Diamond Problem In C++ & Its Resolutions Explained (+Examples)
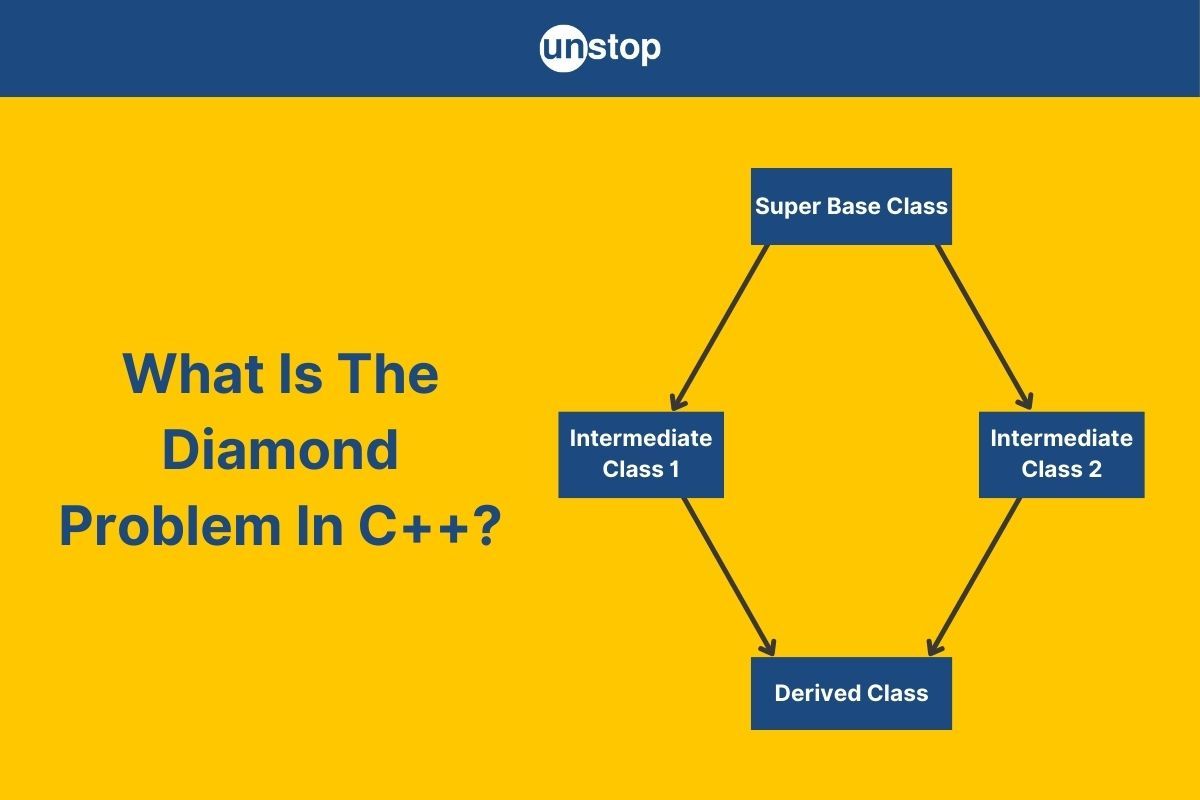
The concept of inheritance in C++ allows a class to acquire the properties and behaviours of another class, promoting code reusability. However, in multiple inheritance with multilevel inheritance, where one class inherits from multiple classes with a common base class, this inheritance may lead to structural problems.
In this article, we will discuss this problem, known as the diamond problem in C++ classes, in detail with examples. We will also discuss how you can resolve the problem or guard against it in your code.
What Is The Diamond Problem In C++?
The infamous diamond problem in C++ programming is a classic issue in object-oriented programming. It occurs when a class inherits from two classes that both derive from the same base class. This situation creates ambiguity and redundancy, as the inheriting class has two instances of the base class—leading to conflicts, particularly with shared members or functions.
Imagine a real-world example of multiple inheritance and the diamond problem in C++ classes:
- Say there is a class called Vehicle, which provides basic functionality like starting the engine. This class is also referred to as the super base class.
- Now, you derive two classes from it: Car and Boat. Both of these subclasses inherit the characteristics of the class Vehicle. In the diamond problem, both of these can be referred to as base classes.
- Finally, you have a new class, AmphibiousVehicle, which inherits from both Car and Boat. This will be referred to as the derived class (with multiple inheritances).
Since both Car and Boat themselves inherit from Vehicle, the class AmphibiousVehicle has two copies of the Vehicle class. This can lead to confusion—such as which startEngine() method should be called if it’s not resolved properly.
The diamond shape comes from how this inheritance is structured:
- At the top, you have the Vehicle class.
- The second level has Car and Boat, where both class share the Vehicle class.
- The third level has AmphibiousVehicle, which inherits from both Car and Boat.
Visually, this forms a diamond-like structure in the class hierarchy, hence the name diamond problem in C++ classes. This design leads to ambiguity as the derived class (AmphibiousVehicle) may have access to members or methods from Vehicle class through both Car and Boat.
