- What Is A Python List?
- What Is A Nested List In Python & How Do They Work?
- How To Create & Initialize A Nested List In Python?
- Add/ Insert Elements To Nested Lists In Python (Step-by-Step Methods)
- Modifying Elements Of Nested List In Python
- Removing Elements From Nested List In Python
- Flattening Nested Lists In Python
- Exploring Negative Indexing In Nested List In Python
- Real Use Cases Of Nested List In Python
- Pitfalls Of Working With Nested Lists In Python
- Advantages & Disadvantages Of Nested List In Python
- Conclusion
- Frequently Asked Questions
Nested List In Python | Initialize, Manipulate & Flatten (+Examples)
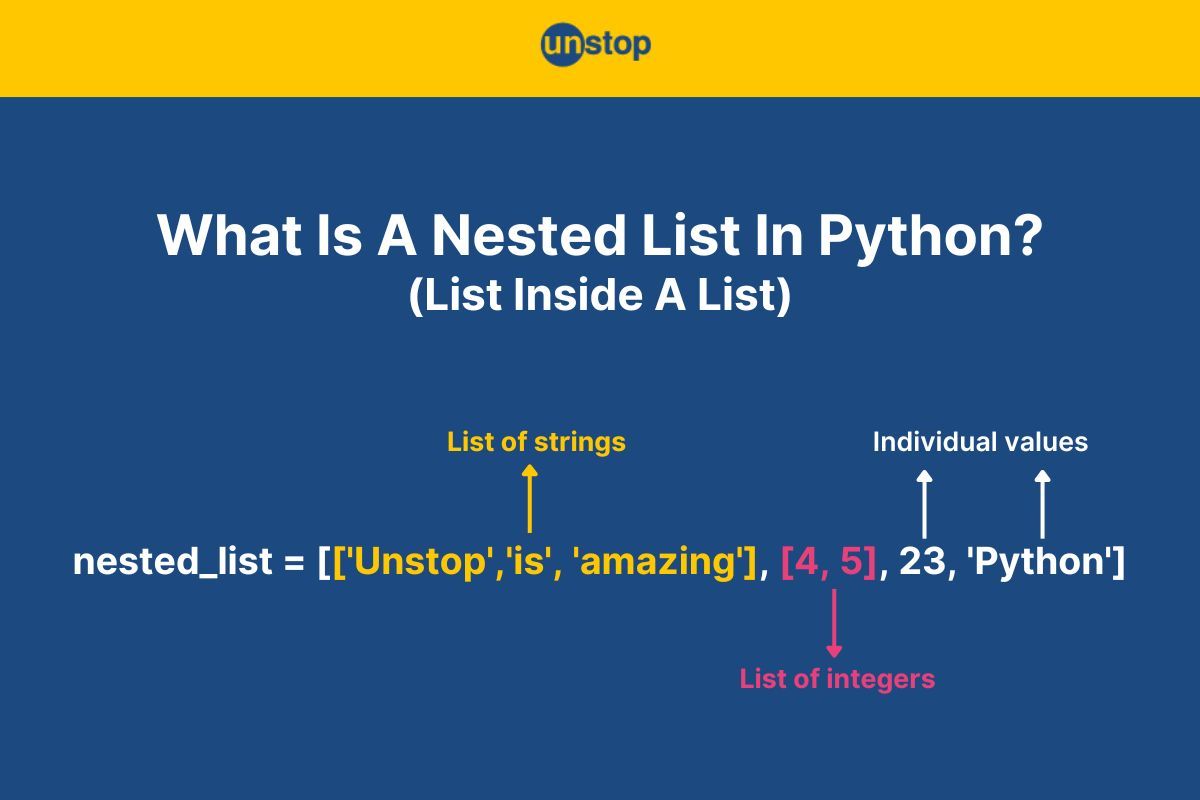
A list in Python is like a versatile container that can hold multiple items, including numbers, strings, or even other lists. When a list contains other lists as its elements, it becomes a nested list. But why would we need such complexity?
Well, nested lists in Python are incredibly useful for representing structured data like matrices, tables, or hierarchical information.
In this article, we will explore the concept of nested list in Python, including the process of its creation, modification, practical use cases, and more. By the end, you’ll have a clear understanding of how to use nested lists effectively in your Python projects.
What Is A Python List?
A list in Python programming is an ordered collection that can store multiple data types, allowing you to organize data flexibly. Lists are mutable, meaning you can add, remove, or modify elements.
Here’s a simple example of a Python list:
# Example of a simple Python list
programming_languages = ['Python', 'Java', 'C++', 'JavaScript']
print(programming_languages)
Output:
['Python', 'Java', 'C++', 'JavaScript']
In this example, programming_languages is a list that holds string elements. You can easily access, modify, or iterate through its elements.
If you’re new to Python lists, check out our Comprehensive Guide to Python Lists for more details.
What Is A Nested List In Python & How Do They Work?
A nested list is simply a list within a list. It's like a storage box that not only holds items but also contains smaller boxes within it, each potentially holding its own set of items. This structure makes nested lists in Python perfect for representing multidimensional data or hierarchical relationships, such as matrices, tables, or trees.
In Python language, nested lists can be used to create lists of lists, allowing for complex data organization. Each sublist in the main list can be accessed using two levels of indices: the first for the main list and the second for the elements within the sublist. The simple Python program example below illustrates the concept of a nested list for better understanding.
Code Example:
# Example of a nested list (list of lists)
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
# Accessing elements
print(matrix[0][2]) # First row, third element
Output:
3
Code Explanation:
In this simple Python code example, we create a nested list called matrix, which contains three sublists, each containing 3 elements.
- In other words, it forms a 3x3 matrix with three rows and columns.
- Then, we use the index expression– matrix[0][2] to access the third element in the first row, which is 3.
- We print this to the console.
Nested lists allow you to model data structures with multiple levels of detail, making them highly versatile for tasks like data analysis, simulations, and more.
How To Create & Initialize A Nested List In Python?
Creating and initializing a nested list in Python is straightforward, and there are multiple methods to suit various needs. In this section, we’ll explore some common techniques, starting with list comprehension and the range() method, followed by other advanced approaches.
Initialize Nested List In Python Using List Comprehension & range() Method
List comprehension is a concise way to create lists in Python, including nested lists. It allows you to generate a list of lists in one line of code. This is especially useful when you need to initialize a list based on a specific pattern, like generating rows of numbers.
The basic Python program example below illustrates how to initialize a nested list in Python using list comprehension with the range() method in a single line.
Code Example:
# Using list comprehension to create a 3x3 matrix
matrix = [[i for i in range(1, 4)] for j in range(3)]
print(matrix)
Output:
[[1, 2, 3], [1, 2, 3], [1, 2, 3]]
Code Explanation:
In the basic Python code example,
- We begin by creating a nested list named matrix and initialize it using list comprehension.
- Inside the list comprehension, we use nested for loops to generate its elements.
- The inner for loop generates a list containing three elements from the range (1,4), i.e., the elements can be 1, 2, and 3.
- The outer for loop stipulates that the process of the inner loop be repeated three times, thus generating three rows.
- This generates a 3x3 matrix where each sublist contains the numbers 1, 2, and 3.
- Then, we use the print() function to display the nested list to the console.
This approach is elegant and efficient, making it ideal for generating nested lists with predictable patterns or structures.
Initialize Nested List In Python Using For Loop
A more traditional way to initialize a nested list is by using a for loop. This method is especially useful if you want more control over the initialization process or need to add specific logic.
Code Example:
#Declaring an empty list
matrix = []
# Using a for loop to initialize a nested list
for i in range(3):
row = [j for j in range(1, 4)] # Create each row with numbers 1 to 3
matrix.append(row)
print(matrix)
Output:
[[1, 2, 3], [1, 2, 3], [1, 2, 3]]
Code Explanation:
In the Python program example,
- We begin by creating an empty list matrix.
- Then, we use a for loop to iterate through the list three times, referring to the number of rows.
- Inside the loop, in every iteration, we use list comprehension to generate a row containing the numbers 1, 2, and 3.
- Then, we use the append() method to add this row to the matrix.
- This creates a 3x3 matrix, which we then printed to the console.
Initialize Nested List In Python With Numpy
If you’re working with large datasets or need advanced functionality (like matrix operations), the numpy Python library provides an efficient way to create nested lists (also known as arrays).
Code Example:
#Importing the library
import numpy as np
# Using numpy to create a 3x3 matrix
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
print(matrix)
Output:
[[1 2 3]
[4 5 6]
[7 8 9]]
Code Explanation:
In the Python code example,
- We first import the Python library numpy, as np; that is, we can use np instead of the full name when using its content.
- Then, we create a nested list matrix and initialise its elements using the array() function from the numpy module, with the elements and sublists provided as arguments.
- The np.array() function creates a 3x3 matrix by converting a nested list into a numpy array.
- Unlike standard Python lists, numpy arrays are optimized for numerical computations, offering better performance and additional capabilities like element-wise operations and reshaping.
This makes numpy a preferred choice for large-scale data processing and matrix manipulation.
Add/ Insert Elements To Nested Lists In Python (Step-by-Step Methods)
When working with nested lists, you might need to add new elements or sublists. Python offers several ways to accomplish this. In this section, we will discuss the three most common ways of adding elements to nested list using Python’s built-in functions: append(), insert(), and extend().
Using append() To Add Element To Nested List In Python
The append() method adds an element to the end of the list. For a nested list in Python, it will add a new sublist (which is the element here) as a new row or item at the end of the main list.
Code Example:
# Creating a 3x3 matrix
matrix = [[1, 2, 3], [4, 5, 6]]
# Adding a new row using append()
matrix.append([7, 8, 9])
print(matrix)
Output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Code Explanation:
In the example Python program,
- We have a nested list matrix with two elements, i.e., sublists containing three elements.
- Then, we use the append() method to add a new sublist [7, 8, 9] at the end of the matrix.
- This modifies the original matri,x which we print to the console.
Using insert() To Add Element To Nested List In Python
The insert() method allows you to add an element at a specific position in the list. It takes two arguments: the index where the element should be inserted and the element itself.
Code Example:
# Creating a 3x3 matrix
matrix = [[1, 2, 3], [4, 5, 6]]
# Inserting a new row at index 1
matrix.insert(1, [7, 8, 9])
print(matrix)
Output:
[[1, 2, 3], [7, 8, 9], [4, 5, 6]]
Code Explanation:
In the example Python code,
- We begin with a nested list matrix containing two elements/ sublists.
- Then, we use the insert() method to add/ insert the sublist [7, 8, 9] at the second position (index 1) of the matrix.
- The function inserts the list and shifts all subsequent elements one position forward.
Using extend() To Add Element To Nested List In Python
The extend() method adds all elements from another iterable (like a list) to the end of the list. For nested lists, this method can add multiple rows at once.
Code Example:
# Creating a 3x3 matrix
matrix = [[1, 2, 3], [4, 5, 6]]
# Extending the matrix with another list of rows
matrix.extend([[7, 8, 9], [10, 11, 12]])
print(matrix)
Output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]]
Code Explanation:
In the sample Python program,
- We begin with the same list as before and use the extend() method to add two new rows ([7, 8, 9] and [10, 11, 12]) to the matrix.
- This is different from append() because it adds multiple rows at once.
These methods provide flexible ways to modify your nested lists. Depending on your use case, you can choose the appropriate method for adding elements to the list.
Check out this amazing course to become the best version of the Python programmer you can be.
Modifying Elements Of Nested List In Python
Once you have a nested list, you may need to modify its individual elements. Python allows you to access and change these elements using indexing. In this section, we will cover how to modify elements in nested lists, including both single elements and entire rows.
Modifying a Single Element (Direct Assignment) Of Nested List In Python
You can modify a single element in a nested list by specifying its row and column index using the direct assignment method. This is the simplest and most direct way to change an element.
Code Example:
# Creating a 3x3 matrix
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Modifying the element in the second row, third column
matrix[1][2] = 10
print(matrix)
Output:
[[1, 2, 3], [4, 5, 10], [7, 8, 9]]
Code Explanation:
- We create a nested list matrix with three sublists (3 elements each).
- Then, we use the direct assignment method to modify the element at position [1][2] (second row, third column) from 6 to 10.
- This updates the value of the element without affecting the rest of the list.
Modifying An Entire Row Of Nested List In Python
You can also modify an entire row in a nested list in Python using the direct assignment method. All you have to do is access the row using its index and assign a new list to replace that with.
Code Example:
# Creating a 3x3 matrix
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Modifying the second row
matrix[1] = [11, 12, 13]
print(matrix)
Output:
[[1, 2, 3], [11, 12, 13], [7, 8, 9]]
Code Explanation:
In the Python code sample,
- We have the nested list matrix with three sublists, and then we use the direct assignment method to modify the second element.
- This replaces the row at index 1 ([4, 5, 6]) with the new row [11, 12, 13].
- If the index is out of range/ bounds, it will throw an error.
This method can be used to change a whole row in the matrix at once.
Modifying Nested List In Python Using Loops
For more complex modifications, you can use loops to iterate over the elements and modify them as needed. This can be particularly useful when applying a transformation to the elements.
Code Example:
# Creating a 3x3 matrix
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Doubling each element in the matrix
for row in matrix:
for i in range(len(row)):
row[i] *= 2
print(matrix)
Output:
[[2, 4, 6], [8, 10, 12], [14, 16, 18]]
Code Explanation:
In the Python program sample,
- We have a nested list matrix with 3 sublists (rows) containing 3 elements each.
- Then, we use a set of nested for loops where:
- The outer loop iterates through each row in the matrix.
- The inner loop iterates each element within the row, using the len() function to set the range for the sublist.
- In every iteration of the inner loop, we multiply the element by 2.
- After the loops are done iterating, they effectively double all elements in the matrix.
Removing Elements From Nested List In Python
Removing elements from nested lists works similarly to regular lists, with the added consideration that you’ll often be targeting elements within sublists.
- If you want to remove entire sublists, you can use the index position of that list alone.
- But if you want to remove elements from within a sublist, then you will have to use two index values, one for the position of the sublist and one for the position of the element inside the sublist.
So, the key difference is understanding how these methods apply to the structure of nested lists, where elements could be individual items or entire sublists.
Common Methods To Remove Elements From Nested List In Python
The common method to remove elements from Python nested lists are:
- remove(): Removes the first occurrence of a value from the list (or sublist).
- pop(): Removes an element at a specified index and returns it.
- del: Deletes a row or element from a list based on its index.
- clear(): Clears all elements from a sublist.
Example Of Using pop() To Remove Element From Python Nested List
The pop() method is often used to remove an element at a specific index, making it useful when dealing with nested lists, especially when removing a specific row or item.
Code Example:
# Example: Removing a row from a nested list using pop
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
removed_row = matrix.pop(1) # Removes the second row
print("Updated Matrix:", matrix)
print("Removed Row:", removed_row)
# Example: Removing a single element from a sublist using pop
removed_element = matrix[0].pop(2) # Removes element '3' from the first sublist
print("Updated Matrix after removing an element from sublist:", matrix)
print("Removed Element:", removed_element)
Output:
Updated Matrix: [[1, 2, 3], [7, 8, 9]]
Removed Row: [4, 5, 6]
Updated Matrix after removing an element from sublist: [[1, 2], [7, 8, 9]]
Removed Element: 3
Code Explanation:
In the Python code, we use the pop() method in two different ways:
- First, we remove the second row of the matrix by specifying its index (1). The method removes and returns the entire row.
- Next, we remove a single element from a sublist (the number 3 from the first sublist). By accessing the sublist at index 0 and calling the function pop() with the index of the element to remove, we get rid of that specific item from the sublist. After both operations, the updated matrix reflects the changes.
For more information on other methods to remove elements, read: Remove Item From Python List | 5 Ways & Comparison (+Code Examples)
Flattening Nested Lists In Python
Flattening a nested list in Python involves converting a multi-level list (a list of lists) into a single-level list, where all elements are placed in a linear sequence. This is especially useful when you need to simplify a structure for processing, searching, or manipulation.
In Python, there are multiple ways to flatten a nested list, depending on the complexity of the structure and the specific needs of your program.
Common Methods To Flatten A Nested List In Python
- Using a for loop
- Using list comprehension
- Using itertools.chain()
- Using a recursive function
Example: Flattening A Nested List In Python Using List Comprehension
List comprehension is a compact and efficient way to flatten a nested list. By iterating over each sublist and extracting its elements, we can create a single, flat list.
Code Example:
# Example: Flattening a nested list using list comprehension
nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]]
flattened_list = [item for sublist in nested_list for item in sublist]
print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8]
Code Explanation:
In this example, we use a nested list comprehension to flatten the nested list as follows:
- The outer loop iterates over each sublist within the nested list.
- The inner loop iterates over each element in the current sublist.
- The result is a single-level list containing all elements of the nested list, now flattened into one sequence.
Example: Flattening A Nested List In Python Using itertools.chain()
The itertools.chain() function is an efficient way to flatten a list. It works by chaining multiple iterables together, effectively flattening them into a single iterable.
Code Example:
import itertools
# Example: Flattening a nested list using itertools.chain()
nested_list = [[1, 2, 3], [4, 5], [6, 7, 8]]
flattened_list = list(itertools.chain(*nested_list))
print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8]
Code Explanation:
In the Python example, we use the itertools.chain() function from the itertools module to flatten the nested list.
- We begin with a nested list named nested_list containing 3 sublists/ elements.
- Then, we call the itertools.chain() function on nested_list to flatten it.
- Here, the chain() function takes multiple iterables as arguments and concatenates them into a single iterable.
- By using the *nested_list syntax, we unpack the nested list.
- This means that instead of passing the entire nested list as a single argument, we pass each sublist (e.g., [1, 2, 3], [4, 5], and [6, 7, 8]) as individual arguments to the chain() function.
- The asterisk symbol (*) here acts as a pointer that unpacks the list, essentially converting the list of lists into separate arguments.
- As chain() processes each sublist, it returns the elements one by one, combining them into a single iterable sequence.
- We then convert this iterable into a list and wrap the result using the list() method.
- The final result is a single flattened list: [1, 2, 3, 4, 5, 6, 7, 8].
This method is particularly efficient for flattening nested lists since itertools.chain() is optimized for handling large datasets without creating unnecessary intermediate lists, making it a good option for performance-sensitive operations.
Level up your coding skills with the 100-Day Coding Sprint at Unstop and get the bragging rights, now!
Example: Flattening A Nested List In Python Using Recursive Function
If the nested lists are of unknown depth (i.e., lists within lists within lists), a recursive function is a great solution for flattening them completely.
Code Example:
# Example: Flattening a nested list using a recursive function
def flatten(nested_list):
flattened = []
for item in nested_list:
if isinstance(item, list):
flattened.extend(flatten(item)) # Recursively flatten sublists
else:
flattened.append(item) # Append non-list items
return flattened
# Testing the recursive flatten function
nested_list = [1, [2, [3, 4], 5], 6, [7, 8]]
flattened_list = flatten(nested_list)
print(flattened_list)
Output:
[1, 2, 3, 4, 5, 6, 7, 8]
Code Explanation:
In the example code,
- We define a function flatten() to recursively traverse a nested list.
- Inside flatten(), we initialize an empty list called flattened to store the result.
- We then use a for loop to iterate over each item in nested_list.
- Inside the loop, for each item, the if-else statement checks if the item is a list using the isinstance() function.
- If the item is a list, the function calls flatten() recursively to flatten that sublist. The resulting elements are added to flattened using extend(), which appends the elements of the flattened sublist to the main list.
- If the item is not a list, it is directly appended to flattened using append().
- This method works for arbitrarily deep nested lists, providing a fully flattened result regardless of the depth of nesting.
- Finally, we test the function by passing a nested_list containing individual elements, sublists, and nested sublists.
- The function returns a flattened list, which is stored in flattened_list and printed.
Exploring Negative Indexing In Nested List In Python
In Python, negative indexing allows you to access elements from the end of a list, which can be extremely handy when dealing with nested lists.
- Instead of starting from the first element, you can use negative indices to access elements from the last to the first.
- This can be particularly useful when you're not sure about the length of the list or want to quickly reference items from the end.
- When applied to nested lists, negative indexing works in the same way as with regular lists but with an additional layer of depth.
It allows you to access sublists or individual elements within those sublists from the end without needing to know the exact length of the list. The example below illustrates how to use negative indexes with a nested list in Python.
Code Example:
# Example: Accessing elements with negative indexing in a nested list
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Accessing the last sublist
last_sublist = nested_list[-1]
# Accessing the last element of the last sublist
last_element = nested_list[-1][-1]
print("Last Sublist:", last_sublist)
print("Last Element of Last Sublist:", last_element)
Output:
Last Sublist: [7, 8, 9]
Last Element of Last Sublist: 9
Code Explanation:
In this Python example:
- We begin with a nested list named nested_list with three sublists.
- Then, we use a single negative index -1 to access the last sublist of the nested_list (i.e., [7, 8, 9]).
- We then use a double negative index [-1][-1] to access the last element of the last sublist (i.e., 9).
- This shows how negative indexing can be used in nested lists to directly reference elements starting from the end of the list or sublists.
Negative indexing simplifies navigation, especially when working with lists of unknown or dynamic lengths, making it a useful feature when manipulating nested structures.
Real Use Cases Of Nested List In Python
Nested lists are incredibly versatile, making them suitable for representing complex data structures.
Use Case 1: Representing Table Of Data & Searching For Information
A common scenario involves storing student data, where each student is represented by a nested list containing their ID and name. Sometimes, you may need to search for a particular student's name or ID within this dataset.
Code Example:
# Nested list representing table of data/ students information with their ID and name
students = [
[101, "Akaash"],
[102, "Aroohi"],
[103, "Shaisha"],
[104, "Aroohi"]
]
# Searching for occurrences of the name 'Alice'
search_name = "Aroohi"
found_indices = [
(index, sublist.index(search_name))
for index, sublist in enumerate(students) if search_name in sublist
]
print("Occurrences of 'Aroohi':", found_indices)
Output:
Occurrences of 'Aroohi': [(0, 1), (3, 1)]
Code Explanation:
In this example,
- We represent student data in the students nested list, where each sublist contains a student's ID and name.
- In other words, each row in the students list is a sublist containing a student's details.
- Then, we use list comprehension to search for occurrences of the name "Aroohi".
- It checks each sublist to see if the name appears, and if so, it records the index of the sublist and the index of the name within that sublist.
- The result is a list of tuples indicating the positions of "Aroohi" in the main list and within each sublist.
This approach is incredibly useful when dealing with large datasets where you're tasked with finding a specific piece of information (like a name or ID) within a collection. Searching for occurrences in nested lists can help streamline data retrieval and analysis.
Use Case 2: Storing Matrix Data
A common use case of a nested list in Python is representing matrices or 2D arrays. Nested lists are an ideal data structure for this, and you can easily manipulate or search for values within a matrix using the same techniques. They are often used in mathematical computations or game development.
Code Example:
# Representing a 3x3 matrix
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
# Accessing the element at row 2, column 3
print(matrix[1][2])
Output:
6
Code Explanation:
Here, a 3x3 matrix is represented as a nested list. We access the element at the second row and third column using double indexing: matrix[1][2]. This is ideal for matrix operations or tabular data.
Use Case 3: Representing Graphs And Trees
Nested lists are also used to represent graphs and trees, where each sublist can represent a node or a relationship. Searching within these structures can help with graph traversal or finding specific nodes.
Code Example:
# Adjacency list representation of a graph
graph = [
[1, 2], # Node 0 connected to Nodes 1, 2
[0, 3], # Node 1 connected to Nodes 0, 3
[0, 3], # Node 2 connected to Nodes 0, 3
[1, 2, 4], # Node 3 connected to Nodes 1, 2, 4
[3] # Node 4 connected to Node 3
]
# Finding all nodes connected to Node 3
connections = graph[3]
print(connections)
Output:
[1, 2, 4]
Code Explanation:
The graph list uses an adjacency list format, where each sublist represents the nodes connected to a specific node. Accessing graph[3] returns all nodes connected to Node 3.
Pitfalls Of Working With Nested Lists In Python
Nested lists are powerful tools for organizing complex data, but they come with a few challenges. Understanding these pitfalls can help you avoid common mistakes and improve the efficiency of your code.
- Indexing Errors: A common mistake when working with nested lists is incorrect indexing. Since nested lists can have varying depths, it's easy to confuse the indices and access elements that don’t exist. Ensuring that you know the structure of the list and the depth of the sublists is key to preventing these errors.
- Modifying Elements in Nested Lists: Since lists in Python are mutable, modifying one list can unintentionally affect other lists if they are references to the same object. This can lead to unexpected side effects, especially if you're working with copies of lists or passing lists to functions. To avoid this, ensure that you're using proper list copying techniques, such as copy() or deepcopy().
- Performance Issues with Deep Nesting: Deeply nested lists can result in performance degradation. As the nesting depth increases, operations such as searching, iterating, or modifying the elements become slower. This is particularly noticeable with large datasets. In such cases, alternative data structures like NumPy arrays or pandas DataFrames can be more efficient.
- Handling Mixed Data Types: Nested lists can sometimes contain a mix of data types (e.g., integers, strings, floats). This can make operations on the list more difficult and error-prone, especially if your code expects elements of a certain type. Ensuring consistency in data types is crucial when working with nested lists to avoid errors.
- Memory Consumption: Nested lists, especially those with multiple layers, can consume significant memory. Each sublist is a separate object, leading to increased memory usage when working with large or deeply nested lists. This can impact performance, especially in resource-constrained environments. Being mindful of the memory footprint of your nested lists is important to optimize performance.
Advantages & Disadvantages Of Nested List In Python
Nested lists offer several advantages, including flexibility in data storage and the ability to represent real-world structures. However, they also come with challenges, such as increased complexity and potential performance overhead.
Advantages |
Disadvantages |
|
|
Looking for guidance? Find the perfect mentor from select experienced coding & software development experts here.
Conclusion
The nested list in Python is a powerful tool for managing complex data, allowing you to create and manipulate multi-dimensional structures like matrices, tables, and hierarchical data.
- There are three common ways of initializing them: for loops, list comprehension with range(), or libraries like NumPy.
- Python provides multiple built-in methods to perform various operations on nested lists.
- For example, the insert(), append() and extend() method to add/ insert elements, direct assignment to modify elements, remove(), pop(), del, and clear() to remove elements.
- We also flatten a nested list in Python using nested list comprehension, itertools.chain(), and recursion.
- Some real-world use cases of these lists include managing student records, handling matrices, and building tree structures.
- While nested lists provide great flexibility, it's important to be mindful of performance when working with deep or large datasets.
By mastering these techniques, you can leverage nested lists in your Python projects for efficient and organized data management.
Frequently Asked Questions
Q1. What is the difference between a list and a nested list in Python?
A regular list contains elements like integers, strings, etc., while a nested list contains other lists as its elements, allowing the storage of more complex data structures like matrices or trees.
Q2. How can I access an element in a nested list?
You can access elements by chaining indices. For example, nested_list[0][1] accesses the second element in the first sublist.
Q3. Can nested lists be of different lengths?
Yes, nested lists in Python can have sublists of different lengths. Each sublist is independent, so their lengths do not need to be the same.
Q4. Are nested lists more memory-intensive than regular lists?
Yes, nested lists can be more memory-intensive because each sublist is a separate list object, and the references to those sublists take up additional space.
Q5. Can I flatten a deeply nested list in Python?
Yes, you can flatten a deeply nested list using recursion or utilities like itertools.chain() for simpler cases.
Q6. What are some real-world applications of nested lists?
Nested lists are often used in scenarios involving multi-dimensional data, such as matrices, tables, and representing graphs or trees.
Q7. What are the performance considerations when using nested lists in Python?
Nested lists can lead to performance issues, especially with large datasets. Accessing elements within deeply nested structures can be slower due to multiple layers of indexing. Additionally, operations like flattening or modifying elements may involve significant computational overhead, depending on the depth and size of the list. It's important to be mindful of memory usage and consider alternative data structures (like NumPy arrays or Pandas DataFrames) for large-scale data manipulation.
By now, you must know all about the nested list in Python. Do explore other important concepts:
- Python Reverse List | 10 Ways & Complexity Analysis (+Examples)
- Remove Duplicates From Python List | 12 Ways With Code Examples
- How To Convert Python List To String? 8 Ways Explained (+Examples)
- Python Assert Keyword | Types, Uses, Best Practices (+Code Examples)
- Python max() Function With Objects & Iterables (+Code Examples)
- Fibonacci Series In Python & Nth Term | Generate & Print (+Codes)
An economics graduate with a passion for storytelling, I thrive on crafting content that blends creativity with technical insight. At Unstop, I create in-depth, SEO-driven content that simplifies complex tech topics and covers a wide array of subjects, all designed to inform, engage, and inspire our readers. My goal is to empower others to truly #BeUnstoppable through content that resonates. When I’m not writing, you’ll find me immersed in art, food, or lost in a good book—constantly drawing inspiration from the world around me.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment