Python Programming
Table of content:
- What Is Python? An Introduction
- What Is The History Of Python?
- Key Features Of The Python Programming Language
- Who Uses Python?
- Basic Characteristics Of Python Programming Syntax
- Why Should You Learn Python?
- Applications Of Python Language
- Advantages And Disadvantages Of Python
- Some Useful Python Tips & Tricks For Efficient Programming
- Python 2 Vs. Python 3: Which Should You Learn?
- Python Libraries
- Conclusion
- Frequently Asked Questions
- It's Python Basics Quiz Time!
Table of content:
- Python At A Glance
- Key Features of Python Programming
- Applications of Python
- Bonus: Interesting features of different programming languages
- Summing up...
- FAQs regarding Python
- Take A Quiz To Rehash Python's Features!
Table of content:
- What Is Python IDLE?
- What Is Python Shell & Its Uses?
- Primary Features Of Python IDLE
- How To Use Python IDLE Shell? Setting Up Your Python Environment
- How To Work With Files In Python IDLE?
- How To Execute A File In Python IDLE?
- Improving Workflow In Python IDLE Software
- Debugging In Python IDLE
- Customizing Python IDLE
- Code Examples
- Conclusion
- Frequently Asked Questions (FAQs)
- How Well Do You Know IDLE? Take A Quiz!
Table of content:
- What Is A Variable In Python?
- Creating And Declaring Python Variables
- Rules For Naming Python Variables
- How To Print Python Variables?
- How To Delete A Python Variable?
- Various Methods Of Variables Assignment In Python
- Python Variable Types
- Python Variable Scope
- Concatenating Python Variables
- Object Identity & Object References Of Python Variables
- Reserved Words/ Keywords & Python Variable Names
- Conclusion
- Frequently Asked Questions
- Rehash Python Variables Basics With A Quiz!
Table of content:
- What Is A String In Python?
- Creating String In Python
- How To Create Multiline Python Strings?
- Reassigning Python Strings
- Accessing Characters Of Python Strings
- How To Update Or Delete A Python String?
- Reversing A Python String
- Formatting Python Strings
- Concatenation & Comparison Of Python Strings
- Python String Operators
- Python String Functions
- Escape Sequences In Python Strings
- Conclusion
- Frequently Asked Questions
- Rehash Python Strings Basics With A Quiz!
Table of content:
- What Is Python Namespace?
- Lifetime Of Python Namespace
- Types Of Python Namespace
- The Built-In Namespace In Python
- The Global Namespace In Python
- The Local Namespace In Python
- The Enclosing Namespace In Python
- Variable Scope & Namespace In Python
- Python Namespace Dictionaries
- Changing Variables Out Of Their Scope & Python Namespace
- Best Practices Of Python Namespace
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Namespaces!
Table of content:
- What Are Logical Operators In Python?
- The AND Python Logical Operator
- The OR Python Logical Operator
- The NOT Python Logical Operator
- Short-Circuiting Evaluation Of Python Logical Operators
- Precedence of Logical Operators In Python
- How Does Python Calculate Truth Value?
- Final Note On How AND & OR Python Logical Operators Work
- Conclusion
- Frequently Asked Questions
- Python Logical Operators Quiz– Test Your Knowledge!
Table of content:
- What Are Bitwise Operators In Python?
- List Of Python Bitwise Operators
- AND Python Bitwise Operator
- OR Python Bitwise Operator
- NOT Python Bitwise Operator
- XOR Python Bitwise Operator
- Right Shift Python Bitwise Operator
- Left Shift Python Bitwise Operator
- Python Bitwise Operations And Negative Integers
- The Binary Number System
- Application of Python Bitwise Operators
- Python Bitwise Operator Overloading
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python Bitwise Operators!
Table of content:
- What Is The Print() Function In Python?
- How Does The print() Function Work In Python?
- How To Print Single & Multi-line Strings In Python?
- How To Print Built-in Data Types In Python?
- Print() Function In Python For Values Stored In Variables
- Print() Function In Python With sep Parameter
- Print() Function In Python With end Parameter
- Print() Function In Python With flush Parameter
- Print() Function In Python With file Parameter
- How To Remove Newline From print() Function In Python?
- Use Cases Of The print() Function In Python
- Understanding Print Statement In Python 2 Vs. Python 3
- Conclusion
- Frequently Asked Questions
- Know The print() Function In Python? Take A Quiz!
Table of content:
- Working Of Normal Print() Function
- The New Line Character In Python
- How To Print Without Newline In Python | Using The End Parameter
- How To Print Without Newline In Python 2.x? | Using Comma Operator
- How To Print Without Newline In Python 3.x?
- How To Print Without Newline In Python With Module Sys
- The Star Pattern(*) | How To Print Without Newline & Space In Python
- How To Print A List Without Newline In Python?
- How To Remove New Lines In Python?
- Conclusion
- Frequently Asked Questions
- Think You Can Print Without a Newline in Python? Prove It!
Table of content:
- What Is A Python For Loop?
- How Does Python For Loop Work?
- When & Why To Use Python For Loops?
- Python For Loop Examples
- What Is Rrange() Function In Python?
- Nested For Loops In Python
- Python For Loop With Continue & Break Statements
- Python For Loop With Pass Statement
- Else Statement In Python For Loop
- Conclusion
- Frequently Asked Questions
- Think You Know Python's For Loop? Prove It!
Table of content:
- What Is Python While Loop?
- How Does The Python While Loop Work?
- How To Use Python While Loops For Iterations?
- Control Statements In Python While Loop With Examples
- Python While Loop With Python List
- Infinite Python While Loop in Python
- Python While Loop Multiple Conditions
- Nested Python While Loops
- Conclusion
- Frequently Asked Questions
- Mastered Python While Loop? Let’s Find Out!
Table of content:
- What Are Conditional If-Else Statements In Python?
- Types Of If-Else Statements In Python
- If Statement In Python
- If-Else Statement In Python
- Nested If-Else Statement In Python
- Elif Statement In Python
- Ladder If-Elif-Else Statement In Python
- Short Hand If-Statement In Python
- Short Hand If-Else Statement In Python
- Operators & If-Esle Statement In Python
- Other Statements With If-Else In Python
- Conclusion
- Frequently Asked Questions
- Quick If-Else Statement Quiz– Let’s Go!
Table of content:
- What Is Control Structure In Python?
- Types Of Control Structures In Python
- Sequential Control Structures In Python
- Decision-Making Control Structures In Python
- Repetition Control Structures In Python
- Benefits Of Using Control Structures In Python
- Conclusion
- Frequently Asked Questions
- Control Structures in Python – Are You the Master? Take A Quiz!
Table of content:
- What Are Python Libraries?
- How Do Python Libraries Work?
- Standard Python Libraries (With List)
- Important Python Libraries For Data Science
- Important Python Libraries For Machine & Deep Learning
- Other Important Python Libraries You Must Know
- Working With Third-Party Python Libraries
- Troubleshooting Common Issues For Python Libraries
- Python Libraries In Larger Projects
- Importance Of Python Libraries
- Conclusion
- Frequently Asked Questions
- Quick Quiz On Python Libraries – Let’s Go!
Table of content:
- What Are Python Functions?
- How To Create/ Define Functions In Python?
- How To Call A Python Function?
- Types Of Python Functions Based On Parameters & Return Statement
- Rules & Best Practices For Naming Python Functions
- Basic Types of Python Functions
- The Return Statement In Python Functions
- Types Of Arguments In Python Functions
- Docstring In Python Functions
- Passing Parameters In Python Functions
- Python Function Variables | Scope & Lifetime
- Advantages Of Using Python Functions
- Recursive Python Function
- Anonymous/ Lambda Function In Python
- Nested Functions In Python
- Conclusion
- Frequently Asked Questions
- Python Functions – Test Your Knowledge With A Quiz!
Table of content:
- What Are Python Built-In Functions?
- Mathematical Python Built-In Functions
- Python Built-In Functions For Strings
- Input/ Output Built-In Functions In Python
- List & Tuple Python Built-In Functions
- File Handling Python Built-In Functions
- Python Built-In Functions For Dictionary
- Type Conversion Python Built-In Functions
- Basic Python Built-In Functions
- List Of Python Built-In Functions (Alphabetical)
- Conclusion
- Frequently Asked Questions
- Think You Know Python Built-in Functions? Prove It!
Table of content:
- What Is A round() Function In Python?
- How Does Python round() Function Work?
- Python round() Function If The Second Parameter Is Missing
- Python round() Function If The Second Parameter Is Present
- Python round() Function With Negative Integers
- Python round() Function With Math Library
- Python round() Function With Numpy Module
- Round Up And Round Down Numbers In Python
- Truncation Vs Rounding In Python
- Practical Applications Of Python round() Function
- Conclusion
- Frequently Asked Questions
- Revisit Python’s round() Function – Take The Quiz!
Table of content:
- What Is Python pow() Function?
- Python pow() Function Example
- Python pow() Function With Modulus (Three Parameters)
- Python pow() Function With Complex Numbers
- Python pow() Function With Floating-Point Arguments And Modulus
- Python pow() Function Implementation Cases
- Difference Between Inbuilt-pow() And math.pow() Function
- Conclusion
- Frequently Asked Questions
- Test Your Knowledge Of Python’s pow() Function!
Table of content:
- Python max() Function With Objects
- Examples Of Python max() Function With Objects
- Python max() Function With Iterable
- Examples Of Python max() Function With Iterables
- Potential Errors With The Python max() Function
- Python max() Function Vs. Python min() Functions
- Conclusion
- Frequently Asked Questions
- Think You Know Python max() Function? Take A Quiz!
Table of content:
- What Are Strings In Python?
- What Are Python String Methods?
- List Of Python String Methods For Manipulating Case
- List Of Python String Methods For Searching & Finding
- List Of Python String Methods For Modifying & Transforming
- List Of Python String Methods For Checking Conditions
- List Of Python String Methods For Encoding & Decoding
- List Of Python String Methods For Stripping & Trimming
- List Of Python String Methods For Formatting
- Miscellaneous Python String Methods
- List Of Other Python String Operations
- Conclusion
- Frequently Asked Questions
- Mastered Python String Methods? Take A Quiz!
Table of content:
- What Is Python String?
- The Need For Python String Replacement
- The Python String replace() Method
- Multiple Replacements With Python String.replace() Method
- Replace A Character In String Using For Loop In Python
- Python String Replacement Using Slicing Method
- Replace A Character At a Given Position In Python String
- Replace Multiple Substrings With The Same String In Python
- Python String Replacement Using Regex Pattern
- Python String Replacement Using List Comprehension & Join() Method
- Python String Replacement Using Callback With re.sub() Method
- Python String Replacement With re.subn() Method
- Conclusion
- Frequently Asked Questions
- Know How To Replace Python Strings? Prove It!
Table of content:
- What Is String Slicing In Python?
- How Indexing & String Slicing Works In Python
- Extracting All Characters Using String Slicing In Python
- Extracting Characters Before & After Specific Position Using String Slicing In Python
- Extracting Characters Between Two Intervals Using String Slicing In Python
- Extracting Characters At Specific Intervals (Step) Using String Slicing In Python
- Negative Indexing & String Slicing In Python
- Handling Out-of-Bounds Indices In String Slicing In Python
- The slice() Method For String Slicing In Python
- Common Pitfalls Of String Slicing In Python
- Real-World Applications Of String Slicing
- Conclusion
- Frequently Asked Questions
- Quick Python String Slicing Quiz– Let’s Go!
Table of content:
- Introduction To Python List
- How To Create A Python List?
- How To Access Elements Of Python List?
- Accessing Multiple Elements From A Python List (Slicing)
- Access List Elements From Nested Python Lists
- How To Change Elements In Python Lists?
- How To Add Elements To Python Lists?
- Delete/ Remove Elements From Python Lists
- How To Create Copies Of Python Lists?
- Repeating Python Lists
- Ways To Iterate Over Python Lists
- How To Reverse A Python List?
- How To Sort Items Of Python Lists?
- Built-in Functions For Operations On Python Lists
- Conclusion
- Frequently Asked Questions
- Revisit Python Lists Basics With A Quick Quiz!
Table of content:
- What Is List Comprehension In Python?
- Incorporating Conditional Statements With List Comprehension In Python
- List Comprehension In Python With range()
- Filtering Lists Effectively With List Comprehension In Python
- Nested Loops With List Comprehension In Python
- Flattening Nested Lists With List Comprehension In Python
- Handling Exceptions In List Comprehension In Python
- Common Use Cases For List Comprehensions
- Advantages & Disadvantages Of List Comprehension In Python
- Best Practices For Using List Comprehension In Python
- Performance Considerations For List Comprehension In Python
- For Loops & List Comprehension In Python: A Comparison
- Difference Between Generator Expression & List Comprehension In Python
- Conclusion
- Frequently Asked Questions
- Rehash Python List Comprehension Basics With A Quiz!
Table of content:
- What Is A List In Python?
- How To Find Length Of List In Python?
- For Loop To Get Python List Length (Naive Approach)
- The len() Function To Get Length Of List In Python
- The length_hint() Function To Find Length Of List In Python
- The sum() Function To Find The Length Of List In Python
- The enumerate() Function To Find Python List Length
- The Counter Class From collections To Find Python List Length
- The List Comprehension To Find Python List Length
- Find The Length Of List In Python Using Recursion
- Comparison Between Ways To Find Python List Length
- Conclusion
- Frequently Asked Questions
- Know How To Get Python List Length? Prove it!
Table of content:
- List of Methods To Reverse A Python List
- Python Reverse List Using reverse() Method
- Python Reverse List Using the Slice Operator ([::-1])
- Python Reverse List By Swapping Elements
- Python Reverse List Using The reversed() Function
- Python Reverse List Using A for Loop
- Python Reverse List Using While Loop
- Python Reverse List Using List Comprehension
- Python Reverse List Using List Indexing
- Python Reverse List Using The range() Function
- Python Reverse List Using NumPy
- Comparison Of Ways To Reverse A Python List
- Conclusion
- Frequently Asked Questions
- Time To Test Your Python List Reversal Skills!
Table of content:
- What Is Indexing In Python?
- The Python List index() Function
- How To Use Python List index() To Find Index Of A List Element
- The Python List index() Method With Single Parameter (Start)
- The Python List index() Method With Start & Stop Parameters
- What Happens When We Use Python List index() For An Element That Doesn't Exist
- Python List index() With Nested Lists
- Fixing IndexError Using The Python List index() Method
- Python List index() With Enumerate()
- Real-world Examples Of Python List index() Method
- Difference Between find() And index() Method In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python List Indexing? Take A Quiz!
Table of content:
- How To Remove Elements From List In Python?
- The remove() Method To Remove Element From Python List
- The pop() Method To Remove Element From List In Python
- The del Keyword To Remove Element From List In Python
- The clear() Method To Remove Elements From Python List
- List Comprehensions To Conditionally Remove Element From List In Python
- Key Considerations For Removing Elements From Python Lists
- Why We Need to Remove Elements From Python List
- Performance Comparison Of Methods To Remove Element From List In Python
- Conclusion
- Frequently Asked Questions
- Quiz– Prove You Know How To Remove Item From Python Lists!
Table of content:
- How To Remove Duplicates From A List In Python?
- The set() Function To Remove Duplicates From Python List
- Remove Duplicates From Python List Using For Loop
- Using List Comprehension Remove Duplicates From Python List
- Remove Duplicates From Python List Using enumerate() With List Comprehension
- Dictionary & fromkeys() Method To Remove Duplicates From Python List
- Remove Duplicates From Python List Using in, not in Operators
- Remove Duplicates From Python List Using collections.OrderedDict.fromkeys()
- Remove Duplicates From Python List Using Counter with freq.dist() Method
- The del Keyword Remove Duplicates From Python List
- Remove Duplicates From Python List Using DataFrame
- Remove Duplicates From Python List Using pd.unique and np.unipue
- Remove Duplicates From Python List Using reduce() function
- Comparative Analysis Of Ways To Remove Duplicates From Python List
- Conclusion
- Frequently Asked Questions
- Think You Know How to Remove Duplicates? Take A Quiz!
Table of content:
- What Is Python List & How To Access Elements?
- What Is IndexError: List Index Out Of Range & Its Causes In Python?
- Understanding Indexing Behavior In Python Lists
- How to Prevent/ Fix IndexError: List Index Out Of Range In Python
- Handling IndexError Gracefully Using Try-Except
- Debugging Tips For IndexError: List Index Out Of Range Python
- Conclusion
- Frequently Asked Questions
- Avoiding ‘List Index Out of Range’ Errors? Take A Quiz!
Table of content:
- What Is the Python sort() List Method?
- Sorting In Ascending Order Using The Python sort() List Method
- How To Sort Items In Descending Order Using Python sort() List Method
- Custom Sorting Using The Key Parameter Of Python sort() List Method
- Examples Of Python sort() List Method
- What Is The sorted() List Method In Python
- Differences Between sorted() And sort() List Methods In Python
- When To Use sorted() & When To Use sort() List Method In Python
- Conclusion
- Frequently Asked Questions
- Take A Quick Python's sort() Quiz!
Table of content:
- What Is A List In Python?
- What Is A String In Python?
- Why Convert Python List To String?
- How To Convert List To String In Python?
- The join() Method To Convert Python List To String
- Convert Python List To String Through Iteration
- Convert Python List To String With List Comprehension
- The map() Function To Convert Python List To String
- Convert Python List to String Using format() Function
- Convert Python List To String Using Recursion
- Enumeration Function To Convert Python List To String
- Convert Python List To String Using Operator Module
- Python Program To Convert String To List
- Conclusion
- Frequently Asked Questions
- Convert Lists To Strings Like A Pro! Take A Quiz
Table of content:
- What Is Inheritance In Python?
- Python Inheritance Syntax
- Parent Class In Python Inheritance
- Child Class In Python Inheritance
- The __init__() Method In Python Inheritance
- The super() Function In Python Inheritance
- Method Overriding In Python Inheritance
- Types Of Inheritance In Python
- Special Functions In Python Inheritance
- Advantages & Disadvantages Of Inheritance In Python
- Common Use Cases For Inheritance In Python
- Best Practices for Implementing Inheritance in Python
- Avoiding Common Pitfalls in Python Inheritance
- Conclusion
- Frequently Asked Questions
- 💡 Python Inheritance Quiz – Are You Ready?
Table of content:
- What Is The Python List append() Method?
- Adding Elements To A Python List Using append()
- Populate A Python List Using append()
- Adding Different Data Types To Python List Using append()
- Adding A List To Python List Using append()
- Nested Lists With Python List append() Method
- Practical Use Cases Of Python List append() Method
- How append() Method Affects List Performance
- Avoiding Common Mistakes When Using Python List append()
- Comparing extend() With append() Python List Method
- Conclusion
- Frequently Asked Questions
- 🧠 Think You Know Python List append()? Take A Quiz!
Table of content:
- What Is A Linked List In Python?
- Types Of Linked Lists In Python
- How To Create A Linked List In Python
- How To Traverse A Linked List In Python & Retrieve Elements
- Inserting Elements In A Linked List In Python
- Deleting Elements From A Linked List In Python
- Update A Node Of Linked List In Python
- Reversing A Linked List In Python
- Calculating Length Of A Linked List In Python
- Comparing Arrays And Linked Lists In Python
- Advantages & Disadvantages Of Linked List In Python
- When To Use Linked Lists Over Other Data Structures
- Practical Applications Of Linked Lists In Python
- Conclusion
- Frequently Asked Questions
- 🔗 Linked List Logic: Can You Ace This Quiz?
Table of content:
- What Is Extend In Python?
- Extend In Python With List
- Extend In Python With String
- Extend In Python With Tuple
- Extend In Python With Set
- Extend In Python With Dictionary
- Other Methods To Extend A List In Python
- Difference Between append() and extend() In Python
- Conclusion
- Frequently Asked Questions
- Think You Know extend() In Python? Prove It!
Table of content:
- What Is Recursion In Python?
- Key Components Of Recursive Functions In Python
- Implementing Recursion In Python
- Recursion Vs. Iteration In Python
- Tail Recursion In Python
- Infinite Recursion In Python
- Advantages Of Recursion In Python
- Disadvantages Of Recursion In Python
- Best Practices For Using Recursion In Python
- Conclusion
- Frequently Asked Questions
- Recursive Thinking In Python: Test Your Skills!
Table of content:
- What Is Type Conversion In Python?
- Types Of Type Conversion In Python
- Implicit Type Conversion In Python
- Explicit Type Conversion In Python
- Functions Used For Explicit Data Type Conversion In Python
- Important Type Conversion Tips In Python
- Benefits Of Type Conversion In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Type Conversion? Take A Quiz!
Table of content:
- What Is Scope In Python?
- Local Scope In Python
- Global Scope In Python
- Nonlocal (Enclosing) Scope In Python
- Built-In Scope In Python
- The LEGB Rule For Python Scope
- Python Scope And Variable Lifetime
- Best Practices For Managing Python Scope
- Conclusion
- Frequently Asked Questions
- Think You Know Python Scope? Test Yourself!
Table of content:
- Understanding The Continue Statement In Python
- How Does Continue Statement Work In Python?
- Python Continue Statement With For Loops
- Python Continue Statement With While Loops
- Python Continue Statement With Nested Loops
- Python Continue With If-Else Statement
- Difference Between Pass and Continue Statement In Python
- Practical Applications Of Continue Statement In Python
- Conclusion
- Frequently Asked Questions
- Python 'continue' Statement Quiz: Can You Ace It?
Table of content:
- What Are Control Statements In Python?
- Types Of Control Statements In Python
- Conditional Control Statements In Python
- Loop Control Statements In Python
- Control Flow Altering Statements In Python
- Exception Handling Control Statements In Python
- Conclusion
- Frequently Asked Questions
- Mastering Control Statements In Python – Take the Quiz!
Table of content:
- Difference Between Mutable And Immutable Data Types in Python
- What Is Mutable Data Type In Python?
- Types Of Mutable Data Types In Python
- What Are Immutable Data Types In Python?
- Types Of Immutable Data Types In Python
- Key Similarities Between Mutable And Immutable Data Types In Python
- When To Use Mutable Vs Immutable In Python?
- Conclusion
- Frequently Asked Questions
- Quiz Time: Mutable vs. Immutable In Python!
Table of content:
- What Is A List?
- What Is A Tuple?
- Difference Between List And Tuple In Python (Comparison Table)
- Syntax Difference Between List And Tuple In Python
- Mutability Difference Between List And Tuple In Python
- Other Difference Between List And Tuple In Python
- List Vs. Tuple In Python | Methods
- When To Use Tuples Over Lists?
- Key Similarities Between Tuples And Lists In Python
- Conclusion
- Frequently Asked Questions
- 🧐 Lists vs. Tuples Quiz: Test Your Python Knowledge!
Table of content:
- Introduction to Python
- Downloading & Installing Python, IDLE, Tkinter, NumPy & PyGame
- Creating A New Python Project
- How To Write Python Hello World Program In Python?
- Way To Write The Hello, World! Program In Python
- The Hello, World! Program In Python Using Class
- The Hello, World! Program In Python Using Function
- Print Hello World 5 Times Using A For Loop
- Conclusion
- Frequently Asked Questions
- 👋 Python's 'Hello, World!'—How Well Do You Know It?
Table of content:
- Algorithm Of Python Program To Add To Numbers
- Standard Program To Add Two Numbers In Python
- Python Program To Add Two Numbers With User-defined Input
- The add() Method In Python Program To Add Two Numbers
- Python Program To Add Two Numbers Using Lambda
- Python Program To Add Two Numbers Using Function
- Python Program To Add Two Numbers Using Recursion
- Python Program To Add Two Numbers Using Class
- How To Add Multiple Numbers In Python?
- Add Multiple Numbers In Python With User Input
- Time Complexities Of Python Programs To Add Two Numbers
- Conclusion
- Frequently Asked Questions
- 💡 Quiz Time: Python Addition Basics!
Table of content:
- Swapping in Python
- Swapping Two Variables Using A Temporary Variable
- Swapping Two Variables Using The Comma Operator In Python
- Swapping Two Variables Using The Arithmetic Operators (+,-)
- Swapping Two Variables Using The Arithmetic Operators (*,/)
- Swapping Two Variables Using The XOR(^) Operator
- Swapping Two Variables Using Bitwise Addition and Subtraction
- Swap Variables In A List
- Conclusion
- Frequently Asked Questions (FAQs)
- Quiz To Test Your Variable Swapping Knowledge
Table of content:
- What Is A Quadratic Equation? How To Solve It?
- How To Write A Python Program To Solve Quadratic Equations?
- Python Program To Solve Quadratic Equations Directly Using The Formula
- Python Program To Solve Quadratic Equations Using The Complex Math Module
- Python Program To Solve Quadratic Equations Using Functions
- Python Program To Solve Quadratic Equations & Find Number Of Solutions
- Python Program To Plot Quadratic Functions
- Conclusion
- Frequently Asked Questions
- Quadratic Equations In Python Quiz: Test Your Knowledge!
Table of content:
- What Is Decimal Number System?
- What Is Binary Number System?
- What Is Octal Number System?
- What Is Hexadecimal Number System?
- Python Program to Convert Decimal to Binary, Octal, And Hexadecimal Using Built-In Function
- Python Program To Convert Decimal To Binary Using Recursion
- Python Program To Convert Decimal To Octal Using Recursion
- Python Program To Convert Decimal To Hexadecimal Using Recursion
- Python Program To Convert Decimal To Binary Using While Loop
- Python Program To Convert Decimal To Octal Using While Loop
- Python Program To Convert Decimal To Hexadecimal Using While Loop
- Convert Decimal To Binary, Octal, And Hexadecimal Using String Formatting
- Python Program To Convert Binary, Octal, And Hexadecimal String To A Number
- Complexity Comparison Of Python Programs To Convert Decimal To Binary, Octal, And Hexadecimal
- Conclusion
- Frequently Asked Questions
- 💡 Decimal To Binary, Octal & Hex: Quiz Time!
Table of content:
- What Is A Square Root?
- Python Program To Find The Square Root Of A Number
- The pow() Function In Python Program To Find The Square Root Of Given Number
- Python Program To Find Square Root Using The sqrt() Function
- The cmath Module & Python Program To Find The Square Root Of A Number
- Python Program To Find Square Root Using The Exponent Operator (**)
- Python Program To Find Square Root With A User-Defined Function
- Python Program To Find Square Root Using A Class
- Python Program To Find Square Root Using Binary Search
- Python Program To Find Square Root Using NumPy Module
- Conclusion
- Frequently Asked Questions
- 🤓 Think You Know Square Roots In Python? Take A Quiz!
Table of content:
- Understanding the Logic Behind the Conversion of Kilometers to Miles
- Steps To Write Python Program To Convert Kilometers To Miles
- Python Program To Convert Kilometer To Miles Without Function
- Python Program To Convert Kilometer To Miles Using Function
- Python Program to Convert Kilometer To Miles Using Class
- Tips For Writing Python Program To Convert Kilometer To Miles
- Conclusion
- Frequently Asked Questions
- 🧐 Mastered Kilometer To Mile Conversion? Prove It!
Table of content:
- Why Build A Calculator Program In Python?
- Prerequisites To Writing A Calculator Program In Python
- Approach For Writing A Calculator Program In Python
- Simple Calculator Program In Python
- Calculator Program In Python Using Functions
- Creating GUI Calculator Program In Python Using Tkinter
- Conclusion
- Frequently Asked Questions
- 🧮 Calculator Program In Python Quiz!
Table of content:
- The Calendar Module In Python
- Prerequisites For Writing A Calendar Program In Python
- How To Write And Print A Calendar Program In Python
- Calendar Program In Python To Display A Month
- Calendar Program In Python To Display A Year
- Conclusion
- Frequently Asked Questions
- Calendar Program In Python – Quiz Time!
Table of content:
- What Is The Fibonacci Series?
- Pseudocode Code For Fibonacci Series Program In Python
- Generating Fibonacci Series In Python Using Naive Approach (While Loop)
- Fibonacci Series Program In Python Using The Direct Formula
- How To Generate Fibonacci Series In Python Using Recursion?
- Generating Fibonacci Series In Python With Dynamic Programming
- Fibonacci Series Program In Python Using For Loop
- Generating Fibonacci Series In Python Using If-Else Statement
- Generating Fibonacci Series In Python Using Arrays
- Generating Fibonacci Series In Python Using Cache
- Generating Fibonacci Series In Python Using Backtracking
- Fibonacci Series In Python Using Power Of Matix
- Complexity Analysis For Fibonacci Series Programs In Python
- Applications Of Fibonacci Series In Python & Programming
- Conclusion
- Frequently Asked Questions
- 🤔 Think You Know Fibonacci Series? Take A Quiz!
Table of content:
- Different Ways To Write Random Number Generator Python Programs
- Random Module To Write Random Number Generator Python Programs
- The Numpy Module To Write Random Number Generator Python Programs
- The Secrets Module To Write Random Number Generator Python Programs
- Understanding Randomness and Pseudo-Randomness In Python
- Common Issues and Solutions in Random Number Generation
- Applications of Random Number Generator Python
- Conclusion
- Frequently Asked Questions
- Think You Know Python's Random Module? Prove It!
Table of content:
- What Is A Factorial?
- Algorithm Of Program To Find Factorial Of A Number In Python
- Pseudocode For Factorial Program in Python
- Factorial Program In Python Using For Loop
- Factorial Program In Python Using Recursion
- Factorial Program In Python Using While Loop
- Factorial Program In Python Using If-Else Statement
- The math Module | Factorial Program In Python Using Built-In Factorial() Function
- Python Program to Find Factorial of a Number Using Ternary Operator(One Line Solution)
- Python Program For Factorial Using Prime Factorization Method
- NumPy Module | Factorial Program In Python Using numpy.prod() Function
- Complexity Analysis Of Factorial Programs In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Factorials In Python? Take A Quiz!
Table of content:
- What Is Palindrome In Python?
- Check Palindrome In Python Using While Loop (Iterative Approach)
- Check Palindrome In Python Using For Loop And Character Matching
- Check Palindrome In Python Using The Reverse And Compare Method (Python Slicing)
- Check Palindrome In Python Using The In-built reversed() And join() Methods
- Check Palindrome In Python Using Recursion Method
- Check Palindrome In Python Using Flag
- Check Palindrome In Python Using One Extra Variable
- Check Palindrome In Python By Building Reverse, One Character At A Time
- Complexity Analysis For Palindrome Programs In Python
- Real-World Applications Of Palindrome In Python
- Conclusion
- Frequently Asked Questions
- Think You Know Palindromes? Take A Quiz!
Table of content:
- Best Python Books For Beginners
- Best Python Books For Intermediate Level
- Best Python Books For Experts
- Best Python Books To Learn Algorithms
- Audiobooks of Python
- Best Books To Learn Python And Code Like A Pro
- To Learn Python Libraries
- Books To Provide Extra Edge In Python
- Python Project Ideas - Reference
- Quiz To Rehash Your Knowledge Of Python Books!
Python extend() Function | Syntax, Techniques & More (+Examples)
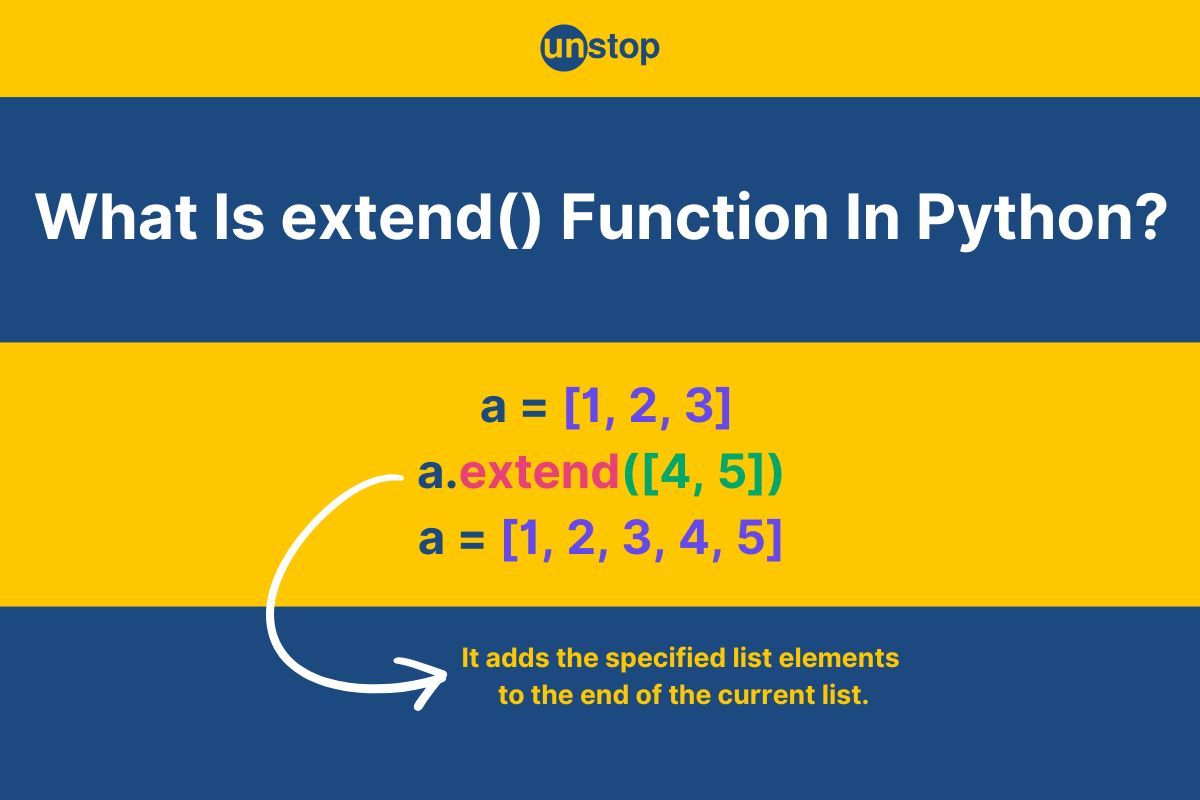
In Python, the extend() function is a built-in method that belongs to list objects. It is used to add all elements of an iterable (such as a list, tuple, or set) to the end of an existing list. Unlike the append() method, which adds a single element to the list, extend() enables you to concatenate multiple elements at once, making it an essential tool for list manipulation and data aggregation.
In this article, we will explore the extend() function in detail, covering its syntax, how it operates with different iterable types, and practical use cases. We will also provide examples to illustrate its functionality, compare it with similar methods, and discuss best practices for using extend() effectively.
What Is Extend In Python?
The extend() method in Python programming is used to add elements from an iterable to the end of a list. This method allows you to combine lists or add multiple items at once. It is important to understand how this method works for effective list management.
Syntax Of Extend In Python
The syntax for using extend() in Python is straightforward:
list.extend(iterable)
Here:
- list: This is the target list that will be modified.
- iterable: This can be any iterable object, such as a list, tuple, or string. Each element of this iterable will be added to the list.
Return Value Of Extend In Python
The extend() function in Python does not return a new list i.e. it returns None. Instead, it modifies the original list in place:
- It iterates over each element in the provided iterable.
- Each element is added one by one to the end of the existing list.
We will now look at some common code examples to understand how to work with extend() method in Python programming.
Extend In Python With List
The extend() method can be used to merge one list into another by appending all elements of the second list to the first. This is useful for combining lists without creating a new list, allowing for efficient data management.
Code Example:
# Initializing two lists
list1 = [10, 20, 30]
list2 = [40, 50, 60]
# Using the extend() method to add elements of list2 to list1
list1.extend(list2)
# Output the modified list1
print(list1)
Output:
[10, 20, 30, 40, 50, 60]
Explanation:
In the above code example-
- We start by initializing two lists, list1 containing the values [10, 20, 30] and list2 containing the values [40, 50, 60].
- Next, we use the extend() method on list1 to add all the elements from list2 to it. This method modifies list1 in place, meaning it will now contain both its original elements and the elements from list2.
- Finally, we print the modified list1 to see the result. The output shows that the elements from list2 have been successfully added to list1.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
Extend In Python With String
Python allows us to extend lists with strings. This is useful when you want to add multiple characters or words into a list. When a string is used with extend(), each character of the string is treated as a separate element, allowing for easy concatenation of characters to a list.
Code Example:
# Initializing a list and a string
list1 = ['A', 'B', 'C']
string1 = 'DEF'
# Using the extend() method to add characters of string1 to list1
list1.extend(string1) # Each character of the string is added individually
# Output the modified list1
print(list1)
Output:
['A', 'B', 'C', 'D', 'E', 'F']
Explanation:
In the above code example-
- We start by initializing a list, list1, with the values ['A', 'B', 'C'], and a string, string1, containing the characters 'DEF'.
- Next, we use the extend() method on list1 to add the characters from string1. Each character of the string is added individually to the list.
- Finally, we print the modified list1 to see the result. The output showcases that the characters from string1 have been successfully appended to list1.
Extend In Python With Tuple
Tuples are a type of data structure in Python. They hold a collection of items. Unlike lists, tuples are immutable, meaning their contents cannot be changed after creation. This characteristic makes them useful for storing fixed collections of data.
The extend() method is typically used with lists. However, you can still use it with tuples by converting them to lists first. This approach allows you to add items from a tuple to a list effectively.
Code Example:
# Initializing a list and a tuple
list1 = [7, 8, 9]
tuple1 = (10, 11, 12)
# Using the extend() method to add elements of tuple1 to list1
list1.extend(tuple1) # Converting tuple to list during extension
# Output the modified list1
print(list1) #
Output:
[7, 8, 9, 10, 11, 12]
Explanation:
In the above Python code example-
- We begin by initializing a list, list1, with the values [7, 8, 9], and a tuple, tuple1, containing the values (10, 11, 12).
- Next, we use the extend() method on list1 to add the elements from tuple1. During this process, the tuple is automatically converted to a list, enabling us to append its elements to list1.
- Finally, we print the modified list1 to see the result. The output confirms that the elements from tuple1 have been successfully added to list1.
Extend In Python With Set
The extend() method cannot be directly used with sets because it is specifically designed for lists. However, we can convert a set to a list and then use extend() to add its elements to another list. This approach allows us to effectively merge elements from a set into a list.
Code Example:
# Initializing a list and a set
list1 = [1, 2, 3]
set1 = {4, 5, 6}
# Using extend() to add elements of set1 to list1
list1.extend(set1) # Converting set to list during extension
# Output the modified list1
print(list1)
Output:
[1, 2, 3, 4, 5, 6]
Explanation:
In the above code example-
- We start by initializing a list, list1, with the values [1, 2, 3], and a set, set1, containing the values {4, 5, 6}.
- Next, we use the extend() method on list1 to add the elements from set1. During this process, the set is automatically converted to a list, allowing us to append its elements to list1.
- Finally, we print the modified list1 to see the result. The output indicates that the elements from set1 have been successfully added to list1.
Extend In Python With Dictionary
Dictionaries in Python are collections of key-value pairs. Each key is unique, and it maps to a specific value. We can extend a list with the keys or values of a dictionary. When using extend(), we can choose to add either the keys or the values, depending on your requirements.
Code Example:
# Initializing a list and a dictionary
list1 = ['X', 'Y', 'Z']
dict1 = {'a': 1, 'b': 2, 'c': 3}
# Using extend() to add keys of dict1 to list1
list1.extend(dict1) # Adding dictionary keys to the list
# Output the modified list1
print(list1)
Output:
['X', 'Y', 'Z', 'a', 'b', 'c']
Explanation:
In the above Python code example-
- We start by initializing a list, list1, with the values ['X', 'Y', 'Z'], and a dictionary, dict1, containing the key-value pairs {'a': 1, 'b': 2, 'c': 3}.
- Next, we use the extend() method on list1 to add the keys from dict1. This adds each key from the dictionary to the list.
- Finally, we print the modified list1 to see the result. The output indicates that the keys from dict1 have been successfully appended to list1.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Other Methods To Extend A List In Python
In addition to the extend() method, there are several other ways to extend a list in Python. Here, we’ll explore three alternative methods: using the + operator, slicing syntax, and the chain() method from the itertools module.
Using The + Operator
The + operator, also known as the concatenation operator, can be used to concatenate two lists, creating a new list that combines both. This method does not modify the original list but returns a new one.
Code Example
# Initializing two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# Using the + operator to combine lists
result = list1 + list2
# Output the new combined list
print(result)
Output:
[1, 2, 3, 4, 5, 6]
Explanation:
In the above Python code example-
- We start by initializing two lists: list1 with the values [1, 2, 3] and list2 with the values [4, 5, 6].
- Next, we use the + operator to combine the two lists, which creates a new list named result.
- This operation does not modify the original lists; instead, it produces a new list that contains the elements of both list1 and list2.
- Finally, we print the new combined list, result, to see the outcome. The output shows that the elements from both lists have been successfully combined into one list.
Using Slicing Syntax
Slicing syntax is another way to extend a list. This method involves using the slice assignment feature of Python. Slicing can insert elements from another list into a specific position within the original list. It allows for more control over where the elements are added.
Code Example:
# Initializing a list and another list to be added
list1 = [7, 8, 9]
list2 = [10, 11, 12]
# Using slicing to extend list1 with elements from list2
list1[len(list1):] = list2 # Adds list2 elements to the end of list1
# Output the modified list1
print(list1)
Output:
[7, 8, 9, 10, 11, 12]
Explanation:
In the above code example-
- We start by initializing a list, list1, with the values [7, 8, 9] and another list, list2, containing the values [10, 11, 12].
- Next, we use slicing to extend list1 with the elements from list2. Specifically, we set the slice list1[len(list1):] to list2, which effectively adds the elements of list2 to the end of list1.
- Finally, we print the modified list1 to see the result. The output indicates that the elements from list2 have been successfully added to the end of list1.
Using The chain() Method
The chain() method from the itertools module can combine multiple tables into a single iterable. This method is especially useful for combining various types of tables, such as lists, tuples, and sets. It avoids creating intermediate lists and is generally more efficient in terms of memory usage.
Code Example:
from itertools import chain
# Initializing a list and a tuple
list1 = [13, 14, 15]
tuple1 = (16, 17, 18)
# Using chain() to combine list1 and tuple1
result = list(chain(list1, tuple1))
# Output the new combined list
print(result)
Output:
[13, 14, 15, 16, 17, 18]
Explanation:
In the above code example-
- We begin by importing the chain() function from the itertools module.
- Next, we initialize a list, list1, with the values [13, 14, 15], and a tuple, tuple1, containing the values (16, 17, 18).
- We then use the function chain() function to combine list1 and tuple1. The chain() function takes multiple iterable inputs and produces a single iterable that yields elements from each input in sequence.
- We convert the result of chain() to a list, storing it in the variable result.
- Finally, we print the new combined list, result, to see the outcome. The output confirms that the elements from both list1 and tuple1 have been successfully combined into one list.
Difference Between append() and extend() In Python
Given below are the key differences between the append() and extend() method in Python:
Feature | append() | extend() |
---|---|---|
Purpose | Adds a single element to the end of the list. | Adds multiple elements from an iterable to the end of the list. |
Parameter Type | Accepts a single element (any data type). | Accepts an iterable (like a list, tuple, or set). |
Return Value | Returns None. | Returns None. |
Modification | Modifies the original list by adding one element. | Modifies the original list by adding multiple elements. |
Usage Example | list.append(item) | list.extend(iterable) |
Code Example:
# Initializing a list
my_list = [1, 2, 3]
# Using append() to add a single element
my_list.append(4)
# Using extend() to add multiple elements
my_list.extend([5, 6, 7])
# Output the modified list
print(my_list)
Output:
[1, 2, 3, 4, 5, 6, 7]
Explanation:
In the above code example-
- We start by initializing a list called my_list with the values [1, 2, 3].
- Next, we use the append() method to add a single element, 4, to the end of my_list. After this operation, the list becomes [1, 2, 3, 4].
- Then, we use the extend() method to add multiple elements at once. We pass the list [5, 6, 7] to extend(), which adds these elements to the end of my_list.
- Finally, we print the modified my_list to see the result. The output will be [1, 2, 3, 4, 5, 6, 7], confirming that both the single element and the multiple elements have been successfully added to the list.
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
Conclusion
The extend() method in Python language is a powerful tool for efficiently adding multiple elements from an iterable to the end of a list. By understanding its syntax, return value, and usage with various data types such as lists, sets, tuples, strings, and dictionaries, you can enhance your list manipulation capabilities.
We also explored alternative methods for extending lists, including the + operator, slicing syntax, and the chain() method from the itertools module. Each offers unique advantages depending on the context. By mastering these techniques, you can choose the most suitable approach for your specific programming needs, leading to cleaner and more effective code.
Frequently Asked Questions
Q. What is Python List extend() method?
The Python extend() method is a built-in function used to add multiple elements from an iterable (such as a list, tuple, set, or string) to the end of an existing list. Unlike append(), which adds a single element as a whole to the list, extend() iterates over the given iterable and adds each element individually to the list.
Key Points:
- Modifies the list in place: The original list is updated directly.
- Takes an iterable: You can pass any iterable (e.g., list, tuple, set, string) to extend(), and each element from the iterable is added to the list.
- No return value: It modifies the list but does not return a new list or any value (None is returned).
Q. Can I use the extend() method to add non-iterable elements like integers to a list?
No, the extend() method only works with iterables, such as lists, tuples, sets, strings, and dictionaries (keys or values). If you try to pass a non-iterable like an integer, Python will raise a TypeError. If you want to add a single non-iterable element (such as an integer), you should use the append() method instead. For Example-
my_list = [1, 2, 3]
my_list.extend(4) # Raises TypeError
# Use append() instead
my_list.append(4)
Q. Does the extend() method return a new list or modify the existing one?
The extend() method modifies the existing list in place and does not return a new list. After using extend in Python, the original list will be updated with the new elements. If you need a new list with the combined elements, you can use the + operator or create a copy of the original list before extending it.
Q. How is extend() different from using the + operator to concatenate lists?
The key difference is that extend() modifies the list in place, while the + operator creates a new list that combines the two. If you want to keep the original list unchanged and create a new list with the combined elements, the + operator is the better option. If you want to update the original list, use extend(). For Example-
# Initializing two lists
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# Using extend()
list1.extend(list2)
print(list1) # Output: [1, 2, 3, 4, 5, 6]
# Using + operator
list1 = [1, 2, 3]
new_list = list1 + list2
print(new_list) # Output: [1, 2, 3, 4, 5, 6]
print(list1) # Original list remains unchanged: [1, 2, 3]
Q. What happens if I extend a list with an empty iterable?
If you extend a list with an empty iterable, such as an empty list [] or an empty tuple (), the original list remains unchanged. The extend() method only adds elements from the iterable, so if the iterable is empty, no elements are added. For Example-
# Initializing a list
my_list = [1, 2, 3]
# Extending with an empty list
my_list.extend([])
print(my_list) # Output: [1, 2, 3]
# Extending with an empty tuple
my_list.extend(())
print(my_list) # Output: [1, 2, 3]
In these cases, the extend() method does nothing because there are no elements to add.
Q. Can I use extend() to combine more than two lists or iterables at once?
No, the extend() method can only take one iterable at a time. If you want to combine more than two lists or iterables, you can call extend() function multiple times or use other methods like the + operator or itertools.chain() to handle multiple iterables simultaneously.
Think You Know extend() In Python? Prove It!
With this, we conclude our discussion on the extend() function in Python. Here are a few other topics that you might be interested in reading:
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Comments
Add commentLogin to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Vishwajeet Singh 1 month ago
kavita Prajapati 1 month ago
niyati m singh 1 month ago
Pardha Venkata Sai Patnam 1 month ago
A Vishnuvardhan 1 month ago