |
Advantages & Disadvantages Of SQL Explained
SQL is an efficient language for database communication. It has various advantages, some of which are listed below:
- Simple and easy: One of the things that makes SQL a popular and reliable choice is that it doesn't require any substantial knowledge about coding and writing programs. SQL has some basic keywords, such as SELECT, INSERT INTO, UPDATE, etc., that can carry out tasks. Syntactical rules are also simple and easy to follow.
- Faster Query Processing: SQL works with an efficient speed. Huge data can be processed in seconds! Querying, manipulating, and conducting calculations on data with analytical queries in a relational database can be done in no time.
- Standard Language: As already mentioned, SQL is a standardized structured query language approved by ISO and ANSI for relational databases. Its long, detailed, and established documentation over the years pose as a strong plus point.
- Portable: It is highly portable as used in PCs, application servers, a few mobile phones, and independent laptops having any operating system such as Windows, Linux, Mac, etc. It can also be embedded with other applications.
- Highly Interactive: As it has simple commands for all purposes, it becomes an interactive language for its users. It is easy to understand, and the commands are also understandable to the non-programmers.
As is evident, there are multiple upsides to using SQL for database management and manipulation. However, this does not mean that the language is free of shortcomings. Explained below are some disadvantages of SQL and you must bear in mind when working with it.
- Poor Interface: SQL has a poor interface, which leads things to look very complex, even if they are not. Users find it difficult to deal with databases due to the difficulty of using the SQL interface.
- Cost Inefficient: SQL Server Standard costs around $1,418/year. The high cost makes it difficult for some programmers to use it.
- Partial Control: SQL doesn't grant its users complete control over databases due to some hidden business rules. This can be a huge drawback for many.
- Security: Regardless of the SQL version, databases in SQL are constantly under threat as they hold huge amounts of sensitive data.
Applications Of SQL
Structured Query Language (SQL) is a powerful tool with a wide range of applications in various fields. Here are some common applications of SQL:
-
Database Management Systems (DBMS): SQL is primarily used for managing and interacting with relational database management systems (RDBMS). Popular RDBMS like MySQL, PostgreSQL, SQLite, Microsoft SQL Server, and Oracle Database use SQL as their standard query language.
-
Web Development: SQL is extensively used in web development for creating and managing databases that store website data. It enables developers to interact with databases to store, retrieve, and manipulate data, providing dynamic content for websites.
-
Business Intelligence (BI): SQL is essential in business intelligence for extracting and analyzing data from different database types. Analysts use SQL queries to gather insights, generate reports, and make data-driven decisions based on the information stored in databases.
-
Data Warehousing: SQL plays a crucial role in data warehousing, where large volumes of data from different sources are consolidated for analysis and reporting. Data warehousing solutions often use SQL for data extraction, drive space, transformation, and loading (ETL) processes.
-
Mobile App Development: Mobile applications often need to store and retrieve data, and SQL is commonly used in the development of mobile apps. SQLite, a lightweight and embedded RDBMS, is frequently used in mobile development and relies on SQL for database operations.
-
Content Management Systems (CMS): CMS platforms, such as WordPress and Joomla, use SQL databases to store and manage content. SQL queries are used to retrieve and update content, manage user data, and handle other database-related tasks.
-
E-commerce: Online stores and e-commerce platforms use SQL databases to manage product catalogs, customer information, order details, and transaction records. SQL queries are employed to retrieve and update information as users interact with the uniform platform.
-
Healthcare Systems: SQL is utilized in healthcare systems for managing patient records, medical histories, and other healthcare-related data. It helps healthcare professionals access and update patient information securely.
-
Financial Systems: SQL is crucial in financial application development for managing transactions, auditing, and reporting. It is used in banking systems, accounting software, and financial analysis tools to handle large volumes of financial data.
-
Gaming: SQL databases are employed in the gaming industry to store and manage game-related data and increase optimal performance, such as player profiles, scores, and achievements. SQL is used to ensure data consistency and provide a reliable gaming experience.
-
Logistics and Supply Chain Management: SQL is applied in logistics and supply chain systems to manage inventory, track shipments, and optimize supply chain processes. It helps in maintaining accurate records of goods movement and inventory levels.
-
Educational Systems: SQL databases are used by suitable database administrators in educational institutions to manage student records, course information, and grades. SQL queries facilitate the retrieval of student-related data for administrative and reporting purposes.
Conclusion
In conclusion, SQL is a robust and widely adopted language for managing relational databases. However, you must carefully evaluate the advantages and disadvantages of SQL in the context of your specific use case before using it. Understanding these aspects can help developers and organizations make informed decisions when selecting a database management system that aligns with their requirements and priorities.
Also read- 70+ Best PL/SQL Interview Questions With Answers 2023
Frequently Asked Questions (FAQs)
Q. What are the key advantages of using SQL in database management?
SQL offers advantages such as ensuring data integrity through constraints, providing a standardized language for interacting with databases, supporting efficient data retrieval with the SELECT statement, and scalability to handle larger datasets and increased loads.
Q. How does SQL contribute to data normalization in relational databases?
SQL supports the normalization process by allowing the definition of constraints like primary and foreign keys, facilitating the creation of efficient and normalized database structures that minimize data redundancy and dependency.
Q. What are some disadvantages of SQL, and how do they impact database management?
Disadvantages include a potentially steep learning curve for beginners, limitations in scalability and lack of flexibility in certain scenarios, challenges with unstructured data, and the complexity of queries with multiple joins. These factors can affect the ease of use, performance, and adaptability of SQL databases.
Q. How does SQL adhere to the ACID properties, and why is this important for database transactions?
SQL databases follow the ACID properties (Atomicity, Consistency, Isolation, Durability) to ensure reliable and secure transactions. This is crucial for maintaining data consistency and integrity, especially in applications where accurate and secure transactions are essential, such as financial or healthcare systems.
Q. Can SQL databases lead to vendor lock-in, and what implications does this have for businesses?
Yes, SQL databases may exhibit vendor lock-in because different database vendors may implement SQL slightly differently. This can make it challenging to switch from one SQL database to another, potentially requiring significant effort and resources. Businesses should consider the long-term implications of vendor lock-in when selecting a database management system.
The advantages and disadvantages of SQL explained above are sure to help you better handle your SQL projects. Here are a few other interesting articles you must read:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Blogs you need to hog!
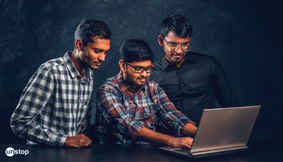
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
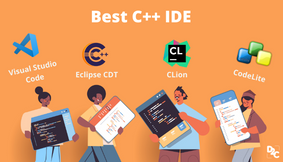
10 Best C++ IDEs That Developers Mention The Most!
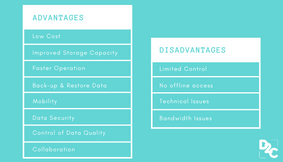
Advantages and Disadvantages of Cloud Computing that you should know!
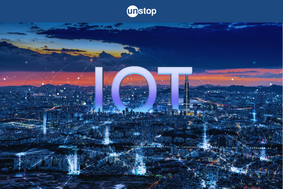
Comments
Add comment