Table of content:
- What Is The Python pop() Function?
- Python pop() With Lists To Remove Element By Index
- Python pop() With Lists To Remove The Last Item Without Index
- Python pop() To Handle IndexErrors In Lists
- Python pop() With Lists For Negative Indices
- Python pop() With Nested Lists
- Python pop() With Dictionaries
- Python pop() With Dictionary To Remove Item By Key With Default Value
- Python pop() With Dictionary To Handle KeyErrors
- Benefits Of Using The pop() Function In Python
- Alternatives To Python’s pop() Method
- Conclusion
- Frequently Asked Questions
Python pop() Function | Syntax, Uses & Alternative With Examples
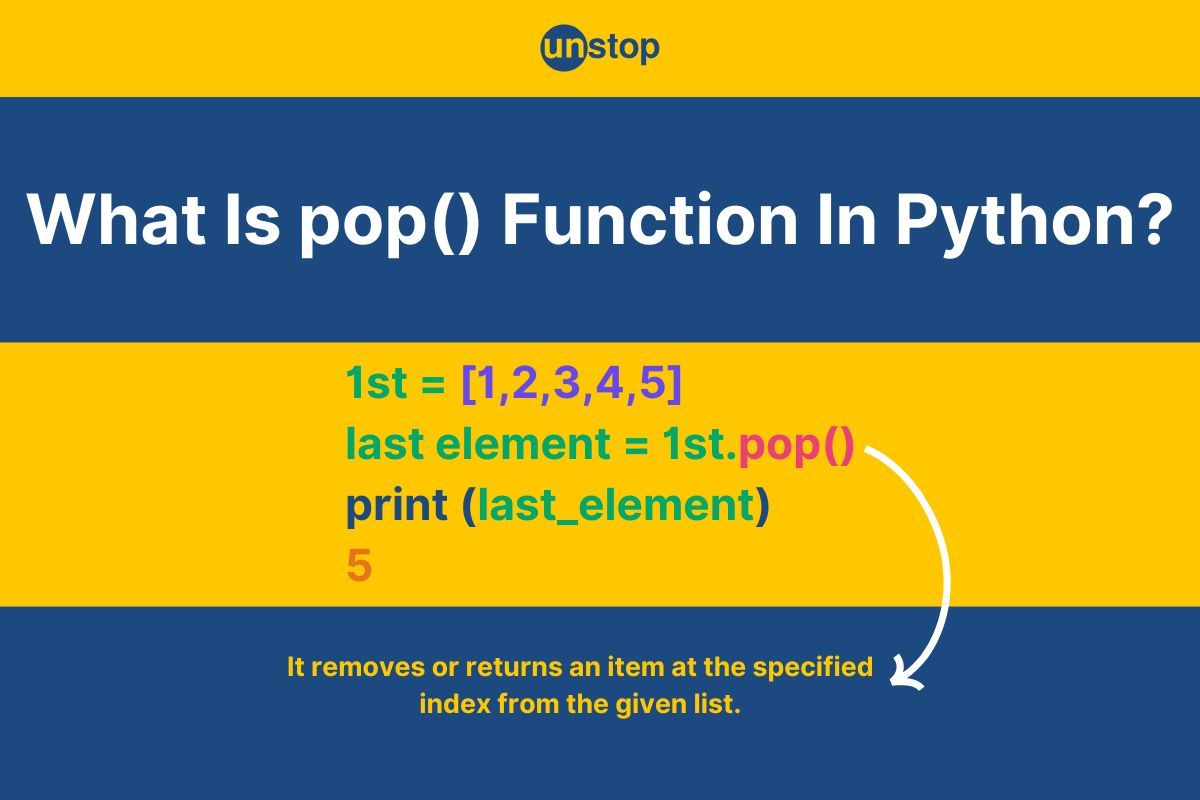
In Python, the pop() function is a built-in method that is used to remove and return an item from a list. It is a versatile and commonly used function that allows us to manipulate list data efficiently. Whether you're looking to remove an item by index or simply need to extract the last element of a list, pop() offers a straightforward solution.
In this article will explore the functionality, syntax, and various use cases of the pop() function in Python programming, providing you with a deeper understanding of how it works and when to use it effectively.
What Is The Python pop() Function?
The Python pop() method is a built-in function used with lists that allows us to remove an element at a specific position (index) and return that element. If no index is provided, it removes and returns the last item in the list by default.
- This makes it an essential tool for manipulating lists, particularly when we need to remove elements dynamically, especially from the end or from specific locations.
- When using the pop() method, the list is modified in-place, meaning the element is permanently removed from the list.
- Additionally, the pop() method returns the value of the item that was removed, which can be assigned to a variable or used in further operations.
Syntax Of Python pop() Function
list.pop([index])
Here:
- list: It refers to the list from which we want to remove an element.
- index (optional): The position (index) of the element to remove. If no index is specified, the last item is removed by default. Indices are zero-based, so 0 refers to the first element, 1 to the second, and so on. Negative indices can also be used to refer to elements from the end of the list, where -1 refers to the last element.
Return Value Of Python pop() Function
- The pop() function returns the value of the element that was removed from the list.
- If no element is removed (e.g., the list is empty), it raises an IndexError.
Explore this amazing course and master all the key concepts of Python programming effortlessly!
Python pop() With Lists To Remove Element By Index
The pop() method can be used to remove an element from a list at a specific index. By providing the index as an argument to the pop() method, we can remove the element at that position. The removed element is returned and can be assigned to a variable for further use.
Code Example:
# List with multiple elements
my_list = [10, 20, 30, 40, 50]
# Removing element at index 2
removed_item = my_list.pop(2)
# Displaying the removed item and updated list
print(removed_item)
print(my_list)
Output:
30
[10, 20, 40, 50]
Explanation:
In the above code example-
- We start by creating a list, my_list, with elements [10, 20, 30, 40, 50].
- We then use the pop() method to remove the element at index 2 (which is 30).
- The pop() method not only removes the item but also returns it, so we store the returned value in the variable removed_item.
- Next, we print the value of removed_item, which displays 30, the element that was removed.
- Finally, we print the updated my_list, which now contains the elements [10, 20, 40, 50] after the removal.
Python pop() With Lists To Remove The Last Item Without Index
If you don't specify an index, pop() will remove and return the last item from the list by default. This is a quick way to remove elements from the end of the list.
Code Example:
# List with multiple elements
my_list = [1, 2, 3, 4, 5]
# Removing the last element
last_item = my_list.pop()
# Displaying the removed item and updated list
print(last_item)
print(my_list)
Output:
5
[1, 2, 3, 4]
Explanation:
In the above code example-
- We begin by defining a list, my_list, with elements [1, 2, 3, 4, 5].
- We then use the pop() method without an index to remove the last element from the list, which is 5.
- Since pop() returns the removed element, we assign it to the variable last_item.
- We print the value of last_item, which is 5, showing the last element that was removed.
- Lastly, we print the updated my_list, which now contains [1, 2, 3, 4] after the removal of the last element.
Python pop() To Handle IndexErrors In Lists
When using pop(), if the provided index is out of bounds (e.g., the index is larger than the size of the list), Python will raise an IndexError. To handle this gracefully, we can use a try-except block.
Code Example:
# List with multiple elements
my_list = [10, 20, 30]
try:
# Trying to pop an element at an invalid index (e.g., index 5)
removed_item = my_list.pop(5)
except IndexError:
print("Error: Index out of range!")
# Displaying the updated list
print(my_list)
Output:
Error: Index out of range!
[10, 20, 30]
Explanation:
In the above code example-
- We start by creating a list, my_list, containing the elements [10, 20, 30].
- Next, we use a try block to attempt removing an element at index 5 using pop(5). However, since index 5 is out of range for the list (which only has indices 0, 1, and 2), this results in an IndexError.
- The except IndexError block catches the error, and we print the message "Error: Index out of range!" to inform us about the issue.
- Finally, we print the updated my_list, which remains unchanged as [10, 20, 30] because the pop() operation was not successful.
Python pop() With Lists For Negative Indices
In Python, the pop() method allows you to remove and return an element from a list, either by specifying its index or using the default behavior of removing the last element.
When dealing with negative indices, Python interprets them as counting from the end of the list rather than the beginning. This can be particularly useful when you want to remove elements from the end without having to calculate the actual index.
How negative indices work with pop():
- -1 refers to the last element.
- -2 refers to the second-to-last element, and so on.
Code Example:
# List of elements
my_list = [10, 20, 30, 40, 50]
# Using pop() with a negative index to remove the last item
last_item = my_list.pop(-1)
# Displaying the removed item and updated list
print(last_item)
print(my_list)
Output:
50
[10, 20, 30, 40]
Explanation:
In the above code example-
- We begin by defining a list, my_list, with elements [10, 20, 30, 40, 50].
- We then use the pop() method with a negative index, -1, to remove the last item in the list, which is 50.
- Since pop() returns the removed item, we store it in the variable last_item.
- We print the value of last_item, which is 50, showing the item that was removed.
- Finally, we print the updated my_list, which now contains [10, 20, 30, 40] after removing the last element.
Python pop() With Nested Lists
The pop() method can also be used on nested lists. This allows us to remove and return items from inner lists by specifying an index for both the outer and inner lists.
Code Example:
# Nested list
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Popping the last item from the outer list (removes [7, 8, 9])
removed_outer = nested_list.pop()
# Popping the second item from the inner list [4, 5, 6]
removed_inner = nested_list[0].pop(1)
# Displaying the removed items and updated list
print(removed_outer)
print(removed_inner)
print(nested_list)
Output:
[7, 8, 9]
2
[[1, 3], [4, 5, 6]]
Explanation:
In the above code example-
- We begin by defining a nested list, nested_list, containing sublists [[1, 2, 3], [4, 5, 6], [7, 8, 9]].
- We use the pop() method on the outer list to remove the last sublist [7, 8, 9]. The removed sublist is stored in the variable removed_outer.
- We then access the first inner list [1, 2, 3] and use pop(1) to remove the second item, 5, from the second inner list [4, 5, 6]. The removed item is stored in the variable removed_inner.
- We print removed_outer, which displays [7, 8, 9], and removed_inner, which displays 5, showing the items that were removed.
- Finally, we print the updated nested_list, which now contains [[1, 2, 3], [4, 6]] after the two pop() operations.
Python pop() With Dictionaries
The pop() method in Python can also be used with dictionaries to remove and return a key-value pair based on the specified key. It is a versatile method that allows for the safe removal of items while returning the value associated with the removed key.
Syntax Of Dictionary pop() Method
dict.pop(key, default)
Here:
- key (Required): This is the key whose associated value needs to be removed from the dictionary.
- default (Optional): This is a value that will be returned if the key is not found in the dictionary. If the default value is not provided and the key is missing, a KeyError will be raised.
Return Value Of Dictionary pop() Method
- If the key is found, pop() removes and returns the corresponding value.
- If the key is not found and a default value is provided, it returns the default value.
- If the key is not found and no default value is provided, it raises a KeyError.
Code Example:
# Dictionary of key-value pairs
my_dict = {'a': 1, 'b': 2, 'c': 3}
# Remove the item with key 'b'
removed_value = my_dict.pop('b')
# Displaying the removed value and updated dictionary
print(removed_value)
print(my_dict)
Output:
2
{'a': 1, 'c': 3}
Explanation:
In the above code example-
- We start by defining a dictionary, my_dict, with key-value pairs {'a': 1, 'b': 2, 'c': 3}.
- We use the pop() method to remove the item with the key 'b'. The pop() method removes the key-value pair and returns the value associated with the key.
- The removed value, 2, is stored in the variable removed_value.
- We print removed_value, which displays 2, showing the value that was removed from the dictionary.
- Finally, we print the updated my_dict, which now contains {'a': 1, 'c': 3} after removing the key 'b'.
Python pop() With Dictionary To Remove Item By Key With Default Value
If the specified key does not exist in the dictionary, pop() will raise a KeyError. However, you can provide a default value as the second argument to avoid this error. If the key is not found, the default value will be returned instead.
Code Example:
# Dictionary with key-value pairs
my_dict = {'a': 1, 'b': 2, 'c': 3}
# Attempting to remove a non-existing key 'd', with a default value 'Not Found'
removed_value = my_dict.pop('d', 'Not Found')
# Displaying the removed value and updated dictionary
print(removed_value)
print(my_dict)
Output:
Not Found
{'a': 1, 'b': 2, 'c': 3}
Explanation:
In the above code example-
- We start by defining a dictionary, my_dict, containing key-value pairs {'a': 1, 'b': 2, 'c': 3}.
- We use the pop() method to try removing the key 'd'. Since 'd' is not present in the dictionary, we provide a default value, 'Not Found', which pop() returns instead of causing an error.
- The returned value, 'Not Found', is stored in the variable removed_value.
- We print removed_value, which displays 'Not Found', indicating that the specified key was missing, and the default value was used.
- Finally, we print the updated my_dict, which remains unchanged as {'a': 1, 'b': 2, 'c': 3} since the key 'd' was not present in the dictionary.
Python pop() With Dictionary To Handle KeyErrors
If you don't provide a default value and attempt to pop a key that doesn't exist, Python raises a KeyError. You can handle this exception using a try-except block.
Code Example:
# Dictionary of key-value pairs
my_dict = {'a': 1, 'b': 2, 'c': 3}
try:
# Attempting to remove a non-existing key 'd' without a default value
removed_value = my_dict.pop('d')
except KeyError:
print("Error: Key not found!")
# Displaying the updated dictionary
print(my_dict)
Output:
Error: Key not found!
{'a': 1, 'b': 2, 'c': 3}
Explanation:
In the above code example-
- We start by defining a dictionary, my_dict, with key-value pairs {'a': 1, 'b': 2, 'c': 3}.
- We then use a try block to attempt to remove the key 'd' with the pop() method. Since 'd' is not in the dictionary and no default value is provided, this causes a KeyError.
- The except KeyError block catches the error, and we print the message "Error: Key not found!" to indicate the missing key.
- Finally, we print the updated my_dict, which remains unchanged as {'a': 1, 'b': 2, 'c': 3} because the pop() operation for the non-existing key was unsuccessful.
Benefits Of Using The pop() Function In Python
The pop() function in Python provides several benefits, especially when dealing with mutable sequences like lists. Here are some of the key advantages:
1. Efficient Removal Of Elements
- From the End:Python pop() is highly efficient (O(1) time complexity) when removing elements from the end of a list. This makes it ideal for situations where you need to process elements sequentially or stack-like structures.
- Direct Access to Removed Element: Python pop() returns the element that is removed, which allows you to use it directly without needing to perform an additional lookup. This can be useful in scenarios like removing elements while simultaneously processing or saving them.
2. Versatile Index Removal
-
Removing by Index: You can remove an element from any position in the list by passing an index to pop(i). This gives you the flexibility to manipulate lists based on specific conditions (e.g., remove an item that meets a certain criterion).
3. Handy For Implementing Stack Operations
-
Stack-like Behavior: Python pop() can be used to implement Last-In-First-Out (LIFO) behavior, which is a fundamental operation of stack data structures. This is useful in algorithms like depth-first search or parsing expressions. Example of stack behavior:
stack = [1, 2, 3]
print(stack.pop()) # Outputs: 3 (LIFO order)
print(stack) # Outputs: [1, 2]
4. In-place Modification
-
No Need for Extra Memory: Python pop() modifies the original list in place without requiring additional memory to create a new list. This can be advantageous in memory-constrained environments or when working with large datasets.
5. Simplifies Error Handling And Cleanup
-
Handling Empty Lists: When popping elements, the pop() function will raise an IndexError if the list is empty, allowing you to handle the situation explicitly with error-handling techniques like try-except. For Example:
my_list = []
try:
my_list.pop() # Will raise IndexError if the list is empty
except IndexError:
print("The list is empty.")
6. Useful For Algorithmic Applications
-
Backtracking Algorithms: The pop() function is a natural fit for backtracking algorithms, such as those used in combinatorial problems (e.g., generating permutations or solving puzzles). You can remove elements as you explore paths and add them back (by append()) when backtracking.
7. Clean And Readable Code
-
Intuitive Syntax: Python pop() provides a straightforward, concise syntax for element removal, enhancing code readability and reducing the need for additional lines of code.
8. Optimized For Performance In Some Scenarios
-
Amortized O(1) for the End: For lists with frequent removals at the end (e.g., implementing queues or stacks), Python pop() is a fast and optimized operation.
9. Supports Multiple Use Cases
-
Unpacking and Multiple Removals: By chaining the Python pop() function with other operations like list comprehension, you can efficiently filter or process items without creating unnecessary intermediate variables.
Sharpen your coding skills with Unstop's 100-Day Coding Sprint and compete now for a top spot on the leaderboard!
Alternatives To Python’s pop() Method
While pop() is a convenient and widely used method to remove elements from lists and dictionaries, Python provides other alternatives that can serve similar or complementary purposes depending on the use case. Let’s explore a few alternatives:
Using The del Statement For Removing Items
The del statement is a powerful tool for removing items from both lists and dictionaries. It can be used to remove an item by its index (for lists) or by its key (for dictionaries). Unlike pop(), del doesn't return the removed item, and it can also be used to delete entire variables or slices of lists.
Code Example:
# List of elements
my_list = [1, 2, 3, 4, 5]
# Removing the item at index 2
del my_list[2]
# Displaying the updated list
print(my_list)
Output:
[1, 2, 4, 5]
Explanation:
In the above code example-
- We begin by defining a list, my_list, containing the elements [1, 2, 3, 4, 5].
- We use the del statement to remove the item at index 2, which is 3. The del statement directly deletes the item without returning it.
- Finally, we print the updated my_list, which now contains [1, 2, 4, 5] after the removal of the element at index 2.
Using The remove() Method For Lists
The remove() method is specifically designed for removing elements by value from a list. It searches for the first occurrence of the specified value and removes it. Unlike pop(), remove() doesn't require an index and will raise a ValueError if the item is not found.
Code Example:
# List of elements
my_list = [10, 20, 30, 40, 50]
# Removing the first occurrence of value 30
my_list.remove(30)
# Displaying the updated list
print(my_list)
Output:
[10, 20, 40, 50]
Explanation:
In the above code example-
- We start by defining a list, my_list, with elements [10, 20, 30, 40, 50].
- We use the remove() method to delete the first occurrence of the value 30 from the list.
- The remove() method finds 30 in the list and removes it without returning any value.
- Finally, we print the updated my_list, which now contains [10, 20, 40, 50] after the removal of 30.
Using The popitem() For Dictionaries
For dictionaries, the popitem() method is an alternative to pop(). It removes and returns a key-value pair. If no key is provided, popitem() removes and returns an arbitrary key-value pair from the dictionary. This is especially useful when working with unordered collections like Python dictionaries before Python 3.7 (though in Python 3.7 and later, dictionaries preserve insertion order).
Code Example:
# Dictionary of key-value pairs
my_dict = {'a': 1, 'b': 2, 'c': 3}
# Removing a specific item by key using popitem
key, value = my_dict.popitem()
# Displaying the removed key-value pair and updated dictionary
print(key, value)
print(my_dict)
Output:
c 3
{'a': 1, 'b': 2}
Explanation:
In the above code example-
- We begin by defining a dictionary, my_dict, with key-value pairs {'a': 1, 'b': 2, 'c': 3}.
- We use the popitem() method to remove and return the last inserted key-value pair in the dictionary, which is ('c', 3).
- The popitem() method provides both the key and value of the removed item, which we store in the variables key and value.
- We then print key and value, which display c and 3, showing the key-value pair that was removed.
- Finally, we print the updated my_dict, which now contains {'a': 1, 'b': 2} after the removal of the last item.
Searching for someone to answer your programming-related queries? Find the perfect mentor here.
Conclusion
The pop() function in Python is a versatile tool for managing elements in both lists and dictionaries. Whether you need to remove an item by index from a list, safely handle missing keys in dictionaries, or simplify your code when dealing with nested structures, pop() offers a flexible and efficient solution.
Alongside its alternatives—like del, remove(), and popitem()—pop() gives you options to handle various data manipulation tasks cleanly and effectively. By understanding the syntax, behavior, and potential pitfalls (like IndexError and KeyError), you can use pop() to write more dynamic, error-resistant code.
Frequently Asked Questions
Q. What does the Python pop() method do?
The pop() method removes the last item from a list and returns it. If you're working with a dictionary, it removes a specified key-value pair and returns the value associated with that key. It’s helpful for dynamic data management.
Q. Can I use pop() on an empty list or dictionary?
No, you cannot use pop() on an empty list or dictionary without causing an error. If you try to pop() from an empty list, it will raise an IndexError. Similarly, if you try to pop() from an empty dictionary, it will raise a KeyError. However, if you're using pop() with a dictionary and provide a default value, it will return that value instead of raising an error if the key doesn't exist. So, it's important to check if the list or dictionary is empty or handle errors properly when using pop().
Q. Is there a way to specify which item to pop from a list?
Yes, you can specify which item to pop from a list by providing its index as an argument to the pop() method. For example, list.pop(2) will remove and return the item at index 2 in the list. If you don't provide an index, pop() will remove and return the last item by default.
Q. Are there performance implications when using pop()?
Yes, there are performance implications when using pop() in Python, and they depend on the type of data structure you're working with:
For Lists:
- Python pop() operates in O(1) time complexity when used to remove the last element (i.e., when no index is specified or the index is -1). This is because removing the last item doesn't require shifting the remaining elements in the list.
- However, if you use pop() with an index other than the last one, such as pop(0) (removing the first item), the time complexity becomes O(n). This is because all elements after the removed item must be shifted to fill the gap.
For Dictionaries:
-
Python pop() is generally O(1) in time complexity for removing a specific key-value pair. Since dictionaries are implemented using hash tables, accessing, removing, and returning a key-value pair using pop() is typically a constant-time operation, assuming no hash collisions.
In general, using pop() on the last element of a list or removing items from a dictionary by key is efficient. However, removing elements from the beginning of a list or performing repetitive pop() operations in a loop with indices can lead to higher time complexity due to the need to shift elements in the list.
Q. What are some alternatives to pop() in Python?
In Python, there are several alternatives to the pop() method, depending on your needs:
- For removing elements from a list, you can use the del statement, which removes an item at a specific index without returning it, or the remove() method, which removes the first occurrence of a specified value.
- For dictionaries, instead of pop(), you can use the popitem() method, which removes and returns a random key-value pair, or simply use del to remove a key-value pair by key.
- These alternatives provide different levels of control and behaviour depending on whether you need to return the removed item or not and whether you're working with lists or dictionaries.
Q. Can I use pop() in a loop?
Yes, you can use pop() in a loop, but be mindful of how it changes the structure as you remove items. For example, if you want to clear all elements from a list, you can use a while loop with pop() to remove items until the list is empty. Here’s how it works:
my_list = [1, 2, 3, 4, 5]
while my_list:
print(my_list.pop()) # This removes and prints the last element each time
In this case, each iteration of the loop removes the last item from my_list until it's empty. Using pop() in this way is efficient and keeps the code straightforward. Just remember that pop() modifies the list in place, so the list’s length changes as you go, which is why a while loop is safer than a for loop in this scenario.
With this, we conclude our discussion on the Python pop() function. Here are a few other topics that you might be interested in reading:
- 12 Ways To Compare Strings In Python Explained (With Examples)
- Python Logical Operators, Short-Circuiting & More (With Examples)
- Python Bitwise Operators | Positive & Negative Numbers (+Examples)
- Python String.Replace() And 8 Other Ways Explained (+Examples)
- How To Reverse A String In Python? 10 Easy Ways With Examples!
I’m a Computer Science graduate with a knack for creative ventures. Through content at Unstop, I am trying to simplify complex tech concepts and make them fun. When I’m not decoding tech jargon, you’ll find me indulging in great food and then burning it out at the gym.
Login to continue reading
And access exclusive content, personalized recommendations, and career-boosting opportunities.

Subscribe
to our newsletter
Comments
Add comment