If both Car and Boat define a method with the same name from the base class, it becomes unclear which version class AmphibiousVehicle should use. If we call this method without specifying the path, the compiler will raise an error. This error indicates that it cannot determine which method to execute.
Example Of The Diamond Problem In C++
In this section, we will continue with the example of the Vehicle and AmphibiousVehicle classes above and see how this translates into code. The program below is a multiple inheritance illustration that highlights the diamond issue.
Code Example:
Output:
error: request for member ‘startEngine’ is ambiguous
Explanation:
In the C++ program example,
- We define the base class Vehicle with a public member function, startEngine() that prints the string message- "Vehicle engine started." to the console.
- Then we define two separate classes: Car and Boat, both of which publicly inherit from Vehicle, meaning they have access to the public member functions/ data members of the parent class Vehicle.
- Next, we define the class AmphibiousVehicle inherits from both Car and Boat, creating a diamond structure. As a result, it inherits two copies of the startEngine() method — one from Car (via Vehicle) and one from Boat (via Vehicle).
- In the main() function, we create an object amphibious of class AmphibiousVehicle.
- We then use the object to call the startEngine() function. As mentioned in the code comment, this will lead to ambiguity as the compiler doesn't know whether to use the startEngine() method from the Car side or the Boat side.
- The compiler throws an error, as shown in the output. In C++ classes, this is called the diamond problem.
Resolution Of The Diamond Problem In C++
There are two primary ways of resolving the diamond problem in C++ classes:
- Virtual Inheritance: This is the most common solution to the diamond problem. By using virtual inheritance, the derived classes share a single instance of the base class, preventing duplication and ambiguity. It ensures that only one copy of the base class is inherited, even when multiple paths exist.
- Scope Resolution Operator: Denoted by the double colon symbol (::), you can use the operator to explicitly specify which base class’s method you want to call. This way, you manually resolve the ambiguity by qualifying the method call with the class name.
Each of these methods has its use case, and they can be used to resolve the diamond issue depending on the complexity of the inheritance structure. We'll explore them in detail in the following sections.
Check out this amazing course to become the best version of the C++ programmer you can be.
Virtual Inheritance To Resolve Diamond Problem In C++
Virtual inheritance is a feature in C++ that allows classes to avoid the diamond problem by sharing a single instance of the base class. This is achieved by marking the inheritance of a class as virtual (using the virtual keyword). When a class is inherited virtually, C++ ensures that only one copy of the base class exists, even if multiple derived classes are inherited from it.
How Virtual Inheritance Resolves The Diamond Problem?
In the diamond problem, two intermediate classes inherit from a common base class, and a final derived class inherits from both intermediates.
- Without virtual inheritance, each intermediate class creates its own copy of the base class, leading to ambiguity. This results in multiple instances of the base class being present in the derived class, causing confusion when accessing members of the base class.
- With virtual inheritance, C++ creates a shared single instance of the base class, regardless of how many intermediate classes inherit from it. This way, when the final derived class accesses the base class, it only refers to this single, shared instance, eliminating ambiguity and ensuring a clean and structured inheritance model.
In the example of a diamond problem in C++ classes discussed above, we can mark the Boat and Car classes as inheriting virtually from the Vehicle class. This virtual inheritance case will ensure that the derived class AmphibiousVehicle has only one instance of the base class (Vehicle) instead of two.
By marking the inheritance as virtual, the ambiguity is resolved, and the structure remains consistent without duplication. The C++ code example below illustrates how this can be done.
Code Example:
Output:
Vehicle constructor called
Car constructor called
Boat constructor called
AmphibiousVehicle constructor called
Vehicle engine started
Explanation:
- We have a base class Vehicle, which contains a constructor and a public member function startEngine() that outputs a message when the engine is started.
- Then, we define two derived classes, Car and Boat, both inheriting from Vehicle. Ideally, by default, this inheritance hierarchy would cause each of them to have their own instance of Vehicle.
- However, we solve this issue using virtual inheritance. The virtual keyword ensures that only one instance of the Vehicle class is shared between Car and Boat. This eliminates ambiguity and redundancy.
- Finally, we define the AmphibiousVehicle class, which inherits from both Car and Boat.
- In the main() function, we instantiate the object av of the AmphibiousVehicle class and use it to call the startEngine() function.
- Here, the compiler knows which Vehicle instance to use, preventing any conflicts, as shown in the diamond problem output. (In this case, the base constructor is called only once since both intermediate classes virtually inherit from a single parent class).
Challenges Of Virtual Inheritance For Diamond Problem In C++
While virtual inheritance is the most common method of resolving the diamond problem in C++ classes, there are some challenges you must be aware of.
Complexity Issues: Virtual inheritance introduces complexity especially when using multiple inheritance feature with multilevel inheritance, where the intermediate class shares a common base class. The inheritance hierarchy can become tangled (as opposed to in single inheritance), leading to difficulties in understanding how data flows through the system.
Performance Overhead: The virtual inheritance process also adds overhead, additional memory usage and increased processing time. Each virtual base class requires a pointer to track its location in memory. As the number of classes increases, this overhead can grow significantly.
Initialization Importance: Proper initialization of virtual base classes is crucial. Failing to do so can lead to runtime errors that are difficult to debug. When a class inherits from multiple sources, it must ensure that all virtual bases are initialized correctly before use. If not, it may access uninitialized data, causing unpredictable behavior.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and claim the bragging rights, now!
Scope Resolution Operator To Resolve Diamond Problem In C++
The scope resolution operator (::
) in C++ defines the context in which an identifier (such as a variable name or a function to be invoked) resides. It helps clarify which class's member function or variable is being referenced, especially in scenarios involving complex inheritance structures.
In the context of the diamond problem in C++, the scope resolution operator can be used to explicitly specify which base class method should be invoked, allowing the programmer to avoid ambiguity when multiple base classes provide the same method. Look at the example C++ program below to see how this is done.
Code Example:
Output:
Vehicle engine started.
Vehicle engine started.
Explanation:
The inheritance order remains the same as in the previous example, with classes Vehicle (super base class), Car and Boat (intermediate class child inheriting from Vehicle) and AphibiousVehicle (derived class inheriting from two different parent classes Boat and Car).
- In the main() function, we create an object av of the AmphibiousVehicle class.
- We then use this object to call the startEngine() function twice.
- In the first case, we use the scope resolution operator to specify that the function member access should be done via the Car parent class.
- Similarly, in the second case, we specify that member access should be done via the Boat class.
Looking for guidance? Find the perfect mentor from select experienced coding & software experts here.
Conclusion
The diamond problem in C++ occurs when a derived class inherits from two different base classes (intermediate base class) that further share a single parent class. Here, the inheritance graph takes a diamond-like structure as we employ various inheritances, namely multiple inheritance with multilevel inheritance.
The problem arises when accessing members from the single parent class without specifying which intermediate class we want to invoke. Since the compiler does not know which route to take, it throws an error due to ambiguity. There are two ways of resolving this problem: the virtual inheritance process and the scope resolution operator. Understanding the resolution to the diamond problem in C++ classes and member access is crucial for any developer. It helps in designing robust systems and avoiding pitfalls in inheritance.
Also Read: 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q1. What is the diamond problem in C++?
The diamond problem in C++ classes arises in scenarios where we have multilevel inheritance with multiple inheritance. That is when a class inherits from two parent classes (multiple inheritance illustration) that already inherit from a common base class (multilevel inheritance).
This inheritance hierarchy creates ambiguity, as the derived class may inherit conflicting methods or properties from the base class or multiple instances of the common base class. So, when we attempt to access the conflicting methods, the compiler will be confused, as it does not know which intermediate base class to use.
Q2. How does virtual inheritance solve the diamond problem in C++ classes?
Virtual inheritance ensures that only one instance of a base class is shared among derived classes. This eliminates ambiguity and prevents multiple copies of the base class. Since the compiler has a clear path for accessing members of base classes, it eliminates the diamond problem in C++ programs.
Q3. Can you provide an example of the diamond problem?
Consider classes A
, B
, and C
, where both B
and C
inherit from A
, while class D
inherits from both B
and C
.
- Here, both
B
andC
will have access to the member functions and data members of class A (through direct inheritance), and class D will have access to the same members via both B and C. - So, if we use an instance of class D to call member functions from A, it will lead to confusion about which intermediate class to use to invoke the method.
- This is the diamond problem in C++ classes that arises when we have multiple inheritances with multilevel inheritances.
Q4. What are the challenges with virtual inheritance?
While virtual inheritance resolves the diamond problem in C++ classes, it also introduces complexity to the inheritance hierarchy. Developers must manage base constructor calls carefully and comprehend how virtual tables work, which can complicate class design and debugging.
Q5. Is the diamond problem specific to C++?
No, the diamond problem can occur in any programming language that supports multiple inheritance. However, C++ language offers specific mechanisms, such as virtual inheritance, to address it effectively.
Q6. How can I avoid the diamond problem in my code?
To avoid the diamond problem, consider using composition over inheritance. Alternatively, limit your use of multiple inheritance or utilize interfaces where possible to maintain clarity in your class hierarchy.
Q7. Are there any performance implications of using virtual inheritance?
Yes, virtual inheritance can introduce slight overhead due to additional indirection and memory management. However, this trade-off is often worthwhile for clearer and more maintainable code when employing numerous inheritances leading to complex hierarchies.
This compiles our discussion on the diamond problem in C++ classes. Do check the following out for more:
- Friend Function In C++ Classes | Types, Uses & More (+Examples)
- Static Member Function In C++: How to Use Them, Properties, & More
- Encapsulation In C++ | Working, Types, Benefits & More (+Examples)
- Array In C++ | Define, Types, Access & More (Detailed Examples)
- C++ Templates | Types, Usage, Overloading & More (+Code Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment