Python Programming
Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Relational Operators In Python?
- Type Of Relational Operators In Python
- Equal-To Relational Operator In Python
- Greater Than Relational Operator In Python
- Less-Than Relational Operator In Python
- Not Equal-To Relational Operator In Python
- Greater-Than Or Equal-To Relational Operator In Python
- Less-Than Or Equal-To Relational Operator In Python
- Why And When To Use Relational Operators?
- Precedence & Associativity Of Relational Operators In Python
- Advantages & Disadvantages Of Relational Operators In Python
- Real-World Applications Of Relational Operators In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues with Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding Python Flow Control
- Break Statement In Python
- Examples Of Break Statements In Python
- Continue Statement In Python
- Examples Of Continue Statements In Python
- Using Break and Continue Statements In A Single Program
- Difference Between Break And Continue Statements In Python
- When To Use Which Statement In Python?
- Conclusion
- Frequently Asked Questions
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
Type Conversion In Python | Implicit & Explicit Types (+Examples)
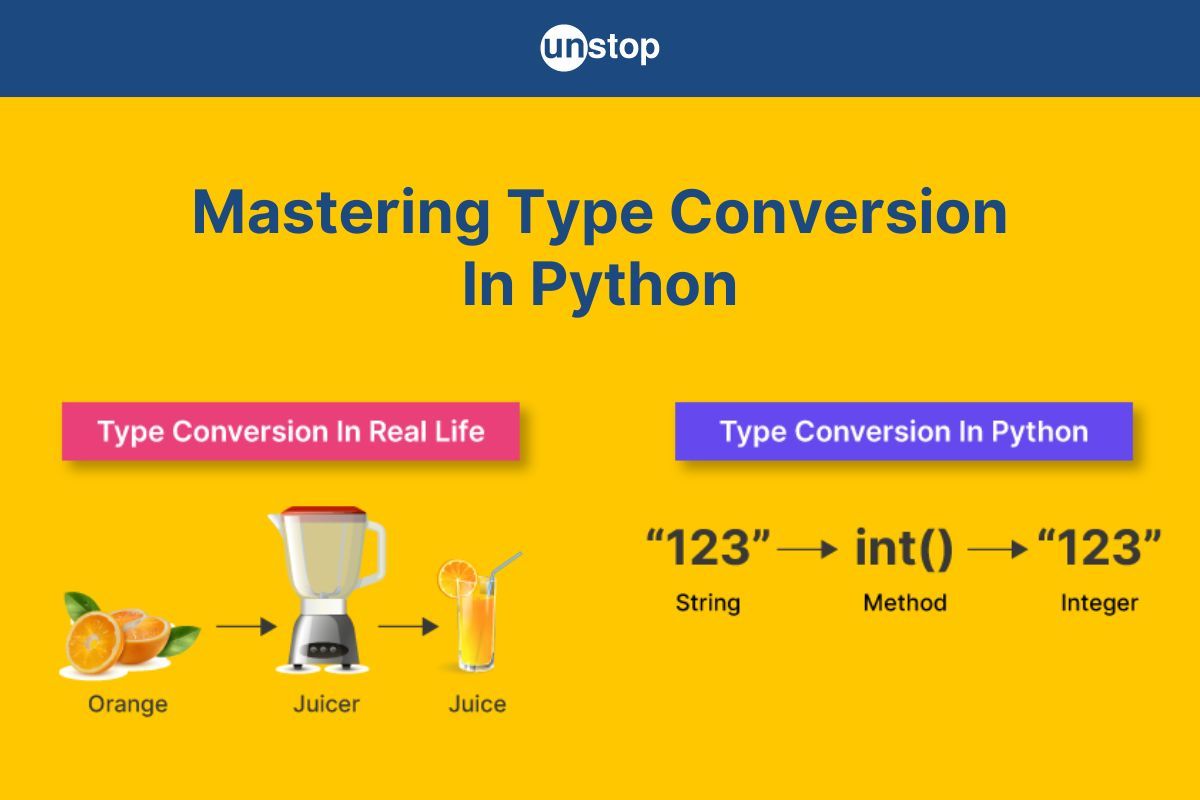
Type conversion in the Python programming language, also known as data type conversion, is a fundamental concept where one data type is converted into another. It is crucial for various operations and data manipulations in your programs, enabling developers to work effectively with different types of data. In this comprehensive tutorial, we will delve into the intricacies of type conversion in Python, exploring the various techniques, functions, and best practices associated with converting data between different types.
What Is Type Conversion In Python?
As discussed above, type conversion in Python refers to the process of changing the data type of a variable from one type to another. These can be implicit type conversions, i.e., those that are implicitly done by Python interpreter itself, or explicit type conversions, where we externally use functions/ methods to convert the data type.
Type conversion is usually done either to ensure that operations between different data types are performed correctly or to adapt data for specific functions and computations.
General Example: Oranges To Juice
The process of type conversion can be likened to converting oranges to juice. Imagine we have whole oranges, which represent one data type. To make juice, we need to process these oranges, transforming them into a different form—juice, analogous to another data type.
Similarly, in Python, we often need to convert data from one type to another to fit the requirements of our code. This is what type conversion in Python and other programming languages broadly entails.
- For instance, we might convert a string representation of a number into an integer to perform arithmetic operations.
- Just as squeezing oranges transforms them into juice, type conversion functions like int(), float(), or str(), transform data into the types we need for our operations.
Let's look at a simple Python program example illustrating type conversion, both implicit and explicit.
