Table of content:
- List Copy In Python
- Python Copy List Using Built-in copy() Method
- Python Copy List Using list() Method
- List Slicing Technique To Copy Python List
- Copying Python List Using Assignment Operator
- Potential Pitfalls Of Using Python Copy List Methods
- Shallow Copy & Deep Copy In Python
- Python copy() List Method From copy Module
- Advanced Techniques To Copy Python Lists
- Common Use Cases Of Copying Python Lists
- Conclusion
- Frequently Asked Questions
Python List Copy Methods | Shallow & Deep Copies (+Code Examples)
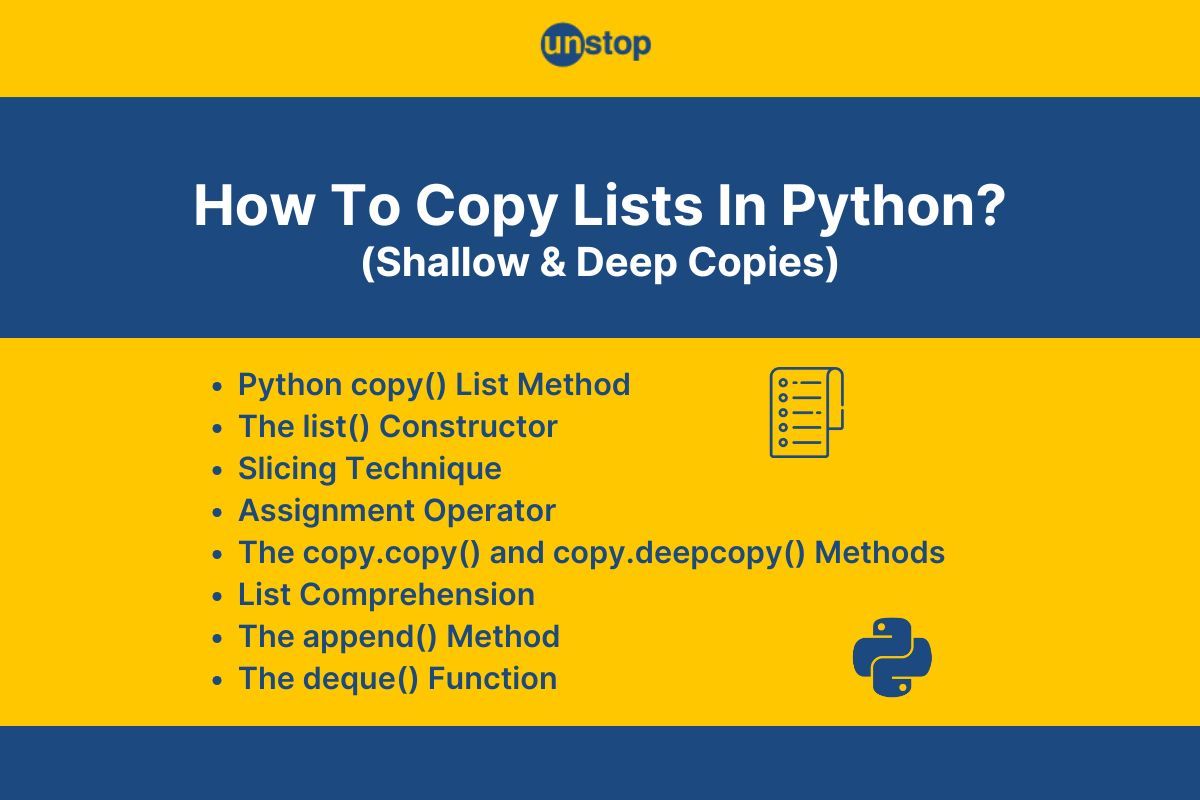
In Python, lists are one of the most versatile data structures, often used to store collections of items. But what happens when you need to make a copy of a list—whether to preserve the original or work with a duplicate in a separate context? It's not as straightforward as it may seem, especially when dealing with mutable data like lists.
In this article, we’ll dive into the various ways to copy lists in Python. Whether you're a beginner or an experienced programmer, understanding these methods is crucial to avoid unexpected behavior in your code.
List Copy In Python
Copying lists in Python may seem simple, but it's important to understand the behavior behind the different techniques available. Lists are mutable, meaning they can be changed after they're created. This mutability creates challenges when trying to duplicate a list. If you're not careful, copying a list could mean you’re only copying a reference to the original, not the actual data. This could lead to unintentional modifications.
Let's start by understanding the concept of list copying.
- When you copy a list, you're creating a new list with the same elements as the original.
- However, there are different ways to achieve this, depending on the method you choose.
- Some methods create shallow copies, meaning the inner elements of the list are still references to the original objects.
- Others, like deep copies, will create a completely independent copy of both the list and its inner elements, making it safer for complex data structures.
In the next sections, we’ll explore the various ways to copy a list in Python, starting with some of the most commonly used methods.
Need For Copying Python Lists
Copying lists in Python is crucial for preserving data integrity and preventing unintended changes. Since lists are mutable, modifications to one list can affect others if they share references. Here are a few reasons to copy lists:
- Prevent Unintended Modifications: Assigning one list to another creates a reference, not a copy. Modifying one will change both. Copying ensures each list is independent.
- Independent Data: When you need to work with a separate version of a list without altering the original (e.g., temporarily modifying or sorting the list).
- Function Flexibility: Passing a copied list to a function ensures the original list remains unchanged.
- Parallel Processing: Independent copies allow safe concurrent operations without data interference.
Copying lists may seem simple, but understanding when it’s necessary can prevent bugs. Now, let’s explore the methods to copy lists in Python.
Ways To Copy Python Lists
There are various ways to copy lists in Python programming, ranging from simple approaches to more advanced techniques for handling complex data structures. They are:
- Using the copy() Method: This creates a shallow copy of the list, meaning a new list is created, but the elements are references to the original objects in memory.
- Using the list() Constructor: This also creates a shallow copy of the list by converting the original list into a new one.
- List Slicing: A concise and efficient way to copy a list, using the slicing syntax new_list = old_list[:]. It creates a shallow copy of the list.
- Using the Assignment Operator: This method does not create a new copy. Instead, it creates a reference to the original list, so changes to one list affect the other.
- Using copy.copy(): This is a function from the copy module that creates a shallow copy of a list, similar to the copy() method, but it is more explicit.
- Using copy.deepcopy(): This method creates a deep copy, meaning it duplicates both the list and all its nested elements, ensuring complete independence between the original and the copy.
- Advanced Techniques for List Duplication:
List Comprehensions: Create a new list by iterating over an existing one.
Using the append() Method: Append elements one by one to a new list.
Using the deque() Function: The deque() function in Python from the collections module can be used to create an efficient copy of the list.
Each method has its advantages depending on the complexity of the list and the type of copy (shallow vs deep) you need. In the following sections, we will explore each of these methods in more detail with examples.
Python Copy List Using Built-in copy() Method
The copy() method is a built-in method in Python that is used to create a shallow copy of a list. That is, the elements of the new copy list are references to the objects from the original one. This method is straightforward and commonly used when you need to duplicate a list but doesn't require deep copying.
Syntax Of Python copy() List Method
list_copy = original_list.copy()
Here:
- The term original_list refers to the name of the list you want to copy.
- The term list_copy refers to the name of the new list that contains the same elements as the original list.
Parameters Of Python copy() List Method
No parameters are required for the copy() method. It simply operates on the list it’s called on and returns a new list with the same elements.
Return Value Of Python copy() List Method
The copy() method returns a shallow copy of the list, meaning:
- The new list is a separate object in memory.
- The elements of the new list are references to the same objects as those in the original list (if the list contains mutable elements).
- This means that while the outer list is duplicated, changes to mutable elements, such as nested lists, will be reflected in both the original and copied lists.
It is important to understand this when working with complex or nested data structures. The simple Python program example below illustrates how the Python copy() list method works. It also shows modifications made to the copy affect the original list.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm9yaWdpbmFsX2xpc3QgPSBbMSwgMiwgMywgWzQsIDVdXQoKIyBDb3B5IHRoZSBsaXN0IHVzaW5nIGNvcHkoKSBtZXRob2QKbGlzdF9jb3B5ID0gb3JpZ2luYWxfbGlzdC5jb3B5KCkKCiMgTW9kaWZ5aW5nIHRoZSBjb3BpZWQgbGlzdApsaXN0X2NvcHlbM11bMF0gPSA5OQoKIyBQcmludCBib3RoIGxpc3RzCnByaW50KCJPcmlnaW5hbCBMaXN0OiIsIG9yaWdpbmFsX2xpc3QpCnByaW50KCJDb3BpZWQgTGlzdDoiLCBsaXN0X2NvcHkp
Output:
Original List: [1, 2, 3, [99, 5]]
Copied List: [1, 2, 3, [99, 5]]
Code Explanation:
In the simple Python code example:
- We begin by creating a list original_list that contains both integers and a nested list.
- Then, we use the copy() method to create a shallow copy of the original list called list_copy.
- After that, we use the index values and direct assignment method to modify the first element (index–0) inside the nested list (index– 3) in list_copy.
- We then print both lists to the console. The output shows that the change is reflected in the original_list as well. This happens because the copy() method creates a shallow copy. The outer list is copied, but the inner list remains a reference to the same object in memory.
Python Copy List Using list() Method
The list() constructor is another way to create a shallow copy of a list. It’s particularly useful when you want to convert an iterable (such as another list or a tuple) into a list, but it can also serve as a way to duplicate an existing list.
Similar to the copy() method, the list() constructor creates a shallow copy. Changes to the outer list won’t affect the original, but modifications to mutable elements like nested lists will be reflected in both. The basic Python program example below illustrates this behaviour of the list() method and the shallow copy it produces.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm9yaWdpbmFsX2xpc3QgPSBbMTAsIDIwLCAzMCwgNDBdCgojIENvcHkgdGhlIGxpc3QgdXNpbmcgbGlzdCgpIGNvbnN0cnVjdG9yCmxpc3RfY29weSA9IGxpc3Qob3JpZ2luYWxfbGlzdCkKCiMgTW9kaWZ5IHRoZSBjb3BpZWQgbGlzdApsaXN0X2NvcHlbMV0gPSA5OQoKIyBQcmludCBib3RoIGxpc3RzCnByaW50KCJPcmlnaW5hbCBMaXN0OiIsIG9yaWdpbmFsX2xpc3QpCnByaW50KCJDb3BpZWQgTGlzdDoiLCBsaXN0X2NvcHkp
Output:
Original List: [10, 20, 30, 40]
Copied List: [10, 99, 30, 40]
Code Explanation:
In the basic Python code example:
- We create an original_list and then use the list() constructor to make a copy called list_copy.
- Next, we modify the element at the index 1 (2nd position) and replace the value 20 with 99 in the copied list.
- Then, we use the print() function to display both the lists to the console.
- It shows that original_list remains unchanged because the list() constructor creates a new list with the same elements, making it independent of the original list.
Note: This independence applies only to the outer list. If the list contains nested mutable objects, those will still be shared between the original and the copied list, as the list() constructor also creates a shallow copy.
List Slicing Technique To Copy Python List
List slicing is a simple and Pythonic way to create a shallow copy of a list. It allows you to extract a part or whole of the list and creates a new list. By using the slicing syntax, you can create a new list that contains the same elements as the original.
Syntax Of List Slicing:
list_copy = original_list[:]
The slicing syntax [:] essentially takes all elements of the original list and copies them into a new list. The Python program example below illustrates the same.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm9yaWdpbmFsX2xpc3QgPSBbJ2xlYXJuJywgJ3ByYWN0aWNlJywgJ2NvbXBldGUnLCAnZ2V0IGhpcmVkJ10KCiMgQ29weSB0aGUgbGlzdCB1c2luZyBzbGljaW5nCmxpc3RfY29weSA9IG9yaWdpbmFsX2xpc3RbOl0KCiMgTW9kaWZ5IHRoZSBjb3BpZWQgbGlzdApsaXN0X2NvcHlbMF0gPSAnZXhwbG9yZScKCiMgUHJpbnQgYm90aCBsaXN0cwpwcmludCgiT3JpZ2luYWwgTGlzdDoiLCBvcmlnaW5hbF9saXN0KQpwcmludCgiQ29waWVkIExpc3Q6IiwgbGlzdF9jb3B5KQ==
Output:
Original List: ['learn', 'practice', 'compete', 'get hired']
Copied List: ['explore', 'practice', 'compete', 'get hired']
Code Explanation:
In the Python code example:
- We begin by creating a list, original_list, containing four string elements.
- Then, we use the slicing technique to create a new list with all elements from the original list. This results in a shallow copy.
- When we modify the first element of list_copy, it doesn’t affect original_list, as the outer lists are independent.
- However, if the list contains nested elements (e.g., a list within a list), changes to those nested objects will reflect in both lists due to shallow copy behavior.
Copying Python List Using Assignment Operator
We can use the assignment operator to copy a Python list, by direct assignment method. It is often mistakenly thought to create a new copy of a list but the new list it creates is a reference to the original list.
This means that both the original and the "copied" lists point to the same memory location, and changes made to one will directly affect the other. The example Python program below illustrates the same.
Code Example:
IyBPcmlnaW5hbCBsaXN0Cm9yaWdpbmFsX2xpc3QgPSBbJ2xlYXJuJywgJ3ByYWN0aWNlJywgJ2dldCBoaXJlZCddCgojIEFzc2lnbiB0aGUgb3JpZ2luYWwgbGlzdCB0byBhIG5ldyB2YXJpYWJsZQpsaXN0X2NvcHkgPSBvcmlnaW5hbF9saXN0CgojIE1vZGlmeSB0aGUgImNvcGllZCIgbGlzdApsaXN0X2NvcHlbMV0gPSAnZXhwbG9yZScKCiMgUHJpbnQgYm90aCBsaXN0cwpwcmludCgiT3JpZ2luYWwgTGlzdDoiLCBvcmlnaW5hbF9saXN0KQpwcmludCgiQ29waWVkIExpc3Q6IiwgbGlzdF9jb3B5KQ==
Output:
Original List: ['learn', 'explore', 'get hired']
Copied List: ['learn', 'explore', 'get hired']
Code Explanation:
In the example Python code:
- We begin with the original_list containing three string elements and then use the assignment operator to assign it to list_copy. Here, the list_copy is not a new list but a reference to the original_list.
- Then, we modify the second element of the copy list (index 1) and replace the string with the string value ‘explore’.
- Next, we print both lists to the console. It shows that changes are done to the second element of original_list as well since both point to the same memory location.
- This behavior highlights why the assignment operator is not suitable for creating an independent copy of a list.
Potential Pitfalls Of Using Python Copy List Methods
Copying lists in Python seems straightforward, but there are some nuances that can lead to unexpected behavior, particularly when dealing with mutable objects or nested structures. Understanding these potential pitfalls can save you from subtle bugs.
- Shallow Copy vs. Deep Copy: Most of the methods we've discussed, such as copy(), list(), and slicing ([:]), create shallow copies. While these methods duplicate the outer list, they only copy references to the elements within it. If the list contains:
- Immutable elements (e.g., integers, strings): Shallow copying works as expected, and changes to the copied list won't affect the original.
- Mutable elements (e.g., nested lists, dictionaries): Shallow copying can lead to shared references. Modifying a mutable element in one list will reflect in the other.
- Assignment Operator Doesn’t Copy: Using the assignment operator (=) doesn’t create a new list but a reference to the original. Changes to one list will directly affect the other, as both point to the same object in memory.
- Memory Considerations for Large Lists: When dealing with large lists, creating multiple copies can increase memory usage significantly. In such cases, you might want to consider using other data structures or sharing references intentionally to conserve memory.
- Unintended Side Effects with Nested Lists: When shallow copies are used on lists with nested structures, unintended side effects can occur.
For example, if your original list contains a nested list like [1, 2, [3, 4]] and you create a shallow copy, both the original and copied lists will share the same nested list. Modifying the nested list (e.g., changing 3 to 99) in one will automatically reflect in the other. This happens because the reference to the nested list is copied, not the actual object.
How To Avoid These Pitfalls
- Use copy.deepcopy(): For deep copies, which recursively duplicate the elements, use the deepcopy() method from the copy module. We will discuss this in a later section.
- Be Mindful of Nested Structures: When working with lists containing nested objects, carefully choose the appropriate copying method based on your requirements.
Check out this amazing course to become the best version of the Python programmer you can be.
Shallow Copy & Deep Copy In Python
When duplicating lists, the terms shallow copy and deep copy describe how the elements of the list are copied. Understanding these concepts helps determine the right method for your use case.
What Is A Shallow Copy?
A shallow copy duplicates the outer list, but it doesn’t recursively copy the objects inside it. Instead, it copies references to the inner elements. This means:
- If the inner elements are immutable (e.g., integers, strings), modifying the copy doesn’t affect the original.
- If the inner elements are mutable (e.g., nested lists), changes to those elements in the copy will reflect in the original.
Imagine a box with a list of fruits and another box nested inside with berries. A shallow copy duplicates the main box and adds a pointer to the same berries box. If you add a new berry, both boxes reflect the change.
What Is A Deep Copy?
A deep copy duplicates not just the outer list but also all objects inside it, recursively creating new objects for every mutable element. The original and copied lists become completely independent.
Using the same analogy, a deep copy would create a new main box and a completely new berries box inside it. Any changes to the berries in one box won’t affect the other.
Difference Between Shallow & Deep Copies In Python
Aspect |
Shallow Copy |
Deep Copy |
Copying Behavior |
Duplicates the outer object but shares references to inner elements. |
Recursively duplicates the entire object, including all nested elements. |
Independence |
Changes to mutable inner elements affect both the original and the copy. |
Changes to any part of the copy do not affect the original. |
Memory Usage |
Uses less memory since it doesn’t duplicate inner elements. |
Uses more memory as it duplicates all elements, even nested ones. |
Use Case |
Suitable for flat lists or when inner elements are immutable. |
Ideal for nested or complex structures requiring complete independence. |
Method |
Methods like copy(), list(), slicing ([:]), or copy.copy(). |
copy.deepcopy() from the copy module. |
When To Use Each Shallow & Deep Copy In Python
- Shallow Copy: Suitable for flat lists or lists with immutable elements where shared references won’t cause issues.
- Deep Copy: Necessary when working with complex nested structures where independence between copies is required.
Python copy() List Method From copy Module
The copy module of Python libraries provides two distinct methods: copy.copy() and copy.deepcopy(), for creating shallow and deep copies of objects (respectively). In this section, we will discuss these methods and how they work with examples.
The Python copy.copy() Method
The copy.copy() method creates a shallow copy of an object.
- It duplicates the outer object but maintains references to the elements inside.
- Changes to mutable inner elements in the copy will affect the original and vice versa.
For example, if you copy a basket of fruits containing a nested container of berries, copy.copy() duplicates the basket but points both baskets to the same berry container.
The Python copy.deepcopy() Method
The copy.deepcopy() method creates a deep copy of an object.
- It duplicates the outer object, as well as every mutable object inside it, recursively.
- Changes made to any part of the deep copy do not affect the original.
Using the same analogy, copy.deepcopy() duplicates the basket and creates a new, independent berry container. Any changes made to one basket don’t affect the other.
The sample Python program below illustrates how both of these methods work for creating copies of lists in Python, and the behavior of the copy lists.
Code Example:
aW1wb3J0IGNvcHkKCiMgT3JpZ2luYWwgbGlzdCB3aXRoIGEgbmVzdGVkIGxpc3QKb3JpZ2luYWxfbGlzdCA9IFsxLCAyLCBbMywgNF1dCgojIFNoYWxsb3cgY29weQpzaGFsbG93X2NvcHkgPSBjb3B5LmNvcHkob3JpZ2luYWxfbGlzdCkKCiMgRGVlcCBjb3B5CmRlZXBfY29weSA9IGNvcHkuZGVlcGNvcHkob3JpZ2luYWxfbGlzdCkKCiMgTW9kaWZ5IHRoZSBuZXN0ZWQgbGlzdCBpbiB0aGUgc2hhbGxvdyBjb3B5CnNoYWxsb3dfY29weVsyXVswXSA9IDk5CgojIE1vZGlmeSB0aGUgbmVzdGVkIGxpc3QgaW4gdGhlIGRlZXAgY29weQpkZWVwX2NvcHlbMl1bMV0gPSA4OAoKIyBQcmludCBhbGwgbGlzdHMKcHJpbnQoIk9yaWdpbmFsIExpc3Q6Iiwgb3JpZ2luYWxfbGlzdCkKcHJpbnQoIlNoYWxsb3cgQ29weToiLCBzaGFsbG93X2NvcHkpCnByaW50KCJEZWVwIENvcHk6IiwgZGVlcF9jb3B5KQ==
Output:
Original List: [1, 2, [99, 4]]
Shallow Copy: [1, 2, [99, 4]]
Deep Copy: [1, 2, [3, 88]]
Code Explanation:
In the sample Python code:
- We first import the copy module to use the copy() and deepcopy() methods.
- Then, we create a list, original_list, containing individual elements and a nested list.
- Next, we use the copy.copy() and copy.deepcopy() methods to create copy lists: shallow_copy and deep_copy respectively.
- We then modify elements of the copy lists to see how they impact the original_list.
- Shallow Copy: Modifying the nested list in shallow_copy changes the original_list because they share references to the same nested object.
- Deep Copy: Changes to deep_copy do not affect the original_list because deepcopy creates entirely independent copies of all elements.
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Advanced Techniques To Copy Python Lists
While the common methods we've covered are suitable for most cases, there are some advanced techniques that offer flexibility and can be especially useful for complex list duplication scenarios.
List Comprehensions To Copy Python Lists
List comprehensions provide a Pythonic and concise way to create copies of lists, particularly when you want to modify elements during the duplication process. You can use list comprehensions to not only duplicate but also apply transformations to the elements. For instance, copying the elements of a list while also squaring the elements.
Code Example:
b3JpZ2luYWxfbGlzdCA9IFsxLCAyLCAzLCA0XQoKI1VzaW5nIGxpc3QgY29tcHJlaGVuc2lvbiB0byBjb3B5IHRoZSBsaXN0IHdoaWxlIHNxdWFyaW5nIGl0cyBlbGVtZW50cwpzcXVhcmVkX2NvcHkgPSBbeCoqMiBmb3IgeCBpbiBvcmlnaW5hbF9saXN0XQoKcHJpbnQoc3F1YXJlZF9jb3B5KQ==
Output:
[1, 4, 9, 16]
Code Explanation:
In the Python program sample, we begin with a list of numbers, original_list. Then, we use list comprehension to create a new list where each element from the original list is squared. It's efficient and works well for simple transformations during duplication.
The append() Method To Copy Python Lists
If you need more control over how elements are added to a new list, you can use the append() method to manually build the duplicate list. This can be useful if you're constructing a more complex list structure.
You can iterate through the original list and use append() to add elements to the copy. This is slower than list comprehension but allows for complex operations within the loop.
Code Example:
b3JpZ2luYWxfbGlzdCA9IFsxLCAyLCAzLCA0XQpjb3B5X3dpdGhfYXBwZW5kID0gW10KCmZvciBpdGVtIGluIG9yaWdpbmFsX2xpc3Q6CiAgY29weV93aXRoX2FwcGVuZC5hcHBlbmQoaXRlbSkKCnByaW50KGNvcHlfd2l0aF9hcHBlbmQp
Output:
[1, 2, 3, 4]
Code Explanation:
In the Python code sample, we create a list of numbers called original_list and an empty list, copy_with_append. Then, we use a for loop to iterate through the original list and use the append() function in Python to create a copy list by appending each element individually to the new list. It’s useful when you need to process or modify elements as they are added.
The deque() Function To Copy Python Lists
The deque class from Python’s collections module offers an alternative approach for copying lists, especially when you’re working with large lists or need fast append and pop operations.
Using deque() to copy a list in Python can be advantageous when you need an efficient data structure that allows for fast insertion to lists and deletion from both ends.
Code Example:
ZnJvbSBjb2xsZWN0aW9ucyBpbXBvcnQgZGVxdWUKCm9yaWdpbmFsX2xpc3QgPSBbMSwgMiwgMywgNF0KZGVxdWVfY29weSA9IGRlcXVlKG9yaWdpbmFsX2xpc3QpCgpwcmludChkZXF1ZV9jb3B5KQ==
Output:
deque([1, 2, 3, 4])
Code Explanation:
The deque class efficiently creates a new list-like object. While it's not technically a list, it behaves like one with improved performance for certain operations (e.g., popping from both ends).
Common Use Cases Of Copying Python Lists
Creating copies of a list in Python is a common operation in many real-world scenarios. Here are some typical cases where creating a copy of a list is essential:
- Managing Independent Data Structures: When working with multiple versions of data, it's often necessary to create independent copies of a list to ensure that operations on one version don't affect others.
Example: Imagine you're working on a simulation where you maintain different configurations of a system. Each configuration is represented as a list. By duplicating the list for each configuration, you ensure that changes made to one configuration do not impact the others. - Undo and Redo Mechanisms: In applications that require undo and redo functionality (like text editors), lists often need to be duplicated to store the current state and restore previous states.
Example: In a to-do list app, whenever a task is added or removed, a duplicate of the list is saved before the change. This allows the user to undo or redo their actions by restoring the previous list state. - Iterating Through Modified Data Without Affecting Original List: Sometimes, while iterating through a list, modifications might be needed. To prevent altering the original data during iteration, it’s crucial to work with a copy.
Example: You have a list of students who completed assignments. As you grade them, you might want to track which students have been graded. By duplicating the list before grading, you can safely update the copy without changing the original student list. - Avoiding Side Effects in Functions: When passing lists to functions, copying the list beforehand can avoid unwanted side effects, especially when the function modifies the list in place.
Example: Consider a function that manipulates a list of numbers by appending values to it. If you pass the original list directly, the changes made by the function will persist outside the function. Creating a copy ensures that the original list remains unchanged. - Maintaining Backup Versions of Lists: Duplication can also be used to maintain backup copies of data, especially when working with critical information or in scenarios where data integrity is crucial.
Example: In a financial application that tracks transactions, you might want to store a backup copy of the transaction list before applying any updates. This allows you to revert to the backup in case an error occurs during processing.
Conclusion
Creating a copy of Python lists is an essential technique that enables developers to work with independent copies of lists without unintentionally modifying the original data. Whether you're dealing with shallow or deep copies, it's important to understand the different methods and when to use each one based on your specific needs.
From simple techniques like the Python copy() list method and list slicing to advanced options like copy.deepcopy() and deque(), the programming language offers a range of tools for duplicating lists. Each method has its unique use cases, and selecting the right one can lead to more efficient, error-free code.
Ready to upskill your Python knowledge? Dive deeper into actual problem statements and elevate your coding game with hands-on practice!
Frequently Asked Questions
Q1. How do you copy a list without references in Python?
To copy a list without references to the original, you need to create a deep copy. A deep copy ensures that all elements, including nested structures, are fully independent of the original list.
- Shallow Copy: It (e.g., using copy() or slicing) creates a new outer list, but nested elements still reference the same objects in memory. This means changes to nested objects will affect both the original and the copied list.
- Deep Copy: It is created using copy.deepcopy(), duplicates not only the outer list but also all nested objects within it, ensuring no shared references between the original and copied list.
Q2. What does copy() do in Python?
The copy() method in Python creates a shallow copy of a list. It duplicates the outer structure of the list but does not create copies of nested elements within the list. Changes to the nested objects in the copied list will also affect the original list, as they reference the same objects in memory.
Q3. What is the difference between list() and copy() in Python?
Both list() and copy() methods create a shallow copy, but the key difference is:
- list(): Creates a new list from the original, which works similarly to a shallow copy in most cases. It's a constructor that can be used to duplicate a list.
- copy(): This method is specifically designed to create a shallow copy of a list. While list() can be used for copying, copy() is the more explicit choice for copying.
In both cases, modifications to mutable elements (such as nested lists) will affect both the original and the copied list.
Q4. What is clone() in Python?
Python does not have a built-in clone() method for lists. However, the concept of cloning a list is similar to copying a list. The methods like copy(), list(), and copy.deepcopy(), can be used to clone or duplicate a list. Some external libraries or Python frameworks may implement a clone() method for specific objects, but in Python’s standard library, it’s not a native method.
Q5. How do you copy a nested list in Python?
To copy a nested list (a list containing other lists or complex objects), you need to use a deep copy. A shallow copy will not duplicate the nested lists or objects inside the main list, meaning that modifications to those nested elements will reflect in both the original and copied lists. To ensure complete independence, use copy.deepcopy().
Q6. Can I copy a list using the assignment operator (=)?
No, using the assignment operator (=) only creates a reference to the original list, not a copy. Any changes made to the copied list will also affect the original list, as both variables point to the same object in memory. To create an actual copy, you need to use one of the copy methods, such as copy(), list(), or deepcopy().
Q7. Can I copy a list using the append() method?
While the append() method itself is not used for copying lists, it can be used as part of a custom solution where you manually copy elements from one list to another. You would iterate through the original list and append each element to the new list, effectively creating a copy of Python lists.
Do check the following out:
- Python Linked Lists | A Comprehensive Guide (With Code Examples)
- Find Length Of List In Python | 8 Ways (+Examples) & Analysis
- Python input() Function (+Input Casting & Handling With Examples)
- Python Reverse List | 10 Ways & Complexity Analysis (+Examples)
- Fix Indexerror (List Index Out Of Range) In Python +Code Examples
- Remove Item From Python List | 5 Ways & Comparison (+Code Examples)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment