Table of content:
- Length Of String In C++ Language
- Ways To Find Length Of String In c++
- How To Find String Length In C++ Without strlen?
- String Length in C++ With User-Defined Function
- String Length In C++ Using Built-In Library Function
- String Length In C++ Using the sizeof() Operator
- Find String Length In C++ Using Recursion
- Function To Find String Length Using Pointers
- Conclusion
- Frequently asked questions
- Test Your Skills: Quiz Time
Ways To Find String Length In C++ Simplified (With Examples)
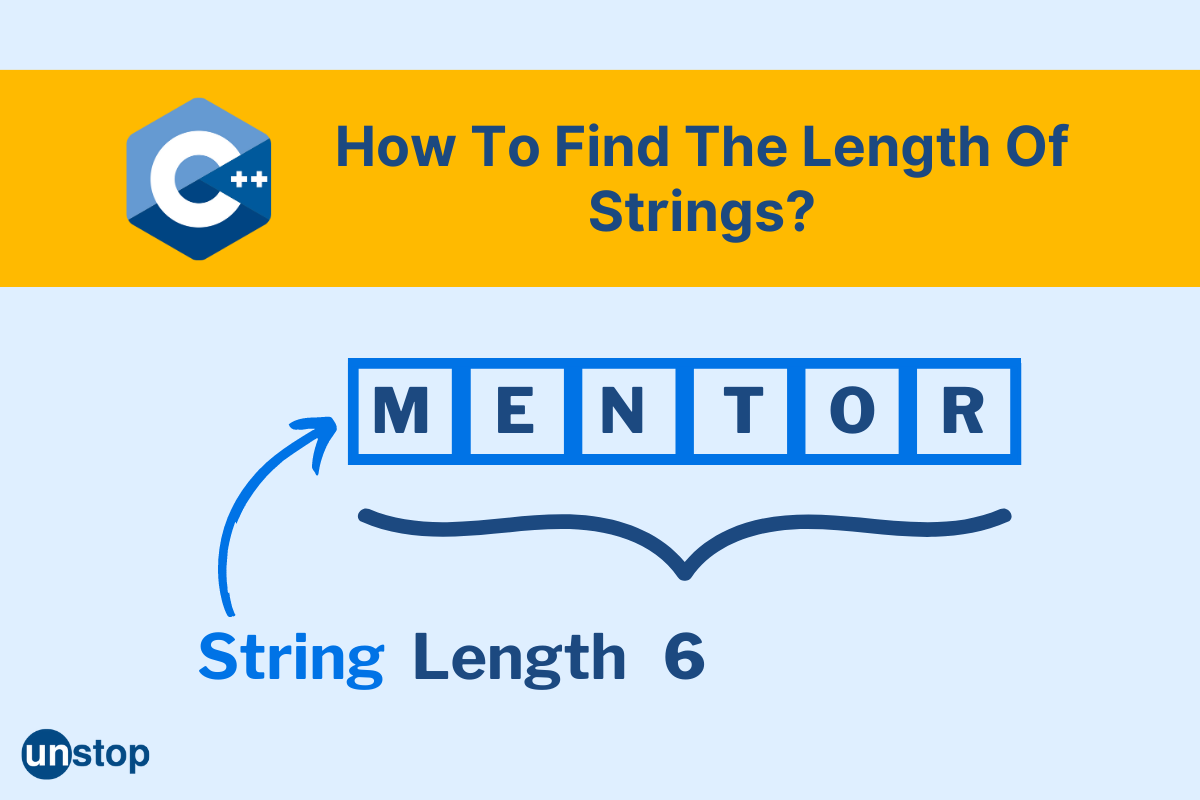
Wondering what is a string in C++? Or how to find its length? Well, an ordered series of characters or an array of characters is referred to as a string in C++. Additionally, there is also something called the class object in C++, which refers to a method of representing a collection of characters. The name of this class is std:: string. While discussing anything related to strings an important topic that comes to the fore is the string length in C++. And in this article, we will explore the length of strings, methods to calculate it, and other related concepts in proper detail, with examples.
Length Of String In C++ Language
The length of a string refers to the measure of a string's magnitude. There are many different procedures/ approaches in C++ that we can use for computing the extent (length) of a given string. For example, one possible course of action would be to employ the length() fucntion of the std::string category. This particular method gauges the numerical value, expressed in bytes, that represents a given string's span. Other approaches include the use of the size() and strlen() functions.
Ways To Find Length Of String In c++
As we've mentioned, there are multiple ways to find the length of string in C++. In this section, we will discuss these in detail, with examples.
Using The strlen() Function
The strlen() function, extracted from the <cstring> header, is a valuable tool in C++ programming that can be employed for ascertaining the length of a null-terminated character array or C-style string. The output yielded by said method corresponds to the number of characters expressed in enumeration with respect to its respective input data set.
Example to find the length of a C-style string in C++ using strlen() function:
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[] = "Hello,Unstop!";
cout << "The length of the string is: " << strlen(str) << endl;
return 0;
}
Output:
The length of the string is: 13
Explanation:
This program uses the strlen() method to determine the string's length after initializing a C-style string with the value "hello,unstop!" A console printout of the length follows. The output will be 13 because the string has 13 characters total, including the comma and the exclamation point.
NOTE: However, there is no need to use strlen() for C++ strings. Instead, it is better to use the std::string class's size() or length() member methods. The aforementioned functions provide the count of bytes that construct a given string, and this is not always equivalent to the extent of its storage capacity. It is noteworthy that size() and length() are interchangeable with each other in producing an identical outcome.
Using The length() Function
The length() and size() functions do not merely return the number of elements contained within a given string, but instead, produce an output reflecting the precise numerical value, in terms of bytes. And this value corresponds to the volume of data occupied by the said string in terms of bytes.
It is crucial to note that this byte total does not always correspond with the total storage capacity allotted for a particular string object, thus introducing another layer of complexity into potential programming implementations. In sequences of multi-byte or variable-length characters, the result returned might not equate to the actual number of encoded characters.
Example Of C++ program using length() function:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello,Unstop!";
cout << "The length of the string is: " << str.length() << endl;
return 0;
}
Output:
The length of the string is: 13
How To Find String Length In C++ Without strlen?
To find string length in C++ without the strlen() function, you can use the for loop and while loop. We'll have a look at both of these approaches with the help of examples, in this section.
Using 'for loop'
Initialize the counter to 0 and increase it from the beginning of the string to the end using the for loop (terminating the null character).
Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello,Unstop!";
int len = 0;
for (int i = 0; str[i] != '\0'; i++) {
len++;
}
cout << "Size of the string is: " << len << endl;
return 0;
}
Output:
Size of the string is: 13
Explanation:
In the above code, we declare a string variable str and initialize it with the value "Hello, Unstop!". Then, we declare an integer variable len to store the size of the string.
Subsequently, we proceed to conduct an iterative process, utilizing a for loop and gradually expanding the variable 'len' with each subsequent individual character observed. This rhythmic incrementation continues until the null character (\0) marks the conclusion of the string's sequence.
Using 'while loop'
You can go through the string and count the characters till you reach the null character in C++ to determine the length of a string using a while loop.
Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string myString = "Hello,Unstop!";
int size = 0;
while (myString[size] != '\0') {
size++;
}
cout << "The size of the string is: " << size << endl;
return 0;
}
Output:
The size of the string is: 13
Explanation:
This sample code initializes an object known as myString with the text "Hello, Unstop!" Furthermore, a variable named "size," which will store the length of the text, is established with a value of 0. The while loop is employed to traverse the characters of the string until it comes to the null character ('0') that denotes the end of the string. With each loop iteration, the size variable is increased.
The value of size, which we print out last, represents the length of the string.
Note: In view of the requisites needed to count the characters, specifically traversing through the whole string, this particular methodological approach exhibits sub-optimal effectiveness when compared against alternative methods that perceive invoking member functions such as size() or length().
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
String Length in C++ With User-Defined Function
An effective technique for computing the length of a string using a user-defined function requires counting all characters present in the string through iteration up to when it reaches the null character (\0), which denotes that we have reached the end of the string.
Example of string length using a user-defined function:
#include <iostream>
#include <string>
using namespace std;
int stringLength(string str) {
int length = 0;
while (str[length] != '\0') {
length++;
}
return length;
}
int main() {
string str;
cout << "Enter a string: ";
getline(cin, str);
cout << "The length of the string is: " << stringLength(str) << endl;
return 0;
}
Input:
Enter a string: UNSTOP
(Note: You can enter any string of your choice.)
Output:
The length of the string is: 6
Explanation:
The function ‘stringLength’ is designed to compute the length of a given input string by iterating through its individual characters in a loop. Once the null character (\0) is detected, which marks the end of the string, the function returns the total number of characters up until that point.
In order to acquire user input for analysis, getline from the string library is used within the main function. After this prompt, the stringLength function calculates and provides output with a numerical representation of the character count for each entered line.
String Length In C++ Using Built-In Library Function
There are a number of inbuilt library functions to determine the length of a string. This includes string::length() or string::size() which is one of the most popular functions to find the length of strings in C++.
Example using the size() function:
#include <iostream>
#include <string>
using namespace std;
int main() {
string myString = "Hello, world!";
int size = myString.size();
cout << "The size of the string is: " << size << endl;
return 0;
}
Output:
The size of the string is: 13
Explanation:
Within this application, an object known as "myString" is established by utilizing the string "Hello, world!" in its initialization process. Subsequently, with the aid of the size() function, we are able to evaluate and quantify myString's length before assigning it to a designated integer variable (referred to as 'size'). Lastly, we print out 'size', which signifies the value indicating myString's length. This method replaces manually scanning through the string with a loop, ultimately resulting in enhanced efficiency and reduced potential for error when assessing its length.
String Length In C++ Using the sizeof() Operator
We generally use the sizeof() operator in C++ to find a data type's size in bytes at build time. Utilizing the sizeof() function to ascertain a string's length may give incongruous results as it involves factoring in the total size of the data type, which might comprise unnecessary bits or padding. Consequently, utilizing this method to gauge a string's length is not considered an ideal approach. Let's look at an example-
Example:
#include <iostream>
#include <string>
using namespace std;
int main() {
string myString = "Hello, world!";
int size = sizeof(myString);
cout << "The size of the string is: " << size << endl;
return 0;
}
Output:
The size of the string is: 32
Explanation:
The output reveals that said object's overall size amounts to 32 bytes. It should be noted, however, that determining size via the sizeof operator involves careful consideration of all elements related to the string, including but not limited to: data pointer(s), length estimate, and maximum yielding capacity.
Find String Length In C++ Using Recursion
Within C++, a viable option to derive the length of a string recursively consists of crafting a function capable of tallying characters up until the null one. Such an algorithmic approach allows for an effective resolution of this task that relies on recursive methods.
Example:
#include <iostream>
#include <string>
using namespace std;
int stringLength(string str) {
if (str == "") {
return 0;
} else {
return 1 + stringLength(str.substr(1));
}
}
int main() {
string myString = "Hello, world!";
int size = stringLength(myString);
cout << "The size of the string is: " << size << endl;
return 0;
}
Output:
The size of the string is: 13
Explanation:
The recursive function stringLength is defined in this example. It accepts the string parameter str and returns an integer that reflects the length of the string.
The code first checks to see if the string is empty. (i.e., has length 0). If it is, the method returns 0. The function returns 1 and the result of performing itself recursively on the substring of str starting with character 2 if such a condition is not present (i.e., str.substr(1)).
In the main() function, we initialize a string object called myString with the phrase "Hello, world!" Utilizing an iterative approach, the length of myString is methodically determined through the implementation of the stringLength function. Subsequently, the numeric output derived from this process is allocated and stored in a designated integer variable called size.
Function To Find String Length Using Pointers
One could construct a function in C++ that utilizes a pointer to traverse through the string and tally up the characters until arriving at the null character, thereby allowing for the determination of said string's length.
Example:
#include <iostream>
int stringLength(const char* str) {
const char* ptr = str;
while (*ptr != '\0') {
ptr++;
}
return ptr - str;
}
int main() {
char str[] = "hello, world!";
std::cout << "The length of the string is: " << stringLength(str) << std::endl;
return 0;
}
Output:
The length of the string is: 13
Explanation:
The mentioned program contains a method called "stringLength()" that serves to identify the length of a C-style string, utilizing said string as its argument. This action transpires subsequent to an initialization process, which establishes a reference point located at the beginning of the string. The ensuing procedure operates via iteration and terminates upon encountering the null character represented in the given string.
Conclusion
The measurement of a string's length in the C++ programming language lends itself to a number of methodologies. One can opt to utilize either the size() or length() member functions belonging to the std::string class, both of which accurately measure length through external values expressed in bytes. Alternatively, another feasible solution is implementing the strlen(), applicable solely for C-style strings and offering an output arbitrary to character count alone. Furthermore, pointers embodying an iterational structure can be employed for manual calculation purposes, across the string until reaching a null-terminating character. It should be duly noted, however, that use of 'sizeof()', even though proposed as viable by certain sources, must herein be deemed unacceptable.
Also read- The Structure of C++ Programs Explained With Examples!
Frequently asked questions
Q. How to find the length of a string in C++?
There are numerous methods available to figure out the length of a string in C++. One method is through the std::string class's size() or length() member functions, which will provide an output in bytes measuring the extent of the string. An alternate approach is the strlen() function which determines the length of C-style strings in terms of characters. Furthermore, it is plausible to employ pointers as a means of traversing the string and tallying the number of characters until the termination point is characterized by the null symbol. One alternative technique involves implementing a loop to systematically cycle through the string and execute an enumeration of each character until arriving at the null character.
It is important to note that the sizeof() operator is not a reliable way to find the length of a string because it returns the size of the entire data type, including any padding or unused space.
Q. What is the difference between the size() function and the length() function for strings?
The size() and length() functions are both used as ways to calculate a string's length in C++ language. But they each take a different approach. On one hand, the size() method discloses the string's scope in terms of bytes, which outlines the overall volume of legitimate bytes that create the contents within the said string. On the other hand, the length() function returns the number of characters in the string.
Q. How do you iterate over a string to find its length?
One approach to determine the length of a string in C++ is to iterate through all of its characters until encountering the null character. This can be achieved with the 'while' loop to inspect each character until the null character is detected. A numerical counter can also be implemented within the loop to keep track of every inspected element and its final incremental value will yield the length of said string.
An alternative approach to determining the size of a string involves implementing recursion. To achieve this, a repetitive function may be used, with the express purpose of counting up the quantity of characters contained within the string until it ultimately encounters a null character.
Q. How can you get the maximum length of a string in C++?
In order to attain the uppermost extent of a character sequence in C++, one may utilize the function max_size() derived from the std::basic_string class. This specific function accomplishes this by delivering the maximum quantity of elements that can be accommodated within the said string.
Q. Can the length of a string change in C++?
In the unparalleled world of programming with C++, modifications to a string can have a rather profound impact on its length; wherein adding or removing characters resultantly changes said length.
Q. What built-in C++ function is used to reverse a string?
In C++, the act of reversing a string can be seamlessly accomplished through the implementation of the "reverse()" function. It's included in the string.h library.
Q. How do you concatenate two strings in C++?
In C++, you can concatenate two strings using the + operator or the append() function of the std::string class. The operator denoted by the plus sign is known to merge two strings and generate an entirely new string that incorporates both of their contents in a collaborative manner.
Q. How do you compare two strings in C++ based on their length?
Using the length() or size() member functions of the std::string class, you can get the lengths of two strings in C++ and then compare them using the appropriate comparison operator (, >, =, >=, ==, or!=).
Q. How do you reverse a string in C++?
To reverse a string, one method is to implement the reverse() function from the algorithm header file. This technique calls upon two iterators that indicate the starting and concluding points, which define the range that is intended to undergo a reversal. An alternative method involves implementing a loop within which each initial character, iteratively progressing towards those occupying positions nearer the center of the string, is swapped with its corresponding opposing counterpart using swap().
Q. How do you count the number of occurrences of a character in a string in C++?
Using a loop to cycle through each character in the string and compare it to the character being searched for is one approach to counting the number of times a character appears in a string in C++. A counter variable is increased if a match is discovered. Utilizing the count() method from the algorithm header file is another option. The start and end of the search range, as well as the value to be looked for, are passed as parameters to this method along with two iterators.
Test Your Skills: Quiz Time
You might also be interested in reading the following:
- Typedef In C++ | Syntax, Application & How To Use It (With Examples)
- What Is GitHub? An Introduction, How-To Use It, Components & More!
- History Of C++ | Detailed Explanation (With Timeline Infographic)
- What Is Bash? Features, Major Concepts, Commands, & More!
- Difference Between C And C++| Features | Application & More!
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Blogs you need to hog!
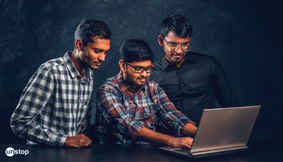
This Is My First Hackathon, How Should I Prepare? (Tips & Hackathon Questions Inside)
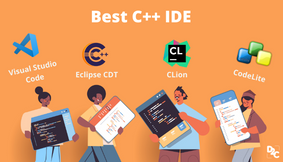
10 Best C++ IDEs That Developers Mention The Most!
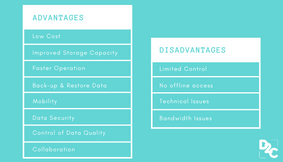
Advantages and Disadvantages of Cloud Computing that you should know!
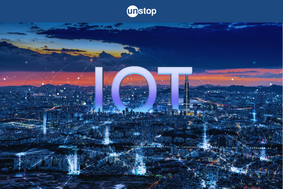
samyak dandge 1 week ago
Mohit kumar Agarwal 2 weeks ago
Anish Malik 2 weeks ago