Code:
aW50X251bSA9IDEwMDAgIyBJbnRlZ2VyIHZhbHVlCmZsb19udW0gPSAzLjE0ICMgRmxvYXRpbmctcG9pbnQgdmFsdWUKCm5ld19udW0gPSBpbnRfbnVtICsgZmxvX251bSAjIEltcGxpY2l0IHR5cGUgY29udmVyc2lvbiBmcm9tIGludCB0byBmbG9hdAoKcHJpbnQoImRhdGF0eXBlIG9mIGludF9udW06IiwgdHlwZShpbnRfbnVtKSkgIyBPdXRwdXQ6IGRhdGF0eXBlIG9mIGludF9udW06IDxjbGFzcyAnaW50Jz4KcHJpbnQoImRhdGF0eXBlIG9mIGZsb19udW06IiwgdHlwZShmbG9fbnVtKSkgIyBPdXRwdXQ6IGRhdGF0eXBlIG9mIGZsb19udW06IDxjbGFzcyAnZmxvYXQnPgpwcmludCgiVmFsdWUgb2YgbmV3X251bToiLCBuZXdfbnVtKSAjIE91dHB1dDogVmFsdWUgb2YgbmV3X251bTogMTAwMy4xNApwcmludCgiZGF0YXR5cGUgb2YgbmV3X251bToiLCB0eXBlKG5ld19udW0pKSAjIE91dHB1dDogZGF0YXR5cGUgb2YgbmV3X251bTogPGNsYXNzICdmbG9hdCc+CgojIEV4cGxpY2l0bHkgY29udmVydCBuZXdfbnVtIHRvIGFuIGludGVnZXIKbmV3X251bSA9IGludChuZXdfbnVtKQpwcmludCgiVmFsdWUgb2YgbmV3X251bSBhZnRlciBleHBsaWNpdCBjb252ZXJzaW9uOiIsIG5ld19udW0pICMgT3V0cHV0OiBWYWx1ZSBvZiBuZXdfbnVtIGFmdGVyIGV4cGxpY2l0IGNvbnZlcnNpb246IDEwMDMKcHJpbnQoImRhdGF0eXBlIG9mIG5ld19udW0gYWZ0ZXIgZXhwbGljaXQgY29udmVyc2lvbjoiLCB0eXBlKG5ld19udW0pKSAjIE91dHB1dDogZGF0YXR5cGUgb2YgbmV3X251bSBhZnRlciBleHBsaWNpdCBjb252ZXJzaW9uOiA8Y2xhc3MgJ2ludCc+
Output:
datatype of int_num: <class 'int'>
datatype of flo_num: <class 'float'>
Value of new_num: 1003.14
datatype of new_num: <class 'float'>
Value of new_num after explicit conversion: 1003
datatype of new_num after explicit conversion: <class 'int'>
Explanation:
In the above Python example,
- We start by defining two variables: integer data type variable int_num with a value of 1000 and flo_num with a floating-point value of 3.14.
- Next, when we add these two variables together, Python performs implicit type conversion, automatically converting the integer int_num into a float so that the addition operation can be performed with both numbers as floats.
- We store the result of this addition in the variable new_num.
- Next, we print the data types of initial variables- int_num and flo_num to show that they are integer and float, respectively.
- After that, we print the value and data type of new_num, which is the sum of int_num and flo_num, resulting in 1003.14 and data type float.
- Next, we use the int() function to explicitly convert new_num from a float to an integer, which truncates the decimal part, resulting in 1003.
- Finally, we again print the value and data type of new_num, which is 1003 and int.
Types Of Type Conversion In Python
Type conversion in Python can be categorized into two main types:
- Implicit Type Conversion
- Explicit Type Conversion
In the sections ahead, we will discuss the types of type conversion in Python in detail, along with code illustrations.
Implicit Type Conversion In Python
Implicit type conversion, also known as automatic type conversion, occurs when Python automatically changes the data type of a variable during operations to maintain consistency and prevent errors. This type of conversion happens without requiring any explicit instructions from us. For example, if we perform arithmetic operations involving distinct data types, Python will convert the lower precision type to a higher precision type to ensure accurate results.
Key Characteristics Of Implicit Type Conversion In Python:
- Automatic: Performed by the Python interpreter automatically.
- No Programmer Input: This does not require explicit commands from the programmer.
- Type Promotion: Usually promotes to a higher or more inclusive data type.
Example 1: Implicit Conversion From Int To Float
In this code example, we will implicitly convert an integer (Int) to a float (Float).
Code:
bnVtX2ludCA9IDUgIyBJbnRlZ2VyCm51bV9mbG9hdCA9IDMuNSAjIEZsb2F0CgpyZXN1bHQgPSBudW1faW50ICsgbnVtX2Zsb2F0ICMgSW1wbGljaXQgY29udmVyc2lvbiBmcm9tIGludCB0byBmbG9hdAoKcHJpbnQoIlJlc3VsdDoiLCByZXN1bHQpCnByaW50KCJEYXRhIHR5cGUgb2YgcmVzdWx0OiIsIHR5cGUocmVzdWx0KSk=
Output:
Result: 8.5
Data type of result: <class 'float'>
Explanation:
In the above Python code,
- We start by assigning the integer value 5 to the variable num_int and the floating-point value 3.5 to the variable num_float.
- Next, when we add these two variables together, Python automatically performs implicit type conversion, converting the integer num_int into a float so that both numbers are the same type for the addition operation.
- We then store the outcome of this addition in the variable result and print it to get the value as 8.5.
- Finally, we use the type() function to display the data type of the result, confirming that it is a floating-point value.
Example 2: Implicit Conversion In Arithmetic Operations
In this code example, we will perform the implicit conversion in arithmetic operations.
Code:
IyBEZWZpbmUgYSBmbG9hdCBhbmQgYW4gaW50ZWdlcgpmbG9hdF92YWwgPSA0Ljc1ICMgRmxvYXQKaW50X3ZhbCA9IDMgIyBJbnRlZ2VyCgojIE11bHRpcGx5IHRoZSBmbG9hdCBieSB0aGUgaW50ZWdlcgpyZXN1bHQgPSBmbG9hdF92YWwgKiBpbnRfdmFsICMgSW1wbGljaXQgY29udmVyc2lvbiBvZiBpbnQgdG8gZmxvYXQKCiMgUHJpbnQgdGhlIHJlc3VsdCBhbmQgaXRzIGRhdGEgdHlwZQpwcmludCgiUmVzdWx0OiIsIHJlc3VsdCkgIyBPdXRwdXQ6IDE0LjI1CnByaW50KCJEYXRhIHR5cGUgb2YgcmVzdWx0OiIsIHR5cGUocmVzdWx0KSkgIyBPdXRwdXQ6IDxjbGFzcyAnZmxvYXQnPg==
Output:
Result: 14.25
Data type of result: <class 'float'>
Explanation:
In the above Python code,
- We begin by defining two variables: float_val with a value of 4.75, and int_val with a value of 3.
- When we multiply them using the multiplication operator (*), Python automatically converts the integer to a float so that the arithmetic operation can be performed with both numbers as floating-point values.
- We then store the outcome of this multiplication in the variable result and print its value using the print() function.
- Finally, we use the type() function to show that the data type of result is a float, which confirms that the implicit type conversion occurred during the multiplication.
Explicit Type Conversion In Python
Explicit type conversion, also known as type casting, requires us to manually convert one data type to another using specific functions or operators. This type of conversion gives us control over how and when the data type change occurs. We use functions like int(), float(), str(), and list() to perform explicit type conversion in Python.
Key Characteristics Of Explicit Type Conversion In Python:
- Manual: Performed by the programmer using specific commands.
- Requires Instructions: Needs explicit commands to perform the conversion.
- Specified Type: Converts data to a specific target data type.
Here is a code example to illustrate explicit type conversion in Python.
Code:
IyBFeHBsaWNpdCB0eXBlIGNvbnZlcnNpb24gZXhhbXBsZQoKbnVtX2Zsb2F0ID0gMy43NSAjIEZsb2F0CgpudW1faW50ID0gaW50KG51bV9mbG9hdCkgIyBFeHBsaWNpdCBjb252ZXJzaW9uIGZyb20gZmxvYXQgdG8gaW50CgpwcmludCgiQ29udmVydGVkIEludGVnZXI6IiwgbnVtX2ludCkKCnByaW50KCJEYXRhIHR5cGUgb2YgY29udmVydGVkIGludGVnZXI6IiwgdHlwZShudW1faW50KSk=
Output:
Converted Integer: 3
Data type of converted integer: <class 'int'>
Explanation:
In the above Python code,
- We start by defining a variable, num_float, with a float value of 3.75.
- To convert this float to an integer, we use the int() function, which explicitly changes the float to an integer, and we store this converted value in the variable num_int.
- Then, we print the value of num_int, which will be 3 because the int() function truncates the decimal part, leaving only the whole number.
- Finally, we use the type() function to check and display that num_int is of type int, confirming that the explicit type conversion from float to int was successful.
Functions Used For Explicit Data Type Conversion In Python
As mentioned before, we use various functions/ built-in methods to carry out explicit type conversion in Python programs. Below is a table that lists common Python functions used for explicit type conversion:
Function Name |
Purpose |
Syntax / Example |
int(a, base) |
Converts a string or number to an integer |
num_str = "42"<br>num_int = int(num_str)<br># Output: 42 |
float() |
Converts a value to a floating-point number |
num_str = "3.14"<br>num_float = float(num_str)<br># Output: 3.14 |
ord() |
Converts a character to its Unicode integer value |
char = 'A'<br>unicode_value = ord(char)<br># Output: 65 |
hex() |
Converts an integer to a lowercase hexadecimal string |
num = 16<br>hex_string = hex(num)<br># Output: '0x10' |
oct() |
Converts an integer to an octal string |
num = 8<br>oct_string = oct(num)<br># Output: '0o10' |
tuple() |
Converts a sequence to a tuple |
list_data = [1, 2, 3]<br>tuple_data = tuple(list_data)<br># Output: (1, 2, 3) |
set() |
Converts a sequence to a set |
list_data = [1, 2, 2, 3, 3, 3]<br>set_data = set(list_data)<br># Output: {1, 2, 3} |
list() |
Converts a sequence to a list |
tuple_data = (1, 2, 3)<br>list_data = list(tuple_data)<br># Output: [1, 2, 3] |
str() |
Converts a value to a string |
num_int = 42<br>num_str = str(num_int)<br># Output: '42' |
dict() |
Converts a sequence of key-value pairs to a dictionary |
list_data = [('a', 1), ('b', 2)]<br>dict_data = dict(list_data)<br># Output: {'a': 1, 'b': 2} |
complex(real, imag) |
Creates a complex number with the given real and imaginary parts |
real_part = 3<br>imag_part = 4<br>complex_num = complex(real_part, imag_part)<br># Output: (3+4j) |
chr(number) |
Converts an integer to its corresponding Unicode character |
unicode_value = 65<br>char = chr(unicode_value)<br># Output: 'A' |
We will now cover each of these functions used for explicit type conversion in Python in detail with code examples.
Type Conversion In Python With int() Function
The int() function is often used for type conversion in Python as it converts a given value to the integer data type. It returns the integer representation of the input parameter.
Syntax:
int(a, base)
Here,
- a: The number or string to be converted.
- base: An integer representing the base of the number in string form (default is 10).
Code:
IyBCaW5hcnkgdG8gaW50ZWdlcgpiaW5hcnlfc3RyaW5nID0gIjEwMTAiCmJpbmFyeV9pbnQgPSBpbnQoYmluYXJ5X3N0cmluZywgMikKCiMgT2N0YWwgdG8gaW50ZWdlcgpvY3RhbF9zdHJpbmcgPSAiMTIiCm9jdGFsX2ludCA9IGludChvY3RhbF9zdHJpbmcsIDgpCgojIEhleGFkZWNpbWFsIHRvIGludGVnZXIKaGV4X3N0cmluZyA9ICJBIgpoZXhfaW50ID0gaW50KGhleF9zdHJpbmcsIDE2KQoKcHJpbnQoYmluYXJ5X2ludCkgIyBPdXRwdXQ6IDEwCnByaW50KG9jdGFsX2ludCkgIyBPdXRwdXQ6IDEwCnByaW50KGhleF9pbnQpICMgT3V0cHV0OiAxMA==
Output:
10
10
10
Explanation:
In the above Python code,
- We start by defining a variable binary_string with the value "1010".
- Then, we use the int() function to convert this binary string to an integer, with the second argument as 2, indicating the base is binary. We store this converted value in binary_int.
- Next, we define octal_string with the value "12". Then, using the int() function, we convert this octal string to an integer, with 8 (octal base) as the second argument. We store this converted value in octal_int.
- Similarly, we define hex_string with the value "A" and use the int() function to convert this hexadecimal string to an integer.
- Here, the second argument, 16, indicates that the base is hexadecimal, and we store this converted value in hex_int.
- Finally, we print the values of binary_int, octal_int, and hex_int. All three will output 10 because the binary "1010", octal "12", and hexadecimal "A" all represent the decimal number 10.
Type Conversion In Python With float() Function
The float() function performs type conversion in Python by converting the input value to a floating-point type. It accepts a single parameter, which can be a number or a string representing a numerical value, and returns the corresponding floating-point representation.
Syntax:
float(value)
Here, the value is the number/ data to be converted to a floating-point number. It can be an integer, a floating-point number, or a string representing a numeric value.
Code:
aW50ZWdlcl9udW1iZXIgPSAxMjMKc3RyaW5nX251bWJlciA9ICIxMjMuNDUiCgpmbG9hdF9mcm9tX2ludCA9IGZsb2F0KGludGVnZXJfbnVtYmVyKQpmbG9hdF9mcm9tX3N0ciA9IGZsb2F0KHN0cmluZ19udW1iZXIpCgpwcmludChmbG9hdF9mcm9tX2ludCkgIyBPdXRwdXQ6IDEyMy4wCnByaW50KGZsb2F0X2Zyb21fc3RyKSAjIE91dHB1dDogMTIzLjQ1
Output:
123.0
123.45
Explanation:
In the above Python code,
- We start by defining a variable integer_number with an integer value of 123. We also define another variable, string_number, with a string value of "123.45".
- Then, we use the float() function to convert the integer to a float and store the result in the variable float_from_int.
- Similarly, we convert the string to a float using the float() function and store the result in the variable float_from_str.
- Finally, we print the values of float_from_int and float_from_str using the print() function.
Type Conversion In Python With ord() Function
The ord() function performs type conversion in Python by converting a single character into its Unicode code point, which is an integer representation of that character.
Syntax:
ord(char1)
Here,
- ord: The name of the function being called.
- char1: The parameter passed to the function.
Code:
Y2hhcl9hID0gJ0EnCmNoYXJfYiA9ICdiJwoKYXNjaWlfYSA9IG9yZChjaGFyX2EpCmFzY2lpX2IgPSBvcmQoY2hhcl9iKQoKcHJpbnQoYXNjaWlfYSkgIyBPdXRwdXQ6IDY1CnByaW50KGFzY2lpX2IpICMgT3V0cHV0OiA5OA==
Output:
65
98
Explanation:
In the above Python code,
- We define two variables, char_a and char_b, with the character values 'A' and 'b', respectively.
- Then, we convert these characters to their corresponding ASCII values by using the ord() function.
- We store the ASCII value of char_a in the variable ascii_a and the ASCII value of char_b in the variable ascii_b.
- Finally, we print the values of ascii_a and ascii_b.
Type Conversion In Python With hex() Function
The hex() function performs type conversion in Python by converting an integer number to a lowercase hexadecimal string prefixed with "0x".
Syntax:
hex(number)
Here,
- number: An integer number to be converted to hexadecimal.
- hex: The hex() function in Python is used to convert an integer number to a lowercase hexadecimal string prefixed with "0x".
Code:
IyBVc2luZyBoZXgoKSBmdW5jdGlvbiB0byBjb252ZXJ0IGludGVnZXJzIHRvIGhleGFkZWNpbWFsIHN0cmluZ3MKCm51bV9pbnQgPSA0MgoKbnVtX2Zsb2F0ID0gMy4xNAoKbnVtX3N0ciA9ICIxMCIKCmhleF9pbnQgPSBoZXgobnVtX2ludCkKCmhleF9mbG9hdCA9IGhleChpbnQobnVtX2Zsb2F0KSkKCmhleF9zdHIgPSBoZXgoaW50KG51bV9zdHIpKQoKcHJpbnQoIkhleGFkZWNpbWFsIHJlcHJlc2VudGF0aW9uIG9mIiwgbnVtX2ludCwgIjoiLCBoZXhfaW50KQoKcHJpbnQoIkhleGFkZWNpbWFsIHJlcHJlc2VudGF0aW9uIG9mIiwgbnVtX2Zsb2F0LCAiOiIsIGhleF9mbG9hdCkKCnByaW50KCJIZXhhZGVjaW1hbCByZXByZXNlbnRhdGlvbiBvZiIsIG51bV9zdHIsICI6IiwgaGV4X3N0cik=
Output:
Hexadecimal representation of 42 : 0x2a
Hexadecimal representation of 3.14 : 0x3
Hexadecimal representation of 10 : 0xa
Explanation:
In the above Python code,
- We start by defining three variables: num_int with an integer value of 42, num_float with a float value of 3.14, and num_str with a string value of "10".
- Then, we convert these values to hexadecimal strings using the hex() function.
- For num_int, we directly pass it to the hex() function and store the result in hex_int.
- For num_float, we first convert it to an integer using the int() function and then the hex() function to convert it to a hexadecimal string, storing the result in hex_float.
- Similarly, for num_str, we first convert the string to an integer using the int() function and then convert it to a hexadecimal string, storing the result in hex_str.
- Finally, we print the hexadecimal representations of num_int, num_float, and num_str.
Type Conversion In Python With oct() Function
The oct() function performs type conversion in Python by converting an integer number into an octal string representation. It takes an integer as a parameter and returns the corresponding octal string. The function returns a string representing the octal value of the input integer.
Syntax:
oct(number)
Here,
- oct: The oct() function returns a string representing the octal value of the input integer.
- number: An integer that needs to be converted into an octal string.
Code:
ZGVjaW1hbF9udW1iZXIgPSA4CgpvY3RfbnVtYmVyID0gb2N0KGRlY2ltYWxfbnVtYmVyKQoKcHJpbnQob2N0X251bWJlcik=
Output:
0o10
Explanation:
In the above Python code,
- We start by defining a variable decimal_number with an integer value of 8.
- Then, we convert this decimal number to its octal representation using the oct() function and store the result in the variable oct_number.
- Finally, we print the value of oct_number.
Type Conversion In Python With tuple() Function
The tuple() function is primarily used for converting a sequence (such as a list, string, or range) into a tuple. It takes a single parameter, which is the sequence to be converted, and returns a tuple containing the elements of the sequence.
Syntax:
tuple(iterable)
Here,
- tuple: The tuple() function in Python is used to convert a sequence (such as a list, string, or range) into a tuple.
- iterable: The sequence (such as a list, string, or range) that will be converted into a tuple.
Code:
IyBUeXBlIENvbnZlcnNpb24gSW4gUHl0aG9uIHdpdGggdHVwbGUoKQoKIyBEZWZpbmUgdmFyaWFibGVzIG9mIGRpZmZlcmVudCB0eXBlcwoKaW50X3ZhciA9IDEwCgpmbG9hdF92YXIgPSAzLjE0CgpzdHJfdmFyID0gIkhlbGxvIgoKbGlzdF92YXIgPSBbMSwgMiwgM10KCnR1cGxlX3ZhciA9ICg0LCA1LCA2KQoKIyBDb252ZXJ0IHZhcmlhYmxlcyBpbnRvIGEgdHVwbGUKCmNvbnZlcnRlZF90dXBsZSA9IHR1cGxlKFtpbnRfdmFyLCBmbG9hdF92YXIsIHN0cl92YXIsIGxpc3RfdmFyLCB0dXBsZV92YXJdKQoKcHJpbnQoIkNvbnZlcnRlZCBUdXBsZToiLCBjb252ZXJ0ZWRfdHVwbGUpCgpwcmludCgiVHlwZSBvZiBjb252ZXJ0ZWRfdHVwbGU6IiwgdHlwZShjb252ZXJ0ZWRfdHVwbGUpKQ==
Output:
Converted Tuple: (10, 3.14, 'Hello', [1, 2, 3], (4, 5, 6))
Type of converted_tuple: <class 'tuple'>
Explanation:
In the above Python code,
- We start by defining variables of different types: int_var with an integer value of 10, float_var with a float value of 3.14, str_var with a string value of "Hello", list_var with a list of integers [1, 2, 3], and tuple_var with a tuple of integers (4, 5, 6).
- Next, we use the tuple() function to convert these variables into a tuple. We pass a list containing all these variables as an argument to tuple(), effectively converting them into a single tuple.
- We store the resulting tuple in the variable converted_tuple.
- Finally, we print the value of converted_tuple to see the combined tuple, which will include all the different variables.
- We also print the type of converted_tuple using the type() function to confirm that it is indeed a tuple.
Type Conversion In Python With set() Function
The set() function is generally used for type conversion in Python as it creates a set data structure. Sets are unordered collections of unique elements. It takes an iterable (such as a list, tuple, string, etc.) as input and returns a set containing the unique elements from the iterable. If no iterable is provided, an empty set is returned.
Syntax:
set(iterable)
Here,
- set: The name of the function.
- iterable: The parameter that specifies the iterable object from which the set will be created.
Code:
bGlzdF92YWx1ZSA9IFsxLCAyLCAyLCAzXQoKc2V0X3ZhbHVlID0gc2V0KGxpc3RfdmFsdWUpCgpwcmludChzZXRfdmFsdWUp
Output:
{1, 2, 3}
Explanation:
In the above Python code,
- We start by defining a variable list_value with a list of integers: [1, 2, 2, 3].
- To convert this list into a set, we use the set() function and store the result in the variable set_value.
- The set() function removes duplicate values and only keeps unique elements.
- Finally, we print the value of set_value.
Type Conversion In Python With list() Function
The list() function in python is primarily used to convert other iterable data types, such as tuples or strings, into a list. It takes a single parameter, which can be any iterable object, and returns a new list containing the elements of that iterable.
Syntax:
list(iterable)
Here,
- list: This is the name of the function being called. In Python, list() is a built-in function used to convert iterable objects into lists.
- iterable: This is the parameter that the list() function takes. It represents the object that you want to convert into a list. It must be an iterable object, such as a string, tuple, or another list.
Code:
IyBDb252ZXJ0IG11bHRpcGxlIHR5cGVzIG9mIHZhcmlhYmxlcyBpbnRvIGxpc3RzIG9mIGludGVnZXJzCgp2YXJpYWJsZTEgPSAxMAoKdmFyaWFibGUyID0gIjIwIgoKdmFyaWFibGUzID0gWzMwLCA0MCwgNTBdCgp2YXJpYWJsZTQgPSAoNjAsIDcwLCA4MCkKCiMgQ29udmVydGluZyB2YXJpYWJsZXMgaW50byBsaXN0cyBvZiBpbnRlZ2VycwoKY29udmVydGVkX2xpc3QxID0gW3ZhcmlhYmxlMV0KCmNvbnZlcnRlZF9saXN0MiA9IFtpbnQodmFyaWFibGUyKV0KCmNvbnZlcnRlZF9saXN0MyA9IGxpc3QobWFwKGludCwgdmFyaWFibGUzKSkKCmNvbnZlcnRlZF9saXN0NCA9IGxpc3QobWFwKGludCwgdmFyaWFibGU0KSkKCiMgT3V0cHV0CgpwcmludCgiQ29udmVydGVkIGxpc3QgMToiLCBjb252ZXJ0ZWRfbGlzdDEpCgpwcmludCgiQ29udmVydGVkIGxpc3QgMjoiLCBjb252ZXJ0ZWRfbGlzdDIpCgpwcmludCgiQ29udmVydGVkIGxpc3QgMzoiLCBjb252ZXJ0ZWRfbGlzdDMpCgpwcmludCgiQ29udmVydGVkIGxpc3QgNDoiLCBjb252ZXJ0ZWRfbGlzdDQp
Output:
Converted list 1: [10]
Converted list 2: [20]
Converted list 3: [30, 40, 50]
Converted list 4: [60, 70, 80]
Explanation:
In the above Python code,
- We start by defining four variables of different types: variable1 with an integer value of 10, variable2 with a string value of "20", variable3 with a list of integers [30, 40, 50], and variable4 with a tuple of integers (60, 70, 80).
- Then we use the list() function in combination with some other function to convert these variables into lists of integers, as follows:
- We convert variable1 to a list using list literals and store the outcome in variable converted_list1.
- Using the int() function with list literals, we convert variable2 from string to list and store the outcome in converted_list2.
- Then, we use the list() function with map() function to convert variables- variable3 and variable4 to Python lists. The outcomes are stored in converted_list3 and converted_list4, respectively.
- Here, the int() function first converts each element of the tuple to an integer, and then the map() function converts the resulting map object to a list.
- Finally, we print the values of converted_list1, converted_list2, converted_list3, and converted_list4.
Also Read: How To Convert Python List To String? 8 Ways Explained (+Examples)
Type Conversion In Python With str() Function
The str() function in Python converts the specified value into a string. It takes any data type as input and returns a string representation of that value.
Syntax:
str(value)
Here,
- str: The str() function returns the string representation of the input value.
- value (mandatory): This parameter specifies the value that you want to convert into a string.
Code:
aW50ZWdlcl9udW1iZXIgPSAxMjMKCnN0cmluZ192YWx1ZSA9IHN0cihpbnRlZ2VyX251bWJlcikKCnByaW50KHN0cmluZ192YWx1ZSk=
Output:
123
Explanation:
In the above Python code,
- We start by defining a variable integer_number containing the integer value 123.
- Next, we use the str() function to convert the integer value integer_number into a string and store it in the variable string_value.
- Finally, we use the print() function to display the value of string_value, confirming that the integer value has been converted to a string.
Type Conversion In Python With dict() Function
The dict() function converts iterable objects (like tuples, lists, etc.) into a dictionary by creating a new dictionary object. It can be used for type conversion in Python even without arguments, in which case it returns an empty dictionary. The function can also take another dictionary or an iterable containing key-value pairs as an argument.
Syntax:
dict(iterable)
Here,
- dict(): Name of the function.
- iterable: Optional argument, an iterable object containing key-value pairs.
Code:
IyBDb252ZXJ0aW5nIG11bHRpcGxlIHR5cGVzIG9mIHZhcmlhYmxlcyBpbnRvIGludGVnZXJzIHVzaW5nIGRpY3QoKQoKbWl4ZWRfZGF0YSA9IHsnYSc6ICcxMCcsICdiJzogMjAuNSwgJ2MnOiBUcnVlLCAnZCc6ICczMCd9Cgpjb252ZXJ0ZWRfZGljdCA9IGRpY3QoKGtleSwgaW50KHZhbHVlKSkgZm9yIGtleSwgdmFsdWUgaW4gbWl4ZWRfZGF0YS5pdGVtcygpKQoKcHJpbnQoY29udmVydGVkX2RpY3Qp
Output:
{'a': 10, 'b': 20, 'c': 1, 'd': 30}
Explanation:
In the above Python code,
- We start by defining a dictionary mixed_data containing values of different types: 'a': '10' (a string), 'b': 20.5 (a float), 'c': True (a boolean), and 'd': '30' (a string).
- Then, we convert all these values to integers using dictionary comprehension within the dict() function.
- This comprehension iterates over each key-value pair in mixed_data, converting each value to an integer using the int() function, and then creates a new dictionary with the same keys but with the integer-converted values in variable converted_dict.
- Finally, we print the converted_dict.
Type Conversion In Python With complex() Function
The complex() function takes two integer-type parameters, real and imag, and converts them into a complex number. Here, the real represents the real part of the complex number and imag represents the imaginary part. If the imag argument is omitted, it defaults to zero.
Syntax:
complex(real[, imag])
Here,
- complex(): This function returns a complex number constructed from its arguments.
- real: Represents the real part of the complex number (can be any numeric type).
- imag: Represents the imaginary part of the complex number (can be any numeric type).
Code:
cmVhbF9wYXJ0ID0gMwppbWFnX3BhcnQgPSA1Cgpjb21wbGV4X251bWJlciA9IGNvbXBsZXgocmVhbF9wYXJ0LCBpbWFnX3BhcnQpCgpwcmludChjb21wbGV4X251bWJlcik=
Output:
(3+5j)
Explanation:
In the above Python code,
- We start by defining two variables: real_part with an integer value of 3 and imag_part with an integer value of 5.
- We then create a complex number using the complex() function, passing real_part to integer 3 and imag_part to integer 5 as arguments, respectively.
- We store the resulting complex number in the variable complex_number.
- Finally, we print the value of complex_number.
Type Conversion In Python With chr(number) Function
The chr() function is commonly used for type conversion in Python to convert an integer representing a Unicode code point into its corresponding character.
Syntax:
chr(number)
Here,
- chr(number): This is the syntax of the chr() function.
- number: The parameter representing the Unicode code point to be converted.
Code:
IyBDb252ZXJ0aW5nIHZhcmlvdXMgdHlwZSBvZiB2YXJpYWJsZXMgaW50byBpbnRlZ2VycyB1c2luZyBjaHIoKQoKIyBDb252ZXJ0aW5nIGludGVnZXJzCgppbnRfbnVtID0gY2hyKDY1KQoKcHJpbnQoIkludGVnZXI6IiwgaW50X251bSkKCiMgQ29udmVydGluZyBoZXhhZGVjaW1hbAoKaGV4X251bSA9IGNocigweDQxKQoKcHJpbnQoIkhleGFkZWNpbWFsOiIsIGhleF9udW0pCgojIENvbnZlcnRpbmcgb2N0YWwKCm9jdF9udW0gPSBjaHIoMG8xMDEpCgpwcmludCgiT2N0YWw6Iiwgb2N0X251bSkKCiMgQ29udmVydGluZyBiaW5hcnkKCmJpbmFyeV9udW0gPSBjaHIoMGIxMDAwMDAxKQoKcHJpbnQoIkJpbmFyeToiLCBiaW5hcnlfbnVtKQoKIyBDb252ZXJ0aW5nIHVuaWNvZGUgY29kZSBwb2ludAoKdW5pY29kZV9udW0gPSBjaHIoOTczMSkKCnByaW50KCJVbmljb2RlOiIsIHVuaWNvZGVfbnVtKQ==
Output:
Integer: A
Hexadecimal: A
Octal: A
Binary: A
Unicode: ☃
Explanation:
In the above Python code:
- We start by using the chr() function to convert various types of numeric representations into characters.
- For int_num, we use chr(65), where 65 is the Unicode code point for the character 'A'. We store this character in int_num and print it.
- For hex_num, we use chr(0x41), where 0x41 is the hexadecimal representation for the Unicode code point 65, which corresponds to the character 'A'. We store this character in hex_num variable and print it.
- For oct_num, we use chr(0o101), where 0o101 is the octal representation for the Unicode code point 65, which corresponds to the character 'A'. We store this character in oct_num variable and print it.
- For binary_num, we use chr(0b1000001), where 0b1000001 is the binary representation for the Unicode code point 65, which corresponds to the character 'A'. We store this character in binary_num variable and print it.
- For unicode_num, we use chr(9731), where 9731 is the Unicode code point for the character ☃ (Snowman). We store this character in unicode_num variable and print it.
Important Type Conversion Tips In Python
When performing type conversion in Python, you must remember a few key concepts to ensure that the conversion is done effectively. They are as follows:
-
Data Loss: Be mindful of potential data loss when converting between different input data types. For example, converting a floating-point number to an integer will truncate the decimal part, potentially leading to a loss of precision. For example:
float_num = 5.78
int_num = int(float_num) # Truncates the decimal part
print(int_num) # Outputs: 5
-
Error Handling: Handle exceptions that may occur during type conversion in Python, especially when dealing with user involvement or external data sources. Invalid inputs may raise ValueError or TypeError. For example:
user_input = "abc"
try:
num = int(user_input) # This will raise a ValueError
except ValueError:
print("Invalid input! Please enter a valid number.")
-
Explicit vs. Implicit Conversion: Understand when to use explicit conversion (using built-in functions like int(), float(), etc.) and when Python performs implicit conversion automatically. Note that explicit type conversion in Python provides greater control over the conversion process.
-
Contextual Conversion: Consider the context in which the type conversion is performed. For example, converting a string representing a date to a datetime object requires knowledge of the date format.
-
Type Checking: Validate data types before performing type conversion in Python, especially when dealing with mixed-type data. You can use isinstance() or type() functions to ensure compatibility.
-
Efficiency: Type conversion in Python may have performance implications, especially in large-scale applications. You must minimize unnecessary conversions to improve performance.
-
Unicode Handling: When converting between string types in Python (str and bytes), consider Unicode encoding/decoding to handle different character encodings properly. For example:
text = "hello"
encoded_text = text.encode('utf-8') # Convert string to bytes using UTF-8 encoding
decoded_text = encoded_text.decode('utf-8') # Convert bytes back to string
print(decoded_text) # Outputs: hello
-
Immutable vs. Mutable Types: Note the difference in behavior when converting between immutable types (e.g., str, tuple) and mutable types (e.g., list, dict). Such type conversion may involve creating new objects or modifying existing ones.
-
Documentation: Document-type conversion operations, especially if they are non-trivial or part of a larger algorithm. Clear documentation helps improve code readability and maintainability. For example:
# Convert a string to a list of integers
def convert_string_to_list(s):
"""
Converts a comma-separated string to a list of integers.
Example:
convert_string_to_list("1,2,3") -> [1, 2, 3]
"""
return [int(x) for x in s.split(',')]
-
Testing: Test type conversion logic thoroughly, covering different edge cases and input scenarios. Automated testing frameworks can assist in verifying the correctness of conversion functions.
Benefits Of Type Conversion In Python
Type conversion in Python programs comes with a wide range of benefits, including:
- Ensures Compatibility: Type conversion in Python ensures that different data types can be used together in operations. For instance, if you add an integer to a float, Python automatically converts the integer to a float to perform the addition.
- Simplifies Coding: Implicit type conversion reduces the need for explicit type conversion commands in simple scenarios. Python handles the conversion for you, which makes the code cleaner and more concise.
- Enhances Code Flexibility: Type conversion in Python allows for the use of various data types in different operations, providing flexibility in how you handle and manipulate data.
- Supports Diverse Data Types: Python’s type conversion functions support a wide range of data types, including numeric data types, string data types, and more complex structures like lists and tuples. For example:
float_num = 3.14
int_num = int(float_num) # Convert float to int
print(int_num) # Outputs: 3
- Improves Data Handling: Explicit type casting helps you control how data types are converted, which is essential for accurate calculations, data formatting, and other operations.
- Avoids Errors: Proper use of type conversion in Python prevents type errors that occur when operations are performed on incompatible data types. For example:
text = "100"
number = 50
result = int(text) + number # Convert text to int to avoid type mismatch
print(result) # Outputs: 150
- Supports Complex Operations: Type conversion is used in complex mathematical operations and data manipulations, allowing you to perform calculations and transformations on different data types. For example:
value = 10
result = value / 3 # Implicitly converts int to float type for division
print(result) # Outputs: 3.3333333333333335
- Ensures Data Integrity: Explicit type conversion in Python helps maintain data integrity by clearly defining how data types should be converted, which prevents unintentional errors.
- Cross-Domain Usage: Type conversion in Python facilitates the reuse of code across different domains or applications by allowing data to be transformed and adapted to suit specific requirements without rewriting significant portions of code.
Conclusion
Type conversion in Python is a fundamental concept that enables you to transform data from one type to another. It helps ensure compatibility, accuracy, and efficiency in your Python programs. There are two types of type conversion in Python, i.e., implicit and explicit. They allow you to manage data types effectively, in various scenarios such as simple arithmetic operations or complex data transformations.
Whether you’re dealing with data type conversion for user inputs, performing mathematical calculations, or integrating data from various sources, a solid grasp of type conversion principles is essential. Utilizing built-in functions like int(), float(), and str() will help you write more reliable and maintainable Python code.
Frequently Asked Questions
Q. What are primitive and non-primitive data types?
Primitive and non-primitive data types are fundamental concepts in programming that help us understand how data is stored and manipulated.
Primitive data types are the most basic data types provided by a programming language, representing single values.
- Examples include integer data type, float data type, characters, and other larger data types.
- These types are directly supported by the language and typically have a fixed size and operations associated with them.
- For instance, an integer type holds whole numbers, and a float holds decimal numbers.
Non-primitive data types, also known as composite or reference types, are built from primitive types and can hold multiple values or more complex structures.
- Examples include arrays, lists, objects, and strings.
- These types can be manipulated using various methods and can contain collections of primitive data types or other non-primitive types.
- For instance, a string is a sequence of characters, and a list can hold integers, floats, strings, or even other lists.
Q. What is type casting in C language and its types?
In C language, typecasting refers to the process of converting a variable from one data type to another. This is done to ensure that operations involving different types of data are performed correctly or to meet specific requirements of functions and operations. Typecasting is essential for managing data precision and performing arithmetic operations where data types differ.
There are two main types of typecasting in C:
-
Implicit Typecasting (Automatic Type Conversion): This occurs automatically when we perform operations involving different data types. For example, if we add an integer datatype to a float datatype, C will implicitly convert the integer to a float before performing the addition. This typecasting is handled by the compiler to ensure that the operation is carried out with the appropriate data type.
-
Explicit Typecasting (Manual Type Conversion): This requires us to manually convert a variable from one type to another using a typecast operator. For example, to convert a float to an integer, we use (int)float_variable, which truncates the decimal part and keeps only the integer part. Explicit typecasting gives us more control over how the conversion is performed and is used to force data types in scenarios where implicit typecasting might not suffice.
Q. What is the difference between implicit and explicit type conversion in Python?
The main difference between implicit and explicit type conversion in Python lies in how they are performed. The table below highlights the difference between the two types.
Implicit Type Conversion | Explicit Type Conversion |
---|---|
Also known as automatic type conversion. | Also known as type casting. |
It occurs automatically, i.e., performed implicitly by a Python interpreter when needed. | Performed manually by the programmer using built-in functions like int(), float(), str(), etc. |
Happens implicitly during operations involving different data types. | Requires the programmer to specify the desired type conversion explicitly. |
The programmer does not have control over implicit conversion since it is done internally by the compiler/ interpreter. | Provides more control over the conversion process. |
Can lead to unexpected results if not managed carefully. | Helps ensure data integrity and avoids unexpected behaviors. |
Q. Can implicit type conversion lead to errors?
Yes, implicit type conversion in Python can lead to unexpected results or errors if not managed carefully. Since the conversion is done automatically by the interpreter, it may not always produce the desired outcome, especially when dealing with operations that involve different data types.
Q. Why is explicit type conversion important?
Explicit type conversion or type casting in Python is important because it provides more control over the conversion process, ensuring data integrity and avoiding unexpected behaviors. It allows the programmer to specify exactly how and when data types should be converted, leading to more predictable and reliable code.
You might also be interested in reading the following:
- Fibonacci Series In Python & Nth Term | Generate & Print (+Codes)
- Swap Two Variables In Python- Different Ways | Codes + Explanation
- Difference Between C and Python | C or Python - Which One Is Better?
- Convert Int To String In Python | Learn 6 Methods With Examples
- How To Reverse A String In Python? 10 Easy Ways With Examples
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment