C++ Find() In Vector | How To Find Element In Vector With Examples
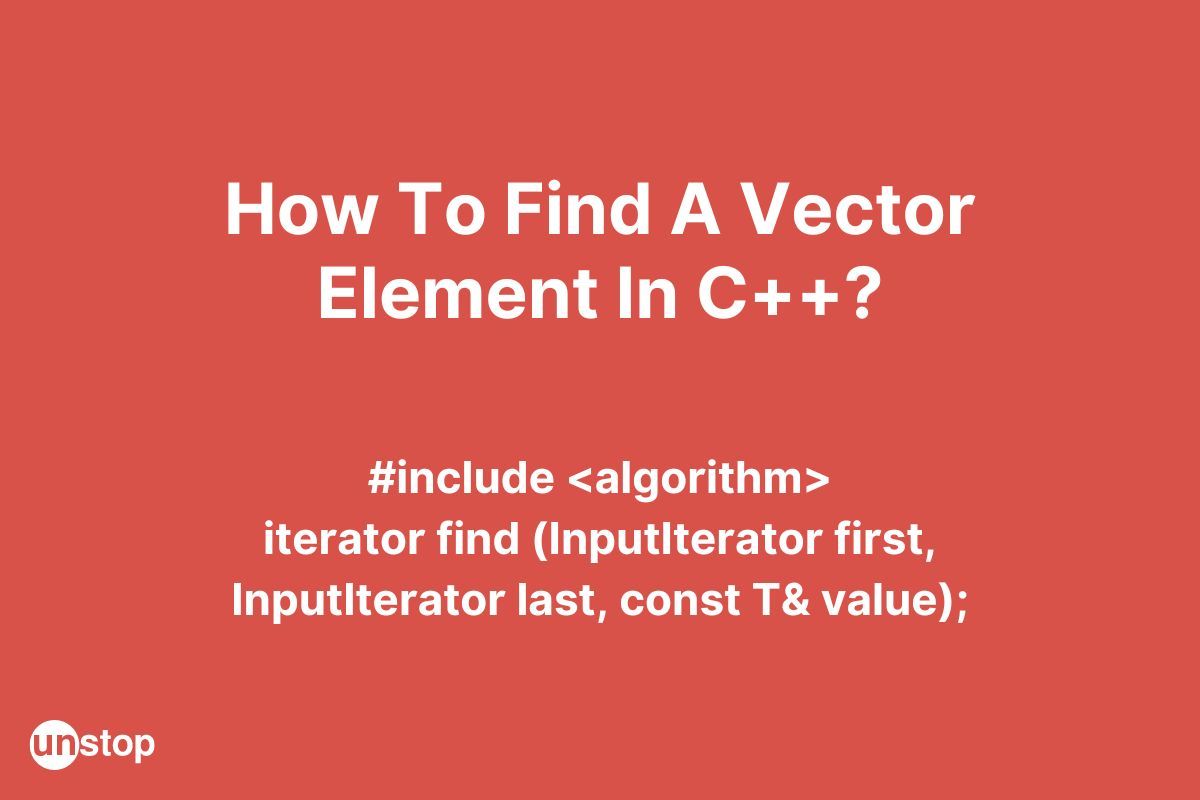
Table of content:
- Definition Of C++ Find In Vector
- Using The std::find() Function
- How Does find() In Vector C++ Function Work?
- Finding An Element By Custom Comparator Using std::find_if() Function
- Use std::find_if() With std::distance()
- Element Find In Vector C++ Using For Loop
- Using The find_if_not Function
- Find Elements With The Linear Search Approach
- Conclusion
- Frequently Asked Questions
Vectors are necessary in C++ for effective data storage and retrieval. With built-in features and automated memory management, they offer a versatile substitute for arrays. The find() function from the C++ Standard Template Library (STL) is frequently used to locate entries within a vector. It provides an iterator pointing to the first instance after receiving the value and search parameters. Finding and using vector data is made easier as a result. Let us get into further depth about find in vector C++.
Definition Of C++ Find In Vector
The find function offered by the C++ Standard Template Library (STL) is used to locate a specific element inside a vector data structure. This is referred to as the C++ find element in the vector.
The vector is a dynamic container that resembles an array and holds a group of elements in a single block of memory. By defining the value to be searched and the search range, programmers may use the find function to look for a specific element inside a vector. It gives back an iterator pointing to the element's first occurrence in the vector, making it simple to retrieve and manipulate the needed data. This feature makes it easier to effectively search through and retrieve data from a vector in C++ language.
Before we get into details of finding a vector element in C++, here's a heads-up for all aspiring coders:
Enhance your skills and prepare for upcoming coding challenges with our dedicated module!
Using The std::find() Function
The C++ Standard Template Library (STL) has the std::find() function, which is used to seek elements inside a range, such as a vector. You may use it to find a certain value or condition inside the range of elements, and it will return an iterator pointing to the element's first appearance/ initial position.
C++ find() Function Syntax
#include <algorithm>
iterator find (InputIterator first, InputIterator last, const T& value);
Parameters of C++ find() Function:
- The Input iterators (first and last), as shown in the syntax above, set the bounds of the search.
- Find is the keyword, which represents the function that will look for the desired element between the first (inclusive) and the last (exclusive) iterator.
- Value: Within the range you wish to locate this value or condition.
Return Value of C++ find() Function:
- The function gives back an iterator that points to the element's first occurrence in the range.
- The function returns the final iterator, which denotes the range's end if the element is not found.
Exceptions of C++ find() Function:
There are no exceptions thrown by the C++ std::find() method. It promises that it will run without ever generating any functionality-related exceptions. As a result, when using std::find(), no special exceptions need to be handled.
- It's crucial to remember that the function presumes the range given by the iterators is accurate.
- Undefined behavior may occur if the iterators supplied are invalid or outside the container's bounds.
- It is up to the programmer to make that the iterators supplied to std::find() are accurate and fall within the right bounds.
In general, it is recommended to handle any exceptions related to memory allocation or other external circumstances that could arise outside the scope of the algorithm itself when utilizing algorithms from the C++ Standard Template Library (STL).
How Does find() In Vector C++ Function Work?
The working mechanism of the find() in vector C++ function is as follows:
- The search is initiated by the first iterator, and the std::find() method iterates across the range until it reaches the last iterator.
- The function applies the == operator or, if a custom predicate is used, the provided predicate to each element in the range before comparing it to the given value.
- If a match is discovered, the method returns an iterator pointing to the element's first appearance.
- The method reaches the final iterator and the end of the range (last) if no match is found.
Here is a sample program that uses std::find and shows its implementation to find an element in a vector in C++:
Code:
Output:
Element found at index: 2
Explanation:
- We begin by including the iostream, vector, and algorithm header files from the Standard Library.
- The program then declares and initializes a vector using the std::vector. The constituents of our vector number are 10, 20, 30, 40, and 50.
- The vector is searched for the number 30 using the std::find() function.
- The numbers.begin() is the first iterator's setting, which points to the start of the vector, and numbers.end() is set as the final iterator (pointing to the vector's final element).
- Each element in the range is first compared to the value 30 by the function before iterating over the elements.
- It finds a match when it gets to the third element (30) and produces an iterator pointing to it.
- We determine if the iterator that was returned is equal to see if the element was located, using number.end().
- Since the element has been located, we use std::distance() to get its index by deducting the starting iterator from the one that was located.
- The element 30 was located at index 2 within the vector, as shown by the output of index 2 at the end.
Complexity Analysis:
The size of the range (the number of elements between the first and last) determines the temporal complexity of std::find(), which is O(n). Until it locates the element or reaches the end, the function does a linear search over the range. Due to the function only requiring a little bit of extra memory to hold the iterators, the space complexity is O(1).
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
Finding An Element By Custom Comparator Using std::find_if() Function
There might be instances where you might need to locate an element that complies with a requirement specified by a custom comparator while dealing with containers in C++. This job may be done quickly using the std::find_if() function from the C++ Standard Template Library (STL). You may provide the comparison logic and look for components that match the required condition by offering a custom predicate or lambda function.
Syntax:
Iterator find_if (Iterator initial, Iterator final, UnaryPredicate pred);
Parameters:
- Initial and final: These are the iterators that specify the search window. The function will look for an element that satisfies the predicate's criterion.
- pred: The comparison logic is defined by a lambda function or a unary predicate. A Boolean value indicating whether the element fulfills the required condition is returned after taking just one input.
Return Value:
- The first element in the range for which the predicate returns true is indicated by the iterator returned by the std::find_if() method.
- The method returns the last iterator if no element meeting the requirement is found.
Exceptions:
There are no exceptions thrown by std::find_if() on its own. However, an exception may propagate if the predicate that was supplied to it throws one.
Code Example:
Output:
Element found: 4
Code Explanation:
- The operator() method, which takes an int input and returns a Boolean indicating if the element is even, is defined as a part of the CustomComparator struct.
- We have a vector of numbers with some integer members in the main() method. To identify the first even number in the vector, we use std::find_if().
- We transfer the digits from numbers to create an instance of CustomComparator to serve as the predicate function and use begin () and end() as the range iterators.
- The operator() method of CustomComparator is called to check each element as the function does a linear search over the range.
- The method returns an iterator pointing to that element and assigns it to it after an even number has been detected.
- To see if it matches, number.end() is used to check if an element matching the criterion was located.
- We output a matching element using *it, if one is discovered. If no element matching the requirement is discovered, the proper message is displayed.
Complexity Analysis:
The size of the range (the number of elements between the first and last) determines the temporal complexity of std::find_if(), which is O(n). Because it just requires a straightforward modulo operation, the custom comparison logic provided in CustomComparator has an O(1) time complexity. The generic function only needs a fixed amount of extra memory to hold the iterators and the predicate object (CustomComparator).
Therefore, the space complexity is O(1). Overall, the code efficiently finds an element using a unique comparator and std::find_if() with a low time complexity and little space cost.
Use std::find_if() With std::distance()
The C++ STL Library provides the std::find_if() method, which enables you to locate the first element in a range that satisfies a certain condition. The utility method std::distance() from the iterator> header determines the separation between two input iterators in a range. You can not only discover the element matching the condition but also figure out its index or location in the container by using the basic functions std::find_if() and std::distance() present in the C++ Library.
Syntax:
#include <algorithm>
#include <iterator>
Iterator find_if (Iterator first, Iterator last, UnaryPredicate pred);
Code Example:
Output:
Element found at index: 2
Code Explanation:
- We begin by declaring a function called isEven(), which uses the modulo operation to determine whether an element is even.
- Next, we create a vector of integers called numbers which is a collection of integers.
- We use the std::find_if() function to find the element in vector numbers. For this, begin() and end() act as the range iterator, isEven is the unary predicate function.
- It looks for the first even integer in the vector, and the iterator points to an element if one is discovered that satisfies the criterion.
- By supplying integers to the std::distance() function, we get the index of the detected element, using it and begin() as an argument list.
- This determines the separation between the starting iterator and the discovered iterator, giving us the index.
- Finally, we use std::cout to report the index of the discovered element.
Complexity Analysis:
The size of the range (the number of elements between the first and last) determines the temporal complexity of std::find_if(), which is O(n). Until it discovers an element meeting the criterion or reaches the end, it does a linear search through the range.
Since std::distance() only subtracts the addresses of two iterators, its temporal complexity is O(1). Due to the code's continual need for extra memory for variables and function calls, its space complexity is O(1).
Element Find In Vector C++ Using For Loop
A for loop is frequently used to cycle through each member of the vector and determine if it satisfies the required value or condition while looking for a certain element in a vector. With this approach, you may personally manage the search procedure and carry out extra tasks as necessary. Given below is an example to help you understand how this works.
Code Example:
Output:
No output as it depends on the further operations performed within the loop.
Explanation:
- The integers 10, 20, 30, 40, and 50 are included in a vector we define as numbers.
- The value we're looking for within the vector is represented by the integer variable target, which in this case, is the number 30.
- We use a for loop to cycle through each member in the vector. Note that index 0 to numbers are included in the loop size_t.
- Using the equality operator == within the loop, we compare each member of numbers[i] with the goal value.
- If a match is discovered, more actions can be carried out inside the relevant if block.
- In this example, we use the break statement to exit the loop, but you can also decide to do so if it's necessary.
- The program moves on to the next sentence if the loop ends without finding a matching element.
Complexity Analysis:
This method has an O(n) time complexity, where n is the length of the vector. The loop linearly iterates through each vector element, looking for a match each time. The lack of further memory allocation results in a space complexity of O(1). For the loop variable and the variables used in comparisons and actions inside the loop, a fixed amount of space is used.
Using The find_if_not Function
The std::find_if_not() function is also part of the C++ Standard Template Library (STL) and is used to search for the first element in a range that does not satisfy a given condition. It is the complement of the std::find_if() function.
Syntax:
#include <algorithm>
Iterator find_if_not (Iterator first, Iterator last, UnaryPredicate pred);
Parameters:
- first and last: These iterators specify the search space to be used. The function will look for an element that does not meet the predicate's criterion.
- pred: The represents the lambda/ unary/ predicate function that specifies the condition to be checked. For the components that ought to be omitted from the search, the predicate ought to return true.
Return Value:
- An iterator pointing to the first element in the range that does not match the criteria is returned by the method.
- The function returns the final iterator if all elements in the range meet the requirement.
Exceptions:
There are no exceptions that the std::find_if_not() method can throw.
Working Mechanism:
- The search is started with the first iterator and continues across the range until it reaches the final iterator using the std::find_if_not() method.
- The function applies the supplied predicate to each element in the range to determine whether or not that element satisfies the condition.
- The method returns an iterator pointing to the element if one is discovered that does not match the criteria.
- The function reaches the end of the range (last) and returns the last iterator if every element in the range satisfies the criteria.
Code Example:
Output:
Element found: 1
Code Explanation:
- We begin with defining a function called isEven which checks if the number is even or note. If the supplied element is even, the method isEven() returns true.
- Then we create an integer-containing vector called numbers.
- Next, apply the std::find_if_not() function on numbers, with begin() and end() as range iterators and isEven as the predicate function.
- The function looks for the first odd (i.e., element that does not meet the requirement of being even) element in the vector.
- The iterator points to an element if one is discovered that satisfies the criterion.
- Using *it, we print the discovered element.
- Numbers are returned by the function if all items meet the requirement. Also, end() is used to show that no element was discovered that does not meet the requirement.
Complexity Analysis:
The size of the range (the number of elements between the first and last) determines the temporal complexity of the std::find_if_not() function, which is O(n). Due to the function only requiring a little bit of extra memory to hold the iterators, the space complexity is O(1).
Find Elements With The Linear Search Approach
Finding an element in a container, like an array or vector, is easy and simple when using the linear search methodology. It entails repeatedly going over each element in turn and comparing it to the target value until a match is made or the container's end is reached.
Syntax:
template <typename T>
int linearSearch(const std::vector<T>& container, const T& value);
Parameters:
- container: This refers to the structure in which the search will be carried out (for example, an array or a vector).
- value: Represents the value that we are looking for within the container.
Return Value:
- If the element is discovered and it is present in the container, the method returns the element's index.
- The function returns -1 or another predefined value to show that the element was not found if it cannot find the element.
Code Example:
Output:
Element found at index: 2
Code Explanation:
- With a vector container and a value as input, we define the function linearSearch().
- Using a for loop, the method iterates through the container's elements from index 0 to container.size() - 1.
- It uses the == operator for each element to determine whether the current element matches the intended value.
- The method instantly returns the index of the element if a match is discovered.
- The method returns -1 to indicate that the element was not found if no match is discovered after iterating over all the items.
- We define a vector of numbers and a goal value of 30 in the main() method. We use the numbers vector and the goal value as inputs when using the linearSearch() method, and we save the outcome in the index variable.
- To find out if the element was discovered, we then check to see if the index is not equal to -1.
- If the element is discovered, we report the index where it was discovered.
- We output a message saying that the element was not found if it is not found.
Complexity Analysis:
When utilizing the linearSearch() function, we pass the numbers vector and the target value as inputs, and we store the results in the index variable. We next check to see whether the index is not equal to -1 to determine if the element was detected. We report the index where the element was found if it is found. If the element is not located, a message stating that it was not found is produced.
Conclusion
In C++, it's crucial to know how to find items in a vector. The find function is an indispensable tool for navigating vectors in C++. With its ability to search efficiently for elements within a specified range, it simplifies the task of locating data. By utilizing the find in vector C++ correctly, programmers can efficiently manage and manipulate collections, making it easier to write efficient and concise code.
Also read- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Frequently Asked Questions
Q. What does find () in C++ return?
The return value of the C++ method find() is an iterator pointing to the first instance of the provided element in the container, which might be a string, array, or entire vector. It returns an iterator pointing to the container's end if the element cannot be located.
Q. What does find () return if not found in C++?
If the find() in C++ vectors does not find the specified element in the container, it returns an iterator pointing to the end of the container. The end iterator represents the position one past the last element in the container, indicating that the element was not found.
Q. Can you use == on vectors C++?
The == operator in C++ may be used to check whether two vectors are equal. The vector items are compared using the == operator to see if they have the same values and the same order. The element comparison will return true if every element in both vectors is identical and is in the same order. It will return false otherwise.
Here is an illustration showing how to use C++'s == operator with vectors:
Output:
vec1 and vec2 are equal.
vec1 and vec3 are not equal.
Explanation:
- The comparison vec1 == vec2 evaluates to true in the example above because vec1 and vec2 have the same components in the same order.
- The comparison vec1 == vec3 evaluates to false because even though vec1 and vec3 have the same components, they are in a different order.
Q. How do you prove a vector is a subset of another vector?
In C++, you may loop over the members of the alleged subset vector and determine whether each element is present in the bigger vector. This will demonstrate that the two vectors are subsets of one another.
Here's a code example to showcase the same:
Output:
The subset is a subset of the superset.
Explanation:
- In the above illustration, the arguments for the isSubset() function are the subset and superset vectors.
- Using std::find(), it iterates through each element in the subset vector and determines if it is present in the superset vector.
- The method returns false if any member from the subset is absent from the superset.
- The method returns true if none of the subset's members can be found in the superset.
- We establish a superset vector with the values 1, 2, 3, 4, and 5 and a subset vector with the values 2 and 4 in the main() method.
- To determine whether a subset is a subset of a superset, we utilize the isSubset() method.
- The result indicates that a subset is definitely a subset of a superset since every member of the subset vector can be found in the superset vector.
Q. How do I find a character in a string in CPP?
The find() method from the string package can be used to locate a character in a string in C++. Given below is an illustration of the same:
Code:
Output:
Character found at position: 4
Explanation:
- In the illustration above, we initialize a string called str with the text "Hello, World!" and a char variable with the letter 'o'.
- On the string, we apply the find() method, supplying the character as a template parameter.
- The point where the character is found is returned by the find() method after looking for the character's first appearance in the string.
- Std::string::npos is returned if the character cannot be located.
- We determine if the returned position (found), which denotes that the character was discovered, is not equal to std::string::npos.
- If it is located, we output the exact location.
- If the character isn't detected, we produce a message to that effect.
This brings us to the end of our discussion on the find in vector C++ functions and their uses. You might also be interested in reading the following:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment