Static Variable In C | Declare, Use, Features & More (+Examples)
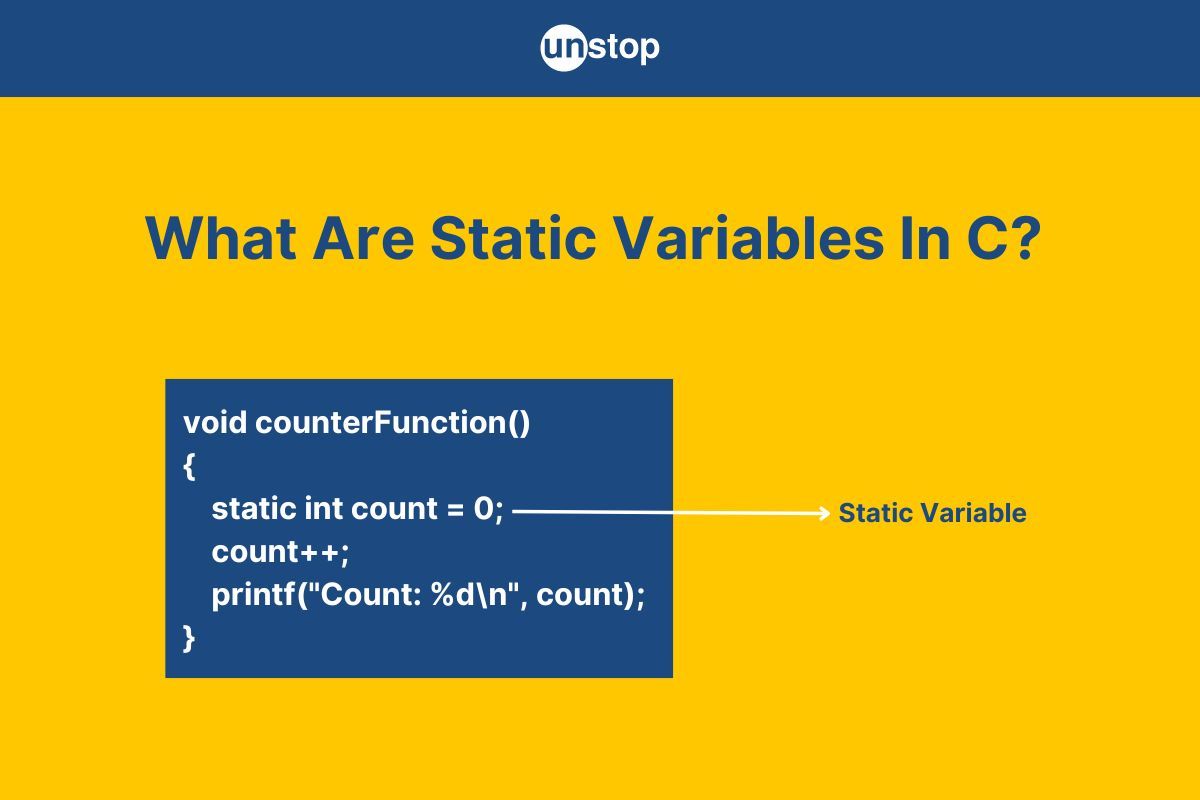
Static variable in C programming language is a powerful feature that allows a variable to maintain its value across multiple function calls and throughout the lifetime of a program. Unlike local variables, which are reinitialized each time a function is called, static variables retain their value between function calls. This makes them particularly useful for tasks that require persistent state information.
They enable flexibility, as they can hold several types of data such as numbers, characters, or even complex data structures like arrays or objects. In this article, we will explore static variables in C, covering their definition, usage, and key characteristics. By the end of this article, you will have a clear understanding of how static variables work and when to use them in your C programming projects.
What Is A Static Variable In C?
A static variable in C programming is a type of variable that retains its value even after the previous scope in which it has been declared has been exited. This means that a static variable persists for the lifetime of the program, maintaining its value across multiple function calls.
Static variables can be declared within a function (local scope) or outside of all functions (file scope). Unlike automatic variables, static variables are initialized only once during the program's execution and maintain their value between function calls.
Real-Life Examples:
- Consider the scenario of a banking application where a function keeps track of the number of transactions made by a user. A static variable within this function could store and increment the transaction count each time the function is called, maintaining an accurate record across multiple interactions.
- Another practical example lies in a weather monitoring system. A function that calculates the average temperature over time might utilize a static variable to store and update the cumulative temperature and the count of readings, allowing for accurate computations without losing previous data.
Declaration & Initialization Of Static Variable In C
The process of declaring and initializing a static variable in C involves specifying the static keyword along with the variable's data type and optionally providing an initial value. Unlike automatic variables, static variables are initialized only once during the program's execution and maintain their value between function calls.
Declaration Of Static Variable In C
Static variables in C are declared using the static keyword. When a static variable is declared, it tells the compiler to allocate memory for the variable in the data segment of the program. The scope of the static variable is determined by its position in the code.
Syntax:
static data_type variable_name;
Here,
- static: It is the keyword indicating the storage class.
- data_type: It represents the type of the variable (e.g., int, char, float).
- variable_name: It is the name of the variable.
Initialization Of Static Variable In C
When a static variable in C is initialized, it is assigned an initial value by the user. If no initial value is provided, the static variable is automatically initialized to zero. This process of initialization happens only once when the program starts, and the variable retains its value throughout the program's execution.
Syntax:
static data_type variable_name = initial_value;
Here, we will declare a static variable with a specific data type and initialize it with a value.
Note: It's important to note that the declaration and initialization of static variables can happen separately in C. Initialization is optional; if not explicitly initialized, the static variable is set to zero (0 for numeric data types, NULL for pointers, etc.).
Let's look at a code example that showcases the usage of a static variable within a function and demonstrates how its value persists across multiple function calls.
Code:
Output:
Count: 1
Count: 2
Count: 3
Explanation:
In the above code example-
- We start by including the standard input-output library using #include <stdio.h> , to use output function printf().
- Next, we define a function called countFunction(). Inside this function, we declare a static integer variable named count and initialize it to 0.
- In the next line, we increment the count variable by 1 using the increment operator (++) and print its value using the printf() function.
- The format specifier %d is the placeholder for an integer value, and the newline escape sequence (\n) adds a new line after the output.
- Next, inside the main() function, we call countFunction() three times in succession. Each call to countFunction() increments the count variable and prints the current count value.
- Since count is declared as static, its value is preserved between function calls.
- As a result, the first call to countFunction() prints Count: 1, the second call prints Count: 2, and the third call prints Count: 3.
- Finally, the main() function returns 0, indicating successful execution of the program.
Static Keyword In C & Its Uses
The static keyword in C is used to define the scope and lifetime of variables and functions. It holds significant importance and its usage varies based on the context within the code:
- Retention of Values: By default, variables in C have local scope and lose their values once their defining function exits. However, when static is applied to a variable or function, it enables them to retain their values between different calls or executions. This ensures the preservation of their last assigned value across various function invocations.
- Controlled Scope: Static keyword also regulates the scope of variables or functions. It confines the visibility of entities within a specific block, function, or file, limiting their accessibility from other scopes. This facilitates encapsulation and restricts access to specific contexts, promoting better code organization.
- Efficient Memory Management: The static keyword aids in managing memory efficiently. It allocates memory for static variables during the program's startup and retains it throughout the entire program's execution. This differs from dynamic memory allocation, which occurs during runtime.
- Static Global Variable: A static global variable is a global variable declared with the static keyword. This variable cannot be accessed from other files due to its file-level scope. Hence it provides file-level encapsulation and prevents external linkage. We will discuss it in detail in the sections below.
- Static Local Variable: A static local variable is a variable declared within a function using the static keyword. It retains its value between function calls, meaning it is initialized only once and persists for the lifetime of the program. We will discuss it in detail in the sections below.
- Static Function: When we use the static keyword in function definition, it restricts its visibility to the file in which it is declared. This ensures that the function cannot be called from other files, providing encapsulation at the file level.
- Static Method: In C++, static methods are functions that belong to the class itself rather than to any specific static object. They can be called on the class itself without needing an instance of the class, and they do not have access to the non-static members of the class.
- Static Member Variables: In C++ programming language, static member variables are shared among all instances of a class. They are not tied to any specific instance, allowing class-wide data management. This feature is used to keep track of information relevant to the class itself. For more, read: Static Data Member In C++ | An Easy Explanation (With Examples)
How To Use Static Variables In C?
Static variables in C have several uses, each serving different purposes depending on where they are declared and how they are utilized. Below, we will explore the different ways to use static variables in C:
Using Static Variable In C To Declare Global Variable
A global variable is a variable declared outside any function and can be accessed and modified by any function within the same file or multiple files (if declared as extern). Using static variables to declare global variables within a single file is a way to limit the scope of these variables to that specific file.
When a static global variable is declared in a file, it cannot be accessed directly by other files using the extern keyword. Instead, it is restricted in scope to only the functions within that file, behaving similarly to a global variable but confined within the file boundaries.
Let's look at a basic program on static global variable in C:
Code:
Output:
Global Variable inside function1: 20
Global Variable in main: 20
Explanation:
In the above code example-
- We start by declaring a static global variable named globalVar and initializing it to 20. The static keyword here means that globalVar is only accessible within this translation unit, which is usually a single source file. It remains global to the file but is not visible to other files that might be linked with this one.
- Next, we define a function called function1(). Inside this function, we use printf() to print the value of globalVar. Since globalVar is declared as static, it can still be accessed within this function.
- In the main() function, we first call function1(). This call prints the value of global variable inside the function1() as 20 because globalVar is 20.
- After calling function1(), we use printf() again to print the value of globalVar from within the main function. The output will be 20 as well, since the globalVar value has not changed.
Using Static Variable In C For User-defined Function
When static variables are declared within user-defined functions, they exhibit a unique behavior distinct from automatic or local variables. A static variable declared inside a function retains its value between function calls. It is initialized only once and maintains its state throughout the program’s execution, which is useful for counters or accumulators.
Code:
Output:
Local Variable: 5
Local Variable: 6
Local Variable: 7
Explanation:
In the above code example-
- We begin by defining a function named staticVariableDemo(). Inside this function, we declare a static local variable named localVar and initialize it to 5.
- The static keyword ensures that localVar retains its value between function calls rather than being reinitialized each time the function is executed.
- Inside staticVariableDemo(), we use printf() function to print the current value of localVar. After printing, we increment localVar by 1. This means that the next time staticVariableDemo() is called, localVar will have its value updated from the previous call.
- Now in the main() function, we call staticVariableDemo() three times in sequence.
- During the first call, localVar is initialized to 5 and printed to the console. After incrementing, localVar becomes 6.
- In the second call, localVar retains its updated value from the previous call, which is 6. Thus, it is printed to the console and then incremented to 7.
- During the third call, localVar again retains its updated value, which is now 7. So it is printed to the console and then incremented to 8.
- Finally, the main() function returns 0, indicating successful execution of the program.
Using Static Variable In C Outside Main Function
Declaring a static variable outside the main() function limits its scope to the file in which it is declared, while its lifetime persists for the entire duration of the program. This use of static provides file-level encapsulation for global data.
Code:
Output:
Outside Main Variable: 100
Explanation:
In the above code example-
- We start by declaring a static variable named outsideMainVar and initialize it to 100. This variable is declared outside of the function, making it a global variable. However, the static keyword limits its visibility to the current translation unit, meaning it can only be accessed within this source file, not from other files.
- We then define a function named displayVar(). Inside this function, we use printf() to print the value of outsideMainVar. Even though outsideMainVar is static and declared outside of main, it is still accessible within displayVar because they are in the same file.
- In the main() function, we call displayVar() to print the value of outsideMainVar. The output will print the value as 100 because outsideMainVar is 100.
Local Variable Vs. Static Variable In C
The table below highlights the key differences between a local variable and a static variable in C programming.
Feature | Local Variable | Static Variable |
---|---|---|
Scope | Limited to the function or block where declared. | Limited to the function or source file where declared. |
Lifetime | Exists only during the execution of the function or block. | Exists for the entire duration of the program. |
Initialization | Initialized every time the function or block is entered. | Initialized only once, retains value between function calls. |
Memory Allocation | Allocated on the stack. | Allocated in the data segment. |
Default Value | Not automatically initialized, may contain garbage value if not explicitly initialized. | Automatically initialized to zero if not explicitly initialized. |
Access Across Calls | Does not retain its value between function calls. | Retains its value between function calls. |
Code:
Output:
Inside main function:
Local Variable: 5, Static Variable: 10
Local Variable: 5, Static Variable: 11
Explanation:
In the above code example-
- We start by defining a function called functionExample(). Inside this function execution, we declare a single variable named localVar and initialize it to 5.
- This variable is a local variable, meaning it is only accessible within this function and gets reinitialized each time the function is called.
- We also declare a static integer variable named staticVar and initialize it to 10. Unlike local variables, static variables retain their value between function calls due to the static keyword.
- Next, inside the function, we use printf() to display the values of localVar and staticVar.
- After printing, we increment both localVar and staticVar by 1 using the increment operator(++). This updates their values for the next time the function is called.
- Inside the main() function, we first print the statement "Inside main function:\n". We then call the functionExample() twice.
- During the first call, localVar is 5 and staticVar is 10, which is reflected in the output. After incrementing, localVar becomes 6 and staticVar becomes 11.
- During the second call to functionExample(), localVar is reinitialized to 5, while staticVar retains its value from the previous call, which is now 11.
- After this call, localVar increments to 6, and staticVar increments to 12.
Check out this amazing online course to become the best version of the C programmer you can be.
Global Variable Vs. Static Variable In C
The key differences between a global variable and a static variable in C are as follows:
Feature | Global Variable | Static Variable |
---|---|---|
Scope | Accessible from any file if declared with extern or if no static keyword is used. | Accessible only within the function or file where declared. |
Lifetime | Exists for the entire duration of the program. | Exists for the entire duration of the program. |
Initialization | Automatically initialized to zero if not explicitly initialized. | Automatically initialized to zero if not explicitly initialized. |
Memory Allocation | Allocated in the data segment of memory, used for global and static data. | Allocated in the data segment of memory, used for global and static data. |
Default Value | Default value is zero if not explicitly initialized. | Default value is zero if not explicitly initialized. |
Access Across Files | Can be accessed across multiple files if declared with extern. | Limited to the file (if declared at file scope) or function (if declared in a function). |
Code:
Output:
Inside main function:
Global Variable: 100, Static Variable: 10
Global Variable: 110, Static Variable: 15
Explanation:
In the above code execution-
- We start by declaring a global variable named globalVar and initializing it to 100. This variable is global, meaning it is accessible from any function within the program.
- We then define a function called functionExample(). Inside this function, we declare a static integer variable named staticVar and initialize it to 10. The static keyword ensures that staticVar retains its value between calls to functionExample().
- Within functionExample(), we use printf() to print the values of globalVar and staticVar.
- After printing, we update globalVar by adding 10 to its current value and staticVar by adding 5.
- In the main() function, we first print the statement "Inside main function:\n". We then call functionExample() twice.
- During the first call, globalVar is 100 and staticVar is 10, as reflected in the output. After updating, globalVar becomes 110 and staticVar becomes 15.
- During the second call to functionExample(), globalVar retains its value from the previous call, which is now 110, and staticVar also retains its value, which is now 15. We print these values to the console.
Key Features Of Static Variables In C
Static variables in C have several distinct features and special properties that affect their behavior and usage in programs.
- Default Initialization: Static variable in C is automatically initialized to zero (eg: auto variable=0) if no other value is specified during their declaration.
- Static Variables & Structures: Static variable in C can be part of entire structure elements but they must be defined outside of the structure definition. These types of variables cannot be declared inside a structure directly. For example-
struct Example {
int data;
};static int count = 0; // Static variable outside the structure
void incrementCount(struct Example *ex) {
count++;
ex->data = count;
}int main() {
struct Example ex1, ex2;
incrementCount(&ex1);
incrementCount(&ex2);
printf("ex1.data: %d\n", ex1.data); // Output will be 1
printf("ex2.data: %d\n", ex2.data); // Output will be 2
return 0;
}
- Maintaining State Information in Functions: Static variables within function implementation retain their value between function calls, allowing the function to remember its state. For Example-
void counter() {
static int count = 0;
count++;
// use count
}
- Improving Efficiency in Loop Constructs: Static variables can optimize performance by avoiding repeated initialization inside loops or functions. For Example-
void initializeOnce() {
static int initialized = 0;
if (!initialized) {
// Perform initialization
initialized = 1;
}
}
- Safeguarding Sensitive Information: Consider a safe deposit box within a room. Static variables serve as this secure space, allowing storage of critical information like encryption keys within the function's confines. This ensures data security, preventing unauthorized access or tampering from external sources.
- Data Logging and Debugging: In the process of debugging code, static variables can act like temporary markers, aiding in tracking and monitoring specific variable values or conditions during program execution. They serve as helpful tools for developers to observe and understand program behavior without altering the code structure.
- Efficient Error Handling: Imagine handling errors like organizing a toolbox. Static variables assist in storing and managing error codes or statuses across function calls. This makes error handling more streamlined, helping propagate and resolve issues more efficiently within the program.
- Controlled Scope and Lifetime Management: Static variables confine their scope and lifetime within a function or block, offering controlled access and ensuring data persistence as long as the program executes.
- Implementing Singleton Patterns: Static variables play a vital role in implementing the Singleton design pattern, ensuring that only one instance of a class or object exists throughout the program's execution.
- Thread Safety Implementation: In multithreaded applications, static variables can be utilized in implementing thread-local storage, allowing each thread to maintain its unique copy of data.
- Enhancing Security Measures: Static variables can be leveraged to implement security measures by storing and managing sensitive information securely within a function's scope.
Memory Allocation For Static Variable In C
In C programming, memory allocation for static variables occurs at the program's startup and remains constant throughout its execution. Static variables in C are allocated in the data segment of the memory. This segment is divided into two parts:
- Initialized Data Segment: This part holds the static variables that are explicitly initialized by the programmer.
- BSS (Block Started by Symbol) Segment: This part holds the static variables that are not explicitly initialized (i.e., they are zero-initialized by default).
Declaration & Memory Allocation Of A Static Variable In C
When a static variable is declared, the compiler sets aside memory for it in the data segment of the program. This is different from local variables, which are allocated on the stack.
-
Inside a Function: The declaration of a static variable inside a function tells the compiler to allocate memory for the variable that persists across multiple function calls. The variable is not reallocated or reinitialized each time the function is called.
void exampleFunction() {
static int count; // Memory allocated in data segment
}
- Outside a Function: A static int variable declared outside any function (file scope) is similarly allocated in the data segment. It is globally accessible within the file but not from other files.
static int globalCount; // Memory allocated in data segment
Initialization & Memory Allocation Of A Static Variable In C
Initialization of a static variable in C occurs only once, regardless of how many times the variable comes into scope.
-
Explicit Initialization: If a static variable is explicitly initialized, it is set to the given value at the start of the program. This value is stored in the initialized data segment.
static int count = 10; // Explicitly initialized, stored in initialized data segment
- Implicit Initialization: If no initialization is provided, the static variable is automatically initialized to zero. This value is stored in the BSS (Block Started by Symbol) segment.
static int count; // Implicitly initialized to 0, stored in BSS segment
Lifetime And Scope Of A Static Variable In C
The lifetime of a static variable in C spans the entire execution time of the program. The scope of a static variable determines where the normal variable can be accessed or modified within a program. This means that once a static variable is allocated and initialized, it remains in memory and retains its value(scope) until the program terminates.
Advantages & Disadvantages Of Static Variable In C
Let's explore some of the important advantages as well as disadvantages of static variables in C programming.
Advantages Of Static Variable In C
- Persistence: Static variable in C can retain its values between function calls. This is useful when maintaining state information across multiple function invocations without using global variables.
- Scope Control: Static variable in C has a limited scope to the block defined by it, reducing the risk of unintended modifications from other parts of the program.
- Data Hiding: By restricting the visibility of static variables to the file or function where they are declared, we can achieve better encapsulation, making our code more modular and easier to manage.
- Encapsulation: Static variable in C can help encapsulate data (hidden variable) within a function or file, limiting scope and reducing the risk of unintended interactions with other parts of the program.
- Memory Efficiency: Since static variables are allocated memory only once, regardless of how many times the function is called, they can be more memory-efficient compared to local variables which are re-allocated on each function call.
- Initial Value: Static variable in C is automatically initialized to zero (for numeric types) if no explicit initializer is provided, ensuring they have a known starting value.
Disadvantages Of Static Variable In C
-
Limited Lifetime: Although static variables persist for the lifetime of the program, they do not get reinitialized automatically on each function call, which may lead to unexpected behavior if not managed properly.
-
Memory Consumption: Static variable in C occupies memory throughout the execution of the program, which might lead to higher memory requirements as compared to local variables that are created and destroyed as needed.
-
Testing Challenges: Static variables in C can make unit testing more challenging because their state is maintained between function calls, making it harder to isolate tests and ensure they don't affect each other.
- Limited Visibility: Static variables declared outside functions have limited visibility, making them accessible only within the file they are declared in.
-
Concurrency Issues: In a multi-threaded environment, accessing static variables can lead to race conditions if proper synchronization mechanisms (like mutexes) are not used.
-
Less Flexibility: The static variable in C is less flexible than dynamic memory allocation, where memory can be allocated and freed as needed, allowing more control over dedicated memory segment.
Conclusion
Static variables in C offer extensive features that make them indispensable for certain programming concepts. Their persistent lifetime allows them to retain values across function calls and throughout the entire program execution. By providing a controlled scope, static variables enable code encapsulation and code reuse which is crucial for managing data privacy and reducing the risk of unintended side effects.
The versatility of static variables is evident in their various applications, from maintaining state within a single function to restricting global variable access to a single file. These attributes make static variables particularly useful for tasks like implementing counters, managing internal states, and ensuring quality of code and data consistency in multi-file programs.
Frequently Asked Questions
Q. What is the difference between static variable and dynamic variable in C?
The table below highlights the key differences and uses of static and dynamic variables in C.
Aspect | Static Variable | Dynamic Variable |
---|---|---|
Lifetime | Retains value for the duration of the program. | Exists until explicitly freed. |
Scope | Limited to the function or file where declared. | Can be accessed via pointers from any function. |
Initialization | Automatically initialized to zero if not explicitly initialized. | Must be explicitly allocated and initialized. |
Memory Location | Allocated in the data segment. | Allocated on the heap. |
Declaration | Declared using the static keyword. | Allocated using functions like malloc(), calloc(), realloc(). |
Memory Management | Managed by the compiler. | Managed manually by the programmer. |
Use Case | Maintaining state between function calls, file-level encapsulation. | Handling variable-sized data, runtime memory allocation. |
Snippet | static int count = 0; | int *ptr = (int *)malloc(sizeof(int) * 10); |
Q. Why use static variable in C?
Static variable in C serves various purposes as follows:
- Maintaining state information across function calls.
- Limiting the variable scope to the block or function where it is declared.
- Retaining values throughout the program execution.
- Optimizing segment memory by allocating memory space only once for a static variable.
Q. What is the lifetime of a static variable in C?
The lifetime of a static variable in C begins when the program starts execution and ends when the program terminates. Static variables persist throughout the program execution, maintaining their values across function calls.
Q. What is the difference between a static variable inside a function and a static variable outside a function?
The key differences between a static variable declared inside a function and one declared outside a function are as follows:
Aspect | Static Variable Inside a Function | Static Variable Outside a Function |
---|---|---|
Scope | Limited to the function where it is declared. | Limited to the file where it is declared. |
Lifetime | Persists for the entire program execution. | Persists for the entire program execution. |
Initialization | Initialized once, retains value between function calls. | Initialized once, retains value throughout the entire code. |
Visibility | Not accessible outside the function. | Not accessible from other code files, only within the declaring file. |
Usage Example | Maintaining state between function calls. | File-level encapsulation, module-specific global state. |
Snippet | void func() { static int x; } | static int x; (at file scope) |
Q. Where are static variables stored in C?
Static variables in C are stored in the data segment of the memory. Specifically, they are stored in either the initialized data segment (if they are explicitly initialized) or the uninitialized data segment (also known as the BSS segment, for "Block Started by Symbol") if they are not explicitly initialized. This memory allocation persists for the entire duration of the program, ensuring that static variables maintain their values across function calls and throughout the program execution.
Q. What is the difference between a constant variable and a static variable in C?
A constant variable, declared using the const keyword, is a regular variable whose value is set at the time of initialization and cannot be modified thereafter. This ensures that the value remains unchanged throughout the program.
On the other hand, a static variable, declared with the static keyword, has a persistent lifetime that spans the entire duration of the program. While its value can be modified, the static variable anytime retains its value between function calls if declared within a function, or maintains file-level scope if declared globally.
Here are a few other interesting topics you must know about:
- C++ Type Conversion & Type Casting Demystified (With Examples)
- Pointers in C++ | A Roadmap To All Types Of Pointers With Examples
- Inline Function In C++ | Declare, Working, Examples & More!
- Array In C++ | Ultimate Guide On Creation, Types & More (Examples)
- Static Member Function In C++: How to Use Them, Properties, & More
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Comments
Add comment