Function Pointers In C | A Comprehensive Guide With Code Examples
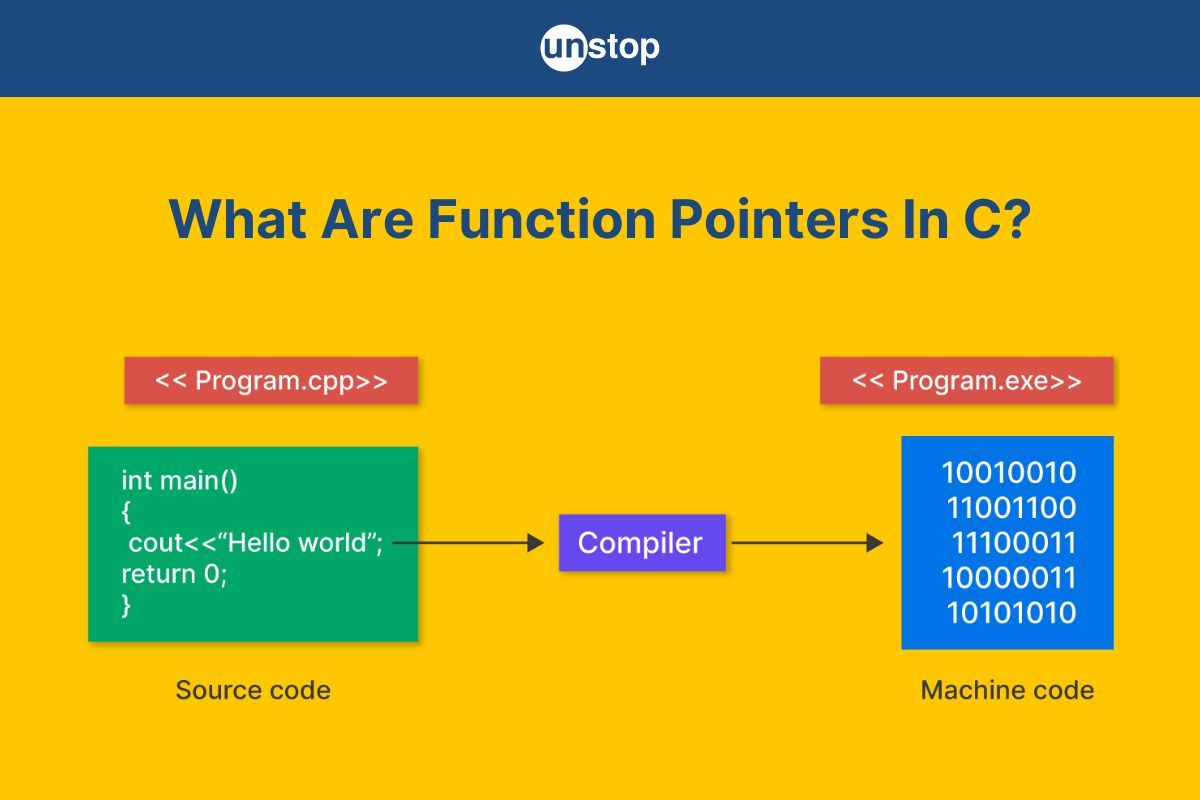
Function pointers in C programming language are a powerful feature that allows us to store the address of a function in a pointer variable. Function pointers are essential in various programming scenarios, such as implementing callback functions, designing state machines, and optimizing performance-critical applications.
In this article, we will explore the concept of function pointers in C, understand their syntax, and examine practical code examples to demonstrate their utility in real-world programming scenarios.
What Is Function Pointer In C?
A function pointer in C is a pointer variable that stores the address of a function, or that points to an executable code instead of just data. One of the primary purposes of this pointer is to invoke a function indirectly instead of calling a function directly by its name, enabling runtime decision-making about which function to execute.
- We can also use them to pass functions as arguments to other functions, store functions in arrays, and create dynamic function call mechanisms.
- In this way, function pointers in C allow for dynamic function calls and greater flexibility in code execution.
For example, consider a sorting algorithm that can sort integers, floats, or custom data types. By using function pointers, we can pass different comparison functions to the sorting algorithm without changing its implementation. This makes our code more modular and reusable.
Declaration Of Function Pointers In C
To declare a function pointer in C, we specify the function's return type, the pointer's name, and the types of the function's parameters
Function Pointer Syntax:
return_type (*pointer_name)(parameter_types);
Here,
- return_type: It represents the data type that the function returns.
- *pointer_name: It is the identifier or name of the function pointer. The asterisk (*) symbol indicates that the variable is a pointer.
- parameter_types: They are the data types of parameters that the function accepts. Note that there can be more than one parameter separated by a comma.
Initialization Of Function Pointers In C
The process of initialization of a function pointer in C entails assigning the address of the respective function to the pointer variable. For this, we use the address of operator followed by the function name. Proper initialization ensures the function pointer references a valid function, preventing undefined behavior or runtime errors.
Syntax:
return_type (*pointer_name)(parameter_types) = &function_name;
Here,
- return_type: It refers to the data type that the function returns.
- *pointer_name: It is the name of the function pointer.
- parameter_types: It represents the types of parameters that the function accepts.
- function_name: It is the name of the function whose address is assigned to the pointer.
Code:
Output:
Result: 7
Explanation:
In the above code example,
- We start by including the necessary standard input-output header file.
- Next, we define a function named add() that takes two integers as parameters and parameters, calculates their sum using the arithmetic addition operator, and returns the same.
- Inside the main function, we declare a function pointer named func_ptr. It will point to a function taking two integers and return an integer.
- Then, we initialize the function pointer func_ptr to point to the add function.
- Next, we use the function pointer to call the add() function with the arguments 3 and 4.
- We store the outcome of the operation in the variable result and use printf() statement to display the same with a formatted string.
- Here, the %d format specifier is the placeholder for an integer value, and the newline escape sequence (\n) shifts the cursor to the next line.
- Finally, the main function returns 0, indicating that the program executed successfully.
Calling A Function Through A Function Pointer In C
As you must have noticed in the example above, we can use function pointers in C programs to call functions indirectly. Calling a function through a function pointer is a two-step process. The first step is to assign the address of the desired function to the function pointer. The next step entails invoking the function through the pointer.
Syntax:
(*pointer_name)(arguments);
Here,
- *pointer_name: This is used to dereference the function pointer to access the function it points to.
- arguments: These are the arguments to be passed to the function, matching the function's parameter list.
Code:
Output:
Result: 30
Explanation:
In the above code example,
- We begin by defining a function named multiply() that takes two integers as parameters and returns their product.
- Inside the main function, we declare a function pointer named func_ptr that can point to a function taking two integers and returning an integer.
- We then initialize the function pointer func_ptr to point to the multiply function.
- Next, we call the multiply() function through the function pointer, passing in the arguments 5 and 6.
- We then store the result of this function call in the variable result and use printf to output it to the console.
- Finally, the main function returns 0 to indicate that the program is executed successfully.
Referencing and Dereferencing Of Function Pointer In C
Referencing a function pointer involves obtaining the address of a function and storing it in a function pointer. This allows you to call the function indirectly through the pointer.
On the other hand, dereferencing a function pointer involves using the pointer to call the function it points to. Unlike regular pointers, dereferencing a function pointer is done by simply using the pointer as if it were the function name to call the respective function with a set of arguments.
Syntax for referencing a function pointer in C:
return_type (*pointer_name)(parameter_types);
pointer_name = &function_name; // Referencing
Here,
- return_type: It is the return type of the function.
- pointer_name: It is the name of the function pointer. The asterisk (*) indicates that this variable is a pointer.
- parameter_types: It represents the types of parameters that the function takes.
- function_name: It refers to the name of the function whose address is being referenced. The address-of operator (&) operator is used to get the function's address.
Syntax for dereferencing a function pointer in C:
pointer_name(arguments); // Dereferencing and calling the function
Here, pointer_name refers to the identifier or name of the function pointer, and arguments are the parameters that we pass to the function.
Code:
Output:
Hello!
Goodbye!
Explanation:
In the above code example,
- We start by defining a function named greet() that prints "Hello!" to the console.
- We define another function named farewell() that prints "Goodbye!" to the console.
- In the main function, we declare two function pointers: func_ptr_greet and func_ptr_farewell, both of which point to functions that do not take any argument and do not return any value, i.e., are void functions.
- Next, we then initialize func_ptr_greet to point to the greet() function using &greet.
- Then, initialize func_ptr_farewell to point to the farewell() function using &farewell.
- Next, we call the greet() function through the func_ptr_greet pointer and call the farewell() function through the func_ptr_farewell pointer.
- These calls invoke the respective function printing messages, Hello and Goodbye to the console.
Array Of Function Pointers In C
An array of function pointers is essentially a collection of pointers where each pointer holds the address of a function. Each element in the array can point to a different function, enabling you to choose which function to call based on an index or other decision criteria.
Here is an example demonstrating the use of an array of function pointers to perform different arithmetic operations.
Code:
Output:
Select an operation:
0: Add
1: Subtract
2: Multiply
3: Divide
Enter your choice (0-3): 2
Result: 200
Explanation:
In the above code example,
- We define four functions: add() for addition, subtract() for subtraction, multiply() for multiplication, and divide() for division.
- Here, the divide function uses an if-statement to check if the denominator is equalt to zero (relational operator), before carrying the division operation out.
- Inside the main function, we declare an array of function pointers named operations, which can point to functions taking two integers and returning an integer.
- Then, we initialize this array with the addresses of our four arithmetic functions.
- Next, we define two integer variables, a and b, and initialize them to 20 and 10, respectively.
- We also declare an integer variable named choice to store the user's menu selection.
- Next, we use printf() function to display 4 menu options for the user, i.e., the available arithmetic operations.
- Then, we prompt the user to select an option between 0 to 3, and read the input using the scanf() function, storing it in the choice variable.
- After that, we check if the user's choice is valid,i.e., between 0 and 3 using the condition if (choice < 0 || choice > 3):
- If the condition is true, i.e., the choice is invalid, we print an error message and return 1 to indicate an error.
- If the condition is false, we move to the next line in the code.
- Next, we call the function corresponding to the user's choice from the operations array and pass a and b as arguments. We store the result of this function call in the variable result.
- We then use printf() to display the result of the selected operation.
- Finally, the main function returns 0 to indicate that the program executed successfully.
Passing A Function Pointer In C As Function Argument
When we pass a function pointer in a function, we are essentially passing another function's address as an argument. This means we are providing the address where the function is located in memory so that the receiving function can call it.
Below are the steps involved in passing a function pointer in C as an argument to a function:
- Define the Function: First, create the function you want to pass as an argument.
- Declare the Function with a Function Pointer Parameter: Next, define a function that takes a function pointer as a parameter. Note that the function signature for the receiving and argument functions must be the same.
- Call the Function with the Function Address: Now, call the function, passing the address (i.e., function pointer) of the function you want to use.
Syntax:
void function_name(void (*func_ptr)(parameters)) {
// Function body
}
Here,
- void: It is the return type of function_name; it doesn’t return a value.
- function_name: It is the name of the function that will receive the function pointer as an argument.
- void (*func_ptr): It is the function pointer with the name func_ptr, which will hold the address of a function with a void return type.
- parameters: It specifies the list of parameters the function pointed to by func_ptr accepts.
Code:
Output:
Sum: 15
Explanation:
In the above code example,
- We start by defining a function named printSum() that takes two integers as parameters and prints their sum.
- We then define another function named performOperation() that takes a function pointer and two integers as parameters.
- Inside this function, we use the function pointer to call the underlying function with the two integers as its arguments.
- Note that the function pointer must point to a function that also takes two integers as parameters and returns nothing.
- In the main function, we call performOperation() function, passing the pointer to printSum() function and integer values 5 and 10 as arguments.
- Here, we do not need to use the pointer notation (*) because the function name itself refers to the address of the function.
- As a result of this call, the performOperation() uses the function pointer to indirectly invoke the printSum() function with the int values and prints the sum of 5 and 10.
Wild Function Pointers In C
A function pointer is referred to as wild when it has been declared but not initialized. Much like wild data pointers, a wild function pointer can point to an undefined or invalid function address, which can cause your program to crash or behave incorrectly if used.
The syntax for the creation of a wild function pointer is the same as for any normal function pointer in C. The only point to note is that it is not initialized with the address of any function.
Syntax:
return_type (*function_pointer_name)(parameter_types);
Let’s look at an example to understand the creation of a wild function pointer in C programs.
Code:
Output:
Function pointer is wild!
Explanation:
In the above code example,
- We begin by declaring a function named sayHello() that prints "Hello, World!" to the console.
- In the main function, we declare a function pointer named funcPtr pointing to functions that do not take any arguments and do not return a value (void functions).
- Here, we have declared the function pointer, but it is uninitialized, i.e., it does not store any specific function's address.
- Next, we have a code comment, where we use funcPtr to call the function it is pointing to, i.e., funcPtr(). If we uncomment the line, it will result in undefined behavior since funcPtr is not initialized (wild).
- Hence, instead of calling funcPtr, we print the string message "Function pointer is wild!" to indicate that funcPtr is currently not pointing to a valid function.
- Finally, the program terminates with a return 0.
Advantages Of Function Pointers In C
Function pointers in the C programming language offer several benefits that contribute to the effectiveness and flexibility of software development. Some of these are:
- Dynamic Function Calls: Function pointers in C allow you to select which function to call at runtime rather than at compile time. This flexibility means you can decide which function to invoke based on conditions or user input, making your programs more dynamic and adaptable to different scenarios.
- Callback Functions: Function pointers in C enable callback mechanisms, where one function can call another function in response to certain events or conditions. This is particularly useful for designing event-driven programs, such as user interfaces or asynchronous operations, where you need to execute specific actions at different points in your code.
- Function Tables: By using function pointers in arrays, you can create function tables or dispatch tables that allow you to call different functions based on an index or a value. This approach is beneficial for implementing state machines, command patterns, or any situation where you need to choose between multiple functions based on some criteria.
- Enhanced Code Flexibility: Function pointers in C programs enhance code flexibility by enabling you to pass functions as arguments, return them from other functions, or store them in complex data structures. This flexibility allows you to design more modular and reusable code, where different functions can be used interchangeably.
- Improved Code Maintainability: Using function pointers in C helps you avoid hardcoding specific functions into your code, which makes it easier to modify and extend your programs. By abstracting functionality, you can change which functions are used without altering the core logic of your program.
- Simplified Event Handling: Function pointers in C simplify event handling by allowing you to register different functions to handle specific events. This approach is commonly used in event-driven programming, where you can easily switch out event handlers or add new ones as needed.
- Efficient Memory Usage: Function pointers can reduce the need for large switch-case or if-else constructs, which can be memory-intensive and difficult to manage. By using function pointers, you can streamline your code and reduce memory overhead associated with managing different function calls.
- Support for Advanced Patterns: Function pointers in C support advanced design patterns like Strategy, Command, and Observer. These patterns rely on the ability to pass and manage functions dynamically, enabling more sophisticated and flexible designs for complex applications.
- Customizable Algorithms: You can pass different functions to algorithms as function pointers, which allows you to customize the behavior of the algorithms without modifying their internal logic. This is particularly useful for sorting functions, searching, or other operations where you can specify different comparison or processing functions.
- Creating Plug-Ins or Modules: Function pointers in C are useful for creating plug-ins or modular systems where different components can be added or swapped out. By defining interfaces with function pointers, you can design systems that support plug-ins or modules that can be easily integrated or replaced.
Normal Pointers Vs. Normal Functions Vs. Function Pointers In C
Below is a comparison table that outlines the key differences between normal pointers, normal functions, and function pointers in C.
Type | Syntax | Purpose | Example |
---|---|---|---|
Normal Pointer | int *ptr; | Holds the address of a variable, enabling indirect access and manipulation of the variable's value. | int a = 10; int *ptr = &a; |
Normal Function | int add(int a, int b); | Defines a block of code that performs a specific task, which can be executed by calling the function by name. | int result = add(3, 4); |
Function Pointer | int (*func_ptr)(int, int); | Holds the address of a function, allowing for dynamic function calls and enabling functions to be passed as arguments or stored in arrays. | func_ptr = &add; int result = func_ptr(3, 4); |
Functions Using Void Pointers
A void* (void pointer) is a generic pointer type that can point to any data type. That is, it does not have a type associated with it, so we can use it to refer to data of any type. However, we must cast it to the appropriate type before dereferencing it.
Void pointers are particularly useful in functions where the type of data is not known in advance. For example, functions like qsort in the C standard library use void pointers to achieve generic sorting.
Syntax:
void function_name(void *data, size_t size) {
// Function body
}
Here,
- function_name: It is the name of the function being defined.
- void *data: It refers to a pointer variable named data, with a void return type, i.e., it can point to any data type.
- size_t size: It refers to the size of the data type being pointed to, which helps in determining the amount of data.
Code:
Output:
Integer: 42
Double: 3.140000
Char: A
Explanation:
In the above code example,
- We start by defining a function named printData() that takes a void pointer and the size of the pointer's data type as parameters.
- The void pointer allows the function to accept different types of data, and size tells the acceptable size of the data type.
- Inside printData(), we use if-else ladder statements with the sizeof() operator to check the size of the data and determine its type.
- We then use the type casting operator to cast the pointer to the appropriate type pointer and dereference it to print the data.
- If the size matches that of an int, we cast void* to int*, dereference it, and print the integer value.
- If the size matches that of a double, we cast void* to double*, dereference it, and print the double value.
- If the size matches that of a char, we cast void* to char*, dereference it, and print the character value.
- If the size doesn’t match any known types, we print a message indicating an unknown data type.
- In the main function, three variables, i, d, and c, with the values 42 (integer type), 3.14 (double type), and 'A' (character type), respectively.
- Next, we call the printData() function three times with different data types:
- First, we pass the address of i and the size of i to printData to print the integer value.
- Then, we pass the address of d and the size of d to printData to print the double value.
- Finally, we pass the address of c and the size of c to printData to print the character value.
- Then, the main function returns 0 to indicate that the program executed successfully.
Functions Using Pointer Variables
C allows for advanced manipulation of data by using pointers as function arguments and return values. Pointers enable a function to access and modify the actual memory locations of variables, a method known as pass by reference. This technique is crucial for efficient memory management and achieving various programming tasks.
When we pass a pointer to a function, we are passing the address of a variable rather than a copy of its value. This means any changes made to the data through the pointer are reflected back in the original variable.
Code:
Output:
Segmentation fault
Explanation:
- In this code example, the compiler throws a segmentation fault error because we are returning a pointer to a local variable from the increment function.
- The local variable b is de-allocated when the function finishes, so when we try to access it afterward, the compiler cannot reference the pointer correctly, leading to a segmentation fault.
- To fix this issue, we need to properly allocate memory for b and ensure it remains valid after the function ends.
Safe Ways To Return A Pointer From A Function
Returning a pointer from a function in C can be tricky and error-prone if not done carefully. Here are some safe ways to return a pointer from a function:
1. Returning a Pointer to Dynamically Allocated Memory: The dynamic memory allocation is done using malloc, calloc, or realloc and persists until explicitly freed, making it a safe option to return from a function. For example:
#include <stdlib.h>
int* createArray(int size) {
int *arr = (int *)malloc(size * sizeof(int));
if (arr == NULL) return NULL;
// Initialize array elements
return arr;
}
2. Returning a Pointer to Static Variables: Static variables retain their value between direct function calls and exist for the lifetime of the program, making them safe to return from a function. For example:
int* getStaticVariable() {
static int num = 42;
return #
}
3. Returning a Function Argument: If a function receives a pointer as an input argument and returns it unchanged, it can be a safe way to return a pointer, provided the caller ensures the pointer is valid. For example:
int* processArray(int *arr, int size) {
for (int i = 0; i < size; i++) {
arr[i] *= 2;
}
return arr;
}
Conclusion
Function pointers in C programming language allow functions to be treated as data, enabling dynamic function calls, callback mechanisms for event handling, and the creation of function tables for modular code design.
Function pointers also help us implement complex algorithms and customizable behaviors. Overall, mastering function pointers equips us with powerful tools for writing robust, efficient, and scalable numeric codes, unlocking new possibilities for advanced programming techniques.
Frequently Asked Question
Q. What are some common mistakes to avoid when using function pointers in C?
Common mistakes with function pointers include:
- Mismatched Function Signatures/ Prototypes: Ensure the function pointer’s signature matches that of the function it points to.
- Uninitialized Pointers: Always initialize function pointers before using them.
- Incorrect Dereferencing: Use function pointers correctly to avoid calling errors and write accurate codes.
- Memory Management Issues: Be careful with dynamically allocated memory when using function pointers for callbacks.
- Null Function Pointers: Check if a function pointer is NULL before dereferencing it to avoid crashes.
Q. What is the type of a function pointer in C?
Generally, the type of a pointer variable refers to the type of data it is pointing to. In the case of function pointers, then, it specifies what kind of function the pointer can point to.
- This is determined by the function's signature, which includes its return type and the parameter types it takes.
- The function's definition comes first, and the function pointer is then defined to match this signature.
- For example, if the return type of a function is void, then the type of it's function pointer will also be void.
Understanding and using these types correctly is vital in C and C++ programming languages.
Q. What is an array of pointers to functions in C?
An array of pointers to functions also known as an array of function pointers in C is a basic data structure that holds multiple function pointers. Each element in the array points to a different function, allowing you to manage and call a group of functions using a single array. This approach is useful for implementing function dispatch mechanisms, handling events, or creating lookup tables for various operations in C.
Code:
Output:
Hello!
Goodbye!
How are you?
In the above executable code example, the funcArray array holds pointers to the functions. The for loop then iterates over the array, calling each display function in sequence.
Q. What are some common uses for function pointers?
Function pointers are commonly used for:
- Callback Functions: Allow functions to be passed as arguments to be called later.
- Event Handling: Register functions to handle specific events or conditions.
- Function Tables: Create tables of functions for dispatching calls based on indices.
- Dynamic Function Selection: Choose functions to execute based on runtime conditions.
- Implementing Design Patterns: Support patterns like Strategy and Command.
You might also be interested in reading the following:
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment