Shift Operators In C | The Ultimate Guide With Code Examples
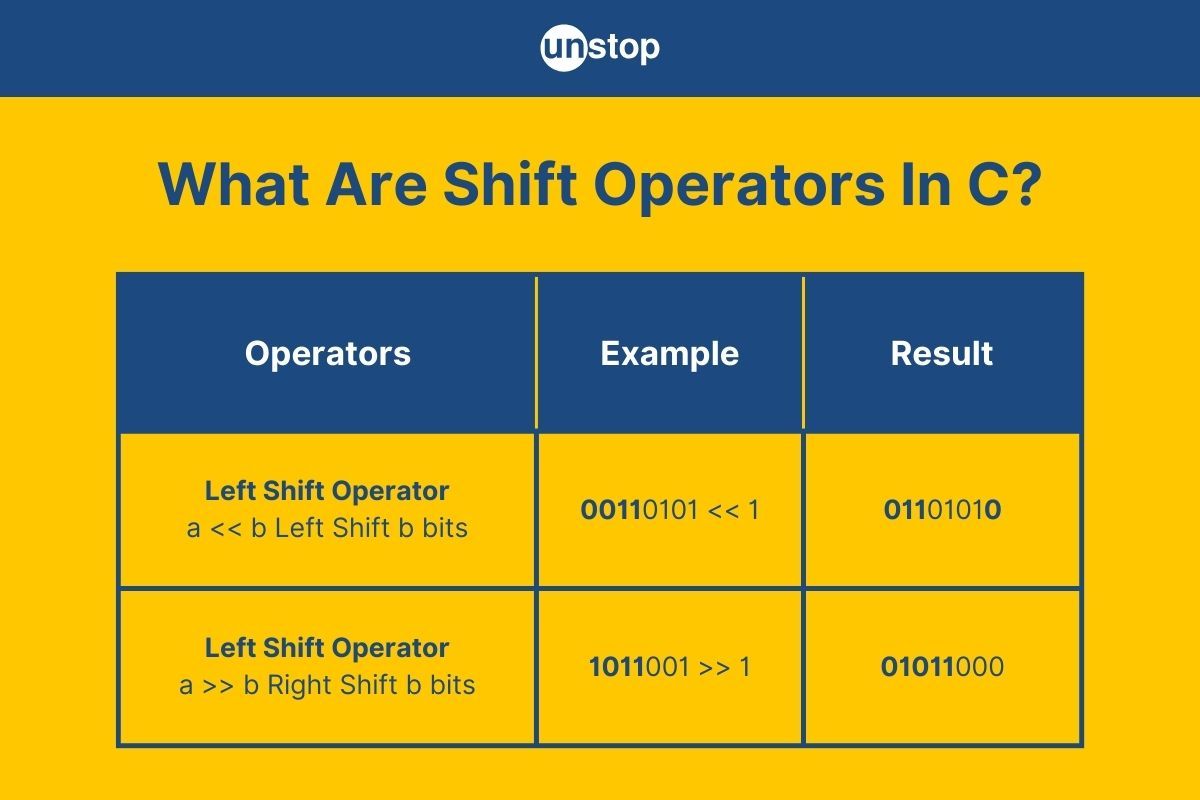
In the field of programming, understanding bitwise operations is crucial for optimizing performance and managing low-level data manipulation. Among these operations, shift operators in C programming hold a special place due to their optimal code efficiency and versatility. In this article, we will discuss the two fundamental shift operators, i.e., the left shift operator and the right shift operator in C. We'll explore how these operators work, their syntax, and practical use cases with the help of examples of shift operators.
What Are Shift Operators In C?
As you must know, shift operators are unary operators that work at the bit level of the operands. They shift the bits by a prespecified number of positions. In other words, the right shift (>>) and the left shift operator in C (<<) allow programmers to move bits within an integer to the left or right, respectively. They enable efficient data manipulation and optimization techniques. We will discuss both types of Bitwise shift operators in detail in the sections ahead.
Left Shift Operators In C
As discussed above, the left shift operator (<<) in C is a bitwise operator that shifts the bits of a binary number to the left by a specified number of positions. It essentially multiplies the value on the left by 2, raised to the power of the shift amount on the right. This ability of the left shift operator to multiply a number by powers of two is one of its primary characteristics.
Syntax Of Left Shift Operator In C
The left shift operators in C has a straightforward syntax. It involves two non-zero operands i.e. the value to be shifted and the number of positions to shift.
result = value << positions;
Here,
- The result is the variable that will store the outcome of the left shift operation performed using the left shift operator(<<).
- Value represents the number whose bits we want to shift, and position is the number of positions to shift the bits by.
Uses Of Left Shift Operator In C
Before we understand the working mechanism of left shift operators in C, let's have a look at some of its common use cases:
1. Bit Masking and Manipulation: The left shift operator is used to create bit masks. Bit masks are used to isolate, set, or clear specific bits in a binary number.
Example:
int mask = 1 << 3; // Creates a mask with the fourth bit set (00001000)
int value = 5; // Binary: 00000101
int result = value & mask; // Masks the larger value, isolating the fourth bit
2. Packing Data into a Single Integer: When dealing with multiple small data items, the left shift operator can pack these items into a single positive integer by shifting bits to appropriate positions.
Example:
unsigned char a = 3; // 00000011
unsigned char b = 5; // 00000101
unsigned int packed = (a << 8) | b; // Packed value: 00000011 00000101
3. Optimizing Performance in Embedded Systems: In embedded systems where memory space efficiency and processing power are limited, bitwise operations, including left shifts, are used to perform tasks more efficiently than using arithmetic operations.
4. Generating Specific Bit Patterns: The left shift operator is used to generate specific bit patterns for tasks such as setting control registers or initializing hardware components.
Example:
unsigned int pattern = (1 << 0) | (1 << 2) | (1 << 4); // Pattern: 00010101
5. Data Serialization and Deserialization: When serializing data (converting data into a format for transmission or storage) or deserializing data (reverting to the original format), the left shift operator helps assemble and disassemble the key space data.
Working Of Left Shift Operators In C
Below is a step-by-step description of how the left shift operators in C work:
Step 1: Initialize the variable whose bits we want to shift and the number of positions by which we want to make the shift.
For example, say the number is 5 (binary representation: 00000000 00000000 00000000 00000101), and the number of bit positions to shift is 3.
Step 2: When the left shift operator in C is applied to these values, it shifts each bit in the binary representation to the left by the provided number of places.
For example, left-shifting the number 5 by 3 position alters its binary representation from 00000000 00000000 00000000 00000101 to 00000000 00000000 00000000 00010100.
Step 3: Once the shift has happened, the bits that move beyond the left end are discarded, and all the empty positions to the right are filled with zeros. Thus, the bit pattern becomes 00000000 00000000 00000000 00010100.
Step 4: The modified binary representation is then converted to a new decimal value. In our example, 00000000 00000000 00000000 00010100 corresponds to the decimal value 40.
Also, we can observe that shifting left by n positions is equivalent to multiplying the number by 2 to the power of n. In this example, shifting left by 3 positions is the same as multiplying 2 to the power of 3, which equals 8. Therefore, the result of the operation 5 << 3 is 5 × 8, which equals 40.
Step 5: Finally, we print the original number and the result to see the effect of the shift. The output will demonstrate the original number and its new value after the shift operation, along with the binary representations before and after the shift.
Example Of Left Shift Operators In C
Let’s understand it better with the help of an example C program implementing left shift operator.
Code:
Output:
Original number: 5
After left shift by 2 positions: 20
Explanation:
In the above code example,
- We start by including the standard input/output library with #include <stdio.h> to use functions like printf.
- Next, inside the main function, we declare an unsigned integer variable named number and assign it value 5 using the assignment operator. In binary, this is represented as 00000000 00000000 00000000 00000101.
- We then declare another integer variable called shift and set it to 2. This variable represents how many positions we want to shift the bits of number to the left.
- Next, we perform the left shift operation using the >> operator. This shifts the bits of number to the left by the number of positions specified by shift. In this case, we shift the bits of 5 to the left by 2 positions, resulting in a new value of 20 in decimal.
- We will now store the result of this shift operation in a variable named result and print it using the print statement.
- Finally, we end the main function and return 0. This return value indicates that the program has finished executing successfully.
Right Shift Operators In C
The right shift operator (>>) in C is a fundamental bitwise operation used to manipulate the bits of an integer. It allows us to shift the bits of a number to the right, effectively dividing the number by powers of two. This operator is commonly used in low-level programming for efficient arithmetic operations and bit manipulation tasks.
Syntax Of Right Shift Operator In C
The syntax for the right shift operators in C is as follows:
result = value >> positions;
Here,
- The result is the variable that will store the outcome of the right shift operation performed using the right shift operator (>>).
- Value represents the number (value) whose bits we want to shift, and position is the number of positions to shift the bits by.
Uses Of Right Shift Operators In C
Here are some of the common and important use cases of right shift operators in C:
- Division by Powers of Two: One of the most common uses of the right shift operators in C is to perform division by powers of two efficiently. Shifting bits to the right by n positions is equivalent to dividing the number by 2 to the power of n.
- Arithmetic Right Shift: Dividing a variable by powers of two is a common use for the right shift operator in C. Moving the bits to the right by the required number of places is an effective method of performing division. In the example below, the value is shifted three places to the right, split by two, and raised to the power of three.
Example:
int result = value >> 3; // Equivalent to dividing by 2^3
- Discarding the Least Significant Bits: The least significant bits of a value can be eliminated by using the right shift operator. In certain situations, it can be helpful to eliminate the least significant bits from a value by right-shifting it.
Example:
int truncatedValue = originalValue >> 2; // Discards the least significant 2 bits
- Implementing Division and Modulo Operation: In cases where both the quotient and remainder are needed, right shifts can be used in conjunction with bitwise AND operations to efficiently compute both.
-
Bitwise Operations and Masking: Right shifts are crucial in bitwise operations to manipulate and check specific bits within a binary representation of data. This is useful in data structures, networking protocols, and cryptography.
Working Of Right Shift Operators In C
Given below is a step-by-step description of how right shift operator in C work:
Step 1: Initialize the variable whose bits we want to shift and the number of positions by which we want to make the shift. For example, say the number is 40 (binary representation: 00000000 00000000 00000000 00101000), and the number of bit positions to shift is 3.
Step 2: When the right shift operator in C is applied to these values, it shifts each bit in the binary representation to the right by the provided number of places. For example, right-shifting the number 40 by 3 positions alters its binary representation from 00000000 00000000 00000000 00101000 to 00000000 00000000 00000000 00000101.
Step 3: Once the shift has happened, the bits that move beyond the right end are discarded. All the empty positions to the left are filled with zeros (for an unsigned right shift or logical right shift). Thus the bit pattern becomes 00000000 00000000 00000000 00000101.
Step 4: The modified binary representation is then converted to a new decimal value. In our example, 00000000 00000000 00000000 00000101 corresponds to the decimal value 5.
Also, we can observe that shifting right by n positions is equivalent to dividing the number by 2 to the power of n. In this example, shifting right by 3 positions is the same as dividing by 2 to the power of 3, which equals 8. Thus, the result of the operation 40 >> 3 is 40 / 8, which equals 5.
Step 5: Finally, we print the original number and the result to see the effect of the shift. The output will demonstrate the original number and its new value after the shift operation, along with the binary representations before and after the shift.
Example Of Right Shift Operators In C
Let’s understand it better with the help of an example in C programming implementing the right shift operator.
Code:
Output:
Original number: 20
After right shift by 2 positions: 5
Explanation:
In the above code example,
- We start by declaring an unsigned integer variable called number inside the main function and initialize it to 20. In binary value, this number is represented as 00000000 00000000 00000000 00010100.
- Next, we declare another integer variable named shift and set it to 2. This variable specifies how many positions we will shift the bits of number to the right.
- We then perform the right shift operation by using the >> operator. This operation moves the bits of number to the right by the number of positions specified by shift. In this case, we are shifting the bits of 20 right by 2 positions. The new value after the shift is 5 in decimal.
- We will now store the result of this right shift operation in a variable named result and print it using the printf statement.
-
Finally, we end the main function and return 0. This return value indicates that the program has finished executing successfully.
Key Points To Remember For Shift Operators In C
Understanding the behavior of shift operators in C and their implications is essential when working with them. Here are some important points that we need to keep in mind while working with them:
- Data type matters: Shift operators in C (<< and >>) are designed to work on integer types (signed and unsigned types). Using them on other data types may lead to unexpected results or errors.
- Non-negative Shift Amount: The shift count should be non-negative. Shifting by a negative value results in undefined behavior.
- Undefined Behavior for Negative Right Shift: Right-shifting a negative signed integer results in implementation-defined behavior. Different compilers may handle this differently due to different compilation processes, so it's best to avoid right-shifting negative numbers unless you are certain about the behavior of your compiler.
- Bitwise Shift Limits: Shifting by a number greater than or equal to the number of bits in the variable results in undefined behavior. For example, in a 32-bit integer, shifting by 32 or more positions is considered undefined.
- Zero-Fill for Unsigned Types: Right-shifting an unsigned integer fills the vacant positions with zeros. This is often referred to as a logical right shift. Ensure that this behavior aligns with your intentions when working with unsigned integers.
- Masking and Unmasking: Shift operators in C are commonly used in combination with bitwise AND (&) and OR (|) operations to create masks or extract specific bits.
Conclusion
Shift operators in C programming language encompass both the left shift (<<) and right shift (>>) operators. They are essential tools for performing efficient bitwise manipulations and arithmetic operations.
- The left shift operator in C, allows us to move bits to the left, effectively multiplying a number by powers of two. It can be leveraged for tasks like quick multiplications and bit field adjustments.
- Conversely, the right shift operator in C moves bits to the right, enabling efficient division by powers of two. It facilitates operations like data extraction and bit masking.
Both shift operators in C offer significant performance benefits in scenarios requiring low-level data manipulation, such as in embedded systems, network protocols, and performance-critical applications. Mastery of these operators enhances your ability to perform complex bitwise shift operations, optimize code performance, and induce code versatility.
Frequently Asked Questions
Q. What are shift operators in C?
Shift operators in C are used to manipulate the individual bits of integer data types. These operators allow you to shift the bits of a variable to the left or right, which can be used for a variety of low-level programming tasks.
There are two types of shift operators in C i.e. Left shift operator and Right shift operator. Shift operators in C are crucial for tasks like bitwise manipulations, bit operations, efficient arithmetic operations, and data encoding.
Q. What is the difference between logical and shift operators?
Logical operators and shift operators serve different purposes in C programming. Here’s a comparison of the two:
- The primary purpose of logical operators, such as AND (&&), OR (||), and NOT (!), is to assess boolean expressions in order to ascertain true or false results predicated on logical circumstances.
- Conversely, bit shift operators in C, such as left shift (<<) and right shift (>>), modify the binary sequence of integers by moving their bits to predetermined places to the left or right.
- Essentially, built-in shift operators in C alter binary sequence form representations, providing flexible tools for various programming requirements, whereas logical operators evaluate boolean conditions.
- Understanding the differences between binary bitwise operators makes manipulation more accurate and efficient.
Q. What happens when you shift bits beyond the size of the data type?
When shifting bits beyond the size of the data type, the behavior of shift operators in C is undefined or implementation-defined. In most cases, only the least significant bits of the shift amount are used. So shifting by a number of larger value than the bit-width of the type results in the same effect as shifting by the remainder of the shift amount divided by the number of bits in the data type.
Example:
int a = 5; // Binary sequence: 00000000 00000000 00000000 00000101
int b = a << 33; // Effective shift amount: 33 % 32 = 1
printf("%d\n", b); // Output will be 10
Q. What is right shift equal to?
The right shift operator (>>) in C is equivalent to dividing the left-hand operand by 2 raised to the power of the right-hand operand. In mathematical terms, the right shift operation x >> n is equivalent to the integer division of x by 2.
This operation shifts the bits of the integer to the right by the specified number of bit positions (n). If the integer is positive, it is akin to performing integer division by 2^n.
For negative integers, the behavior of shift operators may vary depending on the compiler; it might perform an arithmetic right shift, preserving the sign bit and effectively dividing the value by 2^n.
Q. List down the advantages of binary shift operators in C.
Binary shift operators offer several advantages, including enhanced performance, efficient multiplication and division by powers of two, and precise low-level data manipulation. They allow for rapid bit shift operations directly supported by hardware, making them faster than traditional arithmetic operations. Shift operators are essential for tasks such as bit masking, compact data storage, cryptographic algorithms, and control of hardware components in embedded systems.
Q. How do you calculate the left shift assignment operator?
The left shift assignment operator (<<=) is utilized to perform a left shift operation on a variable's binary representation and then assign the result back to the variable.
- This binary operator moves each bit in the variable's binary representation left by a predetermined number of places.
- Then, for every shift, a zero is added to the least significant bit (LSB), and the most significant bits (MSBs) are moved out.
- In the case of int x = 5; for instance, the expression x <<= 2; would assign x the non-negative value 20, since the binary code of 5 (0000 0101) is left-shifted by 2 positions to become 0001 0100, which is decimal equivalent to 20.
Q. What is the difference between left shift and right shift operators in C?
The left shift operator (<<) and the right shift operator (>>) are both bitwise shift operators in C used for manipulating the binary representation of integers. Here are the key differences between these two shift operators in C:
Aspect | Left Shift Operator (<<) | Right Shift Operator (>>) |
---|---|---|
Operation | Shifts bits to the left | Shifts bits to the right |
Mathematical Effect | Multiplies the number by 2^n | Divides the number by 2^n |
Bit Movement | Moves bits left; new positions on the right are filled with zeros | Moves bits right; new positions on the left are filled with zeros for unsigned integers, or the sign bit is extended for signed integers |
Syntax | result = value << shift_amount; | result = value >> shift_amount; |
Common Uses | Fast multiplication by powers of two, bit masking, setting specific bits | Fast division by powers of two, extracting bits, and bit manipulation techniques |
Effect on Value | Increases the value | Decreases the value |
Overflow | Shifting left can cause overflow if bits move beyond the type’s bit-width | Shifting right can cause truncation of bits, especially in signed integers |
Sign Handling | Does not affect the sign bit position | For signed integers, it can perform an arithmetic shift (sign extension); for unsigned integer types, it is a logical shift (fills with zeros) |
Here are a few other topics you might be interested in:
- Structure In C | Create, Access, Modify & More (+Code Examples)
- Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
- 5 Types Of Literals In C & More Explained With Examples!
- Break Statement In C | Loops, Switch, Nested Loops (Code Examples)
- Tokens In C | A Guide For All Types & Their Uses (With Examples)
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment