Table of content:
- What Is Memory Leak In C?
- Causes Of Memory Leak In C
- Types Of Memory Leak In C And C++
- How To Avoid Memory Leak In C?
- Detecting Memory Leaks In C
- Fixing Memory Leaks In C
- Conclusion
- Frequently Asked Questions
Memory Leak In C | Causes, Types, Fixes & More (+Code Examples)
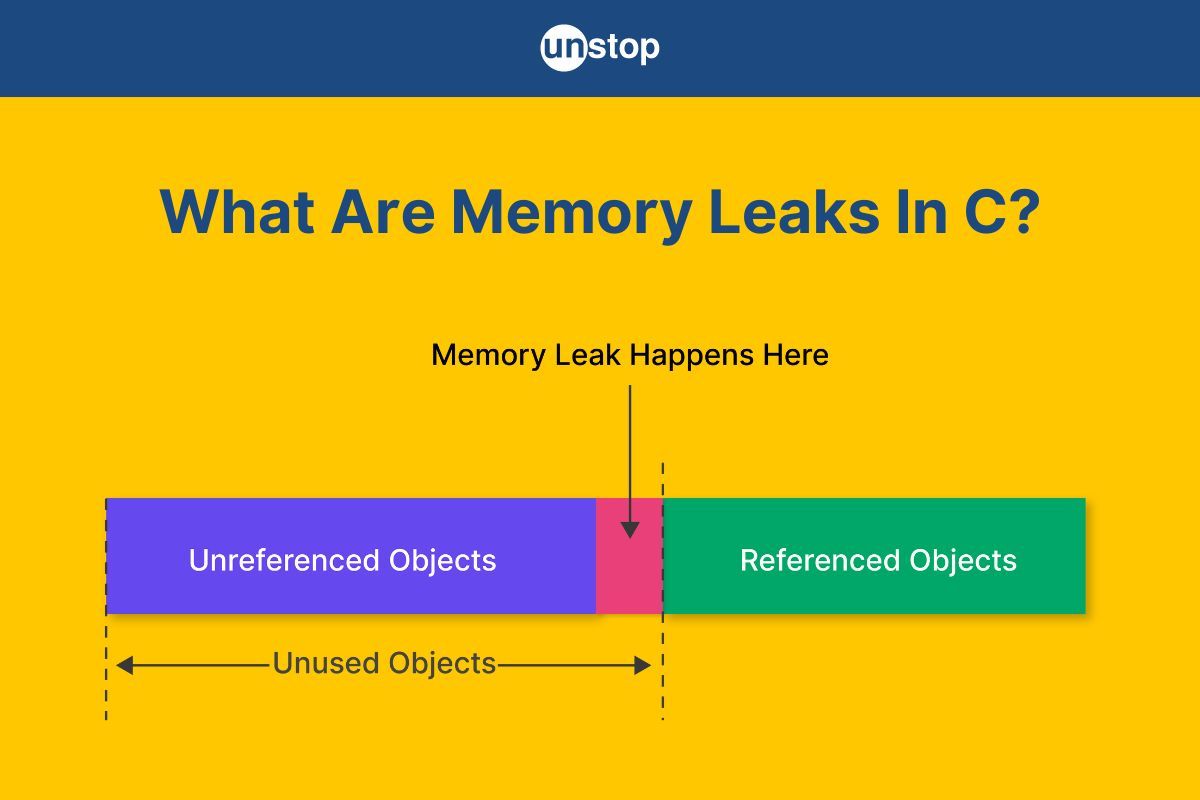
Memory leaks in C programming occur when a program allocates memory dynamically but fails to release it back to the system, leading to inefficient memory usage. This potentially causes the system to run out of memory. These leaks can result in reduced performance, system crashes, and erratic behavior, particularly in long-running applications or those with high memory demands.
Understanding the causes of memory leaks, identifying them during development, and implementing strategies to prevent them are crucial for maintaining the efficiency and stability of C programs. In this article, we will explore the nature of memory leaks in C, examine common pitfalls, and discuss best practices for managing memory effectively.
What Is Memory Leak In C?
A memory leak in C programming occurs when a computer program consumes memory but fails to release it back to the system. Imagine you're working on a desk, and each time you need a piece of paper, you grab one from a drawer. Now, after using the paper, you're expected to put it back into the drawer.
But what happens when you forget to return the paper every time you take one. Over time, your desk accumulates more papers, cluttering the workspace. This is somewhat similar to what happens in programming, specifically in languages like C, when memory leaks occur.
Memory leak happens when the memory that was dynamically allocated using functions like malloc(), calloc(), or realloc() is not properly deallocated using free(). This results in memory remaining occupied even after it is no longer needed by the program.
Why Are Memory Leaks Bad?
Memory leaks are like a silent predator in software development. They might not cause an immediate crash, but their impact becomes evident over time. Here's why they're considered harmful during program execution:
- Resource Depletion: Every time your program runs and memory is leaked, a portion of the system's resources is taken up unnecessarily. If this continues, it can lead to severe resource depletion.
- Performance Issues: As the risk of memory leak in C persists, your program's performance can degrade. It may become slower and less responsive, affecting the user experience.
- System Instability: In extreme cases, continuous memory leaks can lead to system instability or crashes. As a result, the operating system may struggle to allocate the necessary resources, causing unexpected errors.
- Difficulty in Debugging: Identifying the source of a memory leak in C programs can be challenging. The fact that it might not manifest immediately, makes it harder to pinpoint when and where the issue occurred.
Causes Of Memory Leak In C
Memory leak in C can arise from several common programming practices and mistakes. Understanding these causes is essential for preventing and addressing memory leaks effectively. Here are the primary causes of memory leaks in C programs:
- Forgetting to Free Dynamically Allocated Memory: One of the most common causes of memory leak in C is simply forgetting to deallocate memory that was dynamically allocated using malloc(), calloc(), or realloc(). If free() is not called to release the memory, it remains allocated even after it is no longer needed. For example-
void memoryLeakExample() {
int *ptr = (int*)malloc(sizeof(int) * 10); // Memory allocated
// Some operations on ptr
// No free(ptr) here, so memory is not released
}
- Losing Reference to Allocated Memory: Memory leak in C can occur if the reference (pointer) to the allocated memory is overwritten or goes out of scope without freeing the memory. This makes it impossible to release the allocated memory later. For example-
void memoryLeakExample() {
int *ptr = (int*)malloc(sizeof(int) * 10); // Memory allocated
ptr = NULL; // Lost reference to allocated memory, causing a leak
}
- Improper Management of Pointer Reassignments: If a pointer to allocated memory is reassigned without first freeing the memory it points to, the original integer memory block is leaked. For example-
void memoryLeakExample() {
int *ptr = (int*)malloc(sizeof(int) * 10); // Memory allocated
ptr = (int*)malloc(sizeof(int) * 20); // Previous memory not freed, causing a leak
}
- Failure to Free Memory in Error Paths: If a function allocates memory and then encounters an error that causes an early exit, the allocated memory might not be freed, leading to a memory leak. For example-
void memoryLeakExample() {
int *ptr = (int*)malloc(sizeof(int) * 10); // Memory allocated
if (someErrorCondition) {
// Error occurs, no free(ptr), causing a leak
return;
}
free(ptr); // Memory freed only if no error
}
- Incorrect Use of Data Structures: Complex data structures like linked lists, trees, or custom collections can also cause memory leaks if not managed properly. Each node or element must be individually freed. For example-
typedef struct Node {
int data;
struct Node* next;
} Node;void freeList(Node* head) {
Node* temp;
while (head != NULL) {
temp = head;
head = head->next;
free(temp);
}
}
- Memory Leaks in Libraries and APIs: Using third-party libraries or APIs that have memory management issues can also lead to memory leak in C. It’s important to review the documentation and known issues of the libraries you use.
- Unclosed Files: Not closing files opened using fopen() or similar functions can result in resource leaks and may lead to unexpected behavior in your program. Properly closing files ensures that resources are released, preventing potential issues like file corruption and system resource exhaustion. For example-
FILE *file = fopen("example.txt", "r");
// ... processing
// Forgot to close the file
- Dynamic Memory Allocation Inside Loops: Allocating memory inside a loop without freeing it in each iteration can quickly exhaust available memory, causing your program to slow down or crash. It is essential to free the allocated memory within the loop to avoid memory leaks and ensure efficient memory usage. For example-
for (int i = 0; i < 5; ++i) {
int *dynamicVar = (int *)malloc(sizeof(int));
// ... processing
// Memory is not freed in each iteration
}
Types Of Memory Leak In C And C++
Memory leak in C occurs in various forms, each with its distinct characteristics. Let's explore some common types along with code examples:
Dynamic Memory Allocation With malloc() But No Deallocation
Dynamic memory allocation allows programs to request memory at runtime. We frequently use the malloc() function for this purpose, facilitating the creation of variables or arrays with a specified size during runtime. However, if the allocated memory is not appropriately deallocated using free() function when it becomes unnecessary, a memory leak will occur.
Code:
#include <stdio.h>
#include <stdlib.h>
int main() {
// Allocating memory for an integer
int *dynamicVariable = (int *)malloc(sizeof(int));
if (dynamicVariable == NULL) {
// Check if memory allocation is successful
printf("Memory allocation failed!\n");
return 1; // Exit the program with an error code
}
// Print a message to indicate successful memory allocation
printf("Memory allocated successfully!\n");
// No deallocation - Memory leak occurs here
// Print a message to indicate the end of the program
printf("End of the program.\n");
return 0;
}
Output:
Memory allocated successfully!
End of the program.
Explanation:
In the above code example-
- We include the necessary header files, i.e., stdio.h for input and output operations and stdlib.h for memory management functions.
- In the main() function, we use malloc() function to allocate memory for an integer data type variable called dynamicVariable.
- Here, we use the sizeof() operator to calculate the size of the variable and then typecast it to an integer pointer type (int*).
- We then use an if-statement to check if the memory allocation was successful by verifying if dynamicVariable is NULL.
- If it is NULL, it means the allocation failed, so we print an error message and return from the program with an error code of 1.
- If the memory allocation is successful, we print a message indicating that the memory was allocated successfully.
- At this point, we do not deallocate the memory, which leads to a memory leak. This means that the allocated memory is not freed, and it remains allocated even after the program ends.
- Finally, we print a message using printf() to indicate the end of the program.
- The main() function returns 0 to signify that the program executed successfully.
Dynamic Memory Allocation With malloc() & Deallocation With delete()
In C++ programming language, dynamic memory allocation is typically done using new instead of malloc(). The malloc() function is used in C, while new is used in C++ for similar purposes. However, if you allocate memory with malloc() and try to deallocate it using delete, it results in undefined behavior. This mix of memory allocation and deallocation functions from different memory management systems can lead to issues and potential runtime errors.
Code:
#include <iostream>
using namespace std;
int main() {
// Allocating memory for an integer using malloc (C style)
int *dynamicVariable = (int *)malloc(sizeof(int));
if (dynamicVariable == nullptr) {
// Check if memory allocation is successful
cout << "Memory allocation failed!\n";
return 1; // Exit the program with an error code
}
// Print a message to indicate successful memory allocation
cout << "Memory allocated successfully!\n";
// Deallocating memory using delete (C++ style) - Undefined behavior
delete dynamicVariable;
// Print a message to indicate the end of the program
cout << "End of the program.\n";
return 0;
}
Output:
Memory allocated successfully!
End of the program.
Explanation:
In the above C++ code example-
- We start by allocating memory for an integer using the malloc() function, which is a C-style memory allocation.
- Just like before, we cast the result of malloc() to an integer pointer and assign it to dynamicVariable.
- We then check if the memory allocation was successful by verifying if dynamicVariable is nullptr (Null pointer):
- If it is nullptr, it means the allocation failed, so we print an error message indicating that the memory allocation failed and return from the program with an error code of 1.
- If the memory allocation is successful, we print a message indicating that the memory was allocated successfully.
- We then attempt to deallocate the memory using the delete operator, which is a C++-style deallocation. However, this leads to undefined behavior because the memory was allocated using malloc(), which should be paired with free for deallocation.
- Using delete on memory allocated with malloc() can cause unpredictable behavior and potential program crashes.
- Finally, we print a message using cout to indicate the end of the program.
Dynamic Memory Allocation With new & No Deallocation With delete
As mentioned above, in C++, dynamic memory allocation is typically done using the new operator. This operator allocates memory on the heap, and the programmer is responsible for freeing up this memory when it's no longer needed using delete. However, if the allocated memory is not properly deallocated with delete, it results in a memory leak.
Code:
#include <iostream>
int main() {
int *ptr = new int[10]; // Allocate memory for 10 integers
// Using the allocated memory
for (int i = 0; i < 10; i++) {
ptr[i] = i * 2;
}
// Forgot to free the allocated memory
// delete[] ptr;
return 0;
}
Output:
No output is displayed due to undefined behavior
Explanation:
In the above code example-
- We allocate memory for an array of 10 integers using the new operator. This allocation request creates space for 10 integers on the heap, and the address of this memory is stored in ptr.
- Next, we use the allocated memory to initialize the array. We use a for loop to iterate through the array indices from 0 to 9 and set each element at index i to i * 2. This results in the array holding values: 0, 2, 4, 6, 8, 10, 12, 14, 16, and 18.
- However, we neglect to deallocate the memory we previously allocated. In C++, when memory is allocated with new, it must be deallocated with delete[] to release the memory back to the system properly.
- Without this deallocation, we have a memory leak, as the allocated memory is not returned to the system even after the program ends.
- Finally, the program completes and returns 0, indicating successful execution.
Dynamic Memory Allocation With new[] & Deallocation With delete[]
To free up dynamic memory allocated to an array we cannot directly use the delete operator. Using new[] for dynamic memory allocation and delete[] for deallocation is crucial to ensure proper memory management, especially when dealing with arrays.
Using delete instead of delete[] to deallocate memory allocated with new[] can lead to undefined behavior, as it may not correctly release the memory allocated for the entire array. To prevent memory leaks and guarantee the integrity of the memory management system, it is crucial to utilize the right pair of operators.
Code:
#include <iostream>
using namespace std;
int main() {
// Allocating memory for an array of integers using new[] (C++ style)
int *dynamicArray = new int[5];
// Initialize the array with some values (for demonstration purposes)
for (int i = 0; i < 5; ++i) {
dynamicArray[i] = i * 2;
}
for (int i = 0; i < 5; ++i) {
cout<<dynamicArray[i]<<" ";
}
cout<<endl;
// Deallocating memory using delete[]
delete[] dynamicArray;
// Print a message to indicate the end of the program
cout << "End of the program.\n";
return 0;
}
Output:
0 2 4 6 8
End of the program.
Explanation:
In the above code example-
- We first allocate memory for an array of 5 integers using the new[] operator. This creates space for 5 integers on the heap, and we store the pointer to this allocated memory in dynamicArray.
- Then, we initialize the array with values for demonstration purposes. We use a for loop to iterate through each index of the array from 0 to 4, setting each element at index i to i * 2. This results in the array holding the values 0, 2, 4, 6, and 8.
- Next, we print the values stored in the array. Here, we use another for loop to iterate over the array and output each value followed by a space.
- After printing all the values, we use cout and endl to move to the next line for better readability.
- Once we are done using the allocated memory, we properly deallocate it using the delete[] operator.
- This releases the memory that was allocated with new[], ensuring that there is no memory leak and that the system resources are properly returned.
- Finally, we print a message indicating the end of the program.
Dynamic Memory Allocation With new & Deallocation With free()
Using free() to deallocate memory allocated with new is incorrect. The new and delete operators should be used together, while malloc() and free() should be used together. Mixing them leads to undefined behavior and potential memory leaks.
Code:
#include <iostream>
#include <cstdlib>
int main() {
int *ptr = new int[10]; // Allocate memory for 10 integers
// Using the allocated memory
for (int i = 0; i < 10; i++) {
ptr[i] = i * 2;
}
// Incorrectly deallocate with free
free(ptr);
return 0;
}
Output:
No output is displayed due to undefined behavior
Explanation:
In the above code example-
- We start by allocating memory for an array of 10 integers using the new operator, which is a C++-style memory allocation. We assign the address of this allocated memory to ptr.
- Then, we use the allocated memory by initializing the array. Here, we use a for loop to iterate through the array, setting each element at index i to i * 2.
- This means that the array will hold the values 0, 2, 4, 6, 8, 10, 12, 14, 16, and 18 repsectively.
- After using the allocated memory, we attempt to deallocate it using the free() function, which is a C-style deallocation.
- However, this is incorrect because the memory was allocated using the new operator and should be deallocated using the delete operator. Using free() on memory allocated with new can cause undefined behavior and potential program crashes.
- Finally, the main() function returns 0 indicating that the program executed successfully. However, due to the incorrect deallocation, this code may lead to issues during runtime.
How To Avoid Memory Leak In C?
To avoid memory leaks in C, you need to follow good practices for memory management. Here are some guidelines and best practices:
Every malloc Or calloc Should Have A free() Function To Avoid Memory Leaks In C
The malloc() and calloc() are functions used in C to dynamically allocate memory from the heap. When using these functions it is crucial to ensure that you free that memory when it's no longer needed. Failing to do so can result in memory leaks, where the allocated memory is not released, leading to inefficient memory usage.
The free() function is used to deallocate memory that was previously allocated using malloc, calloc, or related functions. It takes a pointer to the beginning of the dynamically allocated memory block as its argument.
Code:
#include <stdlib.h>
#include <stdio.h>
void allocateMemory() {
int *ptr = (int*)malloc(10 * sizeof(int)); // Allocate memory
if (ptr == NULL) {
printf("Memory allocation failed\n");
return;
}
// Use the allocated memory
for (int i = 0; i < 10; i++) {
ptr[i] = i * 2;
}
for (int i = 0; i < 10; i++) {
printf("%d ", ptr[i]);
}
printf("\n");
free(ptr); // Free the allocated memory
}
int main() {
allocateMemory();
return 0;
}
Output:
0 2 4 6 8 10 12 14 16 18
Explanation:
In the above code example-
- We start by including the standard libraries stdlib.h and stdio.h, which provide functions for memory management and input/output operations, respectively.
- Then we define a function allocateMemory(), inside which we allocate memory for an array of 10 integers using the malloc function.
- Here, we also cast the returned pointer from malloc() to an int* type.
- If the memory allocation fails, indicated by a NULL pointer, we print an error message and exit the function.
- If the memory allocation is successful, we use the allocated memory by initializing each element of the array with a value.
- Specifically, we use a for loop to set each element ptr[i] to i * 2, where the counter variable i ranges from 0 to 9.
- After initializing the array, we use another for loop to print out the values stored in the array. We print each value followed by a space, and after the loop, we print a newline to separate the output from any subsequent text.
- Once we are done using the allocated memory, we free it using the free() function to release the memory back to the system.
- This is crucial to avoid memory leaks, which occur when allocated memory is not properly freed.
- In the main() function, we simply call the allocateMemory() function to perform the allocation, initialization, output, and deallocation of memory.
Avoid Memory Leaks In C By Not Orphaning Memory Location
Orphaning memory refers to a situation that occurs when the track of pointers that points to dynamically allocated memory is lost or goes out of scope without freeing the allocated memory. As a result, the program loses the reference to the allocated memory, making it impossible to free and causing a memory leak. To avoid orphaning memory, it's essential to ensure that dynamically allocated memory is properly managed and freed when it's no longer needed.
Code:
#include <stdlib.h>
#include <stdio.h>
void avoidOrphaningMemory() {
int *ptr = (int*)malloc(10 * sizeof(int)); // Allocate memory
if (ptr == NULL) {
printf("Memory allocation failed\n");
return;
}
// Simulate reallocation without freeing
int *temp = (int*)malloc(20 * sizeof(int));
if (temp == NULL) {
printf("Memory allocation failed\n");
free(ptr); // Free previously allocated memory
return;
}
free(ptr); // Free the initial allocation
ptr = temp; // Assign new allocation to ptr
// Use the allocated memory
for (int i = 0; i < 20; i++) {
ptr[i] = i * 2;
}
for (int i = 0; i < 20; i++) {
printf("%d ", ptr[i]);
}
printf("\n");
free(ptr); // Free the allocated memory
}
int main() {
avoidOrphaningMemory();
return 0;
}
Output:
0 2 4 6 8 10 12 14 16 18 20 22 24 26 28 30 32 34 36 38
Explanation:
In the above code example-
- We start by defining a function called avoidOrphaningMemory(). Inside this function:
- We allocate memory for an array of 10 integers using malloc() function.
- Also, we cast the returned pointer to an int* and assign it to ptr.
- If the allocation fails, malloc() returns NULL, and we print an error message and return from the function to avoid further processing.
- We then simulate a scenario where we need more memory than initially allocated. So, we allocate memory for 20 integers and store the pointer in temp.
- Again, we check if the allocation fails. If it does, we print an error message, free the previously allocated memory pointed to by ptr, and return from the function.
- If the second allocation succeeds, we free the initial allocation by calling free(ptr). This prevents memory leaks, ensuring we don't orphan the initially allocated memory.
- Thenm we assign the pointer temp to ptr, updating ptr to point to the new allocation.
- Next, we use the allocated memory by filling the array with values. Here, we use a for loop and iterate from 0 to 19, storing twice the index value at each position in the array.
- After filling the array, we print the values to verify the allocation and initialization. We use another loop to print each element followed by a space, and finally print a newline character for better readability.
- At the end of the function, we call free(ptr) to release the allocated memory, ensuring no memory leaks occur.
- Finally, in the main() function, we call avoidOrphaningMemory() to execute the above logic. The main() function returns 0, indicating successful execution.
Create A Counter To Monitor Allocated Memory & Avoid Memory Leaks In C
Monitoring allocated memory involves keeping track of the number of allocations and deallocations during the execution of a C program.
- This can be achieved by using a counter variable that increments when memory is allocated and decrements when memory is deallocated.
- This practice helps in detecting potential memory leaks and ensures proper memory management.
Code:
#include <stdlib.h>
#include <stdio.h>
int allocationCount = 0; // Global counter for memory allocations
void* myMalloc(size_t size) {
allocationCount++;
return malloc(size);
}
void myFree(void* ptr) {
if (ptr != NULL) {
allocationCount--;
free(ptr);
}
}
int main() {
int *ptr1 = (int*)myMalloc(10 * sizeof(int)); // Allocate memory
int *ptr2 = (int*)myMalloc(20 * sizeof(int)); // Allocate more memory
if (ptr1 == NULL || ptr2 == NULL) {
printf("Memory allocation failed\n");
return 1;
}
// Use the allocated memory
ptr1[0] = 42;
ptr2[0] = 84;
// Print the allocation count
printf("Active allocations: %d\n", allocationCount); // Should be 2
// Free the allocated memory
myFree(ptr1);
myFree(ptr2);
// Print the allocation count after freeing memory
printf("Active allocations after free: %d\n", allocationCount); // Should be 0
return 0;
}
Output:
Active allocations: 2
Active allocations after free: 0
Explanation:
In the above code example-
- We start by defining a global variable allocationCount to keep track of the number of active memory allocations. This global counter will be incremented every time we allocate memory and decremented every time we free memory.
- Next, we define a custom memory allocation function myMalloc() that takes the size of the memory to be allocated as a parameter.
- Inside this function, we increment the allocationCount and then use the malloc() function to allocate the memory. We return the pointer to the allocated memory.
- We also define a custom memory deallocation function myFree() that takes a pointer to the memory to be freed as a parameter. Inside this function, we check if the pointer is not NULL. If it is not NULL, we decrement the allocationCount and then use the free() function to deallocate the memory.
- In the main() function, we allocate memory for two integer arrays, ptr1 and ptr2, using the myMalloc() function.
- Then, we request memory for 10 integers for ptr1 and 20 integers for ptr2.
- After that, we check if either allocation failed by verifying if ptr1 or ptr2 is NULL. If either allocation failed, we print an error message using printf() and return with an error code.
- If the memory allocations are successful, we use the allocated memory by assigning values to the first elements of ptr1 and ptr2.
- We then print the current allocation count, which should be 2, since we have made two allocations.
- Next, we free the allocated memory using the myFree() function. We pass the pointers ptr1 and ptr2 to the myFree() function to deallocate the memory and decrement the allocationCount.
- Finally, we print the allocation count after freeing the memory, which should be 0, indicating that all allocated memory has been successfully freed.
Do Not Work On The Original Pointer To Avoid Memory Leaks In C
Working on the original pointer after it has been deallocated can lead to undefined behavior and memory corruption. Once we free the memory using the free() function, the original pointer becomes a dangling pointer that points to an undefined memory location. To avoid this, it's crucial to cease using the pointer after deallocation and avoid any further operations on it.
Code:
#include <stdio.h>
void modifyValue(int *ptr) {
// Modifying the value pointed to by ptr
*ptr = 20;
}
void doNotModifyPointer(int *ptr) {
// Working with a copy of the pointer
int temporaryValue = 10;
modifyValue(&temporaryValue);
// The original pointer is not modified
// Only the copy of the value is changed
}
int main() {
int originalValue = 5;
int *originalPointer = &originalValue;
printf("Before modification: %d\n", *originalPointer);
// Calling a function that does not modify the original pointer
doNotModifyPointer(originalPointer);
printf("After modification: %d\n", *originalPointer);
return 0;
}
Output:
Before modification: 5
After modification: 5
Explanation:
In the above block of code-
- First, we define the function modifyValue(), which takes an int* (pointer to an integer) as an argument.
- Inside this function, we modify the value pointed to by ptr by setting *ptr to 20.
- This means that the function will change the actual value of the integer to which the pointer is pointing.
- Next, we define the function doNotModifyPointer(), which also takes an int* as an argument. Inside this function, we work with a copy of the pointer.
- Then, we declare a local integer variable temporaryValue and initialize it to 10.
- After that we call the modifyValue() function, passing the address of temporaryValue to it.
- This means that modifyValue will modify the value of temporaryValue to 20.
- However, this modification does not affect the original pointer or the value it points to outside of this function.
- This is because we are modifying only the local variable temporaryValue, and the original pointer ptr passed to doNotModifyPointer() remains unchanged.
- In the main() function, we declare an integer originalValue and initialize it to 5.
- We then declare a pointer originalPointer and assign it the address of originalValue. This means that originalPointer points to originalValue.
- Then, we use printf() to print the value pointed to by originalPointer, which is 5 at this point.
- After that, we call the doNotModifyPointer() function, passing originalPointer to it.
- Since doNotModifyPointer() works with a copy of the pointer and modifies a local variable, the original pointer and its pointed value remain unaffected.
- After returning from doNotModifyPointer(), we again use printf() to print the value pointed to by originalPointer. It still prints 5, confirming that the original pointer and the value it points to have not been modified.
Include Proper Commenting In Your Code
Comments in programming languages are annotations in the code that help explain the purpose and functionality of code segments. They provide information on the code, its logic, and other pertinent facts, acting as an explanation for developers. Proper comments improve code readability and maintainability. Use comments to document memory allocation and deallocation, making it clear where memory is managed.
Code:
#include <stdlib.h>
#include <stdio.h>
// Main function to demonstrate memory management
int main() {
// Allocate memory for 10 integers
int *ptr = (int*)malloc(10 * sizeof(int));
if (ptr == NULL) {
printf("Memory allocation failed\n");
return 1;
}
// Use the allocated memory
ptr[0] = 42; // Initialize first element
// Free the allocated memory
free(ptr);
return 0;
}
Explanation:
In the above code snippet-
- We start by allocating memory for 10 integers using malloc() function and cast the returned pointer to an int* and assign it to ptr.
- The malloc() function allocates a block of memory of the specified size and returns a pointer to it. In this case, we request memory for 10 integers.
- Next, we check if the memory allocation was successful by verifying if ptr is not NULL:
- If malloc() returns NULL, it indicates that the memory allocation failed. In such a case, we print an error message and return 1 from the main() function to indicate an error.
- If the memory allocation is successful, we proceed to use the allocated memory. We initialize the first element of the array by assigning the value 42 to ptr[0].
- This demonstrates how we can access and modify the elements of the dynamically allocated array.
- After using the allocated memory, it is important to free it to avoid memory leaks. We call the free() function, passing the pointer ptr to it. This releases the allocated memory, making it available for future allocations.
Detecting Memory Leaks In C
In programming, it is important to detect memory leaks to ensure efficient memory management and prevent issues like excessive memory consumption. There are various tools and methods available for detecting memory leaks in C. Here are some common approaches along with examples:
- Manual Code Inspection: Regularly inspecting code for proper memory allocation and deallocation involves systematically reviewing the source code to identify potential memory leaks. Developers should look for instances where memory is allocated but not freed, a common source of memory-related issues. For Example-
void potentialLeak() {
int *ptr = (int*)malloc(sizeof(int) * 10);
// Some operations
free(ptr); // Ensure every malloc() has a corresponding free()
}
- Using Debugging Tools: Debugging tools like gdb (GNU Debugger) can help track memory usage and detect leaks. While gdb is primarily a general-purpose debugger, it can be used in conjunction with memory leak detection libraries. For Example-
gcc -g -o program program.c
gdb ./program
run
# Use gdb commands to inspect memory allocations
- Valgrind: Valgrind is a powerful tool for detecting memory erros and memory leaks in C. The memcheck tool in Valgrind can identify memory leaks by tracking all memory allocations and deallocations. For Example-
gcc -g -o program program.c
valgrind --leak-check=yes ./program
- AddressSanitizer (ASan): AddressSanitizer is a runtime memory error detector for C/C++. It is integrated with GCC and Clang and can detect memory leaks, buffer overflows, and other memory-related errors. For Example-
gcc -fsanitize=address -o program program.c
./program
- Electric Fence: Electric Fence is a memory debugger tool that helps detect potential buffer overflows and memory leaks in C programs, by using virtual memory hardware to place inaccessible memory pages immediately after allocated memory. For Example-
gcc -o program program.c -lefence
./program
- Use Memory Leak Detection Libraries: Libraries like mtrace() can detect memory leaks by instrumenting memory allocation and deallocation calls. Developers can enable mtrace(), execute the program, and then disable mtrace() to generate a comprehensive report on memory usage. These libraries offer a practical method to verify memory-related issues, especially in more complex applications. For Example-
#include <mcheck.h>
int main() {
mtrace(); // Enable mtrace
// Code with memory allocations and deallocations
muntrace(); // Disable mtrace and generate a report
return 0;
}
Fixing Memory Leaks In C
Once memory leaks are detected, it’s crucial to fix them to ensure the stability and efficiency of your C programs. Here are some strategies and best practices to address and prevent memory leaks in C/C++:
- Ensure Proper Memory Deallocation: Always free dynamically allocated memory when it is no longer needed. This involves matching every malloc(), calloc(), or realloc() with a corresponding free(). For example-
void fixMemoryLeak() {
int *ptr = (int*)malloc(sizeof(int) * 10);
// Some operations on ptr
free(ptr); // Properly deallocate memory
}
- Use Smart Pointers and RAII (Resource Acquisition Is Initialization): While C does not have built-in smart pointers like C++, you can implement a similar concept by encapsulating memory management in functions or structures to ensure proper deallocation. For example-
typedef struct {
int *data;
} SmartPointer;SmartPointer createSmartPointer(size_t size) {
SmartPointer sp;
sp.data = (int*)malloc(size);
return sp;
}void freeSmartPointer(SmartPointer *sp) {
free(sp->data);
sp->data = NULL;
}int main() {
SmartPointer sp = createSmartPointer(sizeof(int) * 10);
// Some operations on sp.data
freeSmartPointer(&sp);
return 0;
}
- Handle Errors and Clean Up Properly: Ensure all allocated memory is freed, even in error paths. Use goto statements for clean-up in complex functions. For example-
int allocateAndProcess() {
int *ptr = (int*)malloc(sizeof(int) * 10);
if (ptr == NULL) {
return -1; // Allocation failed
}// Simulate an error
if (someErrorCondition) {
goto error;
}// Some operations on ptr
free(ptr);
return 0;error:
free(ptr);
return -1;
}
- Memory Leak Detection Tools: Utilizing memory leak detection tools is a proactive approach to identifying and locating memory leakage in a program. These tools provide insights into potential memory-related issues, enabling developers to address them before deployment and ensuring a more robust and reliable software application. For example-
// Code with potential memory leaks
// Run memory leak detection tool
- Use Automatic Storage Duration (Stack) Whenever Possible: Preferring automatic storage duration (stack) for variables when possible helps avoid explicit memory management. Variables with automatic storage duration are automatically deallocated when they go out of scope, simplifying memory management and reducing the chances of memory-related issues. For example-
void functionWithLocalArray() {
int localArray[100]; // Automatic storage duration
// Use the local array
} // Memory is automatically deallocated when the function exits
Conclusion
Memory leaks in C programming can significantly impact the performance and stability of your applications, leading to reduced efficiency, unpredictable behavior, and even system crashes. Understanding the causes of memory leaks and implementing strategies to detect and fix them is crucial for maintaining code performance and reliability.
By utilizing debugging and memory analysis tools like Valgrind and AddressSanitizer, and following best practices for memory management, you can effectively prevent and address memory leaks. Proper error handling, consistent use of free() for every malloc(), and adopting systematic testing methods are key steps in ensuring your applications remain efficient and stable.
Frequently Asked Questions
Q. How can I prevent memory leaks in my future C projects?
Preventing memory leaks in C projects involves adopting good coding practices and using tools to help manage memory:
- Write clean, well-documented code: Clear code makes it easier to track memory allocations and deallocations.
- Regular code reviews: Peer reviews can help catch potential memory leaks in C programs/ projects.
- Automated testing: Integrate memory leak detection tools into your CI/CD pipeline.
- Use smart pointers and RAII: Although C doesn't have built-in smart pointers, similar practices can be implemented manually.
- Stay informed: Continuously learn and apply best practices for memory management in C programming.
Q. What is a memory leak in C, and why should I be concerned about it?
A memory leak in C occurs when a program allocates memory dynamically using functions like malloc(), calloc(), or realloc() but fails to free that memory using free(). Over time, this leads to inefficient memory usage as the program holds onto memory it no longer needs, potentially causing reduced performance, erratic behavior, and even system crashes, especially in long-running applications.
Q. Is there potential damage to RAM as a result of memory leaks?
Memory leaks themselves do not cause physical damage to RAM or ROM, but they can lead to significant performance degradation issues in a system. When a program leaks memory, it fails to release allocated memory back to the operating system.
- Over time, this can exhaust available memory, causing the system to slow down, become unresponsive, or even crash as it runs out of memory.
- This can lead to increased wear and tear on the system as a whole, as the constant paging and swapping of memory to disk puts extra strain on storage devices and can reduce overall system longevity.
- However, the RAM hardware itself remains physically unaffected by memory leaks in C programs. The issue is strictly related to inefficient memory management by software.
Q. What are the common causes of memory leaks in C?
Memory leaks in C can be caused by several common mistakes:
- Forgetting to free dynamically allocated memory.
- Losing the reference to allocated memory before freeing it.
- Reassigning pointers without freeing the previously allocated memory.
- Failing to free memory in error handling paths.
- Improperly managing complex data structures (e.g., linked lists, trees).
Q. Differentiate between calloc() and malloc()?
Here are the key differences between calloc() and malloc() in C:
Feature | malloc() | calloc() |
---|---|---|
Function | Allocates a specified number of bytes of memory. | Allocates memory for an array of elements and initializes them to zero. |
Syntax | void* malloc(size_t size); | void* calloc(size_t num, size_t size); |
Parameters | size: Number of bytes to allocate. | num: Number of elements to allocate. <br> size: Size of each element. |
Initialization | Does not initialize the allocated memory. | Initializes the allocated memory to zero. |
Use Case | Suitable when the number of bytes required is known. | Suitable when an array of elements needs to be allocated and initialized to zero. |
Performance | Generally faster due to no initialization. | Slightly slower due to zero-initialization of memory. |
Example | int* arr = (int*)malloc(10 * sizeof(int)); | int* arr = (int*)calloc(10, sizeof(int)); |
Q. Is it possible for a memory leak to occur in stack memory?
No, a memory leak can't occur in stack memory. Stack memory is managed automatically by the system, and its allocation and deallocation are handled implicitly through the function call mechanism.
- When a function is called, memory for local variables is allocated on the stack, and when the function returns, this memory is automatically deallocated.
- Because the stack is a Last-In-First-Out (LIFO) data structure, memory management is straightforward and efficient, eliminating the possibility of leaks.
- In contrast, memory leaks occur in the heap, where dynamic memory allocation and deallocation must be explicitly managed by the programmer using functions like malloc() and free().
- Failure to properly manage the heap block of memory leads to memory leaks in C, whereas stack memory is inherently protected against such common issues due to its automatic nature.
You might also be interested in reading the following:
- Ternary (Conditional) Operator In C Explained With Code Examples
- 6 Relational Operators In C & Precedence Explained (+Examples)
- Keywords In C Language | Properties, Usage, Examples & Detailed Explanation!
- Control Statements In C | The Beginner's Guide (With Examples)
- Constant In C | How To Define & Its Types Explained With Examples
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment