Python Programming
Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
String Slicing In Python | Syntax, Usage & More (+Code Examples)
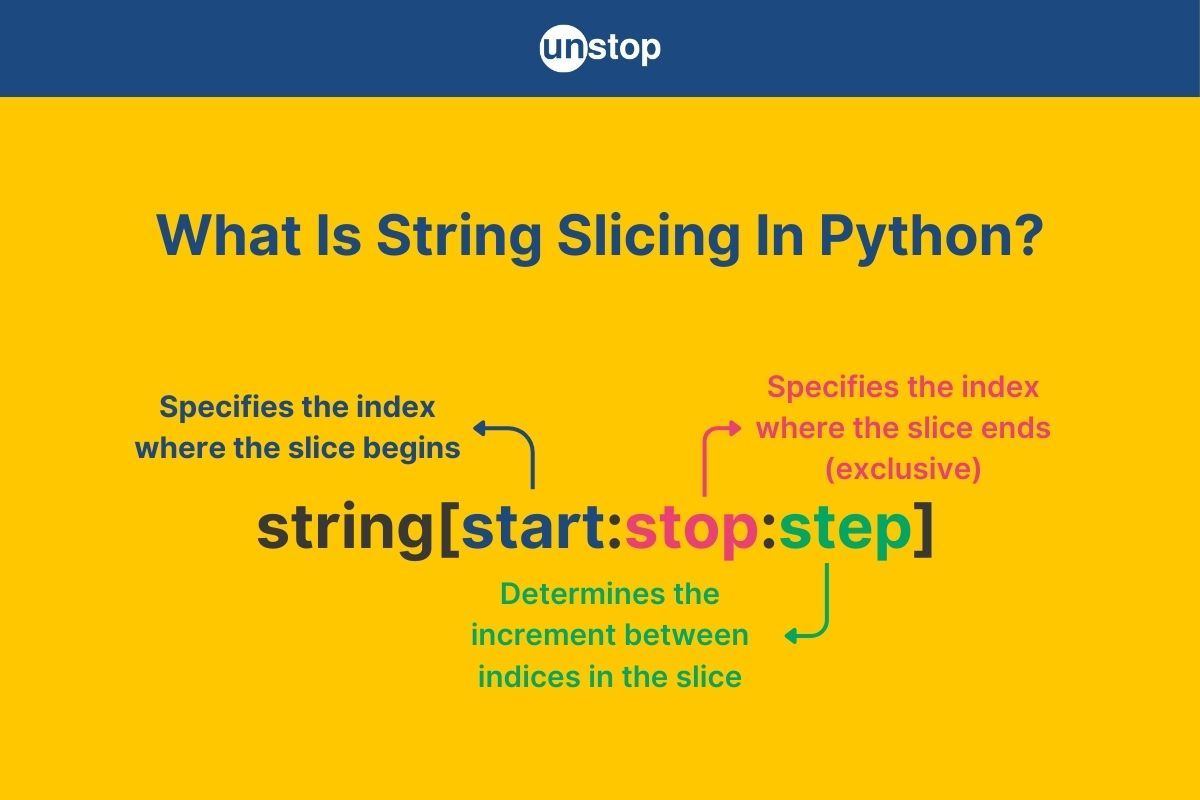
Strings in Python are sequences of characters often used to store and manipulate text data. They are one of the most fundamental data types in Python and are incredibly versatile for various operations, including slicing.
In this article, we'll explore string slicing in Python—how it works, what you can do with it, and how it plays a vital role in many real-world applications. Whether you're a beginner or have some experience with Python, understanding string slicing can significantly improve your ability to handle and manipulate strings effectively.
What Is String Slicing In Python?
String slicing is a technique that allows you to extract portions of a string in Python (or even the entire string) by specifying a range of indices. With slicing, you can easily grab specific characters, subsets of characters (substrings), or even reverse the string, all with a simple syntax.
Syntax Of String Slicing In Python
string[start:end:step]
Parameters Of String Slicing In Python
The string slicing syntax takes three parameters, as follows:
- start: This optional parameter refers to the index where the slice begins. If omitted, the slice starts from the beginning of the string (i.e., default is 0).
- end: This optional parameter provides the index where the slice ends. If omitted, the slice goes until the end of the string (default is the end of the string).
- step: The optional parameter defines the interval between each character. If omitted, the default step is 1.
Return Value Of String Slicing In Python
String slicing returns a new string that consists of the characters from the specified range. If any part of the range is out of bounds, Python will handle it gracefully and return the valid portion of the string or an empty string.
How Indexing & String Slicing Works In Python
String slicing in Python programming is based on the concept of indexing, which is used to identify the position of characters in a string. Indexing can be positive (starting from the left) or negative (starting from the right). This dual indexing system provides flexibility for accessing and slicing strings.
Indexing In Python (Positive & Negative)
Positive indexing starts from 0 and increases as you move from left to right. So, the first character of the string has an index of 0, the second has an index of 1, and so on. You can use this index value to access the characters, as shown below:
string = "Unstop"
print(string[0]) # Output: U
print(string[3]) # Output: t
The term negative indexing, as the name suggests, is the opposite of positive indexing. The negative index starts from -1 for the last character and decreases as you move from right to left. These indexes are especially useful when you do not know the length of a string and want to access the last element. You can simply use the -1 index, as shown in the example below:
string = "Unstop"
print(string[-1]) # Output: p
print(string[-3]) # Output: t
Indexing & String Slicing In Python
String slicing leverages indexing to specify the start and end points of the slice, as well as the step value. For example, if you want to extract a substring, i.e., a portion of the string, you can use the start and end index to specify the beginning and the end of the substring.
Code Example:
#Original string
string = "Unstoppable"
# Slicing characters from index 0 to 2
slice_1 = string[0:3]
# Slicing characters from index -4 to -2
slice_2 = string[-4:-1]
print(slice_1)
print(slice_2)
Output:
Uns
abl
Code Explanation:
In the simple Python program example:
- We begin by creating a variable named string and assign the value “Unstoppable” to it.
- Then, we use the slicing technique to extract portions of the string.
- In the first slice ([0:3]), the slice starts at index 0 and ends just before index 3.
- In the second slice ([-4:-1]), the slice starts at index -4 and ends just before index -1.
Slicing syntax respects the principle of half-open intervals, where the start is inclusive and the end is exclusive.
Also read: Python List index() Method | Use Cases Explained (+Code Examples)
Extracting All Characters Using String Slicing In Python
Sometimes, you may want to extract the entire content of a string without any modifications. For example, duplicating a string to make manipulation while also preserving the original one. Python makes this effortless with string slicing, allowing you to retrieve all characters by omitting the start, end, and step values.
How It Works: When you use the slicing syntax without specifying any parameters (i.e., [:]), Python assumes:
- Start: The first character of the string (index 0).
- End: The last character of the string (index len(string) - 1).
- Step: The default value 1, meaning it selects every character sequentially.
This creates a new string containing all characters from the original string. The simple Python code example below illustrates how to do this.
Code Example:
#Original string
string = "Python Programming"
#Using slicing without any parameters/arguments
all_characters = string[:]
print(all_characters)
Output:
Python Programming
Code Explanation:
- We use the slicing syntax without any parameters [:] on the string variable, i.e., string[:].
- This starts extracting the characters starting from index 0 to the end of the string.
- Since no parameters are specified, it defaults to extracting every character in order.
- The result is a new string identical to the original string, which we display using the print() function.
Extracting Characters Before & After Specific Position Using String Slicing In Python
String slicing can be used to extract characters either before or after a specific position in a string. This allows you to isolate parts of the string based on positional requirements.
Extracting Characters Before Specific Position
To extract characters before a specific position, set the end index to the desired position (exclusive) and omit the start index.
Code Example:
#Original string
string = "Practice on Unstop"
#Extracting characters before index 8 (exclusive)
before_position = string[:8]
print(before_position)
Output:
Practice
Code Explanation:
In the basic Python program example:
- We begin with the string containing– “Practice on Unstop”.
- Then, we use the slicing syntax with the end index 8, i.e., string[:8]. This extracts characters from the start of the string (index 0) up to, but not including, index 8.
- We store the outcome in the variable before_position and print it to the console.
Extracting Characters After Specific Position
To extract characters after a specific position, set the start index to the position and omit the end and start index.
Code Example:
string = "Practice on Unstop"
#Slicing with the start index 12 (included)
after_position = string[12:]
print(after_position)
Output:
Unstop
Code Explanation:
In the basic Python code example:
- We begin with the same string as before. Then, we use the slicing syntax with only the start argument 7, i.e., string[7:].
- This extracts characters starting from index 7 to the end of the string.
- The result excludes characters before index 7, forming the substring "Unstop".
Check out this amazing course to become the best version of the Python programmer you can be.
Extracting Characters Between Two Intervals Using String Slicing In Python
String slicing allows you to extract a substring by specifying both the start and end indices, creating a subset of characters between two intervals. This is especially useful when you need a precise portion of the string.
How It Works: To extract characters between two intervals, use the slicing syntax string[start:end], where start specifies the beginning of extraction (and it is inclusive), and end specifies where the extraction stops (and it is exclusive). The Python program example below illustrates how this works.
Code Example:
string = "Python Programming"
#Extracting a substring between an interval
substring = string[7:18]
print(substring)
Output:
Programming
Code Explanation:
In the Python code example:
- We begin with the string– “Python Programming” and then use the slicing technique to extract the substring from 7 to 18, i.e., string[7:18].
- So, the extraction starts from index 7 (inclusive), which corresponds to the letter P, and ends at index 18 (exclusive), which corresponds to the letter after g.
- The result is the substring "Programming", which lies between these two intervals.
Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
String slicing also allows you to extract characters at specific intervals or steps, enabling selective extraction by skipping characters in the string. This is achieved using the optional step parameter in the slicing syntax.
How It Works: In this case, you use all the parameters with the slicing syntax string[start:end:step].
- The start defines where slicing begins, the end defines where it ends (exclusive,) and the step stipulates the interval at which the characters must be extracted.
- You can omit the start and end indices but not the step index.
- Also, a positive step extracts characters from left to right, while a negative step reverses the extraction direction.
The example Python program below illustrates how the step index affects the slicing process and helps extract characters after a fixed interval.
Code Example:
string = "Python Programming"
#Specifying step 2 helps skip to every other character
step_slice = string[0:18:2]
print(step_slice)
Output:
Pto rgamn
Code Explanation:
In the example Python code:
- We begin with the string– “Python Programming”.
- Then, we use the slicing technique specifying start index of 0, end index of 18, and step index of 2, i.e., string[0:18:2].
- As a result, the slicing starts from index 0 (the letter P), ends just before index 18, and it extracts every second character (step = 2), skipping one character in between.
- The result is "Pto rgamn" which includes characters at indices 0, 2, 4, 6, and so on.
Negative Indexing & String Slicing In Python
Python supports negative indexing, which allows you to reference characters in a string starting from the end. Negative indexing is particularly useful when working with strings where you need to extract characters relative to the string's end rather than the beginning.
- Negative indices count backward from the end of the string:
- The last character is indexed as -1.
- The second-to-last character is indexed as -2, and so on.
- You can use negative indices in string slicing to extract substrings starting from or ending at specific positions relative to the string's end.
Code Example:
string = "Python Programming"
#Using negative indices
negative_slice = string[-18:-7]
print(negative_slice)
Output:
Python Prog
Code Explanation:
In the sample Python code:
- We have the same string as before, but here, we use negative indices, -18 as start and -7 as end, i.e., string[-18:-7].
- As a result, the slicing begins at the character indexed -18, which corresponds to the first character of the string (P).
- And it stops at the character indexed -7 (exclusive). Since negative indexes begin at -1, and not 0, the 7th character is ‘r’, which is why the portion "ramming" is excluded.
Reversing A String Using String Slicing In Python
One powerful use of negative indexing is reversing a string. By specifying a negative step value (-1) in the slicing syntax, you can extract characters from the end of the string back to the beginning, effectively reversing the string.
Code Example:
string = "Python Programming"
#Slicing with negative step, omitting start and end
reversed_string = string[::-1]
print(reversed_string)
Output:
gnimmargorP nohtyP
Code Explanation:
In the sample Python program:
- We use the slice syntax with the negative step of -1, i.e., string[::-1].
- Since we do not specify an end, the slicing starts from the end of the string and moves backward with a step value of -1.
- In addition, since no start or end indices are specified, it defaults to reversing the entire string.
- The result is "gnimmargorP nohtyP", which is the original string in reverse order.
Handling Out-of-Bounds Indices In String Slicing In Python
Python handles out-of-bounds indices gracefully when slicing strings, ensuring the operation does not throw errors. This feature provides flexibility and makes string slicing more robust.
How It Works: When an index in the slicing syntax exceeds the length of the string:
- Python automatically adjusts the index to stay within the valid range.
- No IndexError is raised.
Similarly, negative indices that are less than -len(string) default to the first character of the string.
Code Example:
string = "Unstop"
# End index exceeds the length
slice_out_of_bounds = string[:50]
print(slice_out_of_bounds)
Output:
Unstop
Code Explanation:
In the Python program sample, we use the slicing syntax with an end index of 50, which is beyond the string’s length. Here, Python adjusts the slicing to stop at the last character, returning "Unstop".
Negative Out-of-Bounds Index In String Slicing In Python
When you specify a negative index in string slicing that is smaller than -len(string), Python treats it as if you were starting from the beginning of the string. In simpler terms:
- If the negative start index is more negative than the valid range (-len(string)), Python adjusts it to 0 (the first character of the string).
- Similarly, if a negative end index is smaller than -len(string), Python behaves as though the slice stops at the first character.
Code Example:
string = "Unstop"
#Slice with negative indice that is smaller than the length
negative_out_of_bounds = string[-50:]
print(negative_out_of_bounds)
Output:
Unstop
Code Explanation:
In the Python code sample, the slicing syntax has a start index of -50, which is far smaller than -len(string) (which is -6 for "Python"). So, the Python language adjusts the start index to 0, effectively slicing from the beginning. The result is the entire string, "Unstop".
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
The slice() Method For String Slicing In Python
While slicing strings using the traditional [start:end:step] syntax is common, Python also provides the slice() method as an alternative for more programmatically defined slicing.
- This built-in function in Python can be particularly useful when the slicing parameters are determined dynamically at runtime.
- It creates a slice object, which specifies the start, end, and step parameters for slicing.
- This slice object can then be applied to a string (or other sequence types like lists in Python) using square brackets.
Syntax:
slice(start, stop, step)
Here,
- start: The starting index of the slice (inclusive).
- stop: The ending index of the slice (exclusive).
- step: The interval at which elements are extracted.
Code Example:
string = "Python Programming"
# Using slice() method equivalent to string[0:6]
slice_obj = slice(0, 6)
result = string[slice_obj]
print(result)
Output:
Python
Explanation:
Here, we call the slice() function with arguments starts at index 0 and ends before index 6. This returns a slice object, slice_obj, that specifies the start and stop indices for slicing the string. Then, we apply this object to the string (using square brackets), extracting the substring "Python", as it applies the slice object’s start and stop indices.
Common Pitfalls Of String Slicing In Python
String slicing in Python is intuitive but can sometimes lead to common mistakes, especially for beginners. Here, we’ll cover some of the most frequent pitfalls and how to avoid them.
- Misunderstanding Index Ranges: Many beginners get confused when working with the [start:end] range, especially with the inclusive start index and exclusive end index. Example:
string = "Python"
print(string[1:4]) # Output: 'yth'
In the example above, the slice starts at index 1 and includes indices 1, 2, and 3 but excludes index 4.
- Forgetting the Step Argument: The step argument controls how many indices to skip between elements, but sometimes it's omitted unintentionally, leading to the assumption that it's required. Omitting the step argument defaults it to 1, meaning every character will be included. Example:
string = "Python"
print(string[::2]) # Output: 'Pto'
Here, ::2 skips every second character starting from the beginning, producing "Pto".
- Negative Indexing Confusion: When using negative indices, beginners often mistake how Python handles them, especially when slicing with a negative step. Remember that negative indices count from the end of the string, with -1 referring to the last character. Example:
string = "Python"
print(string[4:-2]) # Output: 'tho'
Here, 4:-2 slices the string from index 4 to two positions before the end (index -2), giving "tho".
- Off-By-One Errors: This happens when you mistakenly include or exclude one extra element because of the exclusive nature of the end index. To fix this, always double-check the range to make sure it's as expected. Example:
string = "Python"
print(string[2:5]) # Output: 'tho'
Here, it starts at index 2 (inclusive) and goes up to index 5 (exclusive), returning "tho". If you wanted to include index 5, you would need to adjust the end index to 6.
Real-World Applications Of String Slicing
String slicing isn’t just a useful tool for technical tasks—it has a variety of real-world applications in different industries. Here's how string slicing can be applied across various domains.
- Data Cleaning in Data Science: In data science, it's common to clean and preprocess large datasets, which often contain strings that need to be split, trimmed, or formatted. For example, when working with dates or phone numbers, string slicing can be used to extract specific parts of the string, such as the year, month, or area code, without affecting other data in the record.
Example: A data analyst working with sales data may need to extract the year from a "YYYY-MM-DD" formatted string to analyze yearly trends. - Parsing Log Files in Software Engineering: In software development, log files often contain large amounts of data. Developers need to extract specific entries such as error codes, timestamps, or user actions. String slicing makes it easy to isolate these components, allowing engineers to quickly analyze log data and diagnose issues in real-time.
Example: A system administrator may use string slicing to extract time stamps or error codes from server logs for troubleshooting and performance monitoring. - Text Manipulation in Web Development: In web development, string slicing plays a vital role in manipulating text-based data, such as URLs, user inputs, or JSON data. Whether it's extracting a query parameter from a URL or formatting a user input string for further processing, string slicing helps developers efficiently handle text.
Example: A web developer may slice strings to extract specific values from URL query parameters (e.g., https://example.com?id=12345), allowing dynamic content rendering on a website. - Bioinformatics for DNA Sequence Analysis: In bioinformatics, string slicing is heavily used for analyzing DNA and RNA sequences. The sequences, made up of long strings of nucleotides (A, T, C, G), are often sliced to extract subsequences for research purposes, such as finding specific gene patterns or mutations.
Example: A bioinformatician may slice DNA sequences to extract specific gene regions, aiding in genetic research and disease prediction. - Financial Analysis in Trading Systems: In financial systems, string slicing is often used to process and extract parts of financial data such as timestamps, stock symbols, or transaction identifiers. For example, slicing can be used to convert string-based time data into more usable formats or to analyze certain aspects of stock market data.
Example: A quantitative analyst may slice transaction timestamps to determine the frequency of trades over specific periods, providing insights into market behavior. - Text Processing in Natural Language Processing (NLP): NLP involves processing and analyzing large volumes of text. String slicing is an essential tool for breaking down text into smaller parts—such as extracting a word, sentence, or paragraph—from a larger body of text. This is particularly useful in tasks such as tokenization, sentiment analysis, and part-of-speech tagging.
Example: An NLP engineer might slice text to extract specific phrases or entities (like company names or dates) for further analysis in a chatbot or voice assistant application.
Conclusion
String slicing in Python is a powerful tool for manipulating strings efficiently. It allows you to extract specific parts of a string using the [start:end:step] syntax, which can be customized to meet various needs.
- However, when working with string slicing in Python, one must be aware of potential mistakes like off-by-one errors, confusion with negative indices, and misuse of the step parameter.
- In addition to the traditional slicing technique, the slice() function in Python provides a programmatically flexible way to handle string slicing. It can be dynamically used in situations where the slice parameters are not predefined.
By mastering string slicing, Python developers can handle text-based data more effectively, enhance performance, and write cleaner, more efficient code.
Ready to upskill your Python knowledge? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Frequently Asked Questions
Q1. What is [:] in Python?
The [:] syntax is a shorthand for slicing a whole string. It essentially means "take the entire string from start to end." Example:
string = "Python"
print(string[:]) # Output: 'Python'
Q2. What is slicing used for?
Slicing is primarily used to extract specific parts of a string (or other sequence types like lists). It can be used to split data, retrieve substrings, reverse sequences, and even manipulate text or other data in various ways.
Q3. How to generate a substring in Python?
A substring in Python can be generated by slicing a string using the [start:end] syntax. You specify the start index and the end index (exclusive), and Python will return the section of the string between these two indices. Example:
string = "Hello, World!"
print(string[7:12]) # Output: 'World'
Here, we use the Hello World! example and extract the second word as a substring.
Q4. How to check if a substring is present within a string in Python?
You can use the in keyword to check if a substring is present within a string. This is a more straightforward approach than slicing but can be useful in combination with slicing for more complex checks. Example:
string = "Hello, World!"
if "World" in string:
print("Substring found!")
Q5. What happens when the start index is greater than the end index?
When the start index is greater than the end index, the result will be an empty string, as no valid elements exist between those two indices. Example:
string = "Python"
print(string[5:2]) # Output: ''
Q6. Can I slice a string with a negative step?
Yes, you can slice a string with a negative step, which allows you to reverse the string or slice it in reverse order. In this case, the start index should be greater than the end index. Example:
string = "Python"
print(string[5:0:-1]) # Output: 'noht' (reversing a portion of the string)
Q7. Is string slicing a time-efficient operation?
Yes, string slicing is an efficient operation. Slicing is performed in constant time, meaning the time taken is proportional to the length of the substring being returned, and it does not affect the original string since strings in Python are immutable.
Q8. Can string slicing be used with lists or other sequences?
Yes, string slicing is not limited to strings; it can be used with any sequence type in Python, including lists, tuples, and even ranges. The syntax and principles remain the same across these sequence types. Example of list slicing in Python:
my_list = [1, 2, 3, 4, 5]
print(my_list[1:4]) # Output: [2, 3, 4]
Quick Python String Slicing Quiz– Let’s Go!
This compiles our discussion on string slicing in Python. Do check the following out:
- Python String Concatenation In 10 Easy Ways Explained (+Examples)
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Convert Int To String In Python | Learn 6 Methods With Examples
- Python List Copy Methods | Shallow & Deep Copies (+Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Pranjul Srivastav 1 day ago
kavita Prajapati 3 weeks ago
niyati m singh 3 weeks ago
Vishwajeet Singh 3 weeks ago
Pardha Venkata Sai Patnam 3 weeks ago
A Vishnuvardhan 3 weeks ago