Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Python List | Everything You Need To Know (With Detailed Examples)
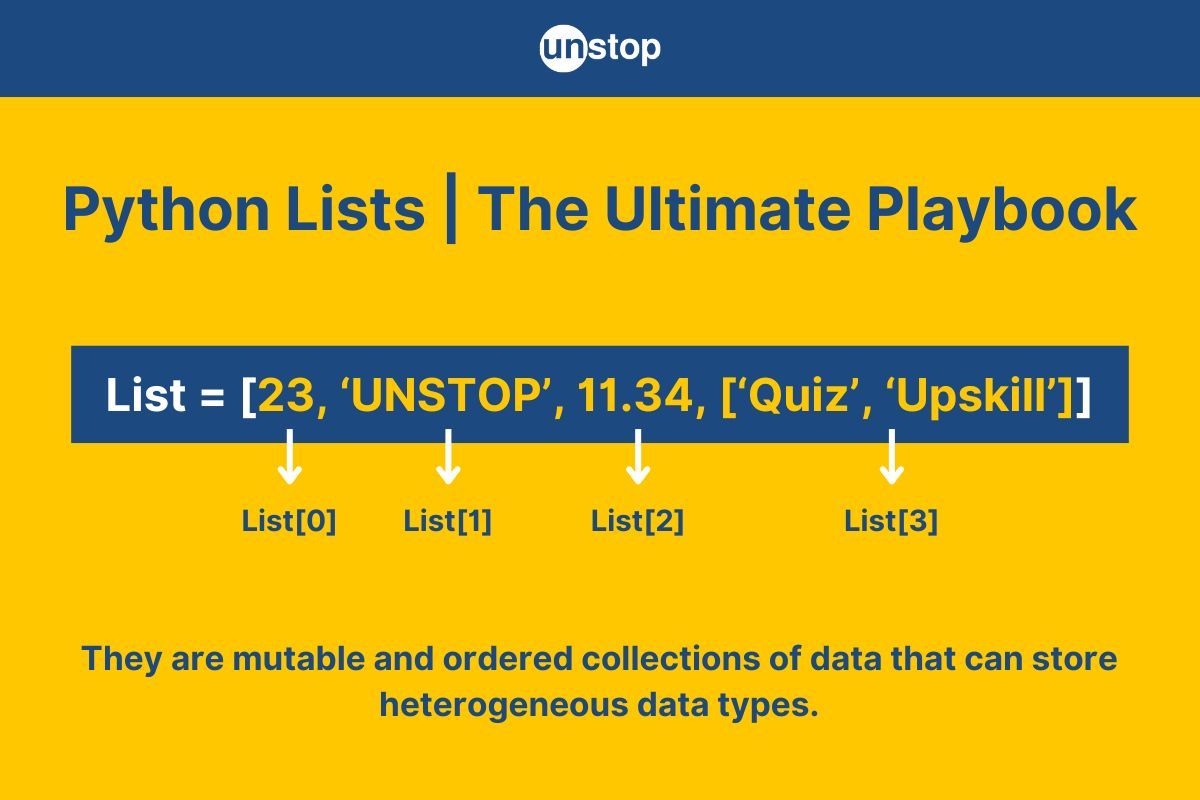
Data structures are essential for storing, organizing, and manipulating data in programming. Multiple types of data structures exist, each with its own set of characteristics, benefits, operations, etc. In this article, we will discuss a powerful tool/ data structure in the Python programming language, namely, the Python list.
Among the various robust data structures Python offers, lists stand out due to their versatility and ease of use. Python lists are one of the most frequently used data structures as they allow you to store and manipulate collections of items of different data types, ranging from numbers and strings to other lists and complex objects. You must have a clear understanding of Python list creation, manipulation, and various functionalities to harness their power when writing your Python programs.
Introduction To Python List
A Python list is an ordered and mutable collection of items. These collections of items can comprise elements of various data types, such as integers, strings, floats, and even other lists. This flexibility makes Python lists incredibly useful for a wide range of applications, from simple data storage to complex data manipulation tasks.
Real-Life Example: Consider a grocery shopping list. You might need to keep track of different items you need to buy, such as fruits, vegetables, dairy products, and snacks. A Python list provides an easy way to not only organize this information but also add new items, remove purchased items, and access specific items based on their position in the list.
grocery_list = ["apples", "bananas", "carrots", "milk", "bread"]
In this example, grocery_list is a Python list containing five string type elements, each representing an item on the shopping list. Note that the collection of elements is enclosed in square brackets. Now, let's look at common list features in Python, after which we will explore how to create these Python lists and perform various operations on them.
Characteristics Of Python Lists
Understanding the basic list features is the key to leveraging their full potential. Here are the important characteristics of lists:
- Ordered: Python lists preserve the order of elements in which they are added. That is, each element has a specific position within the list.
- Mutable: Python lists are mutable, meaning the list elements can be changed after the list is created. In other words, you can add, remove, or modify elements as needed.
- Dynamic: Python lists can grow and shrink dynamically, meaning you don't have to declare the size of a list in advance. This is because you can add or remove elements at any time.
- Heterogeneous: A single list can contain elements of different data types. That is, a Python list can have collection data types ranging from integers, floats, strings, lists, other objects, etc.
- Indexed: Elements in a Python list can be accessed using their index, with indexing starting at 0 for the first element. Negative indexing allows access to elements from the end of the list.
- Slicing: Python lists support slicing, which allows you to slice a section or subset of a list and access its elements.
- Iterable: Lists are iterable, meaning you can loop through the elements using loops such as for loops or comprehensions.
- Comprehensions: Python lists support list comprehensions, which provide a concise way to create lists based on existing lists through a clear and readable syntax.
- Built-in Methods: Lists come with a variety of built-in methods that facilitate different operations of list like adding, removing, sorting, and reversing elements.
- Nested Lists: Lists can contain other lists as elements, allowing the creation of complex data structures such as matrices or lists of lists.
How To Create A Python List?
The most common method we use for creation/ list declaration in Python programming language is list iterals. However, we can also use list comprehension to create a list from an iterable range. Each of these methods to create Python lists is suited for different scenarios and needs. We will discuss all of these in detail in this section.
Python Create Lists Using List Literals
List declaration in Python List literals is the most straightforward way to create a list in Python. In this approach, we provide the name of the list and then directly define/assign the elements to a list within square brackets.
Basic Syntax For List Declaration In Python:
list_name = [element1, element2, element, ...]
Here,
- The term list_name refers to the name you want to give to the list.
- The square brackets [] enclose the collection of items separated by commas, which are given by terms element1, element2,... and so on.
Let's look at a simple Python program example that illustrates how lists are created and printed in Python.
Code Example:
# Creating a list using list literals
fruits = ["apple", "banana", "cherry"]
#Printing the list to the console
print(fruits)
Output:
['apple', 'banana', 'cherry']
Explanation:
In the simple Python code example-
- We first create a list named fruits using list literals, i.e., square brackets [].
- While declaring the list, we also assign the list of items (three string data type values) inside the square brackets.
- This creates a list containing these three string values in the order in which they were listed.
- Next, we use the print() function to display the elements in the string list to the console.
Create Python Lists Using List Comprehension
List comprehension is a concise way to create Python lists. It has a compact syntax that allows you to generate a new list by applying an expression to each element in an existing iterable range, list, tuples, etc. You can also filter elements from the iterable by providing a conditional statement.
Syntax:
[expression for item in iterable if condition]
Here,
- The term expression defines how to evaluate/ calculate each element given by the term item.
- Iterable is the collection of items to be iterated over to find the elements, and the if condition (optional) defines how to filter elements.
- The for keyword marks the loop construct used for iteration.
Let's look at an example Python program that illustrates the creation of Python lists using list comprehension.
Code Example:
# Creating a list using list comprehension
squares = [x**2 for x in range(5)]
#printing the list to the console
print(squares)
Output:
[0, 1, 4, 9, 16]
Explanation:
In the example Python code-
- We declare a list variable called squares and initialize it using list comprehension.
- It requires the program to iterate over each element x in the range 0 to 4, i.e., range(5).
- For each x in the range, the list comprehension's iteration process computes the square of x, i.e., x**2, adding it as an element of the list, creating a list of squares.
- This list is stored in the list variable square, which we then print to the console using the print() function.
Note: Alternatively, we can also create Python lists by converting other iterables like tuples, strings, sets, etc., using the list() built-in function.
How To Access Elements Of Python List?
Once you have created a list, it is important for you to be able to access elements of a Python list to perform operations on the data. In other words, accessing elements from a list in Python is fundamental for retrieving and manipulating data stored in sequential order. There are two primary methods to access elements: basic list indexing and negative indexing.
Access List Elements Using Basic List Indexing
Basic list indexing involves accessing elements by their position (index) in the list. Indexing in Python starts at 0 for the first element and increases sequentially. This is also known as zero-based indexing.
To access components/ items of a list using the index, we must specify the position number inside square brackets after the list name.
Syntax:
element = list_name[index]
Here,
- The term list_name is the identifier/ name of the list whose elements we want to access.
- The [index] is the position number, and the respective item is stored in the variable element.
Given below is a Python program example illustrating this concept for better understanding.
Code Example:
# Example list
numbers = [10, 20, 30, 40, 50]
# Accessing elements using basic list indexing
first_element = numbers[0]
third_element = numbers[2]
last_element = numbers[-1]
# Print accessed elements
print("First Element:", first_element)
print("Third Element:", third_element)
print("Last Element:", last_element)
Output:
First Element: 10
Third Element: 30
Last Element: 50
Explanation:
In the Python code example-
- We create a Python list called numbers using list literals. This list contains five integers: 10, 20, 30, 40, and 50.
- Next, as mentioned in the code comments, we use list indexing to access elements at different positions, as follows:
- We access the element at the 1sh position and store it in the first_elements variable, i.e., first_element = numbers[0].
- Then, elements at the 3rd and (-1)-th/ last position are stored in variables third_element and last_element, respectively.
- Finally, we print these values with a string message describing the element number to the console using a set of print() statements.
Access Python List Elements Using Negative Indexing
The concept of indexing and using indexes to access elements remains the same in this approach. The main distinction is that we use negative indexes, i.e., the indexing starts from the last element.
So, index number -1 indicates the element at the last position; index -2 represents the element at the second-to-last position, and so on. Its syntax follows the same general elements as in concise syntax for basic indexing.
Syntax:
element = list_name[-index]
Here, the difference to more is that the index numbers are negative. Let's look at a sample Python program to illustrate the concept of negative indexing to access list elements.
Code Example:
# Example list
numbers = [10, 20, 30, 40, 50]
# Accessing elements using negative indexing
last_element = numbers[-1]
second_last_element = numbers[-2]
first_element = numbers[-len(numbers)]
# Print accessed elements
print("Last Element:", last_element)
print("Second Last Element:", second_last_element)
print("First Element (with negative indexing):", first_element)
Output:
Last Element: 50
Second Last Element: 40
First Element (with negative indexing): 10
Explanation:
In the sample Python code-
- We first create a Python list called numbers with the values [10, 20, 30, 40, 50].
- Then, we demonstrate how to access elements from this list using negative indexing.
- We use indexes -1 and -2 to retrieve the last and second last elements and store them in variables last_element and second_last_element, respectively.
- To access the first element, we first use the built-in len() function to calculate the length/ total number of elements in the list.
- And then, we use this as the negative index value to access the first element, storing it in variable first_element, i.e., first_element = numbers[-len(numbers)].
- Lastly, we use a set of print() statements to display these values to the console along with a descriptive string message.
Accessing Multiple Elements From A Python List (Slicing)
The concept of slicing allows you to access multiple elements of a Python list. This powerful feature allows you to retrieve portions of lists (and other sequence data types like tuples, strings, etc.) or access a range of elements.
- You can slice a portion or multiple elements from a list and manipulate/ modify that data without affecting the original list.
- This is particularly useful for tasks such as extracting sublists, copying lists, reversing lists, etc.
Typically, you extract a portion of a list by specifying a start, stop, and step index. Slicing uses the colon (:) operator to specify the range of indices.
Syntax For Slicing Python Lists:
sublist = list_name[start:stop:step]
Here,
- The terms list_name and sublist refer to the names/ identifiers of the original list that we are slicing and the sliced portion of the list, respectively.
- The range of slicing is given by start and stop variables. The start variable defines the starting point for slicing (inclusive), and the stop defines the ending index of slicing (exclusive).
- If the start index is missing, the default beginning point is index 0, and if the stop index is missing, the default is the end list.
- The step index is optional, and it defines the interval to keep between elements you want to slice. Its default is 1.
The stop, start, and step indexes help customize the sliced portion of the list. The Python program sample below showcases the most straightforward implementation of the slicing method.
Code Example:
# Creating a list
numbers = [10, 20, 30, 40, 50, 60, 70, 80]
# Slicing elements from index 1 to 4
sublist = numbers[1:5]
print(sublist)
Output:
[20, 30, 40, 50]
Explanation:
In the Python code example/ sample-
- We first create a Python list called numbers using list literals and assign elements [10, 20, 30, 40, 50, 60, 70, 80] to it.
- Then, we use the index numbers to slice out a portion of the initial list and store the outcome in the variable sublist.
- The list slice is made starting from index position 1 (inclusive) and ending at index 5 (not included).
- So, the expression numbers[1:5] extracts/ slices the list with elements 20, 30, 40, and 50.
- We then display this sublist to the console using the print() function.
Access List Elements From Nested Python Lists
A nested list in Python is a type of list that contains other lists as its elements. This technique allows you to create multidimensional lists, such as matrices or grids, where a sublist can represent a row or a specific data group.
- Nested lists are naturally more complex data structures, and accessing elements in nested lists involves indexing multiple levels to retrieve the desired element.
- For example, if you want to access the nth element of a Python list, which itself is the mth element of the outer Python list, then you will use double index values inside individual square brackets.
Look at the syntax below and the basic Python program example to illustrate this approach.
Syntax:
element = list_name[index1][index2]
Here,
- The list_name and element refer to the list whose item we are accessing and the variable's name where the element is stored, respectively.
- Index 1 represents the element position in the outer list, and index 2 indicates the element position in the inner list element.
Code Example:
# Creating a nested list containing only list elementsÂ
nested_list = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
# Accessing an element in the nested list
element = nested_list[1][2]
print(element)
Output:
6
Explanation:
In the Python example-
- We create a nested Python list called nested_list, containing only list elements.
- The nested list contains three sublists: [1, 2, 3], [4, 5, 6], and [7, 8, 9], where each sublist represents a row of numbers.
- Next, we use indexes to access the 3rd element of the 2nd sublist within the nested list, i.e., nested_list[1][2].
- Index 2 represents the 3rd element, and index 1 represents the 2nd element.
- This refers to the element 6 in the second sublist [4, 5, 6], which we display using the print() function.
How To Change Elements In Python Lists?
Python lists are versatile containers as they are mutable, i.e., we can change the list elements by assigning new values (and perform multiple other operations on lists). We can change or replace list elements at specific indices or use slicing for bulk assignments.
Changing Single Element In Python List At Specific Index
In Python, changing a single element in a list is straightforward. You can directly assign a new value to a specific index using the syntax given below.
Syntax:
list_name[index] = new_value
Here, the syntax for accessing the element at a position (using the index) is the same as we discussed above; the only difference is that we assign a new_value to that position.
Code Example:
# Creating a list
numbers = [10, 20, 30, 40, 50]
# Changing the element at index 2
numbers[2] = 35
print(numbers)
Output:
[10, 20, 35, 40, 50]
Explanation:
In the Python code-
- We create a list called numbers with 5 elements (i.e., 10, 20, 30, 40, 50) using list literals.
- Then, we use the index value 2 to access the 3rd element in the list and assign the value 35 to it, i.e., numbers[2] = 35.
- This updates the element at index 2 from 30 to 35, which is shown in the output as we print the modified list.
Changing Multiple Elements In Python List Using Slicing
You can change multiple elements in a list simultaneously by using list slice method and assign new values to elements in that portion. In this approach, you will use the start and stop indexes to specify a range of elements and replace them with new values.
Syntax:
list_name[start:stop] = [new_values]
Here, the syntax for slicing is the same, and the expression [new_values] refers to the list of new values you want to replace in the list.
Code Example:
# Creating a list
numbers = [10, 20, 30, 40, 50]
# Changing elements from index 1 to 3
numbers[1:4] = [25, 35, 45]
print(numbers)
Output:
[10, 25, 35, 45, 50]
Explanation:
In the Python code example-
- We create a list named numbers using list literals containing five integers in the order- [10, 20, 30, 40, 50].
- Next, we change the elements from index 1 to index 3 of the initial list using slicing.
- We use the slicing operator to specify the index range, i.e., numbers[1:4].
- And then use the assignment operator to assign a new list of values, i.e., [25, 35, 45].
- This updates the elements from index 1 up to but does not include index 4. That is, elements 20, 30, and 40 are changed to 25, 35, and 45, respectively.
- After changing the elements, we print the contents of the numbers list using the print() function.
How To Add Elements To Python Lists?
There are several methods to add elements to a Python list, each serving different purposes and offering flexibility depending on the use case. We will discuss them in this section, along with examples.
The Append() Method To Add Element To Python List
The append() method adds a single element to the end of a list. This method modifies the original/ initial list in place and is commonly used to add a single item to a list.
Syntax:
list_name.append(element)
Here,
- The dot operator indicates that we are applying the append() function on the input list with the name list_name.
- The element refers to the value we want to add to the list.
Code Example:
# Creating a list
numbers = [10, 20, 30]
# Appending an element to the list
numbers.append(40)
print(numbers)
Output:
[10, 20, 30, 40]
Explanation:
In the Python code-
- We create a list called numbers and initialize it with three integer values using list literals, i.e., [10, 20, 30].
- Then, we use the append() function to add the value 40 to the end of the initial list.
- After that, we use the print() function to display the modified list as shown in the output window.
The Insert() Method To Add Element To Python Lists
The insert() method allows you to add an individual item/ element at a specific position in the list. For this, you must specify the position (index) where you want to add the element and the value you want to add. This method shifts the elements to the right of the specified index to make room for the new element.
Syntax:
list_name.insert(index, element)
Here, the insert() function takes two parameters: index to specify the position and element to specify the value you want to add.
Code Example:
# Creating a list
numbers = [10, 20, 30, 40]
# Inserting element at index 2
numbers.insert(2, 25)
print(numbers)
Output:
[10, 20, 25, 30, 40]
Explanation:
In the Python code-
- We create a list called numbers with four integer elements, using list literals methods, i.e., [10, 20, 30, 40].
- Next, we use the insert() function to insert an element 25 at the index 2 (3rd position).
- The call numbers.insert(2, 25) inserts 25 at 3rd position, modifying the initial list to [10, 20, 25, 30, 40], which is shown in the output window using print() function.
The extend() Method To Add Element To Python List
The built-in extend() method allows you to add multiple elements to the end of a list. It takes an iterable (like a list, tuple, or set) as the parameter and appends/ adds its elements to the initial list.
Syntax:
list_name.extend(iterable)
Here,
- The name of the input list to which you want to add elements is given by list_name.
- The values you want to add are contained in the iterable variable, which may be a list, tuple, or any iterable object.
Code Example:
# Creating a list
numbers = [10, 20, 30]
# Extending the list with another list
numbers.extend([40, 50, 60])
print(numbers)
Output:
[10, 20, 30, 40, 50, 60]
Explanation:
In the above Python code-
- We create a list named numbers containing three integers using list literals [10, 20, 30].
- Next, we use the extend() method to append a list of elements, i.e., numbers.extend([40, 50, 60]).
- This adds the elements [40, 50, 60] to the end, modifying the numbers list to [10, 20, 30, 40, 50, 60], as shown in the output window using the print() function.
List Concatenation Method To Add Elements To Python Lists
List concatenation combines two lists by joining them together into a new list. We use the concatenation operator/ the addition arithmetic operator (+) to join two input lists. This method creates a new list that includes elements from both original input lists.
Syntax:
new_list = list1 + list2
Here, list1 and list2 are the input lists you want to concatenate, and new_list is the concatenated list.
Code Example:
# Creating two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# Concatenating lists
combined_list = list1 + list2
print(combined_list)
Output:
[1, 2, 3, 4, 5, 6]
Explanation:
In the above Python code-
- We start by creating two lists called list1 and list2 using list literals, with elements [1, 2, 3] and [4, 5, 6], respectively.
- Next, we concatenate the initial lists using the concatenation operator (+) and store the new list in combined_list. It joins the elements of list1 followed by the elements of list2.
- The combined_list becomes [1, 2, 3, 4, 5, 6] which we display using the print() function.
Delete/ Remove Elements From Python Lists
As you must know, removing or deleting elements is an essential list operation needed for data manipulation and memory management. There are multiple ways to remove elements from Python lists, which we will discuss in this section.
The remove() Method To Delete Specific Item | Python Lists
The remove() is a built-in function in Python that deletes the first occurrence of a specified value from a list. For this, you must specify the value of the individual item you want to remove, and the function will remove it the first time it encounters the value.
Syntax:
list_name.remove(value)
Here,
- The value is the parameter inside the remove() function, representing the data/ individual item you want to delete
- The list_name is the identifier/name of the respective list.
Code Example:
# Original list
numbers = [1, 2, 3, 4, 3, 5]
# Remove specific item using remove() method
numbers.remove(3)
# Print updated list
print(numbers)
Output:
[1, 2, 4, 3, 5]
Explanation:
In the example-
- We create a list named numbers containing integers [1, 2, 3, 4, 3, 5].
- Then, we call the remove() method with input value 3 on the list numbers.
- This function call removes the first instance of 3 from the list, i.e., the first occurrence of the value 3.
- Note that the original list contains 2 instances of the value 3, so after modification, the new list becomes [1, 2, 4, 3, 5].
- Finally, we output the new list to the console using the print() function, showing the list after the specified item 3 has been removed.
The pop() Method To Remove Specific Index Element From Python Lists
In the previous method, we used a value to specify the single item we wanted to remove. In this method, we can use the index value to specify the position of the single item we want to remove. So, the pop() method removes the element at the specified index position of a Python list and returns the element.
Syntax :
removed_item = list_name.pop(index)
Here, the list_name refers to the list we want to modify, (index) refers to the position of the element we want to remove, and it is stored in the variable removed_item.
Code Example:
# Original list
numbers = [1, 2, 3, 4, 5]
# Remove specific index using pop() method
removed_item = numbers.pop(2)
# Print the updated list and removed item
print("Updated List:", numbers)
print("Removed Item:", removed_item)
Output:
Updated List: [1, 2, 4, 5]
Removed Item: 3
Explanation:
In the Python code-
- We create a list called numbers using list literals with elements: [1, 2, 3, 4, 5].
- Then, we use the pop() function with argument 2 to remove the element at the 2nd index, i.e., the third position.
- The function call will remove the 3rd element, which is 3, and store it in the variable removed_item.
- As a result, the modified Python list becomes [1, 2, 4, 5].
- Lastly, we use a set of print() statements to display the updated list and the removed item, along with descriptive string messages.
Delete Entire Python List Using Del Keyword
Alternative to the built-in methods, you can use the del keyword to delete specific/ single items or an entire Python list. The syntax is pretty straightforward, as you just need to specify the list name that you want to delete following the del keyword.
Syntax:
del list_name
Code Example:
# Original list
numbers = [1, 2, 3, 4, 5]
# Delete the entire list using the del keyword
del numbers
# Try to print the list (it will throw an error as the list is deleted)
print(numbers)
Output:
NameError: name 'numbers' is not defined
Explanation:
In the above Python code-
- We define a list called numbers containing integers [1, 2, 3, 4, 5].
- Then, we use the del keyword followed by list name- numbers to delete the entire list and remove its reference.
- The statement del numbers deletes or removes the whole list from memory.
- Any attempt to access or print the list numbers will result in unexpected behavior/ an error because the variable no longer exists.
- As shown in the output, when we try to print numbers after it has been deleted, it raises a NameError.
Empty Python List Using clear() Method
The clear() method removes all elements from the list, resulting in an empty list. Note that this is different from the del keyword, as it does not remove the list from the memory but deletes all list items. We are still left with an empty list, which is not the case when using the del keyword.
Syntax:
list_name.clear()
Here, we simply call the clear() function on the list whose name is given by list_name using the dot operator (.).
Code Example:
# Original list
numbers = [1, 2, 3, 4, 5]
# Empty the list using clear() method
numbers.clear()
# Print the empty list
print(numbers)
Output:
[ ]
Explanation:
In the Python example above-
- We create a list called numbers containing integers [1, 2, 3, 4, 5].
- Then, we use the clear() method to empty the entire list and remove all its elements, i.e., numbers.clear(). This leaves us with an empty Python list.
- Finally, we print the list numbers, which output empty square brackets [], showing that the list has been emptied.
How To Create Copies Of Python Lists?
Copying a Python list is a common operation with varied applications, for example if you want to make changes to a list without altering the original one. However, the type of copy created will determine if the changes made in the copy list affects the original list or not. This where the concept of shallow and deep copy come into play.
There are primarily two types of copies that can be created-
- Shallow copy- This is when the changes to the copy list affect the original list.
- Deep copy- This is when changes to the copy Python list do not affect the original/ initial list.
The methods for creating these copies are different based on how they handle the copying of nested objects within the list. In the sections ahead, we will look at the three ways of copying lists in detail.
The copy() Methods To Copy Python Lists
A shallow copy of a list creates a new list object but inserts references to the objects found in the original list. This means that any changes of modifications made to the nested objects within the list will reflect in both the original list and the shallow copy list. We use the copy() function to create a shallow copy of Python lists.
Syntax:
import copy
shallow_copy = copy.copy(original_list)
Here,
- The phrase import copy asks the compiler to import the copy module, which contains the copy() function.
- This function takes a list as input, given by original_list and it is called by using dot operator with module and function name.
- The function creates a shallow copy, which is stored in the shallow_copy list variable.
Code Example:
import copy
# Original list with nested elements
original_list = [1, [2, 3], 4]
# Creating a shallow copy using copy.copy()
shallow_copy = copy.copy(original_list)
# Modify a nested element in shallow copy
shallow_copy[1][0] = 'a'
# Print both lists
print("Original List:", original_list)
print("Shallow Copy:", shallow_copy)
Output:
Original List: [1, ['a', 3], 4]
Shallow Copy: [1, ['a', 3], 4]
Explanation:
In the example Python code, we begin by importing the copy module to use the copy() function.
- Then, we create a Python list named original_list containing elements [1, [2, 3], 4]. This list includes primitive elements (1 and 4) and a nested list element [2, 3].
- Next, we use the copy() function with original_list as an argument to create a shallow copy, i.e., copy.copy(original_list).
- This creates a new list called shallow_copy that initially contains references to the same objects as the original_list.
- We then use the double index method to modify an element of nested list within the shallow_copy.
- That is, we modify the 1st element of the nested list, i.e., shallow_copy[1][0] and assigning the string value a to it.
- This modifies the first element of the nested list from 2 to a within shallow_copy.
- After that, we use a set of print() statements to display both the lists and observe the changes.
- As shown in the output, the modification of the shallow copy also affected the original list, as both of them become [1, ['a', 3], 4].
The deepcopy() Method To Copy Of Python Lists
A deep copy of a list creates a new list object as well as new objects for any nested elements, recursively. We use another function from the copy module to create a deep copy, known as the deepcopy() method.
Using this method ensures that modifications made to nested objects within the copied list do not affect the original list or other copies. The syntax for this is similar to that of the copy() method.
Syntax:
import copy
deep_copy = copy.deepcopy(original_list)
Here,
- The deepcopy() function from the copy module is used for creating deep copies of the list given by original_copy.
- The outcome of the new Python list is stored in the deep_copy list variable.
Code Example:
import copy
# Original list with nested elements
original_list = [1, [2, 3], 4]
# Creating a deep copy using copy.deepcopy()
deep_copy = copy.deepcopy(original_list)
# Modify a nested element in deep copy
deep_copy[1][0] = 'b'
# Print both lists
print("Original List:", original_list)
print("Deep Copy:", deep_copy)
Output:
Original List: [1, [2, 3], 4]
Deep Copy: [1, ['b', 3], 4]
Explanation:
In the above Python code, we import the copy module to use the built-in functions for copying lists.
- We create a Python list named original_list containing elements [1, [2, 3], 4]. This list includes primitive elements (1 and 4) and a nested list [2, 3].
- Then, we use the deepcopy() method from the copy module, to create a deep copy of original_list, i.e., copy.deepcopy(original_list).
- This creates a new list stored in deep_copy where the top-level elements and the nested objects are copied recursively.
- Next, we modify an element of nested list within deep_copy using the double index method.
- That is, we modify the first element of the list (at 2nd position) inside original_list: deep_copy[1][0] and assign the string/ character value 'b' to it.
- Then, we use two print() functions to display both the original and the deep copied list to the console to observe the changes.
- As seen in the output, the original_list remains unaffected by the changes made to the deep_copy list.
Copying Python Lists Using Built-in list() Method
The built-in list() method is commonly used for type conversion in Python to convert other data types to list type. We can also use this function to create a copy of a Python list by passing it as an argument. It creates a shallow copy of the input list, similar to the copy() method.
Syntax:
new_list = list(original_list)
Here,
- The built-in function list() converts the input parameter which can be any iterable to a list type.
- Here, the function parameter is given by a list called original_list, and the outcome is stored in the variable new_list.
Code Example:
# Original list with nested elements
original_list = [1, [2, 3], 4]
print("Original List (before modification):", original_list)
# Create a shallow copy using list() method
shallow_copy = list(original_list)
# Modify a nested element in the shallow copy
shallow_copy[1][0] = 'b'
# Print both lists
print("Original List:", original_list)
print("Shallow Copy:", shallow_copy)
Output:
Original List (before modification): [1, [2, 3], 4]
Original List: [1, ['b', 3], 4]
Shallow Copy: [1, ['b', 3], 4]
Explanation:
In the above Python list example-
- We create a list named original_list containing primitive elements and a nested list, i.e., [1, [2, 3], 4] and print the same to the console.
- Next, we use the list() constructor method to create a shallow copy of original_list, assigning it to shallow_copy variable.
- This creates a new list/ shallow copy of the original input list, with references to the same objects as the original_list.
- Then, we modify an element of the nested list inside the shallow_copy list.
- Here, we replace the 1st element of the nested list with the character value 'b', i.e., shallow_copy[1][0] = 'b'. This changes the 1st element of nested list from 2 to 'b' within shallow_copy.
- After that, we use print() function and display the original as well as the copy list to observe the changes made.
- As seen in the output, modifying shallow_copy affected the original_list for nested objects because both lists share references to the same nested list [2, 3].
Repeating Python Lists
Repeating already existant Python lists is a convenient way of creating multiple copies of the list without having to re-write the code. There are multiple ways in which we can repeat a single Python list, which we will discuss here.
The Multiplication Operator (*) To Repeat Python Lists
The simplest way to repeat Python lists is by using the multiplication operator/ asterisk symbol (*). It allows you to repeat a list by concatenating multiple copies of it.
Syntax:
new_list = original_list * n
Here,
- The asterisk symbol (*) multiplies/ repeates the Python list given by original_list, n number of times.
- The outcome is stored in the new_list variable.
Code Example:
# Original list
numbers = [1, 2, 3]
# Repeat the list three times using the * operator
repeated_list = numbers * 3
print(repeated_list)
Output:
[1, 2, 3, 1, 2, 3, 1, 2, 3]
Explanation:
In the Python list example-
- We create a Python list named numbers containing integers [1, 2, 3].
- Then, we use the multiplication operator (*) to repeat this list 3 times, i.e., numbers * 3.
- This operation creates a new list named repeated_list that contains [1, 2, 3, 1, 2, 3, 1, 2, 3], concatenating the numbers list with itself three times.
- Finally, we print repeated_list, showing the list numbers repeated three times consecutively in a new list.
Repeat Python Lists Using List Comprehension
We have already discussed list comprehension as one of the ways to create Python lists. It also provides a flexible way to repeat list elements based on a specified condition or operation.
Syntax:
new_list = [item for _ in range(n) for item in original_list]
Here,
- The for keyword marks the beginning of the for loop, with the underscore (_) acting as the placeholder of the loop control variable when its value is not used.
- Item represents each element in the initial list given by original_list, and the outcome list is stored in variable new_list.
- The range(n) refer to the range over which the loop will iterate and it encompasses number from 0 to n-1.
Code Example:
# Original list
numbers = [1, 2, 3, 4]
# Repeat the list three times using list comprehension
repeated_list = [item for _ in range(3) for item in numbers]
print(repeated_list)
Output:
[1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4]
Explanation:
In the Python list example-
- We create a list called numbers containing four integers [1, 2, 3, 4].
- Then, we use list comprehension with a nested for loop to create a new list called repeated_list. The comprehension is structured as follows:
- The outer loop iterates 3 times, i.e., for _ in range(3) with the underscore (_) placeholder for a variable we don't actually need to use in the comprehension.
- For every iteration of the outer loop, the inner for loop iterates through each element in the list one by one, adding it to the repeated_list.
- Effectively, for every iteration of the outer loop, all four initial list elements are repeated thrice in the new list.
- After the iterations are done, we get a new list, i.e., repeated_list, with elements [1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4], which we print to the console using print() function.
- This example demonstrates that the list numbers has been repeated three times consecutively in a new list using list comprehension.
Repeat Python Lists Using Loops
Using a loop allows you to dynamically repeat elements of a list based on specified conditions or operations within the loop.
Code Example:
# Original list
numbers = [1, 2, 3]
n = 3 # Number of times to repeat
# Repeat the list using a loop
repeated_list = []
for _ in range(n):
repeated_list.extend(numbers)
print(repeated_list)
Output:
[1, 2, 3, 1, 2, 3, 1, 2, 3]
Explanation:
In the above Python code-
- We create a numbers list containing integer numbers [1, 2, 3] and initialise variable n with the value 3.
- Then, we create another list repeated_list and initialize it with empty square brackets, making it an empty list, to store the new list with repeated elements.
- Next, we create a for loop to iterate over the range n, that is, n times. Inside the loop-
- We use the extend() method to append/ add each element from the numbers list at the end of the repeated_list.
- The extend() method adds elements from an iterable (in this case, numbers) to the end of the list repeated_list.
- This process occurs three times, after which the loop terminates. At the end of the iterations, the repeated_list variable will contain the new list with elements [1, 2, 3, 1, 2, 3, 1, 2, 3].
- Finally, we use the print() function to print the new list, demonstrating that the numbers list has been repeated three times.
Ways To Iterate Over Python Lists
When working with Python lists, you will need to iterate through its elements for various manipulation, modification and computational purposes. There are three primary ways to iterate over a Python list including the traditional loops (for and while) as well as the in-built enumerate() function. We will explore these methods in the following sections.
Iterating Over Python Lists Using For Loop
The for loop is commonly used to iterate over a range which can be elements of an iterable object. In fact, for loops are the most common way to iterate over and access the elements of a Python list. The syntax for the loop is pretty straightforward where the for keyword marks the beginning of the loop.
Syntax:
for element in list_name:
# Perform operations with element
Here, element represents each item in the list that the loop must traverse and the name of the list is given by list_name. Note that in the traditional for loop syntax, we specify the range which here is given by the list.
Code Example:
# Creating a list of numbers
num_list = [1, 2, 3, 4, 5]
# Iterate through the list using a for loop
for num in num_list:
print(num, end= " ")
print()
# Using for loop to sqaure each element of the list
squared_numList = []
for num in num_list:
squared_numList.append(num ** 2)
print(squared_numList)
Output:
1 2 3 4 5
[1, 4, 9, 16, 25]
Explanation:
In the above example-
- We create a list called num_list with integers [1, 2, 3, 4, 5] and then use a for loop to iterate over its elements.
- Inside the first for loop, we have a print() function to output each elements to the console.
- Note that we use the end parameter with a space as the end of the line, to print the elements of the list without a newline.
- After that, we run the print() function without any arguments to shift the cursor to the next line.
- Then, we create a list called squared_numList and initialize it with empty square brackets, making it an empty list.
- Next, we create another for loop to iterate over the num_list once again.
- Inside this loop, we raise each element num of the list to the power 2 (i.e., num**2) and use the append() function to append it to the squared_numList.
- This loop iterates over each element of num_list, adding their square to the squared_numList as an element. By the end of the iterations, the squared_numList contains squares of the elements of the input list.
- Finally, we use a print() function to display the squared_numList, which now contains [1, 4, 9, 16, 25].
- This example demonstrates how we can use the for loop to iterate over a list to access its elements and perform operations on them.
Iterating Over Python Lists Using While Loop
We can also use the while loop to iterate through a Python list. It uses the len() function to determine the length of the iterable (in this case list) and then iterates over each of its elements, incrimenting the index value after each iteration.
Note that while loops are used less commonly in comparison to for loop for traversal purposes in Python lists. This is largely because the implementation of the for loop is simpler.
Syntax:
index = 0
while index < len(list_name):
element = list_name[index]
# Perform operations with element
index += 1
Here,
- The while keyword marks the beginning of the while loop, with the index representing the loop control variable.
- Element refers to items of the list_name that we want to traverse and the len() function calculates the length of the said list.
Code Example:
# Creating a Python list with integer elements
nums_list = [1, 2, 3, 4, 5]
# Iterate through the list using the while loop
index = 0
while index < len(nums_list):
print(nums_list[index], end=' ')
index += 1
Output:
1 2 3 4 5
Explanation:
In the example-
- We create a Python list called nums_list with integer elements [1, 2, 3, 4, 5] and initialize a variable index with value 0 to work as the loop control variable.
- Next, we define a while loop to iterate over the list. Here, we use the len() function to first determine the length of the list and the loop iterates until the value of the index is less than this number.
- Inside the loop we have a print() function which access the element at given index value and output the same to the console.
- Note that we have a space as an end parameter to print all elements without a new line.
- The value of the index is incremented by 1 (index += 1) after every iteration to move the loop to the next element.
- This process continues until the index is equal to the length of the numbers list, at which point the condition index < len(numbers) becomes False, and the loop terminates.
- The example demonstrates how we can use the while loop to iterate over a Python list to access its elements.
Iterating Over Python Lists Using The enumerate() Method
The enumerate() function in Python is useful when you need both the index and the element during iteration. It is also used with the for loop as an alternative to the range and is ideal for scenarios where index tracking is necessary alongside element processing.
Syntax:
for index, element in enumerate(list_name):
# Perform operations with index and element
Here,
- The terms index and element refer to the position of the list item and the item of the list by the name list_name itself.
- The enumerate() function returns an enumerate object containing pairs of index and element.
Code Example:
# Example list
fruits = ['apple', 'banana', 'cherry']
# Iterate through the list using enumerate
for index, fruit in enumerate(fruits):
print(f"Index {index}: {fruit}")
Output:
Index 0: apple
Index 1: banana
Index 2: cherry
Explanation:
In the Python list example code-
- We create a fruits list with string elements ['apple', 'banana', 'cherry'].
- Then, we create a for loop with to iterate through the Python list.
- In every iteration of the loop, the enumerate() function pairs each element of the fruits list with its corresponding index. For example, during the first iteration, the index will be 0, and the fruit will be 'apple'.
- The loop contains a print() function with a formatted string that combines the index and the fruit element name into a readable output.
- This loop iterates through each element in fruits, printing each element's index followed by the element itself.
How To Reverse A Python List?
There are multiple ways to reverse a list in Python, of which the reverse() method is the most commonly used method. The function takes a Python list as input and reverses all its elements in place, meaning it modifies the original list directly.
Syntax:
list_name.reverse()
Here, we use the dot operator (.) to call the reverse() function on a list given by list_name.
Code Example:
# Creating a list
list_num = [1, 2, 3, 4, 5]
print(list_num)
# Reversing the list in place
list_num.reverse()
print(list_num)
Output:
[1, 2, 3, 4, 5]
[5, 4, 3, 2, 1]
Explanation:
In the Python list example-
- We use list literals to create a list called list_num containing five integer elements [1, 2, 3, 4, 5], which we then print to the console.
- Next, we call the reverse() function on the list to reverse its elements in place, i.e., it reverses the order of elements in the list.
- We once again print the list to the console using the print() function to observe that the order of elements is actually reversed in place.
Also read: How To Reverse A String In Python? 10 Easy Ways With Examples
How To Sort Items Of Python Lists?
The built-in sort() method in Python is used to sort the elements of a list in place. That is, the function sorts the element of the original Python list, and does not create a new list. The function by default sorts the elements in an ascending order, however we can sort them in descending order by specifying the parameter reverse asTrue.
Syntax:
list_name.sort(key=None, reverse=False)
Here,
- The sort() function takes two parameters key and reverse where the former refers to the key for sorting comparison and latter is the boolean value to define the sorting order.
- Both these parameter are optional with their default values being none and False, respectively. Here, False means the sorting order will be ascending.
- The dot operator (.) is used to call the function on the Python list given by list_name.
Code Example:
# Creating an unsorted list
numbers_list = [6, 2, 8, 3, 5]
# Sorting the list in place (ascending order)
numbers_list.sort()
print(numbers_list)
# Sorting the list in descending order
numbers_list.sort(reverse=True)
print(numbers_list)
Output:
[2, 3, 5, 6, 8]
[8, 6, 5, 3, 2]
Explanation:
In the basic Python code example-
- We create a list of five integers [6, 2, 8, 3, 5] called numbers_list, using list literals.
- Then, we call the sort() function on the list without specifying any parameters. This sorts the list in place, in ascending order, which we then print to the console.
- After that, we call the sort() function once again with the reverse parameter set to True. This sorts the list in descending order.
- We use the print() function once again to print the revised list and observe the sorting in two different orders.
Built-in Functions For Operations On Python Lists
So far we've discussed how to create Python lists, access and modify their items and perform some basic manipulations on them. Naturally the purpose of lists to store data and then to be able to perform operations on the data.
There are many built-in functions in Python that are readily available for use in your programs without the need for explicit function definitions. In this section we will look at few of the most common Python list operations and their application.
List Of Python List Built-in Functions
Listed in the table below are the functions commonly used with lists in Python, along with a short description.
Function Name | Description |
append() | Adds an element to the end of the Python list. |
extend() | Adds all elements of an iterable (e.g., another list) to the end of the Python list. |
insert() | Inserts an element at a specified position in the Python list. |
remove() | Removes the first occurrence of a specified element from the Python list. |
pop() | Removes and returns the element at a specified position (default is the last element). |
clear() | Removes all elements from the Python list, leaving it empty. |
index() | Returns the index of the first occurrence of a specified element. |
count() | Returns the number of occurrences of a specified element in the Python list. |
sort() | Sorts the elements of the Python list in ascending order (or according to a specified key). |
reverse() | Reverses the order of elements in the Python list. |
copy() | Returns a shallow copy of the Python list. |
len() | Returns the number of elements in the Python list. |
min() | Returns the smallest element in the Python list. |
max() | Returns the largest element in the Python list. |
sum() | Returns the sum of all elements in the Python list. |
list() | Creates a list from an iterable (e.g., a string, tuple, or range). |
sorted() | Returns a new list containing all elements from the original list in ascending order. |
any() | Returns True if at least one element of the list is true, otherwise False. |
all() | Returns True if all elements of the list are true, otherwise False. |
enumerate() | Returns an enumerate object containing pairs of index and elements of the list. |
filter() | Constructs a list from those elements of the list for which a specified function returns true. |
map() | Applies a specified function to all elements in the list and returns a list of the results. |
zip() | Aggregates elements from multiple Python lists (or other iterables) into tuples. |
reduce() | Applies a rolling computation to sequential pairs of values in a list. It is part of the functools module and not a directly built-in Python function. |
cmp() | Compares two objects (say x and y) and returns -1 if x < y, 0 if x == y, and 1 if x > y. It exists in Python2 only and is removed in Python 3. However, the functionality can be achieved with relational comparison and logical operators. |
accumulate() | It makes an iterator that returns accumulated sums or accumulated results of other binary functions. It is part of the itertools module. |
We have already described and used some of these common list methods in the sections above. Let's look at an example for a few of the functions and see how they work with Python lists.
Code Example:
from functools import reduce
from itertools import accumulate
# Creating a Python list
int_list = [1, 2, 3, 4, 5]
# Finding and printing the sum of all elements
total_sum = sum(int_list)
print("Total Sum:", total_sum)
# Finding and printing minimum and maximum elements
minimum = min(int_list)
maximum = max(int_list)
print(f"Minimum Element: {minimum} and Maximum Element: {maximum}")
# Check if all elements are greater than 0
all_positive = all(num > 0 for num in int_list)
print("All Positive Check:", all_positive)
# Check if any element is less than 0
any_negative = any(num < 0 for num in int_list)
print("Any Negative Check:", any_negative)
# Using filter and map with lambda function
filtered_numbers = list(filter(lambda x: x % 2 == 0, int_list)) # Filter even int_list
mapped_numbers = list(map(lambda x: x * 2, int_list)) # Double each element
print("Filtered Numbers (Even):", filtered_numbers)
print("Mapped Numbers (Doubled):", mapped_numbers)
# Using reduce and accumulate
product = reduce(lambda x, y: x * y, int_list) # Product of all elements
accumulated_sum = list(accumulate(int_list)) # Accumulated sums
print("Product of All Elements:", product)
print("Accumulated Sums:", accumulated_sum)
Output:
Total Sum: 15
Minimum Element: 1 and Maximum Element: 5
All Positive Check: True
Any Negative Check: False
Filtered Numbers (Even): [2, 4]
Mapped Numbers (Doubled): [2, 4, 6, 8, 10]
Product of All Elements: 120
Accumulated Sums: [1, 3, 6, 10, 15]
Explanation:
We begin the Python list example by importing the functools module (for reduce) and the itertools module (for accumulate).
- Then, we create a list called int_list containing five integers [1, 2, 3, 4, 5].
- Next, we use the sum() function to calculate the total sum of all elements in the list, store the outcome in total_sum variable and print it.
- After that, we use the min() and max() functions to find the smallest and largest elements in the list. We store the outcomes in the minimum and maximum variables and print them using a formatted string inside the print() function.
- We then use the all() function to check if all elements in the Python list are greater than 0, store the result in all_positive and print the same.
- Similarly, we use the any() function to check if any element in the list is less than 0, store the result in any_negative, and print it.
- Then, we use the filter() function with an anonymous function created using lambda (lambda x: x % 2 == 0), to filter out all positive numbers.
- This creates a new list containing only the even numbers which we store in filtered_numbers and print it.
- Similarly, we use the map() function with a lambda function (lambda x: x * 2), to double each element and create a new list mapped_numbers and print the same.
- Following that, we use the reduce() function with a lambda function (lambda x, y: x * y), to compute the product of all elements in the Python list. We store the outcome in the variable product and print it to the console.
- Lastly, we use the accumulate() function from itertools, to computer the accumulated sums of int_list, store the outcome in the variable accumulated_sum and print it.
Conclusion
Python lists are versatile data structures that allow you to store and manipulate collections of data with ease. The most common method of creating these lists is with the list literals.
- Lists in Python offer flexibility with their dynamic resizing, allowing for the storage of heterogeneous data types and support for various operations.
- The elements of the list are ordered; that is, they maintain the order/ sequence in which they are created.
- Python lists are also mutable, meaning elements can be added, removed, or modified after the list is created, making them suitable for a wide range of programming tasks.
- There are multiple in-built functions that allow you to perform operations on Python lists, like append(), pop(), remove(), sort(), etc.
Understanding the nuances of Python lists and all related concepts will enhance the efficiency and readability of your Python code. Mastering lists will prove invaluable in harnessing the full potential of the Python language for data handling, algorithm development, and application building.
Frequently Asked Questions
Q. What is a list in Python programming?
In Python programming, a list is a versatile and ordered collection of elements that can contain items of different data types, such as integers, floats, strings, and even other lists.
- Python lists are mutable, meaning you can change their elements after they are created.
- They are defined by enclosing comma-separated values within square brackets [ ], i.e., list literals.
- Lists support various operations like indexing, slicing, appending, extending, and more, making them fundamental for storing and manipulating data in Python programs efficiently.
- Python lists are commonly used for tasks ranging from simple data storage to complex data processing and algorithm development.
Q. What is the difference between deepcopy() and copy() methods in Python for lists?
The deepcopy() and copy() are both functions in the copy module and allow us to create copies of Python lists. The primary point of difference between them lies in how they handle the duplication of nested objects.
- The copy() method creates a shallow copy of a list, meaning it duplicates the list structure but maintains references to the objects within the list.
- This means changes made to nested objects in the copied list will also be reflected in the original list.
- In contrast, the deepcopy() method, creates a deep copy of the list, meaning it recursively copies all objects found within the original list, including nested objects.
- This results in completely independent duplicate values of the original list, ensuring that changes made to nested objects in the deep-copied list do not affect the original list and vice versa.
Using deepcopy() is particularly useful when dealing with lists that contain mutable objects such as other lists or dictionaries, where complete independence from the original list is necessary.
Q. Can Python lists store different data types?
Yes, Python lists can store different data types. This flexibility is one of the strengths of Python lists, allowing you to store heterogeneous data within a single list. For example, a single list can contain integers, floats, strings, and even other lists or complex objects like dictionaries and tuples. The example below illustrates this concept.
Code Example:
# Creating a list with different data types
mixed_list = [1, 3.14, 'Python', [1, 2, 3], {'key': 'value'}, (4, 5)]
# Accessing and printing elements of different data types
print("Integer:", mixed_list[0])
print("Float:", mixed_list[1])
print("String:", mixed_list[2])
print("List:", mixed_list[3])
print("Dictionary:", mixed_list[4])
print("Tuple:", mixed_list[5])
Output:
Integer: 1
Float: 3.14
String: Python
List: [1, 2, 3]
Dictionary: {'key': 'value'}
Tuple: (4, 5)
This feature makes Python lists very versatile and useful for various applications, allowing you to group related but different types of data together and manipulate them efficiently.
Q. What is the difference between remove() and del keywords in Python lists?
The difference between the remove() method and the del keyword lies in their usage and functionality for removing elements from a Python list. The table below summarizes the difference between the two.
Feature | remove() Method | del Keyword |
---|---|---|
Purpose | Removes the first occurrence of a specified value | Deletes elements by index, slices, or entire list |
Usage | list_name.remove(value) | del list_name[index] or del list_name |
Removes by | Value | Index or the entire list |
Error Handling | Raises ValueError if value not found | Raises IndexError if index out of range |
Example | numbers.remove(3) | del numbers[2] |
Q. How do you find the length of a list in Python?
The built-in len() function is used to find the length of a Python list. It takes a list as an argument and returns the number of elements/ total count of list items contained within it. Consider the following Python list example:
my_list = [1, 2, 3, 4, 5]
number= len(my_list)
In this example, the len() function will return 5, as there are five elements in the list. It is a simple and efficient way of determining the size of a list for tasks such as iteration and validation or performing basic list operations that depend on the length of a Python list.
Revisit Python Lists Basics With A Quick Quiz!
Do check out the following:
- Fibonacci Series In Python & Nth Term | Generate & Print (+Codes)
- Python Program To Find LCM Of Two Numbers | 5 Methods With Examples
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Convert Int To String In Python | Learn 6 Methods With Examples
- 12 Ways To Compare Strings In Python Explained (With Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.
Divyansh Shrivastava 3 weeks ago
Pranjul Srivastav 1 month ago
Anjali Nimesh 1 month ago
kavita Prajapati 1 month ago
Shradha Manure 1 month ago
niyati m singh 1 month ago